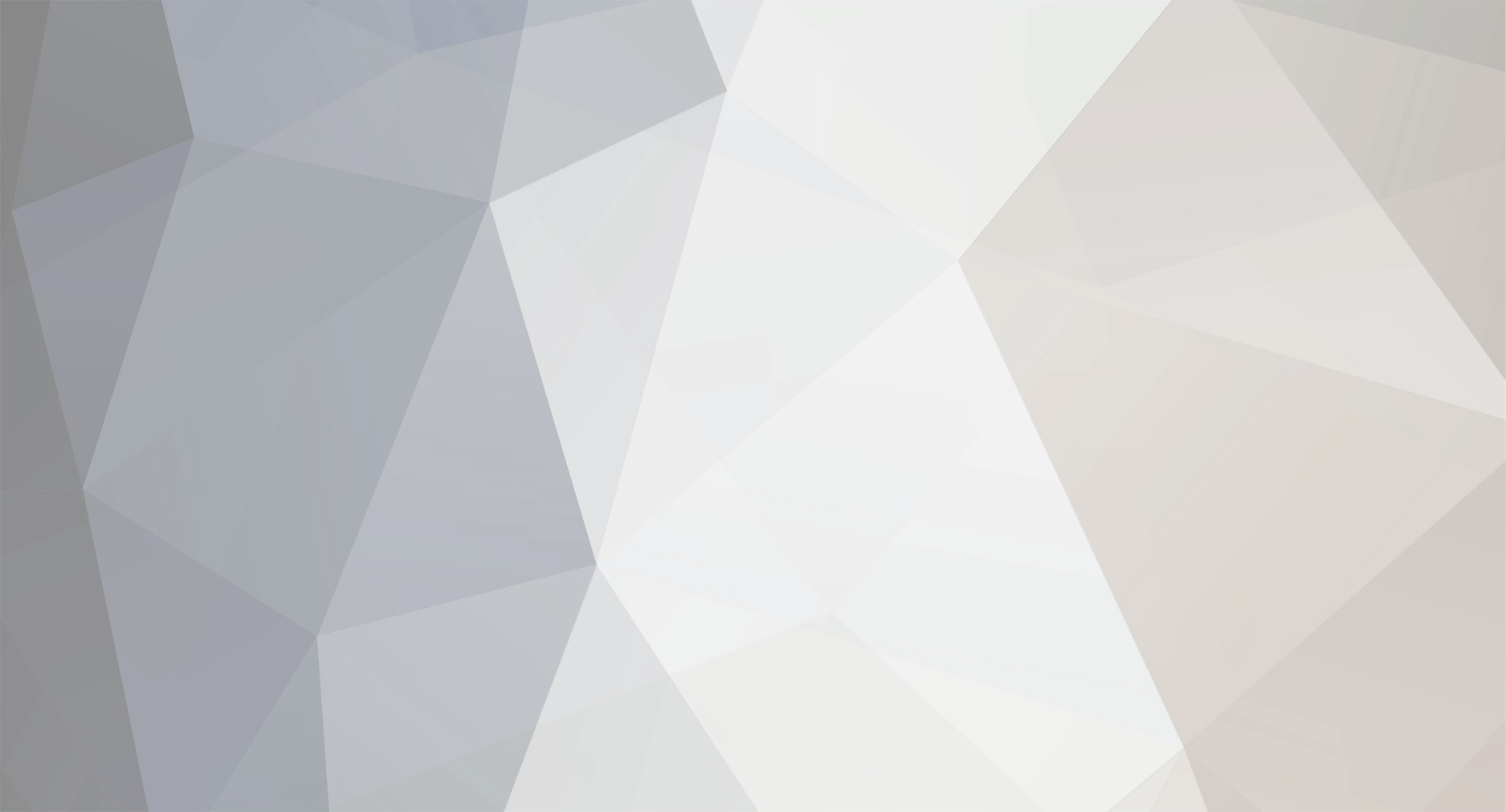
AzizD
Members-
Posts
20 -
Joined
-
Last visited
Everything posted by AzizD
-
[1.16.4] Checking an item with a certain enchantment.
AzizD replied to AzizD's topic in Modder Support
Wow that actually worked. Thank you very much! -
I made an event that ignites tnt if held item has fire aspect. I can ignite the tnt but i how can i detect the fire aspect enchantment? I tried using EnchantmentHelper but didn't find anything useful. Here is my code. @SubscribeEvent public static void fireAspectTnt(final PlayerInteractEvent event){ PlayerEntity player = event.getPlayer(); World world = event.getWorld(); BlockPos blockPos = event.getPos(); BlockState block = world.getBlockState(blockPos); Hand hand = event.getHand(); Item item = player.getHeldItem(hand).getItem(); if(!world.isRemote) { if (block.getBlock() instanceof TNTBlock) { if (item instanceof SwordItem) { //Check the sword if it has Fire Aspect. if(true) { block.getBlock().catchFire(block, world, blockPos, null, null); world.setBlockState(blockPos, Blocks.AIR.getDefaultState(), 11); } } } } }
-
You can change config options without running the game. Config folder is in the same directory as mods folder.
