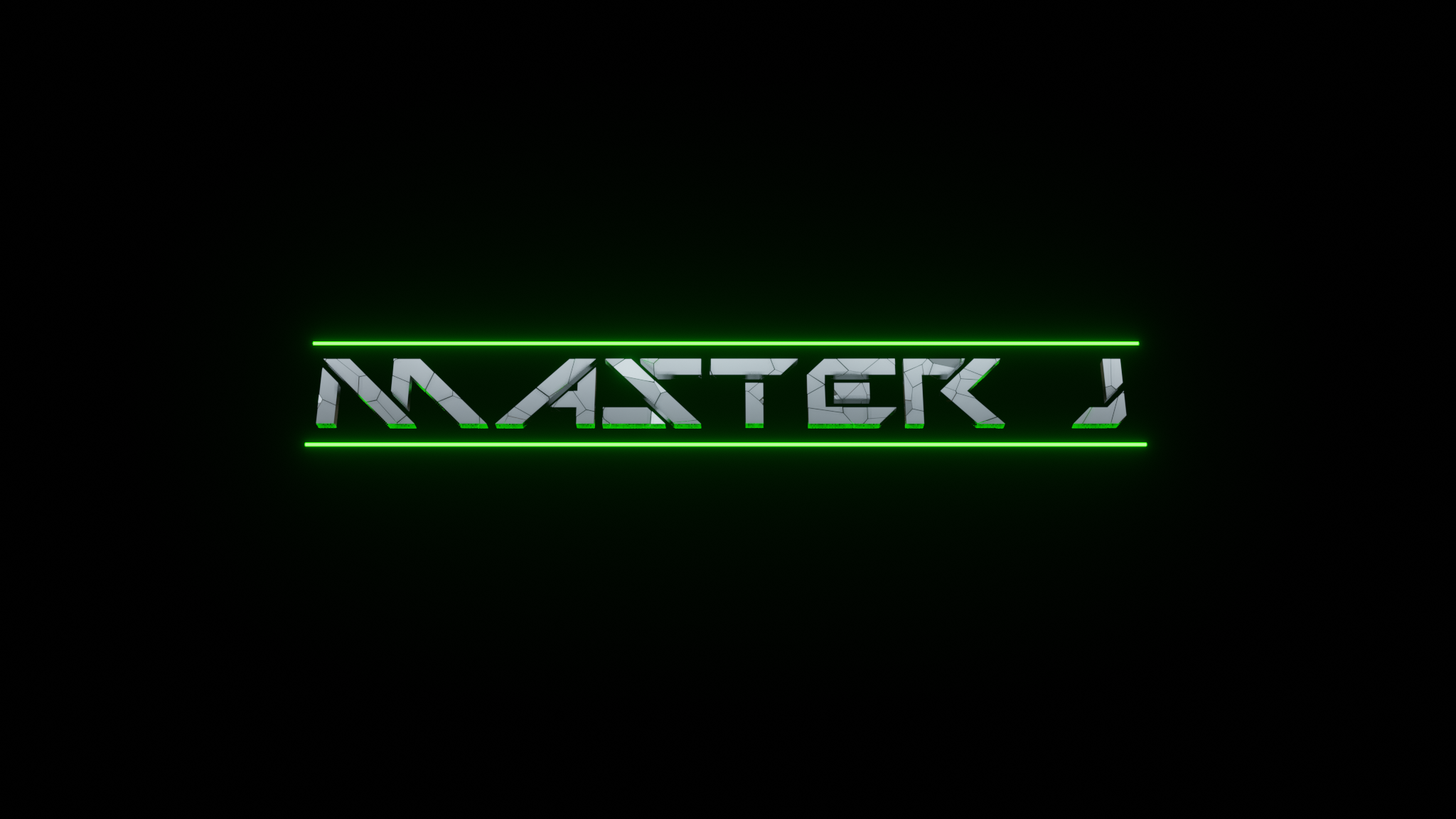
Master_J
Members-
Posts
16 -
Joined
-
Last visited
Everything posted by Master_J
-
I have looked at some other mods, and discovered that they store booleans for movement as entitydata and reference it in the tick method. After implementing this and extending the entity class instead of the boat class, I no longer need any network packets to control the movement. Everything is now working, except the rocket keeps teleporting back to the ground midflight like this: https://www.youtube.com/watch?v=HFX9ZzRyFQQ . https://github.com/MasterJ-06/Neutron_Galaxy/blob/master/src/main/java/neutrongalaxy/masterj/neutrongalaxy/events/ClientEvents.java https://github.com/MasterJ-06/Neutron_Galaxy/blob/master/src/main/java/neutrongalaxy/masterj/neutrongalaxy/entities/RocketEntity.java
-
I thought the problem was only related to those functions. I did read that post, which was why I ran the code to move the rocket up on the client as the player is a passenger, and the code to move it down on the server because it would have no passengers. Here is my Entity class: https://github.com/MasterJ-06/Neutron_Galaxy/blob/master/src/main/java/neutrongalaxy/masterj/neutrongalaxy/entities/RocketEntity.java But I call the methods to control the movement here in the client events for a key press: https://github.com/MasterJ-06/Neutron_Galaxy/blob/master/src/main/java/neutrongalaxy/masterj/neutrongalaxy/events/ClientEvents.java https://github.com/MasterJ-06/Neutron_Galaxy/blob/master/src/main/java/neutrongalaxy/masterj/neutrongalaxy/networking/packet/MoveRocketDownC2SPacket.java
-
If I run the two packets on the client side, then moving up works but moving down does not. If I run them on the server side moving up doesn't work but after a few minutes it sometimes works and moving down works. If I run the moving down packet on the server and the moving up packet on the client, nothing works. My entity needs to move up when it has a passenger and move down when there is no passenger.
-
