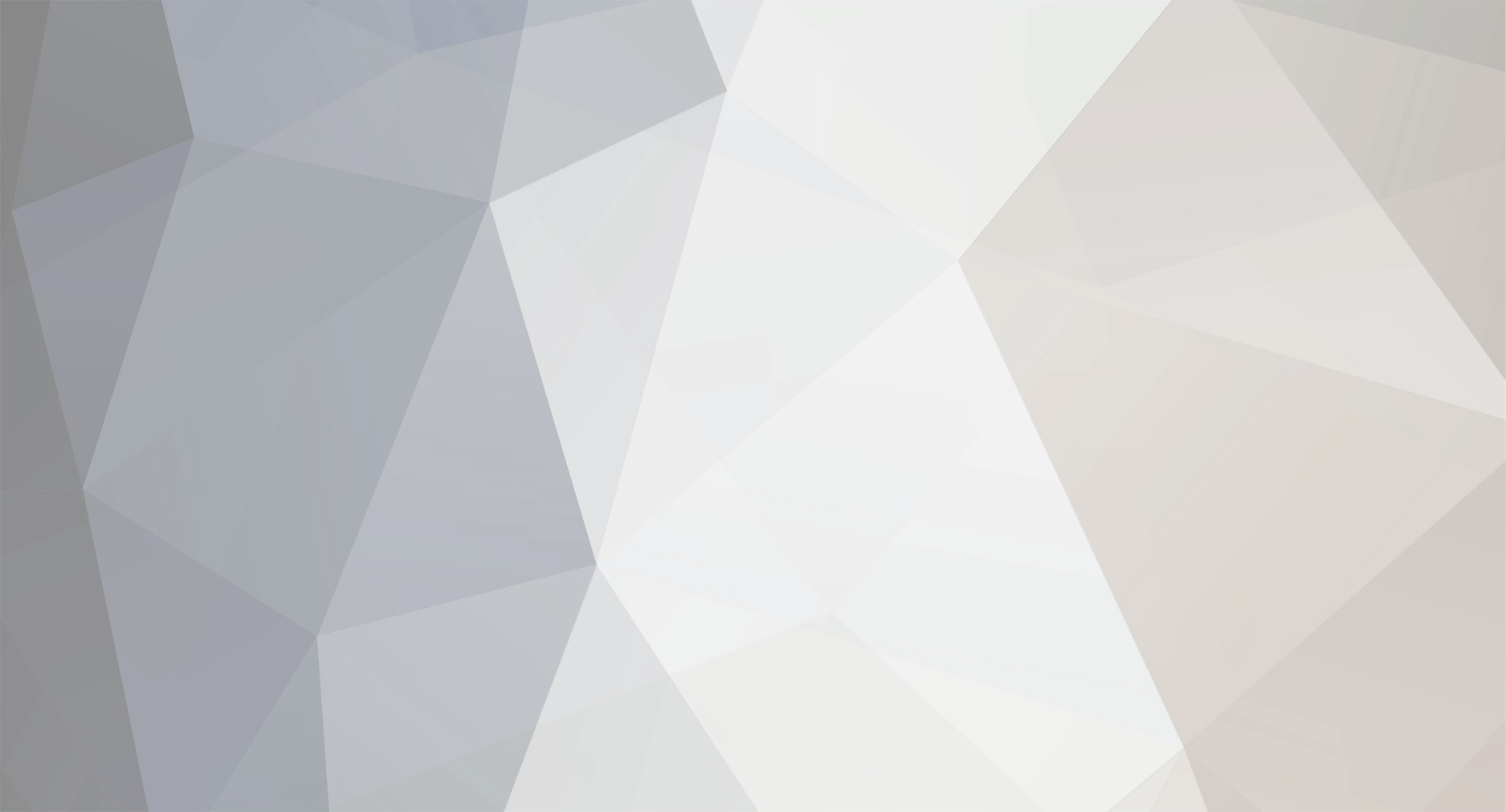
Korlimann
-
Posts
53 -
Joined
-
Last visited
Posts posted by Korlimann
-
-
Hey!
