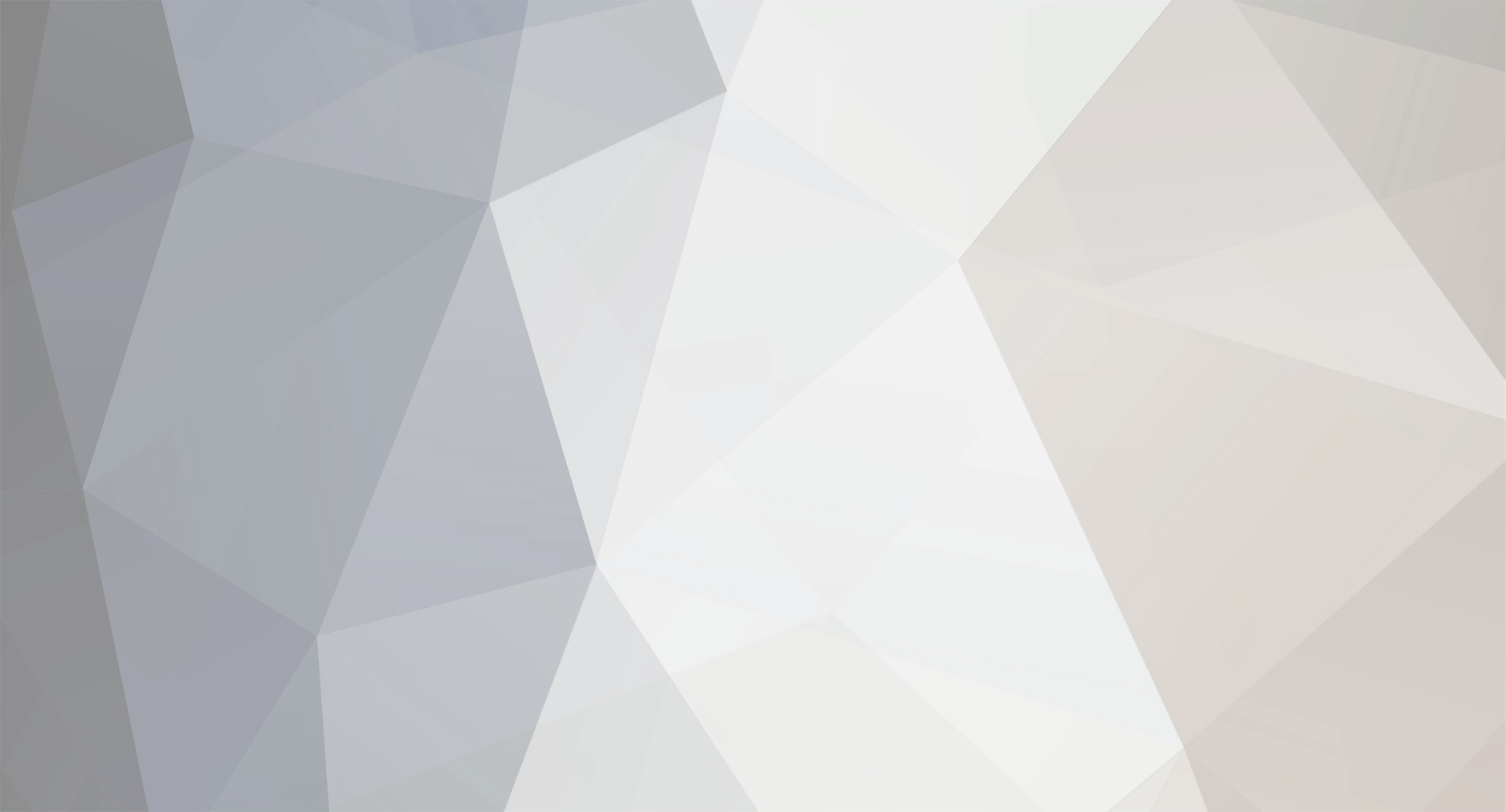
hypov
Members-
Posts
14 -
Joined
-
Last visited
Recent Profile Visitors
The recent visitors block is disabled and is not being shown to other users.