-
Maybe copper ore disabled in the config. Try to enable it.
-
I got it.
-
It looks way better. Thanks for the advice. @Override public boolean canApplyAtEnchantingTable(ItemStack stack, Enchantment enchantment) { Set e = ImmutableSet.of( Enchantments.SHARPNESS, Enchantments.LOOTING, Enchantments.FIRE_ASPECT, Enchantments.UNBREAKING, Enchantments.KNOCKBACK ); return e.contains(enchantment); }
-
@Override public boolean canApplyAtEnchantingTable(ItemStack stack, Enchantment enchantment) { Enchantment[] enchantments = { Enchantments.SHARPNESS, Enchantments.LOOTING, Enchantments.FIRE_ASPECT, Enchantments.UNBREAKING, Enchantments.KNOCKBACK, Enchantments.MENDING, }; for(int i=0;i<enchantments.length;i++){ if(enchantments[i] == enchantment){ return true; } } return false; } I wrote this and it worked. Thx.
-
@Override public boolean canApplyAtEnchantingTable(ItemStack stack, Enchantment enchantment) { if(enchantment == Enchantments.SHARPNESS) { enchantment.canApply(stack); } return true; } I changed with this but same error.
-
@Override public boolean canApplyAtEnchantingTable(ItemStack stack, Enchantment enchantment) { enchantment = Enchantments.SHARPNESS; return enchantment.canApply(stack.getItem().getDefaultInstance()); } I tried to override this method. Whenever i put the item in the enchantment table game crashes with java.lang.StackOverflowError.
-
I created a custom item that extends TieredItem. I want it to be enchantable through the enchantment table with Sharpnes, Looting etc. I can enchant but only the unbreaking enchantment shows up.
-
Finally i fixed the all problems. I added this condition and thats all. world.chunkExists(specialItemEntity.chunkCoordX,specialItemEntity.chunkCoordZ) Final code is : EventHandler class package com.azizd.thunderbird.events; import com.azizd.thunderbird.Thunderbird; import com.azizd.thunderbird.entities.SpecialItemEntity; import com.azizd.thunderbird.init.itemInit; import net.minecraft.entity.Entity; import net.minecraft.entity.item.ItemEntity; import net.minecraft.item.ItemStack; import net.minecraft.world.World; import net.minecraftforge.event.entity.EntityJoinWorldEvent; import net.minecraftforge.eventbus.api.SubscribeEvent; import net.minecraftforge.fml.common.Mod; @Mod.EventBusSubscriber(modid = Thunderbird.MOD_ID, bus = Mod.EventBusSubscriber.Bus.FORGE) public class EventHandler { public static void register(){} @SubscribeEvent public static void crimsonIngotCraft(EntityJoinWorldEvent event){ Entity entity = event.getEntity(); World world = entity.world; double posX = entity.getPosX(); double posY = entity.getPosY(); double posZ = entity.getPosZ(); SpecialItemEntity specialItemEntity = new SpecialItemEntity(world, posX, posY, posZ); if(entity instanceof ItemEntity && !(entity instanceof SpecialItemEntity)){ ItemEntity itemEntity = (ItemEntity) event.getEntity(); ItemStack item = itemEntity.getItem(); if(item.getItem() == itemInit.RED_PEARL.get()){ if(world.chunkExists(specialItemEntity.chunkCoordX,specialItemEntity.chunkCoordZ)) { event.setCanceled(true); specialItemEntity.setItem(itemEntity.getItem().getStack()); specialItemEntity.setPickupDelay(30); specialItemEntity.setMotion(itemEntity.getMotion()); world.addEntity(specialItemEntity); } } } } } SpecialItemEntity class package com.azizd.thunderbird.entities; import com.azizd.thunderbird.init.itemInit; import net.minecraft.entity.item.ItemEntity; import net.minecraft.item.ItemStack; import net.minecraft.world.World; public class SpecialItemEntity extends ItemEntity { private ItemEntity entity = new ItemEntity(this.world,this.getPosX(),this.getPosY(),this.getPosZ()); public SpecialItemEntity(World worldIn, double x, double y, double z) { super(worldIn, x, y, z); } @Override public void tick() { if(this.inWater && !this.world.isRemote){ this.setDead(); int count = this.getItem().getCount(); entity.setPosition(this.getPosX(),this.getPosY(),this.getPosZ()); entity.setItem(new ItemStack(itemInit.CRIMSON_INGOT.get())); entity.getItem().setCount(count); this.world.addEntity(entity); } else{ super.tick(); } } }
-
This is not the issue.
