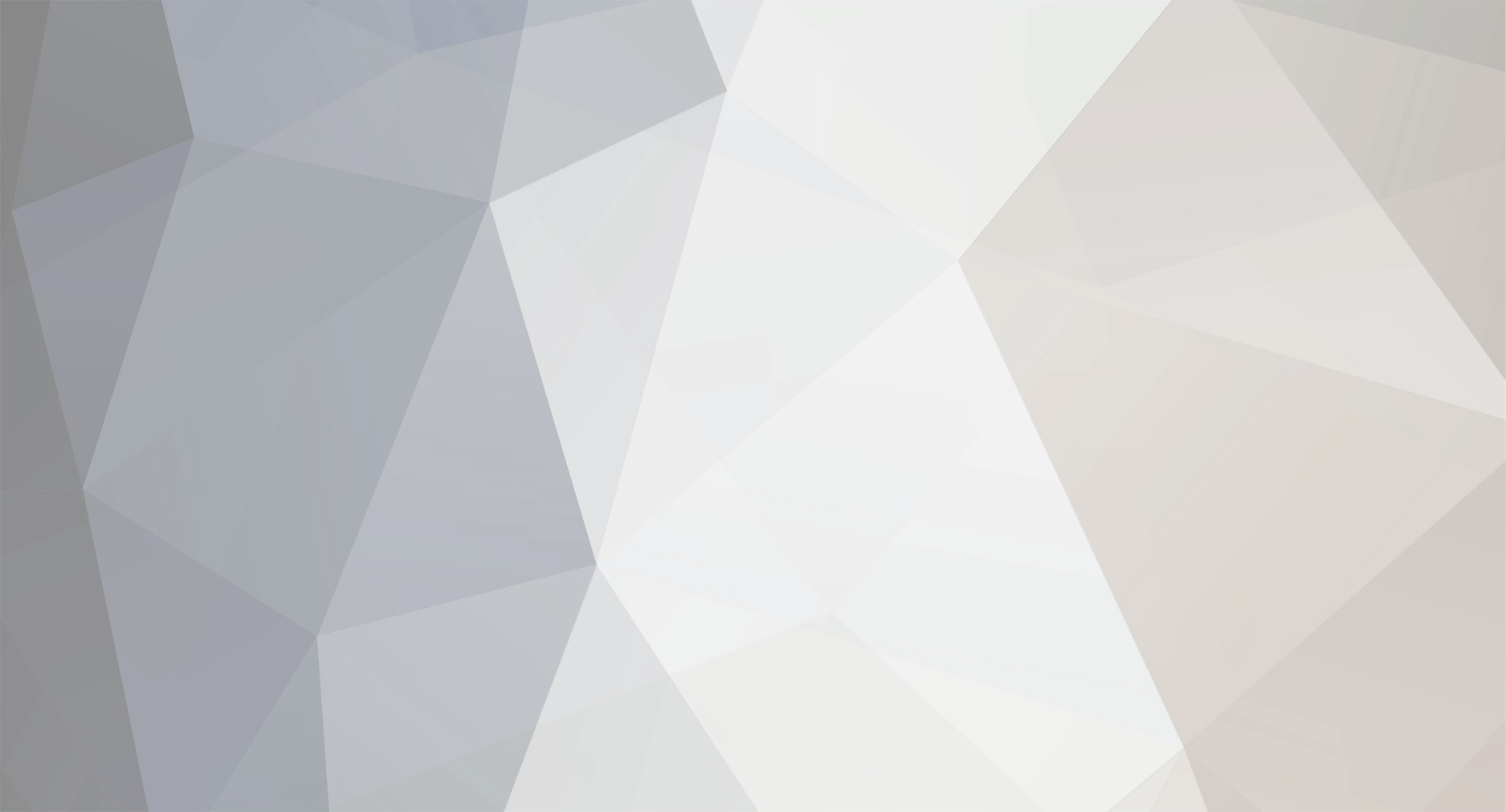
Russoul
-
Posts
27 -
Joined
-
Last visited
Posts posted by Russoul
-
-
Nope, that's not the case
-
I'm writing a custom obj model loader/renderer, not using the existing one because i want to load models dynamically, bypassing ResourceLocation thing + just want to do it myself.
I'm using simple minecraft Tessellator to render a whole 3d object and as a result i'm getting a massive fps drop rendering high poly models. How can i fix that ?
-
AAAAAAAAaaaaaaaaaaaaaa what a derpy derp !!!
