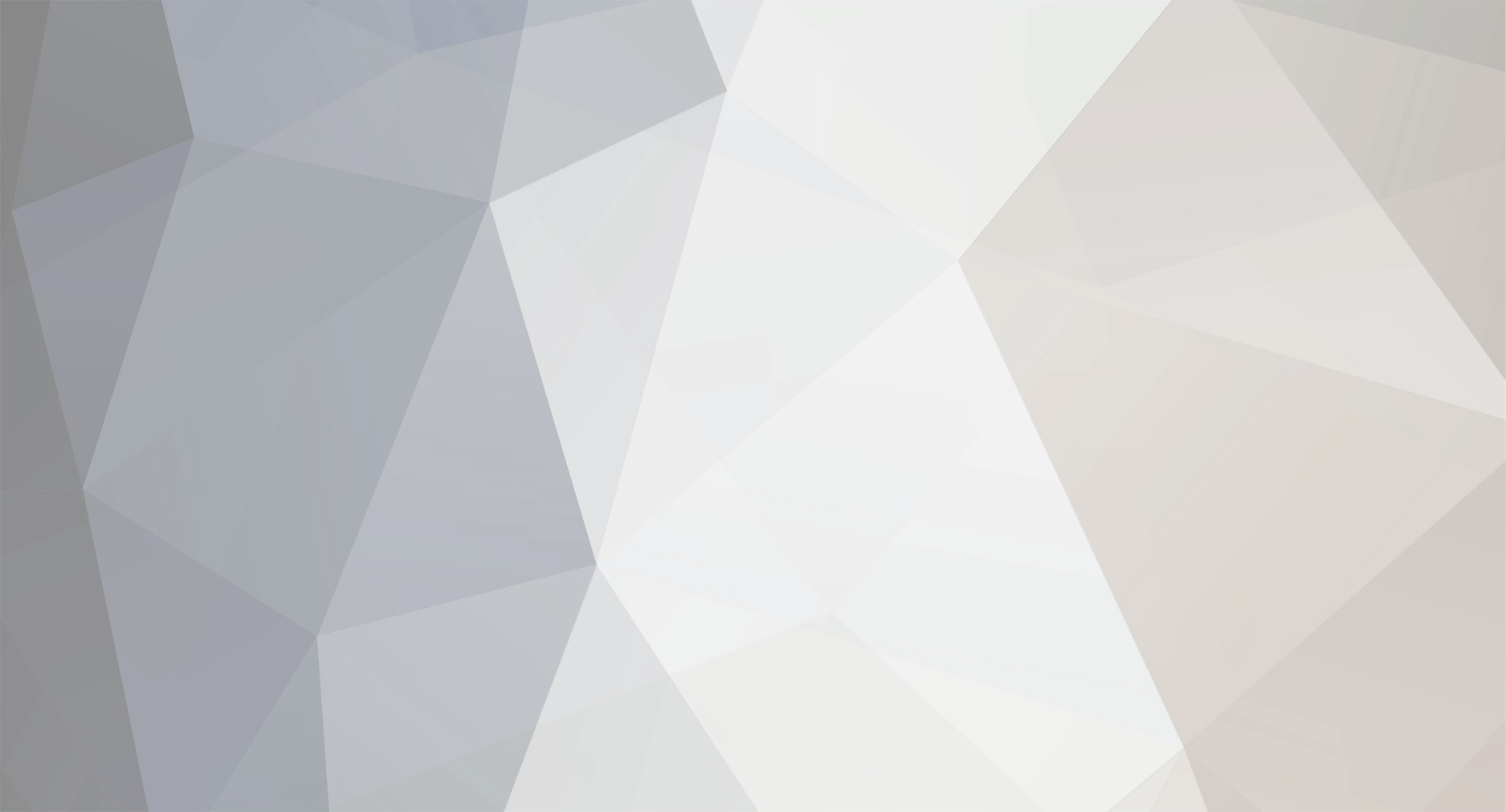
memanlolz
-
Posts
10 -
Joined
-
Last visited
Posts posted by memanlolz
-
-
do you know how exactly to create a tile entity?
-
i got my ItemBlock with
package com.yofungate.mods.YellowMech.misc; import com.yofungate.mods.YellowMech.common.YellowMech; import cpw.mods.fml.relauncher.Side; import cpw.mods.fml.relauncher.SideOnly; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.item.ItemBlock; import net.minecraft.item.ItemStack; import net.minecraft.tileentity.TileEntity; import net.minecraft.util.Icon; import net.minecraft.world.World; public class ItemBlockMegaBlock extends ItemBlock { private static final TileEntity TileEntityMegaBlock = new TileEntityMegaBlock(); public ItemBlockMegaBlock(int par1) { super(par1); this.setMaxDamage(0); this.setHasSubtypes(true); } @SideOnly(Side.CLIENT) public Icon getIconFromDamage(int par1) //Gets the texture { return YellowMech.MegaBlock.getIcon(2, par1); } public int getMetadata(int par1) //Returns the metadata value { return par1; } public boolean onItemUse(ItemStack par1ItemStack, EntityPlayer par2EntityPlayer, World par3World, int par4, int par5, int par6, int par7, float par8, float par9, float par10){ if (par7 == 0) { --par5; } if (par7 == 1) { ++par5; } if (par7 == 2) { --par6; } if (par7 == 3) { ++par6; } if (par7 == 4) { --par4; } if (par7 == 5){ ++par4; } int x=par4, y=par5, z=par6; par3World.setBlock(x, y, z, this.getBlockID()); par3World.setBlockTileEntity(x, y, z, TileEntityMegaBlock); par3World.setBlockMetadataWithNotify(x, y, z, par1ItemStack.getItemDamage(), 3); return true; } public String getItemNameIS(ItemStack is) //Get's the item incode name from an itemstack { return "YellowMech." + BlockMegaBlock.IconNames[is.getItemDamage()]; } }
-
1.if its some kind of tile entity without any gui, or anything wierd, than nop, i dont think so, (IM NOT SURE >.<)
2.containers are a wierd & confusing thing, containers probably do something hidden in the background, something that we cant see or value.
