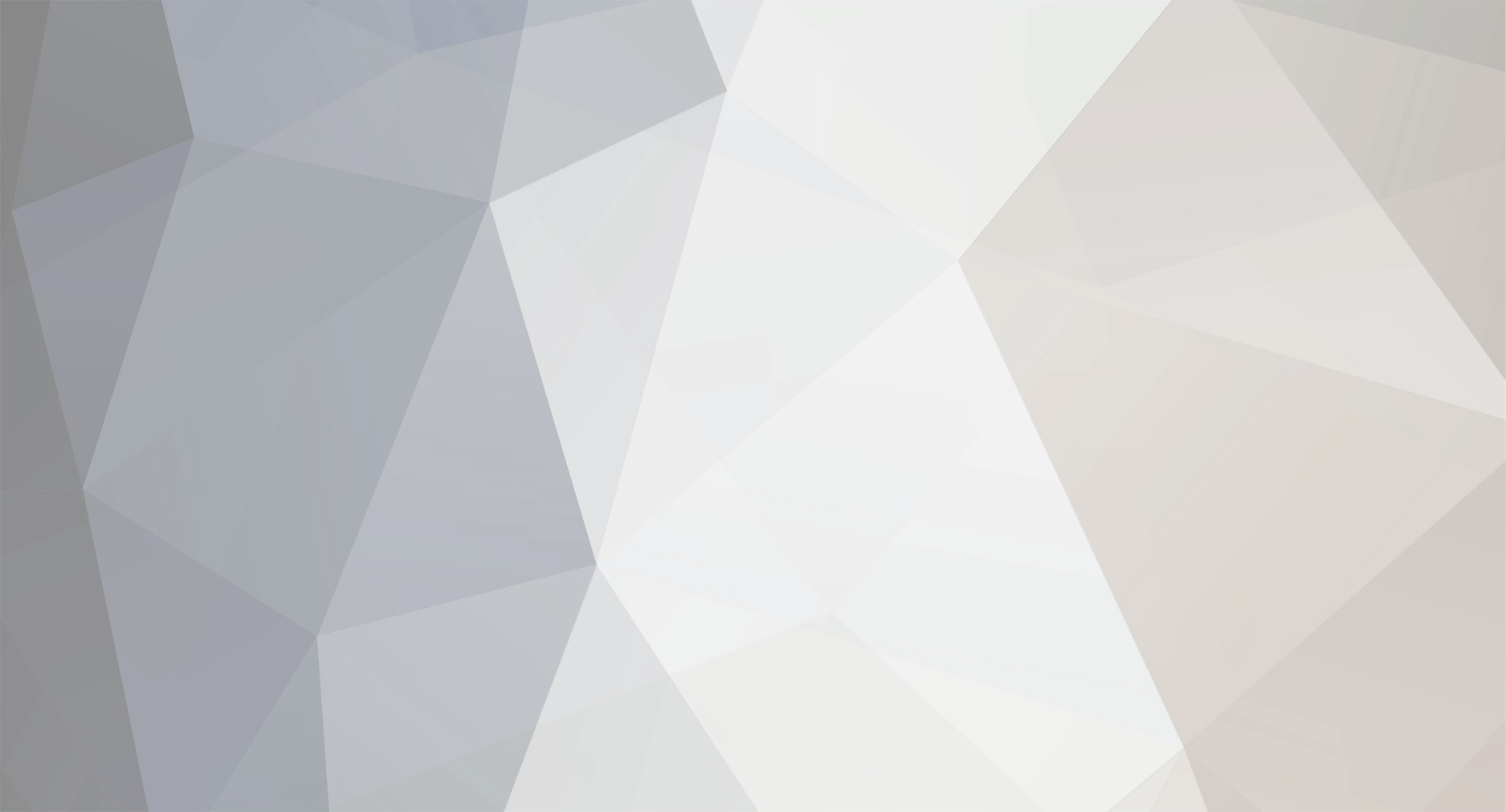
kmccmk9
-
Posts
48 -
Joined
-
Last visited
Posts posted by kmccmk9
-
-
There is no workaround for this. Mods only work for the Minecraft version they are designed for.
ok thanks for the response. If 1.6 telepads is broken then is it possible he recompiled from source to 1.7?
-
Hi, I have a private server with a bunch of 1.6.4 mods on it. I wish to go to 1.7 since one of the mods is broken in 1.6.4. Of course not all of my mods are updated. While I search and searched there seems to be no way to use a mod from 1.6.4 in 1.7 forge. But, I've been watching captain sparklez and he is on 1.7 forge with telepads mod and many others that haven't been updated yet. Is there actually a work around for this?
-
I'm sure you can find a workaround for that if you want to
Okay I'm open to new ideas. I tried to do a cast but that didn't work. Would you recommend extending something different.
-
EntityAIHurtByTarget ?
Thanks for the reply. Although I'm having trouble adding that task since I'm using entityliving instead of entitycreature.
-
Hello, I'm trying to basically do what the title says. I have a passive mob which extends entityliving, and I would like it to attack the player if the player attacks it. I tried taking some code from both the cow and the wolf to try and implement this. Below, is what I have. Currently, it does not attack at all. Also for some reason the movement of my mob in general is very glitchy and random. Any help would be appreciated.
package kmccmk9.witchcraft; import net.minecraft.client.renderer.EntityRenderer; import net.minecraft.entity.Entity; import net.minecraft.entity.EntityCreature; import net.minecraft.entity.EntityLiving; import net.minecraft.entity.EntityLivingBase; import net.minecraft.entity.ai.EntityAIAttackOnCollide; import net.minecraft.entity.ai.EntityAIBeg; import net.minecraft.entity.ai.EntityAIFollowOwner; import net.minecraft.entity.ai.EntityAIHurtByTarget; import net.minecraft.entity.ai.EntityAILeapAtTarget; import net.minecraft.entity.ai.EntityAILookIdle; import net.minecraft.entity.ai.EntityAIMate; import net.minecraft.entity.ai.EntityAIOwnerHurtByTarget; import net.minecraft.entity.ai.EntityAIOwnerHurtTarget; import net.minecraft.entity.ai.EntityAISwimming; import net.minecraft.entity.ai.EntityAITargetNonTamed; import net.minecraft.entity.ai.EntityAIWander; import net.minecraft.entity.ai.EntityAIWatchClosest; import net.minecraft.entity.passive.EntitySheep; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.item.Item; import net.minecraft.util.DamageSource; import net.minecraft.world.World; import net.minecraft.src.*; public class EntityBee extends EntityLiving { public EntityBee(World par1World) { super(par1World); this.getNavigator().setAvoidsWater(true); this.setSize(0.5F, 0.5F); this.setEntityHealth(25F); this.setAIMoveSpeed(2F); this.tasks.addTask(0, new EntityAILookIdle(this)); this.tasks.addTask(1, new EntityAISwimming(this)); this.tasks.addTask(2, new EntityAILeapAtTarget(this, 0.4F)); this.tasks.addTask(3, new EntityAIWatchClosest(this, EntityPlayer.class, 8.0F)); this.tasks.addTask(4, new EntityAILookIdle(this)); } protected int getDropItemId() { return Item.porkRaw.itemID; } /** * Returns true if the newer Entity AI code should be run */ public boolean isAIEnabled() { return true; } /** * Sets the active target the Task system uses for tracking */ public void setAttackTarget(EntityLivingBase par1EntityLivingBase) { super.setAttackTarget(par1EntityLivingBase); } public boolean attackEntityAsMob(Entity par1Entity) { int i = 7; return par1Entity.attackEntityFrom(DamageSource.causeMobDamage(this), (float)i); } }
-
Then try to find a modeling software that works.
I tried both Techne and FMCModeller. The Bee Head is just stick inside the body, if anyone knows how to modify the addbox line for the bee head so that it shows in the right spot...?
-
At a guess I'd say that it's because there isn't really alot in your render file atm, so it might not be connecting the render and entity to the model
erm... I'd suggest taking a look at the bat render file
it's probably the closest you're gunna get to a bee in vanilla
it could be this
public void func_82443_a(EntityBat par1EntityBat, double par2, double par4, double par6, float par8, float par9) { int i = ((ModelBat)this.mainModel).getBatSize(); if (i != this.renderedBatSize) { this.renderedBatSize = i; this.mainModel = new ModelBat(); } super.doRenderLiving(par1EntityBat, par2, par4, par6, par8, par9); }
so try
public void func_82443_a(EntityBee par1EntityBat, double par2, double par4, double par6, float par8, float par9) { int i = ((ModelBee)this.mainModel); this.mainModel = new ModelBee(); super.doRenderLiving(par1EntityBat, par2, par4, par6, par8, par9); }
however, that is just a guess
exploring the render files would be a better option
and there are some 1.6.2 entity tutorials out there that may be a better help than me
the correct texture calling would be for example ("foldername:textures/mob/bee.png")
what this means is that bee.png is located in ("assets/foldername/textures/mob/bee.png")\
for a normal icon texture the calling would be different for example a normal icon texture would be ("foldername:icon")
which means the icon would be in ("assets/foldername/textures/[blocks][items]/icon.png")
I hope this helps
Ok well from what I gathered, what's messed up is the beehead. Nothing else. But if you look at my model class you 'll see I had to comment out a setPosition line. I think that is the problem. But setPosition is valid so I had to comment it. Otherwise everything is right, the head is just inside the body.
