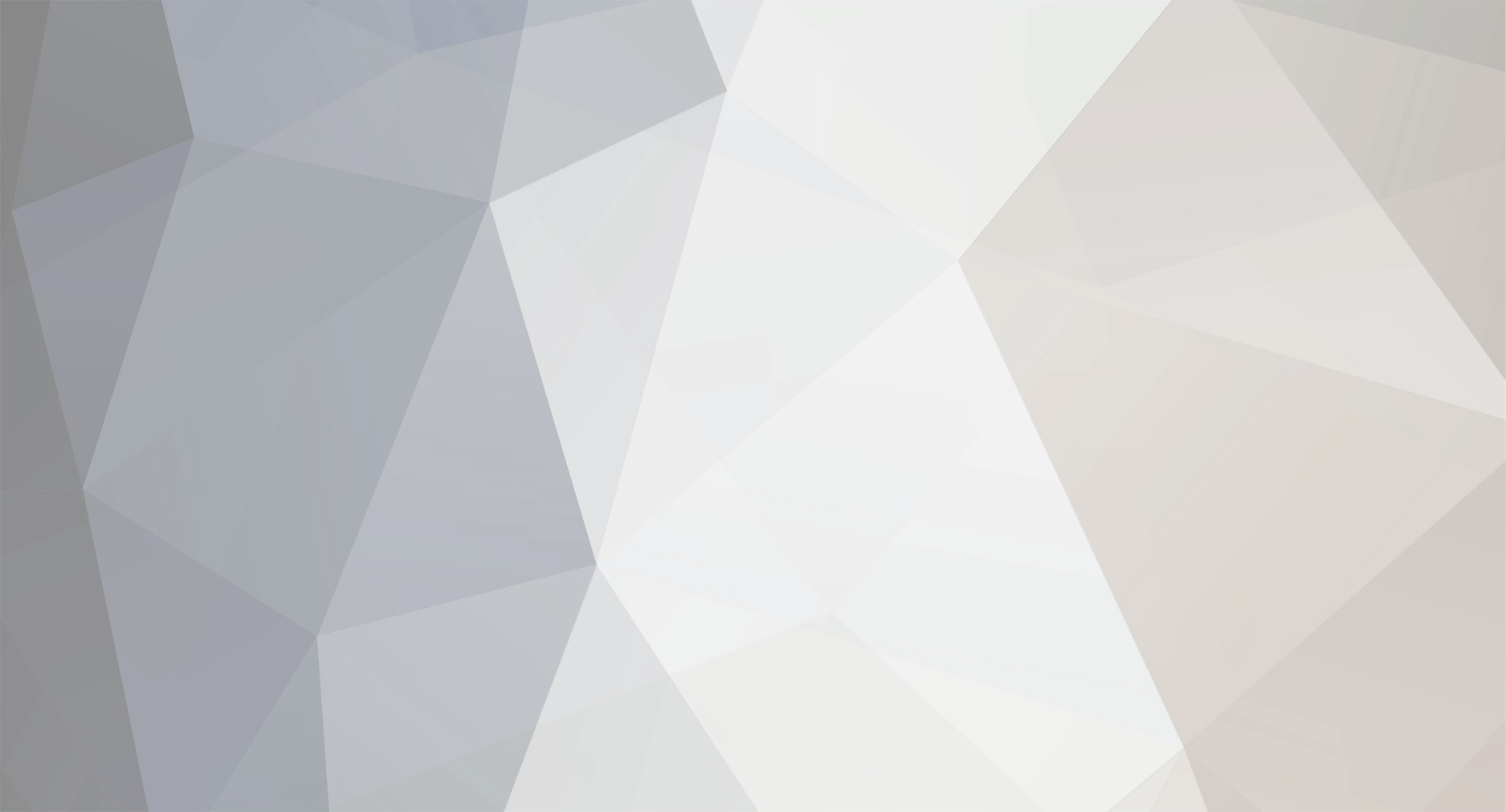
Gulg
Members-
Posts
19 -
Joined
-
Last visited
Recent Profile Visitors
The recent visitors block is disabled and is not being shown to other users.
