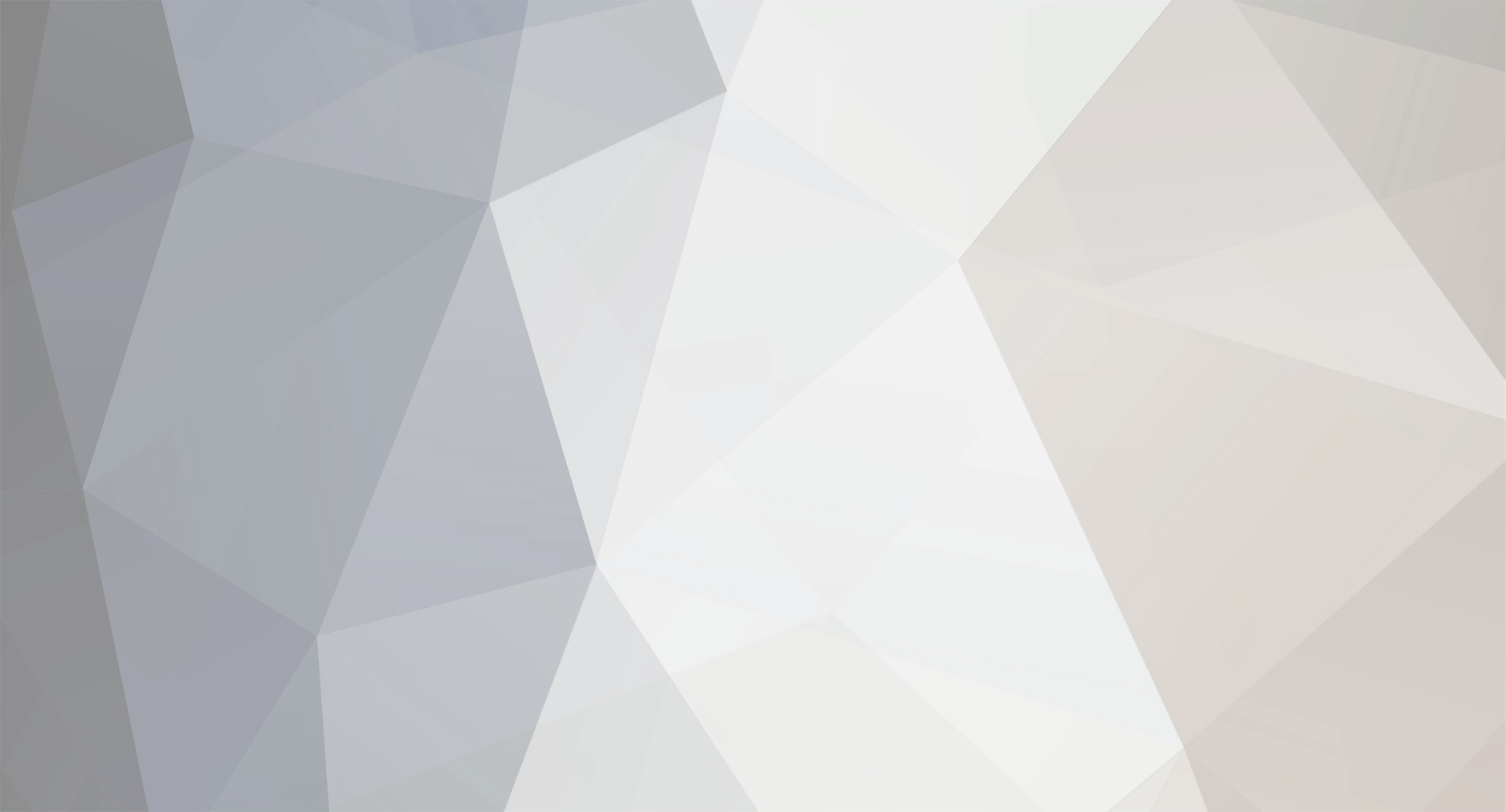
X Squishling X
Members-
Posts
26 -
Joined
-
Last visited
X Squishling X's Achievements

Tree Puncher (2/8)
3
Reputation
-
private static BlockState updateDistance(BlockState blockState, IWorld world, BlockPos blockPos) { int i = distance; try (BlockPos.PooledMutableBlockPos pos = PooledMutableBlockPos.retain().setPos(blockPos)) { loop: for (int k = -1; k < 2; k++) { for (int l = -1; l < 2; l++) { for (int m = -1; m < 2; m++) { if (k == 0 && l == 0 && m == 0) continue; pos.setPos(blockPos.getX() + k, blockPos.getY() + l, blockPos.getZ() + m); i = Math.min(i, getDistance(world.getBlockState(pos)) + 1); if (i <= 1) break loop; } } } } return blockState.with(DISTANCE, Integer.valueOf(i)); } distance is 7, I just did this so I can easily control it.
-
After testing, this updated code still seems to only work horizontally. Does anyone know what's wrong with it? I also updated to this line: if (Math.min(i, getDistance(world.getBlockState(pos)) + 1) < i) i = Math.min(i, getDistance(world.getBlockState(pos)) + 1); Which should take the lowest distance, to see if this could fix something. What else can I try? Multiple people have told me they really want me to keep my current leaves design.