-
no, if you're working in Eclipse, they're placed in forge/mcp/src/minecraft/assets
mine is
forge/mcp/src/minecraft/assets/jnosh_advzelda/textures/entity/chu.png
at least, that's the way that I've seen them all set up since 1.6.1 arrived
you might wanna invest some time into looking up how to update from 1.5.2 to 1.6.x if you've still got things set up the old way
OMG thank you I finally got it! Now do you have any idea what's wrong with my model?
-
no, if you're working in Eclipse, they're placed in forge/mcp/src/minecraft/assets
mine is
forge/mcp/src/minecraft/assets/jnosh_advzelda/textures/entity/chu.png
at least, that's the way that I've seen them all set up since 1.6.1 arrived
you might wanna invest some time into looking up how to update from 1.5.2 to 1.6.x if you've still got things set up the old way
Okay thanks. Also, following another mod I explored, it was done like so kmccmk9/witchCraft/assets/minecraft/textures/entities/file
-
The way that resource locations are done is defaulted to the vanilla minecraft assets folder
you need to change it to so it's directed towards yours
for instance, with mine it's
private static final ResourceLocation field_110890_f = new ResourceLocation("jnosh_advzelda", "textures/entity/chu.png");
as the folder structure goes
"assets" folder > "jnosh_advzelda" folder > "textures" folder > "entity" folder > "chu.png"
assets/jnosh_advzelda/textures/entity/chu
you need to do yours in a similar way, but using your own folders (obviously)
So textures are no longer placed within your mod folder?
-
#RenderingRegistry
Great thanks. I was able to get the resuilt below. Okay, now the model seems to be upside down. The texture problem, I have to figure out still.
Is there something I can do with the mirror part of the model code? What I"m shooting for is like the below image.
-
Ho that, you didn't register your renderer.
Ok, can you explain how to do that?
-
I don't recall Minecraft having a bee texture.
Look for "texture" threads, the proper use of ResourceLocation would be explained.
Even with the wrong texture path, isn't my model messed up?
-
I don't recall Minecraft having a bee texture.
Look for "texture" threads, the proper use of ResourceLocation would be explained.
Ok I'll check it out cause the tutorials explained that the way I wrote it, would be the root of the mod folder, then textures and then bee.png.
-
Your ResourceLocation of course ! that is what the method asks for !
And give the right texture path too...
Okay I changed it and I got this, which seems to still be messed up. But, that is where my texture is?
-
private static final ResourceLocation field_110775_a = new ResourceLocation("textures/bee.png"); @Override protected ResourceLocation func_110775_a(Entity entity) { // TODO Auto-generated method stub return null; }
*facepalm*
Why do you think that should work ?
Ya I had a feeling it may be in that class. But I keep trying to follow different tutorials for different forge versions since no one seems to have one for 1.6.2 as of yet. Okay so what would I need to return then?
-
No, wrong section for that...
I think he means method to call on the server to send a message to all players on the server.
Okay that's what I was thinking too. Although if he could tap into and issue a server command, he could use the say command. Otherwise, I know in bukkit you can do server.broadcastMessage(). I'm sure there is something similar...
-
What do you mean by command? Like when you're running a server and you're an OP?
-
Hello, I've been trying to add a mob to the game and have been able to get an egg that can spawn my new mob. However, it comes out like the picture below instead of what it should be, a bee. What is wrong with my code? Below I have posted everything I got for you.
Main Class:
package kmccmk9.witchcraft; import java.awt.Color; import net.minecraft.entity.Entity; import net.minecraft.entity.EntityEggInfo; import net.minecraft.entity.EntityList; import net.minecraft.entity.EnumCreatureType; import net.minecraft.src.ModLoader; import net.minecraft.world.biome.BiomeGenBase; import kmccmk9.witchcraft.CommonProxy; import cpw.mods.fml.common.Mod; import cpw.mods.fml.common.SidedProxy; import cpw.mods.fml.common.Mod.EventHandler; import cpw.mods.fml.common.Mod.Instance; import cpw.mods.fml.common.event.FMLInitializationEvent; import cpw.mods.fml.common.event.FMLPostInitializationEvent; import cpw.mods.fml.common.event.FMLPreInitializationEvent; import cpw.mods.fml.common.network.NetworkMod; import cpw.mods.fml.common.registry.EntityRegistry; import cpw.mods.fml.common.registry.LanguageRegistry; @Mod(modid="witchcraft", name="WitchCraft", version="0.0.1") @NetworkMod(clientSideRequired=true, serverSideRequired=false) public class WitchCraft { // The instance of your mod that Forge uses. @Instance("WitchCraft") public static WitchCraft instance; // Says where the client and server 'proxy' code is loaded. @SidedProxy(clientSide="kmccmk9.witchcraft.client.ClientProxy", serverSide="kmccmk9.witchcraft.CommonProxy") public static CommonProxy proxy; @EventHandler public void preInit(FMLPreInitializationEvent event) { // Stub Method } @EventHandler public void load(FMLInitializationEvent event) { int background = (Color.YELLOW.getRed() << 16) + (Color.YELLOW.getGreen() << + Color.YELLOW.getBlue(); int foreground = (Color.BLACK.getRed() << 16) + (Color.BLACK.getGreen() << + Color.BLACK.getBlue(); int id = ModLoader.getUniqueEntityId(); EntityRegistry.registerModEntity(EntityBee.class, "Bee", 1, this, 80, 5, true); ModLoader.registerEntityID(EntityBee.class, "Bee", id, background, foreground); EntityRegistry.addSpawn(EntityBee.class, 7, 1, 4, EnumCreatureType.monster, BiomeGenBase.beach, BiomeGenBase.forest, BiomeGenBase.jungle, BiomeGenBase.plains); LanguageRegistry.instance().addStringLocalization("entity.witchcraft.Bee.name", "Bee"); EntityList.IDtoClassMapping.put(id, EntityBee.class); EntityList.entityEggs.put(id, new EntityEggInfo(id, background, foreground)); } @EventHandler public void postInit(FMLPostInitializationEvent event) { // Stub Method } }
EntityClass:
package kmccmk9.witchcraft; import net.minecraft.client.renderer.EntityRenderer; import net.minecraft.entity.EntityLiving; import net.minecraft.entity.ai.EntityAIWander; import net.minecraft.item.Item; import net.minecraft.world.World; import net.minecraft.src.*; public class EntityBee extends EntityLiving { public EntityBee(World par1World) { super(par1World); this.getNavigator().setAvoidsWater(true); this.setSize(0.5F, 0.5F); this.setEntityHealth(15F); this.setAIMoveSpeed(3F); } protected int getDropItemId() { return Item.porkRaw.itemID; } public String getTexture() { return "textures/bee.png"; } }
ModelClass:
package kmccmk9.witchcraft.client; import net.minecraft.client.model.ModelBase; import net.minecraft.client.model.ModelRenderer; import net.minecraft.entity.Entity; public class ModelBee extends ModelBase { //fields ModelRenderer BeeBody; ModelRenderer BeeHead; ModelRenderer ant1; ModelRenderer ant2; ModelRenderer wing2; ModelRenderer wing1; public ModelBee() { //constructor: ant1 = new ModelRenderer(this, 0, 0); ant1.mirror = true; ant1.addBox(2F, 4F, -1F, 1, 4, 1); ant1.rotateAngleZ = 0.17453292519943295F; ant2 = new ModelRenderer(this, 41, 5); ant2.mirror = true; ant2.addBox(2F, 3F, -1F, 1, 4, 1); ant2.rotateAngleZ = 6.1086523819801535F; BeeBody = new ModelRenderer(this, 0, 0); BeeBody.mirror = true; BeeBody.addBox(0F, 0F, 0F, 5, 4, ; BeeHead = new ModelRenderer(this, 19, 2); BeeHead.mirror = true; BeeHead.addBox(0F, 0F, 0F, 3, 3, 2); //BeeHead.setPosition(1F, 1F, -2F); wing1 = new ModelRenderer(this, 31, 1); wing1.mirror = true; wing1.addBox(5F, 1F, 2F, 4, 1, 3); wing1.rotateAngleZ = 6.126105674500097F; wing2 = new ModelRenderer(this, 31, 5); wing2.mirror = true; wing2.addBox(-4F, 2F, 2F, 4, 1, 3); wing2.rotateAngleZ = 0.15707963267948966F; } public void render(Entity entity, float f, float f1, float f2, float f3, float f4, float f5) { //render: ant1.render(f5); ant2.render(f5); BeeBody.render(f5); BeeHead.render(f5); wing1.render(f5); wing2.render(f5); } private void setRotation(ModelRenderer model, float x, float y, float z) { model.rotateAngleX = x; model.rotateAngleY = y; model.rotateAngleZ = z; } public void setRotationAngles(float f, float f1, float f2, float f3, float f4, float f5, Entity entity) { super.setRotationAngles(f, f1, f2, f3, f4, f5, entity); } }
RendererClass:
package kmccmk9.witchcraft; import kmccmk9.witchcraft.client.ModelBee; import net.minecraft.client.Minecraft; import net.minecraft.client.model.ModelBase; import net.minecraft.client.renderer.EntityRenderer; import net.minecraft.client.renderer.entity.RenderLiving; import net.minecraft.entity.Entity; import net.minecraft.util.ResourceLocation; public class RendererBee extends RenderLiving { protected ModelBee model; private static final ResourceLocation field_110775_a = new ResourceLocation("textures/bee.png"); public RendererBee(ModelBee par1ModelBase, float par2) { super(par1ModelBase, par2); model = (ModelBee)par1ModelBase; } @Override protected ResourceLocation func_110775_a(Entity entity) { // TODO Auto-generated method stub return null; } }
Any help is greatly appreciated!
