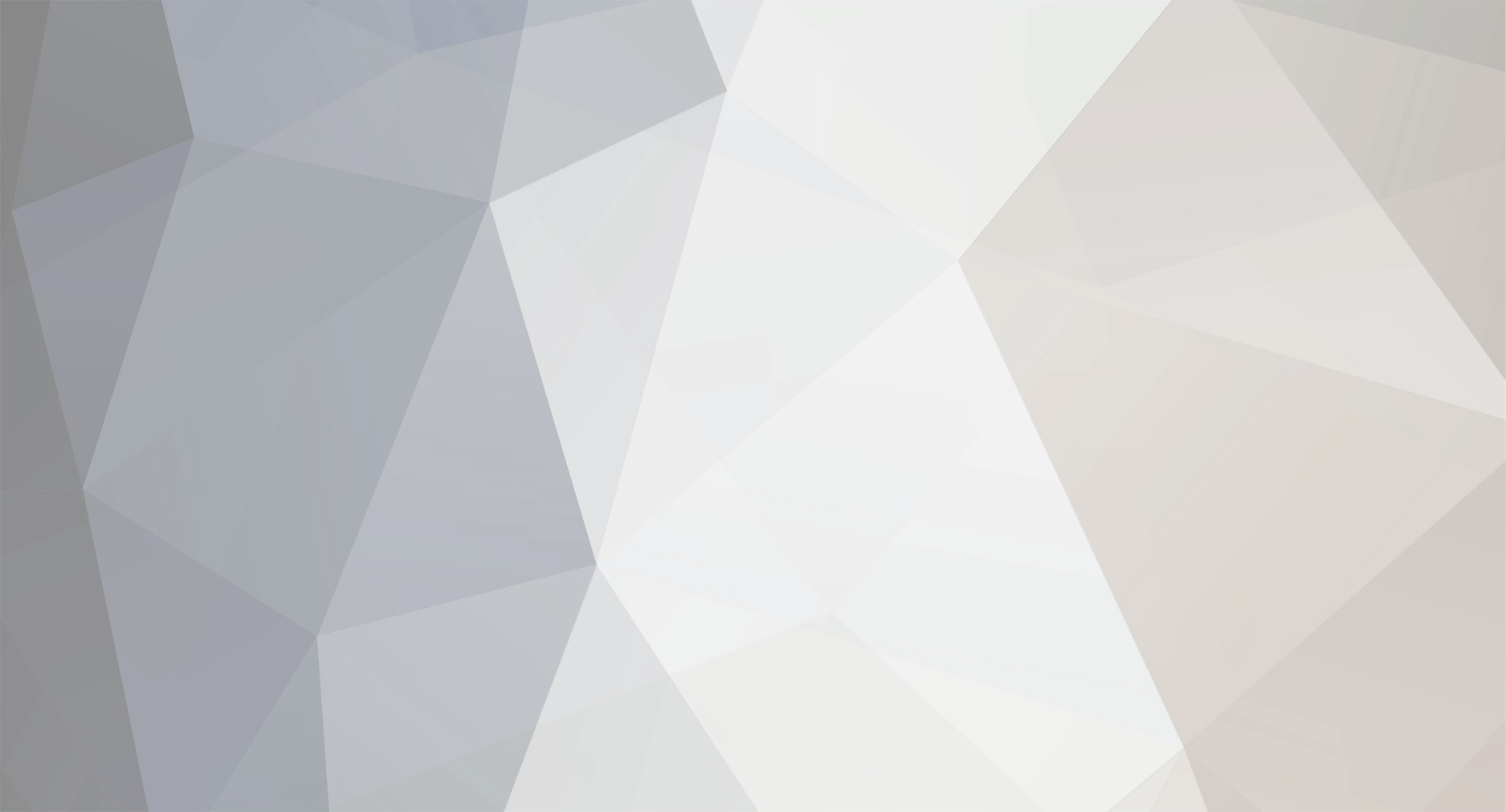
Delilah
-
Posts
40 -
Joined
-
Last visited
Posts posted by Delilah
-
-
Please, someone can tell me why the gui is not opening ?
Thanks.
-
I wrote like that
@Instance("TeR")
public static MainClass instance;
Now the modid is correct but the gui is not opening and I don't have any notification when I'm trying to open the gui.