-
MatterCraft
in Mods
ooo, so one type of port of ee2?
-
i can help you with the textures
show your source codes at least, so i can see what you already have, just show the block and itemblock that you have,
-
you could always do this
public boolean isItemValid(ItemStack stack) { try{ if (stack != null && stack.getItem() != null && Block.blocksList[stack.getItem().itemID] != null) { return true; } }catch(Exception e){ return false; } }
-
Anyone willing to help me?
-
i already have an itemblock.
package com.yofungate.mods.YellowMech.misc; import com.yofungate.mods.YellowMech.common.YellowMech; import cpw.mods.fml.relauncher.Side; import cpw.mods.fml.relauncher.SideOnly; import net.minecraft.item.ItemBlock; import net.minecraft.item.ItemStack; import net.minecraft.util.Icon; public class ItemBlockMegaBlock extends ItemBlock { public ItemBlockMegaBlock(int par1) { super(par1); this.setMaxDamage(0); this.setHasSubtypes(true); } @SideOnly(Side.CLIENT) public Icon getIconFromDamage(int par1) //Gets the texture { return YellowMech.MegaBlock.getIcon(2, par1); } public int getMetadata(int par1) //Returns the metadata value { return par1; } public String getItemNameIS(ItemStack is) //Get's the item incode name from an itemstack { return "YellowMech." + BlockMegaBlock.IconNames[is.getItemDamage()]; } }
-
Well, i have this block, which i want to have 33 subblocks, and everywhere i look, everyone just says "You need TE". i realise i need a TE... just how the heck do i make one!?
Code : MetaDataBlock
private static final TileEntityMegaBlock TileEntityMega = new TileEntityMegaBlock(); private static final TileEntityMegaBlock TE = TileEntityMega; public static Icon[] IconArray; public static String[] IconNames = {"stone","stonebrick","dirt","wood","bedrock","sand","gravel","oreCoal","oreIron","oreGold","oreLapis","oreDiamond","oreRedstone","blockIron","blockGold","blockDiamond","blockLapis","blockRedstone","cloth_0","cloth_1","cloth_2","cloth_3","cloth_4","cloth_5","cloth_6","cloth_7","cloth_8","cloth_9","cloth_10","cloth_11","cloth_12","cloth_13","cloth_14","cloth_15"}; public BlockMegaBlock(int par1) { super(par1, Material.ground); this.setBlockBounds(0.30F, 0.30F, 0.30F, 0.70F, 0.70F, 0.70F); } public boolean hasTileEntity(int meta){ return true; } public TileEntity createNewTileEntity(World world, int meta) { return TE; } @Override public void onBlockAdded(World par1World, int par2, int par3, int par4){ par1World.setBlockTileEntity(par2, par3, par4, TileEntityMega); this.TE.worldObj.setBlockTileEntity(par2, par3, par4, TileEntityMega); super.onBlockAdded(par1World, par2, par3, par4); par1World.setBlockTileEntity(par2, par3, par4, TileEntityMega); this.TE.worldObj.setBlockTileEntity(par2, par3, par4, TileEntityMega); } public boolean isOpaqueCube() { return false; } public void registerIcons(IconRegister par1IconRegister) { this.IconArray = new Icon[iconNames.length]; for(int i=0;i<IconNames.length;i++){ System.out.println("Registered MegaBlock #"+i+" With Image "+this.IconNames[i]); this.IconArray[i] = par1IconRegister.registerIcon(this.IconNames[i]); } } public Icon getIcon(int par1, int par2) { return this.IconArray[par2]; } public int idDropped(int par1, Random par2Random, int par3) { return this.blockID; } public int quantityDropped(Random par1Random) { return 1; } public int damageDropped(int i){ //Tells it what block it drops and also for creative mode pick block return i; } @SideOnly(Side.CLIENT) public void getSubBlocks(int par1, CreativeTabs par2CreativeTabs, List par3List) //Adds the metadata blocks to the creative inventory { for(int i=0;i<IconNames.length;i++){ par3List.add(new ItemStack(par1, 1, i)); } }
Tile Entity
package com.yofungate.mods.YellowMech.misc; import cpw.mods.fml.common.network.IPacketHandler; import cpw.mods.fml.common.network.Player; import net.minecraft.nbt.NBTTagCompound; import net.minecraft.network.INetworkManager; import net.minecraft.network.packet.Packet250CustomPayload; import net.minecraft.tileentity.TileEntity; public class TileEntityMegaBlock extends TileEntity { public static int blockMetadata = 0; @Override public void readFromNBT(NBTTagCompound nbt) { super.readFromNBT(nbt); blockMetadata = nbt.getInteger("BMD") & 0xFF; System.out.print(blockMetadata); } @Override public void writeToNBT(NBTTagCompound nbt) { super.writeToNBT(nbt); System.out.print(blockMetadata); nbt.setInteger("BMD", this.blockMetadata); } public static int GetMeta(){ return blockMetadata; } }
MetaData Block Tile Entity?
in Modder Support
Posted
Okay, i somehow did everything untill now, now i need to know, how do i make it Save the tile entity?
Codes So Far,
Tile Entity
ItemBlock
Block.