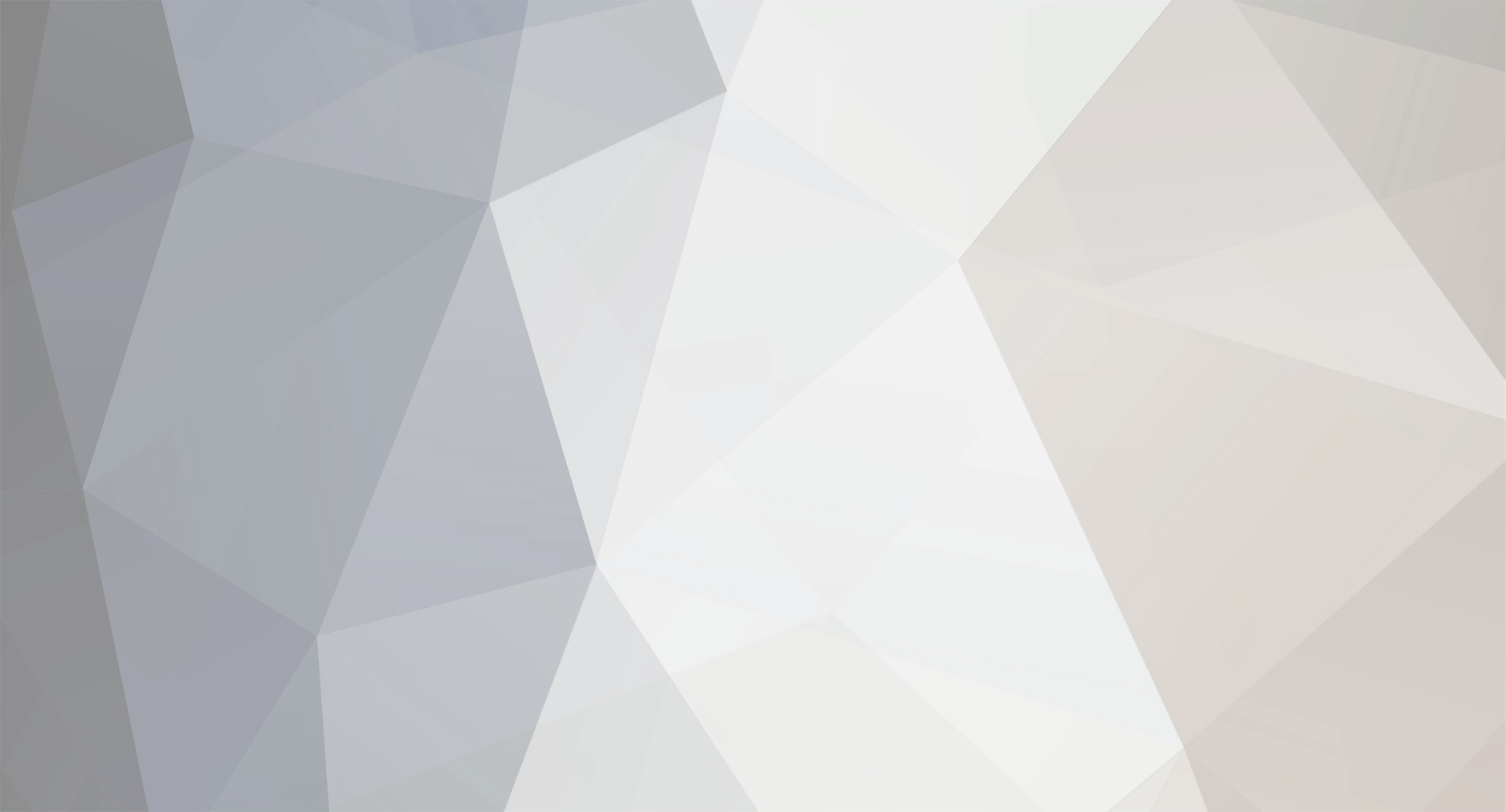
Meastroman
Members-
Posts
14 -
Joined
-
Last visited
Everything posted by Meastroman
-
OK, let's start with this: when coding, no matter what language, GOOGLE IS YOUR FRIEND (on anything else, don't trust google..) So suppose you want you entity that poops out gold ingots. Before you start, think about what classes you need. So to poop gold, you need an entity. To make any entity, see Google, there are MANY tuts on that. However an basic entity is made up of a few classes. Like a render-class, model-class, a main-class etc. Now you got your Entity, it still needs to poop gold. Since we know something in minecraft that already does this, we can use the code from this other entity. (The chicken) The entity shooting chests would be somewhat more complicated, but the basics are the same there. Divide your big problem into smaller, preferably you want every smaller problem to fit in a class. Meaning: "Hey, my entity has no model, so it would be invisible if I were to spawn it!" -> make a ModelEntity class. Since java is an Object Oriented Language, and minecraft follows this very strongly, you need to know more than just java. You might want to read a couple of articles on object oriented languages if you think it will help you out. Good luck
-
To be honest, once you used Github for a month, it's extremely easy. However like a some here said, it can be tricky. I do not know if you are just making an example/test mod right now or if you already started and want to share your code with a friend. However I recommend you creating a testmod or at least a test git repo for the first time. (Just in case you weren't planning on doing this). Now I found that this might be interresting for you: try.github.com/. I know this is a tutorial for using the commandline, but the idea is the same, and I think it would be nice for you to know the basics. I myself have used both commandline git and their git app for mac (not source tree like the guys above, but I have heard some good feedback about it, as other apps around that share the same purpose). I like the git app for mac (and I guess it looks some what the same on Windows/Linux), though it can be confusing since some buttons do not share their name with the commandline counterparts. (For example syncing, because its multiple commands in one button) I think that's all I have to say about it. EDIT: Typo
-
Create a new topic, and include your relevant block and rendering code. Thats better than reusing this topic, especially since OP had a different problem
-
Yes your post fixed it. I found it out a couple minutes before it.. I feel so dumb for this haha. Well thanks guys. So for any of you with this problem, Kudos to TGG his post above me, might fix your problem as well.
