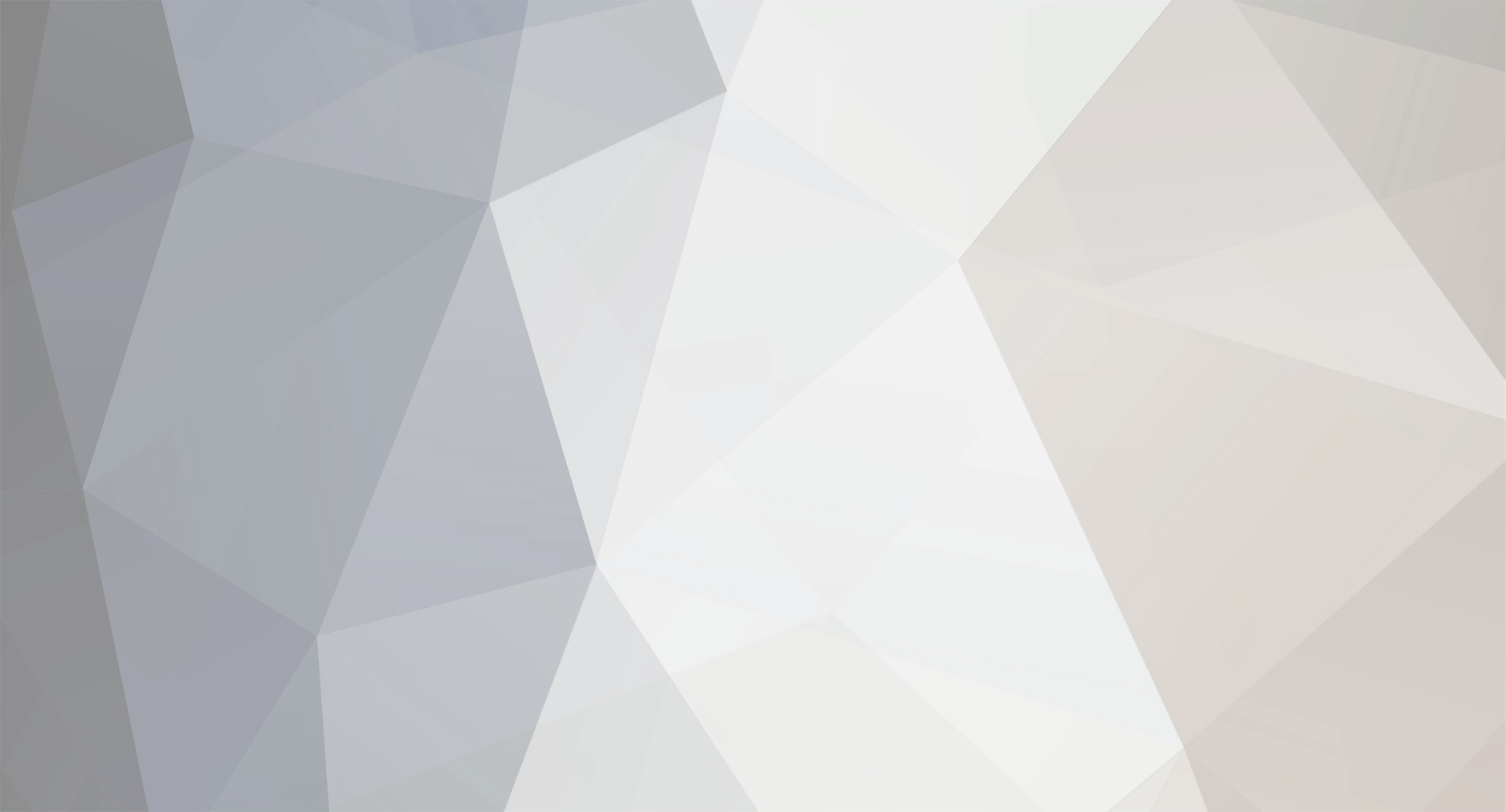
[NoOneButNo]
-
Posts
89 -
Joined
-
Last visited
-
Days Won
1
Posts posted by [NoOneButNo]
-
-
Subscribe to PlayerTickEvent (or LivingUpdateEvent if you want to include entities aside from player) and check there if your enchantment is present using EnchantmentHelper#getEnchantmentLevel. There you can use LivingEntity#addPotionEffect to apply the speed potion.
-
Nevermind. Restarting eclipse did the trick.
-
This is a stupid question, but how to re-import gradle?
-
package com.moveon.modified.lootfunctions; import net.minecraft.loot.LootFunction; public class GuaranteedEnchantWithLevels extends LootFunction{ }
As for the error, a modified screenshot is posted below. Also, haven't even started writing anything on this class.
