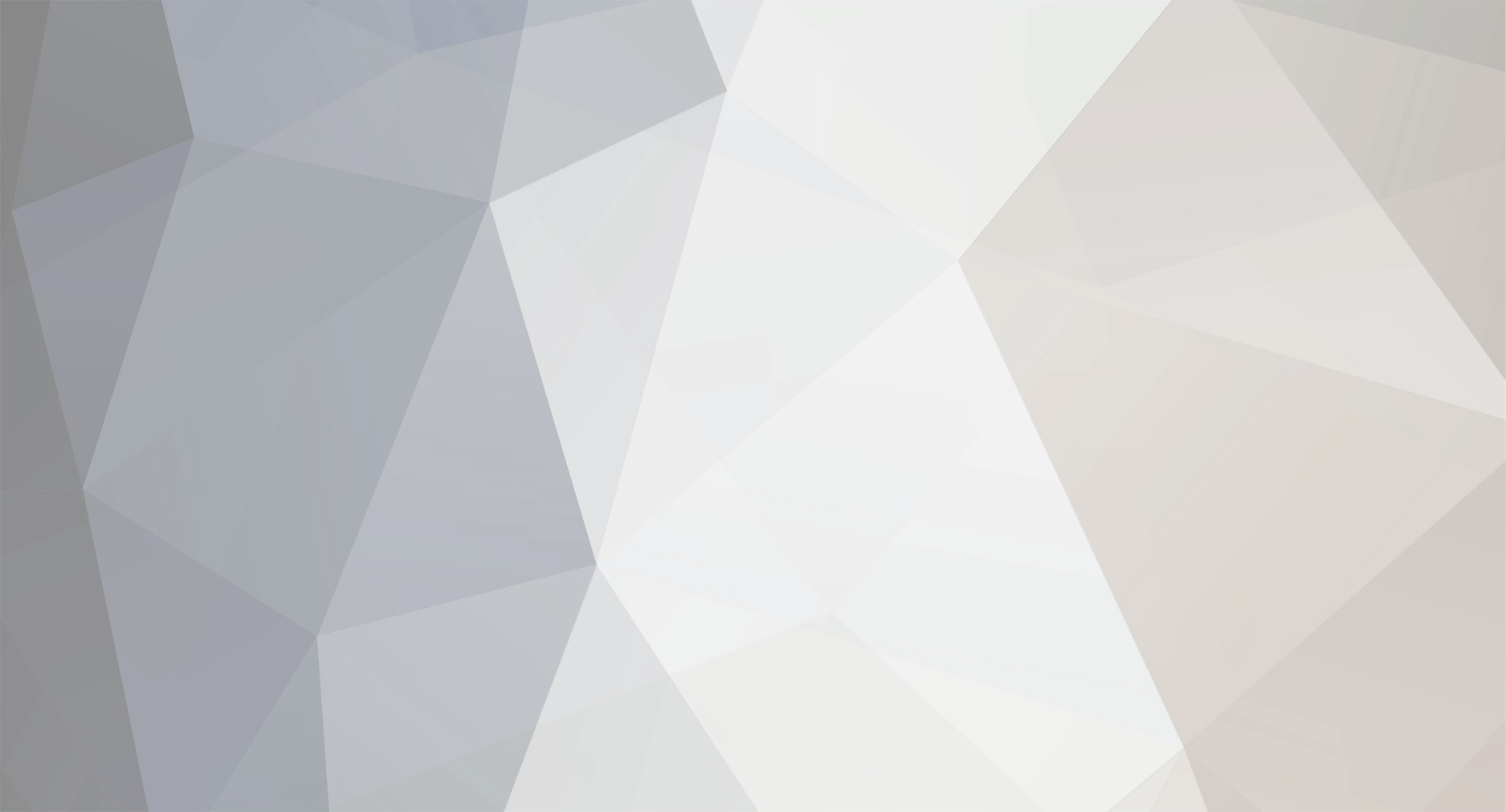
FoxWare
Members-
Posts
5 -
Joined
-
Last visited
Everything posted by FoxWare
-
Minecraft gives error when using custom packets
FoxWare replied to FoxWare's topic in Modder Support
Ok, i do something but are some issues... Here Packet class: public class EmitParticlesPacket { private int entityID; private IParticleData particle; private int lifeTime; public <T extends IParticleData> EmitParticlesPacket(Entity entityIn, T particleIn, int lifeTimeIn) { this.entityID = entityIn.getEntityId(); this.particle = particleIn; this.lifeTime = lifeTimeIn; } public EmitParticlesPacket(PacketBuffer bufIn) throws IOException { ParticleType<?> particleType = Registry.PARTICLE_TYPE.getByValue(bufIn.readInt()); if(particleType == null) { particleType = ParticleTypes.BARRIER; } this.entityID = bufIn.readInt(); this.lifeTime = bufIn.readInt(); this.particle = readParticle(bufIn, particleType); } public void encoder(EmitParticlesPacket msg, PacketBuffer bufIn) throws IOException { bufIn.writeInt(Registry.PARTICLE_TYPE.getId(this.particle.getType())); bufIn.writeInt(this.entityID); bufIn.writeInt(this.lifeTime); this.particle.write(bufIn); } private <T extends IParticleData> T readParticle(PacketBuffer bufIn, ParticleType<T> particleType) { return particleType.getDeserializer().read(particleType, bufIn); } public int getEntityID() { return this.entityID; } public IParticleData getParticle() { return this.particle; } public int getLifeTime() { return this.lifeTime; } public static void handle(EmitParticlesPacket msg, Supplier<NetworkEvent.Context> ctx) { ctx.get().enqueueWork(() -> { if(ctx.get().getDirection().getReceptionSide().equals(LogicalSide.CLIENT)) { Minecraft client = Minecraft.getInstance(); client.particles.emitParticleAtEntity(client.world.getEntityByID(msg.getEntityID()), msg.getParticle(), msg.getLifeTime()); } }); ctx.get().setPacketHandled(true); } } Here the PacketHandler... here eclipse gives some errors at ISTANCE.registerMessage(): 2 arg: The type EmitParticlesPacket does not define encoder(MSG, PacketBuffer) that is applicable here 3 arg: The constructed object of type EmitParticlesPacket is incompatible with the descriptor's return type: MSG 4 arg: The type EmitParticlesPacket does not define handle(MSG, Supplier<NetworkEvent.Context>) that is applicable here Here the class of Packet Handler: public class ModPacketHandler { private static final String PROTOCOL_VER = "1"; public static final SimpleChannel INSTANCE = NetworkRegistry.newSimpleChannel( new ResourceLocation(FurtherPotionsMain.MODID, "main"), () -> PROTOCOL_VER, PROTOCOL_VER::equals, PROTOCOL_VER::equals ); public static void register() { int ID = 0; INSTANCE.registerMessage(ID++, EmitParticlesPacket.class, EmitParticlesPacket::encoder, EmitParticlesPacket::new, EmitParticlesPacket::handle); } } -
