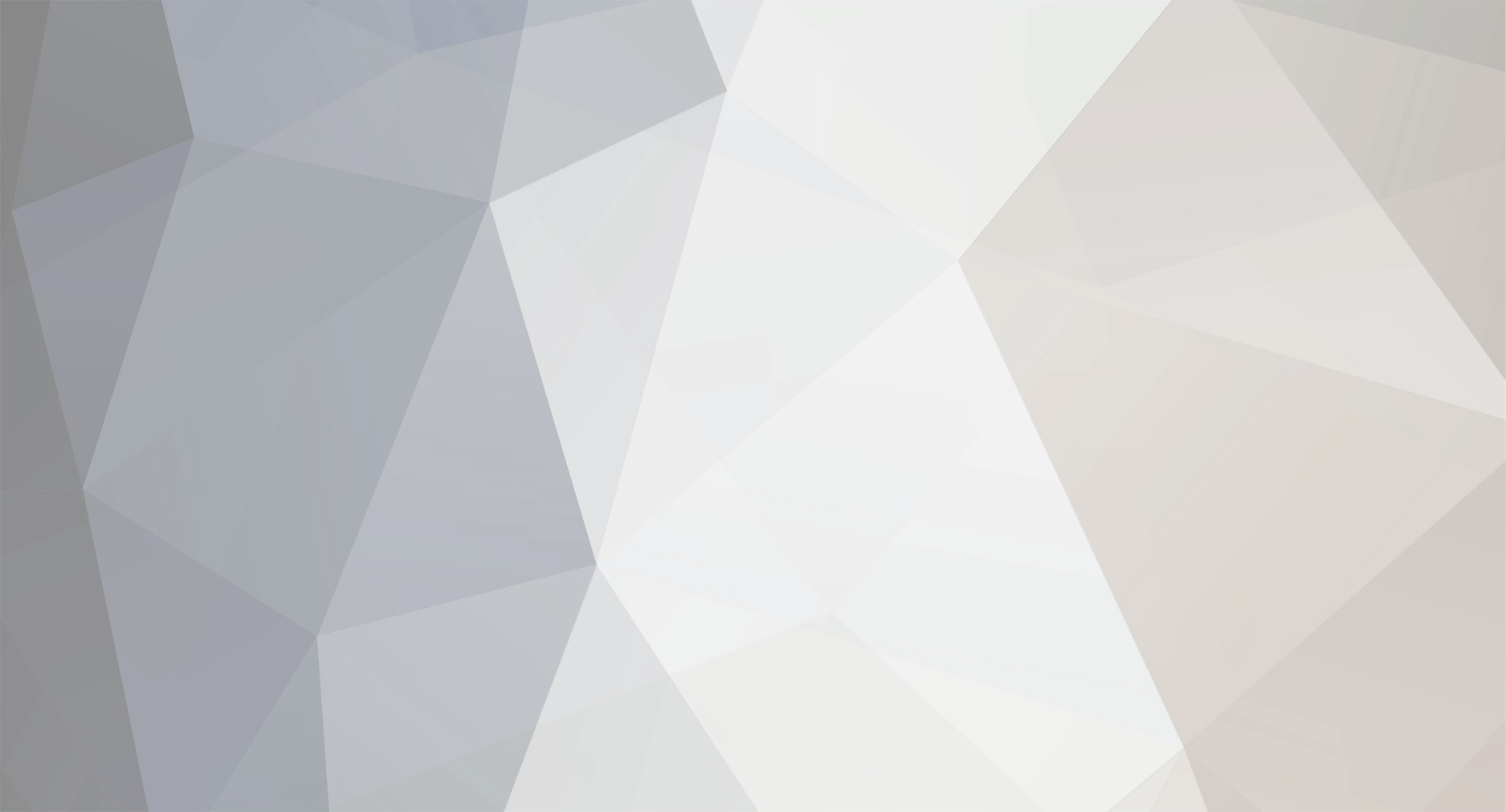
ImpactFlux
Members-
Posts
6 -
Joined
-
Last visited
Converted
-
Gender
Undisclosed
-
Personal Text
I am new!
