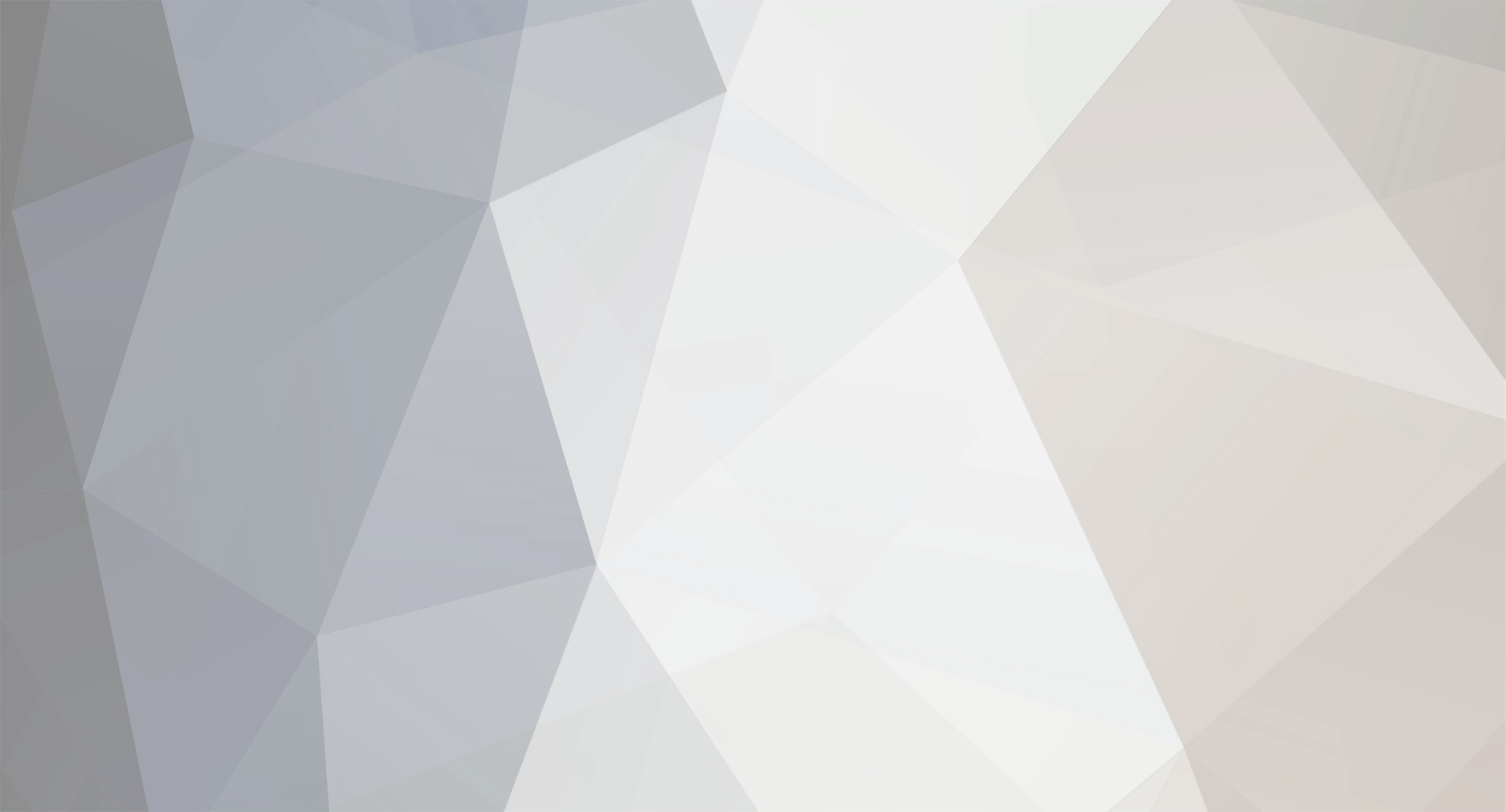
robert135
-
Posts
4 -
Joined
-
Last visited
Posts posted by robert135
-
-
i changed some stuff and now its a pig. if anyone has experienced the same thing pls help
-
public class StickOfDynamiteEntity extends ProjectileItemEntity{ public StickOfDynamiteEntity(EntityType<? extends ProjectileItemEntity> stickOfDynamiteEntity, World worldIn) { super(stickOfDynamiteEntity, worldIn); } public StickOfDynamiteEntity(EntityType<? extends ProjectileItemEntity> type, double x, double y, double z, World worldIn) { super(type, x, y, z, worldIn); // TODO Auto-generated constructor stub } public StickOfDynamiteEntity(EntityType<? extends ProjectileItemEntity> type, LivingEntity throwerIn, World worldIn) { super(type, throwerIn, worldIn); } public StickOfDynamiteEntity(LivingEntity throwerIn, World worldIn) { super(ModEntityTypes.STICK_OF_DYNAMITE.get(), throwerIn, worldIn); } @Override protected Item getDefaultItem() { // TODO Auto-generated method stub return ItemInit.STICK_OF_DYNAMITE; } @OnlyIn(Dist.CLIENT) public ItemStack getItem() { ItemStack itemstack = this.func_213882_k(); return itemstack.isEmpty() ? new ItemStack(this.getDefaultItem()) : itemstack; } @Override protected void onImpact(RayTraceResult result) { // TODO Auto-generated method stub double x = result.getHitVec().getX(); double y = result.getHitVec().getY(); double z = result.getHitVec().getZ(); this.getEntityWorld().createExplosion(this, DamageSource.GENERIC, x, y, z, 1.5F, false, Explosion.Mode.BREAK); this.remove(); } }
Im making a throwable item and it is working, all except for the fact that it is not appearing visually when thrown. I have already looked through how snowballls work and i have based my code off of that. I have determined that when i change ModEntityTypes.STICK_OF_DYNAMITE.get() to EntityType.SNOWBALL it goes from being invisible to being a snowball (the snow ball still explodes on impact like i want it to, but it looks like a snowball). can someone please help me to determine how to get it to appear as my custom texture? heres the item class:
