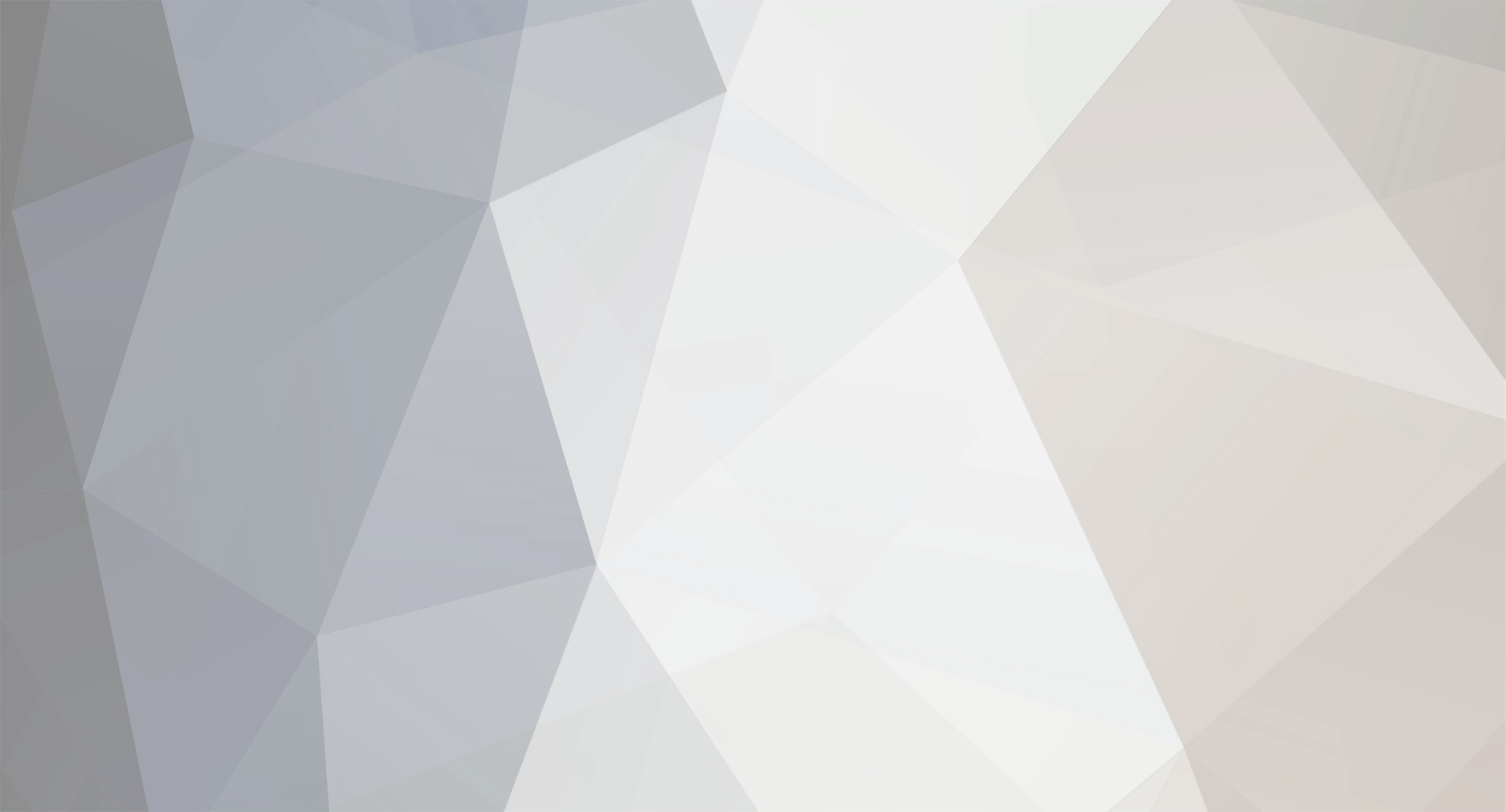
boyx
Members-
Posts
20 -
Joined
-
Last visited
Everything posted by boyx
-
I no longer need to. I found a fix for that problem but it still crashes because of the Capability. I dont know what i did wrong. I made a class where i used @CapabilityInject in and i put that in my preInit. The game only crashes when i get the Item in my inventory.