Gulg's Achievements

Tree Puncher (2/8)
0
Reputation
-
How can I get vanilla Minecraft source code with minimal distortion?
Gulg replied to Gulg's topic in Modder Support
I want to create custom mc client. -
How can I get vanilla Minecraft source code with minimal distortion?
Gulg replied to Gulg's topic in Modder Support
uh, ok -
Here's my Block code: package com.gulgdev.mechanism.block.custom; import com.gulgdev.mechanism.block.ModBlocks; import net.minecraft.core.BlockPos; import net.minecraft.world.level.Level; import net.minecraft.world.level.block.BaseEntityBlock; import net.minecraft.world.level.block.entity.BlockEntity; import net.minecraft.world.level.block.entity.BlockEntityTicker; import net.minecraft.world.level.block.entity.BlockEntityType; import net.minecraft.world.level.block.state.BlockState; import javax.annotation.Nullable; public class Motor extends BaseEntityBlock { public Motor(Properties properties) { super(properties); } @Override public BlockEntity newBlockEntity(BlockPos pos, BlockState state) { return new MotorBlockEntity(pos, state); } @Nullable @Override public <T extends BlockEntity> BlockEntityTicker<T> getTicker(Level level, BlockState state, BlockEntityType<T> type) { return type == ModBlocks.MOTOR_BLOCK_ENTITY.get() ? (pLevel, pPos, pState, pBlockEntity) -> MotorBlockEntity.tick(pLevel, pPos, pState, pLevel.getBlockEntity(pPos)) : null; } } blockstates/motor.json: { "variants" : { "": { "model": "mechanism:block/motor" } } } models/block/motor.json: { "textures": { "0": "mechanism:block/motor_south", "1": "mechanism:block/motor_north", "2": "mechanism:block/motor_pipe", "3": "mechanism:block/motor_east", "4": "mechanism:block/motor_pipe_back", "5": "mechanism:block/motor_bottom", "6": "mechanism:block/motor_pipe_bottom", "particle": "mechanism:block/motor_south" }, "elements": [ { "from": [1, 3, 1], "to": [15, 14, 15], "faces": { "north": {"uv": [0, 0, 16, 16], "texture": "#1"}, "east": {"uv": [16, 0, 0, 16], "texture": "#3"}, "south": {"uv": [16, 0, 0, 16], "texture": "#0"}, "west": {"uv": [0, 0, 16, 16], "texture": "#5"}, "up": {"uv": [16, 16, 0, 0], "texture": "#5"}, "down": {"uv": [16, 0, 0, 16], "texture": "#5"} } }, { "from": [13.25, 1, 1], "to": [15, 3, 2.75], "faces": { "north": {"uv": [0, 0, 16, 16], "texture": "#2"}, "east": {"uv": [0, 0, 16, 16], "texture": "#4"}, "south": {"uv": [0, 0, 16, 16], "texture": "#4"}, "west": {"uv": [0, 0, 16, 16], "texture": "#4"}, "up": {"uv": [0, 0, 0.5, 0.5], "texture": "#missing"}, "down": {"uv": [0, 0, 16, 16], "texture": "#6"} } } ] } In inventory the block renders correct, but when I place it it's invisible.
-
ok, thank you
-
BlockState bs = level.getBlockState(pos); BaseEntityBlock beb = (BaseEntityBlock) bs.getBlock(); BlockEntity be = beb.newBlockEntity(to, bs); CompoundTag nbt_to = be.serializeNBT(); for (String key : nbt_from.getAllKeys()) { if (key != "id" && key != "x" && key != "y" && key != "z") { nbt_src.put(key, nbt.get(key)); } } be.deserializeNBT(nbt_to); nbt_from is nbt data which I want to copy to BE at position pos
-
How can I change block entity data at specified position?
-
Ok, thank you very much.