-
I cba explaining it on here, because I'm tired and about to go to bed, but I wrote out a tutorial on how to get your spawn eggs into a custom creative tab, if you can understand it
http://www.minecraftforum.net/topic/1921545-162-mob-spawn-egg-in-custom-creative-tab-tutorial/
and if you don't care about putting them in a custom creative tab, I uploaded my old way onto my github here
https://github.com/Jacknoshima/Mods/blob/master/Pre-Alt%20Main%20Mod%20File
which puts them into the misc tab
the parts that get you spawn eggs are:
public static int getUniqueID(){ int EntityId = 400; do{ EntityId++; } while(EntityList.getStringFromID(EntityId) != null); return EntityId; } public static void EntityEgg(Class<? extends Entity > entity, int primaryColor, int secondaryColor){ int id = getUniqueID(); EntityList.IDtoClassMapping.put(id, entity); EntityList.entityEggs.put(id, new EntityEggInfo(id, primaryColor, secondaryColor)); }
and
EntityEgg(EntityStatVillager.class, 0xFFCCDD, 0x1900FF);
Hi thanks for the post, it seems to have worked (kinda). I'm having a problem with it. The first is that my model does not render correctly (see photo below), and when I tried to spawn the creature it caused the game to lag very badly (Could be due to the failed model). Below is my modified main class in case I messed up your directions. Thanks.
I added these two lines to my main and everything is working well except for the model. Is it possible my model class is messed up?
EntityList.IDtoClassMapping.put(id, EntityBee.class); EntityList.entityEggs.put(id, new EntityEggInfo(id, background, foreground));
-
Hello, so I've been trying to follow a few tutorials on how to add your own entity in the game. I have a model class, entity class and a render class. Now what am I missing to get an egg to show in the creative menu so I can even test if the model works in game? I did add the line from the wiki EntityRegistry.registerModEntity(EntityBee.class, "Bee", 1, this, 80, 5, true); Or should I be using the register mod ID instead?
EDIT: Currently I'm using these three lines of code.
EntityRegistry.registerModEntity(EntityBee.class, "Bee", 1, this, 80, 5, true); ModLoader.registerEntityID(EntityBee.class, "Bee", 1, background, foreground); EntityRegistry.addSpawn(EntityBee.class, 7, 1, 4, EnumCreatureType.monster, BiomeGenBase.beach, BiomeGenBase.forest, BiomeGenBase.jungle, BiomeGenBase.plains); LanguageRegistry.instance().addStringLocalization("entity.witchcraft.Bee.name", "Bee");
Render class:
package kmccmk9.witchcraft; import kmccmk9.witchcraft.client.ModelBee; import net.minecraft.client.Minecraft; import net.minecraft.client.model.ModelBase; import net.minecraft.client.renderer.EntityRenderer; import net.minecraft.client.renderer.entity.RenderLiving; import net.minecraft.entity.Entity; import net.minecraft.util.ResourceLocation; public class RendererBee extends RenderLiving { protected ModelBee model; private static final ResourceLocation field_110775_a = new ResourceLocation("textures/bee.png"); public RendererBee(ModelBee par1ModelBase, float par2) { super(par1ModelBase, par2); model = (ModelBee)par1ModelBase; } @Override protected ResourceLocation func_110775_a(Entity entity) { // TODO Auto-generated method stub return null; } }
Entity Class:
package kmccmk9.witchcraft; import net.minecraft.client.renderer.EntityRenderer; import net.minecraft.entity.EntityLiving; import net.minecraft.entity.ai.EntityAIWander; import net.minecraft.item.Item; import net.minecraft.world.World; import net.minecraft.src.*; public class EntityBee extends EntityLiving { public EntityBee(World par1World) { super(par1World); this.getNavigator().setAvoidsWater(true); this.setSize(0.5F, 0.5F); this.setEntityHealth(15F); this.setAIMoveSpeed(3F); } protected int getDropItemId() { return Item.porkRaw.itemID; } public String getTexture() { return "textures/body.png"; } }
Model Class:
package kmccmk9.witchcraft.client; import net.minecraft.client.model.ModelBase; import net.minecraft.client.model.ModelRenderer; import net.minecraft.entity.Entity; public class ModelBee extends ModelBase { //fields ModelRenderer BeeBody; ModelRenderer BeeHead; ModelRenderer ant1; ModelRenderer ant2; ModelRenderer wing2; ModelRenderer wing1; public ModelBee() { textureWidth = 64; textureHeight = 32; BeeBody = new ModelRenderer(this, 0, 0); BeeBody.addBox(0F, 0F, 0F, 5, 4, ; BeeBody.setRotationPoint(-3F, -3F, -4F); BeeBody.setTextureSize(64, 32); BeeBody.mirror = true; setRotation(BeeBody, 0F, 0F, 0F); BeeHead = new ModelRenderer(this, 19, 2); BeeHead.addBox(0F, 0F, 0F, 3, 3, 2); BeeHead.setRotationPoint(-2F, -4F, -6F); BeeHead.setTextureSize(64, 32); BeeHead.mirror = true; setRotation(BeeHead, 0F, 0F, 0F); ant1 = new ModelRenderer(this, 0, 0); ant1.addBox(0F, 0F, 0F, 1, 4, 1); ant1.setRotationPoint(1F, -7F, -5F); ant1.setTextureSize(64, 32); ant1.mirror = true; setRotation(ant1, 0F, 0F, 0.2722714F); ant2 = new ModelRenderer(this, 0, 0); ant2.addBox(0F, 0F, 0F, 1, 4, 1); ant2.setRotationPoint(-3F, -7F, -5F); ant2.setTextureSize(64, 32); ant2.mirror = true; setRotation(ant2, 0F, 0F, -0.2617994F); wing2 = new ModelRenderer(this, 29, 0); wing2.addBox(0F, 0F, 0F, 4, 1, 3); wing2.setRotationPoint(-7F, -3F, -1F); wing2.setTextureSize(64, 32); wing2.mirror = true; setRotation(wing2, 0F, 0F, 0.1745329F); wing1 = new ModelRenderer(this, 29, 0); wing1.addBox(0F, 0F, 0F, 4, 1, 3); wing1.setRotationPoint(2F, -2F, -1F); wing1.setTextureSize(64, 32); wing1.mirror = true; setRotation(wing1, 0F, 0F, -0.1745329F); } public void render(Entity entity, float f, float f1, float f2, float f3, float f4, float f5) { super.render(entity, f, f1, f2, f3, f4, f5); setRotationAngles(f, f1, f2, f3, f4, f5, entity); BeeBody.render(f5); BeeHead.render(f5); ant1.render(f5); ant2.render(f5); wing2.render(f5); wing1.render(f5); } private void setRotation(ModelRenderer model, float x, float y, float z) { model.rotateAngleX = x; model.rotateAngleY = y; model.rotateAngleZ = z; } public void setRotationAngles(float f, float f1, float f2, float f3, float f4, float f5, Entity entity) { super.setRotationAngles(f, f1, f2, f3, f4, f5, entity); } }
-
import kmccmk9.commandBlockRecipe.CommonProxy;
That is what you get when you work on two mods
Wrong import !