-
This is my code:
@Mod(modid = "TeR", name = "TeR", version = "v.001")
@NetworkMod(clientSideRequired = true, serverSideRequired = true)
public class MainClass
{
@SidedProxy(clientSide = "JavaBuckets.Modium.Main.ClientProxy", serverSide = "JavaBuckets.Modium.Main.CommonProxy")
public static CommonProxy proxy;
public static String modid = "TeR";
@Instance("MainClass")
public static MainClass instance;
@EventHandler
public void init(FMLInitializationEvent event)
{
NetworkRegistry.instance().registerGuiHandler(this, new GuiHandler());
}
Block
package JavaBuckets.Mods.Modium.Blocks;
import java.util.Random;
import cpw.mods.fml.relauncher.Side;
import cpw.mods.fml.relauncher.SideOnly;
import JavaBuckets.Modium.Main.MainClass;
import JavaBuckets.Mods.TeR.entity.TileEntityTeRWorkBench;
import net.minecraft.block.Block;
import net.minecraft.block.BlockContainer;
import net.minecraft.block.material.Material;
import net.minecraft.client.renderer.texture.IconRegister;
import net.minecraft.entity.EntityLivingBase;
import net.minecraft.entity.item.EntityItem;
import net.minecraft.entity.player.EntityPlayer;
import net.minecraft.inventory.IInventory;
import net.minecraft.item.ItemStack;
import net.minecraft.nbt.NBTTagCompound;
import net.minecraft.tileentity.TileEntity;
import net.minecraft.util.MathHelper;
import net.minecraft.world.World;
public class BlockTeRWorkBench extends BlockContainer
{
public BlockTeRWorkBench(int id)
{
super(id, Material.rock);
setCreativeTab(MainClass.TeRTabItem);
this.setBlockBounds(0F, 0F,
0F, 1F,
1F-1F/16F*6F, 1F);
}
@Override
public TileEntity createNewTileEntity(World world)
{
return new TileEntityTeRWorkBench();
}
public int getRenderType()
{
return -1;
}
public boolean isOpaqueCube()
{
return false;
}
public boolean renderAsNormalBlock()
{
return false;
}
@SideOnly(Side.CLIENT)
public void registerIcons(IconRegister icon)
{
this.blockIcon = icon.registerIcon("modium:work_bench");
}
public int idDropped(int par1, Random par2Random, int par3)
{
return this.blockID;
}
public int quantityDropped(Random par1Random)
{
return 1;
}
//Calculamos la posición del objeto para luego poder rotarlo en la render class
/**
* Called whenever the block is added into the world. Args: world, x, y, z
*/
public void onBlockAdded(World par1World, int par2, int par3, int par4)
{
super.onBlockAdded(par1World, par2, par3, par4);
this.setDefaultDirection(par1World, par2, par3, par4);
}
// set a blocks direction
//
private void setDefaultDirection(World par1World, int par2, int par3, int par4)
{
if (!par1World.isRemote)
{
int l = par1World.getBlockId(par2, par3, par4 - 1);
int i1 = par1World.getBlockId(par2, par3, par4 + 1);
int j1 = par1World.getBlockId(par2 - 1, par3, par4);
int k1 = par1World.getBlockId(par2 + 1, par3, par4);
byte b0 = 3;
if (Block.opaqueCubeLookup[l] && !Block.opaqueCubeLookup[i1])
{
b0 = 3;
}
if (Block.opaqueCubeLookup[i1] && !Block.opaqueCubeLookup[l])
{
b0 = 2;
}
if (Block.opaqueCubeLookup[j1] && !Block.opaqueCubeLookup[k1])
{
b0 = 5;
}
if (Block.opaqueCubeLookup[k1] && !Block.opaqueCubeLookup[j1])
{
b0 = 4;
}
par1World.setBlockMetadataWithNotify(par2, par3, par4, b0, 2);
}
}
public void onBlockPlacedBy(World par1World, int par2, int par3, int par4, EntityLivingBase par5EntityLivingBase, ItemStack par6ItemStack)
{
int l = MathHelper.floor_double((double)(par5EntityLivingBase.rotationYaw * 4.0F / 360.0F) + 0.5D) & 3;
if (l == 0)
{
par1World.setBlockMetadataWithNotify(par2, par3, par4, 2, 2);
}
if (l == 1)
{
par1World.setBlockMetadataWithNotify(par2, par3, par4, 5, 2);
}
if (l == 2)
{
par1World.setBlockMetadataWithNotify(par2, par3, par4, 3, 2);
}
if (l == 3)
{
par1World.setBlockMetadataWithNotify(par2, par3, par4, 4, 2);
}
//if (par6ItemStack.hasDisplayName())
//{
// ((TileEntityFurnace)par1World.getBlockTileEntity(par2, par3, par4)).setGuiDisplayName(par6ItemStack.getDisplayName());
//}
}
private static int getMetadataBasedOnRotation(int rotation)
{
if (rotation >= 315 || rotation < 45)
{
return 1;
}
else if (rotation >= 45 && rotation < 135)
{
return 2;
}