-
Currently, if I'm trying to create a child class of net.minecraft.loot.LootFunction, eclipse yells at me that the hierarchy is inconsistent. Is this intended?
-
1.) Subscribing to an event requires only one parameter. Two or more won't work.
2.) PlayerTickEvent or LivingUpdateEvent is your best option. You need to track the travelled blocks of the player and then do the random chance.
(On the side note: I don't like using speed status effect, I'd rather use an attribute modifier to achieve the same thing. This makes it stackable to other movement speed giving items/abilities/whatever)
3.) Check if you properly registered the events. Sometimes this is the usual problem after a long hour session of coding.
4.) Profit.
-
Get all the enchantment category from
ItemGroup#getEnchantmentCategories
Then add yours (preferably you want to convert the array into a list...)
After that, set the enchantment category to the one you have just updated.
ItemGroup#setEnchantmentCategories
-
I noticed there is a sudden change in the mappings from 1.15 to 1.16. Is there some kind of list (like say .txt file or something) that documents on what is changed and what is not?
-
Just use entity.addPotionEffect that takes in an EffectInstance as its parameter.
-
Search for class EntityTypeTags. This should help you. Also, it should (disclaimer: i didn't bother to try) work the same way as how this docs tell you how to use tags: https://mcforge.readthedocs.io/en/latest/utilities/tags/
-
Try reading this: (note that it doesn't make use of an event) https://mcforge.readthedocs.io/en/latest/models/color/
-
They are just the same. Use whichever you want you find comfortable with.
-
Here's an example of how you override things:
@ObjectHolder("minecraft:quick_charge") public static final Enchantment QUICK_CHARGE = null; @SubscribeEvent public void onRegistry(RegistryEvent.Register<Enchantment> reg){ //note: The class that you are using should be the one you made for the Enchantment Quickcharge. Enchantment class is an abstract class. reg.getRegistry().register(new Enchantment().setRegistryName(new ResourceLocation("minecraft", "quick_charge"))); }
Same thing applies for entity types, etc.
-
I don't know why you have so many hacks set when its not even needed. You even overrided the vanilla enchantments and item wrong. take a look at choonster's github. https://github.com/Choonster-Minecraft-Mods/TestMod3/tree/1.14.4/src/main/java/choonster.
-
No need.
-
Things like this can easily be seen through vanilla classes. Before you actually start asking very basic questions like this, you can actually try to look at how vanilla does its mechanic. For example, in this case, look at ItemStack.class.
-
I won't say its little cluttered, its damn as hell as cluttered as it can be. I will be analysing this but don't expect a fast reply soon.
-
Post your code on github. I will look into it.
-
Sounds like you need to learn inheritance some more. This is actually basic polymorphism...
-
public class RotatedPillarBlock extends Block { public static final EnumProperty<Direction.Axis> AXIS = BlockStateProperties.AXIS; public RotatedPillarBlock(Block.Properties properties) { super(properties); this.setDefaultState(this.getDefaultState().with(AXIS, Direction.Axis.Y)); } /** * Returns the blockstate with the given rotation from the passed blockstate. If inapplicable, returns the passed * blockstate. * @deprecated call via {@link IBlockState#withRotation(Rotation)} whenever possible. Implementing/overriding is * fine. */ public BlockState rotate(BlockState state, Rotation rot) { switch(rot) { case COUNTERCLOCKWISE_90: case CLOCKWISE_90: switch((Direction.Axis)state.get(AXIS)) { case X: return state.with(AXIS, Direction.Axis.Z); case Z: return state.with(AXIS, Direction.Axis.X); default: return state; } default: return state; } } protected void fillStateContainer(StateContainer.Builder<Block, BlockState> builder) { builder.add(AXIS); } public BlockState getStateForPlacement(BlockItemUseContext context) { return this.getDefaultState().with(AXIS, context.getFace().getAxis()); } }
This is an example of a vanilla block that implements it. Take your time and read it meanwhile Im gonna go get some sleep.
-
You can try to reference the pitch by "call hierarchy". See if there is something that snaps it back to zero in vanilla classes.
-
1
-
-
Yes since you are not
overridingthe vanilla class responsible for that blockstate.Edit: Extending. Or making a new one while implementing that blockstate.
-
Sounds like you did not properly implemented your blockstate "axis".
-
1.12.2 is no longer supported on this forum. Update to modern minecraft (1.14.4+) versions to receive support.
[1.16.4] Attributes Modify
in Modder Support
Posted
Oops thought he wanted a speed potion effect.