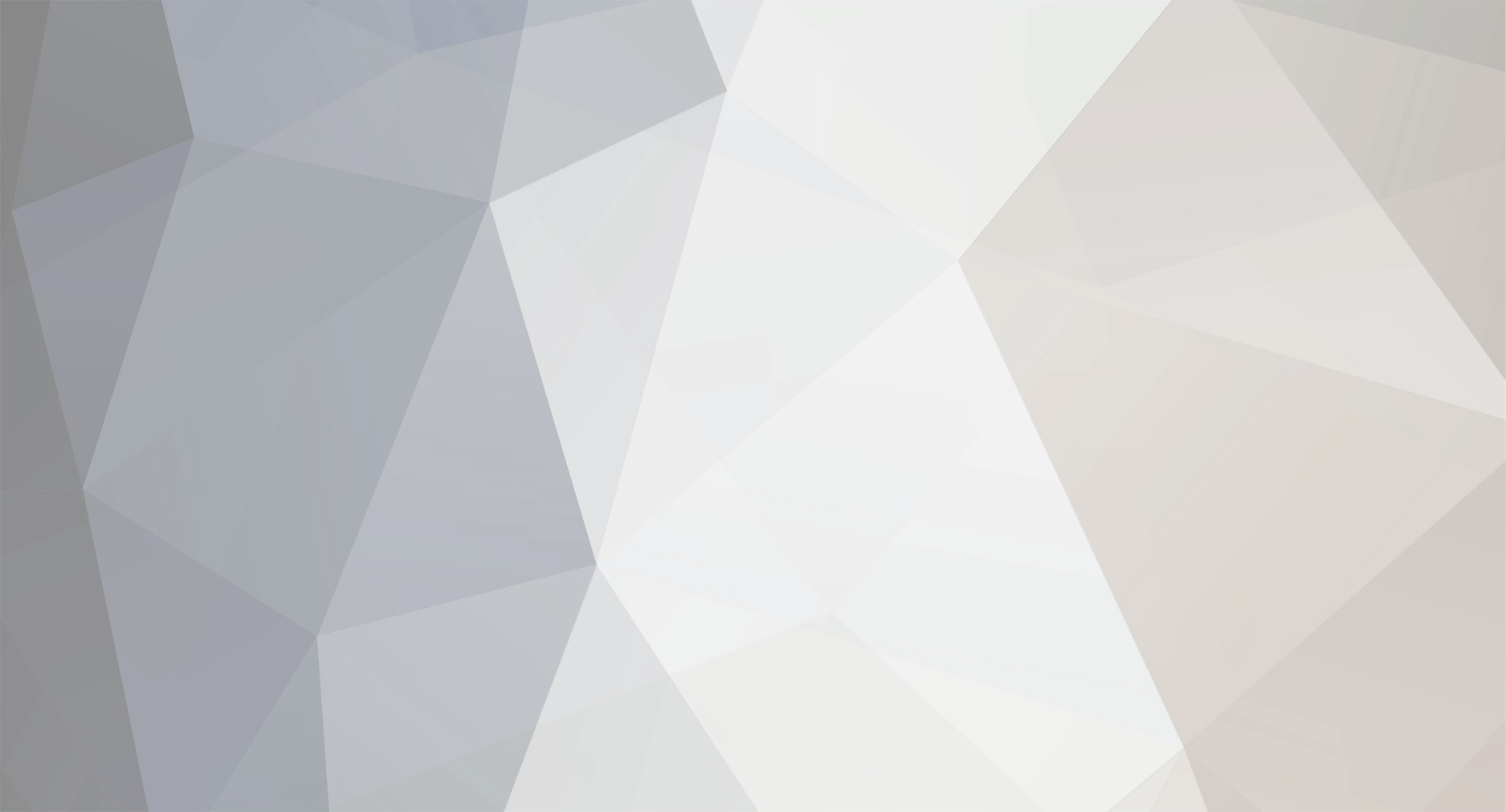
JoppeGames3
Members-
Posts
13 -
Joined
-
Last visited
Everything posted by JoppeGames3
-
Register blocks and items using Data Generators.
