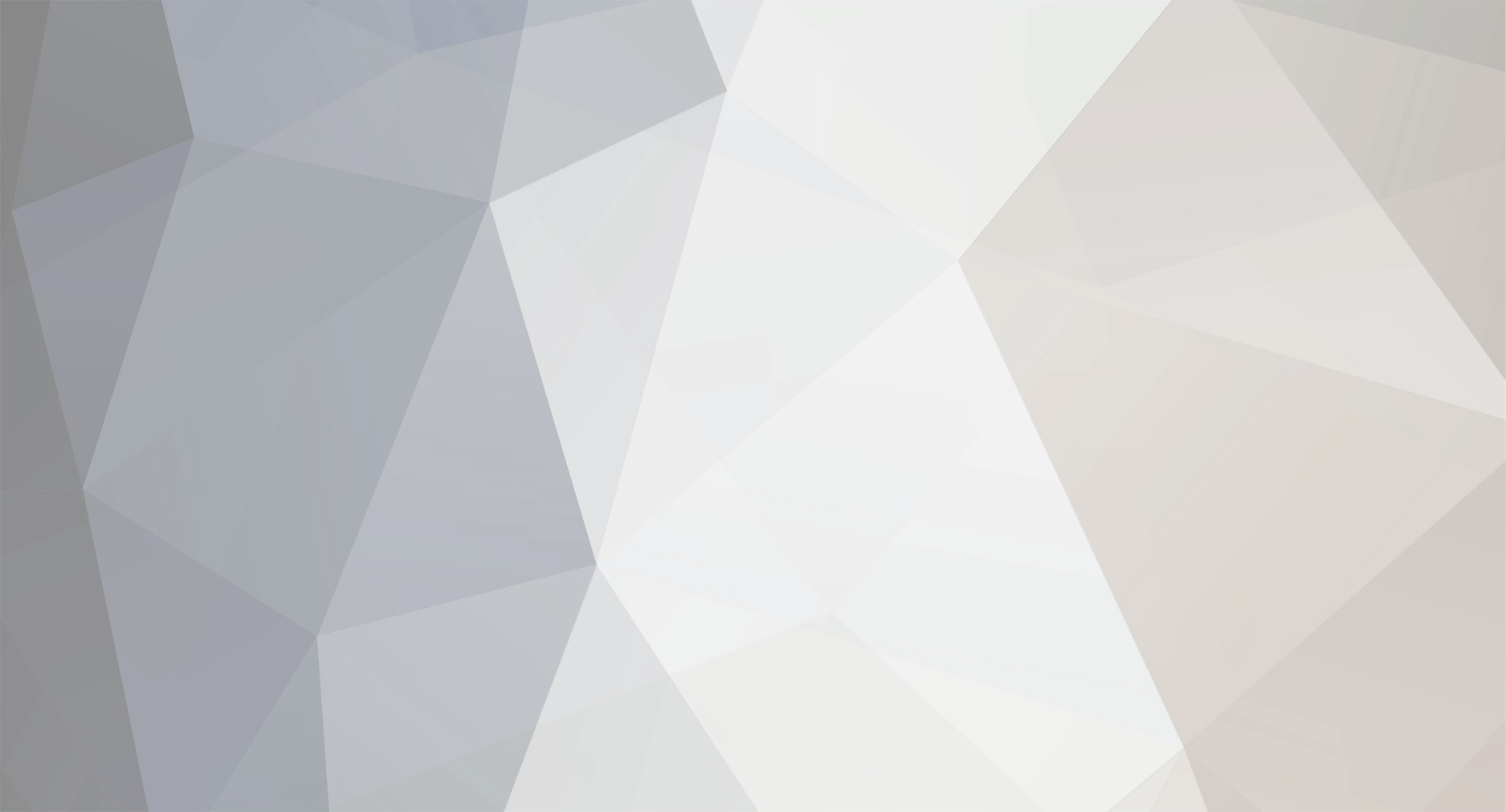
cad97
Forge Modder-
Posts
61 -
Joined
-
Last visited
Everything posted by cad97
-
Ok... this is really weird. With the same code I am getting a different error now ?!!!?? I assume that what you are talking about for caching the getField would be something like this if it weren't giving me the NoSuchFieldException:
-
For the crafting: You will want to make the result of your recipe be a placeholder item as delpi suggested -- I will refer to it as mysteryItem. To accomplish the turning of mysteryItem into one of your random crafting items, I will have the user rightclick whilst holding the mysteryItem. (I can't find a forge event for crafting, just for anvils.) In the mysteryItem class, add this code: private static ItemStack[] craftables = {/*fill this with the appropriate ItemStacks*/}; /** * Called whenever this item is equipped and the right mouse button is * pressed. Args: itemStack, world, entityPlayer */ public ItemStack onItemRightClick(ItemStack par1ItemStack, World par2World, EntityPlayer par3EntityPlayer) { if (!par3EntityPlayer.capabilities.isCreativeMode) { --par1ItemStack.stackSize; } int rand = (int) (Math.random()*craftables.length); par3EntityPlayer.inventory.addItemStackToInventory(craftables[rand]); return par1ItemStack; }
-
Let me do blockdrops first - that is easier. Here is some pseudocode for what you would want to do: public class yourBlock extends Block { yourBlock() { //constructor } /** * Returns the quantity of items to drop on block destruction. */ @Override public int quantityDropped(Random p_149745_1_) { return 1; } /** * Returns the Item dropped by the block when broken. */ @Override public Item getItemDropped(int p_149650_1_, Random p_149650_2_, int p_149650_3_) { Item[] drops; //populate with your possible drops int rand = (int) (Math.random()*drops.length); return drops[rand]; } }
-
I've not messed with Java Reflection before (mainly because I haven't had to and it's such an advanced thing), so I'm probably missing something here, but this is the code that I wrote after doing a bit of research on the javadocs for reflection: @SubscribeEvent public void onSignDone(ActionPerformedEvent.Pre event) throws NoSuchFieldException, SecurityException, IllegalArgumentException, IllegalAccessException // yes I know this throws chain is messy { if (event.gui instanceof GuiEditSign) { GuiEditSign ges = (GuiEditSign) event.gui; TileEntitySign tileSign = (TileEntitySign) ges.getClass().getField("tileSign") .get(null); System.out.println(Arrays.asList(tileSign.signText)); } } However, upon hitting the "Done" on a sign, my Minecraft immediately crashes with this error: (fun side note, the minecraft crash comment was "// Why is it breaking " - exactly what I'm thinking right now )