Aha! Problem fixed. Thank you. Now, my model will not show in the creative menu. :sigh:
-
you still have to point to the new package/class
Oops ya ok I fixed that, but now I"m back to the proxy injector problem.
2013-08-22 15:20:19 [sEVERE] [ForgeModLoader] Attempted to load a proxy type kmccmk9.witchcraft.client.ClientProxy into kmccmk9.witchcraft.WitchCraft.proxy, but the types don't match 2013-08-22 15:20:19 [sEVERE] [ForgeModLoader] An error occured trying to load a proxy into {clientSide=kmccmk9.witchcraft.client.ClientProxy, serverSide=kmccmk9.witchcraft.CommonProxy}.kmccmk9.witchcraft.WitchCraft cpw.mods.fml.common.LoaderException at cpw.mods.fml.common.ProxyInjector.inject(ProxyInjector.java:68) at cpw.mods.fml.common.FMLModContainer.constructMod(FMLModContainer.java:519) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:57) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:601) at com.google.common.eventbus.EventHandler.handleEvent(EventHandler.java:74) at com.google.common.eventbus.SynchronizedEventHandler.handleEvent(SynchronizedEventHandler.java:45) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:313) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:296) at com.google.common.eventbus.EventBus.post(EventBus.java:267) at cpw.mods.fml.common.LoadController.sendEventToModContainer(LoadController.java:194) at cpw.mods.fml.common.LoadController.propogateStateMessage(LoadController.java:174) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:57) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:601) at com.google.common.eventbus.EventHandler.handleEvent(EventHandler.java:74) at com.google.common.eventbus.SynchronizedEventHandler.handleEvent(SynchronizedEventHandler.java:45) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:313) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:296) at com.google.common.eventbus.EventBus.post(EventBus.java:267) at cpw.mods.fml.common.LoadController.distributeStateMessage(LoadController.java:105) at cpw.mods.fml.common.Loader.loadMods(Loader.java:510) at cpw.mods.fml.client.FMLClientHandler.beginMinecraftLoading(FMLClientHandler.java:182) at net.minecraft.client.Minecraft.startGame(Minecraft.java:470) at net.minecraft.client.Minecraft.func_99999_d(Minecraft.java:796) at net.minecraft.client.main.Main.main(Main.java:93) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:57) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:601) at net.minecraft.launchwrapper.Launch.launch(Launch.java:57) at net.minecraft.launchwrapper.Launch.main(Launch.java:18) 2013-08-22 15:20:19 [FINEST] [commandblockrecipe] Sent event FMLConstructionEvent to mod commandblockrecipe 2013-08-22 15:20:19 [FINEST] [witchcraft] Sending event FMLConstructionEvent to mod witchcraft 2013-08-22 15:20:19 [FINEST] [ForgeModLoader] Testing mod witchcraft to verify it accepts its own version in a remote connection 2013-08-22 15:20:19 [FINEST] [ForgeModLoader] The mod witchcraft accepts its own version (0.0.1) 2013-08-22 15:20:19 [FINE] [ForgeModLoader] Attempting to inject @SidedProxy classes into witchcraft 2013-08-22 15:20:19 [sEVERE] [ForgeModLoader] Attempted to load a proxy type kmccmk9.witchcraft.client.ClientProxy into kmccmk9.witchcraft.WitchCraft.proxy, but the types don't match 2013-08-22 15:20:19 [sEVERE] [ForgeModLoader] An error occured trying to load a proxy into {clientSide=kmccmk9.witchcraft.client.ClientProxy, serverSide=kmccmk9.witchcraft.CommonProxy}.kmccmk9.witchcraft.WitchCraft cpw.mods.fml.common.LoaderException at cpw.mods.fml.common.ProxyInjector.inject(ProxyInjector.java:68) at cpw.mods.fml.common.FMLModContainer.constructMod(FMLModContainer.java:519) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:57) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:601) at com.google.common.eventbus.EventHandler.handleEvent(EventHandler.java:74) at com.google.common.eventbus.SynchronizedEventHandler.handleEvent(SynchronizedEventHandler.java:45) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:313) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:296) at com.google.common.eventbus.EventBus.post(EventBus.java:267) at cpw.mods.fml.common.LoadController.sendEventToModContainer(LoadController.java:194) at cpw.mods.fml.common.LoadController.propogateStateMessage(LoadController.java:174) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:57) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:601) at com.google.common.eventbus.EventHandler.handleEvent(EventHandler.java:74) at com.google.common.eventbus.SynchronizedEventHandler.handleEvent(SynchronizedEventHandler.java:45) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:313) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:296) at com.google.common.eventbus.EventBus.post(EventBus.java:267) at cpw.mods.fml.common.LoadController.distributeStateMessage(LoadController.java:105) at cpw.mods.fml.common.Loader.loadMods(Loader.java:510) at cpw.mods.fml.client.FMLClientHandler.beginMinecraftLoading(FMLClientHandler.java:182) at net.minecraft.client.Minecraft.startGame(Minecraft.java:470) at net.minecraft.client.Minecraft.func_99999_d(Minecraft.java:796) at net.minecraft.client.main.Main.main(Main.java:93) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:57) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:601) at net.minecraft.launchwrapper.Launch.launch(Launch.java:57) at net.minecraft.launchwrapper.Launch.main(Launch.java:18) 2013-08-22 15:20:19 [FINEST] [witchcraft] Sent event FMLConstructionEvent to mod witchcraft 2013-08-22 15:20:19 [FINE] [ForgeModLoader] Mod signature data 2013-08-22 15:20:19 [FINE] [ForgeModLoader] mcp(Minecraft Coder Pack:8.04): minecraft.jar (NO VALID CERTIFICATE FOUND) 2013-08-22 15:20:19 [FINE] [ForgeModLoader] FML(Forge Mod Loader:6.2.35.804): coremods (NO VALID CERTIFICATE FOUND) 2013-08-22 15:20:19 [FINE] [ForgeModLoader] Forge(Minecraft Forge:9.10.0.804): coremods (NO VALID CERTIFICATE FOUND) 2013-08-22 15:20:19 [FINE] [ForgeModLoader] commandblockrecipe(CommandBlockRecipe:0.0.1): bin (NO VALID CERTIFICATE FOUND) 2013-08-22 15:20:19 [FINE] [ForgeModLoader] witchcraft(WitchCraft:0.0.1): bin (NO VALID CERTIFICATE FOUND) 2013-08-22 15:20:19 [sEVERE] [ForgeModLoader] Fatal errors were detected during the transition from CONSTRUCTING to PREINITIALIZATION. Loading cannot continue 2013-08-22 15:20:19 [sEVERE] [ForgeModLoader] mcp{8.04} [Minecraft Coder Pack] (minecraft.jar) Unloaded->Constructed FML{6.2.35.804} [Forge Mod Loader] (coremods) Unloaded->Constructed Forge{9.10.0.804} [Minecraft Forge] (coremods) Unloaded->Constructed commandblockrecipe{0.0.1} [CommandBlockRecipe] (bin) Unloaded->Errored witchcraft{0.0.1} [WitchCraft] (bin) Unloaded->Errored 2013-08-22 15:20:19 [sEVERE] [ForgeModLoader] The following problems were captured during this phase 2013-08-22 15:20:19 [sEVERE] [ForgeModLoader] Caught exception from witchcraft cpw.mods.fml.common.LoaderException: cpw.mods.fml.common.LoaderException at cpw.mods.fml.common.ProxyInjector.inject(ProxyInjector.java:75) at cpw.mods.fml.common.FMLModContainer.constructMod(FMLModContainer.java:519) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:57) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:601) at com.google.common.eventbus.EventHandler.handleEvent(EventHandler.java:74) at com.google.common.eventbus.SynchronizedEventHandler.handleEvent(SynchronizedEventHandler.java:45) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:313) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:296) at com.google.common.eventbus.EventBus.post(EventBus.java:267) at