I'm so sorry for that stupid post
-
I'm always getting this error when trying to save my entity extended properties to nbt
java.lang.StackOverflowError
at java.io.DataOutputStream.writeByte(DataOutputStream.java:153) ~[?:1.7.0_67]
at net.minecraft.nbt.NBTTagCompound.func_150298_a(NBTTagCompound.java:469) ~[NBTTagCompound.class:?]
at net.minecraft.nbt.NBTTagCompound.write(NBTTagCompound.java:37) ~[NBTTagCompound.class:?]
at net.minecraft.nbt.NBTTagCompound.func_150298_a(NBTTagCompound.java:474) ~[NBTTagCompound.class:?]
at net.minecraft.nbt.NBTTagCompound.write(NBTTagCompound.java:37) ~[NBTTagCompound.class:?]
at net.minecraft.nbt.NBTTagCompound.func_150298_a(NBTTagCompound.java:474) ~[NBTTagCompound.class:?]
at net.minecraft.nbt.NBTTagCompound.write(NBTTagCompound.java:37) ~[NBTTagCompound.class:?]
Code:
public static void saveInventoryToExtendedProps(EntityPlayer player, InventoryEX inv){ InventoryProperties props = InventoryProperties.get(player); NBTTagCompound tag = new NBTTagCompound(); for(int i = 0;i<size;i++){ ItemStack stack = inv.getStackInSlot(i); if(stack != null)ChatUtils.sendMsg(player,""+i+" "+(stack == null ? "null":stack.getDisplayName())); if(stack != null)tag.setTag(i+"",stack.writeToNBT(tag)); } props.inventory = tag; }
tag is an nbt instance that is being saved.
What's wrong here ?
-
I want to use netty system to send a whole directory of files, their size should be unlimited Client -> Server. I know that netty can perform this kind of a task, but i just need to figure out how to do it ingame, using already established connection between Client and Server. In the worst case i would need to create a separate program that would deal with it.
-
As the subject says, may huge chunks of data (like long strings or even files) be sent via packets or not ? Time is not a problem. Or maybe another method of sending data between client and server ?
-
Got it ! Now it works !!! Thank you
-
Why do i need to do that ? These all are IIcon based ones, renderblocks takes an icon for each side.
I removed
if(pass != block.getRenderBlockPass()) return;
and got that http://i.imgur.com/Tkp0zdO.png
The textures are not derping anymore.
-
Hello !
I'm experiencing a rendring bug, can't imagine what can cause it, so any help ?
Screenshorts http://imgur.com/eNXMSDi,lhf944V,IHP6uuC#0
First img - when i look down, below these 2 multipart blocks
Second img - when i look a little bit above the first position
Third img - looking directly at the block
Code
/** * Render the dynamic, changing faces of this part and other gfx as in a TESR. * The Tessellator will need to be started if it is to be used. * @param pos The position of this block space relative to the renderer, same as x, y, z passed to TESR. * @param frame The partial interpolation frame value for animations between ticks * @param pass The render pass, 1 or 0 */ //This is a meth from ForgeMultipart rendering thing @SideOnly(Side.CLIENT) public void renderDynamic(Vector3 pos, float frame, int pass){ GL11.glPushMatrix(); GL11.glTranslated(pos.x, pos.y, pos.z); GL11.glTranslated((bounds.max.x-bounds.min.x)/2+bounds.min.x, (bounds.max.y-bounds.min.y)/2+bounds.min.y,(bounds.max.z-bounds.min.z)/2+ bounds.min.z); GL11.glRotated(rotX,1,0,0); GL11.glRotated(rotY,0,1,0); GL11.glRotated(rotZ,0,0,1); GL11.glScaled(bounds.max.x-bounds.min.x, bounds.max.y-bounds.min.y, bounds.max.z-bounds.min.z); RenderBlocks renderBlocks = new RenderBlocks(); renderBlocks.setRenderBoundsFromBlock(block); RenderBlockUtils.drawFaces(renderBlocks, null, this.getBlock().getIcon(0, blockMeta), this.getBlock().getIcon(1, blockMeta), this.getBlock().getIcon(2, blockMeta), this.getBlock().getIcon(3, blockMeta), this.getBlock().getIcon(4, blockMeta), this.getBlock().getIcon(5, blockMeta), true); GL11.glPopMatrix(); }
RenderBlockUtils.drawFaces(Args...)
