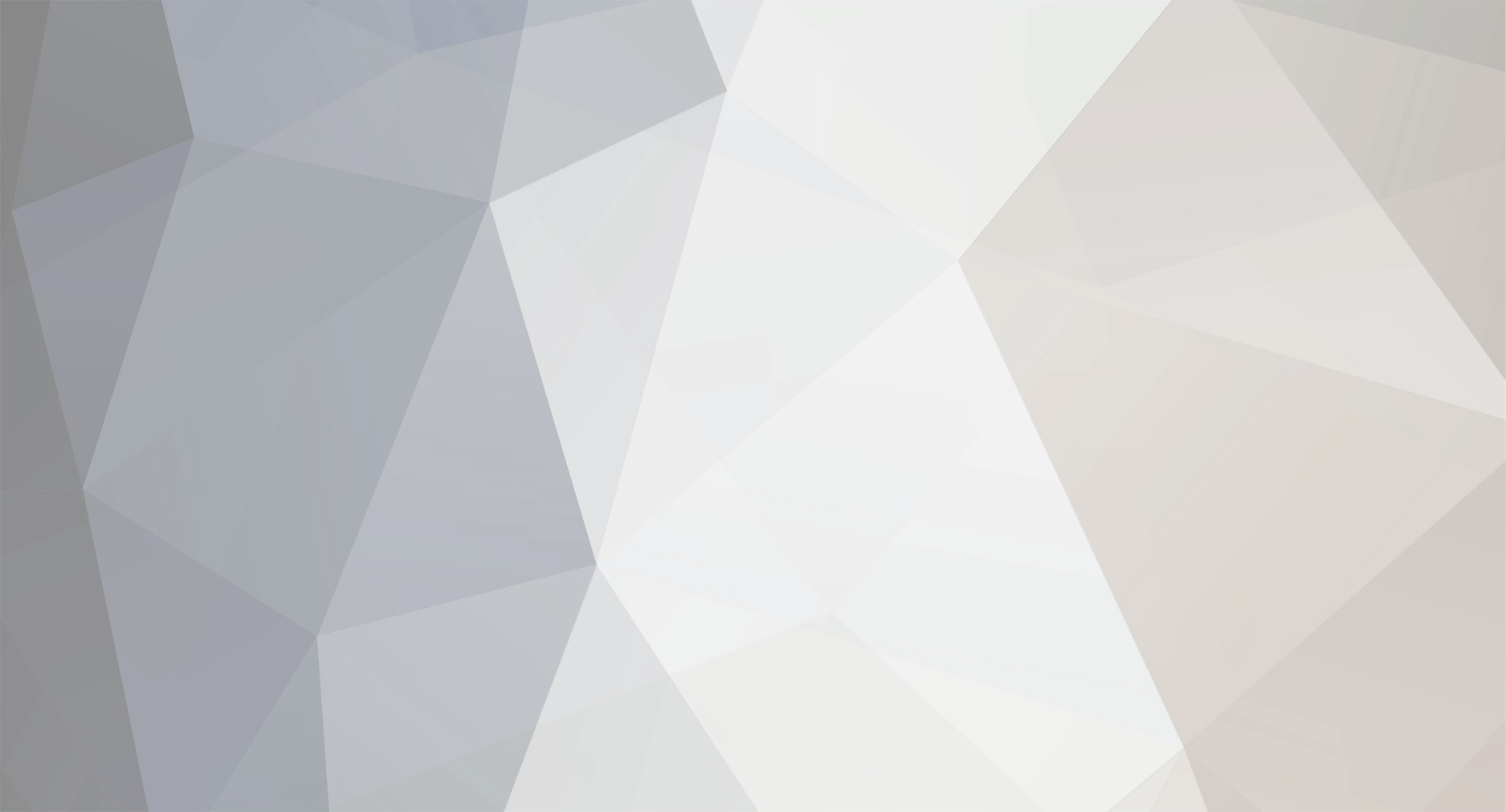
NigNog420
Members-
Posts
16 -
Joined
-
Last visited
Converted
-
Gender
Male
-
Location
Germany
-
Personal Text
I am new!
Recent Profile Visitors
The recent visitors block is disabled and is not being shown to other users.