cpw.mods.fml.common.LoadController.sendEventToModContainer(LoadController.java:194) at cpw.mods.fml.common.LoadController.propogateStateMessage(LoadController.java:174) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:57) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:601) at com.google.common.eventbus.EventHandler.handleEvent(EventHandler.java:74) at com.google.common.eventbus.SynchronizedEventHandler.handleEvent(SynchronizedEventHandler.java:45) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:313) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:296) at com.google.common.eventbus.EventBus.post(EventBus.java:267) at cpw.mods.fml.common.LoadController.distributeStateMessage(LoadController.java:105) at cpw.mods.fml.common.Loader.loadMods(Loader.java:510) at cpw.mods.fml.client.FMLClientHandler.beginMinecraftLoading(FMLClientHandler.java:182) at net.minecraft.client.Minecraft.startGame(Minecraft.java:470) at net.minecraft.client.Minecraft.func_99999_d(Minecraft.java:796) at net.minecraft.client.main.Main.main(Main.java:93) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:57) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:601) at net.minecraft.launchwrapper.Launch.launch(Launch.java:57) at net.minecraft.launchwrapper.Launch.main(Launch.java:18) Caused by: cpw.mods.fml.common.LoaderException at cpw.mods.fml.common.ProxyInjector.inject(ProxyInjector.java:68) ... 33 more 2013-08-22 15:20:19 [sEVERE] [ForgeModLoader] Caught exception from commandblockrecipe cpw.mods.fml.common.LoaderException: cpw.mods.fml.common.LoaderException at cpw.mods.fml.common.ProxyInjector.inject(ProxyInjector.java:75) at cpw.mods.fml.common.FMLModContainer.constructMod(FMLModContainer.java:519) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:57) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:601) at com.google.common.eventbus.EventHandler.handleEvent(EventHandler.java:74) at com.google.common.eventbus.SynchronizedEventHandler.handleEvent(SynchronizedEventHandler.java:45) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:313) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:296) at com.google.common.eventbus.EventBus.post(EventBus.java:267) at cpw.mods.fml.common.LoadController.sendEventToModContainer(LoadController.java:194) at cpw.mods.fml.common.LoadController.propogateStateMessage(LoadController.java:174) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:57) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:601) at com.google.common.eventbus.EventHandler.handleEvent(EventHandler.java:74) at com.google.common.eventbus.SynchronizedEventHandler.handleEvent(SynchronizedEventHandler.java:45) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:313) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:296) at com.google.common.eventbus.EventBus.post(EventBus.java:267) at cpw.mods.fml.common.LoadController.distributeStateMessage(LoadController.java:105) at cpw.mods.fml.common.Loader.loadMods(Loader.java:510) at cpw.mods.fml.client.FMLClientHandler.beginMinecraftLoading(FMLClientHandler.java:182) at net.minecraft.client.Minecraft.startGame(Minecraft.java:470) at net.minecraft.client.Minecraft.func_99999_d(Minecraft.java:796) at net.minecraft.client.main.Main.main(Main.java:93) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:57) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:601) at net.minecraft.launchwrapper.Launch.launch(Launch.java:57) at net.minecraft.launchwrapper.Launch.main(Launch.java:18) Caused by: cpw.mods.fml.common.LoaderException at cpw.mods.fml.common.ProxyInjector.inject(ProxyInjector.java:68) ... 33 more 2013-08-22 15:20:19 [iNFO] [sTDOUT] ---- Minecraft Crash Report ---- 2013-08-22 15:20:19 [iNFO] [sTDOUT] // I bet Cylons wouldn't have this problem. 2013-08-22 15:20:19 [iNFO] [sTDOUT] 2013-08-22 15:20:19 [iNFO] [sTDOUT] Time: 8/22/13 3:20 PM 2013-08-22 15:20:19 [iNFO] [sTDOUT] Description: There was a severe problem during mod loading that has caused the game to fail 2013-08-22 15:20:19 [iNFO] [sTDOUT] 2013-08-22 15:20:19 [iNFO] [sTDOUT] cpw.mods.fml.common.LoaderException: cpw.mods.fml.common.LoaderException 2013-08-22 15:20:19 [iNFO] [sTDOUT] at cpw.mods.fml.common.ProxyInjector.inject(ProxyInjector.java:75) 2013-08-22 15:20:19 [iNFO] [sTDOUT] at cpw.mods.fml.common.FMLModContainer.constructMod(FMLModContainer.java:519) 2013-08-22 15:20:19 [iNFO] [sTDOUT] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) 2013-08-22 15:20:19 [iNFO] [sTDOUT] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:57) 2013-08-22 15:20:19 [iNFO] [sTDOUT] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) 2013-08-22 15:20:19 [iNFO] [sTDOUT] at java.lang.reflect.Method.invoke(Method.java:601) 2013-08-22 15:20:19 [iNFO] [sTDOUT] at com.google.common.eventbus.EventHandler.handleEvent(EventHandler.java:74) 2013-08-22 15:20:19 [iNFO] [sTDOUT] at com.google.common.eventbus.SynchronizedEventHandler.handleEvent(SynchronizedEventHandler.java:45) 2013-08-22 15:20:19 [iNFO] [sTDOUT] at com.google.common.eventbus.EventBus.dispatch(EventBus.java:313) 2013-08-22 15:20:19 [iNFO] [sTDOUT] at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:296) 2013-08-22 15:20:19 [iNFO] [sTDOUT] at com.google.common.eventbus.EventBus.post(EventBus.java:267) 2013-08-22 15:20:19 [iNFO] [sTDOUT] at cpw.mods.fml.common.LoadController.sendEventToModContainer(LoadController.java:194) 2013-08-22 15:20:19 [iNFO] [sTDOUT] at cpw.mods.fml.common.LoadController.propogateStateMessage(LoadController.java:174) 2013-08-22 15:20:19 [iNFO] [sTDOUT] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) 2013-08-22 15:20:19 [iNFO] [sTDOUT] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:57) 2013-08-22 15:20:19 [iNFO] [sTDOUT] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) 2013-08-22 15:20:19 [iNFO] [sTDOUT] at java.lang.reflect.Method.invoke(Method.java:601) 2013-08-22 15:20:19 [iNFO] [sTDOUT] at com.google.common.eventbus.EventHandler.handleEvent(EventHandler.java:74) 2013-08-22 15:20:19 [iNFO] [sTDOUT] at com.google.common.eventbus.SynchronizedEventHandler.handleEvent(SynchronizedEventHandler.java:45) 2013-08-22 15:20:19 [iNFO] [sTDOUT] at com.google.common.eventbus.EventBus.dispatch(EventBus.java:313) 2013-08-22 15:20:19 [iNFO] [sTDOUT] at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:296) 2013-08-22 15:20:19 [iNFO] [sTDOUT] at com.google.common.eventbus.EventBus.post(EventBus.java:267) 2013-08-22 15:20:19 [iNFO] [sTDOUT] at cpw.mods.fml.common.LoadController.distributeStateMessage(LoadController.java:105) 2013-08-22 15:20:19 [iNFO] [sTDOUT] at cpw.mods.fml.common.Loader.loadMods(Loader.java:510) 2013-08-22 15:20:19 [iNFO] [sTDOUT] at cpw.mods.fml.client.FMLClientHandler.beginMinecraftLoading(FMLClientHandler.java:182) 2013-08-22 15:20:19 [iNFO] [sTDOUT] at net.minecraft.client.Minecraft.startGame(Minecraft.java:470) 2013-08-22 15:20:19 [iNFO] [sTDOUT] at net.minecraft.client.Minecraft.func_99999_d(Minecraft.java:796) 2013-08-22 15:20:19 [iNFO] [sTDOUT] at net.minecraft.client.main.Main.main(Main.java:93) 2013-08-22 15:20:19 [iNFO] [sTDOUT] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) 2013-08-22 15:20:19 [iNFO] [sTDOUT] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:57) 2013-08-22 15:20:19 [iNFO] [sTDOUT] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) 2013-08-22 15:20:19 [iNFO] [sTDOUT] at java.lang.reflect.Method.invoke(Method.java:601) 2013-08-22 15:20:19 [iNFO] [sTDOUT] at net.minecraft.launchwrapper.Launch.launch(Launch.java:57) 2013-08-22 15:20:19 [iNFO] [sTDOUT] at net.minecraft.launchwrapper.Launch.main(Launch.java:18) 2013-08-22 15:20:19 [iNFO] [sTDOUT] Caused by: cpw.mods.fml.common.LoaderException 2013-08-22 15:20:19 [iNFO] [sTDOUT] at cpw.mods.fml.common.ProxyInjector.inject(ProxyInjector.java:68) 2013-08-22 15:20:19 [iNFO] [sTDOUT] ... 