I am trying to make an entity move up, but it only moves up a quarter of a block and then stops. I have one packet to move it up when a key is pressed, and another packet to move it back down if there is no player riding it. This is my code to make it move up: public static class ClientForgeEvents { @SubscribeEvent public static void onKeyInput(InputEvent.Key event) { if (KeyBinding.LAUNCH_KEY.consumeClick()) { assert Minecraft.getInstance().player != null; if (Minecraft.getInstance().player.getRootVehicle() instanceof RocketEntity) { launch = true; ModPackets.sendToServer(new MoveRocketC2SPacket()); } } } @SubscribeEvent public static void ticks(TickEvent.PlayerTickEvent event) { if (Minecraft.getInstance().player != null) { if (Minecraft.getInstance().player.getRootVehicle() instanceof RocketEntity) { if (launch) { ModPackets.sendToServer(new MoveRocketC2SPacket()); } } else { launch = false; ModPackets.sendToServer(new MoveRocketDownC2SPacket()); } } } } And this is the handle method for the two packets public boolean handle(Supplier<NetworkEvent.Context> supplier) { NetworkEvent.Context ctx = supplier.get(); ctx.enqueueWork(() -> { // Here we are on the server ServerPlayer player = ctx.getSender(); ServerLevel level = player.getLevel(); if (player.getRootVehicle().getY() <= 151) { player.getRootVehicle().setDeltaMovement(player.getRootVehicle().getDeltaMovement().add(0.0D, 0.05D, 0.0D)); player.getRootVehicle().move(MoverType.SELF, player.getRootVehicle().getDeltaMovement()); } else if (player.getRootVehicle().getY() >= 151) { player.getRootVehicle().setDeltaMovement(Vec3.ZERO); player.getRootVehicle().move(MoverType.SELF, player.getRootVehicle().getDeltaMovement()); ModPackets.sendToPlayer(new RequestDestPlanetS2CPacket(), player); } }); return true; } public boolean handle(Supplier<NetworkEvent.Context> supplier) { NetworkEvent.Context ctx = supplier.get(); ctx.enqueueWork(() -> { // Here we are on the server ServerPlayer player = ctx.getSender(); ServerLevel level = player.getLevel(); level.getAllEntities().forEach(x -> { if (x instanceof RocketEntity) { if (!(x.getFirstPassenger() instanceof ServerPlayer)) { x.setDeltaMovement(x.getDeltaMovement().normalize().add(0.0D, -0.14D, 0.0D)); x.move(MoverType.SELF, x.getDeltaMovement()); } } }); }); return true; } I have found that the Delta movement changes correctly, but it does not make the rocket move up.