JoppeGames3 replied to JoppeGames3's topic in Modder Support
this is the BlockClass: package com.joppegames1.tvstudio.common.blocks; import net.minecraft.block.AbstractBlock; import net.minecraft.block.Block; import net.minecraft.block.BlockState; import net.minecraft.item.BlockItemUseContext; import net.minecraft.state.DirectionProperty; import net.minecraft.state.StateContainer; import net.minecraft.state.properties.BlockStateProperties; import net.minecraft.util.Direction; import net.minecraft.util.Mirror; import net.minecraft.util.Rotation; import net.minecraft.util.math.BlockPos; import net.minecraft.util.math.shapes.IBooleanFunction; import net.minecraft.util.math.shapes.ISelectionContext; import net.minecraft.util.math.shapes.VoxelShape; import net.minecraft.util.math.shapes.VoxelShapes; import net.minecraft.world.IBlockReader; import net.minecraft.world.IWorld; import java.util.HashMap; import java.util.Map; import java.util.stream.Stream; public class TvCameraOnAStand extends Block { public static final DirectionProperty HORIZONTAL_FACING = BlockStateProperties.HORIZONTAL_FACING; protected static final Map<Direction, VoxelShape> SHAPES = new HashMap<Direction, VoxelShape>(); // The voxelshape is this line: public static final VoxelShape SHAPE = Stream.of( Block.makeCuboidShape(6.5, 7, 6.5, 9.75, 14.5, 9.75), Block.makeCuboidShape(6.5, 20.75, 7, 10, 21.75, 11.25), Block.makeCuboidShape(6.5, 21.75, 7.75, 10, 22.25, 11.25), Block.makeCuboidShape(6.5, 22.25, 7.75, 10, 23, 14), Block.makeCuboidShape(6, 20.25, 10.75, 10.75, 21.25, 11.75), Block.makeCuboidShape(6.5, 20.25, 5.5, 10, 21.25, 7), Block.makeCuboidShape(6.5, 17.5, 7, 10, 20.75, 9.25), Block.makeCuboidShape(6.5, 17, 6.75, 10, 17.75, 9.75), Block.makeCuboidShape(6.5, 15.75, 3.25, 9.75, 17, 13.25), Block.makeCuboidShape(3.25, 15.75, 6.5, 13.25, 17, 9.75), Block.makeCuboidShape(4, 5.25, 6.75, 12.25, 7, 9.5), Block.makeCuboidShape(7, 5.25, 4, 9.25, 7, 12.25), Block.makeCuboidShape(7.5, 14.5, 7.5, 8.75, 15.75, 8.75), Block.makeCuboidShape(-2, 0, 8, 18.5, 5.25, 17.75), Block.makeCuboidShape(3, 0, 3, 13.25, 5.25, 8), Block.makeCuboidShape(5, 0, -3, 11, 5.25, 13.5), Block.makeCuboidShape(9.75, 23.5, -0.25, 10.25, 27, 0), Block.makeCuboidShape(6.25, 23.5, -0.25, 6.75, 27, 0), Block.makeCuboidShape(9.75, 24, -0.5, 10.25, 27, -0.25), Block.makeCuboidShape(6.25, 24, -0.5, 6.75, 27, -0.25), Block.makeCuboidShape(9.75, 24.75, -0.75, 10.25, 27, -0.5), Block.makeCuboidShape(6.25, 24.75, -0.75, 6.75, 27, -0.5), Block.makeCuboidShape(9.75, 25.25, -1, 10.25, 27, -0.75), Block.makeCuboidShape(6.25, 25.25, -1, 6.75, 27, -0.75), Block.makeCuboidShape(6.75, 26.25, -1, 9.75, 27, 0), Block.makeCuboidShape(9.75, 25.75, -1.25, 10.25, 27, -1), Block.makeCuboidShape(6.25, 25.75, -1.25, 6.75, 27, -1), Block.makeCuboidShape(6.75, 26.25, -1.25, 9.75, 27, -1), Block.makeCuboidShape(6.25, 26.5, -1.5, 10.25, 26.75, -1.25), Block.makeCuboidShape(9.75, 23, 0, 10.25, 27, 7.75), Block.makeCuboidShape(6.25, 23, 0, 6.75, 27, 7.75), Block.makeCuboidShape(6.75, 23, 0, 9.75, 27, 7.75), Block.makeCuboidShape(6, 23, 7.75, 10.5, 27, 9.5), Block.makeCuboidShape(6.25, 25.75, 11, 10.25, 28, 15.25), Block.makeCuboidShape(6.25, 27.25, 15.25, 6.75, 28, 16.75), Block.makeCuboidShape(9.75, 27.25, 15.25, 10.25, 28, 16.75), Block.makeCuboidShape(6.75, 27.5, 15.25, 9.75, 28, 16.75), Block.makeCuboidShape(6.75, 26, 14.5, 9.75, 27.5, 15.5), Block.makeCuboidShape(6.25, 26.75, 15.25, 6.75, 27, 16.25), Block.makeCuboidShape(9.75, 26.75, 15.25, 10.25, 27, 16.25), Block.makeCuboidShape(6.25, 27, 15.25, 6.75, 27.25, 16.5), Block.makeCuboidShape(9.75, 27, 15.25, 10.25, 27.25, 16.5), Block.makeCuboidShape(6.25, 26.5, 15.25, 6.75, 26.75, 16), Block.makeCuboidShape(9.75, 26.25, 15.25, 10.25, 26.5, 15.75), Block.makeCuboidShape(6.25, 26.25, 15.25, 6.75, 26.5, 15.75), Block.makeCuboidShape(9.75, 26, 15.25, 10.25, 26.25, 15.5), Block.makeCuboidShape(6.25, 26, 15.25, 6.75, 26.25, 15.5), Block.makeCuboidShape(6.25, 25.5, 10.75, 10.25, 28, 11), Block.makeCuboidShape(6, 23, 7.75, 10.5, 25.5, 14.5), Block.makeCuboidShape(6, 25.5, 7.75, 10.5, 27, 9.5), Block.makeCuboidShape(6, 25.5, 9.5, 10.5, 27, 10), Block.makeCuboidShape(6, 25.5, 10, 10.5, 26.5, 10.25), Block.makeCuboidShape(6, 25.5, 10.25, 10.5, 26.25, 10.5), Block.makeCuboidShape(6, 25.5, 10.5, 10.5, 26, 10.75), Block.makeCuboidShape(6, 25.5, 10.75, 10.5, 25.75, 11), Block.makeCuboidShape(6.25, 25.5, 10.5, 10.25, 27.75, 10.75), Block.makeCuboidShape(7.75, 27, 9.75, 8.75, 28, 10.75), Block.makeCuboidShape(7.75, 28, 9.75, 8.75, 28.25, 10.5), Block.makeCuboidShape(7.75, 27.75, 7.75, 8.75, 28, 8.75), Block.makeCuboidShape(5.5, 24.75, 7.25, 11, 25.25, 14.5), Block.makeCuboidShape(6.25, 24.75, 14.5, 10.75, 25.25, 15), Block.makeCuboidShape(10.75, 24.75, 14.5, 11, 25.25, 14.75), Block.makeCuboidShape(5.75, 24.75, 14.5, 6.25, 25.25, 14.75), Block.makeCuboidShape(6, 24.75, 14.75, 6.25, 25.25, 15), Block.makeCuboidShape(7.75, 27.5, 7.75, 8.75, 27.75, 9), Block.makeCuboidShape(7.75, 27.25, 7.75, 8.75, 27.5, 9.25), Block.makeCuboidShape(7.75, 27, 7.75, 8.75, 27.25, 9.5), Block.makeCuboidShape(6.25, 25.5, 10.25, 10.25, 27.5, 10.5), Block.makeCuboidShape(6.25, 25.75, 10, 10.25, 27.25, 10.25), Block.makeCuboidShape(6, 25.5, 10.5, 10.5, 26, 10.75), Block.makeCuboidShape(6, 24, 1.75, 6.25, 24.75, 2), Block.makeCuboidShape(6, 24.25, 1.5, 6.25, 24.5, 1.75), Block.makeCuboidShape(6, 