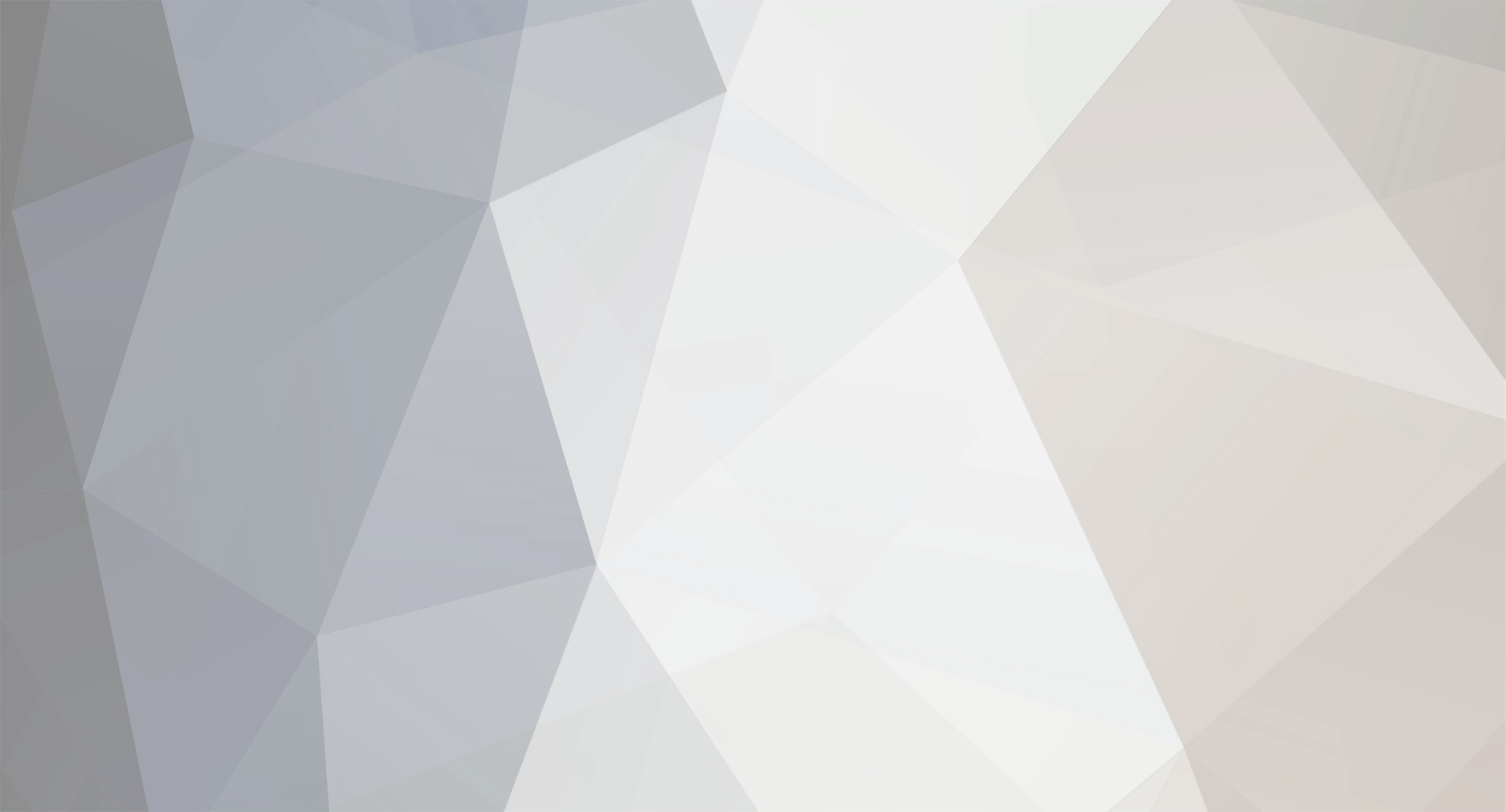
Xuluf
-
Posts
7 -
Joined
-
Last visited
Posts posted by Xuluf
-
-
only getServerGuiElement is called now as before.
-
For some odd reason the getClientGuiElement in the GuiHandler wont be called. Do I need to setup something or shouldn't I use that?
-
Should I remove the WHOLE of that or just a bit of it?