-
I did some testing, and discovered that this.getLootTable() always equals null. Upon looking at the chest boat class, it does not seem to ever assign a value to it. I also discovered that the output of this.getItemStacks() was [1 air, 1 air, 1 air, 1 air, 1 air, 1 air, 1 air, 1 air, 1 air]
-
I don't set the loottable field to null at all.
-
I have added an inventory menu to my custom entity which is based off the chest boat class, and the container entity interface, but when I put an item in its inventory and close and reopen then inventory menu, the items disappear. I suspect it has somethings to do with the two additional save data methods, but I can't seem to fix it. @Nullable private ResourceLocation lootTable; private long lootTableSeed; @Override @Nullable public ResourceLocation getLootTable() { return this.lootTable; } @Override public void setLootTable(@Nullable ResourceLocation pLootTable) { this.lootTable = pLootTable; } @Override public long getLootTableSeed() { return this.lootTableSeed; } @Override public void setLootTableSeed(long pLootTableSeed) { this.lootTableSeed = pLootTableSeed; } @Override protected void readAdditionalSaveData(CompoundTag pCompound) { if (pCompound.contains("Type", 0)) { this.setType(RocketEntity.Type.byName(pCompound.getString("Type"))); } this.clearItemStacks(); if (pCompound.contains("LootTable", 0)) { this.setLootTable(new ResourceLocation(pCompound.getString("LootTable"))); this.setLootTableSeed(pCompound.getLong("LootTableSeed")); } else { ContainerHelper.loadAllItems(pCompound, this.getItemStacks()); } } @Override protected void addAdditionalSaveData(CompoundTag pCompound) { pCompound.putString("Type", this.getRocketType().getName()); if (this.getLootTable() != null) { pCompound.putString("LootTable", this.getLootTable().toString()); if (this.getLootTableSeed() != 0L) { pCompound.putLong("LootTableSeed", this.getLootTableSeed()); } } else { ContainerHelper.saveAllItems(pCompound, this.getItemStacks()); } }
-
I had a look at their code using sponge powered mixins, and used sponge powered's mixins to supress all the experimental warnings including the warning when you create a new world, which that mod does not do. Thanks for the help.
-
I used multiple biomes by setting the type to minecraft:multi_noise. The dimension is working fine now, but I was wondering if there is a way to supress this warning when creating a new world: Warning! These settings are using experimental features. These settings are experimental and could one day stop working. Do you wish to proceed?
-
I had a look at the forge repo, but when I switched the branch to 1.19.2, there was no dimension data generator, but I did find this comment: /* TODO: Make this a data gen: data/dimension_settings_test/dimension/test_overworld.json { "#comment": [ "This is an example Dimension showing how it can be configured to use a custom", "DimensionSettings that possess similar generation settings to overworld but", "has independently configurable structure settings.", "", "See the 'settings' field below" ], "type": "minecraft:overworld", "generator": { "type": "minecraft:noise", "seed": 12345, "settings": "dimension_settings_test:test_overworld", "biome_source": { "preset": "minecraft:overworld", "type": "minecraft:multi_noise" } } } */ I would also like to know how to use multiple biomes in this dimension json file like the overworld, instead of making everything the plains biome: { "type": "neutrongalaxy:ng_moon", "generator": { "type": "minecraft:noise", "seed": -485105919, "settings": "minecraft:overworld", "biome_source": { "type": "minecraft:fixed", "biome": "minecraft:plains" } } }
-
Hi, I have managed to create dimensions using public static final ResourceKey<Level> NG_MOON = ResourceKey.create(Registry.DIMENSION_REGISTRY, new ResourceLocation(NeutronGalaxy.MODID, "ng_moon")); public static final ResourceKey<DimensionType> NG_MOON_TYPE = ResourceKey.create(Registry.DIMENSION_TYPE_REGISTRY, new ResourceLocation(NeutronGalaxy.MODID, "ng_moon")); and these two json files { "type": "neutrongalaxy:ng_moon", "generator": { "type": "minecraft:noise", "seed": -485105919, "settings": "minecraft:overworld", "biome_source": { "type": "minecraft:fixed", "biome": "minecraft:plains" } } } { "ultrawarm": false, "natural": true, "piglin_safe": false, "respawn_anchor_works": false, "bed_works": false, "has_raids": true, "has_skylight": true, "has_ceiling": false, "coordinate_scale": 1, "ambient_light": 0, "logical_height": 384, "effects": "minecraft:overworld", "infiniburn": "#minecraft:infiniburn_overworld", "min_y": -64, "height": 384, "monster_spawn_light_level": { "type": "minecraft:uniform", "value": { "min_inclusive": 0, "max_inclusive": 7 } }, "monster_spawn_block_light_limit": 0 } But I would like to know if it is possible to create the dimensions using code instead of json files, or generate the json files like the loot table and recipe data generators.
