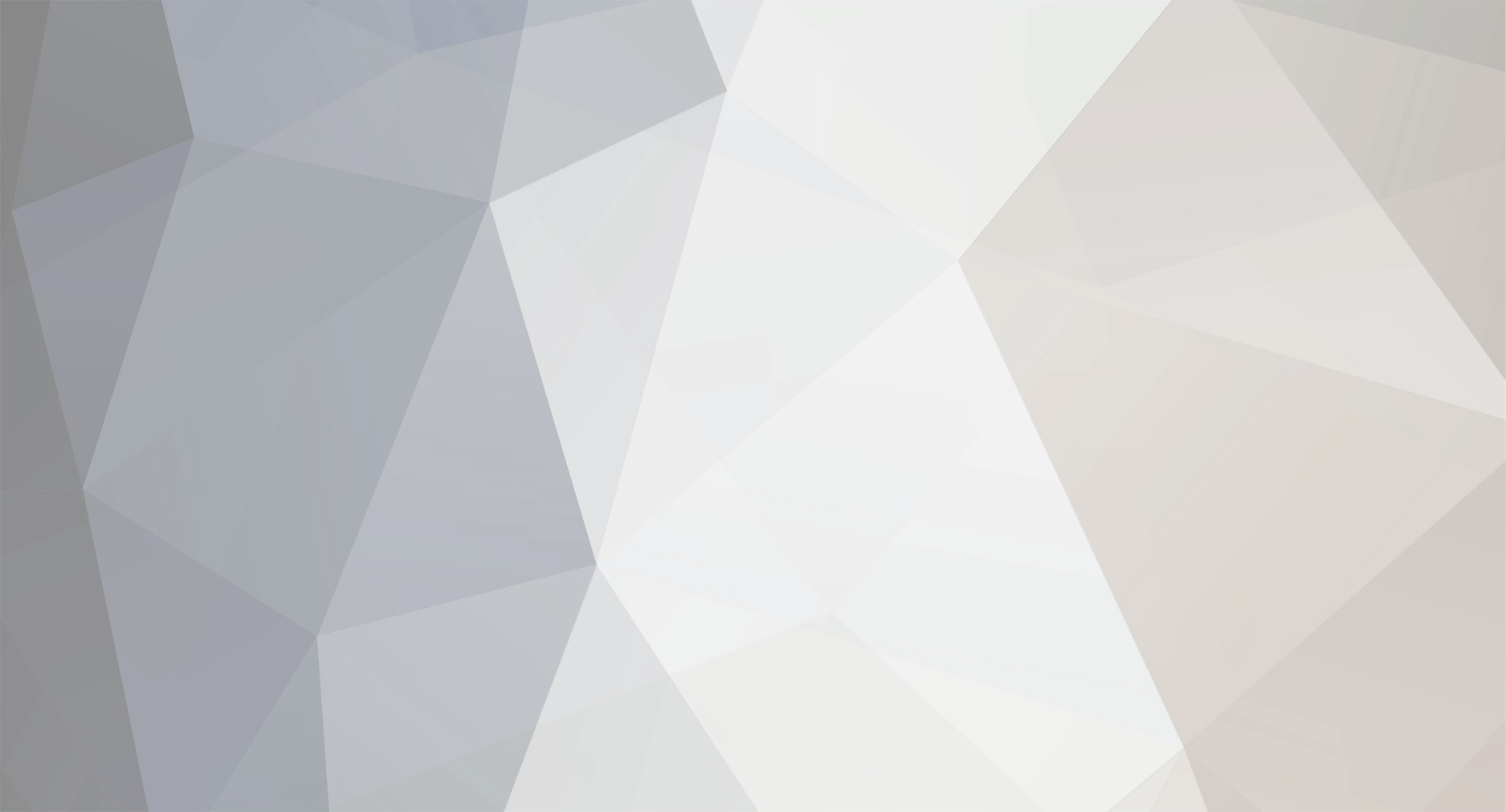
Hive Mind
Members-
Posts
5 -
Joined
-
Last visited
Recent Profile Visitors
The recent visitors block is disabled and is not being shown to other users.