24, 1.25, 6.25, 24.75, 1.5), Block.makeCuboidShape(6, 24, 1, 6.25, 24.25, 1.25), Block.makeCuboidShape(6, 24, 0.75, 6.25, 24.75, 1), Block.makeCuboidShape(6, 24.5, 1, 6.25, 24.75, 1.5), Block.makeCuboidShape(10.25, 24, 2.75, 10.5, 24.75, 3), Block.makeCuboidShape(10.25, 24.25, 2.5, 10.5, 24.5, 2.75), Block.makeCuboidShape(10.25, 24, 2.25, 10.5, 24.75, 2.5), Block.makeCuboidShape(10.25, 24, 1.75, 10.5, 24.75, 2), Block.makeCuboidShape(10.25, 24, 2, 10.5, 24.25, 2.25), Block.makeCuboidShape(10.25, 24.5, 2, 10.5, 24.75, 2.5), Block.makeCuboidShape(5.75, 27, 12.75, 6, 27.25, 13.5), Block.makeCuboidShape(5.75, 26.5, 12.75, 6, 26.75, 13.5), Block.makeCuboidShape(5.75, 26.75, 12.75, 6, 27, 13), Block.makeCuboidShape(5.75, 26.75, 13.25, 6, 27, 13.5), Block.makeCuboidShape(5.75, 26.75, 13, 6.25, 27, 13.25), Block.makeCuboidShape(10.5, 26.5, 13.25, 10.75, 27.25, 13.5), Block.makeCuboidShape(10.5, 26.5, 12.75, 10.75, 27.25, 13), Block.makeCuboidShape(10.5, 26.5, 13, 10.75, 26.75, 13.25), Block.makeCuboidShape(10.5, 27, 13, 10.75, 27.25, 13.25), Block.makeCuboidShape(10.25, 26.75, 13, 10.75, 27, 13.25) ).reduce((v1, v2) -> {return VoxelShapes.combineAndSimplify(v1, v2, IBooleanFunction.OR);}).get(); public TvCameraOnAStand(AbstractBlock.Properties properties) { super(properties); runCalculation(SHAPE); this.setDefaultState(this.stateContainer.getBaseState().with(HORIZONTAL_FACING, Direction.NORTH)); } @Override public VoxelShape getShape(BlockState state, IBlockReader worldIn, BlockPos pos, ISelectionContext context) { return SHAPES.get(state.get(HORIZONTAL_FACING)); } @SuppressWarnings("deprecation") @Override public BlockState mirror(BlockState state, Mirror mirrorIn) { return state.rotate(mirrorIn.toRotation(state.get(HORIZONTAL_FACING))); } @Override public BlockState rotate(BlockState state, IWorld world, BlockPos pos, Rotation direction) { return state.with(HORIZONTAL_FACING, direction.rotate(state.get(HORIZONTAL_FACING))); } //.getOpposite() for mirroring the block @Override public BlockState getStateForPlacement(BlockItemUseContext context) { return this.getDefaultState().with(HORIZONTAL_FACING, context.getPlacementHorizontalFacing().getOpposite()); } @Override protected void fillStateContainer(StateContainer.Builder<Block, BlockState> builder) { super.fillStateContainer(builder); builder.add(HORIZONTAL_FACING); } protected static void calculateShapes(Direction to, VoxelShape shape) { VoxelShape[] buffer = new VoxelShape[] { shape, VoxelShapes.empty() }; int times = (to.getHorizontalIndex() - Direction.NORTH.getHorizontalIndex() + 4) % 4; for (int i = 0; i < times; i++) { buffer[0].forEachBox((minX, minY, minZ, maxX, maxY, maxZ) -> buffer[1] = VoxelShapes.or(buffer[1], VoxelShapes.create(1 - maxZ, minY, minX, 1 - minZ, maxY, maxX))); buffer[0] = buffer[1]; buffer[1] = VoxelShapes.empty(); } SHAPES.put(to, buffer[0]); } protected void runCalculation(VoxelShape shape) { for (Direction direction : Direction.values()) { calculateShapes(direction, shape); } } } and here is the BlockInit: package com.joppegames1.tvstudio.core.init; import com.joppegames1.tvstudio.TvStudio; import com.joppegames1.tvstudio.common.blocks.DirectorChair; import com.joppegames1.tvstudio.common.blocks.Pole; import com.joppegames1.tvstudio.common.blocks.SetWall; import com.joppegames1.tvstudio.common.blocks.SetWallSupport; import com.joppegames1.tvstudio.common.blocks.StudioBar; import com.joppegames1.tvstudio.common.blocks.StudioLight; import com.joppegames1.tvstudio.common.blocks.TvCamera; import com.joppegames1.tvstudio.common.blocks.TvCameraOnAStand; import com.joppegames1.tvstudio.common.blocks.TvStand; import com.joppegames1.tvstudio.common.blocks.TvStandHead; import net.minecraft.block.AbstractBlock; import net.minecraft.block.Block; import net.minecraft.block.SoundType; import net.minecraft.block.WallBlock; import net.minecraft.block.material.Material; import net.minecraftforge.common.ToolType; import net.minecraftforge.fml.RegistryObject; import net.minecraftforge.registries.DeferredRegister; import net.minecraftforge.registries.ForgeRegistries; public class BlockInit { public static final DeferredRegister<Block> BLOCKS = DeferredRegister.create(ForgeRegistries.BLOCKS, TvStudio.MOD_ID); //screen blocks public static final RegistryObject<Block> BLUE_SCREEN_BLOCK = BLOCKS.register("blue_screen_block", () -> new Block(AbstractBlock.Properties.create(Material.ROCK).hardnessAndResistance(1.5F, 6.0F).harvestTool(ToolType.PICKAXE).harvestLevel(2).sound(SoundType.STONE))); public static final RegistryObject<Block> GREEN_SCREEN_BLOCK = BLOCKS.register("green_screen_block", () -> new Block(AbstractBlock.Properties.create(Material.ROCK).hardnessAndResistance(1.5F, 6.0F).harvestTool(ToolType.PICKAXE).harvestLevel(2).sound(SoundType.STONE))); public static final RegistryObject<Block> GREEN_SCREEN_WALL = BLOCKS.register("green_screen_wall", () -> new WallBlock(AbstractBlock.Properties.create(Material.WOOL).hardnessAndResistance(1.5F, 6.0F).sound(SoundType.METAL).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> BLUE_SCREEN_WALL = BLOCKS.register("blue_screen_wall", () -> new WallBlock(AbstractBlock.Properties.create(Material.WOOL).hardnessAndResistance(1.5F, 6.0F).sound(SoundType.METAL).setLightLevel((state) -> {return 2;}))); //non coloured public static final RegistryObject<Block> TV_CAMERA = BLOCKS.register("tv_camera", () -> new TvCamera(AbstractBlock.