else if (rotation >= 135 && rotation < 225)
{
return 0;
}
else
{
return 3;
}
}
//Añadimos todo lo de la interfaz Gui
@Override
public boolean onBlockActivated(World world, int x, int y, int z, EntityPlayer player, int metadata, float what, float these, float are)
{
TileEntity tileEntity = world.getBlockTileEntity(x, y, z);
if (tileEntity == null || player.isSneaking())
{
return false;
}
//code to open gui explained later
player.openGui(MainClass.instance, 0, world, x, y, z);
return true;
}
@Override
public void breakBlock(World world, int x, int y, int z, int par5, int par6)
{
dropItems(world, x, y, z);
super.breakBlock(world, x, y, z, par5, par6);
}
private void dropItems(World world, int x, int y, int z)
{
Random rand = new Random();
TileEntity tileEntity = world.getBlockTileEntity(x, y, z);
if (!(tileEntity instanceof IInventory))
{
return;
}
IInventory inventory = (IInventory) tileEntity;
for (int i = 0; i < inventory.getSizeInventory(); i++)
{
ItemStack item = inventory.getStackInSlot(i);
if (item != null && item.stackSize > 0)
{
float rx = rand.nextFloat() * 0.8F + 0.1F;
float ry = rand.nextFloat() * 0.8F + 0.1F;
float rz = rand.nextFloat() * 0.8F + 0.1F;
EntityItem entityItem = new EntityItem(world,
x + rx, y + ry, z + rz,
new ItemStack(item.itemID, item.stackSize, item.getItemDamage()));
if (item.hasTagCompound())
{
entityItem.getEntityItem().setTagCompound((NBTTagCompound) item.getTagCompound().copy());
}
float factor = 0.05F;
entityItem.motionX = rand.nextGaussian() * factor;
entityItem.motionY = rand.nextGaussian() * factor + 0.2F;
entityItem.motionZ = rand.nextGaussian() * factor;
world.spawnEntityInWorld(entityItem);
item.stackSize = 0;
}
}
}
/*
@Override
public TileEntity createNewTileEntity(World world) {
return new TileEntityTiny();
}
*/
}
Container
package JavaBuckets.Modium.Main;
import JavaBuckets.Mods.TeR.entity.TileEntityGui;
import net.minecraft.entity.player.EntityPlayer;
import net.minecraft.entity.player.InventoryPlayer;
import net.minecraft.inventory.Container;
import net.minecraft.inventory.Slot;
import net.minecraft.item.ItemStack;
public class ContainerGui extends Container
{
protected TileEntityGui tileEntity;
public ContainerGui (InventoryPlayer inventoryPlayer, TileEntityGui te)
{
tileEntity = te;
//the Slot constructor takes the IInventory and the slot number in that it binds to
//and the x-y coordinates it resides on-screen
for (int i = 0; i < 3; i++)
{
for (int j = 0; j < 3; j++)
{
addSlotToContainer(new Slot(tileEntity, j + i * 3, 62 + j * 18, 17 + i * 18));
}
}
//commonly used vanilla code that adds the player's inventory
bindPlayerInventory(inventoryPlayer);
}
@Override
public boolean canInteractWith(EntityPlayer player) {
return tileEntity.isUseableByPlayer(player);
}
protected void bindPlayerInventory(InventoryPlayer inventoryPlayer) {
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 9; j++) {
addSlotToContainer(new Slot(inventoryPlayer, j + i * 9 + 9,
8 + j * 18, 84 + i * 18));
}
}
for (int i = 0; i < 9; i++) {
addSlotToContainer(new Slot(inventoryPlayer, i, 8 + i * 18, 142));
}
}
@Override
public ItemStack transferStackInSlot(EntityPlayer player, int slot) {
ItemStack stack = null;
Slot slotObject = (Slot) inventorySlots.get(slot);
//null checks and checks if the item can be stacked (maxStackSize > 1)
if (slotObject != null && slotObject.getHasStack()) {
ItemStack stackInSlot = slotObject.getStack();
stack = stackInSlot.copy();
//merges the item into player inventory since its in the tileEntity
if (slot < 9) {
if (!this.mergeItemStack(stackInSlot, 0, 35, true)) {
return null;
}
}
//places it into the tileEntity is possible since its in the player inventory
else if (!this.mergeItemStack(stackInSlot, 0, 9, false)) {
return null;
}
if (stackInSlot.stackSize == 0) {
slotObject.putStack(null);
} else {
slotObject.onSlotChanged();
}
if (stackInSlot.stackSize == stack.stackSize) {
return null;
}
slotObject.onPickupFromSlot(player, stackInSlot);
}
return stack;
}
}
GuiHandler
package JavaBuckets.Modium.Main;
import JavaBuckets.Mods.TeR.entity.TileEntityGui;