NigNog420's Achievements

Tree Puncher (2/8)
0
Reputation
-
Hi, this seems like a very tall order and I get that it's probably not supported any more because of 1.7's age, but I've been looking to update my old mod that I last touched years ago and with almost 2000 errors in the code, it feels like it might just be better to start over from scratch. Nonetheless, I want to at least try and see if I can't get it to work and was looking for any resources/documentations that might assist me in updating the old codebase I have. I was also thinking of trying to update it step by step across multiple older versions until I arrive at 1.13.2, but I've been having too much trouble getting those old Forge builds with deprecated Gradle versions set up as they don't support Java 11 so I just skipped that for now. In particular, I was looking for what happened to things like @EventHandler, the FMLIntializationEvent where you used to handle all the block, item and recipe registration and stuff, world and structure generation, entities and entity rendering and what changed with the ClientProxy and CommonProxy as well as the @SidedProxy annotation in the main mod class. Any help would be appreciated.
-
Oh, seems I forgot them... Thanks, works now!
-
I have wanted to create an item that stores a player's experience, it uses a GUI inside of which I have created an int (xpStored) that should increase/decrease when you press a button, while simultaneously removing your experience/giving it back. But it doesn't seem to work. The GUI has three buttons, one that adds 1 to xpStored and removes 1 level, one that adds 1 level and decreases xpStored by 1, and the other one which closes the GUI and writes a chat message to the player, telling him how much Experience he has stored. When I have an amount of levels over 0 and try to press the first button, it executes the action of the close button. My GUI code: package com.nignog.mymod.gui; import org.lwjgl.opengl.GL11; import com.nignog.mymod.MainClass; import net.minecraft.client.Minecraft; import net.minecraft.client.entity.EntityClientPlayerMP; import net.minecraft.client.gui.GuiButton; import net.minecraft.client.gui.GuiScreen; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.util.ChatComponentText; import net.minecraft.util.ResourceLocation; import net.minecraft.world.World; public class XPStorageGui extends GuiScreen { ResourceLocation texture = new ResourceLocation(MainClass.MODID, "textures/gui/background.png"); private final int xSizeTexture = 248; private final int ySizeTexture = 166; public XPStorageGui(EntityPlayer player) { } @Override public void drawScreen(int x, int y, float f) { drawDefaultBackground(); GL11.glColor4f(1F, 1F, 1F, 1F); mc.getMinecraft().getTextureManager().bindTexture(texture); int posX = (this.width - xSizeTexture) / 2; int posY = (this.height - ySizeTexture) / 2; drawTexturedModalRect(posX, posY, 0, 0, xSizeTexture, ySizeTexture); drawCenteredString(fontRendererObj, "XP Storage", posX + 125, (int) (posY + 7.5), 16777215); super.drawScreen(x, y, f); } @Override public boolean doesGuiPauseGame() { return false; } @Override public void initGui() { this.buttonList.clear(); int posX = (this.width - xSizeTexture) / 2; int posY = (this.height - ySizeTexture) / 2; GuiButton XPAdd = new GuiButton(0, posX + 75, posY + 55, 100, 20, "Add XP to storage"); GuiButton XPGet = new GuiButton(0, posX + 75, posY + 85, 100, 20, "Add XP to your bar"); GuiButton close = new GuiButton(0, posX + 100, posY + 115, 50, 20, "Close"); XPAdd.id = 1; XPGet.id = 2; close.id = 3; this.buttonList.add(close); this.buttonList.add(XPAdd); this.buttonList.add(XPGet); } @Override protected void actionPerformed(GuiButton button) { EntityClientPlayerMP player = mc.getMinecraft().thePlayer; World world = mc.getMinecraft().theWorld; int xpStored = 0; switch(button.id) { case 1: if(player.experienceLevel > 0) { player.addExperienceLevel(-1); xpStored++; } else if (player.experienceLevel < 0){ player.closeScreen(); if(world.isRemote) { player.addChatMessage(new ChatComponentText("You don't have any levels!")); } } case 2: if(xpStored > 0) { player.addExperienceLevel(1); xpStored--; } case 3: player.closeScreen(); if(world.isRemote) { player.addChatMessage(new ChatComponentText("Your XP Storage currently holds:" + " " + xpStored + " " + "Levels.")); } } } } I tried adding System.out.println(player.experienceLevel); in case 1 to test whether it can tell the player's experience level amount, and it prints it out correctly.