Just wanted to ask, did you find a solution? If so, could you tell me what the problem was, and did you use a tutorial or research yourself on how custom structures work? I want to try to make my own structure, but wasn't able to find a good tutorial on how to do it yet
-
14 minutes ago, Cadiboo said:
Chests are a TileEntity, not a normal block. What do you mean by "or similar"?
It rotates the current GL matrix with the values you specify. The values are angle (in degrees), x (either 1 or -1), y (either 1 or -1), z (either 1 or -1).
Thanks a lot! I think I confused what you meant with Texturemaps, sorry.
I just pushed all my changes. I still get a block with no textures though. Do you maybe have an idea why it won't render correctly?
-
7 minutes ago, Cadiboo said:
Don't do this if your trying to render an actual model
It stores all the normal textures of the game (Block & Item textures) in 1 texture for performance and a number of other reasons
Ah, thanks! So stuff like a chest or similar use Texturemaps?
And, do you know how the rotation method works?
-
22 hours ago, Cadiboo said:
Hey there again
I'm now in the process of trying to get my Entity to render correctly and had a few question regarding the classes you sent.So, I saw that the GlStateManager has a rotate() mehod, and I need my Model to be turned so that the blade will be horizontal to the player.
Furthermore, you get an ItemStack from your Dagger which appears really complicated to me. I tried to get it to work by simply creating the class and registering it in my ModItems and ItemRegistry Class. I was able to replace the green square by a block with no textures that rotates weirdly when shot.
So in order to maybe get the block to work, I replaced the TextureMap.LOCATION_BLOCKS_TEXTURE with the path to my textures, but the only thing that changed is that the block now has complete black textures instead of just the "missing textures" texture. What does the TextureMap actually do, and how can I get the Renderer to render my custom model?
-
2 hours ago, V0idWa1k3r said:
Are you sure about that? I can't replicate the issue. In fact I have debugged the entities and can confirm that there only exists one entity instance per respective side after the swing.
Sorry for the wait, I wasn't at home. I tried to replicate the issue https://imgur.com/JTOstpO
I hope you can see it here in the gif. When shooting through the wall, most of the times it (seems to) split up into two entities, I don't know though if this description is accurate. But when simply shooting in any direction, it looks like there's only one.
EDIT: Just to clarify, I haven't changed any of the code since the last push. -
14 minutes ago, V0idWa1k3r said:
Nope, didn't debug it to that extend but I suspect that is a client-side only glitch anyway.
Ah, okay. Either way, quite interesting, I think
Okay, so I followed your advice and it works a lot better now, although on every click, it spawns two entities at once now which seem to lay perfectly on each other. I experimented a little bit and when shooting through a wall, those two entities seem to change their direction a little, so you can actually see that it spawns twice everytime. I pushed my changes. Any idea why it get's spawned twice?
-
3 minutes ago, V0idWa1k3r said:
Store the entity's starting position and check when the distance between the current position and the starting one is greater or equal to 100*100(don't do the check that calculates the sqrt for you that's not needed in this case). Of course you must make sure that your entity won't stop - so it needs to go through all blocks and have a constant velocity.
Thanks, that's actually way smarter
-
Just now, V0idWa1k3r said:
You are not creating your throwable entitiy correctly. You need to tell it to be shot into a direction, and you are not telling it to do so. See how vanilla shoots it's projectiles at ItemSnowball for example. Literally every single one of your issues is fixed by adding the EntityProjectile#shoot call. Yes, I've debugged it
If you want to play the "swing" animation return false in your override of Item#onEntitySwing.
Thanks a bunch! I'm gonna check that out right now. By the way, do you have an idea why exactly the Projectile kept following the Players cursor? I'm curious ?
-
3 hours ago, Cadiboo said:
Can you push your changes to your repo too please?
Alrighty. So I finally arrived home and started working on it and I see the following problem.
I tried setting some fixed values for the motion variables. This stopped the Entity from following my mouse, but now it always shoots in the same direction, no matter which way I'm facing.
