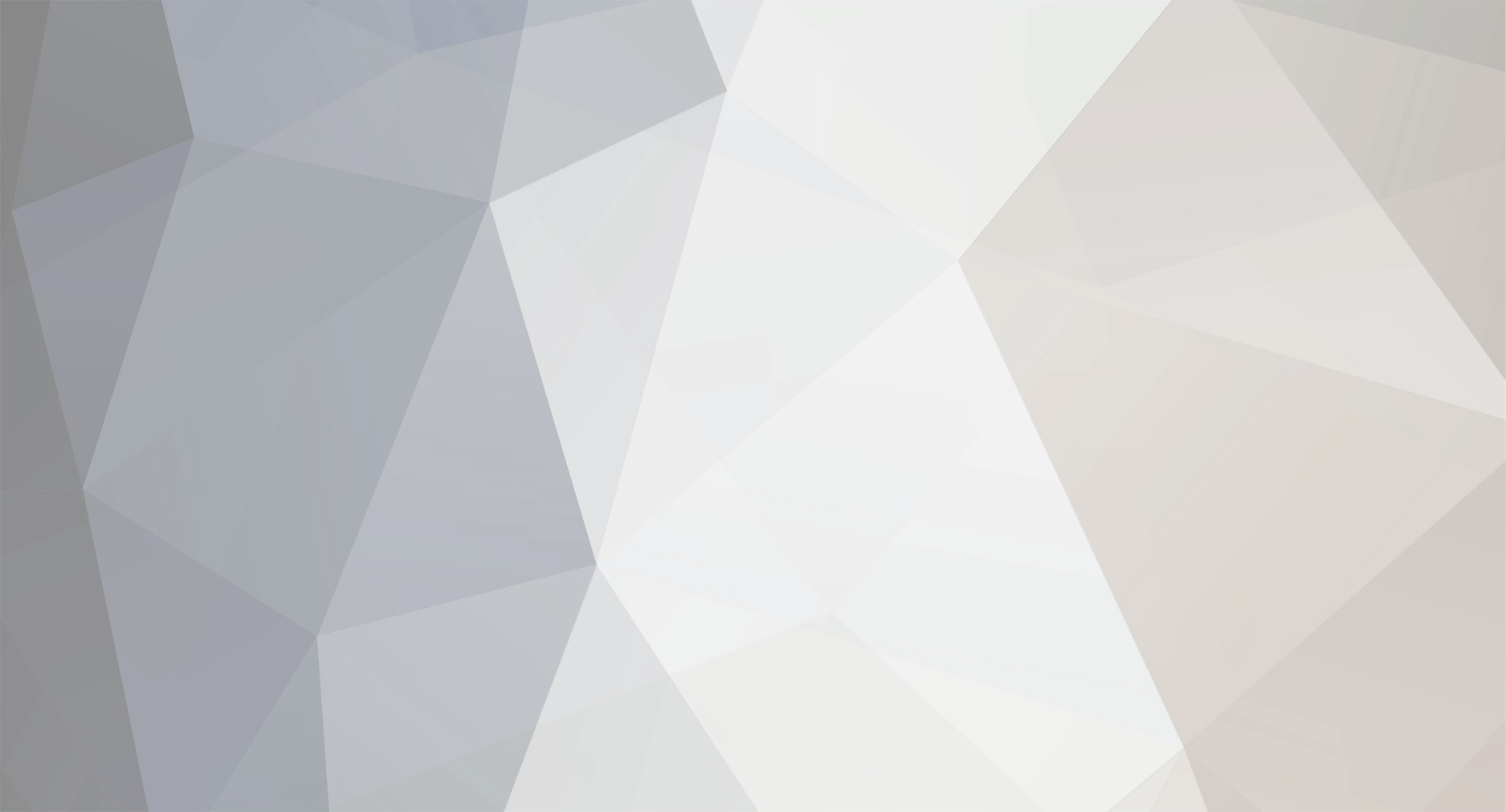
Smyler
Members-
Posts
16 -
Joined
-
Last visited
Recent Profile Visitors
The recent visitors block is disabled and is not being shown to other users.
Smyler's Achievements

Tree Puncher (2/8)
0
Reputation
-
I am looking for a way to detect a double click in a Minecraft GUI. I have successfully implemented something which works quite well, but I am relying on a config value for the double click delay and would like to follow the user's system preferences. However after some research I found out from this Github issue that using awt is not a good practice as it conflicts with lwjgl and behaves badly on macOS. I can't find any way other than something like this to get the sytsem delay, and it uses awt: Toolkit.getDefaultToolkit().getDesktopProperty("awt.multiClickInterval"); Does anyone know of a way to do that without awt?