import net.minecraft.entity.player.EntityPlayer;
import net.minecraft.tileentity.TileEntity;
import net.minecraft.world.World;
import cpw.mods.fml.common.network.IGuiHandler;
public class GuiHandler implements IGuiHandler
{
//returns an instance of the Container you made earlier
@Override
public Object getServerGuiElement(int id, EntityPlayer player, World world, int x, int y, int z)
{
TileEntity tileEntity = world.getBlockTileEntity(x, y, z);
if(tileEntity instanceof TileEntityGui)
{
return new ContainerGui(player.inventory, (TileEntityGui) tileEntity);
}
return null;
}
//returns an instance of the Gui you made earlier
@Override
public Object getClientGuiElement(int id, EntityPlayer player, World world,
int x, int y, int z) {
TileEntity tileEntity = world.getBlockTileEntity(x, y, z);
if(tileEntity instanceof TileEntityGui)
{
return new TeRGui(player.inventory, (TileEntityGui) tileEntity);
}
return null;
}
}
Gui
package JavaBuckets.Modium.Main;
import net.minecraft.client.gui.inventory.GuiContainer;
import net.minecraft.entity.player.InventoryPlayer;
import net.minecraft.util.ResourceLocation;
import net.minecraft.util.StatCollector;
import org.lwjgl.opengl.GL11;
import JavaBuckets.Mods.TeR.entity.TileEntityGui;
public class TeRGui extends GuiContainer
{
public TeRGui (InventoryPlayer inventoryPlayer,
TileEntityGui tileEntity)
{
//the container is instanciated and passed to the superclass for handling
super(new ContainerGui(inventoryPlayer, tileEntity));
}
@Override
protected void drawGuiContainerForegroundLayer(int param1, int param2) {
//draw text and stuff here
//the parameters for drawString are: string, x, y, color
fontRenderer.drawString("Tiny", 8, 6, 4210752);
//draws "Inventory" or your regional equivalent
fontRenderer.drawString(StatCollector.translateToLocal("container.inventory"), 8, ySize - 96 + 2, 4210752);
}
@Override
protected void drawGuiContainerBackgroundLayer(float par1, int par2,
int par3)
{
//draw your Gui here, only thing you need to change is the path
//int texture = mc.renderEngine.getTexture("modium:craftinggui.png");
final ResourceLocation texture = new ResourceLocation("modium:textures/blocks/craftinggui.png");
GL11.glColor4f(1.0F, 1.0F, 1.0F, 1.0F);
this.mc.renderEngine.bindTexture(texture);
int x = (width - xSize) / 2;
int y = (height - ySize) / 2;
this.drawTexturedModalRect(x, y, 0, 0, xSize, ySize);
}
}
ClientProxy
package JavaBuckets.Modium.Main;
import JavaBuckets.Mods.TeR.Models.slimegreen;
import JavaBuckets.Mods.TeR.Models.slimenblue;
import JavaBuckets.Mods.TeR.Models.tercorruptor;
import JavaBuckets.Mods.TeR.Renders.SlimeBlueRender;
import JavaBuckets.Mods.TeR.Renders.SlimeGreenRender;
import JavaBuckets.Mods.TeR.Renders.TeRCorruptorRender;
import JavaBuckets.Mods.TeR.entity.Entityslimeblue;
import JavaBuckets.Mods.TeR.entity.Entityslimegreen;
import JavaBuckets.Mods.TeR.entity.Entityter_corruptor;
import cpw.mods.fml.client.registry.RenderingRegistry;
import cpw.mods.fml.relauncher.Side;
import cpw.mods.fml.relauncher.SideOnly;
public class ClientProxy extends CommonProxy
{
@SideOnly(Side.CLIENT)
public void registerRenderInformation()
{
}
}
CommonProxy
package JavaBuckets.Modium.Main;
public class CommonProxy
{
public void registerRenderInformation()
{
}
}
TileEntityGui
package JavaBuckets.Mods.TeR.entity;
import net.minecraft.entity.player.EntityPlayer;
import net.minecraft.inventory.IInventory;
import net.minecraft.item.ItemStack;
import net.minecraft.nbt.NBTTagCompound;
import net.minecraft.nbt.NBTTagList;
import net.minecraft.tileentity.TileEntity;
public class TileEntityGui extends TileEntity implements IInventory {
private ItemStack[] inv;
public TileEntityGui(){
inv = new ItemStack[9];
}
@Override
public int getSizeInventory() {
return inv.length;
}
@Override
public ItemStack getStackInSlot(int slot) {
return inv[slot];
}
@Override
public void setInventorySlotContents(int slot, ItemStack stack) {
inv[slot] = stack;
if (stack != null && stack.stackSize > getInventoryStackLimit()) {
stack.stackSize = getInventoryStackLimit();
