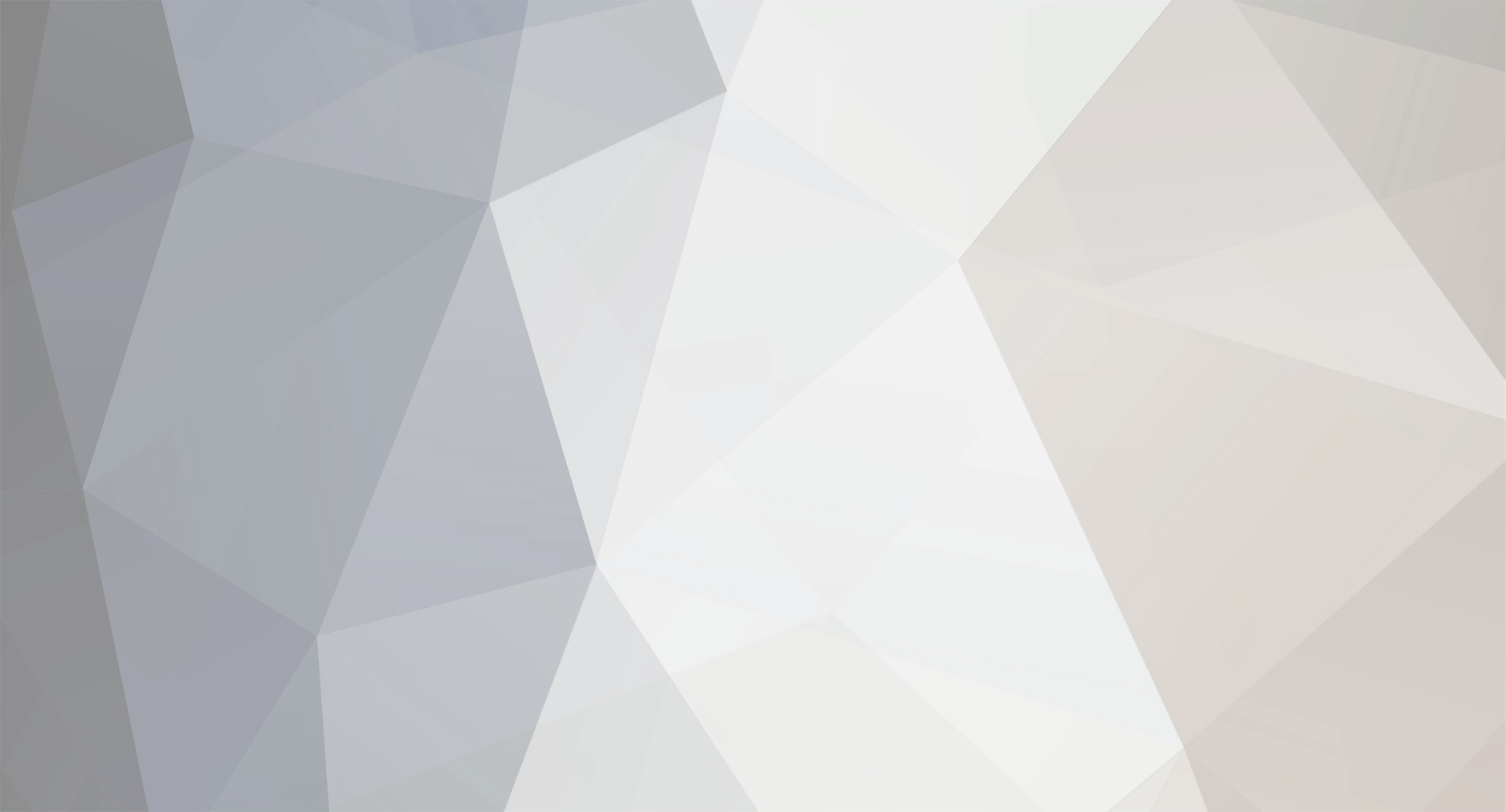
cptcorndog
-
Posts
7 -
Joined
-
Last visited
Posts posted by cptcorndog
-
-
Thank you that's exactly what I've done. For some reason, the forge example is making the subcategory class private which makes it more cumbersome to access from main code
-
Trying to figure out best way to access Config file variables from code when subcategories are used... (using @Config annotation per Forge). Below is their example of how to make subcategories, however, there seems to be some access issues with this
@Config(modid = "modid") public class Configs { public static SubCategory subcat = new SubCategory(); private static class SubCategory { public boolean someBool; public int relatedInt; } }
Anyone have a good example of how they are doing this using the @config way
Thanks all!