-
Custom Texture Loading From Outside The Mod's JAR
Smyler replied to Smyler's topic in Modder Support
I found the solution myself, so here it is for anyone which may have a similar problem: use TextureManager::getDynamicTextureLocation(String, BufferredImage) -
Hi, I am doing a web map client inside Minecraft, and I am facing a problem. This kind of maps uses 256*256 tiles which I download from the internet at run time and save to a directory inside the .minecraft directory. I then need to render them on a GUI in the game, and that's were the problem is: I can't get how I am supposed to properly load a texture from a given file BufferedImage (bypassing the minecraft ResourceLocation system). I believe I do not fully understand how Minecraft handles texture handling, could someone explain it? This is what I am currently doing: public class TileTexture extends SimpleTexture{ //A class holding informations about the tile (position, zoom level, and a BufferedImage (which is the texture I need) protected ImageWebTile tile; /** * @param textureResourceLocation */ public TileTexture(ResourceLocation textureResourceLocation, ImageWebTile tile) { super(textureResourceLocation); this.tile = tile; } @Override public void loadTexture(IResourceManager resourceManager) throws IOException{ this.deleteGlTexture(); BufferedImage bufferedimage = tile.getImage(); TextureUtil.uploadTextureImageAllocate(this.getGlTextureId(), bufferedimage, false, false); } } public class GuiTiledMap extends Gui{ //FIXME MEMORY LEAK /* * The position of the map on the GUI */ protected int x; protected int y; /* * Its dimensions */ protected int width; protected int height; protected boolean visible; protected boolean hovered; protected TiledMap<?> map; //An object representing the map itself // Delta of the position of the map (in pixel) protected long upperLeftX; protected long upperLeftY; protected float zoomLevel; public GuiTiledMap(int x, int y, int width, int height, TiledMap<?> map) { this.x = x; this.y = y; this.width = width; this.height = height; this.visible = true; this.hovered = false; this.map = map; this.zoomLevel = map.getZoomLevel(); this.upperLeftX = 0; this.upperLeftY = 0; } public void draw() { this.handleMouseInput(); Minecraft mc = Minecraft.getMinecraft(); TextureManager textureManager = mc.getTextureManager(); ImageWebTile upperLeftTile = this.map.getTileAt(this.upperLeftX, this.upperLeftY); ImageWebTile tile = upperLeftTile; int renderSize = getTileRenderSize(tile); long maxX = this.upperLeftX + this.width; long maxY = this.upperLeftX + this.width; while(tile.getY() * renderSize < maxY) { //The problem is here, I need to reload the texture into the same resource location everytime, which is far from ideal textureManager.bindTexture(tile.getTexture()); drawModalRectWithCustomSizedTexture( (int)(this.x + tile.getX() * renderSize - this.upperLeftX), (int)(this.y + tile.getY() * renderSize - this.upperLeftY), 0, 0, renderSize, renderSize, renderSize, renderSize); if(tile.getX() * renderSize + renderSize >= maxX) tile = this.map.getTile(upperLeftTile.getX(), tile.getY() + 1); else tile = this.map.getTile(tile.getX() + 1, tile.getY()); renderSize = getTileRenderSize(tile); } } /** * Handles mouse input. */ public void handleMouseInput(){ int i = Mouse.getDWheel(); float z; if (i != 0){ if (i > 0) z = 0.1f; else z = -0.1f; this.zoom(z); IRLW.logger.info(this.zoomLevel); } } public void zoom(float val) { if(this.zoomLevel + val > 0) this.zoomLevel += val; this.setTiledMapZoom(); } private void setTiledMapZoom() { this.map.setZoomLevel((int)this.zoomLevel); } public int getTileRenderSize(ImageWebTile tile) { float factor = this.zoomLevel - (float)tile.getZoom(); int size = (int) (Math.pow(2, factor) * tile.getSideSize()); return size / 2; } } public abstract class ImageWebTile implements Cachable { protected int x; protected int y; protected int zoom; protected int[] defaultPixel = {0, 0, 0, 0}; //What to return when the tile doesn't exist but should protected int size; protected BufferedImage image; protected ResourceLocation texture = null; public ImageWebTile(int size, int x, int y, int zoom) { this.x = x; this.y = y; this.zoom = zoom; this.size = size; } public ImageWebTile(int size, int x, int y, int zoom, int[] defaultPixel) { this(size, x, y, zoom); this.defaultPixel = defaultPixel; } @Override public abstract URL getURL(); @Override public abstract String getFileName(); public BufferedImage getImage() throws IOException, InvalidMapboxSessionException { //Loads the BufferedImage if needed return this.image; } public ResourceLocation getTexture() { if(true) { //TODO TEMP //I want to do something like this to avoid reloading the texture at each frame //if(this.texture == null) { //This displays the debug texture (ping and black) //this.texture = new ResourceLocation(IRLW.MOD_ID, "textures/gui/maps/" + this.x + "/" + this.y + "/" + this.zoom); //But this works (the file exists) this.texture = new ResourceLocation(IRLW.MOD_ID, "textures/gui/worldmap.png"); Minecraft mc = Minecraft.getMinecraft(); TextureManager textureManager = mc.getTextureManager(); textureManager.loadTexture(this.texture, new TileTexture(this.texture, this)); } return this.texture; //TODO We should be able to unload textures, there is a big memory leak there } ///// Various uninteresting getters and setters from here ///// } Just tell me if want a git repo, I just haven't make one yet as it is still very early development. I think a solution would be to have a custom ResourceLocation, but I currently can't find out how to that. Thanks in advance!
