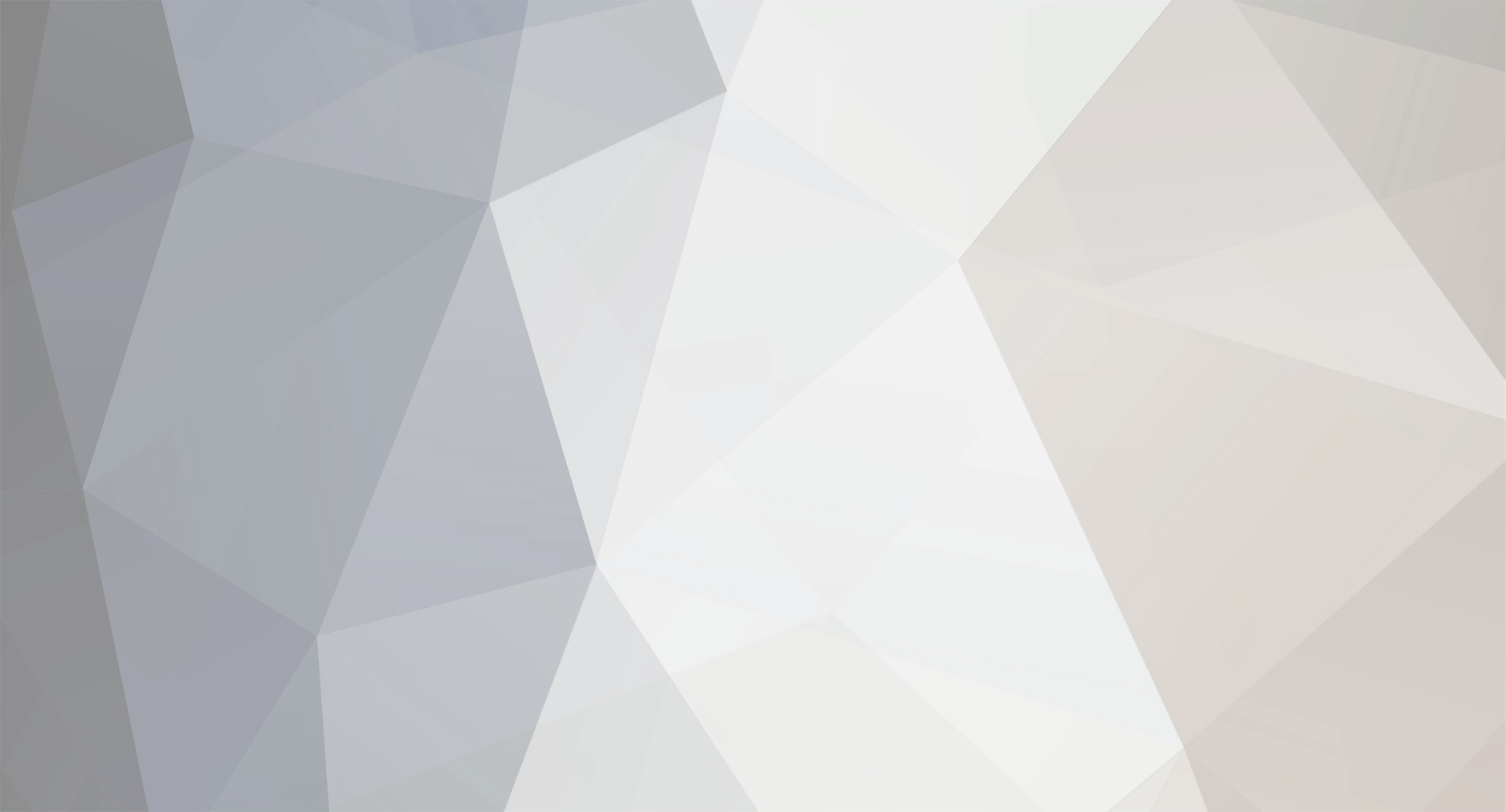
DoktuhParadox
Members-
Posts
21 -
Joined
-
Last visited
Everything posted by DoktuhParadox
-
How would I go about getting the damage from items used in a recipe? Say I wanted to craft a paxel, and it's crafted from all of the tools. To avoid exploitation, the pickaxes and other tools cannot be damaged in order to craft the recipe, but this is bothersome. Is there a way to scan the crafting table, getting the damages of all of the items in the table, and damaging the paxel with the sum of the damages?