-
Out of curiosity and desire to have not only working but well-written code, wondering the best way to implement IGuiHandler while respecting sidedness
The below code works (but only with a @SideOnly annotation in TileEntity when run on dedicated server). If not using annotation will throw:
java.lang.RuntimeException: Attempted to load class net/minecraft/client/gui/inventory/GuiContainer for invalid side SERVER
I have currently implemented my IGuiHandler as:
public class GuiHandler implements IGuiHandler { @Nullable @Override public Object getServerGuiElement(int ID, EntityPlayer player, World world, int x, int y, int z) { BlockPos pos = new BlockPos(x, y, z); TileEntity te = world.getTileEntity(pos); if (te instanceof IGuiTile) { return ((IGuiTile) te).createContainer(player); } return null; } @Nullable @Override public Object getClientGuiElement(int ID, EntityPlayer player, World world, int x, int y, int z) { BlockPos pos = new BlockPos(x, y, z); TileEntity te = world.getTileEntity(pos); if (te instanceof IGuiTile) { return ((IGuiTile) te).createGui(player); } return null; } }
IGuiTile
public interface IGuiTile { Container createContainer(EntityPlayer player); GuiContainer createGui(EntityPlayer player); }
and my Tile Entity subclass has the following implementation:
@Override public Container createContainer(EntityPlayer player) { return new ContainerFurnace(player.inventory, this); } @SideOnly(Side.CLIENT) @Override public GuiContainer createGui(EntityPlayer player) { return new GuiFurnace(this, new ContainerFurnace(player.inventory, this)); }
ps. above code was largely from a Mcjty tutorial as I was learning, and his didn't use the @SideOnly. I don't think he ran his on dedicated server during the tutorial to find this error...
Thanks all!
-
1 hour ago, MrDireball said:
I'm trying to register the TileEnity for my custom block called "data_block", but Forge says that the method I'm using is obsolete, and also I don't even know if I'm doing it right.
GameRegistry.registerTileEntity(DataTileEntity.class, Reference.MOD_ID + ".data_block"); //This is in preInit of Main.
I say that I don't know if I'm doing it right because I've also seen different people us all of the following when entering the path string for TileEntites.
Reference.MOD_ID + "data_block"
Reference.MOD_ID + ".data_block"
Reference.MOD_ID + "_data_block"
Reference.MOD_ID + ":data_block"
I believe it needs to be a ResourceLocation instead of just a String
e.g.,
GameRegistry.registerTileEntity(DataTileEntity.class, new ResourceLocation(Reference.MOD_ID + ".data_block"));
-
1
-
-
47 minutes ago, unassigned said:
You should make a custom ItemStackHandler that extends ItemStackHandler in which you overwrite insertItem and extractItem, and check if the itemstack is valid. I have a small example of what I mean here:
But this isn't necessarily needed, as you should be able to modify isItemValid when instantiating the ItemStackHandler:
public final ItemStackHandler inv = new ItemStackHandler(1) { @Override public boolean isItemValid(int slot, @Nonnull ItemStack stack) { return super.isItemValid(slot, stack); //check stack. } };
Also, make sure you are exposing the ItemHandler capability, or your inventory will not work properly.
The latter is what I'm trying to accomplish but Eclipse is raising an error when I try to override this method, and when I search through ItemStackHandler and IItemHandler I don't see this method declaration...
-
Other sources have suggested overriding the isItemValid method of IItemHandler to check if an item can be placed in a slot (e.g., only allow smeltable items in a furnace). I don't see this method available in documentation
Should I be performing this check inside an overridden insertItem method instead?
Thanks! brand new to modding so bear with me
[1.12.2] @Config subcatgories
in Modder Support
Posted
Yup, on it, just thought I was missing something obvious for it to be otherwise