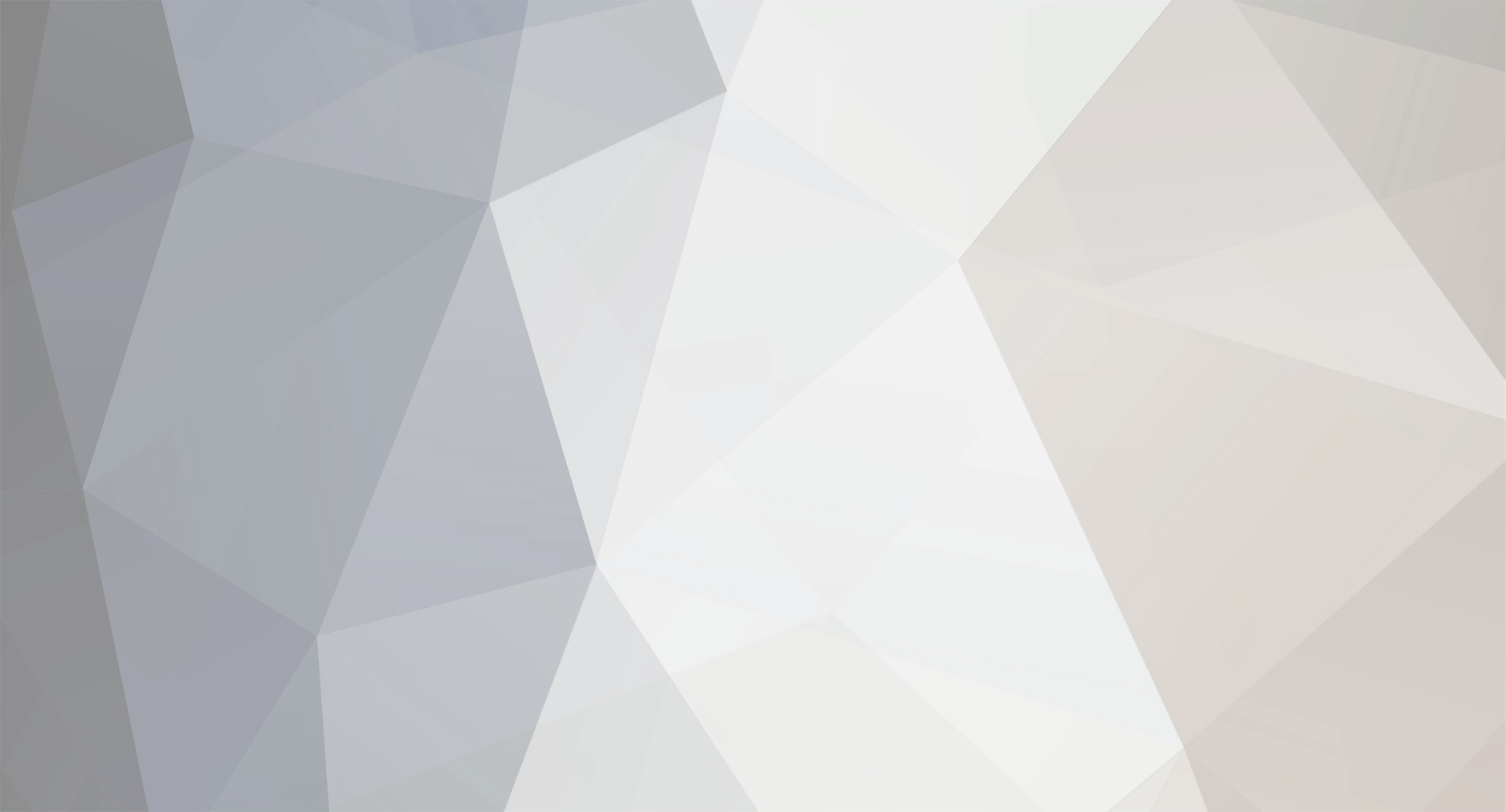
Kuuu
-
Posts
62 -
Joined
-
Last visited
Posts posted by Kuuu
-
-
Have you registered your generator?
-
Seems I removed registering line while was testing other things and forgot to return it back
thanks for help.
