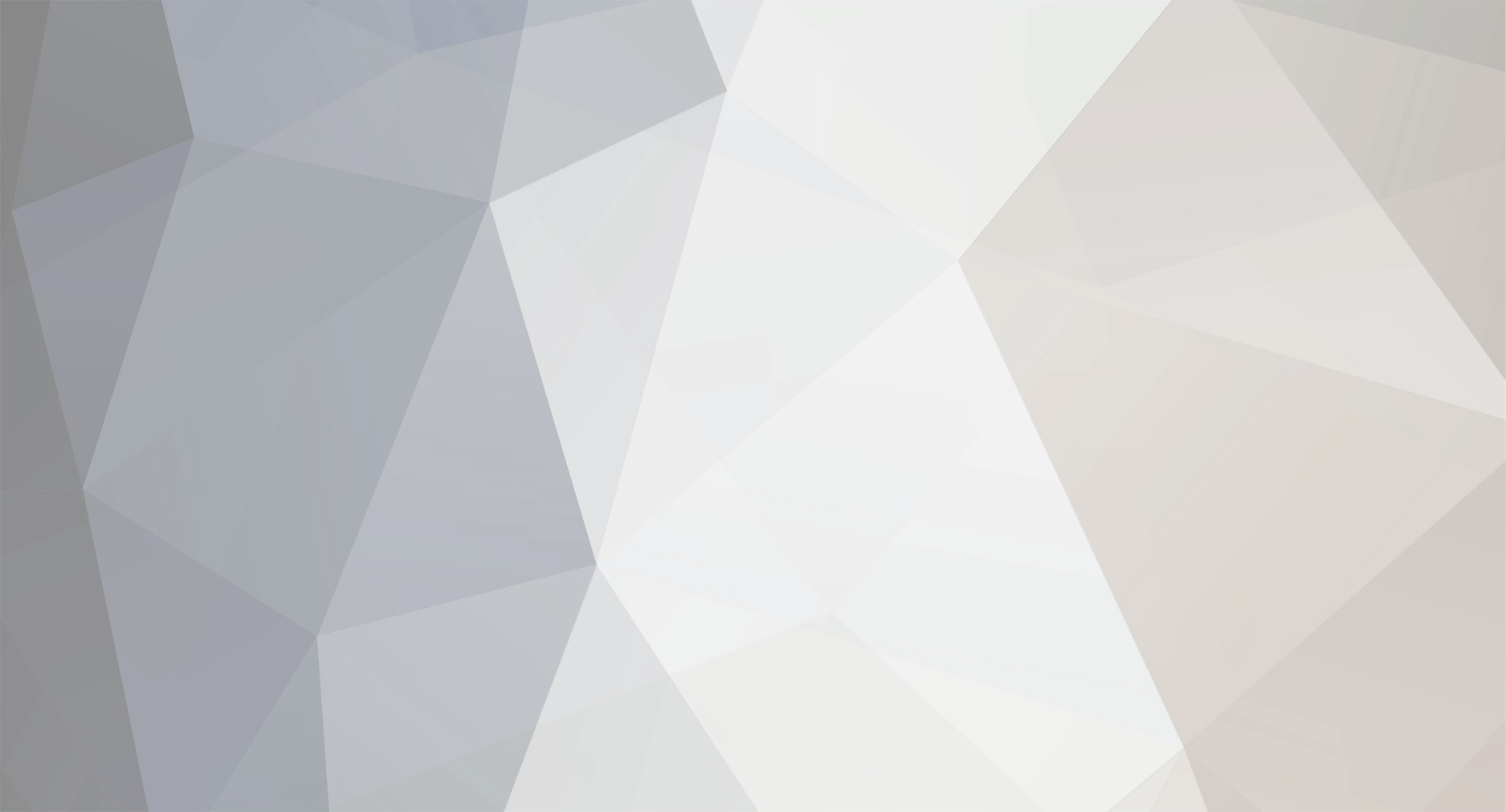
DjGiannuzz
Members-
Posts
24 -
Joined
-
Last visited
-
Days Won
1
Everything posted by DjGiannuzz
-
This is the first time I'm working with ASM, so please, be gentle. My mod is finished and works perfectly. I have my IFMLLoadingPlugin class and my IClassTransformer that do their job, but when I export the mod and test it with MultiMC the mod doesn't load as it should. The transform method get called and gives no error at all, but when minecraft launches my mod isn't in the modlist and nothing happens, like it wasn't there. I've been searching on Google for a while but i haven't find anyhing helpful, maybe I'm forgetting something.
-
Ok, I fixed the code, now it finally works. I'll post it, just in case anybody needs it. public static final double TWICE_PI = Math.PI*2; private static Tessellator tessellator = Tessellator.getInstance(); private static WorldRenderer worldRenderer = tessellator.getWorldRenderer(); public static void drawRegularPolygon(double x, double y, int radius, int sides) { GL11.glEnable(GL11.GL_BLEND); GL11.glDisable(GL11.GL_TEXTURE_2D); GL11.glBlendFunc(GL11.GL_SRC_ALPHA, GL11.GL_ONE_MINUS_SRC_ALPHA); worldRenderer.begin(GL11.GL_TRIANGLE_FAN, DefaultVertexFormats.POSITION); worldRenderer.pos(x, y, 0).endVertex(); for(int i = 0; i <= sides ;i++) { double angle = (TWICE_PI * i / sides) + Math.toRadians(180); worldRenderer.pos(x + Math.sin(angle) * radius, y + Math.cos(angle) * radius, 0).endVertex(); } tessellator.draw(); GL11.glEnable(GL11.GL_TEXTURE_2D); GL11.glDisable(GL11.GL_BLEND); } This will draw a regular polygon with <sides>sides. If you set it high enough, obviosly, you will get a circle.
-
Ok, looks like the problem was another one. I came up with this: private static final double twicePI = Math.PI*2; private static void drawCircle(double x, double y, int radius, int slices) { GL11.glBegin(GL11.GL_TRIANGLE_FAN); GL11.glVertex2d(x, y); for(int i = 0; i <= slices;i++) { GL11.glVertex2d(x + (radius * Math.cos(i * twicePI / slices)), y + (radius * Math.sin(i * twicePI / slices))); } GL11.glEnd(); } This should render a filled circle, centered at (x,y), but i doesn't render anything. If i replace "GL_TRIANGLE_FAN" with "GL_TRIANGLE_STRIPES" and I add "GlStateManager.disableTexture2D();" before I get something, but it's not what i want. Am I forgetting anything?
-
Yes, I know, but I need to draw it in slices of different colors and I have a dynamic number of slices. I really can't find anything on the new renderer class.
-
I just need to draw a filled circle, that's it, but I can't find anything helpful online. Everything I tried doesn't work. I'm inside the drawScreen method of a GuiScreen class.
-
diesieben07 helped me on the #minecraftforge irc channel. You just need to add this to the class that extends CommandBase: @Override public int getRequiredPermissionLevel() { return 0; }
-
I've tried this, but it doesn't work: @EventHandler public void init(FMLInitializationEvent event) { proxy.init(); ClientCommandHandler.instance.registerCommand(new CommandParty()); } I moved the line into my ClientProxy but, as expected, nothing changed.
-
Hi guys, again after several researches on Google I'm asking for your help. I'm writing a ClientOnly mod and I would like to add a Chat Command and register it only on the Client Side. In all the tutorials that I've found they register the Command class on the server. It is possible to register the Command on the client side only?
-
That's exactly what I was looking for. That list will help me a lot. Thank you!
-
I'm looking for some kind of event that triggers when a client receive a Chat message. I've tried using the ChatListener, but it seems to be removed from Forge. I can't find anything similar on Google. There's the ServerChatEvent but it triggers when a chat message is sent, and not received. I'm making a Client Only mod.
-
Thank you
-
I've seen many modders using the "@VERSION@" String instead of the actual version in the mod references. I've tried to google it many times, without result. Anyone have a good tutorial that I can follow?
-
THAT is what I was looking for. Thank you!
-
Read carefully. In my gui, that the player can be open from any dimension I have many dimensionId. If one of the dimensionIds is -1(while the player is in the overworld) I have to print Nether, not Overworld.
-
I know, but I need only the Name of the dimension. I'm quite sure that is saved somewhere in the client, i would like to know where.. The only thing that comes to mind at the moment is to send a packet from the server to the client with all dimension name and is respective dimensionId when the world loads.
-
Hi all, I have to draw in a Gui the name of a dimension having only the dimensionId. On the server i just do DimensionManager.getWorld(dimensionId).provider.getDimensionName(), but if I call it from the client it will obviously crash the client. So, anyone know a method to get the provider(or directly the name) of a dimension having the dimensionID?
-
Have you tried it with a minimap mod? I saw this type of rendering having issues with minimaps.
-
[Solved]TileEntity with world set to null
DjGiannuzz replied to DjGiannuzz's topic in Modder Support
I did that before reading you reply. It works, thank you! (I have to learn how that threads stuff works.) -
[Solved]TileEntity with world set to null
DjGiannuzz replied to DjGiannuzz's topic in Modder Support
So when? I don't like to do that every tick or every 'n' tick. -
Hi all, I have a problem with a TileEntity on World load. I save the dimensionId, xCoord, yCoord, zCoord of the TileEntity in a global variable every time the method readFromNBT is called. The problems come if there are no player around and it seems that the world of the TileEntity is set to null. @Override public void readFromNBT(NBTTagCompound par1NBTTagCompound) { super.readFromNBT(par1NBTTagCompound); this.isLocked = par1NBTTagCompound.getBoolean("isLocked"); this.isPrivate = par1NBTTagCompound.getBoolean("isPrivate"); this.isGlobal = par1NBTTagCompound.getBoolean("isGlobal"); String owner = par1NBTTagCompound.getString("Owner"); this.ownerUsername =owner.equals("undef")?"":owner; String privName = par1NBTTagCompound.getString("privateName"); this.privateName = privName.equals("undef")?"":privName; String globName = par1NBTTagCompound.getString("globalName"); this.globalName = globName.equals("undef")?"":globName; if(worldObj != null) { if(isPrivate) FastTravelHandler.addPrivatePoint(ownerUsername, worldObj.provider.dimensionId, xCoord, yCoord, zCoord, privateName); if(isGlobal) FastTravelHandler.addGlobalPoint(worldObj.provider.dimensionId, xCoord, yCoord, zCoord, globalName); } else LogHelper.error("The world is null"); The TileEntity is Chunkloaded and it works for sure.(if I put a print inside the updateEntity() method I get the message in the console) Unfortunately I get the "The world is null" message from the TileEntity that is far away from the player every time I load the world. I've tried to save the dimensionId in the NBTTag and use it instead of worldObj.provider.dimensionId but I get this crashlog that looks like a strange loop: http://pastebin.com/2wAVQwiH
-
I just had an idea, and it worked. You have to register your EventHandler on the FML bus to make it works. Like this: FMLCommonHandler.instance().bus().register(new HEventHandler()); instead of MinecraftForge.EVENT_BUS.register(new HEventHandler());
-
Hi, I have an EvenHandler class with the PlayerRespawnEvent in it and it seems to not want to behave. I was using the Recommended version of Forge(10.13.0.1180), but I tried it even on the Latest version(10.13.0.1208) and it still doesn't work. This is my EventHandler class: public class HEventHandler { @SubscribeEvent(priority = EventPriority.NORMAL) public void playerRespawn(PlayerInteractEvent event) { LogHelper.info("INTERACT"); } @SubscribeEvent(priority = EventPriority.NORMAL) public void playerRespawn(PlayerRespawnEvent event) { LogHelper.info("RESPAWN"); } } And it's registered here: @EventHandler public void init(FMLInitializationEvent event) { MinecraftForge.EVENT_BUS.register(new HEventHandler()); proxy.init(); } In the console I get the "INTERACT" message when a player triggers it, so the EventHandler is working fine, I don't get the "RESPAWN" message when a player die and respawn.