}
}
@Override
public ItemStack decrStackSize(int slot, int amt) {
ItemStack stack = getStackInSlot(slot);
if (stack != null) {
if (stack.stackSize <= amt) {
setInventorySlotContents(slot, null);
} else {
stack = stack.splitStack(amt);
if (stack.stackSize == 0) {
setInventorySlotContents(slot, null);
}
}
}
return stack;
}
@Override
public ItemStack getStackInSlotOnClosing(int slot) {
ItemStack stack = getStackInSlot(slot);
if (stack != null) {
setInventorySlotContents(slot, null);
}
return stack;
}
@Override
public int getInventoryStackLimit() {
return 64;
}
@Override
public boolean isUseableByPlayer(EntityPlayer player) {
return worldObj.getBlockTileEntity(xCoord, yCoord, zCoord) == this &&
player.getDistanceSq(xCoord + 0.5, yCoord + 0.5, zCoord + 0.5) < 64;
}
@Override
public void openChest() {}
@Override
public void closeChest() {}
@Override
public void readFromNBT(NBTTagCompound tagCompound) {
super.readFromNBT(tagCompound);
NBTTagList tagList = tagCompound.getTagList("Inventory");
for (int i = 0; i < tagList.tagCount(); i++) {
NBTTagCompound tag = (NBTTagCompound) tagList.tagAt(i);
byte slot = tag.getByte("Slot");
if (slot >= 0 && slot < inv.length) {
inv[slot] = ItemStack.loadItemStackFromNBT(tag);
}
}
}
@Override
public void writeToNBT(NBTTagCompound tagCompound) {
super.writeToNBT(tagCompound);
NBTTagList itemList = new NBTTagList();
for (int i = 0; i < inv.length; i++) {
ItemStack stack = inv;
if (stack != null) {
NBTTagCompound tag = new NBTTagCompound();
tag.setByte("Slot", (byte) i);
stack.writeToNBT(tag);
itemList.appendTag(tag);
}
}
tagCompound.setTag("Inventory", itemList);
}
@Override
public String getInvName() {
return "tco.tileentitytiny";
}
//Métodos implementados
@Override
public boolean isInvNameLocalized()
{
// TODO Auto-generated method stub
return false;
}
@Override
public boolean isItemValidForSlot(int i, ItemStack itemstack)
{
// TODO Auto-generated method stub
return false;
}
}
Thanks
-
Hi hello, I did at the end the gui following the tutorial of Containers and Guis here
http://www.minecraftforge.net/wiki/Containers_and_GUIs
Apparently there is no errors in the code and the game is working, but when I'm doing right click on the block that should open the gui I can see the text "A mod tried to open a gui on the server without being a NetworkMod" I was looking for information but in the tutorial I can't see nothing about that and I don't know how to fixed. If someone know the solution would be a help. Is really complicated the first time that you are doing that.
Thanks.
-
Hi hello, I want to ask if anyone know a good tutorial for minecraft 1.6.2 or 1.6.4
I need to create a custom gui for my mod and I have a lot of work to do in the gui
after the creation of the gui so I would like to know if someone know a tutorial or
a place where I can find or see the information about the guis development.
Thanks.
-
Hi hello,
I open this post to ask if anyone know a good tutorial to create a custom crafting table
in minecraft 1.6 series. I'm looking in google but the tutorials that I'm watching are
not clear or are for old versions.
Thanks.
-
Omg, is so complex to create a whole new crafting table... I was thinking in using the minecraft workbench ui to try to do the things more easy, but I'm watching that create a custom crafting table is going to be another real pain....
Anyone know a good tutorial about that for mc 1.6 series ? Thanks.
-
Hi hello, I continued with my mod, and now I'm telling to the blocks that they are
crafting stations to display the crafting table ui. I was reading the code of the
Workbench block and I saw this code
/**
* Called upon block activation (right click on the block.)
*/
public boolean onBlockActivated(World par1World, int par2, int par3, int par4, EntityPlayer par5EntityPlayer, int par6, float par7, float par8, float par9)
{
if (par1World.isRemote)
{
return true;
}
else
{
par5EntityPlayer.displayGUIWorkbench(par2, par3, par4);
return true;
}
}
So I decided to add this code to my block to see what happen, but the code is not working.