-
Stupid me. Forgot to register the event. Works now. For other people having this problem, the first code bit works fine.
-
Hello, so, I've created an Entity which extends EntitySnowman, using the same model, scaled to a smaller size, and shooting arrows instead of snowballs. Now, I've wanted to create an event which makes it so if the EntityArrow fired by the Tiny Snowman hits either a player, another Tiny Snowman or the vanilla snowmans, the damage of the arrow is being set to 0, but it doesn't happen. My code (The "ammount" field is not a spelling error on my side): package com.nignog.mymod.events; import net.minecraft.entity.monster.EntitySnowman; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.entity.projectile.EntityArrow; import net.minecraftforge.event.entity.living.LivingHurtEvent; import com.nignog.mymod.entity.EntityTinySnowGolem; import cpw.mods.fml.common.eventhandler.SubscribeEvent; public class TinySnowmanNoPlayerDamage { @SubscribeEvent public void noPlayerDamage(LivingHurtEvent e) { if (e.source.getSourceOfDamage() instanceof EntityArrow && e.source.getEntity() instanceof EntityTinySnowGolem && e.entityLiving instanceof EntityPlayer || e.entityLiving instanceof EntityTinySnowGolem || e.entityLiving instanceof EntitySnowman) { e.ammount = 0F; } } } Before that, I tried this, but that didn't work either: package com.nignog.mymod.events; import net.minecraft.entity.monster.EntitySnowman; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.entity.projectile.EntityArrow; import net.minecraftforge.event.entity.living.LivingHurtEvent; import com.nignog.mymod.entity.EntityTinySnowGolem; import cpw.mods.fml.common.eventhandler.SubscribeEvent; public class TinySnowmanNoPlayerDamage { @SubscribeEvent public void noPlayerDamage(LivingHurtEvent e) { EntityArrow arrow = e.source.getSourceOfDamage(); if (arrow.shootingEntity instanceof EntityTinySnowGolem && e.entityLiving instanceof EntityPlayer || e.entityLiving instanceof EntityTinySnowGolem || e.entityLiving instanceof EntitySnowman) { e.ammount = 0F; } } } Any help? Thanks in advance.
-
[1.7.2] Loot generated in chests multiplies
NigNog420 replied to NigNog420's topic in Modder Support
Apparently, it didn't seem to work. I put it like this: world.setBlock(x + 1, y + 1, z + 1, Blocks.chest); TileEntityChest entityChest = (TileEntityChest) world.getTileEntity(x + 1, y + 1, z + 1); if (entityChest != null) { if (!world.isRemote) { WeightedRandomChestContent.generateChestContents(rand, snipersChestContent, entityChest, 6); } } -
The title is a bit irritating. To better explain: I have created a structure which contains a chest to generate in my biome and most of it works, but I have trouble getting the loot to stay in the chest. I have added a few items to it, they are being generated in the chest as wanted, but the problem is that they multiply around of it. I noticed this happens to the torches, too, as you can see in the picture. First, I tried it by simply adding the item stacks to the tile entity's inventory, now I tried it with the WeightedRandomChestContent function used in WorldGenDungeons, same result. A screenshot: My code for the structure: package com.nignog.mymod.world.gen.structure; import java.util.Random; import net.minecraft.init.Blocks; import net.minecraft.init.Items; import net.minecraft.item.ItemStack; import net.minecraft.tileentity.TileEntityChest; import net.minecraft.util.WeightedRandomChestContent; import net.minecraft.world.World; import net.minecraft.world.gen.feature.WorldGenerator; import com.nignog.mymod.MainClass; public class WorldGenCustomStructure extends WorldGenerator { public static final WeightedRandomChestContent[] snipersChestContent = new WeightedRandomChestContent[] { new WeightedRandomChestContent(MainClass.jarate, 0, 5, 10, 10), new WeightedRandomChestContent(Items.bow, 150, 1, 1, 10), new WeightedRandomChestContent(Items.arrow, 0, 10, 15, 15), new