Hive Mind's Achievements

Tree Puncher (2/8)
0
Reputation
-
I've been trying to create a sword with a special Riptide-like ability but I've ran into the problem of not being able to call the method that performs said ability, which is called "startObliterationAttack". The code where I'm trying to call the method from is in one "ObliteratorBlade" class and the abilities actual code is in another "SHPlayer" class, both shown below: ObliteratorBlade package net.hivethemodder.superheated.common.item; import net.minecraft.util.Mth; import net.minecraft.world.InteractionHand; import net.minecraft.world.InteractionResultHolder; import net.minecraft.world.entity.LivingEntity; import net.minecraft.world.entity.MoverType; import net.minecraft.world.entity.player.Player; import net.minecraft.world.item.*; import net.minecraft.world.level.Level; import net.minecraft.world.phys.Vec3; public class ObliteratorBlade extends SwordItem implements Vanishable { public ObliteratorBlade(Tier tier, int damage, float attackSpeed, Properties props) { super(tier, damage, attackSpeed, props); } @Override public UseAnim getUseAnimation(ItemStack itemStack) { return UseAnim.BOW; } @Override public int getUseDuration(ItemStack itemStack) { return 72000; } @Override public void releaseUsing(ItemStack itemStack, Level level, LivingEntity livingEntity, int p_41415_) { if (livingEntity instanceof Player player) { int i = this.getUseDuration(itemStack) - p_41415_; if (i >= 10) { float playerRotX = player.getXRot(); float playerRotY = player.getYRot(); float VecX = -Mth.sin(playerRotY * ((float)Math.PI / 180f)) * Mth.cos(playerRotX * ((float)Math.PI / 180f)); float VecY = -Mth.sin(playerRotX * ((float)Math.PI / 180f)); float VecZ = Mth.cos(playerRotY * ((float)Math.PI / 180f)) * Mth.cos(playerRotX * ((float)Math.PI / 180f)); float modifier = Mth.sqrt(VecX * VecX + VecY * VecY + VecZ * VecZ); float force = 1.5f; VecX *= force / modifier; VecY *= force / modifier; VecZ *= force / modifier; player.push(VecX, VecY, VecZ); //Where I want to call the method startObliterationAttack() if (player.isOnGround()) { player.move(MoverType.SELF, new Vec3(0.0d, 1.1999999d, 0.0d)); } } } } @Override public InteractionResultHolder<ItemStack> use(Level level, Player player, InteractionHand hand) { ItemStack itemStack = player.getItemInHand(hand); player.startUsingItem(hand); return InteractionResultHolder.consume(itemStack); } } SHPlayer package net.hivethemodder.superheated.client; import com.mojang.authlib.GameProfile; import net.minecraft.core.BlockPos; import net.minecraft.world.entity.Entity; import net.minecraft.world.entity.LivingEntity; import net.minecraft.world.entity.player.Player; import net.minecraft.world.entity.player.ProfilePublicKey; import net.minecraft.world.level.Explosion; import net.minecraft.world.level.Level; import net.minecraft.world.phys.AABB; import org.jetbrains.annotations.Nullable; import java.util.List; public abstract class SHPlayer extends Player { public int obliterationAttackTicks = 0; public SHPlayer(Level level, BlockPos blockPos, float p_219729_, GameProfile profile, @Nullable ProfilePublicKey publicKey) { super(level, blockPos, p_219729_, profile, publicKey); } //The method I want to call public void startObliterationAttack(int time) { this.autoSpinAttackTicks = time; if (!this.level.isClientSide) { this.removeEntitiesOnShoulder(); } } @Override public void aiStep() { AABB aabb = this.getBoundingBox(); if (obliterationAttackTicks > 0) { obliterationAttackTicks--; this.checkObliterationAttack(aabb, this.getBoundingBox()); } } protected void checkObliterationAttack(AABB aabb1, AABB aabb2) { AABB aabb = aabb1.minmax(aabb2); List<Entity> list = this.level.getEntities(this, aabb); if (!list.isEmpty()) { for (Entity entity : list) { if (entity instanceof LivingEntity) { this.doAutoAttackOnTouch((LivingEntity) entity); Explosion.BlockInteraction interaction = Explosion.BlockInteraction.NONE; level.explode(entity, this.getX(), this.getY(), this.getZ(), 3f, interaction); this.obliterationAttackTicks = 0; this.setDeltaMovement(this.getDeltaMovement().scale(-0.2d)); break; } } } else if (this.horizontalCollision) { this.obliterationAttackTicks = 0; } } } What I've already tried: 1. Import SHPlayer as a variable and then call the method: SHPlayer shPlayer; shPlayer.startObliterationAttack(time); However this produces a NullPointerException when I try to use the ability in-game. 2. Cast Player into SHPlayer and then call the method: SHPlayer shPlayer = (SHPlayer)livingEntity; shPlayer.startObliterationAttack(time); Doing this generates a "java.lang.ClassCastException" error when I try to use the ability. Any help would be greatly appreciated!
-
I've made a block with custom shapes and hitbox using VoxelShapes and when texting it in-game two problems became apparent. First of the lightning didn't work correctly as shown below: Light glitch And some wierd problem with the hitbox. The hitbox works fine except when you try to destroy or interact with the upper half of the block: Hitbox problem Below is the blocks class: package com.hivemind.superheated.block; import net.minecraft.core.BlockPos; import net.minecraft.core.Direction; import net.minecraft.world.item.context.BlockPlaceContext; import net.minecraft.world.level.BlockGetter; import net.minecraft.world.level.LevelAccessor; import net.minecraft.world.level.block.Block; import net.minecraft.world.level.block.HorizontalDirectionalBlock; import net.minecraft.world.level.block.Mirror; import net.minecraft.world.level.block.Rotation; import net.minecraft.world.level.block.state.BlockState; import net.minecraft.world.level.block.state.StateDefinition.Builder; import net.minecraft.world.level.block.state.properties.DirectionProperty; import net.minecraft.world.phys.shapes.BooleanOp; import net.minecraft.world.phys.shapes.CollisionContext; import net.minecraft.world.phys.shapes.Shapes; import net.minecraft.world.phys.shapes.VoxelShape; public class Forge extends Block{ public static final DirectionProperty