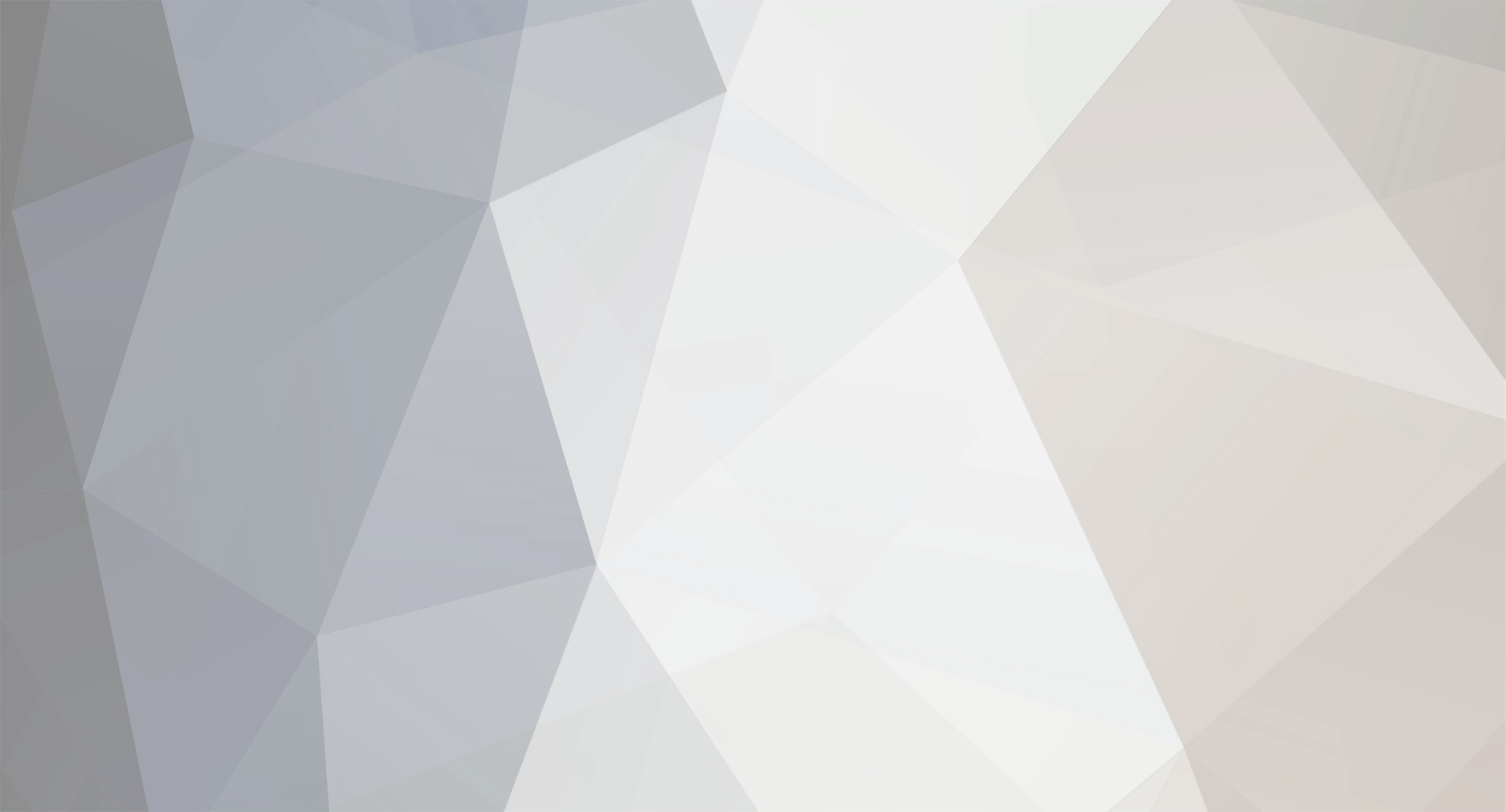
mahidhoni123
-
Posts
57 -
Joined
-
Last visited
Posts posted by mahidhoni123
-
-
Does this work?
public static final RegistryObject<ForgeSpawnEggItem> SKELETON_SPAWN_EGG = ITEMS.register("skeleton_spawn_egg", () -> new ForgeSpawnEggItem(new Supplier<EntityType<? extends Mob>>() { @Override public EntityType<? extends Mob> get() { return EntityType.SKELETON; } }, 0x00FFA8, 0x326D59, new Item.Properties().tab(CreativeModeTab.TAB_MATERIALS)));
-
Something like this -
public static final RegistryObject<ForgeSpawnEggItem> SKELETON_SPAWN_EGG = ITEMS.register("skeleton_spawn_egg", () -> new ForgeSpawnEggItem(null , 0x00FFA8, 0x326D59, new Item.Properties().tab(CreativeModeTab.TAB_MATERIALS)));
So what should I replace null with?