My block is a custom block with custom model but is an extension of the minecraft block.
I did a short modification in the code
/**
* Called upon block activation (right click on the block.)
*/
public boolean onBlockActivated(World par1World, int par2, int par3, int par4, EntityPlayer par5EntityPlayer, int par6, float par7, float par8, float par9)
{
{
par5EntityPlayer.displayGUIWorkbench(par2, par3, par4);
return true;
}
}
With the modification my crafting table is working but the ui is opening for half a second and after that
the game is closing the ui so you can't use the ui...
If someone can help me... I need a hand again sorry is my first crafting table and I'm looking for information.
Thanks.
-
Thanks your solution was magic, thank you so much.
That is the solution for someone with the same ploblem
/**
* Updates the blocks bounds based on its current state. Args: world, x, y, z
*/
public void setBlockBoundsBasedOnState(IBlockAccess par1IBlockAccess, int par2, int par3, int par4)
{
//this.setBlockBounds(MinX, MinY, MinZ, maxX, maxY, maxZ);
int l = par1IBlockAccess.getBlockMetadata(par2, par3, par4) & 3;
System.out.println(l);
if (l != 3 && l != 2)
{
this.setBlockBounds(0.2F, 0.0F, 0.1F, 0.7F, 0.6F, 0.9F);
}
else
{
this.setBlockBounds(0.1F, 0.0F, 0.2F, 0.9F, 0.6F, 0.7F);
}
}
In the else condition I swap the x coord with the z coord and now the object is having the proper blockbound in all the moments. Thank you so much....
-
Sorry, I don't know really, I had the problem and I was looking for information, I saw this code in a forum I think and I used it to see if it working of something was happening because with the render code the only the orientation was the same every time and after I copied this code the orientation was correct but I don't know the reason really...
Now, I have I think the last problem of the block orientation... the bounding box of the block is every time the same... But now the block is having the orientation from where you are placing the block but the bounding box is not having the orientation...I should write something for the bounding box of the object in the render class as well
Sorry is my first time doing a mod and my first custom rendered block.
-
Omg omg omg, I hate to do the things in this way, looking information everywhere because there isn't a place where you can see the full doc....
In the minecraft forum I saw a bit of code but wasn't completed so I had to print a line to see all the parameters at the end the this is the code that is working to set the block orientation with the player orientation.
public void renderTileEntityAt(TileEntity tileentity, double x, double y, double z, float f)
{
GL11.glPushMatrix();
GL11.glTranslatef((float)x+0.5F, (float)y+1.5F, (float)z+0.5F);
GL11.glRotatef(180, 0F, 0F, 1F);
int meta = tileentity.getWorldObj().getBlockMetadata(tileentity.xCoord, tileentity.yCoord, tileentity.zCoord);
System.out.println(meta);
if (meta==5) meta = 1;
else if (meta==2) meta=0;
if (meta==4) meta = 3;
else if (meta==3) meta=2;
GL11.glRotatef(90 * meta, 0F, 1F, 0F);
this.bindTexture(basicfurnacetexture);
//GL11.glPushMatrix();
this.model.renderModel(0.0625F);
//GL11.glPopMatrix();
GL11.glPopMatrix();
}
But this code wasn't enough, I was thinking that the code was only in the render class but again I was wrong...I don't know where at the moment but in other place looking for the same problem I saw that I had to add code as well in the class for the block of the object.
This is the code for the block class.
