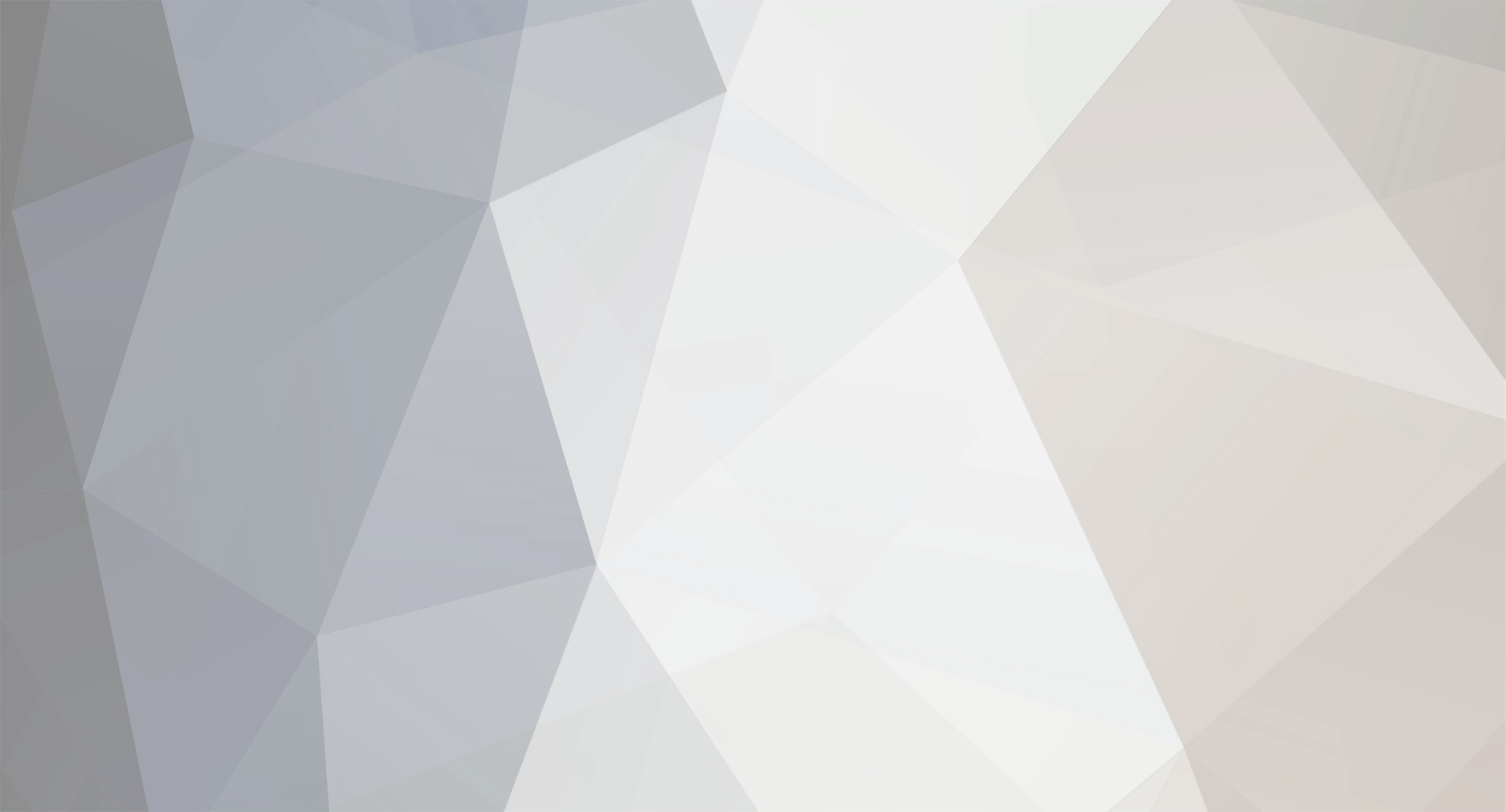
Alron
Forge Modder-
Posts
25 -
Joined
-
Last visited
Converted
-
Gender
Undisclosed
-
Personal Text
I am new!
Alron's Achievements

Tree Puncher (2/8)
2
Reputation
-
Thanks. I'll see if I can find out how the .tnc file is structured. A shame though that the old minecraft models no longer work.
-
Alright, I might take a look at that. Any pointers on where to start learning about making a custom model loader?
-
So there is still no way to render items in the inventory through a TESR or something similar? Do all models of items now have to be done via json files or obj files etc?
-
[1.7.10]problem with server won'y run , infinity 2.3.0
Alron replied to liliexx's topic in Support & Bug Reports
Since you are running FTB Infinity, you should propably go to the FTB forums http://forum.feed-the-beast.com/forum/tech-support.210/. I doubt that people here can help you. -
Hi, Is there a way to register an Item with my own ISmartModel, so that it shows up in my inventory using that model? As of now I hve only seen how to do it with a ModelResourceLocation. Or alternatively a way to render the Item with a TileEntitySpecialRenderer. I would hate to convert all my Techne models to json models if there is a way to avoid that.