-
package com.gulgdev.mechanism.block.custom; import com.gulgdev.mechanism.block.ModBlocks; import net.minecraft.client.Minecraft; import net.minecraft.core.BlockPos; import net.minecraft.core.Direction; import net.minecraft.network.chat.TextComponent; import net.minecraft.world.entity.Entity; import net.minecraft.world.entity.Mob; import net.minecraft.world.item.ItemStack; import net.minecraft.world.level.Level; import net.minecraft.world.level.block.BaseEntityBlock; import net.minecraft.world.level.block.Block; import net.minecraft.world.level.block.ChestBlock; import net.minecraft.world.level.block.Blocks; import net.minecraft.world.level.block.entity.BlockEntity; import net.minecraft.world.level.block.state.BlockState; import net.minecraft.world.level.block.state.properties.BlockStateProperties; import net.minecraft.world.phys.AABB; import net.minecraft.world.phys.Vec3; import net.minecraftforge.items.ItemStackHandler; import oshi.util.tuples.Pair; import java.lang.reflect.InvocationTargetException; import java.util.*; import static java.lang.Math.abs; public class MotorBlockEntity extends BlockEntity { public static final int movingLimit = 8; public static final List<Block> nonMovable = Arrays.asList( Blocks.BEDROCK, Blocks.OBSIDIAN ); public final ItemStackHandler items; public MotorBlockEntity(BlockPos pos, BlockState state) { super(ModBlocks.MOTOR_BLOCK_ENTITY.get(), pos, state); items = new ItemStackHandler(4) { @Override public ItemStack extractItem(int slot, int amount, boolean simulate) { return super.extractItem(slot, amount, simulate); } @Override public ItemStack insertItem(int slot, ItemStack stack, boolean simulate) { return super.insertItem(slot, stack, simulate); } }; } public static <T extends BlockEntity> void tick(Level level, BlockPos pos, BlockState state, T be) { if (level.isClientSide()) return; new Thread(() -> { MotorBlockEntity entity = (MotorBlockEntity) be; Map<BlockPos, Pair<BlockState, BlockEntity>> blocks = FindStructureBlocks(level, pos, movingLimit); Map<BlockPos, BlockPos> moving = GetMovingMap(level, blocks, Direction.UP);//state.getValue(BlockStateProperties.FACING)); if (moving == null) return; MoveStructure(level, pos, movingLimit, blocks, moving, true); }).start(); } private static Map<BlockPos, Pair<BlockState, BlockEntity>> FindStructureBlocks(Level level, BlockPos start, int limit) { Map<BlockPos, Pair<BlockState, BlockEntity>> blocks = new HashMap<BlockPos, Pair<BlockState, BlockEntity>>(); blocks.put(start, new Pair<BlockState, BlockEntity>(level.getBlockState(start), level.getBlockEntity(start))); for (Direction direction : Direction.values()) { FindingBranch(level, blocks, start, start, direction, limit); } return blocks; } private static void FindingBranch(Level level, Map<BlockPos, Pair<BlockState, BlockEntity>> blocks, BlockPos center, BlockPos start, Direction direction, int limit) { BlockPos pos = start.relative(direction); if (!(level.getBlockState(pos).isAir() || nonMovable.contains(level.getBlockState(pos).getBlock()) || abs(center.getX() - pos.getX()) >= limit || abs(center.getY() - pos.getY()) >= limit || abs(center.getZ() - pos.getZ()) >= limit)) { try { Minecraft.getInstance().player.chat(level.getBlockEntity(pos).serializeNBT().toString()); } catch (NullPointerException e) { Minecraft.getInstance().player.chat("no nbt at " + pos.toString()); } blocks.put(pos, new Pair<BlockState, BlockEntity>(level.getBlockState(pos), level.getBlockEntity(pos))); for (Direction dir : Direction.values()) { if (!blocks.containsKey(pos.relative(dir))) { FindingBranch(level, blocks, center, pos, dir, limit); } } } } private static Map<BlockPos, BlockPos> GetMovingMap(Level