-
For now I have set it to null, but you can help -
public static final RegistryObject<ModSpawnEggItem> SKELETON_SPAWN_EGG = ITEMS.register("skeleton_spawn_egg", () -> new ModSpawnEggItem(null , 0x00FFA8, 0x326D59, new Item.Properties().tab(CreativeModeTab.TAB_MATERIALS)));
-
1 hour ago, mahidhoni123 said:
I typed ForgeSpawnEggItem.fromEntityType(EntityType.SKELETON) but now I saw a complimation error -
Required Registry Object extends<? net.minecraft.entity.EntityType
Provided SpawnEggItem
Luis_ST Can you please show me an example I am trying to create a ForgeSpawnEggItem in my ModSpawnEgg class, but it keeps on saying the same error as shown above
-
Please can you HELP instead of posting learn java learn java, PLEASE
-
Please check this link, hopefully you can check it out - https://docs.google.com/presentation/d/e/2PACX-1vQ3x-VnSHEmme9bIfJ26CeSuQHWZE8Atl4dRQvp9xtAItt5ucIZc6E8p8Edn1DMws35tJn6D4oR6x5p/pub?start=true&loop=false&delayms=3000
-
I typed ForgeSpawnEggItem.fromEntityType(EntityType.SKELETON) but now I saw a complimation error -
Required Registry Object extends<? net.minecraft.entity.EntityType
Provided SpawnEggItem
-
but how do you access this class? The forge provided spawn egg
-
Error =
Required RegistryObject<?extends EntityType<?>>
Provided EntityType<SKELETON>
-
I think the error comes from this java class even though it dosen't show any error -
package com.mahidhoni123.forgemod.items; import net.minecraft.core.BlockSource; import net.minecraft.core.Direction; import net.minecraft.core.dispenser.DefaultDispenseItemBehavior; import net.minecraft.nbt.CompoundTag; import net.minecraft.world.entity.EntityType; import net.minecraft.world.entity.MobSpawnType; import net.minecraft.world.item.ItemStack; import net.minecraft.world.item.SpawnEggItem; import net.minecraft.world.level.block.DispenserBlock; import net.minecraftforge.common.util.Lazy; import net.minecraftforge.fml.util.ObfuscationReflectionHelper; import net.minecraftforge.fmllegacy.RegistryObject; import java.util.ArrayList; import java.util.List; import java.util.Map; public class ModSpawnEggItem extends SpawnEggItem { protected static final List<ModSpawnEggItem> UNADDED_EGGS = new ArrayList<>(); private final Lazy<? extends EntityType<?>> entityTypeSupplier; public ModSpawnEggItem(final RegistryObject<? extends EntityType<?>> entityTypeSupplier, int p_43208_, int p_43209_, Properties p_43210_) { super(null, p_43208_, p_43209_, p_43210_); this.entityTypeSupplier = Lazy.of(entityTypeSupplier::get); UNADDED_EGGS.add(this); } public static void initSpawnEggs(){ final Map<EntityType<?>, SpawnEggItem> EGGS = ObfuscationReflectionHelper.getPrivateValue(SpawnEggItem.class, null, "field_195987_b"); DefaultDispenseItemBehavior dispenseBehavior = new DefaultDispenseItemBehavior() { @Override protected ItemStack execute(BlockSource p_123385_, ItemStack p_123386_) { Direction direction = p_123385_ .getBlockState().getValue(DispenserBlock.FACING); EntityType<?> type = ((SpawnEggItem) p_123386_.getItem()).getType(p_123386_.getTag()); type.spawn(p_123385_.getLevel(), p_123386_, null, p_123385_.getPos(), MobSpawnType.DISPENSER, direction != Direction.UP, false); p_123386_.shrink(1); return p_123386_; } }; for (final SpawnEggItem spawnEgg : UNADDED_EGGS) { EGGS.put(spawnEgg.getType(null ), spawnEgg); DispenserBlock.registerBehavior(spawnEgg, dispenseBehavior); } UNADDED_EGGS.clear(); } @Override public EntityType<?> getType(CompoundTag nbt) { return this.entityTypeSupplier.get(); } }
-
I tried this code but I got an error while making custom spawn egg for a skeleton(this skeleton is a vanilla minecraft entity) I think I know why this error is coming, but I don't know how to solve it, can you please help, I am sharing the code -