FACING = HorizontalDirectionalBlock.FACING; @Override public VoxelShape getShape( BlockState state, BlockGetter getter, BlockPos pos, CollisionContext context) { switch (state.getValue(FACING)) { case EAST: VoxelShape EAST = Shapes.empty(); EAST = Shapes.join(EAST, Shapes.box(0, 0, 0, 1, 0.0625, 1), BooleanOp.OR); EAST = Shapes.join(EAST, Shapes.box(0.25, 0.3125, 0.75, 0.875, 0.875, 0.9375), BooleanOp.OR); EAST = Shapes.join(EAST, Shapes.box(0.25, 0.3125, 0.0625, 0.875, 0.875, 0.25), BooleanOp.OR); EAST = Shapes.join(EAST, Shapes.box(0.25, 0.875, 0.0625, 0.875, 1, 0.9375), BooleanOp.OR); EAST = Shapes.join(EAST, Shapes.box(0.25, 0.0625, 0.0625, 0.875, 0.3125, 0.9375), BooleanOp.OR); EAST = Shapes.join(EAST, Shapes.box(0.0625, 0.0625, 0.0625, 0.25, 1, 0.9375), BooleanOp.OR); EAST = Shapes.join(EAST, Shapes.box(0, 1, 0, 0.9375, 1.125, 1), BooleanOp.OR); EAST = Shapes.join(EAST, Shapes.box(0.0625, 1.125, 0.0625, 0.875, 1.375, 0.9375), BooleanOp.OR); EAST = Shapes.join(EAST, Shapes.box(0.0625, 1.375, 0.1875, 0.75, 1.625, 0.8125), BooleanOp.OR); EAST = Shapes.join(EAST, Shapes.box(0.0625, 1.625, 0.3125, 0.625, 1.875, 0.6875), BooleanOp.OR); EAST = Shapes.join(EAST, Shapes.box(0, 0.0625, 0.375, 0.0625, 1, 0.625), BooleanOp.OR); EAST = Shapes.join(EAST, Shapes.box(0, 1.125, 0.375, 0.0625, 1.875, 0.625), BooleanOp.OR); EAST = Shapes.join(EAST, Shapes.box(0, 1.875, 0.375, 0.5, 2, 0.625), BooleanOp.OR); return EAST; case SOUTH: VoxelShape SOUTH =Shapes.empty(); SOUTH =Shapes.join(SOUTH,Shapes.box(0, 0, 0, 1, 0.0625, 1), BooleanOp.OR); SOUTH =Shapes.join(SOUTH,Shapes.box(0.0625, 0.3125, 0.25, 0.25, 0.875, 0.875), BooleanOp.OR); SOUTH =Shapes.join(SOUTH,Shapes.box(0.75, 0.3125, 0.25, 0.9375, 0.875, 0.875), BooleanOp.OR); SOUTH =Shapes.join(SOUTH,Shapes.box(0.0625, 0.875, 0.25, 0.9375, 1, 0.875), BooleanOp.OR); SOUTH =Shapes.join(SOUTH,Shapes.box(0.0625, 0.0625, 0.25, 0.9375, 0.3125, 0.875), BooleanOp.OR); SOUTH =Shapes.join(SOUTH,Shapes.box(0.0625, 0.0625, 0.0625, 0.9375, 1, 0.25), BooleanOp.OR); SOUTH =Shapes.join(SOUTH,Shapes.box(0, 1, 0, 1, 1.125, 0.9375), BooleanOp.OR); SOUTH =Shapes.join(SOUTH,Shapes.box(0.0625, 1.125, 0.0625, 0.9375, 1.375, 0.875), BooleanOp.OR); SOUTH =Shapes.join(SOUTH,Shapes.box(0.1875, 1.375, 0.0625, 0.8125, 1.625, 0.75), BooleanOp.OR); SOUTH =Shapes.join(SOUTH,Shapes.box(0.3125, 1.625, 0.0625, 0.6875, 1.875, 0.625), BooleanOp.OR); SOUTH =Shapes.join(SOUTH,Shapes.box(0.375, 0.0625, 0, 0.625, 1, 0.0625), BooleanOp.OR); SOUTH =Shapes.join(SOUTH,Shapes.box(0.375, 1.125, 0, 0.625, 1.875, 0.0625), BooleanOp.OR); SOUTH =Shapes.join(SOUTH,Shapes.box(0.375, 1.875, 0, 0.625, 2, 0.5), BooleanOp.OR); return SOUTH; case WEST: VoxelShape WEST = Shapes.empty(); WEST = Shapes.join(WEST, Shapes.box(0, 0, 0, 1, 0.0625, 1), BooleanOp.OR); WEST = Shapes.join(WEST, Shapes.box(0.125, 0.3125, 0.0625, 0.75, 0.875, 0.25), BooleanOp.OR); WEST = Shapes.join(WEST, Shapes.box(0.125, 0.3125, 0.75, 0.75, 0.875, 0.9375), BooleanOp.OR); WEST = Shapes.join(WEST, Shapes.box(0.125, 