33 more 2013-08-22 15:20:19 [iNFO] [sTDOUT] 2013-08-22 15:20:19 [iNFO] [sTDOUT] 2013-08-22 15:20:19 [iNFO] [sTDOUT] A detailed walkthrough of the error, its code path and all known details is as follows: 2013-08-22 15:20:19 [iNFO] [sTDOUT] --------------------------------------------------------------------------------------- 2013-08-22 15:20:19 [iNFO] [sTDOUT] 2013-08-22 15:20:19 [iNFO] [sTDOUT] -- System Details -- 2013-08-22 15:20:19 [iNFO] [sTDOUT] Details: 2013-08-22 15:20:19 [iNFO] [sTDOUT] Minecraft Version: 1.6.2 2013-08-22 15:20:19 [iNFO] [sTDOUT] Operating System: Windows 7 (amd64) version 6.1 2013-08-22 15:20:19 [iNFO] [sTDOUT] Java Version: 1.7.0_02, Oracle Corporation 2013-08-22 15:20:19 [iNFO] [sTDOUT] Java VM Version: Java HotSpot(TM) 64-Bit Server VM (mixed mode), Oracle Corporation 2013-08-22 15:20:19 [iNFO] [sTDOUT] Memory: 777302888 bytes (741 MB) / 1038876672 bytes (990 MB) up to 1038876672 bytes (990 MB) 2013-08-22 15:20:19 [iNFO] [sTDOUT] JVM Flags: 3 total; -Xincgc -Xmx1024M -Xms1024M 2013-08-22 15:20:19 [iNFO] [sTDOUT] AABB Pool Size: 0 (0 bytes; 0 MB) allocated, 0 (0 bytes; 0 MB) used 2013-08-22 15:20:19 [iNFO] [sTDOUT] Suspicious classes: FML and Forge are installed 2013-08-22 15:20:19 [iNFO] [sTDOUT] IntCache: cache: 0, tcache: 0, allocated: 0, tallocated: 0 2013-08-22 15:20:19 [iNFO] [sTDOUT] FML: MCP v8.04 FML v6.2.35.804 Minecraft Forge 9.10.0.804 5 mods loaded, 5 mods active 2013-08-22 15:20:19 [iNFO] [sTDOUT] mcp{8.04} [Minecraft Coder Pack] (minecraft.jar) Unloaded->Constructed 2013-08-22 15:20:19 [iNFO] [sTDOUT] FML{6.2.35.804} [Forge Mod Loader] (coremods) Unloaded->Constructed 2013-08-22 15:20:19 [iNFO] [sTDOUT] Forge{9.10.0.804} [Minecraft Forge] (coremods) Unloaded->Constructed 2013-08-22 15:20:19 [iNFO] [sTDOUT] commandblockrecipe{0.0.1} [CommandBlockRecipe] (bin) Unloaded->Errored 2013-08-22 15:20:19 [iNFO] [sTDOUT] witchcraft{0.0.1} [WitchCraft] (bin) Unloaded->Errored 2013-08-22 15:20:19 [iNFO] [sTDOUT] #@!@# Game crashed! Crash report saved to: #@!@# C:\Users\Kyle\Downloads\minecraftforge-src-1.6.2-9.10.0.804\forge\mcp\jars\.\crash-reports\crash-2013-08-22_15.20.19-client.txt
-
Use lowercase for packages and modid.
Thanks for the reply, okay well here is my error log now. Seems to still be along the same lines.
2013-08-22 15:11:35 [sEVERE] [ForgeModLoader] An error occured trying to load a proxy into {clientSide=kmccmk9.witchCraft.client.ClientProxy, serverSide=kmccmk9.witchCraft.CommonProxy}.kmccmk9.witchcraft.WitchCraft java.lang.ClassNotFoundException: kmccmk9.witchCraft.client.ClientProxy at net.minecraft.launchwrapper.LaunchClassLoader.findClass(LaunchClassLoader.java:179) at java.lang.ClassLoader.loadClass(ClassLoader.java:423) at java.lang.ClassLoader.loadClass(ClassLoader.java:356) at cpw.mods.fml.common.ModClassLoader.loadClass(ModClassLoader.java:58) at java.lang.Class.forName0(Native Method) at java.lang.Class.forName(Class.java:264) at cpw.mods.fml.common.ProxyInjector.inject(ProxyInjector.java:58) at cpw.mods.fml.common.FMLModContainer.constructMod(FMLModContainer.java:519) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:57) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:601) at com.google.common.eventbus.EventHandler.handleEvent(EventHandler.java:74) at com.google.common.eventbus.SynchronizedEventHandler.handleEvent(SynchronizedEventHandler.java:45) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:313) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:296) at com.google.common.eventbus.EventBus.post(EventBus.java:267) at cpw.mods.fml.common.LoadController.sendEventToModContainer(LoadController.java:194) at cpw.mods.fml.common.LoadController.propogateStateMessage(LoadController.java:174) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:57) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:601) at com.google.common.eventbus.EventHandler.handleEvent(EventHandler.java:74) at com.google.common.eventbus.SynchronizedEventHandler.handleEvent(SynchronizedEventHandler.java:45) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:313) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:296) at com.google.common.eventbus.EventBus.post(EventBus.java:267) at cpw.mods.fml.common.LoadController.distributeStateMessage(LoadController.java:105) at cpw.mods.fml.common.Loader.loadMods(Loader.java:510) at cpw.mods.fml.client.FMLClientHandler.beginMinecraftLoading(FMLClientHandler.java:182) at net.minecraft.client.Minecraft.startGame(Minecraft.java:470) at net.minecraft.client.Minecraft.func_99999_d(Minecraft.java:796) at net.minecraft.client.main.Main.main(Main.java:93) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:57) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:601) at net.minecraft.launchwrapper.Launch.launch(Launch.java:57) at net.minecraft.launchwrapper.Launch.main(Launch.java:18) Caused by: java.lang.NoClassDefFoundError: kmccmk9/witchCraft/client/ClientProxy (wrong name: kmccmk9/witchcraft/client/ClientProxy) at java.lang.ClassLoader.defineClass1(Native Method) at java.lang.ClassLoader.defineClass(ClassLoader.java:791) at java.security.SecureClassLoader.defineClass(SecureClassLoader.java:142) at net.minecraft.launchwrapper.LaunchClassLoader.findClass(LaunchClassLoader.java:171) ... 39 more 2013-08-22 15:11:35 [FINEST] [commandblockrecipe] Sent event FMLConstructionEvent to mod commandblockrecipe 2013-08-22 15:11:35 [FINEST] [witchcraft] Sending event FMLConstructionEvent to mod witchcraft 2013-08-22 15:11:35 [FINEST] [ForgeModLoader] Testing mod witchcraft to verify it accepts its own version in a remote connection 2013-08-22 15:11:35 [FINEST] [ForgeModLoader] The mod witchcraft accepts its own version (0.0.1) 2013-08-22 15:11:35 [FINE] [ForgeModLoader] Attempting to inject @SidedProxy classes into witchcraft 2013-08-22 15:11:35 [sEVERE] [ForgeModLoader] An error occured trying to load a proxy into {clientSide=kmccmk9.witchCraft.client.ClientProxy, serverSide=kmccmk9.witchCraft.CommonProxy}.kmccmk9.witchcraft.WitchCraft java.lang.ClassNotFoundException: kmccmk9.witchCraft.client.ClientProxy at net.minecraft.launchwrapper.LaunchClassLoader.findClass(LaunchClassLoader.java:94) at java.lang.ClassLoader.loadClass(ClassLoader.java:423) at java.lang.ClassLoader.loadClass(ClassLoader.java:356) at cpw.mods.fml.common.ModClassLoader.loadClass(ModClassLoader.java:58) at java.lang.Class.forName0(Native Method) at java.lang.Class.forName(Class.java:264) at cpw.mods.fml.common.ProxyInjector.inject(ProxyInjector.java:58) at cpw.mods.fml.common.FMLModContainer.constructMod(FMLModContainer.java:519) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:57) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:601) at com.google.common.eventbus.EventHandler.handleEvent(EventHandler.java:74) at com.google.common.eventbus.SynchronizedEventHandler.handleEvent(SynchronizedEventHandler.java:45) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:313) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:296) at com.google.common.eventbus.EventBus.post(EventBus.java:267) at cpw.mods.fml.common.LoadController.sendEventToModContainer(LoadController.java:194) at cpw.mods.fml.common.LoadController.propogateStateMessage(LoadController.java:174) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:57) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:601) at com.google.common.eventbus.EventHandler.handleEvent(EventHandler.java:74) at