level, Map<BlockPos, Pair<BlockState, BlockEntity>> blocks, Direction direction) { Map<BlockPos, BlockPos> moving = new HashMap<BlockPos, BlockPos>(); for (BlockPos position : blocks.keySet()) { BlockPos target = position.relative(direction); if (!level.getBlockState(target).isAir() && !blocks.containsKey(target)) return null; moving.put(position, target); } return moving; } private static void UpdateBlocks(Level level, BlockPos[] blocks) { for (BlockPos block : blocks) { BlockState state = level.getBlockState(block); if (state.getMaterial().isSolid()) level.sendBlockUpdated(block, state, state, 0); } for (BlockPos block : blocks) { BlockState state = level.getBlockState(block); if (!state.getMaterial().isSolid()) level.sendBlockUpdated(block, state, state, 0); } } private static void ClearStructure(Level level, Map<BlockPos, Pair<BlockState, BlockEntity>> blocks) { for (BlockPos pos : blocks.keySet()) { level.setBlock(pos, Blocks.AIR.defaultBlockState(), 0); } UpdateBlocks(level, blocks.keySet().toArray(new BlockPos[0])); } private static void MoveStructure(Level level, BlockPos center, int limit, Map<BlockPos, Pair<BlockState, BlockEntity>> blocks, Map<BlockPos, BlockPos> moving, boolean moveEntities) { ClearStructure(level, blocks); List<Entity> entities = null; if (moveEntities) { entities = level.getEntities(null, AABB.ofSize(new Vec3(center.getX(), center.getY(), center.getZ()), limit * 2 + 1, limit * 2 + 1, limit * 2 + 1)); } for (Map.Entry<BlockPos, Pair<BlockState, BlockEntity>> entry : blocks.entrySet()) { BlockPos from = entry.getKey(); BlockPos to = moving.get(from); BlockState blockState = entry.getValue().getA(); BlockEntity blockEntity = entry.getValue().getB(); if (moveEntities) { for (Entity entity : entities) { if (to.getX() == entity.getBlockX() && from.getY() + 1 == entity.getBlockY() && to.getZ() == entity.getBlockZ()) { entity.moveTo(new Vec3(to.getX(), to.getY() + 1, to.getZ())); } } } if (blockState.getMaterial().isSolid()) { level.setBlock(to, blockState, 0); try { BlockEntity be = blockEntity.getClass().getConstructor().newInstance(new Object[] { to, blockState }); be.deserializeNBT(blockEntity.serializeNBT()); level.setBlockEntity(be); } catch (InstantiationException | IllegalAccessException | InvocationTargetException | NoSuchMethodException | NullPointerException e) { e.printStackTrace(); } } } for (Map.Entry<BlockPos, Pair<BlockState, BlockEntity>> entry : blocks.entrySet()) { BlockPos from = entry.getKey(); BlockPos to = moving.get(from); BlockState blockState = entry.getValue().getA(); BlockEntity blockEntity = entry.getValue().getB(); if (!blockState.getMaterial().isSolid()) { level.setBlock(to, blockState, 0); try { BlockEntity be = blockEntity.getClass().getConstructor().newInstance(new Object[] { to, blockState }); Minecraft.getInstance().player.chat(blockEntity.serializeNBT().toString()); be.deserializeNBT(blockEntity.serializeNBT()); level.setBlockEntity(be); } catch (NullPointerException | InstantiationException | IllegalAccessException | InvocationTargetException | NoSuchMethodException e) { e.printStackTrace(); } } } UpdateBlocks(level, moving.values().toArray(new BlockPos[0])); } }
-
The position is loaded and block has BlockEntity (chest). But it returns null.
-
BlockEntity otherBE = level.getBlockEntity(blockEntityPos); otherBE always null.
-
It always returns null.
-
public static <T extends BlockEntity> void tick(Level level, BlockPos pos, BlockState state, T be) { if (level.isClientSide()) return; // I want to get other BE by position which I have in other variable (otherPos) }
-
But I need to get BE before. I have only position.