public static void drawFaces(RenderBlocks renderblocks, Block block, IIcon i1, IIcon i2, IIcon i3, IIcon i4, IIcon i5, IIcon i6, boolean solidtop) { Tessellator tessellator = Tessellator.instance; GL11.glTranslatef(-0.5F, -0.5F, -0.5F); tessellator.startDrawingQuads(); tessellator.setNormal(0.0F, -1.0F, 0.0F); renderblocks.renderFaceYNeg(block, 0.0D, 0.0D, 0.0D, i1); tessellator.draw(); if (solidtop) GL11.glDisable(3008); tessellator.startDrawingQuads(); tessellator.setNormal(0.0F, 1.0F, 0.0F); renderblocks.renderFaceYPos(block, 0.0D, 0.0D, 0.0D, i2); tessellator.draw(); if (solidtop) GL11.glEnable(3008); tessellator.startDrawingQuads(); tessellator.setNormal(0.0F, 0.0F, 1.0F); renderblocks.renderFaceXNeg(block, 0.0D, 0.0D, 0.0D, i3); tessellator.draw(); tessellator.startDrawingQuads(); tessellator.setNormal(0.0F, 0.0F, -1.0F); renderblocks.renderFaceXPos(block, 0.0D, 0.0D, 0.0D, i4); tessellator.draw(); tessellator.startDrawingQuads(); tessellator.setNormal(1.0F, 0.0F, 0.0F); renderblocks.renderFaceZNeg(block, 0.0D, 0.0D, 0.0D, i5); tessellator.draw(); tessellator.startDrawingQuads(); tessellator.setNormal(-1.0F, 0.0F, 0.0F); renderblocks.renderFaceZPos(block, 0.0D, 0.0D, 0.0D, i6); tessellator.draw(); GL11.glTranslatef(0.5F, 0.5F, 0.5F); }
-
They are both my mods. I don't really want to make it dependent. If its not there, it just won't drop the item.
You listed Blockregistry, assuming I can use Itemregistry similar, but it doesn't look like it has as nice of methods.
Something like this? I know how to test for a mod and could do that before, but could I just test to see if it returned something?
Item drop = (Item) Item.itemRegistry.getObject("mymodmodi:hammer");
if (drop != null) {
}
if you don't find a good method of doing your stuff, you can always use reflection to check if your mod is available and if it is, get items or mobs for further usage
-
I had a link to this at one point but can't find it now.
I want to have a mob in one mod drop an item from another mod in certain cercumstances.
Anyone able to point me at a code snipet or example where this was done. I can find in minecraft how to load and item from a string, but i'm unsure of the context of that string. Is it modid:name?
You can make your mod dependant on both mods: mobs one and items one. Then link those mods to your IDE and use Item from needed mod using linked object for other mod mob drops
-
Thank you all for your answers ! I did deobfuscation manually, just figuring out what every func_blabla means, and that was not the best time
-
Any way of deobfuscating a 1.7.2 mod ?
-
I need to make a independent thread that can run an infinite loop while minecraft is up. But all my attempts led to the freeze of the game. Is there a way of doing that ?
EDIT: Managed to do it myself !
-
-
When i tried to build my mod with gradle, it errored with symbol: class 'SOME_KIND_OF_CLASS_FROM_SOME_KIND_OF_MOD'.
I m pretty sure that gradle don't compile my mod with a dependency correctly. So how do i setup one right ?
-
I need to draw my stuff on the item of another mod or draw overlay when the item is hold by specified entity . Event handlers, tickhandlers where should i start ? Any suggestions ?
-
Also if problem is defined right see this : http://www.minecraftforge.net/forum/index.php/topic,14250.msg73036.html
-
Without scanning all your code, im 99% sure that the cause is not syncing server-client, you should send a packet to client with data you need
-
I have some odd issues while rendering a hud
Here is my code from rendertickhander :