ImpactFlux's Achievements

Tree Puncher (2/8)
1
Reputation
-
Does anyone have any good resources on using models created from Cubik for entities? I looked at McJty's tutorial, and I have it rendering using a simple texture, but I would like to move past that and use the model generated from `Cubik`. I noticed it gives the following options for export: JSON: Minecraft model Java: minecfraft Mod Entity model OBJ: Wavefront obj model I figure that I need to do the following. Export it as a JAVA Rename it to the existing ModelMeteor, which extends ModelBase. public class MeteorModel extends ModelBase { //fields ModelRenderer e1; ModelRenderer e2; ModelRenderer e3; ModelRenderer e4; ModelRenderer e5; ModelRenderer e6; public CubikModel() { textureWidth = 64; textureHeight = 64; e1 = new ModelRenderer(this, 0, 55); e1.addBox(3F, 7F, 3.5F, 11, 1, 8); e1.setRotationPoint(3F, 7F, 3.5F); e1.setTextureSize(64, 64); e1.mirror = false; setRotation(e1, 0F, 0F, 0F); e2 = new ModelRenderer(this, 0, 48); e2.addBox(4F, 5F, 4.5F, 9, 1, 6); e2.setRotationPoint(4F, 5F, 4.5F); e2.setTextureSize(64, 64); e2.mirror = false; setRotation(e2, 0F, 0F, 0F); e3 = new ModelRenderer(this, 0, 48); e3.addBox(4F, 8F, 4.5F, 9, 1, 6); e3.setRotationPoint(4F, 8F, 4.5F); e3.setTextureSize(64, 64); e3.mirror = false; setRotation(e3, 0F, 0F, 0F); e4 = new ModelRenderer(this, 38, 58); e4.addBox(5.5F, 4F, 5F, 6, 1, 5); e4.setRotationPoint(5.5F, 4F, 5F); e4.setTextureSize(64, 64); e4.mirror = false; setRotation(e4, 0F, 0F, 0F); e5 = new ModelRenderer(this, 38, 58); e5.addBox(5.5F, 9F, 5F, 6, 1, 5); e5.setRotationPoint(5.5F, 9F, 5F); e5.setTextureSize(64, 64); e5.mirror = false; setRotation(e5, 0F, 0F, 0F); e6 = new ModelRenderer(this, 0, 55); e6.addBox(3F, 6F, 3.5F, 11, 1, 8); e6.setRotationPoint(3F, 6F, 3.5F); e6.setTextureSize(64, 64); e6.mirror = false; setRotation(e6, 0F, 0F, 0F); } public void render(Entity entity, float f, float f1, float f2, float f3, float f4, float f5) { super.render(entity, f, f1, f2, f3, f4, f5); setRotationAngles(f, f1, f2, f3, f4, f5, entity); e1.render(f5); e2.render(f5); e3.render(f5); e4.render(f5); e5.render(f5); e6.render(f5); } private void setRotation(ModelRenderer model, float x, float y, float z) { model.rotateAngleX = x; model.rotateAngleY = y; model.rotateAngleZ = z; } public void setRotationAngles(float f, float f1, float f2, float f3, float f4, float f5, Entity entity) { super.setRotationAngles(f, f1, f2, f3, f4, f5, entity); } } Update RenderMeteor somehow to bind to the new model. public class RenderMeteor extends Render<EntityMeteor> { private ModelMeteor modelMeteor = new ModelMeteor(); private ResourceLocation meteorTexture = new ResourceLocation(MeteorsMod.MODID, "textures/entity/fallingmeteor.png"); public RenderMeteor(RenderManager renderManager, ModelBase model, float shadow) { super(renderManager); this.modelMeteor = new ModelMeteor(); this.shadowSize = shadow; } @Override public void doRender(EntityMeteor entity, double d, double d1, double d2, float f, float f1) { renderMeteor(entity, d, d1, d2, f, f1); } public void renderMeteor(EntityMeteor entityMeteor, double d, double d1, double d2, float f, float f1) { GL11.glPushMatrix(); GL11.glTranslatef((float)d, (float)d1, (float)d2); GL11.glRotatef(entityMeteor.prevRotationYaw + (entityMeteor.rotationYaw - entityMeteor.prevRotationYaw) * f1, 0.0F, 1.0F, 0.0F); GL11.glRotatef(entityMeteor.prevRotationPitch + (entityMeteor.rotationPitch - entityMeteor.prevRotationPitch) * f1, 0.0F, 0.0F, 1.0F); this.bindTexture(meteorTexture); float f2 = 1.0F * (float)entityMeteor.size; GL11.glScalef(f2, f2, f2); modelMeteor.renderWithSize(entityMeteor.size, 0.0625F); GL11.glPopMatrix(); } @Override public boolean canRenderName(EntityMeteor entity){ return false; } @Override protected ResourceLocation getEntityTexture(EntityMeteor entity) { return meteorTexture; } public static class Factory implements IRenderFactory<EntityMeteor> { @Override public Render<? super EntityMeteor> createRenderFor(RenderManager manager) { return new RenderMeteor(manager, ModelManager.models.get("meteor"), 0.5f); } } } ModelManager will likely stay the same public class ModelManager { public static Map<String, ModelBase> models = new HashMap<String, ModelBase>(); public static void init(){ models.put("meteor",new ModelMeteor()); } } I'm also assuming my EntityRegistration will also stay the same? ResourceLocation meteorRL = new ResourceLocation(MeteorsMod.MODID + ":falling_meteor"); EntityRegistry.registerModEntity(meteorRL, EntityMeteor.class, "falling_meteor", entityId++, MeteorsMod.instance, 256, 1, true); And then I need to put the entity model json inside the resources/assets/meteors/models/entity folder? But after trying the above, I'm still not getting it to render properly.. so any resources would be most appreciated.