0.875, 0.0625, 0.75, 1, 0.9375), BooleanOp.OR); WEST = Shapes.join(WEST, Shapes.box(0.125, 0.0625, 0.0625, 0.75, 0.3125, 0.9375), BooleanOp.OR); WEST = Shapes.join(WEST, Shapes.box(0.75, 0.0625, 0.0625, 0.9375, 1, 0.9375), BooleanOp.OR); WEST = Shapes.join(WEST, Shapes.box(0.0625, 1, 0, 1, 1.125, 1), BooleanOp.OR); WEST = Shapes.join(WEST, Shapes.box(0.125, 1.125, 0.0625, 0.9375, 1.375, 0.9375), BooleanOp.OR); WEST = Shapes.join(WEST, Shapes.box(0.25, 1.375, 0.1875, 0.9375, 1.625, 0.8125), BooleanOp.OR); WEST = Shapes.join(WEST, Shapes.box(0.375, 1.625, 0.3125, 0.9375, 1.875, 0.6875), BooleanOp.OR); WEST = Shapes.join(WEST, Shapes.box(0.9375, 0.0625, 0.375, 1, 1, 0.625), BooleanOp.OR); WEST = Shapes.join(WEST, Shapes.box(0.9375, 1.125, 0.375, 1, 1.875, 0.625), BooleanOp.OR); WEST = Shapes.join(WEST, Shapes.box(0.5, 1.875, 0.375, 1, 2, 0.625), BooleanOp.OR); return WEST; default: VoxelShape NORTH =Shapes.empty(); NORTH =Shapes.join(NORTH,Shapes.box(0, 0, 0, 1, 0.0625, 1), BooleanOp.OR); NORTH =Shapes.join(NORTH,Shapes.box(0.75, 0.3125, 0.125, 0.9375, 0.875, 0.75), BooleanOp.OR); NORTH =Shapes.join(NORTH,Shapes.box(0.0625, 0.3125, 0.125, 0.25, 0.875, 0.75), BooleanOp.OR); NORTH =Shapes.join(NORTH,Shapes.box(0.0625, 0.875, 0.125, 0.9375, 1, 0.75), BooleanOp.OR); NORTH =Shapes.join(NORTH,Shapes.box(0.0625, 0.0625, 0.125, 0.9375, 0.3125, 0.75), BooleanOp.OR); NORTH =Shapes.join(NORTH,Shapes.box(0.0625, 0.0625, 0.75, 0.9375, 1, 0.9375), BooleanOp.OR); NORTH =Shapes.join(NORTH,Shapes.box(0, 1, 0.0625, 1, 1.125, 1), BooleanOp.OR); NORTH =Shapes.join(NORTH,Shapes.box(0.0625, 1.125, 0.125, 0.9375, 1.375, 0.9375), BooleanOp.OR); NORTH =Shapes.join(NORTH,Shapes.box(0.1875, 1.375, 0.25, 0.8125, 1.625, 0.9375), BooleanOp.OR); NORTH =Shapes.join(NORTH,Shapes.box(0.3125, 1.625, 0.375, 0.6875, 1.875, 0.9375), BooleanOp.OR); NORTH =Shapes.join(NORTH,Shapes.box(0.375, 0.0625, 0.9375, 0.625, 1, 1), BooleanOp.OR); NORTH =Shapes.join(NORTH,Shapes.box(0.375, 1.125, 0.9375, 0.625, 1.875, 1), BooleanOp.OR); NORTH =Shapes.join(NORTH,Shapes.box(0.375, 1.875, 0.5, 0.625, 2, 1), BooleanOp.OR); return NORTH; } } public Forge( Properties properties) { super(properties); this.registerDefaultState(this.defaultBlockState().setValue(FACING, Direction.NORTH)); } @Override protected void createBlockStateDefinition( Builder<Block, BlockState> builder) { builder.add(FACING); super.createBlockStateDefinition( builder); } @Override public BlockState getStateForPlacement(BlockPlaceContext context) { return this.defaultBlockState().setValue(FACING, context.getHorizontalDirection().getOpposite()); } @SuppressWarnings("deprecation") @Override public BlockState mirror(BlockState state, Mirror mirror) { return state.rotate(mirror.getRotation(state.getValue(FACING))); } @Override public BlockState rotate(BlockState state, LevelAccessor world, BlockPos pos, Rotation direction) { return state.setValue(FACING, direction.rotate(state.getValue(FACING))); } } If you need anything else to find the problem just ask.