-
What should i update? The forge version or the mapping or what?
-
I am exactly in the same class and dont find this
-
package boyx.arandomstuff.handlers; import net.minecraft.util.EnumFacing; import net.minecraft.util.ResourceLocation; import net.minecraftforge.common.capabilities.Capability; import net.minecraftforge.common.capabilities.CapabilityInject; import net.minecraftforge.common.capabilities.ICapabilityProvider; import javax.annotation.Nonnull; import javax.annotation.Nullable; import boyx.arandomstuff.Reference; public class InternalTimers { @CapabilityInject(InternalTimers.class) public static final Capability<InternalTimers> CAPABILITY = null; public static final ResourceLocation NAME = new ResourceLocation(Reference.MODID, "internal_timers"); private final Timer repair = new Timer(); private final Timer heal = new Timer(); private final Timer feed = new Timer(); public void tick() { if (repair.shouldUpdate) { repair.tickCount++; repair.shouldUpdate = false; } if (heal.shouldUpdate) { heal.tickCount++; heal.shouldUpdate = false; } if (feed.shouldUpdate) { feed.tickCount++; feed.shouldUpdate = false; } } public void activateRepair() { repair.shouldUpdate = true; } public void activateHeal() { heal.shouldUpdate = true; } public void activateFeed() { feed.shouldUpdate = true; } public boolean canRepair() { if (repair.tickCount >= 19) { repair.tickCount = 0; repair.shouldUpdate = false; return true; } return false; } public boolean canHeal() { if (heal.tickCount >= 19) { heal.tickCount = 0; heal.shouldUpdate = false; return true; } return false; } public boolean canFeed() { if (feed.tickCount >= 19) { feed.tickCount = 0; feed.shouldUpdate = false; return true; } return false; } public static class Provider implements ICapabilityProvider { private final InternalTimers capInstance = new InternalTimers(); @Override public boolean hasCapability(@Nonnull Capability<?> capability, @Nullable EnumFacing facing) { return capability == CAPABILITY; } @Override public <T> T getCapability(@Nonnull Capability<T> capability, @Nullable EnumFacing facing) { if (capability == CAPABILITY) return CAPABILITY.cast(capInstance); else return null; } } private static class Timer { public int tickCount = 0; public boolean shouldUpdate = false; } } Thats the class i did the Capabilitys in. I dont know much and i didnt understand the documentation well.
-
In Minecraft the class ItemStack doesnt own a Method called isEmpty() somehow... I dont even know if this is the error. How i said some post above the crashlog says there too many ticks and something with the Capability doesnt work. [Edit] Btw the game crashes whenever i get the Repairer Item in my inventory.
-
It is undefined and not working in ItemStack...
-
Yes i tried that but it also doesnt work. I know many basic things in java. I also programmed some programs but not too complicated. I never did something like this advanced modding.
-
After i deleted the stack.isEmpty then yes it does
-
@CapabilityInject(InternalTimers.class) public static final Capability<InternalTimers> CAPABILITY = null; public static final ResourceLocation NAME = new ResourceLocation(Reference.MODID, "internal_timers"); I did this with the capability.
-
Minecraft Crash Report says this line is the error?
-
This is the crash report. Maybe it has nothing to do with the isEmpty error...
-
Do you know something i could use instead of this?
-
There are many things that can return a boolean but nothing i need i think. What i need is that it returns true if the ItemStack is empty for that i always used ItemStack.Empy or ItemStack.isEmpty and both dont work.
-
When i hover over it it says : isEmpty cannot be resolved or is not a field In the other mods ive just did the same thing. I created a new ItemStack stack = inv.getStackInSlot(i); and then just did invStack.isEmpty; to get a boolean.