-
Hello, I'm updating my mod to 1.9, can't get GUIs to open.
onBlockActivated is called but GUI doesn't show up
@Override public boolean onBlockActivated(World worldIn, BlockPos pos, IBlockState state, EntityPlayer playerIn, EnumHand hand, ItemStack heldItem, EnumFacing side, float hitX, float hitY, float hitZ) { if (worldIn.isRemote) { return true; } else { System.out.println("onBlockActived was called! Trying to open GUI"); playerIn.openGui(More.instance, 0, worldIn, pos.getX(), pos.getY(), pos.getZ()); return true; } }
Here is my GuiHandler:
public class MoreGuiHandler implements IGuiHandler { @Override public Object getServerGuiElement(int id, EntityPlayer player, World world, int x, int y, int z) { BlockPos pos = new BlockPos(x, y, z); TileEntity tile_entity = world.getTileEntity(pos); switch (id) { case 0: return id == 0 && world.getBlockState(pos).getBlock() == MoreBlocks.inventor_table ? new ContainerInventorTable( player.inventory, world, pos) : null; case 1: return id == 1 && ((world.getBlockState(pos).getBlock() == MoreBlocks.uranium_furnace) || (world.getBlockState(pos).getBlock() == MoreBlocks.lit_uranium_furnace)) ? new ContainerUraniumFurnace( player.inventory, (TileEntityUraniumFurnace)tile_entity) : null; case 2: return id == 2 && ((world.getBlockState(pos).getBlock() == MoreBlocks.uranium_compressor) || (world.getBlockState(pos).getBlock() == MoreBlocks.lit_uranium_compressor)) ? new ContainerUraniumCompressor( player.inventory, (TileEntityUraniumCompressor) tile_entity) : null; } return null; } @Override public Object getClientGuiElement(int id, EntityPlayer player, World world, int x, int y, int z) { BlockPos pos = new BlockPos(x, y, z); TileEntity tile_entity = world.getTileEntity(pos); switch (id) { case 0: return id == 0 && world.getBlockState(pos).getBlock() == MoreBlocks.inventor_table ? new InventorTableGui( player.inventory, world) : null; case 1: return id == 1 && ((world.getBlockState(pos).getBlock() == MoreBlocks.uranium_furnace) || (world.getBlockState(pos).getBlock() == MoreBlocks.lit_uranium_furnace)) ? new UraniumFurnaceGui( player.inventory, (TileEntityUraniumFurnace)tile_entity) : null; case 2: return id == 2 && ((world.getBlockState(pos).getBlock() == MoreBlocks.uranium_compressor) || (world.getBlockState(pos).getBlock() == MoreBlocks.lit_uranium_compressor)) ? new UraniumCompressorGui( player.inventory, (TileEntityUraniumCompressor) tile_entity) : null; } return null; } }
Thanks in advance!
-
Put this in init()
ChestGenHooks.getInfo(ChestGenHooks.CATEGORY).addItem(new WeightedRandomChestContent(new ItemStack(YourItem),Min,Max,Rarity));
-
Awesome_Spider, I copied all the code from my working mod, so I can't understand what's the problem. Do you use big or small oak tree generation?
Hmm, try to remove IWorldGenerator implementation and remove this from MaztrixTreeGen:
@Override public void generate(Random random, int chunkX, int chunkZ, World world, IChunkProvider chunkGenerator, IChunkProvider chunkProvider) { int x = random.nextInt(16); int z = random.nextInt(16); this.generate(world, random, x, world.getHeightValue(x, z), z); }
-
Is it just me or would your code for the tree Y position have some nasty sideeffects?
int randPosY=random.nextInt(250); //Max Y coordinate
afaik, random.nextInt would give a random height from 0 to 250 in this case, and the tree should be on the ground level, not randomly flying or appearing underground which would be the case here.
It won't generate in air because MaztrixTreeGen should check if the block is grass, but i think i've got long world generating because of this, thanks, i'll use
int randPosY= world.getHeightValue(randPosX, randPosZ);
-
At first you have to make a class that implements IWorldGenerator and register it. Let's call it Generate.java:
Generate.java
package your.package; import java.util.Random; import net.minecraft.block.Block; import net.minecraft.world.World; import net.minecraft.world.biome.BiomeGenBase; import net.minecraft.world.chunk.IChunkProvider; import net.minecraft.world.gen.MapGenBase; import net.minecraft.world.gen.feature.WorldGenMinable; import cpw.mods.fml.common.IWorldGenerator; public class Generate implements IWorldGenerator { public void generate(Random random, int x, int z, World world, IChunkProvider chunkGenerator, IChunkProvider chunkProvider) { switch (world.provider.dimensionId) { case -1: generateNether(world, random, x * 16, z * 16); case 0: generateSurface(world, random, x * 16, z * 16); case 1: generateEnd(world, random, x * 16, z * 16); } } private void generateEnd(World world, Random random, int x, int z) { } private void generateSurface(World world, Random random, int x, int z) { } private void generateNether(World world, Random random, int x, int z) { } }
Then create a var to register it:
Main mod class:
Generate generate = new Generate();
And register it in init():
GameRegistry.registerWorldGenerator(generate, 0);
And in Generate.java you can generate your trees
Write this in generateSurface method:
for(int i = 0; i < 15; i++) // 15 is rarity { int randPosX=x + random.nextInt(16); int randPosY=random.nextInt(250); //Max Y coordinate int randPosZ=z + random.nextInt(16); (new MaztrixTreeGen(false)).generate(world, random, randPosX, randPosY, randPosZ); }
I hope it helps.