I suppose that, for what ever reason, the onUpdate method keeps getting the way the player is facing and updating the motion factors accordingly. I have absolutely no idea how and why this works.
But I did manage to let the Entity despawn by making an int that counts 1 up for every time the onUpdate method get's called. If the counter reaches 100, it despawns (and this should be approx. 100 blocks)
I also fixed the problem that it slowed down and just stood still by deleting the part where the motion get's multiplied by the motionFactor.
Do you maybe have any idea how I can stop the entity from "chasing the cursor"?
I also noticed that, when the motion variables are not fixed, the Entity won't spawn until I hit a second time, and when I do it spawns two of them right after each other.
When having the motion variables fixed, clicking twice with almost no delay makes the second Entity bounce off the first and change direction.
Any ideas?
EDIT: I just noticed that the Entity stops following the cursor when you turn really fast
EDIT 2: I managed to stop the Entity from following the cursor by setting the RayTracerResult only in the constructor and making a private variable to it, so no new RayTracerResult gets created every onUpdate, however, I noticed why you need to click 2 times before it starts flying. The first one apparently get's spawned right were you stand and every Entity that spawns after that collides with the first one and get's shot of in the direction you're looking. If you move fast while clicking, you will have many entities just floating around. -
Just now, Cadiboo said:
Can you push your changes to your repo too please?
Done. You wanna have a look at my new magic missile class?
-
Just now, Cadiboo said:
The way I would do it would be to extend entity throwable, move the entity & check how long it’s been alive in the onUpdate method and handle collisions (blocks and entities) in the onImpact method
Okay, I think I'll start with that as soon as I'm back home. Thanks!
-
2 minutes ago, Cadiboo said:
So, you’ve somehow extended a generic class with no specific functionality and suddenly have a magic missile that follows your cursor? I want programing magic like that to happen to me!
I think it's awesome too, although it's not really what I wanted
-
8 minutes ago, Cadiboo said:
I suggested entity throwable because it has a good collision method that you can override, I didn’t know that you were making a magic missile (it’s been a long time since I played terraria) type entity. For the collision you need to do appropriate stuff in the onImpact method. How are you implementing the magic missile functionality?
No no no, I don't want to make a magic missile. I want to do something like this. I don't know how or why it works like that, but it does and I have no idea on how to fix it.
On 11/18/2018 at 2:30 PM, Korlimann said:So, basically I want this sword to spawn one of those green swords in the gif as entity that shoots out until it has either traveled 100 blocks OR hit 3 enemies. It should move approx. like a fireball, but with more accuracy. So, you shoot it and it shoots exactly in the direction you're looking at. It doesn't drop down, go up or anyone else, just travels into the direction you looked at when firing it with the same speed and everything, until it should be declared dead.
-
3 minutes ago, Cadiboo said:
Thanks a lot! I'll check it out as soon as possible. Right now, I changed the Entity to be extending EntityThrowable, which gave me some funny results.
https://imgur.com/a/USerdh4
As you can see in the gif, the block now gets... ...somewhat rendered? And seems to behave like a fireball (which might be because I took the code from the RenderEntityFireball class, but I'm not sure anymore)
I always need to doubleclick for it to shoot, altough shooting only once does the job too, but only sometimes, which is quite confusing. Also, after shooting, while moving it behaves somewhat like a "magic missile" and follows the cursor, but stops after maybe 3-4 seconds of flying. I think this is probably due to EntityThrowable not having acceleration, so I had to cut a lot of code pieces out. Also, the entitys have no collisions and just disappear in the underground BUT they can push each other, when you shoot them at each other after they stopped and float in the sky.I don't even know where to begin first to fix all of this.
-
On 11/20/2018 at 12:48 AM, Cadiboo said:
That link works, as I said, you should probably be extending entity throwable instead of entity fireball. Take a look at https://github.com/Cadiboo/WIPTechAlpha/blob/fb5883e9d76ef0361ec1ebbcb9c508611dd2ef6b/src/main/java/cadiboo/wiptech/entity/projectile/EntityNapalm.java#L18-L89
Ignore the igniteBlocks method (it sets everything on fire) and lines 83-85 (they deal with approximating the nearest air block to the collision point)
Hey there! Sorry for not answering so long.