package com.anirudh.forgemod.init; import com.anirudh.forgemod.ForgeMod; import com.anirudh.forgemod.items.ItemBase; import com.anirudh.forgemod.items.ModSpawnEggItem; import com.anirudh.forgemod.items.StrongestApple; import com.anirudh.forgemod.util.enums.ModArmorMaterial; import net.minecraft.world.entity.EntityType; import net.minecraft.world.entity.EquipmentSlot; import net.minecraft.world.item.ArmorItem; import net.minecraft.world.item.CreativeModeTab; import net.minecraft.world.item.Item; import net.minecraftforge.fmllegacy.RegistryObject; import net.minecraftforge.registries.DeferredRegister; import net.minecraftforge.registries.ForgeRegistries; public class ModItems { public static DeferredRegister<Item> ITEMS = DeferredRegister.create(ForgeRegistries.ITEMS, ForgeMod.MOD_ID); //Items public static final RegistryObject<Item> RUBY = ITEMS.register("ruby", ItemBase::new); public static final RegistryObject<StrongestApple> POISON_APPLE = ITEMS.register("strongest_apple", StrongestApple::new); public static final RegistryObject<ModSpawnEggItem> SKELETON_SPAWN_EGG = ITEMS.register("skeleton_spawn_egg", () -> new ModSpawnEggItem(EntityType.SKELETON, 0x00FFA8, 0x326D59, new Item.Properties().tab(CreativeModeTab.TAB_MATERIALS))); //Armor public static final RegistryObject<ArmorItem> RUBY_HELMET = ITEMS.register("ruby_helmet", () -> new ArmorItem(ModArmorMaterial.RUBY, EquipmentSlot.HEAD, new Item.Properties().tab(CreativeModeTab.TAB_COMBAT))); public static final RegistryObject<ArmorItem> RUBY_CHESTPLATE = ITEMS.register("ruby_chestplate", () -> new ArmorItem(ModArmorMaterial.RUBY, EquipmentSlot.CHEST, new Item.Properties().tab(CreativeModeTab.TAB_COMBAT))); public static final RegistryObject<ArmorItem> RUBY_LEGGINGS = ITEMS.register("ruby_leggings", () -> new ArmorItem(ModArmorMaterial.RUBY, EquipmentSlot.LEGS, new Item.Properties().tab(CreativeModeTab.TAB_COMBAT))); public static final RegistryObject<ArmorItem> RUBY_BOOTS = ITEMS.register("ruby_boots", () -> new ArmorItem(ModArmorMaterial.RUBY, EquipmentSlot.FEET, new Item.Properties().tab(CreativeModeTab.TAB_COMBAT))); }
-
but I don't have the forge bot on discord, can you tell me the name for OreFeatureConfig, Please
-
Please can someone reply
-
Please can someone reply
-
Please can someone reply
-
Please can someone reply
-
my code -
package com.anirudh.forgemod.world.gen; import com.anirudh.forgemod.ForgeMod; import net.minecraft.world.level.biome.Biome; import net.minecraft.world.level.block.state.BlockState; import net.minecraftforge.eventbus.api.SubscribeEvent; import net.minecraftforge.fml.common.Mod; import net.minecraftforge.fml.event.lifecycle.FMLLoadCompleteEvent; import net.minecraftforge.registries.ForgeRegistries; @Mod.EventBusSubscriber(modid = ForgeMod.MOD_ID, bus = Mod.EventBusSubscriber.Bus.MOD) public class ModOreGen { @SubscribeEvent public static void generateOres(FMLLoadCompleteEvent event){ for (Biome biome : ForgeRegistries.BIOMES){ if (biome.getBiomeCategory() == Biome.BiomeCategory.NETHER){ } else if (biome.getBiomeCategory() == Biome.BiomeCategory.THEEND){ } else { } } } private static void genOre(Biome biome, int count, int bottomOffset, int topOffset, int max, OreFeatureConfig.FillerBlockType filler,BlockState defaultBlockstate, int size){ } }
Error - Cannot resolve symbol 'OreFeatureConfig' :31
-
please can someone reply
-
and what is called OreFeatureConfig, while making ore gen
-
-
The method is tab but while registering which tab the tutorial says .group(ItemGroup), but their is no ItemGroup, so is their a name for that as well?
-
In registry Handler -
public static final RegistryObject<ArmorItem> RUBY_HELMET = ITEMS.register("ruby_helmet", () -> new ArmorItem(ModArmorMaterial.RUBY, EquipmentSlot.HEAD, new Item.Properties().group()));
the same error as the previous one
-
In one of the lines I wrote
RUBY(ForgeMod.MOD_ID + ":ruby",1, new int[] { 2, 5, 6, 2, }, 999999999, SoundEvents.AMBIENT_CAVE, 1000.0F, () ->{ return Ingredient.of(RegistryHandler.RUBY.get()).group(); });
The error is - Cannot resolve method 'group' in Ingredient, I think the name has changed but to which name?
-
ok, one last question
I can't import IItemTier in forge mod minecraft
in Modder Support
Posted
and it was not IItemTier, rather Tier and now I have made it!