-
Well, I'm able to grab the GUI with the new GUI hooks! @SubscribeEvent public void on(ActionPerformedEvent.Pre event) { if (event.gui instanceof GuiEditSign) { GuiEditSign ges = (GuiEditSign) event.gui; } } The one problem is this line in GuiEditSign: /** Reference to the sign object. */ private TileEntitySign tileSign; Looks like I'll be overwriting the GuiEditSign after all. Oh well, at least I got a Forge update!
-
At the very least this got me to update Forge I was on 10.12.1.1060 which was the Recommended when I installed Forge on this machine in my enviroment, but now the Recommended build has been updated to 10.12.2.1121, literally last night Aaaaaanyway: in 1060, the only GUI event was GuiOpenEvent. In 1069, however, "bspkrs: New GuiScreen events" was pushed. I'm updating now to have a look. (In other notes, in looking through "all" of the events to find one for this I had only looked in subpackages of net.minecraftforge.event and not net.minecraftforge.client.event. You learn something new every day!)
-
I've been trying to replicate the function of TrazLander's CommandBlockSigns+ MCEdit filter within modded Minecraft. I came up with a system that would work, but I don't know if it is possible to execute within Forge as it is in the latest release. TL;DR : is there a way to hook into TileEntitySign's completion so that I can do things with the text of a sign when placed? (The easy way to do this would just be to make a new block as extension of BlockSign (i.e. CommandBlockSign) but I would like to keep convertibility to vanilla for exporting.) Longer version : The way this mod would work is any time a sign is placed and the player is finished writing to it, the mod would grab it, check if it is a valid sign for the system, and if so, log it into a .json file (the json part works wonderfully, though I spent an evening figuring out how to do it - I'm noobish with Java I/O). When a sign is destroyed the mod would check for a log for the sign and if there was one, remove it. (This is an easy hook.) When executing commands the mod would intercept the command, find tokens (as defined by the filter) and replace them as appropriate, then forward the command (and I believe I have found the token for this). The problem with this system, however, is that I have been unable to find a @SubscribeEvent that I could subscribe to to allow me to do this logging of signs. Any help is appreciated.