-
uhm, nope... It does not print "ITS A HIT" sadly, sorry I forgot to inlcude it in my post I thought entityHit would be the entity taking damage (and after printing it I know it is), and attackEntityFrom would be the function that inflicts damage, and checks if this type of damage does harm the entity. I hope I am on the right track. And yes I did try looking into attackEntityFrom, but since you hinted me there, I looked at it again. Previous time something bothered me. Whenever I printed this.worldObj.isRemote within my onUpdate function it returns true. However EntityLivingBase.attackEntityFrom returns false whenever my world is remote.. But even if I print it at the start of my onUpdate method, it does this. It's never called on the serverside I don't really know why though. I thought my entity was registered properly
-
Well I did, and as I said, the problem is not dispensing my projectile, but damaging entities. I took the code from EntityArrow and removed some lines, mainly parts with this.shootingEntity. However I don't think I need this, since arrows do hurt even if this.shootingEntity is null.
-
Hmm, that must be something from the EntityArrow, I know I tried replacing it with 6.0F, but it made no difference
-
@Helrato Did you add this to your code: @Override public int getRenderType() { return -1; } @Override public boolean shouldSideBeRendered(IBlockAccess iblockaccess, int i, int j, int k, int l){ return false; } @Override public boolean isOpaqueCube(){ return false; } I think it's one of those functions you are missing, I do not understand how one of these might be influenced by your world gen type, though..
-
I think I can help you with 1 and 3: 1. I don't know if I fully understand this, but I'll give it a try. The function updateEntity() might be able to do what you want, or else some of those onNeighborChange() functions, or the equivalent function for redstone 2. Sorry. So far I never tried rendering my own armor, have you been able to find tuts on these? 3. When I tried using integrated git in eclipse, I couldn't get it to work, so instead my buddy and I used Terminal/Commandprompt/app git. Requires an extra window on our desktop but it was way easier... Hope it helps out.
-
I am making my own projectile, and I took quite some code from the EntityArrow class. However, my projectile is unable to inflict damage. I've been staring and testing my code, and for some reason attackEntityFrom() always returns false. Now I think I need to solve one of these 3 problems: 1. Within attackEntityFrom() something with a ForgeHook is being called at the start of the function, and I need to do something with that. 2. I accidentally removed a function I shouldn't have 3. I still need to add some stuff As I said before, most of my code is from EntityArrow.onUpdate(), however I removed some major stuff, this includes getting stopped by blocks and everything with arrowshake, yaw and pitch, inGround. And because I want my projectile spawned by a block, I removed this.shootingEntity as well. I think that last might be my problem, however I'm not sure because an arrow still does damage, even though its not from an entity. My code basically does the following: Look for entities, like the arrow does See if it collides with one Do damage if necessary Update position and motion (homing projectile) Here is my code in case you would like to see it: It's probably something really stupid and easy. However I can't figure it out, even after a couple days, and I'm hoping one of you guys see it..
-
Hey brah, I think you might want to take a look at how BlockStairs or BlockFence render. Meaning it is possible. But I believe these models are included in Minecraft and the way to specify the right model would be through this method: public int getRenderType() { return 10; } where 10 in this case refers to the stair model. Now, as far as I know, forge doesn't allow you to add these render types, so you might be better off with a TileEntity, unless you want to be able to move your block of course. Then it does have to be a normal block. If you REALLY want this, I suggest looking at RenderBlocks.renderBlockByRenderType and the getRenderType() methods
-
[1.7] [SOLVED] Problem with item spawning TileEntity
Meastroman replied to Meastroman's topic in Modder Support
Wow... Thanks so much! I could not believe that actually worked, but it makes a lot of sense now haha. I wasted almost a week of my time on this problem. CASE CLOSED -
Hey fellow Modders! I am trying to make an item that spawns a Multi-block structure in the world. I started with an item that creates 1 BlockContainer with TileEntity. I can't get it to work. Here's what I know so far: - Replacing my custom block for a vanilla block does make it spawn in the world. - Replacing my custom block for a vanilla TileEntity does make it spawn. (I tried the anvil block). - I use all necessary functions used ItemSkull, TileEntitySkull, BlockSkull. (Or at least as far as I know). - world.getTileEntity(x, y, z); returns null. - world.getTileEntity also returns null after I used world.setTileEntity(x, y, z, tileentity);. - Placing the block by hand does make it a working TileEntity - Placing a block by hand calls createNewTileEntity() multiple times (mostly 2 times, but I've seen 3 as well (print statement)). - I did look at numerous Tutorials, topics on this forum, and minecraft code examples (like the skull). Most of them are 1.6 though (some older).. I'll update this list whenever I found a new usefull clue. My guess is I'm forgetting to call a function, but I wouldn't know which one. The main thing I don't understand is why my TileEntity works whenever I place the block by hand, but whenever I use the Item it fails. Here is the code: ItemTurret TileEntityTurret BlockTurret