-
Two of four subblock's names are the same
DoktuhParadox replied to DoktuhParadox's topic in Modder Support
Fixed it! It turns out that the unlocalized name switch needed the cases for the two other damage values in order to make a different unlocalized name. -
Two of four subblock's names are the same
DoktuhParadox replied to DoktuhParadox's topic in Modder Support
Well, here's the block file now: public class XailiteBlock extends Block { public XailiteBlock(int id) { super(id, Material.rock); this.setCreativeTab(Xailite.xailiteTab); this.setHardness(5.5F); } @SideOnly(Side.CLIENT) private Icon[] blockTextures; public void registerIcons(IconRegister iconRegister) { blockTextures = new Icon[4]; //Change this according to how many blocks there are for (int i = 0; i < blockTextures.length; i++) { blockTextures[i] = iconRegister.registerIcon("xailite:" + (i == 0 ? "XailiteOre" : i == 1 ? "XailiteBlock" : i == 2 ? "TemperedXailiteOre" : i == 3 ? "RefinedXailiteBlock" : null)); } } @Override public Icon getIcon(int id, int metadata) { return blockTextures[metadata]; } @Override public void getSubBlocks(int id, CreativeTabs tab, List subBlockList) { for (int i = 0; i < blockTextures.length; ++i) { subBlockList.add(new ItemStack(id, 1, i)); } } @Override public int damageDropped(int metadata) { this.setUnlocalizedName("XailiteBlock|" + metadata); return metadata; } @Override public boolean isBeaconBase(World worldObj, int x, int y, int z, int beaconX, int beaconY, int beaconZ) { return worldObj.getBlockMetadata(x, y, z) == 1; } public String getUnlocalizedName(ItemStack itemStack) { return this.getUnlocalizedName() + "Xailite"; } } But it doesn't seem to work. And it's definitely the last two blocks having the same unlocalized name. -
Two of four subblock's names are the same
DoktuhParadox replied to DoktuhParadox's topic in Modder Support
Move that to where? The block class or the item block class? -
It may be your tick handler, then. Make sure it implements ITickHandler and it's server side, then use ModLoader.getMinecraftInstance.thePlayer for the player.
-
Wait, the item you're checking for has a damage value?
-
With getCurrentItemOrArmor you're checking the four armor slots. To get the current held item, it's getCurrentEquippedItem which returns an itemstack.
-
I have a block with four subblocks including itself. The first two have their appropriate names, the last two have the same name. I tested adding a fifth sub block, and its name was the same as the other two as well. Here are the snippets of interest: In the Block.class: private Icon[] icons; ... public void registerIcons(IconRegister iconRegister) { blockTextures = new Icon[4]; //Change this according to how many blocks there are for (int i = 0; i < blockTextures.length; i++) { this.setUnlocalizedName("XailiteBlock|" + i); blockTextures[i] = iconRegister.registerIcon("xailite:" + (i == 0 ? "XailiteOre" : i == 1 ? "XailiteBlock" : i == 2 ? "TemperedXailiteOre" : i == 3 ? "RefinedXailiteBlock" : null)); } } In the ItemBlock.class: public String getUnlocalizedName(ItemStack itemstack) { String name; switch (itemstack.getItemDamage()) { case 0: name = "world"; break; case 1: name = "nether"; break; default: name = "broken"; break; } return this.getUnlocalizedName() + "." + name; } Registering the block: GameRegistry.registerBlock(xailiteBlock, ItemXailiteBlock.class, "Xailite" + xailiteBlock.getUnlocalizedName().substring(5)); Giving them display names: LanguageRegistry.addName(new ItemStack(xailiteBlock, 1, 2), "Refined Xailite Block"); LanguageRegistry.addName(new ItemStack(xailiteBlock, 1, 3), "Tempered Xailite Ore"); The two blocks with the same name are the refined block and the tempered ore. They return the correct block when broken, the recipes are correct, etc. the only discrepancy is them having the same name.
-
In the mod I'm developing, the armor has a special attribute; it disappears with the player when the player uses an invisibility potion. It works well, but since the armor disappearance is only visual (it just sets the armor skin texture to an empty .png), mobs can still track the player normally. I already have a tick handler set up for my other armor effects, so it's just a matter of removing the player from mob trackers after a potion check. How would I go about doing this?