-
I belive I have fixed it, this is for everyone else that finds this thread: This is the update distance method I have created. I loop through every block in a 3x3 cube around the block, ignoring (0, 0, 0) because that is the block itself (continue will just skip to the next cycle of the loop). I now add these offsets (-1 to 1) to the position to get the blocks around the leaves block. I then set i to the min (it takes the lower) of the distance of this block plus one (because this leaves block is one block over). i is 7 usually, so this means a max range for checking of 7, but you can change that although that also means you will have to change the 7 in multiple places in the code. The get distance method handles what block this is and getting the distance property. If the distance is 1 (or less) we exit. This is because it means we are right next to a log, and otherwise we will keep on searching in case we find another, shorter distance. Finally, we return a block state with the distance property set to the value of i, aka our distance. private static BlockState updateDistance(BlockState p_208493_0_, IWorld p_208493_1_, BlockPos p_208493_2_) { int i = 7; try (BlockPos.PooledMutableBlockPos pos = PooledMutableBlockPos.retain().setPos(p_208493_2_)) { loop: for (int k = -1; k < 2; k++) { for (int l = -1; l < 2; l++) { for (int m = -1; m < 2; m++) { if (k == 0 && l == 0 && m == 0) continue; pos.setPos(p_208493_2_.getX() + k, p_208493_2_.getY() + l, p_208493_2_.getZ() + m); i = Math.min(i, getDistance(p_208493_1_.getBlockState(pos)) + 1); if (i <= 1) break loop; } } } } return p_208493_0_.with(DISTANCE, Integer.valueOf(i)); } For our leaves to work properly, we will also need to update the distance method to ensure it works with our leaves class: private static int getDistance(BlockState neighbor) { if (BlockTags.LOGS.contains(neighbor.getBlock())) { return 0; } else { return (neighbor.getBlock() instanceof LeavesBlock || neighbor.getBlock() instanceof CustomLeaves) ? neighbor.get(DISTANCE) : 7; } } All other methods in the leaves class are copied from LeavesBlock, so you will have to copy the contents of that. Warning: Because we can't edit the normal leaves block class without a core mod, normal leaves won't be able to see that our leaves exist, and so therefore will only work if a normal leaves block is connected to the log. This is usually not an issue, because almost always only one type of leaves is connected to a log.
-
I know what a mutable block position is, but I've had issues with them in the past so I decided to do this. The reason I am asking for help on this is because I want to know the correct way to do it. I get what you are saying on the other things, but some of it was left over from vanilla where I just tried to edit it, such as the '+ 1' check. Am I looking at the right method? The vanilla code is hard to read sometimes with the lack of comments, and the weird names I assume come from decompiling the code. This is also my first time working with 1.14, although I am sure the leaves aren't very different in 1.12. Oh, and it doesn't work if I move the label below because I get an error that at the time of the break 'loop' wasn't defined.
-
I have a palm tree where some leaves stick out diagonally. I'm wondering what the best way to make the leaves support this is? I have tried to override the updateDistance method like so: private static BlockState updateDistance(BlockState p_208493_0_, IWorld p_208493_1_, BlockPos p_208493_2_) { int i = 7; try (BlockPos.PooledMutableBlockPos blockpos$pooledmutableblockpos = BlockPos.PooledMutableBlockPos.retain()) { loop: for (int k = -1; k < 2; k++) { for (int l = -1; l < 2; l++) { for (int m = -1; m < 2; m++) { if (k == 0 && l == 0 && m == 0) break loop; BlockPos pos = new BlockPos(blockpos$pooledmutableblockpos.getX() + k, blockpos$pooledmutableblockpos.getY() + l, blockpos$pooledmutableblockpos.getZ() + m); i = Math.min(i, getDistance(p_208493_1_.getBlockState(pos)) + 1); if (i == 1) break loop; } } } } return p_208493_0_.with(DISTANCE, Integer.valueOf(i)); } But this just makes my leaves decay even next to the log (it is a vanilla spruce log so the log shouldn't matter). How could I make it support my palm tree? The 'fronds' are about 4-5 blocks long, for reference and some are diagonal.
-
I have a dimension which uses similar generation to the nether. I have made a biome to go in the dimension, along with a custom tree that I give to the biome using getRandomTreeFeature. Even if I set the top block of the biome to grass (I have made a custom system to handle this), the trees do not generate. Looking at the logs, it seems like every time it tries to generate a tree, it either picks bedrock, air, or stone (the main block of the dimension), and always within 20 blocks vertically of the top roof bedrock, however if I use a vanilla biome it spawns their trees just fine. Does anyone know how to change the trees to spawn on the different layers of my dimension?
-
Grass generation in nether-type dimension
X Squishling X replied to X Squishling X's topic in Modder Support
Place the replaceTopBlocks method in decorate. -
Grass generation in nether-type dimension
X Squishling X replied to X Squishling X's topic in Modder Support
You'd probably want to replace the air check with something else, because it means it wont replace the block under tallgrass and such. -
Grass generation in nether-type dimension
X Squishling X replied to X Squishling X's topic in Modder Support
private void replaceTopBlocks(int chunkX, int chunkZ) { int x = (chunkX * 16) + 8; int z = (chunkZ * 16) + 8; for (int a = x; a < x + 16; a++) { for (int b = 0; b < world.getHeight(); b++) { for (int c = z; c < z + 16; c++) { replaceTopBlock(a, b, c); } } } } private void replaceTopBlock(int x, int y, int z) { BlockPos position = new BlockPos(x, y, z); if (topBlockReplacements.containsKey(world.getBlockState(position)) && world.getBlockState(position.up()).getBlock() == Blocks.AIR) { world.setBlockState(position, topBlockReplacements.get(world.getBlockState(position))); } } This is the code I am using, topBlockReplacments is a HashMap of two IBlockStates; the first being the original block, second being the block to replace it with. -
Grass generation in nether-type dimension
X Squishling X replied to X Squishling X's topic in Modder Support
Nevermind, fixed.