-
But i still have a bug. If specialItemEntity is in the world. I can't join that world.
-
Finally i fixed all the problems. Now it works exactly what i want. This is my EventHandler Method @SubscribeEvent public static void crimsonIngotCraft(EntityJoinWorldEvent event){ Entity entity = event.getEntity(); World world = entity.world; double posX = entity.getPosX(); double posY = entity.getPosY(); double posZ = entity.getPosZ(); if(entity instanceof ItemEntity && !(entity instanceof SpecialItemEntity)){ ItemEntity itemEntity = (ItemEntity) event.getEntity(); if(itemEntity.getItem().getItem() == itemInit.RED_PEARL.get()){ event.setCanceled(true); SpecialItemEntity specialItemEntity = new SpecialItemEntity(world,posX,posY,posZ); specialItemEntity.setItem(itemEntity.getItem().getStack()); specialItemEntity.setPickupDelay(50); specialItemEntity.setMotion(itemEntity.getMotion()); world.addEntity(specialItemEntity); } } } And this is my SpecialItemEntity class package com.azizd.thunderbird.entities; import com.azizd.thunderbird.init.itemInit; import net.minecraft.entity.item.ItemEntity; import net.minecraft.item.ItemStack; import net.minecraft.world.World; import net.minecraftforge.common.extensions.IForgeEntity; public class SpecialItemEntity extends ItemEntity implements IForgeEntity { public SpecialItemEntity(World worldIn, double x, double y, double z) { super(worldIn, x, y, z); } ItemEntity entity = new ItemEntity(getEntityWorld(),this.getPosX(),this.getPosY(),this.getPosZ()); @Override public void tick() { if(this.inWater){ this.setDead(); int count = this.getItem().getCount(); entity.setPosition(this.getPosX(),this.getPosY(),this.getPosZ()); entity.setItem(new ItemStack(itemInit.CRIMSON_INGOT.get())); entity.getItem().setCount(count); this.world.addEntity(entity); } else{ super.tick(); } } } Thanks for the helps.
-
I solved the pickup bug. The only problem is SpecialItemEntity motion.
-
I am settting the pickup delay to default every tick. This is why i cant pick up the item. The motion part is incomplete so item drop part is bad.
-
It is kinda working right now. This is EventHandler part public static void crimsonIngotCraft(EntityJoinWorldEvent event){ Entity entity = event.getEntity(); World world = entity.world; double posX = entity.getPosX(); double posY = entity.getPosY(); double posZ = entity.getPosZ(); if(entity instanceof ItemEntity && !(entity instanceof SpecialItemEntity)){ ItemEntity itemEntity = (ItemEntity) event.getEntity(); if(itemEntity.getItem().getItem() == itemInit.RED_PEARL.get()){ event.setCanceled(true); SpecialItemEntity specialItemEntity = new SpecialItemEntity(world,posX,posY,posZ); specialItemEntity.setItem(itemEntity.getItem()); world.addEntity(specialItemEntity); } } } and this is the special item entity class package com.azizd.thunderbird.entities; import com.azizd.thunderbird.init.itemInit; import net.minecraft.entity.item.ItemEntity; import net.minecraft.item.ItemStack; import net.minecraft.world.World; import net.minecraftforge.common.extensions.IForgeEntity; public class SpecialItemEntity extends ItemEntity implements IForgeEntity { public SpecialItemEntity(World worldIn, double x, double y, double z) { super(worldIn, x, y, z); } @Override public void tick() { ItemEntity entity = new ItemEntity(getEntityWorld(),this.getPosX(),this.getPosY(),this.getPosZ()); if(this.inWater){ this.setDead(); entity.setItem(new ItemStack(itemInit.CRIMSON_INGOT.get())); this.world.addEntity(entity); } ItemStack stack = this.getItem(); if (stack != null && stack.getItem() != null && stack.getItem().onEntityItemUpdate(this.getItem(),entity)) return; if(entity == null) this.setDead(); else{ super.tick(); this.setDefaultPickupDelay(); this.prevPosX = this.getPosX(); this.prevPosY = this.getPosY(); this.prevPosZ = this.getPosZ(); this.setMotion(0,-0.098,0); } } } The item converting part is complete but i can't pickup the item i threw and motion part is bad. When i throw a whole stack it gives 1 single item rather than giving a stack.
-
@SubscribeEvent public static void crimsonIngotCraft(EntityJoinWorldEvent event){ Entity entity = event.getEntity(); World world = entity.world; double posX = entity.getPosX(); double posY = entity.getPosY(); double posZ = entity.getPosZ(); if(entity instanceof ItemEntity && !(entity instanceof SpecialItemEntity)){ ItemEntity itemEntity = (ItemEntity) event.getEntity(); if(itemEntity.getItem().getItem() == itemInit.RED_PEARL.get()){ SpecialItemEntity ie = new SpecialItemEntity(entity.world,entity.getPosX(),entity.getPosY(),entity.getPosZ(),((ItemEntity) entity).getItem()); event.setCanceled(true); SpecialItemEntity specialItemEntity = new SpecialItemEntity(world,posX,posY,posZ,((ItemEntity) entity).getItem().getStack()); } } } I changed code into this and SpecialItemEntity clas is: package com.azizd.thunderbird.entities; import com.azizd.thunderbird.init.blockInit; import net.minecraft.entity.item.ItemEntity; import net.minecraft.item.ItemStack; import net.minecraft.util.math.BlockPos; import net.minecraft.world.World; public class SpecialItemEntity extends ItemEntity { public SpecialItemEntity(World worldIn, double x, double y, double z, ItemStack stack) { super(worldIn, x, y, z, stack); } @Override public void tick() { BlockPos pos= new BlockPos(getPosX(),getPosY(),getPosZ()); if(inWater){ this.setDead(); this.world.setBlockState(pos,blockInit.BLOCK_OF_RED_PEARL.get().getDefaultState()); } } } The item deletes when i press the q button.
-
@SubscribeEvent public static void crimsonIngotCraft(EntityJoinWorldEvent event){ Entity entity = event.getEntity(); if(entity instanceof ItemEntity){ ItemEntity itemEntity = (ItemEntity) event.getEntity(); if(itemEntity.getItem().getItem() == itemInit.RED_PEARL.get()){ System.out.println("Working!!"); if(itemEntity.isInWater()){ System.out.println("Not working :("); itemEntity.remove(); //FIXME Continue. } } } } Everything is working except the if(itemEntity.isInWater()){ System.out.println("Not working :("); itemEntity.remove(); } this part. I wanna create a recipe that you throw an item into water and water transforms into another block.
-
package com.azizd.thunderbird.enchant; import com.google.gson.JsonObject; import net.minecraft.enchantment.Enchantment; import net.minecraft.enchantment.EnchantmentType; import net.minecraft.enchantment.Enchantments; import net.minecraft.inventory.EquipmentSlotType; import net.minecraft.inventory.Inventory; import net.minecraft.item.ItemStack; import net.minecraft.item.crafting.FurnaceRecipe; import net.minecraft.item.crafting.IRecipeType; import net.minecraft.loot.LootContext; import net.minecraft.loot.conditions.ILootCondition; import net.minecraft.util.ResourceLocation; import net.minecraftforge.common.loot.GlobalLootModifierSerializer; import net.minecraftforge.common.loot.LootModifier; import net.minecraftforge.items.ItemHandlerHelper; import javax.annotation.Nonnull; import java.util.ArrayList; import java.util.List; public class EnchantAutoSmelt extends Enchantment{ public EnchantAutoSmelt(Rarity rarityIn, EnchantmentType typeIn, EquipmentSlotType... slots) { super(rarityIn, typeIn, slots); } @Override public int getMaxLevel() { return 1; } @Override protected boolean canApplyTogether(Enchantment ench) { return ench != Enchantments.SILK_TOUCH && ench != Enchantments.FORTUNE && super.canApplyTogether(ench); } private static class EnchantAutoSmeltLoot extends LootModifier{ public EnchantAutoSmeltLoot(ILootCondition[] conditionsIn) { super(conditionsIn); } @Nonnull @Override public List<ItemStack> doApply(List<ItemStack> generatedLoot, LootContext context) { ArrayList<ItemStack> ret = new ArrayList<ItemStack>(); generatedLoot.forEach((stack) -> ret.add(smelt(stack, context))); return ret; } private static ItemStack smelt(ItemStack stack, LootContext context) { return context.getWorld().getRecipeManager().getRecipe(IRecipeType.SMELTING, new Inventory(stack), context.getWorld()) .map(FurnaceRecipe::getRecipeOutput) .filter(itemStack -> !itemStack.isEmpty()) .map(itemStack -> ItemHandlerHelper.copyStackWithSize(itemStack, stack.getCount() * itemStack.getCount())) .orElse(stack); } private static class Serializer extends GlobalLootModifierSerializer<EnchantAutoSmeltLoot> { @Override public EnchantAutoSmeltLoot read(ResourceLocation name, JsonObject json, ILootCondition[] conditionsIn) { return new EnchantAutoSmeltLoot(conditionsIn); } @Override public JsonObject write(EnchantAutoSmeltLoot instance) { return null; } } } } This is my code. Everything works but the smelting part. The drops are not smelted.