WeightedRandomChestContent(MainClass.flyingStaff, 0, 1, 1, 4), new WeightedRandomChestContent(MainClass.australiumIngot, 0, 1, 5, 12), new WeightedRandomChestContent(new ItemStack( MainClass.hardenedObsidian), 2, 4, 15) }; public boolean generate(World world, Random rand, int x, int y, int z) { if (world.getBlock(x, y, z) != MainClass.australiumDust || world.getBlock(x, y + 1, z) != Blocks.air || world.getBlock(x + 5, y, z) != MainClass.australiumDust || world.getBlock(x + 5, y, z + 6) != MainClass.australiumDust || world.getBlock(x, y, z + 6) != MainClass.australiumDust || world.getBlock(x + 5, y + 1, z) != Blocks.air || world.getBlock(x + 5, y + 1, z + 6) != Blocks.air || world.getBlock(x, y + 1, z + 6) != Blocks.air) { return false; } for (int x1 = 0; x1 < 5; x1++) { for (int y1 = 0; y1 < 5; y1++) { for (int z1 = 0; z1 < 6; z1++) { if ((x1 >= 1 && x1 <= 4) && (y1 >= 1 && y1 <= 4) && (z1 >= 1 && z1 <= 5)) { continue; } world.setBlock(x, y + y1 + 1, z, Blocks.planks); world.setBlock(x + x1, y + 1, z, Blocks.planks); world.setBlock(x + x1, y + 2, z, Blocks.planks); world.setBlock(x + x1, y + 3, z, Blocks.planks); world.setBlock(x + x1, y + 4, z, Blocks.planks); world.setBlock(x + x1, y + 5, z, Blocks.planks); world.setBlock(x, y + 1, z + z1, Blocks.planks); world.setBlock(x, y + 2, z + z1, Blocks.planks); world.setBlock(x, y + 3, z + z1, Blocks.planks); world.setBlock(x, y + 4, z + z1, Blocks.planks); world.setBlock(x, y + 5, z + z1, Blocks.planks); world.setBlock(x + 4, y + y1 + 1, z, Blocks.planks); world.setBlock(x + 4, y + y1 + 1, z + 1, Blocks.planks); world.setBlock(x + 4, y + y1 + 1, z + 2, Blocks.planks); world.setBlock(x + 4, y + y1 + 1, z + 3, Blocks.planks); world.setBlock(x + 4, y + y1 + 1, z + 4, Blocks.planks); world.setBlock(x + 4, y + y1 + 1, z + 5, Blocks.planks); world.setBlock(x + 3, y + y1 + 1, z + 5, Blocks.planks); world.setBlock(x + 1, y + y1 + 1, z + 5, Blocks.planks); world.setBlock(x + 2, y + 3, z + 5, Blocks.planks); world.setBlock(x + 2, y + 4, z + 5, Blocks.planks); world.setBlock(x + 2, y + 5, z + 5, Blocks.planks); for (int xx = 0; xx < 4; xx++) { for (int zz = 0; zz < 4; zz++) { world.setBlock(x + xx + 1, y + 5, z + zz + 1, Blocks.planks); } } world.setBlock(x + x1, y, z + z1, MainClass.australiumDust); for (int z5 = 0; z5 < 5; z5++) { world.setBlock(x, y, z + z5, MainClass.australiumDust); } for (int x5 = 0; x5 < 5; x5++) { world.setBlock(x + x5, y, z, MainClass.australiumDust); } world.setBlock(x + 2, y + 2, z, Blocks.air); world.setBlock(x + 2, y, z + 2, MainClass.australiumBlock); world.setBlock(x + 1, y + 3, z + 2, Blocks.torch); world.setBlock(x + 3, y + 3, z + 2, Blocks.torch); world.setBlock(x + 1, y + 1, z + 1, Blocks.chest); TileEntityChest entityChest = (TileEntityChest) world .getTileEntity(x + 1, y + 1, z + 1); if (entityChest != null) { WeightedRandomChestContent.generateChestContents(rand, snipersChestContent, entityChest, ; } } } } return true; } } My code for the World Generator: package com.nignog.mymod.world.gen; import java.util.Random; import net.minecraft.world.World; import net.minecraft.world.chunk.IChunkProvider; import com.nignog.mymod.world.gen.structure.WorldGenSniper; import cpw.mods.fml.common.IWorldGenerator; public class WorldGeneratorCustom implements IWorldGenerator { public void generate(Random random, int chunkX, int chunkZ, World world, IChunkProvider chunkGenerator, IChunkProvider chunkProvider) { if (world.provider.dimensionId == 0) { generateSurface(world, random, chunkX * 16, chunkZ * 16); } } private void generateSurface(World world, Random random, int blockX, int blockZ) { int Xcoord = blockX + random.nextInt(16); int Ycoord = random.nextInt(128); int Zcoord = blockZ + random.nextInt(16); (new WorldGenCustomStructure()).generate(world, random, Xcoord, Ycoord, Zcoord); } } In my preinit fuction in the MainClass: GameRegistry.registerWorldGenerator(new WorldGeneratorCustom(), 1); Any ideas? Thanks in advance.