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> TV_STAND_HEAD = BLOCKS.register("tv_stand_head", () -> new TvStandHead(AbstractBlock.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> POLE = BLOCKS.register("pole", () -> new Pole(AbstractBlock.Properties.create(Material.IRON).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> CHIPBOARD_BLOCK = BLOCKS.register("chipboard_block", () -> new Block(AbstractBlock.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).harvestLevel(2).sound(SoundType.WOOD))); public static final RegistryObject<Block> SET_WALL_SUPPORT = BLOCKS.register("set_wall_support", () -> new SetWallSupport(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).harvestLevel(2).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); //Tv Camera On A Stand public static final RegistryObject<Block> WHITE_TV_CAMERA_ON_STAND = BLOCKS.register("white_tv_camera_on_stand", () -> new TvCameraOnAStand(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> ORANGE_TV_CAMERA_ON_STAND = BLOCKS.register("orange_tv_camera_on_stand", () -> new TvCameraOnAStand(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> MAGENTA_TV_CAMERA_ON_STAND = BLOCKS.register("magenta_tv_camera_on_stand", () -> new TvCameraOnAStand(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> LIGHT_BLUE_TV_CAMERA_ON_STAND = BLOCKS.register("light_blue_tv_camera_on_stand", () -> new TvCameraOnAStand(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> YELLOW_TV_CAMERA_ON_STAND = BLOCKS.register("yellow_tv_camera_on_stand", () -> new TvCameraOnAStand(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> LIME_TV_CAMERA_ON_STAND = BLOCKS.register("lime_tv_camera_on_stand", () -> new TvCameraOnAStand(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> PINK_TV_CAMERA_ON_STAND = BLOCKS.register("pink_tv_camera_on_stand", () -> new TvCameraOnAStand(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> GRAY_TV_CAMERA_ON_STAND = BLOCKS.register("gray_tv_camera_on_stand", () -> new TvCameraOnAStand(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> LIGHT_GRAY_TV_CAMERA_ON_STAND = BLOCKS.register("light_gray_tv_camera_on_stand", () -> new TvCameraOnAStand(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> CYAN_TV_CAMERA_ON_STAND = BLOCKS.register("cyan_tv_camera_on_stand", () -> new TvCameraOnAStand(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> PURPLE_TV_CAMERA_ON_STAND = BLOCKS.register("purple_tv_camera_on_stand", () -> new TvCameraOnAStand(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> BLUE_TV_CAMERA_ON_STAND = BLOCKS.register("blue_tv_camera_on_stand", () -> new TvCameraOnAStand(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> BROWN_TV_CAMERA_ON_STAND = BLOCKS.register("brown_tv_camera_on_stand", () -> new TvCameraOnAStand(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> GREEN_TV_CAMERA_ON_STAND = BLOCKS.register("green_tv_camera_on_stand", () -> new TvCameraOnAStand(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> RED_TV_CAMERA_ON_STAND = BLOCKS.register("red_tv_camera_on_stand", ()-> new TvCameraOnAStand(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> BLACK_TV_CAMERA_ON_STAND = BLOCKS.register("black_tv_camera_on_stand", () -> new TvCameraOnAStand(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 2;}))); //Tv Stand public static final RegistryObject<Block> WHITE_TV_STAND = BLOCKS.register("white_tv_stand", () -> new TvStand(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> ORANGE_TV_STAND = BLOCKS.register("orange_tv_stand", () -> new TvStand(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> MAGENTA_TV_STAND = BLOCKS.register("magenta_tv_stand", () -> new TvStand(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> LIGHT_BLUE_TV_STAND = BLOCKS.register("light_blue_tv_stand", () -> new TvStand(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> YELLOW_TV_STAND = BLOCKS.register("yellow_tv_stand", () -> new TvStand(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> LIME_TV_STAND = BLOCKS.register("lime_tv_stand", () -> new TvStand(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> PINK_TV_STAND = BLOCKS.register("pink_tv_stand", () -> new TvStand(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> GRAY_TV_STAND = BLOCKS.register("gray_tv_stand", () -> new TvStand(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> LIGHT_GRAY_TV_STAND = BLOCKS.register("light_gray_tv_stand", () -> new TvStand(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> CYAN_TV_STAND = BLOCKS.register("cyan_tv_stand", () -> new TvStand(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> PURPLE_TV_STAND = BLOCKS.register("purple_tv_stand", () -> new TvStand(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> BLUE_TV_STAND = BLOCKS.register("blue_tv_stand", () -> new TvStand(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> BROWN_TV_STAND = BLOCKS.register("brown_tv_stand", () -> new TvStand(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> GREEN_TV_STAND = BLOCKS.register("green_tv_stand", () -> new TvStand(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> RED_TV_STAND = BLOCKS.register("red_tv_stand", ()-> new TvStand(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> BLACK_TV_STAND = BLOCKS.register("black_tv_stand", () -> new TvStand(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 2;}))); //Studio