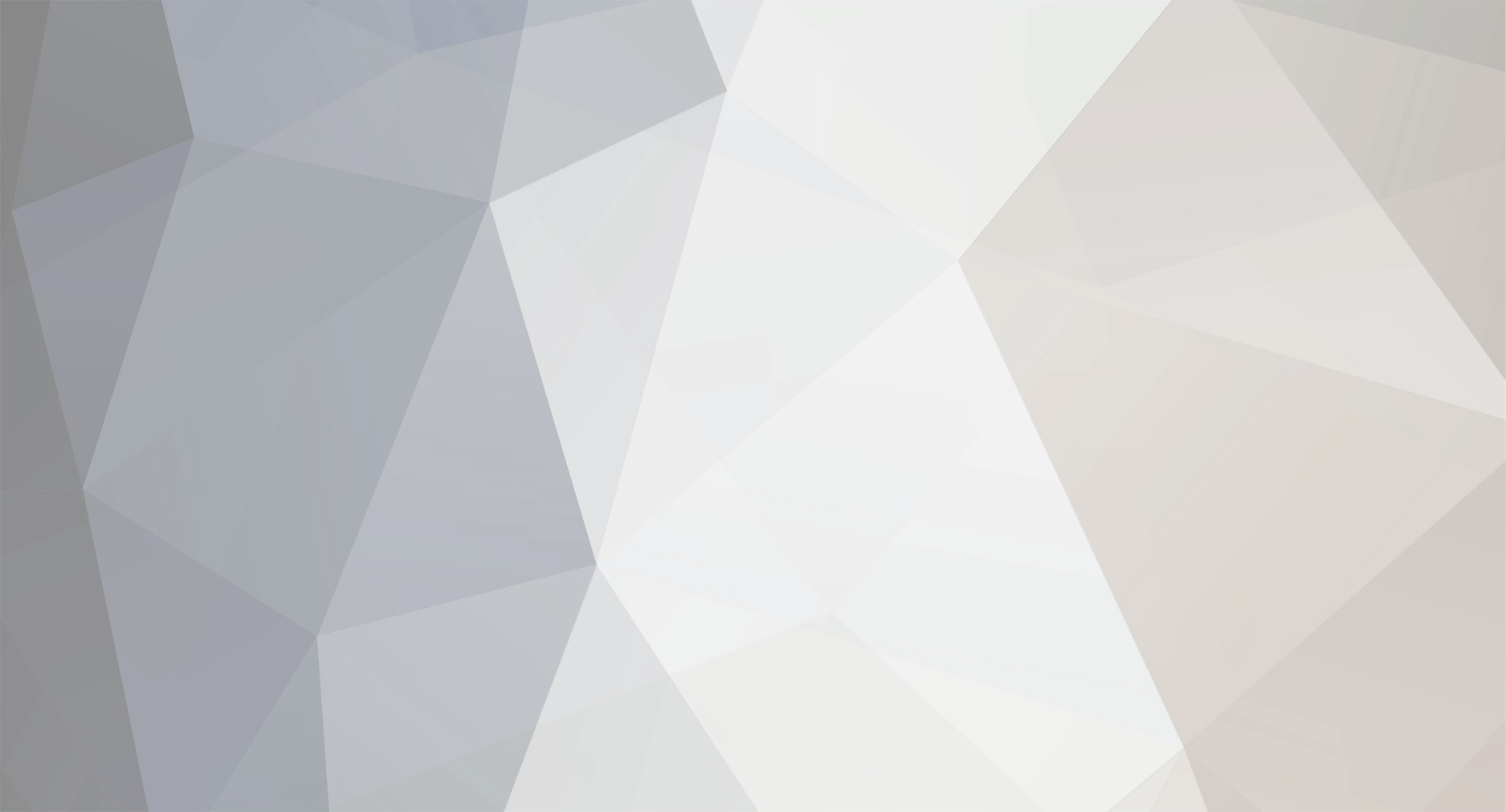
bossmania
Members-
Posts
13 -
Joined
-
Last visited
Everything posted by bossmania
-
Hi, so I was making an item that sends info to the server to do some work, and I want the server to send the client back the results? How do I do that? Here is my code: The snippet that sends the packet to the server SimpleChannelNetwork.CHANNEL.sendToServer(new CreateStructureCompass("fortress")); The file that manage the traffic package io.github.bossmania.NetherandEndMaps.core.network; import io.github.bossmania.NetherandEndMaps.NetherandEndMaps; import io.github.bossmania.NetherandEndMaps.core.network.message.CreateStructureCompass; import net.minecraft.util.ResourceLocation; import net.minecraftforge.fml.network.NetworkRegistry; import net.minecraftforge.fml.network.simple.SimpleChannel; public class SimpleChannelNetwork { public static final String NETWORK_VERSION = "0.1.0"; public static final SimpleChannel CHANNEL = NetworkRegistry.newSimpleChannel( new ResourceLocation(NetherandEndMaps.MOD_ID, "network"), () -> NETWORK_VERSION, version -> version.equals(NETWORK_VERSION), version -> version.equals(NETWORK_VERSION)); public static void init() { CHANNEL.registerMessage(0, CreateStructureCompass.class ,CreateStructureCompass::encode, CreateStructureCompass::decode, CreateStructureCompass::handle); } } the code that handle the packet receive and where I want the server to send back a packet to the client package io.github.bossmania.NetherandEndMaps.core.network.message; import java.util.function.Supplier; import io.github.bossmania.NetherandEndMaps.common.items.TemporaryItem; import net.minecraft.entity.player.ServerPlayerEntity; import net.minecraft.item.ItemStack; import net.minecraft.network.PacketBuffer; import net.minecraft.util.math.BlockPos; import net.minecraft.world.gen.feature.structure.Structure; import net.minecraft.world.server.ServerWorld; import net.minecraftforge.fml.network.NetworkEvent; import net.minecraft.item.Item; import net.minecraft.item.ItemGroup; public class CreateStructureCompass { public String structureType; public CreateStructureCompass() { } //create the constructor and store the structureType public CreateStructureCompass(String structureType) { this.structureType = structureType; } //encode the message public static void encode(CreateStructureCompass message, PacketBuffer buffer) { buffer.writeString(message.structureType); } //decode the message public static CreateStructureCompass decode(PacketBuffer buffer) { return new CreateStructureCompass(buffer.readString()); } //execute function when message is sent to server public static void handle(CreateStructureCompass message, Supplier<NetworkEvent.Context> contextSupplier) { NetworkEvent.Context context = contextSupplier.get(); context.enqueueWork(() -> { //get the player and the server ServerPlayerEntity player = context.getSender(); ServerWorld SEWorld = player.getServerWorld(); //get the location of the structure BlockPos structureLocation = SEWorld.func_241117_a_(Structure.FORTRESS, player.getPosition(),100, false); //this is where I want the server to send structureLocation back to the player }); context.setPacketHandled(true); } }
-
After doing some digging, I found out that ServerWorld.func_24117_a_(Structure<?>, BlockPos, int, Boolean) return the BlockPos of the structure. Thanks for your help.
-