com.google.common.eventbus.SynchronizedEventHandler.handleEvent(SynchronizedEventHandler.java:45) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:313) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:296) at com.google.common.eventbus.EventBus.post(EventBus.java:267) at cpw.mods.fml.common.LoadController.distributeStateMessage(LoadController.java:105) at cpw.mods.fml.common.Loader.loadMods(Loader.java:510) at cpw.mods.fml.client.FMLClientHandler.beginMinecraftLoading(FMLClientHandler.java:182) at net.minecraft.client.Minecraft.startGame(Minecraft.java:470) at net.minecraft.client.Minecraft.func_99999_d(Minecraft.java:796) at net.minecraft.client.main.Main.main(Main.java:93) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:57) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:601) at net.minecraft.launchwrapper.Launch.launch(Launch.java:57) at net.minecraft.launchwrapper.Launch.main(Launch.java:18) 2013-08-22 15:11:35 [FINEST] [witchcraft] Sent event FMLConstructionEvent to mod witchcraft 2013-08-22 15:11:35 [FINE] [ForgeModLoader] Mod signature data 2013-08-22 15:11:35 [FINE] [ForgeModLoader] mcp(Minecraft Coder Pack:8.04): minecraft.jar (NO VALID CERTIFICATE FOUND) 2013-08-22 15:11:35 [FINE] [ForgeModLoader] FML(Forge Mod Loader:6.2.35.804): coremods (NO VALID CERTIFICATE FOUND) 2013-08-22 15:11:35 [FINE] [ForgeModLoader] Forge(Minecraft Forge:9.10.0.804): coremods (NO VALID CERTIFICATE FOUND) 2013-08-22 15:11:35 [FINE] [ForgeModLoader] commandblockrecipe(CommandBlockRecipe:0.0.1): bin (NO VALID CERTIFICATE FOUND) 2013-08-22 15:11:35 [FINE] [ForgeModLoader] witchcraft(WitchCraft:0.0.1): bin (NO VALID CERTIFICATE FOUND) 2013-08-22 15:11:35 [sEVERE] [ForgeModLoader] Fatal errors were detected during the transition from CONSTRUCTING to PREINITIALIZATION. Loading cannot continue 2013-08-22 15:11:35 [sEVERE] [ForgeModLoader] mcp{8.04} [Minecraft Coder Pack] (minecraft.jar) Unloaded->Constructed FML{6.2.35.804} [Forge Mod Loader] (coremods) Unloaded->Constructed Forge{9.10.0.804} [Minecraft Forge] (coremods) Unloaded->Constructed commandblockrecipe{0.0.1} [CommandBlockRecipe] (bin) Unloaded->Errored witchcraft{0.0.1} [WitchCraft] (bin) Unloaded->Errored 2013-08-22 15:11:35 [sEVERE] [ForgeModLoader] The following problems were captured during this phase 2013-08-22 15:11:35 [sEVERE] [ForgeModLoader] Caught exception from witchcraft cpw.mods.fml.common.LoaderException: java.lang.ClassNotFoundException: kmccmk9.witchCraft.client.ClientProxy at cpw.mods.fml.common.ProxyInjector.inject(ProxyInjector.java:75) at cpw.mods.fml.common.FMLModContainer.constructMod(FMLModContainer.java:519) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:57) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:601) at com.google.common.eventbus.EventHandler.handleEvent(EventHandler.java:74) at com.google.common.eventbus.SynchronizedEventHandler.handleEvent(SynchronizedEventHandler.java:45) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:313) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:296) at com.google.common.eventbus.EventBus.post(EventBus.java:267) at cpw.mods.fml.common.LoadController.sendEventToModContainer(LoadController.java:194) at cpw.mods.fml.common.LoadController.propogateStateMessage(LoadController.java:174) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:57) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:601) at com.google.common.eventbus.EventHandler.handleEvent(EventHandler.java:74) at com.google.common.eventbus.SynchronizedEventHandler.handleEvent(SynchronizedEventHandler.java:45) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:313) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:296) at com.google.common.eventbus.EventBus.post(EventBus.java:267) at cpw.mods.fml.common.LoadController.distributeStateMessage(LoadController.java:105) at cpw.mods.fml.common.Loader.loadMods(Loader.java:510) at cpw.mods.fml.client.FMLClientHandler.beginMinecraftLoading(FMLClientHandler.java:182) at net.minecraft.client.Minecraft.startGame(Minecraft.java:470) at net.minecraft.client.Minecraft.func_99999_d(Minecraft.java:796) at net.minecraft.client.main.Main.main(Main.java:93) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:57) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:601) at net.minecraft.launchwrapper.Launch.launch(Launch.java:57) at net.minecraft.launchwrapper.Launch.main(Launch.java:18) Caused by: java.lang.ClassNotFoundException: kmccmk9.witchCraft.client.ClientProxy at net.minecraft.launchwrapper.LaunchClassLoader.findClass(LaunchClassLoader.java:94) at java.lang.ClassLoader.loadClass(ClassLoader.java:423) at java.lang.ClassLoader.loadClass(ClassLoader.java:356) at cpw.mods.fml.common.ModClassLoader.loadClass(ModClassLoader.java:58) at java.lang.Class.forName0(Native Method) at java.lang.Class.forName(Class.java:264) at cpw.mods.fml.common.ProxyInjector.inject(ProxyInjector.java:58) ... 33 more 2013-08-22 15:11:35 [sEVERE] [ForgeModLoader] Caught exception from commandblockrecipe cpw.mods.fml.common.LoaderException: java.lang.ClassNotFoundException: kmccmk9.witchCraft.client.ClientProxy at cpw.mods.fml.common.ProxyInjector.inject(ProxyInjector.java:75) at cpw.mods.fml.common.FMLModContainer.constructMod(FMLModContainer.java:519) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:57) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:601) at com.google.common.eventbus.EventHandler.handleEvent(EventHandler.java:74) at com.google.common.eventbus.SynchronizedEventHandler.handleEvent(SynchronizedEventHandler.java:45) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:313) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:296) at com.google.common.eventbus.EventBus.post(EventBus.java:267) at cpw.mods.fml.common.LoadController.sendEventToModContainer(LoadController.java:194) at cpw.mods.fml.common.LoadController.propogateStateMessage(LoadController.java:174) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:57) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:601) at com.google.common.eventbus.EventHandler.handleEvent(EventHandler.java:74) at com.google.common.eventbus.SynchronizedEventHandler.handleEvent(SynchronizedEventHandler.java:45) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:313) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:296) at com.google.common.eventbus.EventBus.post(EventBus.java:267) at cpw.mods.fml.common.LoadController.distributeStateMessage(LoadController.java:105) at cpw.mods.fml.common.Loader.loadMods(Loader.java:510) at cpw.mods.fml.client.FMLClientHandler.beginMinecraftLoading(FMLClientHandler.java:182) at net.minecraft.client.Minecraft.startGame(Minecraft.java:470) at net.minecraft.client.Minecraft.func_99999_d(Minecraft.java:796) at net.minecraft.client.main.Main.main(Main.java:93) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:57) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:601) at net.minecraft.launchwrapper.Launch.launch(Launch.java:57) at net.minecraft.launchwrapper.Launch.main(Launch.java:18) Caused by: java.lang.ClassNotFoundException: kmccmk9.witchCraft.client.ClientProxy at net.minecraft.launchwrapper.LaunchClassLoader.findClass(LaunchClassLoader.java:179) at java.lang.ClassLoader.loadClass(ClassLoader.java:423) at java.lang.ClassLoader.loadClass(ClassLoader.java:356) at cpw.mods.fml.common.ModClassLoader.loadClass(ModClassLoader.java:58) at java.lang.Class.forName0(Native Method) at java.lang.Class.forName(Class.java:264) at cpw.mods.fml.common.ProxyInjector.inject(ProxyInjector.java:58) ... 