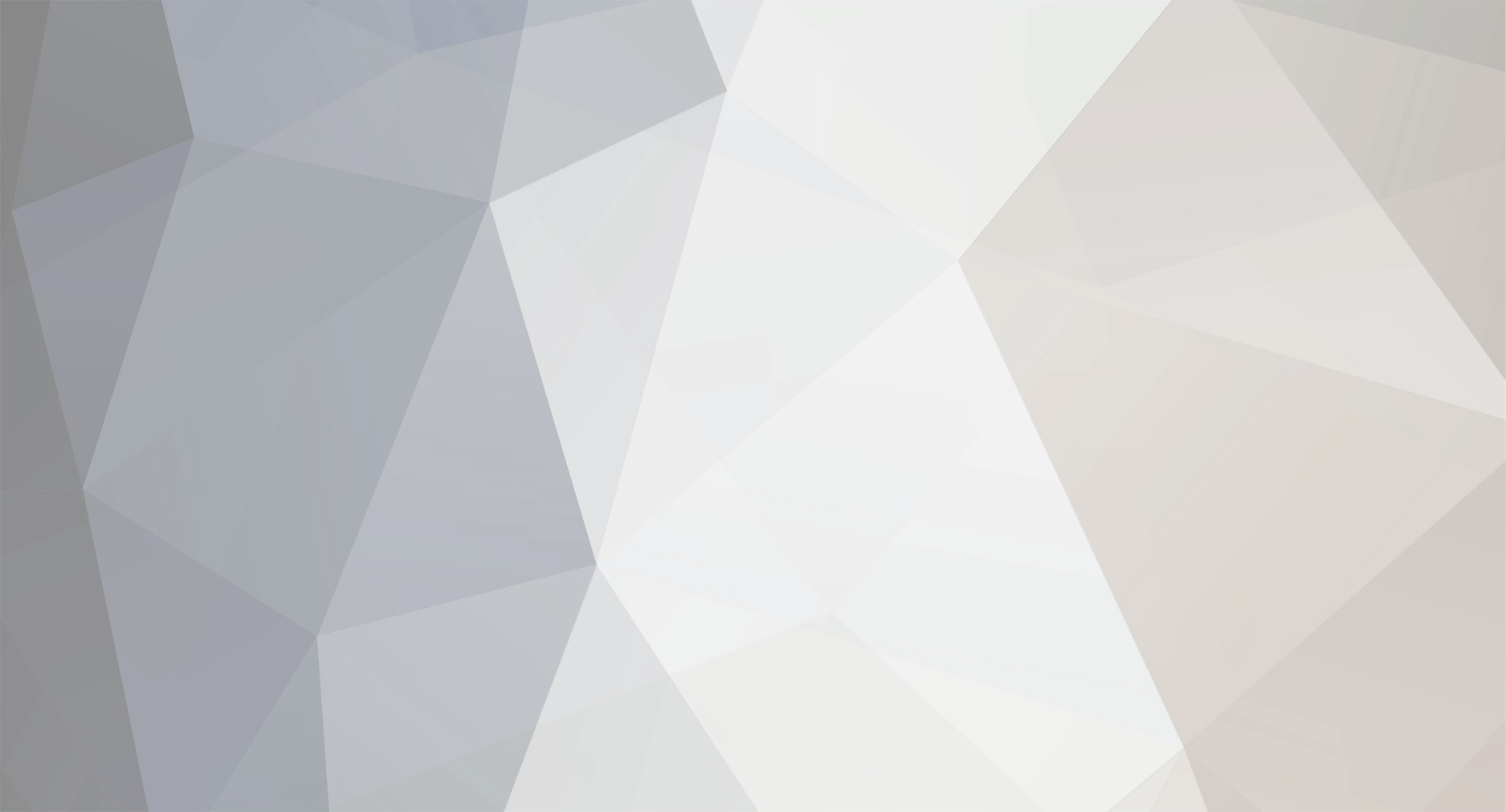
Bomb787
-
Posts
7 -
Joined
-
Last visited
Posts posted by Bomb787
-
-
21 hours ago, Toma™ said:
Yeah, I heard about it too, but isn't it really meant only for work with flans mod? I don't really know, I have worked with it only once and it looks like it's done only for the flans mod because of some different rendering stuff which wouldn't work with other mods unless I would create or copy flan's model rendering system.
You have the option to export as either a flans mod or generic java model
-
Toolbox 2.0 is a pretty good program that we use for Flans Mod
-
What would be a good tutorial for me to follow? 1.7.10 is the only version I've made a mod for.
-
I'm making a mod that adds decorations and I can't seem to get the textures working. When I hold the item I in my hand it shows up as a black and purple square in the middle of my screen with "vmm:LH_new#inventory" in front.