-
Thanks for explaining how players and entities are controlled, after looking at the Pig class and how it determined if the player was controlling it, I modified the getControllingPassenger method to make my entity unable to be controlled be the player so I can control it from the server, which fixed the issue. These are the modified methods from the Pig class that I used: @Nullable public Entity getControllingPassenger() { Entity entity = this.getFirstPassenger(); return entity != null && this.canBeControlledBy(entity) ? entity : null; } private boolean canBeControlledBy(Entity p_218248_) { return false; }
-
I am now sending a packet every tick, and updated the handle to make sure that the player is a passenger of my entity, but even when both of my if statements are true it seems to take a random amount of time from 1 to 30+ seconds to start moving my entity. The updated handle: public boolean handle(Supplier<NetworkEvent.Context> supplier) { NetworkEvent.Context ctx = supplier.get(); ctx.enqueueWork(() -> { // Here we are on the server ServerPlayer player = ctx.getSender(); if (player.getRootVehicle() instanceof RocketEntity) { if (player.getRootVehicle().getY() <= 91) { player.getRootVehicle().setDeltaMovement(player.getRootVehicle().getDeltaMovement().add(0.0D, 0.05D, 0.0D)); } } }); return true; }
-
After applying the logic to the server instead of the client via network packets, everything works except I cannot seem to create a loop to continually check if the entity is above 91 blocks using a while loop, because it freezes the game. At the moment it only runs once every time the key is pressed. Any help would be appreciated. This is my packet handler, and the packets are sent every time a key is pressed: public boolean handle(Supplier<NetworkEvent.Context> supplier) { NetworkEvent.Context ctx = supplier.get(); ctx.enqueueWork(() -> { // This is the server ServerPlayer player = ctx.getSender(); if (player.getRootVehicle().getY() < 91) { player.getRootVehicle().setDeltaMovement(player.getRootVehicle().getDeltaMovement().add(0.0D, 1.0D, 0.0D)); } }); return true; }
-
I have this if statement to check if the boolean launch = true, and a player is riding my entity every tick, and if both requirements are met then it moves the entity upwards. This works flawlessly if there is only one instance of the entity in the world. If I summon another instance of my entity, then this.getPassengers().get(0) returns an Array Index Out Of Bounds Exception for both entities. if (ClientEvents.launch) { try { // This stops code from running until a passenger is detected Entity getPassenger = this.getPassengers().get(0); if (this.getY() < 91) { this.setDeltaMovement(this.getDeltaMovement().add(0.0D, 0.001D, 0.0D)); } else { System.out.println("above 90 blocks"); ClientEvents.launch = false; } } catch (ArrayIndexOutOfBoundsException e) { ClientEvents.launch = false; System.out.println("No passenger so not moving up"); } } I have tried a few other ways to get the passenger, but they all have the same issue. Most of the code for my entity is based on parts of the Boat class if that helps.