33 more Caused by: java.lang.NoClassDefFoundError: kmccmk9/witchCraft/client/ClientProxy (wrong name: kmccmk9/witchcraft/client/ClientProxy) at java.lang.ClassLoader.defineClass1(Native Method) at java.lang.ClassLoader.defineClass(ClassLoader.java:791) at java.security.SecureClassLoader.defineClass(SecureClassLoader.java:142) at net.minecraft.launchwrapper.LaunchClassLoader.findClass(LaunchClassLoader.java:171) ... 39 more 2013-08-22 15:11:35 [iNFO] [sTDOUT] ---- Minecraft Crash Report ---- 2013-08-22 15:11:35 [iNFO] [sTDOUT] // This doesn't make any sense! 2013-08-22 15:11:35 [iNFO] [sTDOUT] 2013-08-22 15:11:35 [iNFO] [sTDOUT] Time: 8/22/13 3:11 PM 2013-08-22 15:11:35 [iNFO] [sTDOUT] Description: There was a severe problem during mod loading that has caused the game to fail 2013-08-22 15:11:35 [iNFO] [sTDOUT] 2013-08-22 15:11:35 [iNFO] [sTDOUT] cpw.mods.fml.common.LoaderException: java.lang.ClassNotFoundException: kmccmk9.witchCraft.client.ClientProxy 2013-08-22 15:11:35 [iNFO] [sTDOUT] at cpw.mods.fml.common.ProxyInjector.inject(ProxyInjector.java:75) 2013-08-22 15:11:35 [iNFO] [sTDOUT] at cpw.mods.fml.common.FMLModContainer.constructMod(FMLModContainer.java:519) 2013-08-22 15:11:35 [iNFO] [sTDOUT] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) 2013-08-22 15:11:35 [iNFO] [sTDOUT] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:57) 2013-08-22 15:11:35 [iNFO] [sTDOUT] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) 2013-08-22 15:11:35 [iNFO] [sTDOUT] at java.lang.reflect.Method.invoke(Method.java:601) 2013-08-22 15:11:35 [iNFO] [sTDOUT] at com.google.common.eventbus.EventHandler.handleEvent(EventHandler.java:74) 2013-08-22 15:11:35 [iNFO] [sTDOUT] at com.google.common.eventbus.SynchronizedEventHandler.handleEvent(SynchronizedEventHandler.java:45) 2013-08-22 15:11:35 [iNFO] [sTDOUT] at com.google.common.eventbus.EventBus.dispatch(EventBus.java:313) 2013-08-22 15:11:35 [iNFO] [sTDOUT] at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:296) 2013-08-22 15:11:35 [iNFO] [sTDOUT] at com.google.common.eventbus.EventBus.post(EventBus.java:267) 2013-08-22 15:11:35 [iNFO] [sTDOUT] at cpw.mods.fml.common.LoadController.sendEventToModContainer(LoadController.java:194) 2013-08-22 15:11:35 [iNFO] [sTDOUT] at cpw.mods.fml.common.LoadController.propogateStateMessage(LoadController.java:174) 2013-08-22 15:11:35 [iNFO] [sTDOUT] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) 2013-08-22 15:11:35 [iNFO] [sTDOUT] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:57) 2013-08-22 15:11:35 [iNFO] [sTDOUT] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) 2013-08-22 15:11:35 [iNFO] [sTDOUT] at java.lang.reflect.Method.invoke(Method.java:601) 2013-08-22 15:11:35 [iNFO] [sTDOUT] at com.google.common.eventbus.EventHandler.handleEvent(EventHandler.java:74) 2013-08-22 15:11:35 [iNFO] [sTDOUT] at com.google.common.eventbus.SynchronizedEventHandler.handleEvent(SynchronizedEventHandler.java:45) 2013-08-22 15:11:35 [iNFO] [sTDOUT] at com.google.common.eventbus.EventBus.dispatch(EventBus.java:313) 2013-08-22 15:11:35 [iNFO] [sTDOUT] at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:296) 2013-08-22 15:11:35 [iNFO] [sTDOUT] at com.google.common.eventbus.EventBus.post(EventBus.java:267) 2013-08-22 15:11:35 [iNFO] [sTDOUT] at cpw.mods.fml.common.LoadController.distributeStateMessage(LoadController.java:105) 2013-08-22 15:11:35 [iNFO] [sTDOUT] at cpw.mods.fml.common.Loader.loadMods(Loader.java:510) 2013-08-22 15:11:35 [iNFO] [sTDOUT] at cpw.mods.fml.client.FMLClientHandler.beginMinecraftLoading(FMLClientHandler.java:182) 2013-08-22 15:11:35 [iNFO] [sTDOUT] at net.minecraft.client.Minecraft.startGame(Minecraft.java:470) 2013-08-22 15:11:35 [iNFO] [sTDOUT] at net.minecraft.client.Minecraft.func_99999_d(Minecraft.java:796) 2013-08-22 15:11:35 [iNFO] [sTDOUT] at net.minecraft.client.main.Main.main(Main.java:93) 2013-08-22 15:11:35 [iNFO] [sTDOUT] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) 2013-08-22 15:11:35 [iNFO] [sTDOUT] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:57) 2013-08-22 15:11:35 [iNFO] [sTDOUT] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) 2013-08-22 15:11:35 [iNFO] [sTDOUT] at java.lang.reflect.Method.invoke(Method.java:601) 2013-08-22 15:11:35 [iNFO] [sTDOUT] at net.minecraft.launchwrapper.Launch.launch(Launch.java:57) 2013-08-22 15:11:35 [iNFO] [sTDOUT] at net.minecraft.launchwrapper.Launch.main(Launch.java:18) 2013-08-22 15:11:35 [iNFO] [sTDOUT] Caused by: java.lang.ClassNotFoundException: kmccmk9.witchCraft.client.ClientProxy 2013-08-22 15:11:35 [iNFO] [sTDOUT] at net.minecraft.launchwrapper.LaunchClassLoader.findClass(LaunchClassLoader.java:94) 2013-08-22 15:11:35 [iNFO] [sTDOUT] at java.lang.ClassLoader.loadClass(ClassLoader.java:423) 2013-08-22 15:11:35 [iNFO] [sTDOUT] at java.lang.ClassLoader.loadClass(ClassLoader.java:356) 2013-08-22 15:11:35 [iNFO] [sTDOUT] at cpw.mods.fml.common.ModClassLoader.loadClass(ModClassLoader.java:58) 2013-08-22 15:11:35 [iNFO] [sTDOUT] at java.lang.Class.forName0(Native Method) 2013-08-22 15:11:35 [iNFO] [sTDOUT] at java.lang.Class.forName(Class.java:264) 2013-08-22 15:11:35 [iNFO] [sTDOUT] at cpw.mods.fml.common.ProxyInjector.inject(ProxyInjector.java:58) 2013-08-22 15:11:35 [iNFO] [sTDOUT] ... 33 more 2013-08-22 15:11:35 [iNFO] [sTDOUT] 2013-08-22 15:11:35 [iNFO] [sTDOUT] 2013-08-22 15:11:35 [iNFO] [sTDOUT] A detailed walkthrough of the error, its code path and all known details is as follows: 2013-08-22 15:11:35 [iNFO] [sTDOUT] --------------------------------------------------------------------------------------- 2013-08-22 15:11:35 [iNFO] [sTDOUT] 2013-08-22 15:11:35 [iNFO] [sTDOUT] -- System Details -- 2013-08-22 15:11:35 [iNFO] [sTDOUT] Details: 2013-08-22 15:11:35 [iNFO] [sTDOUT] Minecraft Version: 1.6.2 2013-08-22 15:11:35 [iNFO] [sTDOUT] Operating System: Windows 7 (amd64) version 6.1 2013-08-22 15:11:35 [iNFO] [sTDOUT] Java Version: 1.7.0_02, Oracle Corporation 2013-08-22 15:11:35 [iNFO] [sTDOUT] Java VM Version: Java HotSpot(TM) 64-Bit Server VM (mixed mode), Oracle Corporation 2013-08-22 15:11:35 [iNFO] [sTDOUT] Memory: 775779424 bytes (739 MB) / 1038876672 bytes (990 MB) up to 1038876672 bytes (990 MB) 2013-08-22 15:11:35 [iNFO] [sTDOUT] JVM Flags: 3 total; -Xincgc -Xmx1024M -Xms1024M 2013-08-22 15:11:35 [iNFO] [sTDOUT] AABB Pool Size: 0 (0 bytes; 0 MB) allocated, 0 (0 bytes; 0 MB) used 2013-08-22 15:11:35 [iNFO] [sTDOUT] Suspicious classes: FML and Forge are installed 2013-08-22 15:11:35 [iNFO] [sTDOUT] IntCache: cache: 0, tcache: 0, allocated: 0, tallocated: 0 2013-08-22 15:11:35 [iNFO] [sTDOUT] FML: MCP v8.04 FML v6.2.35.804 Minecraft Forge 9.10.0.804 5 mods loaded, 5 mods active 2013-08-22 15:11:35 [iNFO] [sTDOUT] mcp{8.04} [Minecraft Coder Pack] (minecraft.jar) Unloaded->Constructed 2013-08-22 15:11:35 [iNFO] [sTDOUT] FML{6.2.35.804} [Forge Mod Loader] (coremods) Unloaded->Constructed 2013-08-22 15:11:35 [iNFO] [sTDOUT] Forge{9.10.0.804} [Minecraft Forge] (coremods) Unloaded->Constructed 2013-08-22 15:11:35 [iNFO] [sTDOUT] commandblockrecipe{0.0.1} [CommandBlockRecipe] (bin) Unloaded->Errored 2013-08-22 15:11:35 [iNFO] [sTDOUT] witchcraft{0.0.1} [WitchCraft] (bin) Unloaded->Errored 2013-08-22 15:11:35 [iNFO] [sTDOUT] #@!@# Game crashed! Crash report saved to: #@!@# C:\Users\Kyle\Downloads\minecraftforge-src-1.6.2-9.10.0.804\forge\mcp\jars\.\crash-reports\crash-2013-08-22_15.11.35-client.txt
Running older mods? (Captain Sparklz Ex.)
in General Discussion
Posted
Oh duh you're right I'm sorry, I meant chicken bones. Well two of his mods but the essential ones like ender pouch and ender chests are not. Is there a different mod that would allow for dimension teleportation?