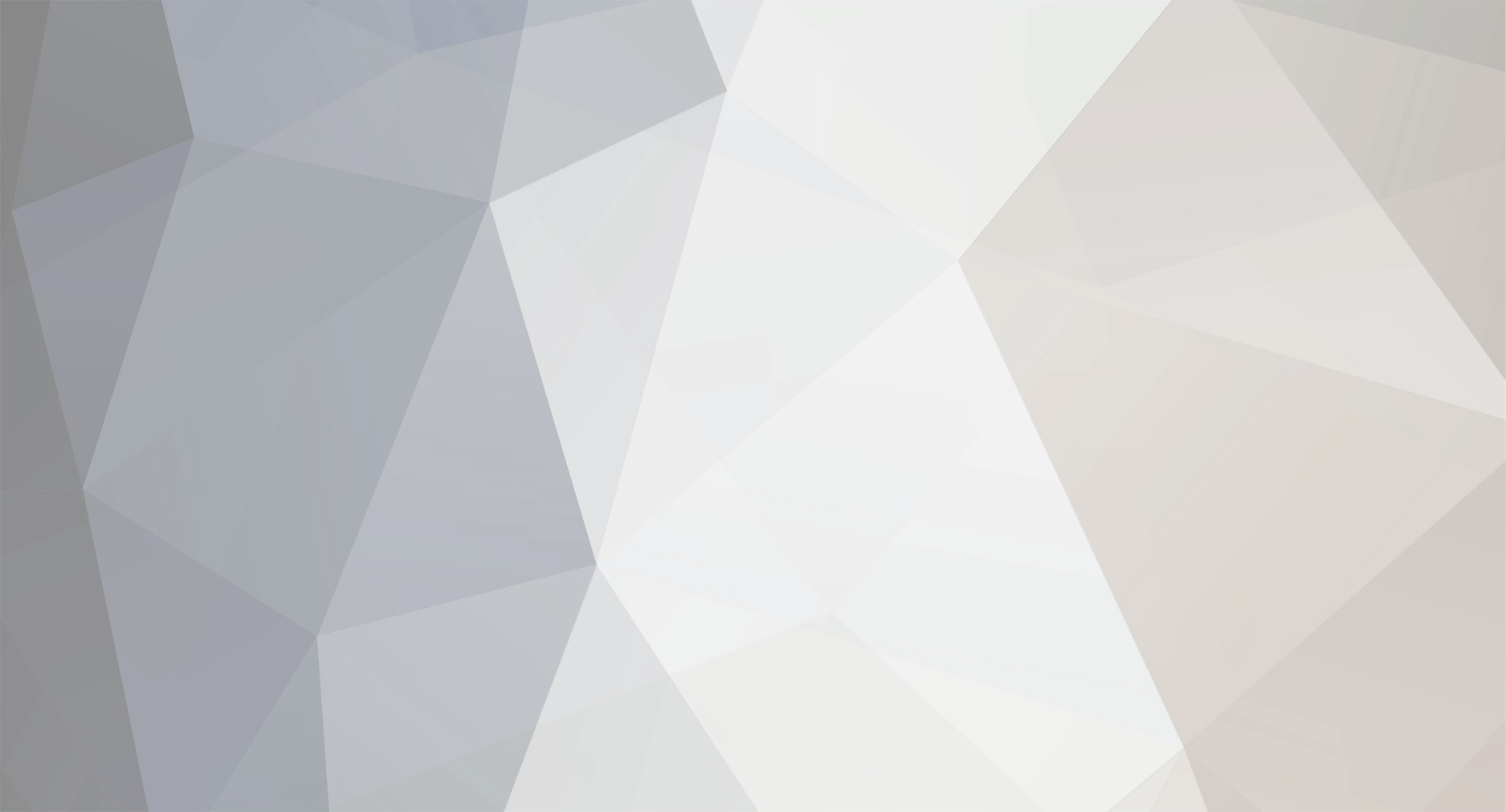
Lua
Members-
Posts
191 -
Joined
-
Last visited
Everything posted by Lua
-
nobody at all?
-
Bbbbbump
-
Hi, Im adding background music but Ive ran into a problem making sure no other music is playing before I play mine. Heres what I got @SubscribeEvent public void playMyMusic(ClientTickEvent event) { Random rand = new Random(); ISound soundCache = null; SoundHandler handler = Minecraft.getMinecraft().getSoundHandler(); int i = rand.nextInt(1000); if(soundCache == null || !handler.isSoundPlaying(soundCache)) { if(i == 0) { System.out.println("playing sound"); soundCache = new PositionedSoundRecord(new ResourceLocation(Arcticraft.MOD_ID + ":records.frozen_feelings"), 1.0F, 1.0F, 1.0F, 1.0F, 1.0F); handler.playSound(soundCache); } } } My sound plays but it will play over the top of any currently playing which I dont want. Obviously, its playing because soundCache does = null but thats because I have no idea how ISound works and didnt get any google results.