public class StickOfDynamiteItem extends Item { public StickOfDynamiteItem(Properties properties) { super(properties); } public ActionResult<ItemStack> onItemRightClick(World worldIn, PlayerEntity playerIn, Hand handIn) { ItemStack itemstack = playerIn.getHeldItem(handIn); worldIn.playSound((PlayerEntity)null, playerIn.getPosX(), playerIn.getPosY(), playerIn.getPosZ(), SoundEvents.ENTITY_SNOWBALL_THROW, SoundCategory.NEUTRAL, 0.5F, 0.4F / (random.nextFloat() * 0.4F + 0.8F)); if (!worldIn.isRemote) { StickOfDynamiteEntity dynamiteEntity = new StickOfDynamiteEntity(playerIn, worldIn); dynamiteEntity.setItem(itemstack); dynamiteEntity.setInvisible(false); dynamiteEntity.shoot(playerIn, playerIn.rotationPitch, playerIn.rotationYaw, 0.0F, 1.5F, 1.0F); worldIn.addEntity(dynamiteEntity); } if (!playerIn.abilities.isCreativeMode) { itemstack.shrink(1); } return ActionResult.resultSuccess(itemstack); } }
and heres the ModEntityTypes class where i made the registry object for the entity:
public class ModEntityTypes { public static final DeferredRegister<EntityType<?>> ENTITY_TYPES = new DeferredRegister<>(ForgeRegistries.ENTITIES, Battle.MOD_ID); public static final RegistryObject<EntityType<StickOfDynamiteEntity>> STICK_OF_DYNAMITE = ENTITY_TYPES.register("stick_of_dynamite", () -> EntityType.Builder.<StickOfDynamiteEntity>create(StickOfDynamiteEntity::new, EntityClassification.MISC).size(.25F, .25F).build(new ResourceLocation(Battle.MOD_ID, "stick_of_dynamite").toString())); }
-
im working on a similar thing. if you do
super(ModEntityType.SHURIKEN_ENTITY.get(), throwerIn, worldIn);
instead of
super(ModEntityType.SHURIKEN_ENTITY, throwerIn, worldIn);
it should work
[1.15.2] [SOLVED] thrown object is not appearing
in Modder Support
Posted
ok i got it working now. thanks for the help!