-
Thanks, it works now, here's the code if someone needs it:
@Override public boolean doesContainerItemLeaveCraftingGrid(ItemStack itemstack) { return true; } @Override public boolean isDamageable() { return true; } @Override public boolean hasContainerItem(ItemStack itemstack) { return true; } @Override public ItemStack getContainerItem(ItemStack itemStack) { ItemStack cStack = itemStack.copy(); cStack.setItemDamage(cStack.getItemDamage() + 1); cStack.stackSize = 1; return cStack; }
-
You don't need to write shape for shapeless recipe
new Object[]{"SCO"}
just do this:
GameRegistry.addShapelessRecipe(new ItemStack(Items.diamond, 1), new Object[]{Blocks.stone,MBlocks.ComStone, Blocks.obsidian});
-
Hello. I'm trying to make it so my tool will get back in inventory with little damage after crafting.
I have this code:
@Override public boolean doesContainerItemLeaveCraftingGrid(ItemStack itemstack) { return true; } @Override public ItemStack getContainerItem(ItemStack itemStack) { ItemStack cStack = itemStack.copy(); cStack.setItemDamage(cStack.getItemDamage() + 1); cStack.stackSize = 1; return cStack; }
But i don't get the item back after crafting, and i can't understand why. Please help.
-
Fixed, the problem was in Minions mod.
-
Hello. I want to play minecraft 1.7.2 with mods on my server, I installed cauldron-1.7.2-1.1147.04.91-server and some mods:
Archimedes Ships
BiblioCraft
BuildCraft
Carpenters Blocks
Flat Bedrock
Forestry
Industrial Craft
GregTech
Minions
Parachute Mod
RailCraft
When i join my server I get an error "Fatally missing blocks and items"
I re-created my world but still can't join.
here's server log:
[12:20:23 INFO]: Client attempting to join with 22 mods : gregtech@MC172,FlatBed [email protected],BuildCraft|[email protected],[email protected],[email protected]. 7,[email protected],BuildCraft|[email protected],BuildCraft|[email protected],Railcraft @9.1.0.0,BuildCraft|[email protected],[email protected],[email protected], BuildCraft|[email protected],[email protected],[email protected],[email protected],AS_Mini [email protected],[email protected],[email protected] v1.7.0,BuildCraft|Build [email protected],[email protected],[email protected] [12:20:23 INFO]: Attempting connection with missing mods [] at CLIENT [12:20:24 INFO]: ya_kudinov lost connection: Disconnected
-
Replace EnumToolMaterial with ToolMaterial
-
Tried these both, but it didn't work, I think my problem is in another place.
I'll create a new topic and will post all code in it.
Thanks!
-
I need to know if the item isRepairable.
in 1.6.4 I used this:
Item.itemsList[var4.itemID].isRepairable()
Item.field_150901_e.getObject() //this returns Object, I need something that will return Item
-
I know what there's no more itemID
-
Hello!
I need to get an Item from list of Items, in 1.6.4 I used:
Item.itemsList[itemstack.itemID];
But now I can't find itemsList in Item.class
-
Hello!
I'm updating my mod from 1.6.4 to 1.7.2
In this mod I have a block with gui, onContainerClosed I need to drop an item, in 1.6.4 I used:
entityplayer.dropPlayerItem(itemstack);
now dropPlayerItem doesn't exist and I couldn't find similar function.
-
-
Hello!
I need to compare my block with block, which is at x,y,z
I used this in 1.6.4:
if(worldObj.getBlockId(posX, posY, posZ) != MyMod.MyBlock)
but in 1.7 I can't get id of my block
I couldn't find function in World.java what takes coords and returns Block
-
-
Thanks for reply!
PlayerEvent.ItemCraftedEvent() //Did you mean this?
It takes 3 args: player, itemstack, iinventory
I just need to register my crafting handler
I used
GameRegistry.registerCraftingHandler(new MyCraftingHandler());
It needs only my crafting handler to register it
-
Hello!
How can I register crafting handler in 1.7.2? In 1.6.4 I used
GameRegistry.registerCraftingHandler();
But now registerCraftingHandler() doesn't exist in GameRegistry
Thanks in advance!
-
Take a look at Cauldron code
Modding help?
in Modder Support
Posted
Make sure your folder with textures has lower case letters. Your modid is 'MTM', folder in assets should be called 'mtm'.