/**
* Called whenever the block is added into the world. Args: world, x, y, z
*/
public void onBlockAdded(World par1World, int par2, int par3, int par4)
{
super.onBlockAdded(par1World, par2, par3, par4);
this.setDefaultDirection(par1World, par2, par3, par4);
}
// set a blocks direction
//
private void setDefaultDirection(World par1World, int par2, int par3, int par4)
{
if (!par1World.isRemote)
{
int l = par1World.getBlockId(par2, par3, par4 - 1);
int i1 = par1World.getBlockId(par2, par3, par4 + 1);
int j1 = par1World.getBlockId(par2 - 1, par3, par4);
int k1 = par1World.getBlockId(par2 + 1, par3, par4);
byte b0 = 3;
if (Block.opaqueCubeLookup[l] && !Block.opaqueCubeLookup[i1])
{
b0 = 3;
}
if (Block.opaqueCubeLookup[i1] && !Block.opaqueCubeLookup[l])
{
b0 = 2;
}
if (Block.opaqueCubeLookup[j1] && !Block.opaqueCubeLookup[k1])
{
b0 = 5;
}
if (Block.opaqueCubeLookup[k1] && !Block.opaqueCubeLookup[j1])
{
b0 = 4;
}
par1World.setBlockMetadataWithNotify(par2, par3, par4, b0, 2);
}
}
public void onBlockPlacedBy(World par1World, int par2, int par3, int par4, EntityLivingBase par5EntityLivingBase, ItemStack par6ItemStack)
{
int l = MathHelper.floor_double((double)(par5EntityLivingBase.rotationYaw * 4.0F / 360.0F) + 0.5D) & 3;
if (l == 0)
{
par1World.setBlockMetadataWithNotify(par2, par3, par4, 2, 2);
}
if (l == 1)
{
par1World.setBlockMetadataWithNotify(par2, par3, par4, 5, 2);
}
if (l == 2)
{
par1World.setBlockMetadataWithNotify(par2, par3, par4, 3, 2);
}
if (l == 3)
{
par1World.setBlockMetadataWithNotify(par2, par3, par4, 4, 2);
}
//if (par6ItemStack.hasDisplayName())
//{
// ((TileEntityFurnace)par1World.getBlockTileEntity(par2, par3, par4)).setGuiDisplayName(par6ItemStack.getDisplayName());
//}
}
private static int getMetadataBasedOnRotation(int rotation)
{
if (rotation >= 315 || rotation < 45)
{
return 1;
}
else if (rotation >= 45 && rotation < 135)
{
return 2;
}
else if (rotation >= 135 && rotation < 225)
{
return 0;
}
else
{
return 3;
}
}
I don't know seriously what this code is doing 100% but I think this code is storing the number when we set the block that we are using in the variable meta in the render class, because the variable meta is not working is this code is not in the block class.
Anyway the problem is solved. Thanks. I hope this code can help to someone with the same problem.
This is a photo with the problem solved.
Before the code that I'm showing in this post, the block was every time in the same direction....omg how complicated was....
-
No no, the rotation of the model is working. This is not mi question. My question is that the model is every time in the same
direction, and the model need to have the player orientation because the model is not symmetric.
-
I was reading a post in the forum of minecraft about the same problem, this is my code now:
public void renderTileEntityAt(TileEntity tileentity, double x, double y, double z, float f)
{
GL11.glPushMatrix();
GL11.glTranslatef((float)x+0.5F, (float)y+1.5F, (float)z+0.5F);
GL11.glRotatef(180, 0F, 0F, 1F);
int meta = tileentity.getWorldObj().getBlockMetadata(tileentity.xCoord, tileentity.yCoord, tileentity.zCoord);
if (meta==3) meta =2;
else if (meta==2) meta=3;
GL11.glRotatef(90 * meta, 0F, 1F, 0F);
this.bindTexture(basicfurnacetexture);
this.model.renderModel(0.0625F);
GL11.glPopMatrix();
}
In the forum of minecraft they told that these solution was working for the orientation of the custom rendered block but
my block is not working, is still in the same direction, if someone know what happen, I'm getting crazy... I don't know what
happen and I can't see a proper information about everything... is just follow this tutorial or follow another tutorial... but
is crazy to work like that without a proper doc....
-
Hi hello, I'm doing at the moment the models for my mod and the models
are working. But I have two questions.
My models are every time in the same direction I would like that when you set a model
in the world is taking your orientation and I would like to know if can change the size
of the model, I don't know how, is my first custom model. This is the code that I'm using.
package JavaBuckets.Mods.TeR.Renders;
import org.lwjgl.opengl.GL11;
import JavaBuckets.Mods.TeR.Models.terraria_furnace;
import JavaBuckets.Mods.TeR.entity.TileEntityTeRBasicFurnace;
import net.minecraft.client.renderer.tileentity.TileEntitySpecialRenderer;
import net.minecraft.tileentity.TileEntity;
import net.minecraft.util.ResourceLocation;
public class BasicFurnaceRender extends TileEntitySpecialRenderer
{
private static final ResourceLocation basicfurnacetexture = new ResourceLocation("modium:textures/blocks/terraria_furnace.png");
private terraria_furnace model;
public BasicFurnaceRender()
{
this.model = new terraria_furnace();
}
public void renderTileEntityAt(TileEntity tileentity, double x, double y, double z, float f)
{
GL11.glPushMatrix();
GL11.glTranslatef((float)x+0.5F, (float)y+1.5F, (float)z+0.5F);
GL11.glRotatef(180, 0F, 0F, 1F);
this.bindTexture(basicfurnacetexture);
GL11.glPushMatrix();
this.model.renderModel(0.0625F);
GL11.glPopMatrix();
GL11.glPopMatrix();
}
}
Thanks.