-
Yeah
-
I bind the texture to the model in TESR but I just wanted the icons to be different and now they are. getIconFromDamage was exactly what I needed.
-
Yeah, i just need the icons to be differenet thats all.
-
In the item class I did register em with and now theyre just invisible. @SideOnly(Side.CLIENT) public static final String[] iciclesTextures = new String[]{"icicle", "icicle", "icicle", "icicle_ud","icicle_ud", "icicle_ud"}; @SideOnly(Side.CLIENT) private IIcon[] texture; public void registerIcons(IIconRegister iconRegister) { this.texture = new IIcon[iciclesTextures.length]; for (int i = 0; i < iciclesTextures.length; ++i) { this.texture[i] = iconRegister.registerIcon(Arcticraft.MOD_ID + ":" + iciclesTextures[i]); } } Im not quite sure how I would just render em on RenderItem
-
Ok so I tried making a IItemRenderer and I came up with this and yes I registered it in the clientProxy. package net.arcticraft.item.render; import net.arcticraft.main.Arcticraft; import net.minecraft.item.ItemStack; import net.minecraftforge.client.IItemRenderer; public class ItemIcicleRender implements IItemRenderer{ public ItemIcicleRender(){} @Override public boolean handleRenderType(ItemStack item, ItemRenderType type) { return true; } @Override public boolean shouldUseRenderHelper(ItemRenderType type, ItemStack item, ItemRendererHelper helper) { return true; } @Override public void renderItem(ItemRenderType type, ItemStack item, Object... data) { System.out.println(item.getItemDamage()); if(item.getItemDamage() == 0 && item.getItemDamage() == 1 && item.getItemDamage() == 2) { item.getItem().setTextureName(Arcticraft.MOD_ID + ":icicle"); } else { item.getItem().setTextureName(Arcticraft.MOD_ID + ":icicle_ud"); } } } But they just come up as the missing texture texture(the purple black squares one). Is this because I commented out the getIcon and registerIcon in the block method? Probably, but I shouldnt need em should since its a tileentity w/ model and it uses the same texture.
-
Is there a way to check that no background music is already playing first before I play mine?
-
Okay, thank you.
-
Yeah, the icon in your hand. Not the actual block texture.
-
Noooo, the texture for the actual blocks should be the same, I just want the icons to differ.
-
package net.arcticraft.tileentity.renderers; import net.arcticraft.block.BlockIcicle; import net.arcticraft.main.Arcticraft; import net.arcticraft.tileentity.TileEntityIcicle; import net.arcticraft.tileentity.models.ModelBlockIcicle; import net.minecraft.client.renderer.tileentity.TileEntitySpecialRenderer; import net.minecraft.tileentity.TileEntity; import net.minecraft.util.ResourceLocation; import org.lwjgl.opengl.GL11; import cpw.mods.fml.client.FMLClientHandler; public class TileEntityIcicleRender extends TileEntitySpecialRenderer{ private ModelBlockIcicle model; public TileEntityIcicleRender(){ model = new ModelBlockIcicle(); } public void renderAModelAt(TileEntityIcicle tileEntity, double x, double y, double z, float f) { TileEntityIcicle icicle = (TileEntityIcicle) tileEntity; icicle = (TileEntityIcicle) tileEntity.getWorldObj().getTileEntity(tileEntity.xCoord, tileEntity.yCoord, tileEntity.zCoord); int meta = 0; if(tileEntity.getWorldObj() != null) { meta = tileEntity.getBlockMetadata(); icicle.rotation = meta; } FMLClientHandler.instance().getClient().renderEngine.bindTexture(new ResourceLocation(Arcticraft.MOD_ID, "textures/blocks/modeled_blocks/icicle.png")); GL11.glPushMatrix(); GL11.glEnable(GL11.GL_NORMALIZE); GL11.glEnable(GL11.GL_BLEND); GL11.glBlendFunc(GL11.GL_SRC_ALPHA, GL11.GL_ONE_MINUS_SRC_ALPHA); GL11.glTranslatef((float) x + 0.5F, (float) y + 1.5F, (float) z + 0.5F); GL11.glScalef(1.0F, -1F, -1F); GL11.glRotatef(icicle.rotation * 90, 0.0F, 1.0F, 0.0F); if(icicle.type == 0 || icicle.type == 3) { // large GL11.glScalef(2.0F, 1.0F, 1F); } else if(icicle.type == 1 || icicle.type == 4) { // regular GL11.glScalef(1.5F, 1F, 1F); } else if(icicle.type == 2 || icicle.type == 5) { // small GL11.glScalef(1.0F, 1F, 1F); } if(icicle.upsideDown){ GL11.glRotatef(180, 1, 0, 0); GL11.glTranslatef(0, (float) -1.5F, 0); } model.renderAll(); GL11.glPopMatrix(); } @Override public void renderTileEntityAt(TileEntity tileEntity, double x, double y, double z, float par8) { this.renderAModelAt((TileEntityIcicle) tileEntity, x, y, z, par8); } }
-
So what could I do instead? Because right now they all just use the icicle icon.