hypov's Achievements

Tree Puncher (2/8)
0
Reputation
-
Entity Code: package io.github.withonetee.entities; import io.github.withonetee.init.ModEntity; import net.minecraft.block.BlockState; import net.minecraft.enchantment.Enchantments; import net.minecraft.entity.*; import net.minecraft.entity.ai.attributes.AttributeModifierMap; import net.minecraft.entity.ai.attributes.Attributes; import net.minecraft.entity.ai.goal.*; import net.minecraft.inventory.EquipmentSlotType; import net.minecraft.item.IDyeableArmorItem; import net.minecraft.item.ItemStack; import net.minecraft.item.Items; import net.minecraft.util.DamageSource; import net.minecraft.util.SoundEvent; import net.minecraft.util.SoundEvents; import net.minecraft.util.math.BlockPos; import net.minecraft.util.text.ITextComponent; import net.minecraft.world.BossInfo; import net.minecraft.world.World; import net.minecraft.world.server.ServerBossInfo; public class FightingEntity extends MonsterEntity { private final ServerBossInfo bossInfo = (ServerBossInfo) (new ServerBossInfo(this.getDisplayName(), BossInfo.Color.RED, BossInfo.Overlay.PROGRESS)).setDarkenSky(true); public final ItemStack leather_helmet = new ItemStack(Items.LEATHER_HELMET); public final ItemStack cookie = new ItemStack(Items.COOKIE); public FightingEntity (EntityType<? extends MonsterEntity> type, World worldIn) { super(type, worldIn); } @Override public void onAddedToWorld() { super.onAddedToWorld(); this.setSprinting(true); ((IDyeableArmorItem) leather_helmet.getItem()).setColor(leather_helmet, 0xBFA290); cookie.addEnchantment(Enchantments.KNOCKBACK, 2); this.setItemStackToSlot(EquipmentSlotType.MAINHAND, cookie); this.setItemStackToSlot(EquipmentSlotType.HEAD, leather_helmet); } public static AttributeModifierMap.MutableAttribute setCustomAttributes() { return MobEntity.func_233666_p_() .createMutableAttribute(Attributes.MAX_HEALTH, 300.0F) .createMutableAttribute(Attributes.MOVEMENT_SPEED, 0.4F) .createMutableAttribute(Attributes.ATTACK_KNOCKBACK, 3.0F) .createMutableAttribute(Attributes.ATTACK_SPEED, 100.0F) .createMutableAttribute(Attributes.ATTACK_DAMAGE, 5.0F) .createMutableAttribute(Attributes.ARMOR, 0.0F) .createMutableAttribute(Attributes.ARMOR_TOUGHNESS, 0.0F); } @Override public void registerGoals() { super.registerGoals(); } @Override public int getExperiencePoints(PlayerEntity player) { return 25 + this.world.rand.nextInt(50); } @Override public SoundEvent getAmbientSound() { return SoundEvents.ENTITY_CAT_AMBIENT; } @Override public SoundEvent getDeathSound() { return SoundEvents.ENTITY_CAT_DEATH; } public SoundEvent getHurtSound(DamageSource damageSourceIn) { return SoundEvents.ENTITY_CAT_HURT; } @Override public void playStepSound(BlockPos pos, BlockState blockIn) { this.playSound(SoundEvents.ENTITY_PIG_STEP, 1.0F, 1.0F); } @Override public boolean canSwim() { return true; } @Override public SoundEvent getSwimSound() { return SoundEvents.ENTITY_PLAYER_SWIM; } @Override public SoundEvent getSplashSound() { return SoundEvents.ENTITY_PLAYER_SPLASH; } @Override public SoundEvent getFallSound(int heightIn) { return SoundEvents.ENTITY_PLAYER_BIG_FALL; } @Override public boolean canBreatheUnderwater() { return true; } @Override public boolean preventDespawn() { return true; } @Override public boolean canDespawn(double distanceToClosestPlayer) { return false; } @Override public boolean isDespawnPeaceful() { return false; } @Override public boolean onLivingFall(float distance, float damageMultiplier) { return super.onLivingFall(distance, 0); } @Override public boolean isNonBoss() { return false; } @Override public void updateAITasks() { super.updateAITasks(); bossInfo.setPercent(getHealth() / getMaxHealth()); } @Override public void addTrackingPlayer(ServerPlayerEntity player) { super.addTrackingPlayer(player); bossInfo.addPlayer(player); } @Override public void removeTrackingPlayer(ServerPlayerEntity player) { super.removeTrackingPlayer(player); bossInfo.removePlayer(player); } } Renderer: package io.github.withonetee.client.render; import io.github.withonetee.Main; import io.github.withonetee.client.model.EntityPlayerModel; import io.github.withonetee.entities.FightingEntity; import net.minecraft.client.renderer.entity.EntityRendererManager; import net.minecraft.client.renderer.entity.LivingRenderer; import net.minecraft.client.renderer.entity.layers.BipedArmorLayer; import net.minecraft.client.renderer.entity.layers.HeldItemLayer; import net.minecraft.util.ResourceLocation; public class EntityFightingRenderer extends LivingRenderer<FightingEntity, EntityPlayerModel<FightingEntity>> { public EntityFightingRenderer(EntityRendererManager manager) { super(manager, new EntityPlayerModel(0.0F, false), 0.5F); this.addLayer(new BipedArmorLayer<>(this, new EntityPlayerModel<>(0.5F, false), new EntityPlayerModel<>(1.0F, false))); this.addLayer(new HeldItemLayer<>(this)); } @Override public ResourceLocation getEntityTexture(FightingEntity entity) { return new ResourceLocation(Main.MOD_ID, "textures/entity/entity.png"); } }
-
I need help rendering my armor. It's rendering weirdly. I apply my armor via setItemStackToSlot: public final ItemStack leather_helmet = new ItemStack(Items.LEATHER_HELMET); public NewEntity(EntityType<? extends MonsterEntity> type, World worldIn) { super(type, worldIn); } @Override public void onAddedToWorld() { super.onAddedToWorld(); this.setSprinting(true); ((IDyeableArmorItem) leather_helmet.getItem()).setColor(leather_helmet, 0xBFA290); this.setItemStackToSlot(EquipmentSlotType.MAINHAND, new ItemStack(Items.; thisHEAD, leather_helmet; Please help, thank you so much.
-
Uhm yes, I have problems though 1. How do I check if there's ground below an entity? 2. I'm registering the event handler in another class, so how would I set the item of the entity in the other class?
-
Okay Basically if an entity is falling, the entity would place a water bucket below them to counteract the fall damage
-
oh right How would I fix this? Also I'm trying to add mlg features to the entity.. how would I do that? Thank you!
-
I'm confused What does that mean?
-
Why can't I re-use ItemStack instances?
-
The entity class? DreamEntity.java
-
My mod keeps crashing the client. Can anyone help? crash-2020-11-10_22.15.47-server.txt
-
Solved thanks
-
@Override public void setItemStackToSlot(EquipmentSlotType slotIn, ItemStack stack) { this.setItemStackToSlot(EquipmentSlotType.MAINHAND, netherite_axe); }
-
Doesn't work for some reason? I put it in my entity java class, and it's not rendering help please
-
Oh, cool thanks. I'll try it out!
-
I need help making a MonsterEntity hold an item (netherite_axe) Thank you!