Thanks for the suggestion, I'm gonna try to implement it like that, but I still think that the main issue is, that my Renderclass doesn't work, because I don't know how to operate the GLStateManager
-
3 hours ago, Cadiboo said:
Your GitHub links all return
I don't know why that is, everything works fine for me. Does that link work for you?
-
10 hours ago, Cadiboo said:
Make an entity that dies after a few ticks, and doesn’t stop/die on collision. You should be able to just extend entity throwable and spawn some particles on its path
Well, for what I know, the green swords actually flies 2500 blocks (in Terraria) and then dies, or it dies after colliding with a wall OR hitting 3 enemies, so it's not that easy. Currently, it's working that the entity flies at least somewhat far before the particle track disappears, but I have two rather big problems.
First one is, that the entity doesn't render, and I don't know how to render it.
Second problem is, that it's somewhat random in which direction it shoots. I want it to fly directly at the point where the players crosshair is, like a fireball, without changing direction. But as it is of now, it feels more like shooting a shotgun, sometimes the entity will even come out behind me or on my side, and I don't know which piece of code makes it do that. -
1 hour ago, V0idWa1k3r said:
Well, technically you didn't. You can't do something wrong if you don't do anything in the first place.
Unless your repository is outdated your entity renderer does absolutely nothing apart from existing. You need to actually tell the game how to render your entity, it's not going to read your mind and magically work. You do have some code there, but it's commented out and thus isn't even compiled.
@V0idWa1k3r I commented it out since it did not work but I didn't want to throw it away, so I left it there. Sorry, I should've mentioned it.
-
Good evening everybody!
I'm trying to create a custom projectile, but I'm having trouble to get it to render.
As of now this is what my mod looks likeRight now, when left clicking the sword, the particles get generated behind the flying entity and when it hits something, the sound gets played, but the Entity itself doesn't render.
I suppose I did something wrong in the Render class for the Entity (and to be honest I haven't really understood how the Render classes work)
I'd be very happy if someone could push me in the right direction.Thanks
-
2 hours ago, Cadiboo said:
I think for the item you should extend ItemSword and override the leftClick method (I’ve forgotten what it’s called). What is your entity meant to be? A thrown sword or something else?
That's what my Item is currently doing. It extends ItemSword.The method I think you mean is called onLeftClickEntity and it's for attacking an Entity directly, so it will only be called when the player actually hits another entity with the sword, which would kind of miss the point of the sword, because it should not only be a melee weapon but also some sort of magic ranged weapon, so if I wanted to hit a mob further away, with this method it wouldn't work.
EDIT: I just noticed I didn't answer your question. It's meant to be the Terra Blade from Terraria
This is how it looks like in Terraria.
I want to create a sword that shoots the green sword as seen in the gif upon attacking or rather left clicking.
As of now, I have the sword, but now I need an Entity that behaves like the green sword. -
46 minutes ago, V0idWa1k3r said:
Well, you still have a CommonProxy, for one.
You are not actually doing any rendering, just screwing up the matrix stack, but I assume this is still unfinished, similar to how the sword currently does nothing.
Don't have your proxy as an event subscriber. You already have a dedicated class for that.
Why are you calling onUpdate in the constructor? That is a very bad idea that is likely to crash the game.
Okay, so I think I fixed everything you mentioned here. I looked at your code and kinda confused ClientRegistry and ClientProxy, but I think it should be fine now
Also, yes, the Render class is unfinished. I'm currently trying to figure out everything I'll need to get the entity to work.
So, looking at it now, my ItemTerraBlade will be the class that "spawns" the Entity. I think the method I want to use for this is "onEntitySwing", which should be called everytime when the Player that holds the item swings it, if I'm not mistaken.