Hi, I maked a custom packet to with the scope to use Minecraft.partciles.emitParticlesAtEntity(), but minecraft give me an error: Internal Exception: io.netty.handler.codec.EncoderException: java.lang.RuntimeException: ConnectionProtocol unknown: me.foxware.potions.network.packets.SEmitParticlesPacket@389b00f Here the packet code: public class SEmitParticlesPacket implements IPacket<IClientPlayNetHandler> { private Minecraft client = Minecraft.getInstance(); private int entityID; private IParticleData particle; private int lifeTime; public <T extends IParticleData> SEmitParticlesPacket(Entity entityIn, T particleIn, int lifeTimeIn) { this.entityID = entityIn.getEntityId(); this.particle = particleIn; this.lifeTime = lifeTimeIn; } @Override public void readPacketData(PacketBuffer bufIn) throws IOException { ParticleType<?> particleType = Registry.PARTICLE_TYPE.getByValue(bufIn.readInt()); if(particleType == null) { particleType = ParticleTypes.BARRIER; } this.entityID = bufIn.readInt(); this.lifeTime = bufIn.readInt(); this.particle = readParticle(bufIn, particleType); } @Override public void writePacketData(PacketBuffer bufIn) throws IOException { bufIn.writeInt(Registry.PARTICLE_TYPE.getId(this.particle.getType())); bufIn.writeInt(this.entityID); bufIn.writeInt(this.lifeTime); this.particle.write(bufIn); } private <T extends IParticleData> T readParticle(PacketBuffer bufIn, ParticleType<T> particleType) { return particleType.getDeserializer().read(particleType, bufIn); } public int getEntityID() { return this.entityID; } public IParticleData getParticle() { return this.particle; } public int getLifeTime() { return this.lifeTime; } @Override public void processPacket(IClientPlayNetHandler handlerIn) { this.client.particles.emitParticleAtEntity(this.client.world.getEntityByID(getEntityID()), getParticle(), getLifeTime()); } } Here where is used: @SubscribeEvent public static void onEntityDeath(LivingDeathEvent eventIn) throws InterruptedException { LivingEntity entity = eventIn.getEntityLiving(); if(entity.getActivePotionEffect(ModEffects.IMMORTALITY.get()) != null) { entity.setHealth(entity.getMaxHealth() * 25 / 100); entity.removePotionEffect(ModEffects.IMMORTALITY.get()); entity.world.playSound(null, entity.getPosX(), entity.getPosY(), entity.getPosZ(), SoundEvents.ITEM_TOTEM_USE, entity.getSoundCategory(), 1.0F, 1.0F); for(PlayerEntity p : entity.world.getPlayers()) { ((ServerPlayerEntity) p).connection.sendPacket(new SEmitParticlesPacket(entity, ParticleTypes.TOTEM_OF_UNDYING, 30)); } eventIn.setCanceled(true); } } Thanks for everyone helps me!