if (player.getCurrentEquippedItem() != null && !TCKeyHandler.radialActive && ThaumcraftApiHelper.isResearchComplete(player.username, "Complex Essentia Draw")){ if ((player.getCurrentEquippedItem().getItem() instanceof ItemWandCasting)){ ItemStack wand = player.getCurrentEquippedItem(); ItemWandCasting wandINTR = (ItemWandCasting) player.getCurrentEquippedItem().getItem(); GL11.glPushMatrix(); GL11.glClear(256); GL11.glMatrixMode(5889); GL11.glLoadIdentity(); GL11.glOrtho(0.0D, res.getScaledWidth_double(), res.getScaledHeight_double(), 0.0D, 1000.0D, 3000.0D); GL11.glMatrixMode(5888); GL11.glLoadIdentity(); int k = res.getScaledWidth(); int l = res.getScaledHeight(); ResourceLocation hud = new ResourceLocation("thaumcraft", "textures/gui/hud.png"); ResourceLocation utilR = new ResourceLocation("rs","textures/utils/stuff.png"); GL11.glTranslatef(k, 0, -2000.0F); GL11.glColor4f(1.0F, 1.0F, 1.0F, 1.0F); GL11.glEnable(3042); GL11.glBlendFunc(770, 771); GL11.glPushMatrix(); GL11.glScaled(0.5D, 0.5D, 0.5D); Minecraft.getMinecraft().renderEngine.bindTexture(hud); GL11.glTranslatef(-32, 32, 0); GL11.glRotatef(270, 0, 0, 1); GL11.glTranslatef(32, -32, 0); UtilsFX.drawTexturedQuad(-64, 0, 0, 0, 64, 64, -90.0D); GL11.glPopMatrix(); /*for testing GL11.glPushMatrix(); Minecraft.getMinecraft().renderEngine.bindTexture(utilR); GL11.glTranslatef(-32, 32, 0); UtilsFX.drawTexturedQuad(0,0, 10, 0, 1, 1, -90F); GL11.glPopMatrix(); */ Minecraft.getMinecraft().renderEngine.bindTexture(hud); int max = wandINTR.getMaxVis(wand); for(int i = 0;i<6;i++){ GL11.glPushMatrix(); GL11.glScaled(0.5D, 0.5D, 0.5D); GL11.glTranslatef(-32, 32, 0); GL11.glRotatef(105-i*24, 0, 0, 1); GL11.glTranslatef(-8+i*0.2F, 26, 0); GL11.glPushMatrix(); UtilsFX.drawTexturedQuad(0, 0, 72, 4, 16, 42, -90F); GL11.glPopMatrix(); if(player.getCurrentEquippedItem().stackTagCompound.hasKey(EDUtils.COMPLEX_POOL)){ NBTTagCompound taggy = player.getCurrentEquippedItem().stackTagCompound.getCompoundTag(EDUtils.COMPLEX_POOL); AspectList visA = new AspectList(); visA.readFromNBT(taggy); if(visA.getAspects().length-1 >= i && visA.getAspects()[i] != null){ GL11.glPushMatrix(); GL11.glPushMatrix(); int loc = (int)(30.0F * visA.getAmount(visA.getAspects()[i])/ max); Color ac = new Color(visA.getAspects()[i].getColor()); GL11.glColor4f(ac.getRed() / 255.0F, ac.getGreen() / 255.0F, ac.getBlue() / 255.0F, 0.8F); UtilsFX.drawTexturedQuad(4, 4, 104, 0, 8, loc, -90.0D); GL11.glColor4f(1.0F, 1.0F, 1.0F, 1.0F); GL11.glPopMatrix(); if(player.isSneaking()){ GL11.glPushMatrix(); GL11.glRotatef(-90, 0, 0, 1); Minecraft.getMinecraft().ingameGUI.drawCenteredString(Minecraft.getMinecraft().fontRenderer, Math.round(visA.getAmount(visA.getAspects()[i])/100)+"", -50, 50, 16777215); GL11.glPopMatrix(); } GL11.glPopMatrix(); } } GL11.glPopMatrix(); } GL11.glPopMatrix(); } }
I confess that a bunch of code is just pasted, i did only some basics.
Here is an issue :
http://s29.postimg.org/wi0c7exgn/2014_03_17_21_49_27.png
right top corner (not the locations of 100s, white scribble on the hud is the problem and it happens when player is sneaking)
-
You should replace your onrightclick method with onItemUseFirst and onUsingItemTick (see them in Item.class) and here is a sounds tut : http://www.minecraftforum.net/topic/1886370-forge16x-mazs-tutorials-custom-sounds-fluids190713/
Edit: some stuff maybe outdated, so try to search for the equivalents.
-
Hi
This link might give you some help on where to start looking.
http://greyminecraftcoder.blogspot.com.au/2013/10/user-input.html
-TGG
I looked at http://greyminecraftcoder.blogspot.com.au/2013/10/user-input.html and found out where that is done but here follows another question : i noticed that Container.slotclick is called twice in my case, the first call is MyContainer.slotclick and then follows
Container.slotclick, whats interesting that i don't call super.slotclick. And one more problem that can be ralated to the first one :
when i tried to print what is the side Client or Server im on than initing Container, it return CLIENT. Is that ok ? I call player.opengui from my packethandler class, packets work right .
-
I need to disable/block/restrict vanilla 1-9 keyboard clicking mechanics (swapping hotbar items with the clicked slot item) when my container is opened. I thought that its done in slotclick method of the Container.class but its not.
-
Don't use a Tickhandler. Use RenderGameOverlayEvent, then you can specify EventPriority.HIGHEST and your handler will be run first (or "as first as possible").
Thanks, this helped ! :0
How does thaumcraft's warding effect work?
in Modder Support
Posted
Find it out yourself. Download dev build of thaumcraft and scan it