-
Hi, I've created a metadata block with 6 subtypes(0-5). The block is a tile entity with a special renderer and it uses the same model but just rotated a bit. However, I want the icon for the 0-2 blocks to be one icon and the 3-5 blocks be another. Obviously, its got to with getIcon method but I can't get them to be different and I thought this would be a really simple task. Heres the relevant code: @SideOnly(Side.CLIENT) @Override public void registerBlockIcons(IIconRegister iconRegister) { this.blockIcon = iconRegister.registerIcon(Arcticraft.MOD_ID + ":icicle_ud"); this.icicle = iconRegister.registerIcon(Arcticraft.MOD_ID + ":icicle"); } @SideOnly(Side.CLIENT) public IIcon getIcon(int side, int meta) { if(meta == 3 || meta == 4 || meta == 5){ return this.blockIcon; } else{ return this.icicle; } }
-
hmmm, still confused
-
Okay, I've got the classes setup but I have no idea how I'd change my old code to make it fit with this new system.
-
Hi, I'm currently updating a mod from 1.6.2 to 1.7. I've managed to do most things but I've recently ran into a issue with updating a packet. Basically, I need someone to explain how I update this packet and so I can use it. public class AC_PacketHandler implements IPacketHandler { @Override public void onPacketData(INetworkManager manager, Packet250CustomPayload packet, Player player) { if(packet.channel.equals(Strings.CHANNEL_ROPE_POSITION)) { this.handleRopePosition(packet, player); } } private void handleRopePosition(Packet250CustomPayload packet, Player plyr) { DataInputStream inputStream = new DataInputStream(new ByteArrayInputStream(packet.data)); int captainId; int hookId; try { captainId = inputStream.readInt(); hookId = inputStream.readInt(); } catch(IOException e) { e.printStackTrace(); return; } WorldClient world; if(plyr instanceof EntityPlayer) { world = (WorldClient) ((EntityPlayer) plyr).worldObj; } else { return; } AC_EntityPirateHook hook = (AC_EntityPirateHook) world.getEntityByID(hookId); AC_EntityCaptain captain = (AC_EntityCaptain) world.getEntityByID(captainId); hook.setThrower(captain); captain.resetHookCooldown(); captain.setHookAirBorne(true); double rotation = (captain.rotationYaw + 70.0F) / (180.0F / Math.PI); double hookLaunchX = Math.cos(rotation); double hookLaunchY = 1.4D; double hookLaunchZ = Math.sin(rotation); for (int i = 0; i < 10; i++) { captain.worldObj.spawnParticle("smoke", captain.posX + hookLaunchX, captain.posY + hookLaunchY, captain.posZ + hookLaunchZ, (captain.getRNG().nextDouble() - 0.5D) / 5D, (captain.getRNG().nextDouble() - 0.5D) / 5D, (captain.getRNG().nextDouble() - 0.5D) / 5D); } } This is how I used it before Packet250CustomPayload packet = new Packet250CustomPayload(); packet.channel = Strings.CHANNEL_ROPE_POSITION; packet.data = bos.toByteArray(); packet.length = bos.size(); ((WorldServer) this.worldObj).getEntityTracker().sendPacketToAllPlayersTrackingEntity(hook, packet); urh it messed up my formatting.