I already noticed that when using this class in addition to my EntityTerraBladeProjectile, since it's extended from the Fireball, the sword doesn't actually "swing" anymore, but instead does the animation that comes up when shooting a fireball. So my first question would be, should I use another method? Or is there another way to maintain the swinging animation.
Moving on from ItemTerraBlade, everytime it gets swung, a new EntityTerraBladeProjectile should spawn. I'm a little unsure, but the EntityTerraBladeProjectile class should declare how fast and in which direction my Entity in this case moves and also what happens on impact or when it moves out of sight.
Since I want it to behave like a fireball, it should shoot straight in the direction of where the player is looking at, which I figured shouldn't be too hard, since this is basically what a fireball does anyways. But since I made a custom model for the entity, it should have specific rotation. Basically, it should always be that the blade of the entity (which currently looks like this:)
is horizontal to the players face
Despite that, this should basically be it for the Entity class. Of course it will still need to damage entitys it hits and I also wanted to make some sort of animation when it hits something, but that's something for later I suppose.
Next would be the RenderEntityTerraBladeProjectile class. I think this is the class where the Entity actually gets rendered, so I suppose the code that makes it so that the entity has the right rotation when spawning should be placed inside this class.Other than that there should be a custom resource location declared so that the Entity will have textures, but it also leaves me wondering if I'll need a class that extends from ModelBase in order for my custom model to work.
Last but not least, the Entity will need to be registered, once the entity itself in the EntityRegistry, but also the Renderer in the ClientProxy (so I'm guessing I won't need the RenderRegistry class I made?)
I believe this should be everything I need for an entity to work, right?
-
Thanks again for the explanation and the help yesterday. I tried to follow as many point as you mentioned and basically started over, threw the IHasModel out, created ObjectHolders, renamed the mod_id and name and so on. I also deleted all the entity related classes and started over. I recreated them but more like the general class and constructor without copying too much code, trying to write only those parts I'm sure I understand.
I would be very thankful if you could have a quick look and tell me if there's anything I'm still doing wrong and what I'll need now to get the Entity to work.
-
@V0idWa1k3r Thanks a ton for all the suggestions, the links and the help in general. I just wanted to let you know that I'm going to be away from home soon so I don't have time to respond. I'll answer tomorrow. Thanks!
-
Quote
I mean you have them right there, above your code and still go against what they tell you to do.
As for the issue - you are not setting the position of the entity you are spawning so it spawns at [0,0,0]. You can see an example of an item creating a projectile at ItemBow for example.
I know, that wasn't really a smart idea. I just thought that maybe the entity is not spawning because of this, so I wanted to make sure that it spawns anyway. I already deleted the else but haven't pushed it yet.
QuoteWhat explanation would you like exactly? I am happy to provide, but you need to ask your questions first. Entities are kinda complex.
Well, how entities work in general. As far as I understood, certain items, such as snowballs for example generate (is that the correct term?) a entitythrowable when, for example, right clicked. This entity will need to be rendered seperatly, and I saw someone use some kind of ModelBase instead of a Render if I remember correctly, so I guess if you want the entity to have it's own model, you'll need a class that extends ModelBase.
You also need to register the entity, for me this would be in the ObjectRegistry, but also in the ClientProxy.
Is this correct, or did I mess anything up?Also I wondered how my entity will be moving when spawned. I saw some variables like velocity or motion, but I don't think I understood them yet.
QuoteCommonProxy makes no sense. Proxies exist to separate sided-only code that only exists on a specific physical side. If your code is common it goes anywhere else but your proxy.
If had made a mod some time ago, also in 1.12.2 and the tutorial I followed to create this had me create a ClientProxy and CommonProxy class. I have no idea why it's called like that and there wasn't much of an explanation going on, so I figured it wouldn't be that important and is simply needed to register stuff. (And since I'm unable to find any good tutorial on 1.12.2 and the documentation is far from existent, I didn't bother searching for it, just like for many other things)
1 hour ago, V0idWa1k3r said:This makes even less sense. A server proxy either provides methods that would crash the client if executed on it or noop implementations for client-only stuff. Your common proxy can't be your server proxy because if it would it would crash the client.
