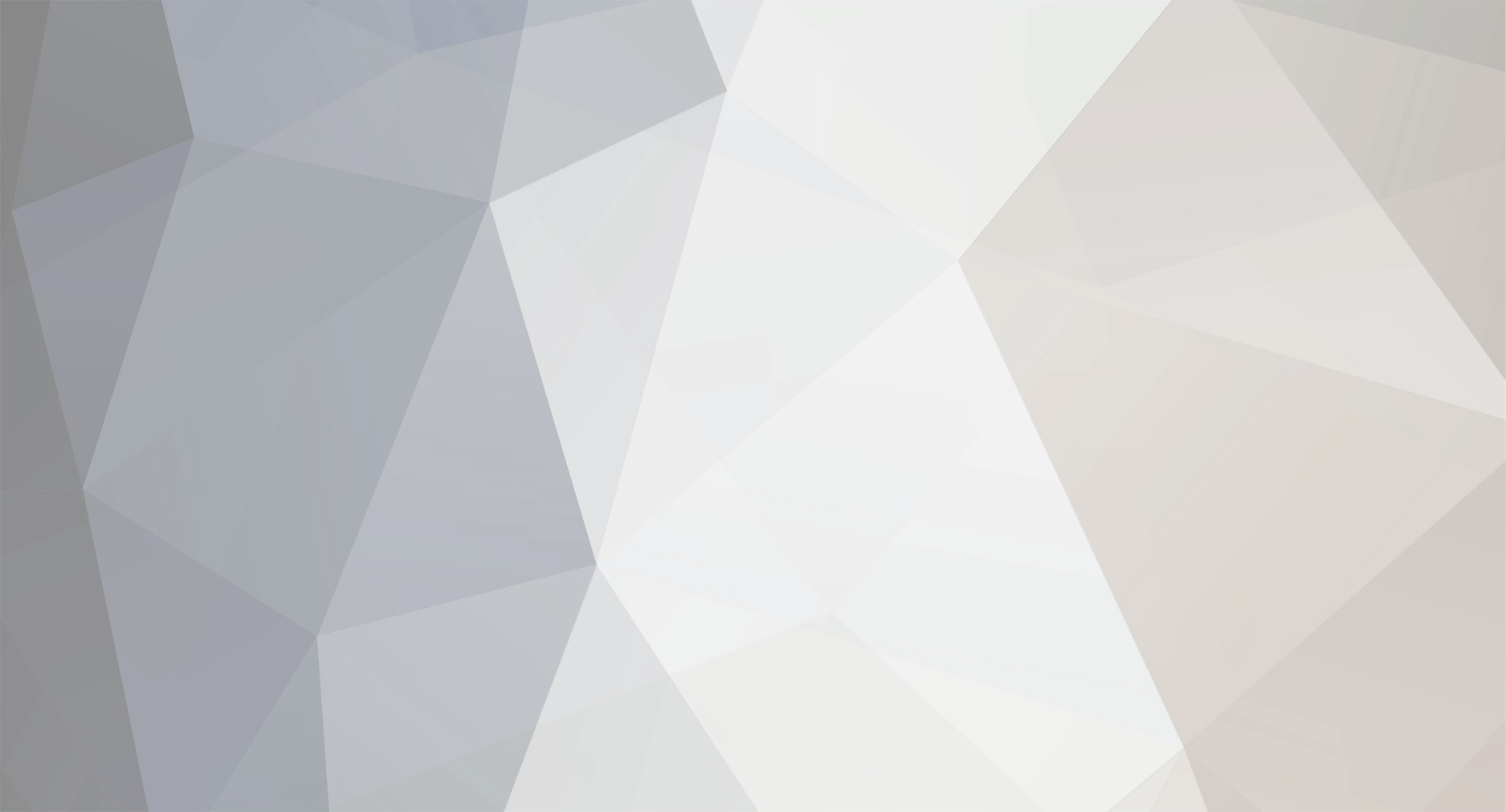
PermskyKit
-
Posts
12 -
Joined
-
Last visited
Posts posted by PermskyKit
-
-
12 minutes ago, diesieben07 said:
Block != RopeBlock.
Should it be a piece of code or a separate class? I don't know, since I'm just starting to learn Java and was writing this code from a tutorial.
-
3 minutes ago, diesieben07 said:
Ahem.
What? What? What?
-
10 hours ago, diesieben07 said:
Show where you register your block.
I'm sorry, for some reason I overlooked this question..