-
Yeah I didn't know if there was a good way to define a basic list, and then look at the crafting recipes or if anyone had any better ideas. What I may do, is build a seperate config with an entire block list, and default everything to having a massive burn time and then go through and assign times to the ones that make sense and let it be a user configuration.
-
Anyone know of a good way to build a map/table of blocks in relation to their rarity/difficulty to obtain? I'm looking to dynamically build a hash of blocks on init that iterates through the existing block set and can assign a default value of its rarity or some value to it. Essentially I need a way to determine how rare a block is for my terraforming mod. I'm allowing any block to be apart of the terraforming process, but so it won't be too OP I just want to raise the consume time considerably to get it to that point. The easy/long way would be to just list every block out and build a massive config file by hand, but I would rather do it dynamically.
-
[Solved][1.7.2]Can someone explain adding new liquids to me?
ImpactFlux replied to Taji34's topic in Modder Support
Glad you got it worked out! Since its still pretty vanilla you may even want to update the fluid tutorial on the wiki. -
[Solved][1.7.2]Can someone explain adding new liquids to me?
ImpactFlux replied to Taji34's topic in Modder Support
Here is an example fluid I've added.. I followed the tutorial awhile ago. Note: The naming convention is case sensative also your textures need to be in the following \texture\blocks\yourfluidflow.png \texture\blocks\yourfluidflow.png.mcmeta \texture\blocks\yourfluidstill.png \texture\blocks\yourfluidstill.png.mcmeta This is for BlockYourFluid.java + import net.minecraft.block.material.Material; + import net.minecraft.client.renderer.texture.IIconRegister; + import net.minecraft.creativetab.CreativeTabs; + import net.minecraft.util.IIcon; + import net.minecraft.world.IBlockAccess; + import net.minecraft.world.World; + import net.minecraftforge.fluids.BlockFluidClassic; + import net.minecraftforge.fluids.Fluid; + import cpw.mods.fml.relauncher.Side; + import cpw.mods.fml.relauncher.SideOnly; + + public class BlockYourFluidFluid extends BlockFluidClassic + { + @SideOnly(Side.CLIENT) + protected IIcon stillIcon; + @SideOnly(Side.CLIENT) + protected IIcon flowingIcon; + + public BlockEcopoiesisFluid(Fluid fluid, Material material) { + super(fluid, material); + fluid.setUnlocalizedName("yourFluid"); + setCreativeTab(CreativeTabs.tabMisc); + } + + @Override + public IIcon getIcon(int side, int meta) { + return (side == 0 || side == 1)? stillIcon : flowingIcon; + } + + @SideOnly(Side.CLIENT) + @Override + public void registerBlockIcons(IIconRegister register) { + stillIcon = register.registerIcon(YourMod.MODID + ":yourfluidstill"); + flowingIcon = register.registerIcon(YourMod.MODID + ":yourfluidflow"); + } + /* + @Override + public boolean canDisplace(IBlockAccess world, int x, int y, int z) { + if (world.getBlock(x, y, z).isLiquid()) return false; + return super.canDisplace(world, x, y, z); + } + + @Override + public boolean displaceIfPossible(World world, int x, int y, int z) { + if (world.getBlock(x, y, z).isLiquid()) return false; + return super.displaceIfPossible(world, x, y, z); + }*/ + + } In your Block Class: declare them like so: public static Fluid fluidYourFluid; public static BlockYourFluidFluid fluidYourFluidBlock; In Your Block Register Method This is how you register it: fluidYourFluidBlock = new BlockYourFluidFluid(fluidYourFluid, Material.water); GameRegistry.registerBlock(fluidYourFluidBlock, "fluidYourFluid"); Sorry for the fragmentation - I'm working from my change logs to get this in place as I've redone how I handle my fluids but this should get you on the right path. -
Morning all, I'm in the process of working on my mod (TerraCraft) and I'm new (*gasp*) to minecraft mod development, however I am a software developer (c++) by profession and I have made significant strides in my progression. However I need some pointers going forward. I've got the main mechanic of my mod completed, which is biome replacement. Currently I've done the following: - Added new ores/generation - Added respective armors/tools to coincide with the new ores. - Added effects to tools when being used. - Added a basic biome changing block to the game, that can be set down, activated and changes entire biomes (in a server friendly & grief friendly way) - Added a new fluid that when unleashed, will generate new terrain. - Added effects to the world that are under terraformation. What I'm looking to do, and why I'm here is that I'm looking to expand upon the basics and any direction would be good. Ideally, I would like to have a multiblock structure with the following aspects: 1) Intakes fluid mentioned earlier. 2) Intakes power (any kind, RF/MJ/etc) 3) Has an big reactors type frame, but hollow with a custom render in the center. 4) Has a gui that specifies the mode of terraforming 5) Integrate my fluid/ores with the TE/TiCon Api That said - I've seen tutorials for guis, and I've looked at the multi-block tutorial in the minecraft forge, but does anyone else have any resources they used that could help me accomplish these next goals? Also - I am guessing with the rendering/textures that paint.net isn't quite enough.. what tools do you recommend? Thanks for any advice!