Light public static final RegistryObject<Block> WHITE_STUDIO_LIGHT = BLOCKS.register("white_studio_light", () -> new StudioLight(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 15;}))); public static final RegistryObject<Block> ORANGE_STUDIO_LIGHT = BLOCKS.register("orange_studio_light", () -> new StudioLight(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 15;}))); public static final RegistryObject<Block> MAGENTA_STUDIO_LIGHT = BLOCKS.register("magenta_studio_light", () -> new StudioLight(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 15;}))); public static final RegistryObject<Block> LIGHT_BLUE_STUDIO_LIGHT = BLOCKS.register("light_blue_studio_light", () -> new StudioLight(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 15;}))); public static final RegistryObject<Block> YELLOW_STUDIO_LIGHT = BLOCKS.register("yellow_studio_light", () -> new StudioLight(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 15;}))); public static final RegistryObject<Block> LIME_STUDIO_LIGHT = BLOCKS.register("lime_studio_light", () -> new StudioLight(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 15;}))); public static final RegistryObject<Block> PINK_STUDIO_LIGHT = BLOCKS.register("pink_studio_light", () -> new StudioLight(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 15;}))); public static final RegistryObject<Block> GRAY_STUDIO_LIGHT = BLOCKS.register("gray_studio_light", () -> new StudioLight(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 15;}))); public static final RegistryObject<Block> LIGHT_GRAY_STUDIO_LIGHT = BLOCKS.register("light_gray_studio_light", () -> new StudioLight(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 15;}))); public static final RegistryObject<Block> CYAN_STUDIO_LIGHT = BLOCKS.register("cyan_studio_light", () -> new StudioLight(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 15;}))); public static final RegistryObject<Block> PURPLE_STUDIO_LIGHT = BLOCKS.register("purple_studio_light", () -> new StudioLight(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 15;}))); public static final RegistryObject<Block> BLUE_STUDIO_LIGHT = BLOCKS.register("blue_studio_light", () -> new StudioLight(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 15;}))); public static final RegistryObject<Block> BROWN_STUDIO_LIGHT = BLOCKS.register("brown_studio_light", () -> new StudioLight(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 15;}))); public static final RegistryObject<Block> GREEN_STUDIO_LIGHT = BLOCKS.register("green_studio_light", () -> new StudioLight(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 15;}))); public static final RegistryObject<Block> RED_STUDIO_LIGHT = BLOCKS.register("red_studio_light", ()-> new StudioLight(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 15;}))); public static final RegistryObject<Block> BLACK_STUDIO_LIGHT = BLOCKS.register("black_studio_light", () -> new StudioLight(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 15;}))); //Studio Bar public static final RegistryObject<Block> WHITE_STUDIO_BAR = BLOCKS.register("white_studio_bar", () -> new StudioBar(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> ORANGE_STUDIO_BAR = BLOCKS.register("orange_studio_bar", () -> new StudioBar(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> MAGENTA_STUDIO_BAR = BLOCKS.register("magenta_studio_bar", () -> new StudioBar(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> LIGHT_BLUE_STUDIO_BAR = BLOCKS.register("light_blue_studio_bar", () -> new StudioBar(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> YELLOW_STUDIO_BAR = BLOCKS.register("yellow_studio_bar", () -> new StudioBar(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> LIME_STUDIO_BAR = BLOCKS.register("lime_studio_bar", () -> new StudioBar(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> PINK_STUDIO_BAR = BLOCKS.register("pink_studio_bar", () -> new StudioBar(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> GRAY_STUDIO_BAR = BLOCKS.register("gray_studio_bar", () -> new StudioBar(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> LIGHT_GRAY_STUDIO_BAR = BLOCKS.register("light_gray_studio_bar", () -> new StudioBar(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> CYAN_STUDIO_BAR = BLOCKS.register("cyan_studio_bar", () -> new StudioBar(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> PURPLE_STUDIO_BAR = BLOCKS.register("purple_studio_bar", () -> new StudioBar(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> BLUE_STUDIO_BAR = BLOCKS.register("blue_studio_bar", () -> new StudioBar(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> BROWN_STUDIO_BAR = BLOCKS.register("brown_studio_bar", () -> new StudioBar(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> GREEN_STUDIO_BAR = BLOCKS.register("green_studio_bar", () -> new StudioBar(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> RED_STUDIO_BAR = BLOCKS.register("red_studio_bar", ()-> new StudioBar(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> BLACK_STUDIO_BAR = BLOCKS.register("black_studio_bar", () -> new StudioBar(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 2;}))); //Director Chair public static final RegistryObject<Block> WHITE_DIRECTOR_CHAIR = BLOCKS.register("white_director_chair", () -> new DirectorChair(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> ORANGE_DIRECTOR_CHAIR = BLOCKS.register("orange_director_chair", () -> new DirectorChair(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> MAGENTA_DIRECTOR_CHAIR = BLOCKS.register("magenta_director_chair", () -> new DirectorChair(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> LIGHT_BLUE_DIRECTOR_CHAIR = BLOCKS.register("light_blue_director_chair", () -> new DirectorChair(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> YELLOW_DIRECTOR_CHAIR = BLOCKS.register("yellow_director_chair", () -> new DirectorChair(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> LIME_DIRECTOR_CHAIR = BLOCKS.register("lime_director_chair", () -> new DirectorChair(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> PINK_DIRECTOR_CHAIR = BLOCKS.register("pink_director_chair", () -> new DirectorChair(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> GRAY_DIRECTOR_CHAIR = BLOCKS.register("gray_director_chair", () -> new DirectorChair(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> LIGHT_GRAY_DIRECTOR_CHAIR = BLOCKS.register("light_gray_director_chair", () -> new DirectorChair(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> CYAN_DIRECTOR_CHAIR = BLOCKS.register("cyan_director_chair", () -> new DirectorChair(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> PURPLE_DIRECTOR_CHAIR = BLOCKS.register("purple_director_chair", () -> new DirectorChair(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> BLUE_DIRECTOR_CHAIR = BLOCKS.register("blue_director_chair", () -> new DirectorChair(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> BROWN_DIRECTOR_CHAIR = BLOCKS.register("brown_director_chair", () -> new DirectorChair(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> GREEN_DIRECTOR_CHAIR = BLOCKS.register("green_director_chair", () -> new DirectorChair(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> RED_DIRECTOR_CHAIR = BLOCKS.register("red_director_chair", ()-> new DirectorChair(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> BLACK_DIRECTOR_CHAIR = BLOCKS.register("black_director_chair", () -> new DirectorChair(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 2;}))); //Set public static final RegistryObject<Block> SET_WALL = BLOCKS.register("set_wall", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_STONE = BLOCKS.register("set_wall_stone", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_GRANITE = BLOCKS.register("set_wall_granite", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_POLISHED_GRANITE = BLOCKS.register("set_wall_polished_granite", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_DIORITE = BLOCKS.register("set_wall_diorite", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_POLISHED_DIORITE = BLOCKS.register("set_wall_polished_diorite", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_ANDESITE = BLOCKS.register("set_wall_andesite", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_POLISHED_ANDESITE = BLOCKS.register("set_wall_polished_andesite", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_DIRT = BLOCKS.register("set_wall_dirt", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_COARSE_DIRT = BLOCKS.register("set_wall_coarse_dirt", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_CRIMSON_NYLIUM = BLOCKS.register("set_wall_crimson_nylium", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_WARPED_NYLIUM = BLOCKS.register("set_wall_warped_nylium", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_COBBLESTONE = BLOCKS.register("set_wall_cobblestone", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_OAK_PLANKS = BLOCKS.register("set_wall_oak_planks", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_SPRUCE_PLANKS = BLOCKS.register("set_wall_spruce_planks", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_BIRCH_PLANKS = BLOCKS.register("set_wall_birch_planks", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_JUNGLE_PLANKS = BLOCKS.register("set_wall_jungle_planks", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_ACACIA_PLANKS = BLOCKS.register("set_wall_acacia_planks", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_DARK_OAK_PLANKS = BLOCKS.register("set_wall_dark_oak_planks", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_CRIMSON_PLANKS = BLOCKS.register("set_wall_crimson_planks", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_WARPED_PLANKS = BLOCKS.register("set_wall_warped_planks", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_BEDROCK = BLOCKS.register("set_wall_bedrock", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_SAND = BLOCKS.register("set_wall_sand", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_RED_SAND = BLOCKS.register("set_wall_red_sand", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_GRAVEL = BLOCKS.register("set_wall_gravel", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_GOLD_ORE = BLOCKS.register("set_wall_gold_ore", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_IRON_ORE = BLOCKS.register("set_wall_iron_ore", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_COAL_ORE = BLOCKS.register("set_wall_coal_ore", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_NETHER_GOLD_ORE = BLOCKS.register("set_wall_nether_gold_ore", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_OAK_LOG = BLOCKS.register("set_wall_oak_log", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_SPRUCE_LOG = BLOCKS.register("set_wall_spruce_log", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_BIRCH_LOG = BLOCKS.register("set_wall_birch_log", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_JUNGLE_LOG = BLOCKS.register("set_wall_jungle_log", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_ACACIA_LOG = BLOCKS.register("set_wall_acacia_log", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_DARK_OAK_LOG = BLOCKS.register("set_wall_dark_oak_log", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_CRIMSON_STEM = BLOCKS.register("set_wall_crimson_stem", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_WARPED_STEM = BLOCKS.register("set_wall_warped_stem", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_STRIPPED_OAK_LOG = BLOCKS.register("set_wall_stripped_oak_log", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_STRIPPED_SPRUCE_LOG = BLOCKS.register("set_wall_stripped_spruce_log", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_STRIPPED_BIRCH_LOG = BLOCKS.register("set_wall_stripped_birch_log", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_STRIPPED_JUNGLE_LOG = BLOCKS.register("set_wall_stripped_jungle_log", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_STRIPPED_ACACIA_LOG = BLOCKS.register("set_wall_stripped_acacia_log", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_STRIPPED_DARK_OAK_LOG = BLOCKS.register("set_wall_stripped_dark_oak_log", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_STRIPPED_CRIMSON_STEM = BLOCKS.register("set_wall_stripped_crimson_stem", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_STRIPPED_WARPED_STEM = BLOCKS.register("set_wall_stripped_warped_stem", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_SPONGE = BLOCKS.register("set_wall_sponge", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_WET_SPONGE = BLOCKS.register("set_wall_wet_sponge", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_LAPIS_ORE = BLOCKS.register("set_wall_lapis_ore", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_LAPIS_BLOCK = BLOCKS.register("set_wall_lapis_block", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_DISPENSER = BLOCKS.register("set_wall_dispenser", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_SANDSTONE = BLOCKS.register("set_wall_sandstone", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_CHISELED_SANDSTONE = BLOCKS.register("set_wall_chiseled_sandstone", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_CUT_SANDSTONE = BLOCKS.register("set_wall_cut_sandstone", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_NOTE_BLOCK = BLOCKS.register("set_wall_note_block", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_WHITE_WOOL = BLOCKS.register("set_wall_white_wool", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_ORANGE_WOOL = BLOCKS.register("set_wall_orange_wool", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_MAGENTA_WOOL = BLOCKS.register("set_wall_magenta_wool", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_LIGHT_BLUE_WOOL = BLOCKS.register("set_wall_light_blue_wool", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_YELLOW_WOOL = BLOCKS.register("set_wall_yellow_wool", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_LIME_WOOL = BLOCKS.register("set_wall_lime_wool", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_PINK_WOOL = BLOCKS.register("set_wall_pink_wool", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_GRAY_WOOL = BLOCKS.register("set_wall_gray_wool", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_LIGHT_GRAY_WOOL = BLOCKS.register("set_wall_light_gray_wool", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_CYAN_WOOL = BLOCKS.register("set_wall_cyan_wool", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_PURPLE_WOOL = BLOCKS.register("set_wall_purple_wool", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_BLUE_WOOL = BLOCKS.register("set_wall_blue_wool", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_BROWN_WOOL = BLOCKS.register("set_wall_brown_wool", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_GREEN_WOOL = BLOCKS.register("set_wall_green_wool", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_RED_WOOL = BLOCKS.register("set_wall_red_wool", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_BLACK_WOOL = BLOCKS.register("set_wall_black_wool", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_GOLD_BLOCK = BLOCKS.register("set_wall_gold_block", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_IRON_BLOCK = BLOCKS.register("set_wall_iron_block", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_SMOOTH_QUARTZ = BLOCKS.register("set_wall_smooth_quartz", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_SMOOTH_RED_SANDSTONE = BLOCKS.register("set_wall_smooth_red_sandstone", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_SMOOTH_SANDSTONE = BLOCKS.register("set_wall_smooth_sandstone", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_SMOOTH_STONE = BLOCKS.register("set_wall_smooth_stone", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_BRICKS = BLOCKS.register("set_wall_bricks", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); public static final RegistryObject<Block> SET_WALL_TNT = BLOCKS.register("set_wall_tnt", () -> new SetWall(Block.Properties.create(Material.WOOD).hardnessAndResistance(3f, 3f).harvestTool(ToolType.AXE).sound(SoundType.WOOD).setLightLevel((state) -> {return 2;}))); } -
Register blocks and items using Data Generators.
JoppeGames3 replied to JoppeGames3's topic in Modder Support
It is true, when I delete them it runs fast... -
is there a way to register multiple variants of one block so that instead of 16 lines like this: public static final RegistryObject<Block> WHITE_STUDIO_LIGHT = BLOCKS.register("white_studio_light", () -> new StudioLight(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 15;}))); public static final RegistryObject<Block> ORANGE_STUDIO_LIGHT = BLOCKS.register("orange_studio_light", () -> new StudioLight(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 15;}))); public static final RegistryObject<Block> MAGENTA_STUDIO_LIGHT = BLOCKS.register("magenta_studio_light", () -> new StudioLight(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 15;}))); public static final RegistryObject<Block> LIGHT_BLUE_STUDIO_LIGHT = BLOCKS.register("light_blue_studio_light", () -> new StudioLight(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 15;}))); public static final RegistryObject<Block> YELLOW_STUDIO_LIGHT = BLOCKS.register("yellow_studio_light", () -> new StudioLight(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 15;}))); public static final RegistryObject<Block> LIME_STUDIO_LIGHT = BLOCKS.register("lime_studio_light", () -> new StudioLight(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 15;}))); public static final RegistryObject<Block> PINK_STUDIO_LIGHT = BLOCKS.register("pink_studio_light", () -> new StudioLight(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 15;}))); public static final RegistryObject<Block> GRAY_STUDIO_LIGHT = BLOCKS.register("gray_studio_light", () -> new StudioLight(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 15;}))); public static final RegistryObject<Block> LIGHT_GRAY_STUDIO_LIGHT = BLOCKS.register("light_gray_studio_light", () -> new StudioLight(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 15;}))); public static final RegistryObject<Block> CYAN_STUDIO_LIGHT = BLOCKS.register("cyan_studio_light", () -> new StudioLight(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 15;}))); public static final RegistryObject<Block> PURPLE_STUDIO_LIGHT = BLOCKS.register("purple_studio_light", () -> new StudioLight(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 15;}))); public static final RegistryObject<Block> BLUE_STUDIO_LIGHT = BLOCKS.register("blue_studio_light", () -> new StudioLight(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 15;}))); public static final RegistryObject<Block> BROWN_STUDIO_LIGHT = BLOCKS.register("brown_studio_light", () -> new StudioLight(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 15;}))); public static final RegistryObject<Block> GREEN_STUDIO_LIGHT = BLOCKS.register("green_studio_light", () -> new StudioLight(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 15;}))); public static final RegistryObject<Block> RED_STUDIO_LIGHT = BLOCKS.register("red_studio_light", ()-> new StudioLight(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 15;}))); public static final RegistryObject<Block> BLACK_STUDIO_LIGHT = BLOCKS.register("black_studio_light", () -> new StudioLight(Block.Properties.create(Material.ROCK).hardnessAndResistance(0f, 0f).sound(SoundType.METAL).setLightLevel((state) -> {return 15;}))); it is just one line. because the way I do it now with the 16 lines makes loading the game too slow
-
anyone knows a tutorial on how to create vehicles or wants to explain it here?
-
how to make custom tags, so not add to one already existing but make your own. and how to implement them in recipes
-
my custom ones do...
-
I meant same rotation.. what do I have to check and how to replace it with another block
-
how to check what block gets right clicked by your item, and then replace it to another block, but with the same blockstate as the first block? I know I have to use the "onItemUse" method but don't know what to put after the 'if' this is what I got so far.... public ActionResultType onItemUse(ItemUseContext context) { World world = context.getWorld(); BlockPos blockpos = context.getPos(); BlockState blockstate = world.getBlockState(blockpos); BlockState block = blockstate.getBlockState(); if (block != null) {
-
I'm sorry
-
how to add my own on and off model there?
-
that isn't another model right?
-
well, the LIT part confuses me, the rest I can understand
-
Yeah, I looked at the Redstone lamp class but this: [removed vanilla code] doesn't help me with creating my own Redstone lamp