-
Hi, I'm looking to replace the vanilla which is placed by bucket in my custom dimension. So I first looked how vanilla does with the water in the nether and they do by a method in ItemBucket which I obviously can't edit. So I looked at Forge events for some answers and I came across the PlayerInterectEvent so this is what I've came up. I've just tried cancelling the interaction at first but it doesn't seem to work. But it gets called and If I try it with a torch it gets cancelled so Im not sure what Im doing wrong here. Heres the code: @SubscribeEvent public void playerInteract(PlayerInteractEvent event) { ItemStack hand = event.entityPlayer.inventory.getCurrentItem(); if(event.entityPlayer != null && hand != null) { if(event.entityPlayer.dimension == 3 && hand.getItem() == Items.water_bucket) { if(event.action == event.action.RIGHT_CLICK_BLOCK) { System.out.println("call"); event.useItem = Result.DENY; event.setCanceled(true); //event.world.setBlock(event.x, event.y, event.z, ACBlocks.frostWaterIce); } } } }
-
Nevermind, I've managed to do it. For anyone who wants to know: Create an int in your entity, in the constructor of the entity, randomise the int using Javas random class. Create a method to return the int. In your render class, in the getEntityTexture method which returns a resourcelocation, you gotta get an object of your entity class, to do that, cast it to the entity param. Then, it simples, just use an else if and check the ints value. EG: @Override protected ResourceLocation getEntityTexture(Entity ent){ EntityGreedling greedlingObj = (EntityGreedling)ent; int texture = greedlingObj.getTexture(); if(texture == 0){ return greedlingOriginal; } else if(texture == 1){ return greedlingEndling; } else{ return greedlingSpecial; } }
-
Okay, I setup a onEntityConstructingEvent, heres my code: package fl.main; import java.util.Random; import net.minecraft.util.ResourceLocation; import net.minecraftforge.event.ForgeSubscribe; import net.minecraftforge.event.entity.EntityEvent.EntityConstructing; import fl.entities.EntityGreedling; public class ForgeEvents { private Random rand = new Random(); public ResourceLocation greedlingTexture; private ResourceLocation greedlingOriginal = new ResourceLocation(Reference.MOD_ID, "textures/entities/greedling/greedling_original.png"); private ResourceLocation greedlingEndling = new ResourceLocation(Reference.MOD_ID, "textures/entities/greedling/greedling_endling.png"); private ResourceLocation greedlingSpecial = new ResourceLocation(Reference.MOD_ID, "textures/entities/greedling/greedling_special.png"); @ForgeSubscribe public void greedlingConstruction(EntityConstructing event){ if(event.entity instanceof EntityGreedling) { switch(rand.nextInt(3)) { case 0: greedlingTexture = greedlingOriginal; break; case 1: greedlingTexture = greedlingEndling; break; case 2: greedlingTexture = greedlingSpecial; break; } } } } Render Class package fl.renders; import java.util.Random; import net.minecraft.client.model.ModelBase; import net.minecraft.client.renderer.entity.RenderLiving; import net.minecraft.entity.Entity; import net.minecraft.util.ResourceLocation; import fl.main.ForgeEvents; import fl.main.Reference; import fl.models.ModelGreedling; public class RenderGreedling extends RenderLiving { public RenderGreedling(ModelBase modelBase, float par2) { super(new ModelGreedling(), 0.3F); } @Override protected ResourceLocation getEntityTexture(Entity ent){ ForgeEvents fe = new ForgeEvents(); return fe.greedlingTexture; } } I had to create an object to the class so I could access greedlingTexture, the alternative would be making it static which would lead to all mobs being the same texture I must be missing something However, I hit an NPE for the texture registration. Error: 2014-01-30 00:25:45 [iNFO] [sTDERR] Caused by: java.lang.NullPointerException 2014-01-30 00:25:45 [iNFO] [sTDERR] at net.minecraft.client.resources.SimpleReloadableResourceManager.getResource(SimpleReloadableResourceManager.java:59) 2014-01-30 00:25:45 [iNFO] [sTDERR] at net.minecraft.client.renderer.texture.SimpleTexture.loadTexture(SimpleTexture.java:31) 2014-01-30 00:25:45 [iNFO] [sTDERR] at net.minecraft.client.renderer.texture.TextureManager.loadTexture(TextureManager.java:84) 2014-01-30 00:25:45 [iNFO] [sTDERR] ... 