Class path:
TrueRopesMod/src/main/java/core.init/BlockInit
Code:
package core.init; import com.PermskyKit.TrueRopesMod.TrueRopesMod; import net.minecraft.block.Block; import net.minecraft.block.SoundType; import net.minecraft.block.material.Material; import net.minecraft.block.material.MaterialColor; import net.minecraft.block.AbstractBlock; import net.minecraftforge.fml.RegistryObject; import net.minecraftforge.registries.DeferredRegister; import net.minecraftforge.registries.ForgeRegistries; public class BlockInit { public static final DeferredRegister<Block> BLOCKS = DeferredRegister.create(ForgeRegistries.BLOCKS, TrueRopesMod.MOD_ID); public static final RegistryObject<Block> ROPE_BLOCK = BLOCKS.register("rope_block", () -> new Block(AbstractBlock.Properties.of(Material.GRASS, MaterialColor.TERRACOTTA_BROWN) .strength((float) 0.4) .sound(SoundType.CROP))); }
-
4 minutes ago, Logix said:
You've renamed
func_220053_a
to
getShape
, was this intentional?
Yes, because with func_220053_a Eclipse gives an error and suggests removing @Override.
-
3 minutes ago, Logix said:
All you need to do is to move the
VoxelShape shape = Block.box(6, 0, 6, 10, 16, 10);
declaration out of the function, into the class, and preferably mark it as static. That should fix your issue.
Yes, I did it and was able to change the code, now it looks like this:
package com.PermskyKit.TrueRopesMod.blocks; import net.minecraft.block.Block; import net.minecraft.block.BlockState; import net.minecraft.util.math.BlockPos; import net.minecraft.util.math.shapes.ISelectionContext; import net.minecraft.util.math.shapes.VoxelShape; import net.minecraft.world.IBlockReader; public class RopeBlock extends Block{ public RopeBlock(Properties properties) { super(properties); } private static final VoxelShape PART_1 = Block.box(6.0, 0.0, 6.0, 10.0, 16.0, 10.0); @Override public VoxelShape getShape(BlockState state, IBlockReader block, BlockPos pos, ISelectionContext context) { return PART_1; } }
But alas, the usual full block hitbox remains in the game...
-
10 hours ago, Logix said:
private static final VoxelShape PART_1 = Block.box(0.0, 0.0, 0.0, 16.0, 16.0, 16.0); // 16.0 is the full size of one block (in pixels) @Override public VoxelShape getShape(BlockState state, BlockGetter block, BlockPos pos, CollisionContext context) { return PART_1; } // If you want multiple hitboxes, combine them like: private static final VoxelShape AABB = Shapes.or(PART_1, PART_2); // You can add more parts here if necessary // Then simply return this from getShape() instead of the individual part.
BlockGetter and CollisionContext do not work in version 1.16.5. Do you know how they were designated in this version?
-
52 minutes ago, Luis_ST said:
in your IDE, in Eclipse you can search for the class when using CTRL + SHIFT + H,
im not familiar with the Intellij ShortcutsThanks a lot, I was not aware of this Eclipse feature. But even this method did not help, I am tormented by the question, maybe there should be some additional files besides "BlockInit", or the path to this "RopeBlock" file should be different.
I've already tried many variations of the code and .. Maybe I just don't know something else about Eclipse or the file structure.
-
7 hours ago, diesieben07 said:
Look at e.g. FlowerPotBlock.
Where can i find this example?
-
package com.PermskyKit.TrueRopesMod.block; import net.minecraft.block.Block; import net.minecraft.block.BlockState; import net.minecraft.util.math.BlockPos; import net.minecraft.util.math.shapes.ISelectionContext; import net.minecraft.util.math.shapes.VoxelShape; import net.minecraft.world.IBlockReader; public class RopeBlock extends Block{ public RopeBlock(Properties p_i48440_1_) { super(p_i48440_1_); } @Override public VoxelShape getShape(BlockState state, IBlockReader worldIn, BlockPos pos, ISelectionContext context) { VoxelShape shape = Block.box(6, 0, 6, 10, 16, 10); return shape; } }
1 hour ago, Draco18s said:Unfortunately it didn't help. Now my code looks like this, but the problem persists.
-
Where exactly should I write this?
4 minutes ago, Luis_ST said:use @Override when overwrite methods
-
Hi. I have a big question. I created a small block in the game, but I can't adjust the size of its hitbox in any way, and no errors are generated. I am attaching a screenshot and block code, I really hope for help.
package com.PermskyKit.TrueRopesMod.block; import net.minecraft.block.Block; import net.minecraft.block.BlockState; import net.minecraft.util.math.BlockPos; import net.minecraft.util.math.shapes.ISelectionContext; import net.minecraft.util.math.shapes.VoxelShape; import net.minecraft.world.IBlockReader; public class RopeBlock extends Block{ public RopeBlock(Properties p_i48440_1_) { super(p_i48440_1_); } public VoxelShape func_220053_a(BlockState state, IBlockReader worldIn, BlockPos pos, ISelectionContext context) { VoxelShape shape = Block.box(6, 0, 6, 10, 16, 10); return shape; } }
VoxelShapes 1.16.5
in Modder Support
Posted
Ahem, I'm sorry, I did a bit reworking of the mod structure, here's how the block is registered: