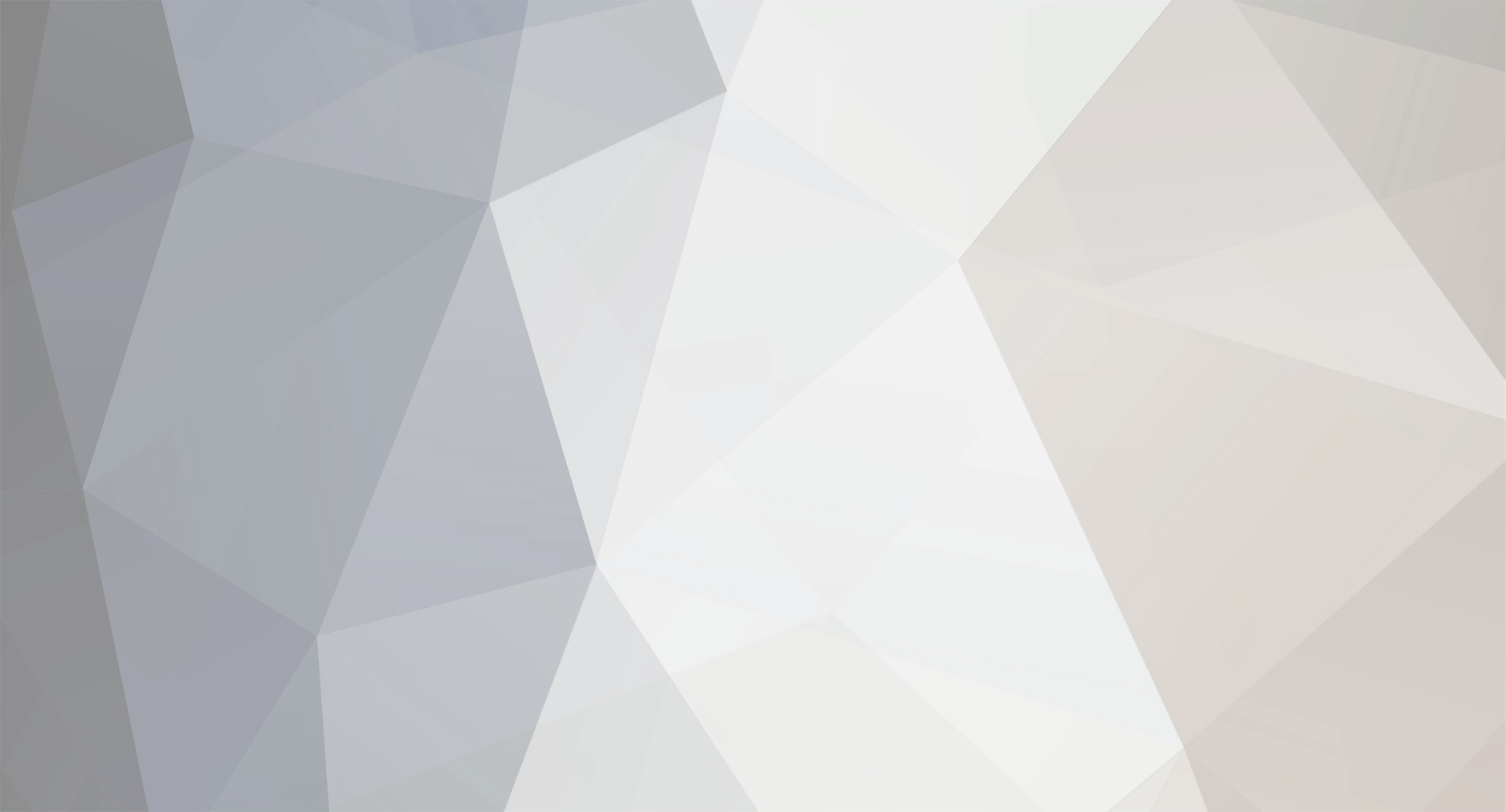
nightscout01
Members-
Posts
7 -
Joined
-
Last visited
Everything posted by nightscout01
-
So, I'm trying to make a trap for one of my new structures that's going to generate in the world. However, I cannot get it to rotate properly. The schematic to java converter gave me this line of code for the dispenser rotation: world.setBlock(x + 4, y + -4, z + 9, Blocks.dispenser, 11, 3); I made a new block for debugging. It just raised more questions. Here is the block's code: package net.morestructures.mod.blocks; import net.minecraft.block.Block; import net.minecraft.block.material.Material; import net.minecraft.client.renderer.texture.IIconRegister; import net.minecraft.entity.Entity; import net.minecraft.entity.EntityLivingBase; import net.minecraft.init.Blocks; import net.minecraft.item.ItemStack; import net.minecraft.tileentity.TileEntityMobSpawner; import net.minecraft.world.World; import net.morestructures.mod.Morestructures; import cpw.mods.fml.relauncher.Side; import cpw.mods.fml.relauncher.SideOnly; public class TestBlock extends Block { Blocks b; public TestBlock(Material material) { super(material); this.setHardness(3.0F); this.setResistance(5.0F); this.setStepSound(soundTypeMetal); this.setCreativeTab(Morestructures.morestructuresTab); } @Override public void onBlockPlacedBy(World world, int x, int y, int z, EntityLivingBase entity, ItemStack itemStack) { super.onBlockPlacedBy(world, x, y, z, entity, itemStack); world.setBlockToAir(x,y,z); world.setBlock(x + 1, y, z, Blocks.dispenser, 11, 5); world.setBlock(x + 2, y, z, Blocks.dispenser, 11, 2); world.setBlock(x + 3, y, z, Blocks.dispenser, 11, 1); world.setBlock(x, y, z, Blocks.dispenser, 11, 3); world.setBlock(x + 4, y, z, Blocks.dispenser, 11, 4); } @SideOnly(Side.CLIENT) public void registerBlockIcons(IIconRegister iconRegister) { this.blockIcon = iconRegister.registerIcon(Morestructures.modid + ":" + this.getUnlocalizedName().substring(5)); } } *Spoilers did not open for me, sorry about that. I did not make a texture for it just to keep it simple. So, This is what happens when I place the block: Then, I broke off the block on the end, and placed my block in the exact same spot as before. Then this happened: On a side note, If anyone knows how to make a dispenser's tile entity inventory be many potions of harming II, please tell me how! Thanks for your help, nightscout01
-
[1.7.2] world.getblock and check room for spawning
nightscout01 replied to nightscout01's topic in Modder Support
Thank you very much for all your help . I am currently only using the array once, so I will use your second option. Thanks again, nightscout01. -
[1.7.2] world.getblock and check room for spawning
nightscout01 replied to nightscout01's topic in Modder Support
Pretty much all I'm trying to do right now, is trying to make it check for two blocks. Such as a list of Valid spawn blocks, like "It can spawn on grass or dirt or stone". Thanks for your help, nightscout01. -
So, I am trying to get the current block of some cords: namely (x,y,z) and then return if the block is different then the one listed. However, I do not know how to check for multiple blocks. I have tried the java "or" function and tried an array, how does one go about this, nighscout01 My world.getblock code: if (world.getBlock(x, y, z) !=Blocks.grass) return; if (world.getBlock(x + 15, y, z) != Blocks.grass) return; if (world.getBlock(x + 15, y, z + 12) != Blocks.grass) return; if (world.getBlock(x, y, z + 12) != Blocks.grass) return; On a similar note, this is the "check room and blocks for spawning" file the converter gives me, however, I cannot figure out on how to implement this method of doing it into my current structure gen code. protected Block[] GetValidSpawnBlocks() { return new Block[] { Blocks.grass, }; } public boolean LocationIsValidSpawn(World world, int i, int j, int k) { int distanceToAir = 0; Block checkBlock = world.getBlock(i, j, k); while (checkBlock != Blocks.air) { distanceToAir++; checkBlock = world.getBlock(i, j + distanceToAir, k); } if (distanceToAir > 1) { return false; } j += distanceToAir - 1; Block block = world.getBlock(i, j, k); Block blockAbove = world.getBlock(i, j + 1, k); Block blockBelow = world.getBlock(i, j - 1, k); for (Block l : GetValidSpawnBlocks()) { if (blockAbove != Blocks.air) { return false; } if (block == l) { return true; } else if (block == Blocks.snow_layer && blockBelow == l) { return true; } else if (block.getMaterial() == Material.plants && blockBelow == l) { return true; } } return false; } My current structure gen file Thanks, and please help, nightscout01
-
bump for more help
-
There are no reported errors in my code and everything but the structure generation works fine. It simply does not generate the structure.
-
Hi everyone, I am trying to make a mod that allows more structures to generate in the world, however, my code does not seem to be working. Can anyone tell me what's wrong with it? My main mod class file package net.morestructures.mod; import net.minecraft.block.Block; import net.minecraft.block.material.Material; import net.minecraft.creativetab.CreativeTabs; import net.minecraft.item.Item; import net.minecraft.item.ItemStack; import net.morestructures.mod.blocks.BuildersBlock; import net.morestructures.mod.blocks.OreBlock; import net.morestructures.mod.items.MCItems; import net.morestructures.mod.worldgen.MorestructureWorldGen; import net.morestructures.mod.worldgen.Test; import cpw.mods.fml.common.IWorldGenerator; import cpw.mods.fml.common.Mod; import cpw.mods.fml.common.Mod.EventHandler; import cpw.mods.fml.common.event.FMLInitializationEvent; import cpw.mods.fml.common.event.FMLPostInitializationEvent; import cpw.mods.fml.common.event.FMLPreInitializationEvent; import cpw.mods.fml.common.registry.GameRegistry; import cpw.mods.fml.relauncher.Side; import cpw.mods.fml.relauncher.SideOnly; @Mod(modid = Morestructures.modid, version = Morestructures.version) public class Morestructures { public static final String modid = "Morestructures"; public static final String version = "Alpha 0.1"; MorestructureWorldGen eventWorldGen = new MorestructureWorldGen(); public static CreativeTabs morestructuresTab; public static Item itemBuildersIngot; public static Item itemStructureIngot; public static Item itemUnusedIngot; public static IWorldGenerator test = new Test(); public static Block oreBuildersOre; public static Block oreStructureOre; public static Block oreUnusedOre; public static Block blockBuildersBlock; @EventHandler public void PreInit(FMLPreInitializationEvent preEvent){ morestructuresTab = new CreativeTabs("Morestructures") { @SideOnly(Side.CLIENT) public Item getTabIconItem() { return Item.getItemFromBlock(Morestructures.oreBuildersOre); } }; itemBuildersIngot = new MCItems().setUnlocalizedName("BuildersIngot"); GameRegistry.registerItem(itemBuildersIngot, "Builders Ingot"); itemStructureIngot = new MCItems().setUnlocalizedName("StructureIngot"); GameRegistry.registerItem(itemStructureIngot, "Structure Ingot"); itemUnusedIngot = new MCItems().setUnlocalizedName("UnusedIngot"); GameRegistry.registerItem(itemUnusedIngot, "UnusedIngot"); oreBuildersOre = new OreBlock(Material.rock).setBlockName("BuildersOre"); GameRegistry.registerBlock(oreBuildersOre, "BuildersOre"); oreStructureOre = new OreBlock(Material.rock).setBlockName("StructureOre"); GameRegistry.registerBlock(oreStructureOre, "StructureOre"); oreUnusedOre = new OreBlock(Material.rock).setBlockName("UnusedOre"); GameRegistry.registerBlock(oreUnusedOre, "UnusedOre"); blockBuildersBlock = new BuildersBlock(Material.iron).setBlockName("BuildersBlock"); GameRegistry.registerBlock(blockBuildersBlock, "BuildersBlock"); //Spawn GameRegistry.registerWorldGenerator(eventWorldGen, 0); } @EventHandler public void Init(FMLInitializationEvent event){ //Recipes GameRegistry.addRecipe(new ItemStack(blockBuildersBlock), new Object[]{"CCC", "CCC", "CCC", 'C', itemBuildersIngot}); //Smelting GameRegistry.addSmelting(oreBuildersOre, new ItemStack(itemBuildersIngot), 0); GameRegistry.addSmelting(oreStructureOre, new ItemStack(itemStructureIngot), 0); GameRegistry.addSmelting(oreUnusedOre, new ItemStack(itemUnusedIngot), 0); GameRegistry.registerWorldGenerator(test, 1); } @EventHandler public void PostInit(FMLPostInitializationEvent postEvent){ } } [/Code] My Test world Gen file: [Code] package net.morestructures.mod.worldgen; import java.util.Random; import net.minecraft.block.Block; import net.minecraft.init.Blocks; import net.minecraft.world.World; import net.minecraft.world.biome.BiomeGenBase; import net.minecraft.world.biome.BiomeGenPlains; import net.minecraft.world.chunk.IChunkProvider; import net.minecraft.world.gen.feature.WorldGenMinable; import cpw.mods.fml.common.IWorldGenerator; public class Test implements IWorldGenerator { @Override public void generate(Random random, int chunkX, int chunkZ, World world, IChunkProvider chunkGenerator, IChunkProvider chunkProvider) { switch(world.provider.dimensionId) { case -1: generateNether(world, random, chunkX * 16, chunkZ * 16); break; case 0: generateSurface(world, random, chunkX * 16, chunkZ * 16); break; case 1: generateEnd(world, random, chunkX * 16, chunkZ * 16); break; } } private void generateEnd(World w, Random r, int i, int j) { } private void generateSurface(World world, Random random, int i2, int k2) { int i = i2 + random.nextInt(15); int k = k2 + random.nextInt(15); int j = world.getHeightValue(i,k)-1; if(world.getWorldChunkManager().getBiomeGenAt(i,j)==BiomeGenBase.plains){{ place(true); if((random.nextInt(1000000)+1)<=100000){ boolean place = true; if(place){ world.setBlock(i+0, j+0, k+0, Block.getBlockById(44), 3, 2); world.setBlock(i+1, j+0, k+0, Block.getBlockById(44), 3, 2); world.setBlock(i+2, j+0, k+0, Block.getBlockById(44), 3, 2); world.setBlock(i+3, j+0, k+0, Block.getBlockById(44), 3, 2); world.setBlock(i+4, j+0, k+0, Block.getBlockById(44), 3, 2); world.setBlock(i+0, j+0, k+1, Block.getBlockById(44), 3, 2); world.setBlock(i+1, j+0, k+1, Block.getBlockById(4), 0, 2); world.setBlock(i+2, j+0, k+1, Block.getBlockById(4), 0, 2); world.setBlock(i+3, j+0, k+1, Block.getBlockById(4), 0, 2); world.setBlock(i+4, j+0, k+1, Block.getBlockById(44), 3, 2); world.setBlock(i+0, j+0, k+2, Block.getBlockById(44), 3, 2); world.setBlock(i+1, j+0, k+2, Block.getBlockById(4), 0, 2); world.setBlock(i+2, j+0, k+2, Block.getBlockById(9), 0, 2); world.setBlock(i+3, j+0, k+2, Block.getBlockById(4), 0, 2); world.setBlock(i+4, j+0, k+2, Block.getBlockById(44), 3, 2); world.setBlock(i+0, j+0, k+3, Block.getBlockById(44), 3, 2); world.setBlock(i+1, j+0, k+3, Block.getBlockById(4), 0, 2); world.setBlock(i+2, j+0, k+3, Block.getBlockById(4), 0, 2); world.setBlock(i+3, j+0, k+3, Block.getBlockById(4), 0, 2); world.setBlock(i+4, j+0, k+3, Block.getBlockById(44), 3, 2); world.setBlock(i+0, j+0, k+4, Block.getBlockById(44), 3, 2); world.setBlock(i+1, j+0, k+4, Block.getBlockById(44), 3, 2); world.setBlock(i+2, j+0, k+4, Block.getBlockById(44), 3, 2); world.setBlock(i+3, j+0, k+4, Block.getBlockById(44), 3, 2); world.setBlock(i+4, j+0, k+4, Block.getBlockById(44), 3, 2); world.setBlock(i+1, j+1, k+1, Block.getBlockById(4), 0, 2); world.setBlock(i+3, j+1, k+1, Block.getBlockById(4), 0, 2); world.setBlock(i+1, j+1, k+3, Block.getBlockById(4), 0, 2); world.setBlock(i+3, j+1, k+3, Block.getBlockById(4), 0, 2); world.setBlock(i+1, j+2, k+1, Block.getBlockById(4), 0, 2); world.setBlock(i+3, j+2, k+1, Block.getBlockById(4), 0, 2); world.setBlock(i+1, j+2, k+3, Block.getBlockById(4), 0, 2); world.setBlock(i+3, j+2, k+3, Block.getBlockById(4), 0, 2); world.setBlock(i+1, j+3, k+1, Block.getBlockById(4), 0, 2); world.setBlock(i+3, j+3, k+1, Block.getBlockById(4), 0, 2); world.setBlock(i+1, j+3, k+3, Block.getBlockById(4), 0, 2); world.setBlock(i+3, j+3, k+3, Block.getBlockById(4), 0, 2); world.setBlock(i+1, j+4, k+1, Block.getBlockById(4), 0, 2); world.setBlock(i+2, j+4, k+1, Block.getBlockById(4), 0, 2); world.setBlock(i+3, j+4, k+1, Block.getBlockById(4), 0, 2); world.setBlock(i+1, j+4, k+2, Block.getBlockById(4), 0, 2); world.setBlock(i+2, j+4, k+2, Block.getBlockById(4), 0, 2); world.setBlock(i+3, j+4, k+2, Block.getBlockById(4), 0, 2); world.setBlock(i+1, j+4, k+3, Block.getBlockById(4), 0, 2); world.setBlock(i+2, j+4, k+3, Block.getBlockById(4), 0, 2); world.setBlock(i+3, j+4, k+3, Block.getBlockById(4), 0, 2); }}}} } private void place(boolean b) { } private void generateNether(World w, Random rand, int i, int j) { } } [/Code] Thanks for your help, nightscout01