-
Ok i expressed myself wrong. I know Java and i also know why its marked red but i dont understand why because i used this alot in some mods from me but only here it doesnt work. Thats what i ment by this
-
package boyx.arandomstuff.items; import boyx.arandomstuff.Reference; import boyx.arandomstuff.api.item.IModeChanger; import boyx.arandomstuff.handlers.InternalTimers; import boyx.arandomstuff.init.ModItems; import boyx.arandomstuff.util.ItemHelper; import net.minecraft.entity.Entity; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.inventory.EntityEquipmentSlot; import net.minecraft.item.Item; import net.minecraft.item.ItemStack; import net.minecraft.util.ResourceLocation; import net.minecraft.world.World; import net.minecraftforge.items.CapabilityItemHandler; import net.minecraftforge.items.IItemHandler; public class ItemRepairer extends Item { public ItemRepairer(String unlocalizedName, String registryName) { this.setUnlocalizedName(unlocalizedName); this.setRegistryName(new ResourceLocation(Reference.MODID, registryName)); this.setMaxStackSize(1); this.setMaxDamage(100); } @Override public void onUpdate(ItemStack stack, World world, Entity entity, int par4, boolean par5) { if (world.isRemote || !(entity instanceof EntityPlayer)) { return; } EntityPlayer player = (EntityPlayer) entity; player.getCapability(InternalTimers.CAPABILITY, null).activateRepair(); if (player.getCapability(InternalTimers.CAPABILITY, null).canRepair()) { repairAllItems(player); } } private void repairAllItems(EntityPlayer player) { IItemHandler inv = player.getCapability(CapabilityItemHandler.ITEM_HANDLER_CAPABILITY, null); for (int i = 0; i < inv.getSlots(); i++) { ItemStack invStack = inv.getStackInSlot(i); if (invStack.isEmpty || invStack.getItem() instanceof IModeChanger || !invStack.getItem().isRepairable()) { continue; } if (invStack == player.getItemStackFromSlot(EntityEquipmentSlot.MAINHAND) && player.isSwingInProgress) { //Don't repair item that is currently used by the player. continue; } if (ItemHelper.isDamageable(invStack) && invStack.getItemDamage() > 0) { invStack.setItemDamage(invStack.getItemDamage() - 1); } } } } This should repair every Items in my Inventory in every few seconds. Ive got one problem whenever i get the Item in my inventory my MC crash's. It could be that I removed invStack.isEmpty (i didnt removed it in the code above) is marked red and i dont know why. If you want me to post the crash report just ask. Thank you!
-
Ok i know how to time a repair but i want to repair other Items in my inventory. How do i do this?
-
package boyx.arandomstuff.items; import boyx.arandomstuff.Reference; import net.minecraft.entity.Entity; import net.minecraft.item.Item; import net.minecraft.item.ItemStack; import net.minecraft.util.ResourceLocation; import net.minecraft.world.World; public class ItemRepairer extends Item { public ItemRepairer(String unlocalizedName, String registryName) { this.setUnlocalizedName(unlocalizedName); this.setRegistryName(new ResourceLocation(Reference.MODID, registryName)); this.setMaxStackSize(1); this.setMaxDamage(100); } @Override public void onUpdate(ItemStack stack, World worldIn, Entity entityIn, int itemSlot, boolean isSelected) { stack.setItemDamage(stack.getItemDamage() - 1); } } This is the code i recently tried.
-
I recently coded another mod but i ran into a problem. I wanted to do an Item that repairs every other Item in the inventory of the player who got the Item. In my Class ive got a onUpdate method but it dont work out like i want. It should repair EVERY Item in the inventory every 3 - 6 seconds (60 Ticks - 120 Ticks) and it should work without holding it in the hand. So i need a tick timer and a method that repairs everything.
-
I recently coded another mod but i ran into a problem. I wanted to do an Item that repairs every other Item in the inventory of the player who got the Item. In my Class ive got a onUpdate method but it dont work out like i want. It should repair EVERY Item in the inventory every 3 - 6 seconds (60 Ticks - 120 Ticks) and it should work without holding it in the hand. So i need a tick timer and a method that repairs everything. [EDIT] = The version i am coding on is 1.7.10 Thanks for you suggestions. -boyx