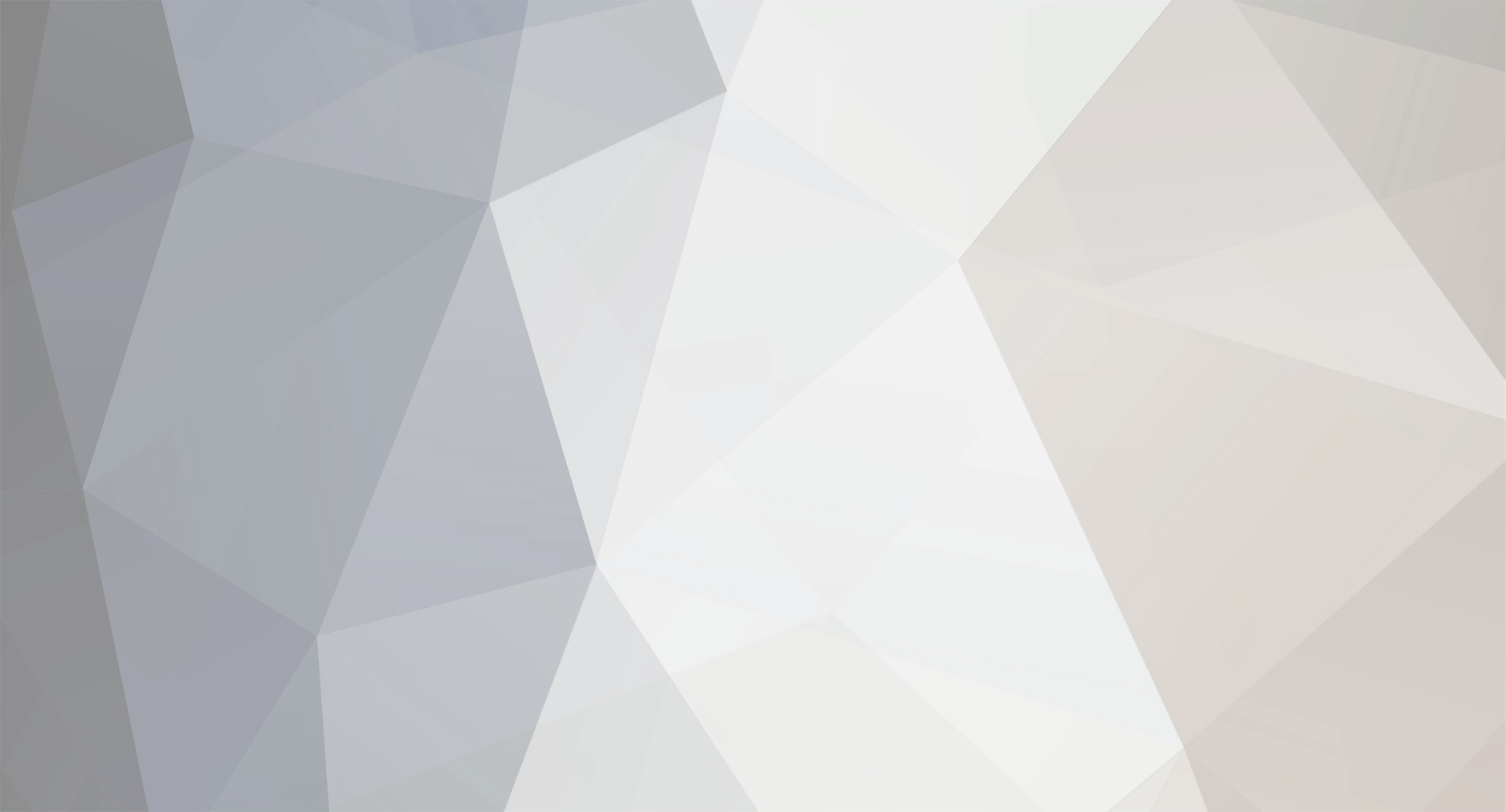
Nomnomab
Members-
Posts
21 -
Joined
-
Last visited
Recent Profile Visitors
The recent visitors block is disabled and is not being shown to other users.
Nomnomab's Achievements

Tree Puncher (2/8)
0
Reputation
-
Ah, thanks I'll try that, and yeah I meant getActualState sorry haha. Been a bit tired recently
-
Like, the getActiveState from a block and the "server data" is the tile entity's nonremote data I guess. Not even sure how to go about this haha @diesieben07
-
Ok, code is changed correctly for that part. Also, the data seems to save and load properly if I check with !worldIn.isRemote. Am I able to just set the Active State from the Server data? @draganz @diesieben07
-
Ah ok, I'll give it a try.
-
Then how would you suggest I do this?