-
Okay, I got an error that said that it tried to open a GUI without being a network mod. Code
package xul.mods.adventuremod; import java.lang.annotation.Annotation; import net.minecraft.block.Block; import net.minecraft.client.Minecraft; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.item.Item; import net.minecraft.item.ItemStack; import net.minecraft.world.World; import net.minecraftforge.client.MinecraftForgeClient; import xul.mods.adventuremod.common.AdvCommonProxy; import cpw.mods.fml.common.Mod; import cpw.mods.fml.common.Mod.Init; import cpw.mods.fml.common.Mod.Instance; import cpw.mods.fml.common.Mod.PreInit; import cpw.mods.fml.common.SidedProxy; import cpw.mods.fml.common.event.FMLInitializationEvent; import cpw.mods.fml.common.event.FMLPreInitializationEvent; import cpw.mods.fml.common.network.IConnectionHandler; import cpw.mods.fml.common.network.IPacketHandler; import cpw.mods.fml.common.network.ITinyPacketHandler; import cpw.mods.fml.common.network.NetworkMod; import cpw.mods.fml.common.network.NetworkRegistry; import cpw.mods.fml.common.registry.GameRegistry; import cpw.mods.fml.common.registry.LanguageRegistry; @Mod(modid = "Xuluf_Adventuremod", name = "Adventuremod", version = "1.0 RC1") @NetworkMod(clientSideRequired = true, serverSideRequired = false, channels = {"GenericRandom"}, packetHandler = PacketHandler.class) public class Adventuremod { @Instance public static Adventuremod instance = new Adventuremod(); private GuiHandler guiHandler = new GuiHandler(); @SidedProxy(clientSide = "xul.mods.adventuremod.AdvClientProxy", serverSide = "xul.mods.adventuremod.common.AdvCommonProxy") public static AdvCommonProxy proxy; /** Weapons */ public static Item battleaxewood; public static Item battleaxestone; public static Item battleaxeiron; public static Item battleaxegold; public static Item battleaxediamond; public static Item battleaxedeamon; @Init public void Init(FMLInitializationEvent event) { proxy.registerRenderThings(); battleaxewood = new ItemAdv(5000).setIconIndex(0).setItemName("BATTLEAXEWOOD"); battleaxestone = new ItemAdv(5001).setIconIndex(1).setItemName("BATTLEAXESTONE"); battleaxeiron = new ItemAdv(5002).setIconIndex(2).setItemName("BATTLEAXEIRON"); battleaxegold = new ItemAdv(5003).setIconIndex(3).setItemName("BATTLEAXEGOLD"); battleaxediamond = new ItemAdv(5004).setIconIndex(4).setItemName("BATTLEAXEDIAMOND"); battleaxedeamon = new ItemAdv(5005).setIconIndex(5).setItemName("BATTLEAXEDEAMON"); GameRegistry.registerItem(battleaxewood, "Wooden Battleaxe"); LanguageRegistry.addName(battleaxewood, "Wooden Battleaxe"); GameRegistry.registerItem(battleaxestone, "Stone Battleaxe"); LanguageRegistry.addName(battleaxestone, "Stone Battleaxe"); GameRegistry.registerItem(battleaxeiron, "Iron Battleaxe"); LanguageRegistry.addName(battleaxeiron, "Iron Battleaxe"); GameRegistry.registerItem(battleaxegold, "Gold Battleaxe"); LanguageRegistry.addName(battleaxegold, "Gold Battleaxe"); GameRegistry.registerItem(battleaxediamond, "Diamond Battleaxe"); LanguageRegistry.addName(battleaxediamond, "Diamond Battleaxe"); GameRegistry.registerItem(battleaxedeamon, "Deamon Battleaxe"); LanguageRegistry.addName(battleaxedeamon, "Deamon Battleaxe"); GameRegistry.addRecipe(new ItemStack(battleaxewood, 1), new Object[]{ "***","***","***", '*', Block.dirt }); GameRegistry.addRecipe(new ItemStack(battleaxestone, 1), new Object[]{ "***","* ","***", '*', Block.dirt }); GameRegistry.addRecipe(new ItemStack(battleaxeiron, 1), new Object[]{ "* *","***","***", '*', Block.dirt }); GameRegistry.addRecipe(new ItemStack(battleaxegold, 1), new Object[]{ "***","* *","***", '*', Block.dirt }); GameRegistry.addRecipe(new ItemStack(battleaxediamond, 1), new Object[]{ "***","***","* *", '*', Block.dirt }); GameRegistry.addRecipe(new ItemStack(battleaxedeamon, 1), new Object[]{ "*", '*', Block.dirt }); MinecraftForgeClient.preloadTexture("/adventure/gui/items.png"); GameRegistry.registerPlayerTracker(new PlayerTracker()); NetworkRegistry.instance().registerGuiHandler(this, guiHandler); } }
package xul.mods.adventuremod; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.nbt.NBTTagCompound; import net.minecraft.src.ModLoader; import net.minecraft.world.World; import cpw.mods.fml.common.IPlayerTracker; public class PlayerTracker implements IPlayerTracker{ @Override public void onPlayerLogin(EntityPlayer player) { NBTTagCompound tag = player.getEntityData(); if(tag.getBoolean("onceConnected") != true){ player.sendChatToPlayer("Hi! You just connected."); player.openGui(Adventuremod.instance, 26, player.worldObj, 0, 0, 0); } else{ player.sendChatToPlayer("You are not a new player "); }tag.setBoolean("onceConnected", true); } @Override public void onPlayerLogout(EntityPlayer player) { // TODO Auto-generated method stub } @Override public void onPlayerChangedDimension(EntityPlayer player) { // TODO Auto-generated method stub } @Override public void onPlayerRespawn(EntityPlayer player) { // TODO Auto-generated method stub } }
package xul.mods.adventuremod; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.tileentity.TileEntity; import net.minecraft.world.World; import cpw.mods.fml.common.network.IGuiHandler; public class GuiHandler implements IGuiHandler { //returns an instance of the Container you made earlier @Override public Object getServerGuiElement(int id, EntityPlayer player, World world, int x, int y, int z) { System.out.println("Server is working!"); return null; } //returns an instance of the Gui you made earlier @Override public Object getClientGuiElement(int id, EntityPlayer player, World world, int x, int y, int z) { System.out.println("Client is working!"); switch(id) { case 26: System.out.println("Whatever"); return new GuiStart(); default: return null; } } }
Tell me if I forgot any class or code
-
Okay I've already got that part. But I want to open a gui on the open of a NEW world. So I tried the PlayerTracker but that didn't work :I
-
Okay so I recently made a forum post on the Minecraftforum.net and they said a bunch of things then after like 2 days one said that I was trying to open the gui on a singleplayer world and I had to use a PacketHandler for that. Now my question is, I don't understand the tutorial on the wiki. And I really need help with this. Minecraftforum.net link: http://www.minecraftforum.net/topic/1674623-i-need-help-with-a-guihandler
Opening a Gui with a packethandler.
in Modder Support
Posted
I got suggested that I should use a packethandler to open my gui which isn't a container it is a gui with text only. Now I have no idea on how to open the gui in the packethandler, so I would require some help
thanks! If it is unclear, sorry 