-
How I can set the tooltips info's (lore) after enchantment grows?
-
I "adjusted" my code but still don't work package me.foxware.lm.items; import java.awt.Color; import java.util.List; import net.minecraft.client.util.ITooltipFlag; import net.minecraft.entity.player.PlayerEntity; import net.minecraft.item.Item; import net.minecraft.item.ItemStack; import net.minecraft.util.ActionResult; import net.minecraft.util.Hand; import net.minecraft.util.math.vector.Vector3d; import net.minecraft.util.text.ITextComponent; import net.minecraft.util.text.TranslationTextComponent; import net.minecraft.world.World; public class RemoteItem extends Item { public RemoteItem(Properties properties) { super(properties.maxDamage(100)); } @Override public ActionResult<ItemStack> onItemRightClick(World world, PlayerEntity player, Hand hand) { ItemStack item = player.getHeldItem(hand); if(!world.isRemote()) { Vector3d pos = player.getPositionVec(); int X = Math.round((float) pos.x); int Y = Math.round((float) pos.y); int Z = Math.round((float) pos.z); if(!player.abilities.isCreativeMode) item.damageItem(1, player, (damagePlayer) -> {damagePlayer.sendBreakAnimation(hand);}); player.getCooldownTracker().setCooldown(this, 20); return ActionResult.resultSuccess(item); } return ActionResult.resultFail(item); } @Override public boolean showDurabilityBar(ItemStack item) { if(item.getDamage() != 0) return true; return false; } @Override public int getRGBDurabilityForDisplay(ItemStack item) { return Color.CYAN.getRGB(); } @Override public void addInformation(ItemStack item, World world, List<ITextComponent> tooltip, ITooltipFlag flag) { if(item.getDamage() != 0) tooltip.add(new TranslationTextComponent("info.lm.battery", Integer.toString(item.getMaxDamage() - item.getDamage()))); super.addInformation(item, world, tooltip, flag); } @Override public int getItemStackLimit(ItemStack item) { return 1; } }
-
I'm trying to make a Item with battery, so I use stack.damageItem() to damage the itemstack, but don't works. Here's the register: public static final RegistryObject<Item> REMOTE = ITEMS.register("remote", () -> new RemoteItem(new Item.Properties().group(ModItemGroup.LM_TAB))); Here's the class of the item: package me.foxware.lm.items; import java.awt.Color; import java.util.List; import net.minecraft.client.util.ITooltipFlag; import net.minecraft.entity.player.PlayerEntity; import net.minecraft.item.Item; import net.minecraft.item.ItemStack; import net.minecraft.util.ActionResult; import net.minecraft.util.Hand; import net.minecraft.util.math.vector.Vector3d; import net.minecraft.util.text.ITextComponent; import net.minecraft.util.text.TranslationTextComponent; import net.minecraft.world.World; public class RemoteItem extends Item { public RemoteItem(Properties properties) { super(properties.maxDamage(100)); } @Override public ActionResult<ItemStack> onItemRightClick(World world, PlayerEntity player, Hand hand) { ItemStack item = player.getHeldItem(hand); if(!world.isRemote()) { if(item.getDamage() < item.getMaxDamage()) { Vector3d pos = player.getPositionVec(); int X = Math.round((float) pos.x); int Y = Math.round((float) pos.y); int Z = Math.round((float) pos.z); item.damageItem(1, player, null); player.getCooldownTracker().setCooldown(this, 20); return ActionResult.resultSuccess(item); } } return ActionResult.resultFail(item); } @Override public boolean showDurabilityBar(ItemStack item) { if(item.getDamage() != 0) return true; return false; } @Override public int getRGBDurabilityForDisplay(ItemStack item) { return Color.CYAN.getRGB(); } @Override public void addInformation(ItemStack item, World world, List<ITextComponent> tooltip, ITooltipFlag flag) { tooltip.add(new TranslationTextComponent("item.battery", Integer.toString(item.getMaxDamage() - item.getDamage()))); super.addInformation(item, world, tooltip, flag); } @Override public int getItemStackLimit(ItemStack item) { return 1; } }