-
Hello again, I did a photo to show the problem this is my first air mob.
As you can see in the mob is in the air, but the hitbox of the mob in on the ground
so when you want to kill the mob you need to attack the invisible hitbox on the ground.
Someone knows how to set the hitbox where the mob is ?
Thanks.
-
Hi hello, again, I continue with the mod and I did the first flying mob. Well, is not flying
really but the position of the mod is like two meters from the floor, the question that I have
is. For the game the mob is on the floor. I can see the shadow on the floor and to attack this enemy
I have to attack an invisible enemy under the real mob.
How I can setup the hitbox of the mob to the position of the model ? and I was looking in the
blaze code to see how they can fly, but I couldn't find, if anyone know how I can tell to mob
that he can fly would be a help.
Thanks.
Pd.Sorry for my english, my level of english to explain that wasn't enough...
-
Thanks I'll try.
-
Hi hello, I did my first mob for the mod and is working at the end.
But I have only one thing more for finish the mob... I would like to add to the mob custom jumps to move instead to travel following lines. I would like to ask if there is any AI or function that I can use to tell to the mob to move using jumps.
Thanks.
-
Thanks is working I solved two question
the forum is amazing, I couldn't do my mod, without the help of the people.
If anyone know small routine to add random jumps to an entity is the last thing for my mod, thanks.
-
Thanks more or less is working but how I can do that the new mob attack the player?
-
Hi hello again, I continued with my mod and I finished the world
generation, was so hard, is my first mod and every is new but
I'm following tutorials and they are helping a lot.
I was working yesterday in my first custom mob, I had a few models
from techne and I started all the tutorials for mob generation.
And at the moment my mob is working, is having the specific health,
size, texture and is appearing in the custom biome only. But is not
totally ready and I need to ask a few things that I can't see in the
tutorials.
1º My mob is spawning only during the night I think is because in the Registry
the type of creature is a monster.
EntityRegistry.addSpawn(MyMob.class, 80, 6, 10, EnumCreatureType.monster, Mycustombiome);
But I think in the code of the mob I can set the condition, here is my condition for spawn.
public boolean getCanSpawnHere()
{
//return this.worldObj.difficultySetting > 0 && this.isValidLightLevel() && super.getCanSpawnHere();
return this.worldObj.difficultySetting > 0 && super.getCanSpawnHere();
}
2º My second question is because the mob is passive is not attacking even if you are
attacking him. I don't know how to tell to attack de player because if my first time doing a mob.
I don't know if there is a task for attack de player for the moment I have only the 3 tasks
of the tutorial.
public MyMob(World par1World)
{
super(par1World);
this.setSize(1F, 1F);
this.getNavigator().setAvoidsWater(true);
this.tasks.addTask(0, new EntityAIWander(this, 0.4F));
this.tasks.addTask(1, new EntityAIWatchClosest(this, EntityPlayer.class, 6.0F));
this.tasks.addTask(2, new EntityAILookIdle(this));
}
3º And the last thing, sorry is a large question. My mob is a slime that I want to have
in specifics biomes but I'm looking in the original code of slimes to add the jumps to the mob.
At the moment my mob is working and moving, but is only jumping when the mob have a
block in front of. How I can add random jumps ?
Thanks.
-
Thanks is working really good
This is the code that I did in my worldgenerator in case that someone need to do the same thing.
BiomeGenBase biomeGenBase = world.getWorldChunkManager().getBiomeGenAt(x+16, y+16);
if (biomeGenBase == Biome that we want to add the specific ore)
{
this.addOreSpawn(Our Ore, world, random, x, y, 16, 16, 5+random.nextInt(5), 5, 0, 100);
}
-
Hi hello, I continued working with my mod and at the moment I have
a full new world generation with my own world generator and my
own ores, but the question that I want to do is...
At the moment I want to add a specific ore to a specific biome because
my world generator is creating all my ores in all my biomes. I think is
possible to do but is the first time that I doing this case. I was trying to
do all the morning watching the code of the e.hills to see the
code but the code of the e.hills is not working.
I hope someone know how to do to help me.
Thanks.
-
Omg.Thanks, the code is working, that what I was looking for. Now watching your code I can
understand the process but is something that is I'm doing for myself I can't do because my
creativity is zero. Thanks.
[1.6.4] A mod tried to open a gui on the server without being a NetworkMod
in Modder Support
Posted
Yes I did, but still is not working. Is someone can tell me what is wrong in code would be a help. I can't continue with the mod until I finish with this problem.