21 more 2014-01-30 00:25:45 [iNFO] [sTDERR] net.minecraft.util.ReportedException: Registering texture 2014-01-30 00:25:45 [iNFO] [sTDERR] at net.minecraft.client.renderer.texture.TextureManager.loadTexture(TextureManager.java:99) 2014-01-30 00:25:45 [iNFO] [sTDERR] at net.minecraft.client.renderer.texture.TextureManager.bindTexture(TextureManager.java:41) 2014-01-30 00:25:45 [iNFO] [sTDERR] at net.minecraft.client.renderer.entity.Render.bindTexture(Render.java:53) 2014-01-30 00:25:45 [iNFO] [sTDERR] at net.minecraft.client.renderer.entity.Render.bindEntityTexture(Render.java:48) 2014-01-30 00:25:45 [iNFO] [sTDERR] at net.minecraft.client.renderer.entity.RendererLivingEntity.renderModel(RendererLivingEntity.java:296) 2014-01-30 00:25:45 [iNFO] [sTDERR] at net.minecraft.client.renderer.entity.RendererLivingEntity.doRenderLiving(RendererLivingEntity.java:156) 2014-01-30 00:25:45 [iNFO] [sTDERR] at net.minecraft.client.renderer.entity.RenderLiving.doRenderLiving(RenderLiving.java:28) 2014-01-30 00:25:45 [iNFO] [sTDERR] at net.minecraft.client.renderer.entity.RenderLiving.doRender(RenderLiving.java:142) 2014-01-30 00:25:45 [iNFO] [sTDERR] at net.minecraft.client.renderer.entity.RenderManager.renderEntityWithPosYaw(RenderManager.java:312) 2014-01-30 00:25:45 [iNFO] [sTDERR] at net.minecraft.client.renderer.entity.RenderManager.renderEntity(RenderManager.java:281) 2014-01-30 00:25:45 [iNFO] [sTDERR] at net.minecraft.client.renderer.RenderGlobal.renderEntities(RenderGlobal.java:524) 2014-01-30 00:25:45 [iNFO] [sTDERR] at net.minecraft.client.renderer.EntityRenderer.renderWorld(EntityRenderer.java:1160) 2014-01-30 00:25:45 [iNFO] [sTDERR] at net.minecraft.client.renderer.EntityRenderer.updateCameraAndRender(EntityRenderer.java:1006) 2014-01-30 00:25:45 [iNFO] [sTDERR] at net.minecraft.client.Minecraft.runGameLoop(Minecraft.java:945) 2014-01-30 00:25:45 [iNFO] [sTDERR] at net.minecraft.client.Minecraft.run(Minecraft.java:837) 2014-01-30 00:25:45 [iNFO] [sTDERR] at net.minecraft.client.main.Main.main(Main.java:93) 2014-01-30 00:25:45 [iNFO] [sTDERR] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) 2014-01-30 00:25:45 [iNFO] [sTDERR] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) 2014-01-30 00:25:45 [iNFO] [sTDERR] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) 2014-01-30 00:25:45 [iNFO] [sTDERR] at java.lang.reflect.Method.invoke(Unknown Source) 2014-01-30 00:25:45 [iNFO] [sTDERR] at net.minecraft.launchwrapper.Launch.launch(Launch.java:131) 2014-01-30 00:25:45 [iNFO] [sTDERR] at net.minecraft.launchwrapper.Launch.main(Launch.java:27)
-
Hi, I attempted to do a seemingly simple task; randomise mob textures. Here is what I tried to do: package fl.renders; import java.util.Random; import net.minecraft.client.model.ModelBase; import net.minecraft.client.renderer.entity.RenderLiving; import net.minecraft.entity.Entity; import net.minecraft.util.ResourceLocation; import fl.main.Reference; import fl.models.ModelGreedling; public class RenderGreedling extends RenderLiving { private Random rand = new Random(); private static ResourceLocation greedlingTexture; private static final ResourceLocation greedlingOriginal = new ResourceLocation(Reference.MOD_ID, "textures/entities/greedling/greedling_original.png"); private static final ResourceLocation greedlingEndling = new ResourceLocation(Reference.MOD_ID, "textures/entities/greedling/greedling_endling.png"); private static final ResourceLocation greedlingSpecial = new ResourceLocation(Reference.MOD_ID, "textures/entities/greedling/greedling_special.png"); public RenderGreedling(ModelBase modelBase, float par2) { super(new ModelGreedling(), 0.3F); } @Override protected ResourceLocation getEntityTexture(Entity ent){ switch(rand.nextInt(3)) { case 0: greedlingTexture = greedlingOriginal; break; case 1: greedlingTexture = greedlingEndling; break; case 2: greedlingTexture = greedlingSpecial; break; } return greedlingTexture; } } However, getEntityTexture is constantly being called which makes it choose a new textures each time. I'm not sure how to get around it, advice?
-
@Diesieben07 Thank you for the information.
-
I wasn't aware of that. So, don't you need the @NetworkMod annotation anymore?
-
Hi, After setting up ForgeGradle I noticed there was a short example mod but I accidentally deleted it. Please can someone upload theirs to a file sharing site, or just post the code below. Thank you.