-
[1.12.1] Canceled PlaceEvent removes block from inventory
Smyler replied to Pixtar's topic in Modder Support
Maybe you should sync your config from server to client? -
It seems to be exactly what I need and I didn't know about it, thanks!
-
I need to be able to be able to stop the server if the player moves quickly to a zone which needs to be generated, before the server tries to generate it. I don't want to generate a default chunk in this case. I will recommend not to use my mod offline, but I still need to properly handles the connection being lost at the middle of the game. Well when a chunk is being generated by my IChunkGenerator implementation's provideChunk(int x, int z) method and is on a tile of the map which needs to be downloaded from the Mapbox's server, it's too late to cancel the chunk generation, and the method has to return something. Returning null crashes the game. At this stage, a two things can happened: The network is disconnected The API http response code is 403, telling that the access token is not valid. In the first case, the server should be stopped for both the integrated and the physical server. In the second one, the physical server should stop and the integrated server should ask for a valid token through the client and wait for it (I already implemented this). If no token is provided, it should close the server. This is impossible to do from IChunkGenerator::provideChunk(), as the server can't be stopped from there. As I mentioned in my previous post, the data being downloaded from IChunkGenerator::provideChunk() is my fallback option, and I have a daemon thread running to download tiles. So when the tile at (x;y) is being loaded to be used for world generation, the tiles surrounding tiles are added to a download queue, where any problem can be solved by shutting down the server. The problem is that when the player moves quickly to a new zone, this wont work because I have no way to have added the tile to the download queue before, so it's being downloaded from the server thread, within IChunkGenerator::provideChunk().
-
I am making a mod which generates the real world in Minecraft, using manly the Mapbox API. Querying the API works fine, but the fact the the mod can create an error when generating a chunk if the internet access drops is a very big problem, as it's impossible to cancel the generation of the chunk to stop the server. To counter this, my mod uses a daemon thread to download the data of the area that could potentially be generated in a near future. This system works great when you are just flying over the world in creative, but the game will still crash if the player teleports somewhere where the data hasn't been downloaded yet. How do you think I could detect such an action to stop the server before it tries to generate the area where the player moves? You can find the sources here: https://github.com/ZeSmiler/irlw/tree/0.2_dev . I will create a new repository when the mod will get playable, I know this one doesn't follow the guidelines you guys are giving on the forum about creating a repository, so I will fix it then. Thanks in advance.
-
Ok, thanks for your help, I think I will try the hard thing first for the client game as I don't want to completely crash the clientit, but maybe I will come back at it later with more experience if I can't find a way to work around it.
-
And how can I stop the dedicated server without just crashing it? I can't find any way to get an instance like I would do on the client with Minecratf#getMinecraft()
-
Even on the client game? There is no way to show a GUI when the world is being generated by the integrated server?
-
Sorry, I don't really understand what you mean, English is not my primary language. I am using Mapbox's API to download the heightmap used by my IChunkGenerator, and so I need to be able to cancel the world creation if the token is invalid (If I get a 401 response from the web API), from IChunkGenerator::provideChunk(), as each chunk can be on a new tile to download and as the token can be invalidated if the player makes more requests than he is able to with his Mapobox account. Ideally, a GUI prompting for a valid token should be displayed on the client and a message asking to edit the config file printed to stdout on the dedicated server. I don't think this is possible but if you have any idea how to make something similar please tell because I want to do something user-friendly and at the moment, the token is stored in the config and having an invalid one will simply crash the game. Here is the github: https://github.com/ZeSmiler/irlw/tree/0.2_dev
-
Mapbox, I am generating a map of the world, so it has to be done while generating.
-
I have a world generator that needs internet access to fetch data through a web API which requires a token. I want to stop the integrated server if the token is invalid and display a message in the logs. I need to do something equivalent for the integrated server, I think through a GUI, but I can't so far because the spawn area is generated before the server starts, and because I can't get an instance of the dedicated server (not the integrated, I made a mistake in my last post).
-
Hi, I need to stop the dedicated server, but I can't figure out how to do it. I thought about using MinecraftServer::initiateShutdown(), as it's the only thing I found to do it, but I can't figure out how to get the MinecraftServer instance. Please help, thanks
-
That fixes it, thanks.