-
First off, I am setting the tile entitie's data here: @Override public void onBlockPlacedBy(World worldIn, BlockPos pos, IBlockState state, EntityLivingBase placer, ItemStack stack) { System.out.print("BlockMultiTanningRack set in world.\n"); // set this data getTileEntity(worldIn, pos).setIsMaster(true); // grab direction EnumFacing enumFacing = BlockPistonBase.getFacingFromEntity(pos, placer); // grab positions BlockPos right = getDirectionalOffset(pos, enumFacing.getIndex(), 1, 0, 0); BlockPos topLeft = getDirectionalOffset(pos, enumFacing.getIndex(), 0, 1, 0); BlockPos topRight = getDirectionalOffset(pos, enumFacing.getIndex(), 1, 1, 0); // set blocks worldIn.setBlockState(pos, state.withProperty(FACING, enumFacing).withProperty(PIECE, Enum4Piece.BOTTOMLEFT)); worldIn.setBlockState(right, ModBlocks.TANNING_RACK.getDefaultState().withProperty(FACING, enumFacing).withProperty(PIECE, Enum4Piece.BOTTOMRIGHT)); worldIn.setBlockState(topLeft, ModBlocks.TANNING_RACK.getDefaultState().withProperty(FACING, enumFacing).withProperty(PIECE, Enum4Piece.TOPLEFT)); worldIn.setBlockState(topRight, ModBlocks.TANNING_RACK.getDefaultState().withProperty(FACING, enumFacing).withProperty(PIECE, Enum4Piece.TOPRIGHT)); // set tiles Tile4PieceVerticalBlock rightEntity = (Tile4PieceVerticalBlock)createTileEntity(worldIn, state); Tile4PieceVerticalBlock topLeftEntity = (Tile4PieceVerticalBlock)createTileEntity(worldIn, state); Tile4PieceVerticalBlock topRightEntity = (Tile4PieceVerticalBlock)createTileEntity(worldIn, state); // set masters rightEntity.setMasterPos(pos.getX(), pos.getY(), pos.getZ()); topLeftEntity.setMasterPos(pos.getX(), pos.getY(), pos.getZ()); topRightEntity.setMasterPos(pos.getX(), pos.getY(), pos.getZ()); // set tile in world worldIn.setTileEntity(right, rightEntity); worldIn.setTileEntity(topLeft, topLeftEntity); worldIn.setTileEntity(topRight, topRightEntity); super.onBlockPlacedBy(worldIn, pos, state, placer, stack); } And then debugging here: @Override public boolean onBlockActivated(World worldIn, BlockPos pos, IBlockState state, EntityPlayer playerIn, EnumHand hand, @Nullable ItemStack heldItem, EnumFacing side, float hitX, float hitY, float hitZ) { TileEntity tile = worldIn.getTileEntity(pos); if(tile != null && tile instanceof Tile4PieceVerticalBlock) { Tile4PieceVerticalBlock tileTanningRack = (Tile4PieceVerticalBlock)tile; playerIn.addChatMessage(new TextComponentString("Master = " + tileTanningRack.isMaster() + ", Slave = " + tileTanningRack.hasMaster())); } return super.onBlockActivated(worldIn, pos, state, playerIn, hand, heldItem, side, hitX, hitY, hitZ); }
-
Considering when I debug before I reload the world, it contains the data I set. But then when I reload the world and then debug, the data is completely reset. (I debug through onBlockActivated btw to do quick checks)
-
Ok so, I can successfully set data on my TileEntity for my ModelTanningRack, but for some reason if I reload the world, the data is not loaded up at all and only reset. My TileEntity: public class Tile4PieceVerticalBlock extends TileEntity implements ITickable { private boolean isMaster, hasMaster; private int masterX, masterY, masterZ; // reset info when master is gone public void reset(){ masterX = masterY = masterZ = 0; isMaster = hasMaster = false; } // check that the master exists public boolean checkForMaster(){ TileEntity tile = worldObj.getTileEntity(getMasterPos()); return (tile != null && (tile instanceof Tile4PieceVerticalBlock)); } @Override public NBTTagCompound writeToNBT(NBTTagCompound compound) { System.out.println("WRITE:" + getMasterPos() + "\n"); compound.setInteger("masterX", masterX); compound.setInteger("masterY", masterY); compound.setInteger("masterZ", masterZ); compound.setBoolean("isMaster", isMaster); compound.setBoolean("hasMaster", hasMaster); super.writeToNBT(compound); // if(hasMaster() && isMaster()){ // masterWriteToNBT(compound); // } return compound; } @Override public void readFromNBT(NBTTagCompound compound) { this.masterX = compound.getInteger("masterX"); this.masterY = compound.getInteger("masterY"); this.masterZ = compound.getInteger("masterZ"); this.isMaster = compound.getBoolean("isMaster"); this.hasMaster = compound.getBoolean("hasMaster"); super.readFromNBT(compound); System.out.println("READ:" + getMasterPos() + "\n"); // if(hasMaster() && isMaster()){ // masterReadToNBT(compound); // } } public boolean hasMaster(){ return hasMaster; } public boolean isMaster(){ return isMaster; } public int getMasterX(){ return masterX; } public int getMasterY(){ return masterY; } public int getMasterZ(){ return masterZ; } public BlockPos getMasterPos(){ return new BlockPos(getMasterX(), getMasterY(), getMasterZ()); } public void setHasMaster(boolean bool){ hasMaster = bool; } public void setIsMaster(boolean bool){ isMaster = bool; hasMaster = bool; } public void setMasterPos(int x, int y, int z){ masterX = x; masterY = y; masterZ = z; setHasMaster(true); } public int xCoord(){ return pos.getX(); } public int yCoord(){ return pos.getY(); } public int zCoord(){ return pos.getZ(); } @Override public void update() { } } Any ideas? (I could very well be missing something)
-
Were you syncing the data to the client? Yep, just could never find the right way to set the state to the Block. Since I kept getting NullPointers for the block state. I do that for one of my structures. A 3x3 millstone. Ah sweet, currently making the base classes for this so that I can do it as large as I need Hopefully it doesn't explode too much
-
Haha yeah Let us know how it turns out
-
Ah ok, then yeah, it should be fine for you. Unless it doesn't act like a door, then you might have to do a bit of a rewrite
-
Maybe? Depends on what you are trying to do
-
Ouch, that might be why it freaks out
-
Tried that, although only tried to use data from the TileEntity to set the state there. Should I use placement instead in the "group" of multiblocks? Like parent is x,y,z and child is using that parent's position to determine the state? What would you recommend
-
Quick question then, do TileEntities support loading of BlockStates to a Block it is housed in? Trying to make this as painless as possible to load up states over 16. I can share what I've done once I make sure it doesn't blow up in my face anymore