Here is my code:
Common Proxy:
Spoilerpackage bomb787.vmm.proxy;
import net.minecraft.item.Item;
public class CommonProxy {
public void registerItemRenderer(Item item, int meta, String id) {
}}
Client Proxy:
Spoilerpackage bomb787.vmm.proxy;
import net.minecraft.client.renderer.block.model.ModelResourceLocation;
import net.minecraft.item.Item;
import net.minecraftforge.client.model.ModelLoader;public class ClientProxy extends CommonProxy {
public void registerItemRenderer(Item item, int meta, String id) {
ModelLoader.setCustomModelResourceLocation(item, meta, new ModelResourceLocation(item.getRegistryName(), id));
}}
RegistryHandler:
Spoilerpackage bomb787.vmm.util.handlers;
import bomb787.vmm.init.ItemInit;
import bomb787.vmm.util.IHasModel;
import net.minecraft.item.Item;
import net.minecraftforge.client.event.ModelRegistryEvent;
import net.minecraftforge.event.RegistryEvent;
import net.minecraftforge.fml.common.Mod.EventBusSubscriber;
import net.minecraftforge.fml.common.eventhandler.SubscribeEvent;@EventBusSubscriber
public class RegistryHandler {
@SubscribeEvent
public static void onItemRegister(RegistryEvent.Register<Item> event) {
event.getRegistry().registerAll(ItemInit.ITEMS.toArray(new Item[0]));
}
@SubscribeEvent
public static void onModelRegister(ModelRegistryEvent event) {
for(Item item : ItemInit.ITEMS) {
if(item instanceof IHasModel) {
((IHasModel)item).registerModels();
}
}
}}
IHasModel:
Spoilerpackage bomb787.vmm.util;
public interface IHasModel {
public void registerModels();
}
Item Base:
Spoilerpackage bomb787.vmm.items;
import bomb787.vmm.Main;
import bomb787.vmm.init.ItemInit;
import bomb787.vmm.proxy.ClientProxy;
import bomb787.vmm.util.IHasModel;
import net.minecraft.creativetab.CreativeTabs;
import net.minecraft.item.Item;public class ItemBase extends Item implements IHasModel{
public ItemBase(String name) {
setUnlocalizedName(name);
setRegistryName(name);
setCreativeTab(CreativeTabs.MATERIALS);
ItemInit.ITEMS.add(this);
}
@Override
public void registerModels() {
Main.proxy.registerItemRenderer(this, 0, "inventory");
}
}ItemInit:
Spoilerpackage bomb787.vmm.init;
import java.util.ArrayList;
import java.util.List;import bomb787.vmm.items.ItemBase;
import net.minecraft.item.Item;public class ItemInit {
public static final List<Item> ITEMS = new ArrayList<Item>();
public static final Item NewLHLogo = new ItemBase("LH_new");
}Am I doing something wrong? I'm following this as the tutorial:
-
-
I'm making a mod focused on 3d models but I can't figure out how to set the model rotation when placed.
Block:
package com.bomb787.modelingmod.Blocks; import com.bomb787.modelingmod.rendering.tiles.TileEntityTable; import cpw.mods.fml.relauncher.Side; import cpw.mods.fml.relauncher.SideOnly; import net.minecraft.block.BlockContainer; import net.minecraft.block.material.Material; import net.minecraft.entity.EntityLivingBase; import net.minecraft.init.Blocks; import net.minecraft.item.ItemStack; import net.minecraft.tileentity.TileEntity; import net.minecraft.util.AxisAlignedBB; import net.minecraft.util.IIcon; import net.minecraft.util.MathHelper; import net.minecraft.world.World; public class BlockTable extends BlockContainer{ public BlockTable() { super(Material.wood); this.setBlockName("table"); this.setResistance(1.0F); this.setHardness(1.0F); } @Override public int getRenderType() { return -1; } public boolean isOpaqueCube() { return false; } public boolean isNormalCube() { return false; } public boolean renderAsNormalBlock() { return false; } @SideOnly(Side.CLIENT) public IIcon getIcon(int p_149691_1_, int p_149691_2_) { return Blocks.planks.getBlockTextureFromSide(p_149691_1_); } @Override public TileEntity createNewTileEntity(World world, int id) { return new TileEntityTable(); } }
Render Class:
package com.bomb787.modelingmod.rendering.tileentities; import org.lwjgl.opengl.GL11; import com.bomb787.modelingmod.ModelingMod; import com.bomb787.modelingmod.models.ModelTable; import com.bomb787.modelingmod.rendering.tiles.TileEntityTable; import net.minecraft.client.renderer.tileentity.TileEntitySpecialRenderer; import net.minecraft.entity.Entity; import net.minecraft.tileentity.TileEntity; import net.minecraft.util.ResourceLocation; import net.minecraft.world.World; public class RenderTable extends TileEntitySpecialRenderer{ private ModelTable model; private ResourceLocation texture = new ResourceLocation("modelingmod:textures/blocks/Table.png"); public RenderTable() { this.model = new ModelTable(); } public void renderTileEntityAt(TileEntity tile, double x, double y, double z, float scale) { GL11.glPushMatrix(); GL11.glTranslated(x+1, y, z-0.5); GL11.glRotated(180, 0, 0, 1); this.bindTexture(texture); this.model.render((Entity)null, 0, -0.1f, 0, 0, 0, 0.0625f); GL11.glPopMatrix(); } }
Tile Entity Class:
package com.bomb787.modelingmod.rendering.tiles; import cpw.mods.fml.common.registry.GameRegistry; import net.minecraft.tileentity.TileEntity; public class TileEntityTable extends TileEntity{ }
How do I make it rotate?
Packet was larger than expected
in Support & Bug Reports
Posted
Whenever I try to play on my server it occasionally kicks me because a packet was larger than expected. It's getting really annoying to have to restart it every time and I want to fix it.
Latest log: https://pastebin.com/1EeqBHag I had to delete the stuff that didn't have to do with the server because pastebin didn't let me have one that big.
The debug log didn't have anything to do with the server.