-
[1.7.2] Biome Top and Filler Blocks not working with custom blocks
NigNog420 replied to NigNog420's topic in Modder Support
Thank you very much! It works now. -
[1.7.2] Biome Top and Filler Blocks not working with custom blocks
NigNog420 replied to NigNog420's topic in Modder Support
I have left out anything unrelated MainClass: package com.nignog.mymod; @Mod(modid = MainClass.MODID, name = MainClass.NAME, version = MainClass.VERSION) public class MainClass { @SidedProxy(clientSide = "com.nignog.mymod.ClientProxy", serverSide = "com.nignog.mymod.CommonProxy") public static CommonProxy proxy; public static final String MODID = "mymod"; public static final String VERSION = "3.14159"; public static final String NAME = "My Mod"; public static BiomeGenBase biomeAustralia = new BiomeAustralia(42); // Blocks public static Block australiumDust = new AustraliumDust(Material.ground); public static Block diamondOreDisguise = new DiamondOreDisguise( Material.ground); @EventHandler public void preinit(FMLPreInitializationEvent e) { // Biome Registry ModBiomeRegistry.init(); // Block Registry ModBlockRegistry.init(); } @EventHandler public void load(FMLInitializationEvent event) { } @EventHandler public void init(FMLPostInitializationEvent event) { } } BiomeAustralia: package com.nignog.mymod.biomes; import net.minecraft.world.biome.BiomeGenBase; import com.nignog.mymod.MainClass; public class BiomeAustralia extends BiomeGenBase { public BiomeAustralia(int id) { super(id); setBiomeName("Australia"); fillerBlock = MainClass.diamondOreDisguise; topBlock = MainClass.australiumDust; setDisableRain(); setHeight(height_MidPlains); theBiomeDecorator.treesPerChunk = -999; theBiomeDecorator.generateLakes = true; } } ModBiomeRegistry: package com.nignog.mymod; import net.minecraftforge.common.BiomeManager; public class ModBiomeRegistry { public static void init() { BiomeManager.warmBiomes.add(new BiomeManager.BiomeEntry( MainClass.biomeAustralia, 420)); BiomeManager.addSpawnBiome(MainClass.biomeAustralia); } } -
Hello, I wanted to add a new biome, after a few tries and hours of flying around (I even added an Item for increasing the flying speed just to find it), I found my biome, but the top and filler blocks don't generate, but when I choose vanilla blocks, it works. Now, I guess this is because my custom block's ID exceeds 256, as it was in 1.6.4, but since GameRegistry.registerBlock(); automatically registers an ID, I don't know how to go about this problem. Anyone know what to do? Thanks in advance.
-
Alright, thanks for the clarification. Guess I'll have to wait, then.
-
Oh, well, at least you tried, thanks for your help, anyway.
-
GameRegistry.addShapedRecipe(new ItemStack(YourMod.YourItem), "SSS", "SZS", "SZS", 'S', YourMod.YourItem, 'Z', Items.spawn_egg, 1, 54); you need to set it yo be zombie spawn egg like this It is, you don't see it well, but after new ItemStack(NewOres.spawnerBars, 1), there is the 'Z', but then the new line starts, which made it harder to see. I tried without new Object[], but it just works the same way. No zombie spawner, I get a pig spawner, instead.
-
GameRegistry.addShapedRecipe(new ItemStack(YourMod.YourItem), "SSS", "SZS", "SZS", 'S', YourMod.YourItem, 'Z', Items.spawn_egg, 1, 54); you need to set it yo be zombie spawn egg like this It is, you don't see it well, but after new ItemStack(NewOres.spawnerBars, 1), there is the 'Z', but then the new line starts, which made it harder to see.
-
The "Z" is the zombie spawn egg.