-
To clarify, any json file assigning the tags to blocks and items aswell as any recipes using the tags returns the error I mentioned.
-
I've been trying to create custom tags for items in the mod I am making so any future recipes I add can use items with ore dictonary tags instead of specific items, but I've run into a problem. I already have a recipe that uses oredict tags rather than items but when I try to load into a world to test if it works the game says: "Errors in currently selected datapacks prevented the world from loading. You can either try to load it with only the vanilla datapack ("safe mode"), or go to the title screen and fix it manually." It seems as, despite the TagInit class being free of errors, the tags I have created doesn't exist (?). I've been following a 1.18.1 modding tutorial that explained how to create custom tags, but I am making a mod in 1.18.2 and I've heard that tags went through some changes between those versions and I guess the problems lay there. I've triple-checked that I got the namespaces and tag names right. Below is the TagInit class, two of the tag json files and the recipe that uses said tags. TagInit class: package com.hivemind.superheated.init; import com.hivemind.superheated.Superheated; import net.minecraft.resources.ResourceLocation; import net.minecraft.tags.BlockTags; import net.minecraft.tags.ItemTags; import net.minecraft.world.item.Item; import net.minecraft.world.level.block.Block; import net.minecraft.tags.TagKey; public final class TagInit { public static final class Blocks { public static final TagKey<Block> BLOCK_CRUDE_STEEL = mod("blockCrudeSteel"); private static TagKey<Block> mod(String path) { return BlockTags.create(new ResourceLocation(Superheated.MOD_ID, path)); } } public static final class Items { public static final TagKey<Item> INGOT_CRUDE_STEEL = mod("ingotCrudeSteel"); public static final TagKey<Item> BLOCK_CRUDE_STEEL = mod("blockCrudeSteel"); public static final TagKey<Item> PLATE_IRON = mod("plateIron"); public static final TagKey<Item> LIGHT_PLATE_IRON = mod("lightPlateIron"); private static TagKey<Item> mod(String path) { return ItemTags.create(new ResourceLocation(Superheated.MOD_ID, path)); } } } The custom tags and thier names: blockCrudeSteel.json { "replace": false, "values": [ { "id": "superheated:block_crude_steel" } ] } plateIron.json { "replace": false, "values": [ { "id": "superheated:plate_iron" } ] } The recipe: { "type": "minecraft:crafting_shaped", "pattern": [ "AAA", "BCB", "DDD" ], "key": { "A": { "tag": "superheated:blockCrudeSteel" }, "B": { "tag": "superheated:plateIron" }, "C": { "item": "minecraft:anvil" }, "D": { "item": "minecraft:polished_deepslate" } }, "result": { "count": 1, "item": "superheated:forging_anvil" } }
-
I've been trying to make an infinite fuel item by returning said item after it has been used as fuel the same way a Lava Bucket returns an empty Bucket after it has been used. I am aware of the methods getContainerItem, hasContainerItem and hasCraftingRemainingItem but I don't know which one to use (if it even is one of them) nor do I know how they work. Can anyone help me get this to work? Below is the items current code. package com.hivemind.superheated.item; import net.minecraft.world.item.Item; import net.minecraft.world.item.ItemStack; import net.minecraft.world.item.crafting.RecipeType; public class BlazingCore extends Item { public BlazingCore(Properties properties) { super(properties); } @Override public int getBurnTime(ItemStack itemStack, RecipeType<?> recipeType) { return 400; } }