-
Bump. Please, I need help.
-
I've made a tile entity similar to a furnace, and it used to work. Now, when I right click it, the game crashes with this: I will post code when/if necessary.
-
Overwriting a configuration file key?
DoktuhParadox replied to DoktuhParadox's topic in Modder Support
Bump because I need this. -
I'm trying to make an ingame GUI to be able to configure my mod easily. I have all of the buttons and other GUI elements down, but I need to know how to overwrite a key in the configuration file. I'm also doing this in a class that's outside the class that the configuration file keys and the file itself are defined in, if that makes a difference.
-
Making a custom configuration category
DoktuhParadox replied to DoktuhParadox's topic in Support & Bug Reports
Thanks, Lex, got it now -
I made a configuration file, and some of the stuff should really be in a different category that isn't a default one. Is there a way to make a custom one?
-
Configurable item ID question
DoktuhParadox replied to DoktuhParadox's topic in Support & Bug Reports
Isn't that why I'm here? -
Configurable item ID question
DoktuhParadox replied to DoktuhParadox's topic in Support & Bug Reports
Well, the file's there, but it keeps saying this in the console 2012-08-22 17:31:09 [iNFO] [sTDOUT] CONFLICT @ 0 item slot already occupied by net.minecraft.src.ItemSpade@62029d75 while adding com.rushmods.powermod.PowerModItemPickaxe@19af9e98 Even when the file's there and the property is, too. Here are the areas of interest: private static int PickItemID; public static final Item PowerPick = new PowerModItemPickaxe(PickItemID, POWER_TOOL); @PreInit public void preInit(FMLPreInitializationEvent event) { event.getModMetadata().version = Version.getVersion(); Configuration cfg = new Configuration(event.getSuggestedConfigurationFile()); try { cfg.load(); PickItemID = cfg.getOrCreateBlockIdProperty("PowerPickID", 9000).getInt(9000); } catch (Exception e) { } finally { cfg.save(); } } And I get no errors but the one about the conflict, and the tool doesn't show up. -
Hi, I would like to add configurable item IDs in to my mod. I know how to make the file and things, but I looked around and found out you need the item declaration inside your @Init (load) method, but I can't do that because of achievements and a few other conditions (the functions that the achievements are in void methods themselves, like onItemPickup and stuff). So, is there a way I could add configurable item ids without completely reformatting everything?
-
The new information file format?
DoktuhParadox replied to DoktuhParadox's topic in Support & Bug Reports
Fixed the parse errors, still doesn't work. Do I need to ship it as a jar, zip, etc.? -
The new information file format?
DoktuhParadox replied to DoktuhParadox's topic in Support & Bug Reports
Still doesn't work. Using 1.3.2, forge 4.x, and here's my file, if you see a problem. Also, if there's something i need to add to my mod_ file to make it load (it loads in MCP and everything) I will do so. { "modinfoversion": 2, "modlist" : [ { "modid": "mod_PowerMod", "name": "The PowerMod", "description": "A mod that adds a new toolset, armor, and more.", "version": "1.3", "mcversion": "1.3.2", "url": "", "updateUrl": "", "authors": [ "DoktuhParadox" ], "credits": "Mien berts!", "logoFile": "", "screenshots": [ "" ], "parent":"mod_PowerMod", "requiredMods": [ "" ], "dependencies": [ "" ], "dependants": [ "", ], "useDependencyInformation": "true", } ] } -
Does anyone have it? I think I do, but my mod still doesn't load, and gives me this in the console: 2012-08-18 00:17:24 [FINE] [ForgeModLoader] Found a candidate zip or jar file Archive.zip 2012-08-18 00:17:24 [FINE] [ForgeModLoader] Examining file minecraft.jar for potential mods 2012-08-18 00:17:25 [FINE] [ForgeModLoader] Examining file Archive.zip for potential mods 2012-08-18 00:17:25 [FINER] [ForgeModLoader] Located mcmod.info file in file Archive.zip 2012-08-18 00:17:25 [WARNING] [ForgeModLoader] Zip file Archive.zip failed to read properly, it will be ignored 2012-08-18 00:17:25 [iNFO] [ForgeModLoader] Forge Mod Loader has identified 2 mods to load 2012-08-18 00:17:25 [FINE] [ForgeModLoader] Received a system property request '' And so on. Anyone have the new format/can give me an example or help me?