-
http://i1279.photobucket.com/albums/y523/textcraft/Jun%202014%20-%202/a77dd69ddfa9e622422c5e5cd7e377b14d5cdedec1b7a8e19dde68c9e22be6dfbf81219d3893f419da39a3ee5e6b4b0d3255bfef95601890afd8070929aa338b0dfc68d48355_zps0c847cf3.png[/img] Hi guys, I'm a long-term student coder and short-term modder that recently got into modding Minecraft. I've never been into big mods/modpacks that change the game dramatically and prefer the smaller mods that enhance or expand upon the magic that is vanilla Minecraft and keep a sense of the same balance. The idea of these mods is to do this - by adding a small and Minecraft-y thing to the game to enhance the vanilla experience. http://i1279.photobucket.com/albums/y523/textcraft/Jun%202014%20-%202/b4c7169dfe3e78612028143ba24081d8751fc806da39a3ee5e6b4b0d3255bfef95601890afd80709da39a3ee5e6b4b0d3255bfef95601890afd80709f1ed27cba5cbba094db5_zps9f2cc829.png[/img] SpawnerCraft allows you to have the power and convenience of moving and creating (standard) spawners in survival. By collecting the essence of the mob, you are able to infuse a mob cage with the mob to create a spawner, or to create spawn eggs. Now, to get villagers into your farm, you just need to murder them, collect their essence, and turn them into spawn eggs! To create a pig farm, just hook up a spawner! (Warning: does not edit the functionality of spawners so mob spawners are subject to the standard rules of mob spawning: conditions must be favorable to mob spawning; i.e. passives have light and grass, aggressive have dark and space.) You can even create a blaze spawner in the overworld so you can finally use that perfect design that requires water. Download here: http://minecraft.curseforge.com/mc-mods/221268-spawnercraft Direct Download: http://minecraft.curseforge.com/mc-mods/221268-spawnercraft/files/2204720/download (CurseForge has a review system to check that files uploaded are not malicious. If a file is not available, that is because it is still under review. If it is, please check back in an hour or so - it should be available then. Thanks!) Source on GitHub: https://github.com/CAD97/SpawnerCraft [spoiler=install] [*]Make sure you have Forge installed. If you don't, grab the Recommended Installer build here and run it. [*]Place the .jar from the download into the mods folder that is generated by Forge. [*]That's it! Launch the game and enjoy! This mod was built with MCForge 10.12.1.1060 and therefore for maximum performance you should use this build. It should work on any higher 1.7.2 Forge build but I cannot guarantee its performance. Other tested Forge releases: 10.12.2.1121 (works) [spoiler=details] Killing mob drops 1 Essence of Mob Four Essence of Mob creates one Agglomeration of Mob Two Agglomeration of Mob (shapeless) creates one Spawn Mob (8 Essence of Mob) Four Agglomeration of Mob creates one Spirit of Mob (16 Essence of Mob) A Spirit of Mob can be placed in the crafting grid to get two Spawn Mob Mob spawners when destroyed drop 1 Empty Spawner Clicking on a Mob Cage with a Spirit of Mob turns it into a Mob Spawner Essence of Mob, Agglomeration of Mob, and Spirit of Mob are colored the same way as and follow the same naming conventions as Spawn Mob; i.e., the sprite is grey and colored by the game and a Spawn Mob for squid is “Spawn Squid,” similarly “Essence of Squid,” “Agglomeration of Squid,” and “Spirit of Squid.” Essence of Mob, Agglomeration of Mob, and Spirit of Mob follow the same damage value assignation of mobs as Spawn Mob Current version: 1.1.1-mc1.7.2 [spoiler=changelog] 1.1.1-mc1.7.2: some refactoring of codebase and commenting for clarity for release of codebase 1.1-mc1.7.2: VASTLY improved sprite images. If someone who can actually sprite wants to make me some, though, please go ahead! 1.0-mc1.7.2: Initial release
-
Oh, and thanks for answering Degubi, but you were a little too slow
-
As seems always the case, the OP has solved their own problem moments after posting it due to finding something they originally overlooked. When looking one more time in net.minecraftforge.event I found that net.minecraftforge.event.BlockEvent held a class HarvestDropsEvent that does exactly what I wanted to. By pushing a(n?) @SubscribeEvent on(HarvestDropsEvent) to MinecraftForge.EVENT_BUS I was able to hook an event that if the block that checks for dropping happens to be an instanceof BlockMonsterSpawner to add an ItemStack(mobCage, 1) to the drop queue. I hope that in the future people searching for how to do Custom Vanilla Block Drops in Forge can find this thread or do what I have done and find it themself so they can continue on and make great (or at least working) mods. My Listening class for those interested to accomplish this task: https://gist.github.com/CAD97/0f67b8f95804a0bd2d90
-
In the mod that I'm working on I need to make it so that BlockMonsterSpawner (vanilla) drops a MobCage (my mod) when it is destroyed. I've looked for a @SubscribeEvent to use but cannot find one for block destruction, and as such have come to believe that there is not a way to do so without editing BlockMonsterSpawner.class. My question, therefore, is twofold: [*]Is there a way to modify or add to vanilla block drops in Forge? (The version I have in my environment is the current Recommended (10.12.1.1060).) [*]If not, as I suspect and have seen several times from repeated googling, how should I go about changing the getItemDropped(int, Random, int) and quantityDropped(Random) methods to make BlockMonsterSpawner drop a MobCage? As is in Eclipse I cannot just edit the Minecraft files as they are read-only (as they should be). Thank you in advance for your help for a beginning modder. If you're interested, my workspace is Eclipse as set up by Wuppy in his tutorial on setting up Forge development enviroment (http://www.wuppy29.com/minecraft/modding-tutorials/wuppys-minecraft-forge-modding-tutorials-for-1-7-set-up-part-2b-advanced-forge-setup/).