-
Thanks. Will try to make it work without.
-
Hi I want to use a couple APIs for the mod I'm currently making, so I followed a tutorial (https://raw.githubusercontent.com/coolAlias/Forge_Tutorials/master/ModdingWithAPIs.java) on how to use them. Loaded the Buildcraft api in and minecraft runs nicely. Now the problem is that I want the mod to work even without any other mods installed, so I use the @Optional annotations: @Optional.InterfaceList(value={ @Optional.Interface(iface="buildcraft.api.power.IPowerReceptor", modid="BuildCraft|Energy", striprefs=true) }) public class TileEntityPowerReceptor extends TileEntity implements IPowerReceptor{ //is that gonna work without bc? public PowerHandler BCPowerHandler = new PowerHandler(this, PowerHandler.Type.MACHINE); private int power; public TileEntityPowerReceptor(){ } @Method(modid="BuildCraft|Energy") @Override public PowerReceiver getPowerReceiver(ForgeDirection side) { return BCPowerHandler.getPowerReceiver(); } @Method(modid="BuildCraft|Energy") @Override public void doWork(PowerHandler workProvider) { // TODO Auto-generated method stub } @Method(modid="BuildCraft|Energy") @Override public World getWorld() { return worldObj; } public int getPower() { return power; } public void setPower(int power) { this.power = power; } } I don't think that will work though since it doesn't know what a PowerHandler object is without BC. Can I strip that as well somehow?
-
[Solved][1.7.10] Rendering a cube with scale not working
Alron replied to Alron's topic in Modder Support
Hi yeah it just occured to me that there really was no reason not to do it in glScalef. So I moved it there and it works fine now, thanks! -
[Solved][1.7.10] Rendering a cube with scale not working
Alron replied to Alron's topic in Modder Support
Thanks for the suggestion! Unfortunately that method doesn't seem to exist in Render (which is the parent of the RenderShot) . The shot is also extends EntityFireball and not EntityLivingBase (should have mentioned that in OP). The -1 is because this shot is fired from a cannon, the model for which I made in Techne. When I imported it it screwed up the rotations and such so I just started to change values until it looked right. Thats also the reason for the "shot.getRenderYaw() - (float)Math.PI * 0.5f". Will clean all that rotation stuff up later though, its kinda messy right now. The scaling I'm worried about happens here though: float scale = 0.0625F * shot.getSizeFactor(); LogHelper.info(scale); cubeShot.render(scale); -
[Solved][1.7.10] Rendering a cube with scale not working
Alron replied to Alron's topic in Modder Support
What is that/how do I do it? I've never heard of it. -
I'd guess you have to remove the @SideOnly annotation at the top of your repair table class. I think it causes the constructor of that class to only exist on the client since you commented out the variable below the annotation.
-
[Solved][1.7.10] Rendering a cube with scale not working
Alron replied to Alron's topic in Modder Support
I'm actually doing that, its not the problem. shot.getSizeFactor() returns the correct value on the client and the server side. As I said, the scale is calculated properly and as far as I can tell it really should work. -
Hey everyone I've got a small problem with rendering cubes. I have a RenderShot that should render a shot for an EntityShot, and it basically just renders one cube with varying size. Or at least it should. Here's the code for the renderer: public class RenderShot extends Render{ private ModelBase model = new ModelBase(){}; private ModelRenderer cubeShot; public RenderShot(){ model.textureHeight = 32; model.textureWidth = 64; cubeShot = new ModelRenderer(model); cubeShot.addBox(-2F, -2F, -8F, 4, 4, 16);; cubeShot.setTextureSize(64, 32); } @Override public void doRender(Entity entity, double x, double y, double z, float yaw, float partialTickTime) { EntityShot shot = (EntityShot)entity; GL11.glPushMatrix(); GL11.glTranslatef((float)x, (float)y, (float)z); GL11.glScalef(-1F, -1F, 1F); Minecraft.getMinecraft().getTextureManager().bindTexture(Textures.Model.ENERGY_SHOT); //rotation works cubeShot.rotateAngleX = shot.getRenderPitch(); cubeShot.rotateAngleY = shot.getRenderYaw() - (float)Math.PI * 0.5f; //also correctly calculated float scale = 0.0625F * shot.getSizeFactor(); LogHelper.info(scale); cubeShot.render(scale); GL11.glPopMatrix(); } @Override protected ResourceLocation getEntityTexture(Entity entity) { return Textures.Model.ENERGY_SHOT; } } The problem is that it doesn't vary the size even though the scale calculation clearly works (I know cause I print it out). It always renders all shots the same size, either max scale(wich is a scale of 0.0625) or min scale (wich is about 0.015). It might switch from min to max or the other way around when I restart the game, but it always renders every shot the same size. Does anyone have an idea what could cause that?
-
I think that explains it well enough. Thanks!
-
Ok, I understand why you would use an API. So if I make a mod with Forge, why do I need things from FML in my base mod class? Or could I make a Forge mod without using anything from FML?