I have tried using that, but it says that the method is undefined. Where can I find the locate command?
-
Hi, so I am currently making a custom compass that points to a structure. I was able to get the compass to point to a hard coded location, but I want it to point to a structure, like a village. I have tried looking through the web to find an answer, but no results have shown up. So, how do I get a coordinate of a structure?
-
How to generate a explorer map that points to a village in code?
bossmania replied to bossmania's topic in Modder Support
So then, how else do I get a loot table to run in the code? -
How to generate a explorer map that points to a village in code?
bossmania replied to bossmania's topic in Modder Support
So how do I access the server, and send the ServerWorld to the client? -
How to generate a explorer map that points to a village in code?
bossmania replied to bossmania's topic in Modder Support
How do I check it? And if it's not an instance of ServerWorld, then how do I get the ServerWorld? -
How to generate a explorer map that points to a village in code?
bossmania replied to bossmania's topic in Modder Support
Ok, so after doing some digging into the Block class, I have found out that it uses this function to run a loot table. public static List<ItemStack> getDrops(BlockState state, ServerWorld worldIn, BlockPos pos, @Nullable TileEntity tileEntityIn, @Nullable Entity entityIn, ItemStack stack) { LootContext.Builder lootcontext$builder = (new LootContext.Builder(worldIn)).withRandom(worldIn.rand).withParameter(LootParameters.field_237457_g_, Vector3d.copyCentered(pos)).withParameter(LootParameters.TOOL, stack).withNullableParameter(LootParameters.THIS_ENTITY, entityIn).withNullableParameter(LootParameters.BLOCK_ENTITY, tileEntityIn); return state.getDrops(lootcontext$builder); } But, how do I get a ServerWorld to pass into the function? I have tried passing "(ServerWorld)World", like how the Block class did it, but it crashes the game. This is the error message. The game crashed whilst unexpected error Error: java.lang.ClassCastException: net.minecraft.client.world.ClientWorld cannot be cast to net.minecraft.world.server.ServerWorld Exit Code: -1 -
How to generate a explorer map that points to a village in code?
bossmania replied to bossmania's topic in Modder Support
So I tried to look for how Blocks call the loot table, but I was not able to find it. Can you provide how it calls the loot table? -
How to generate a explorer map that points to a village in code?
bossmania replied to bossmania's topic in Modder Support
Can you elaborate more on that, and give an example of it? -
How to generate a explorer map that points to a village in code?
bossmania replied to bossmania's topic in Modder Support
I was able to get a recipe loot table to generate an explorer map, but the problem with this method is that I want the explorer maps to also point to structures in all three dimensions, and I can't prevent the player from crafting the map in the wrong dimension. -
Hi, I'm new to modding and to this forum, and I'm trying to get an item that gives the player an explorer map that points to a village. I was able to do this with a loot table, but I was only able to get the loot table to run when a block is broken. Is there a way to have a loot table to run in code, or is there a different way to create an explorer map that points to a village?