^^See above. I'd be happy if you could quickly explain the concept of the Client, Server and CommonProxy classes to me.
1 hour ago, V0idWa1k3r said:es is a terrible modid. You have 64 characters for a reason.
Well, thanks. I'm going to change this as soon as possible.
1 hour ago, V0idWa1k3r said:The name property is supposed to be a user-friendly name of your mod.
Just like the modid, I just typed something in real quick. I don't even really have a name for the mod yet, I just thought the general concept of the mod was nice and wanted to get to work as soon as possible. After all, there should be enough time to think of one during the process of making the mod. But I'm also going to change this, as soon as I want to publish it/have a name for it.
1 hour ago, V0idWa1k3r said:IHasModel is stupid. All items need models, no exceptions, and nothing about model registration requires access to private/protected data of the item. Register your models in the ModelRegistryEvent directly.
I also created this, basically copied it from a tutorial. So, if I get you correctly, I don't need the Interface "IHasModel" at all, right? But, where is the ModelRegistryEvent, and how do I register models without the class?
1 hour ago, V0idWa1k3r said:This is kinda nitpicky but why is your entity renderer in your proxy package of all places?
It's like that because I checked the original minecraft files and found the renderer.entity package inside the client package. And because my client package is inside the proxy package, I simply put it there, because I thought it would have more structure like that, but I guess it doesn't
1 hour ago, V0idWa1k3r said:ItemBase is an antipattern. There is already an ItemBase, it's called Item.
I'm starting to question the tutorials I watched. A lot, actually. Why is there not a single good tutorial for 1.12.2?
1 hour ago, V0idWa1k3r said:Don't ever use static initializers. Instantinate your stuff in the appropriate registry event. If you need a reference to the thing later use ObjectHolders. Same applies to your entity entry btw.
So, I would be fine with this line of code if I simply deleted the static, or do I need anything else to make it work then?
1 hour ago, V0idWa1k3r said:This is probably the most, um, interesting way to use IHasModel. Especially considering that the renderer must be registered in preinit, not in a ModelRegistry event. Also the implementation
@Override public void registerModels() { Main.proxy.registerItemRenderer(this, 0, "inventory"); }
Entity is not an item. It makes negative sense to call a "registerItemRenderer" for an Entity. Actually now when I look at your methods this doesn't even compile. Are you using an IDE?
After 10 hours of trying to get this to work, I became kinda desperate and tried all sort of random (and dumb) stuff. Since there was no model showing up for the entity, I just put this there and tried, but it didn't work either. And yes it did compile, since I was "smart" enough to create another registerItemRenderer() method only for EntityTerraBladeBeam
And yes, I am using eclipse.So far, thanks a bunch for all the stuff you made me realize is wrong and thanks for the quick answer. I'll probably just throw everything out and start all over again.
EDIT: I just thought, would it maybe be smarter to extend EntityArrow? Can I change my EntityTerraBladeBeam to fly until it hits something or is not in sight anymore?
[1.14.4] Custom Animated Java Models?
in Modder Support
Posted
Hello everyone.
I'm currently trying to figure out how to use Java Classes for Models in my Mod. A friend of mine created a .java Model with Blockbench, but it appears the class generated is not for 1.14.4, but probably for 1.12, since most of the Classes used in this Class are relocated somewhere else, or renamed entirely.
Specifically, I'm searching for these classes:
I was able to find ModelBox, but that was the only class, all the others seem to be renamed or relocated, and I'm not sure which one is which.
Furthermore, I'm guessing that these classes have changed in how they work between 1.12 and 1.14.
(It appears pastebin is down so I'm posting the class here)
If any of you guys could tell me what needs to be changed, or have a link so I can read up on Models in 1.14 (I've looked through the forge docs, maybe I overlooked it but I couldn't see anything regarding .java models) or a link to a working example or tutorial, I'd greatly appreciate that.
Thanks!