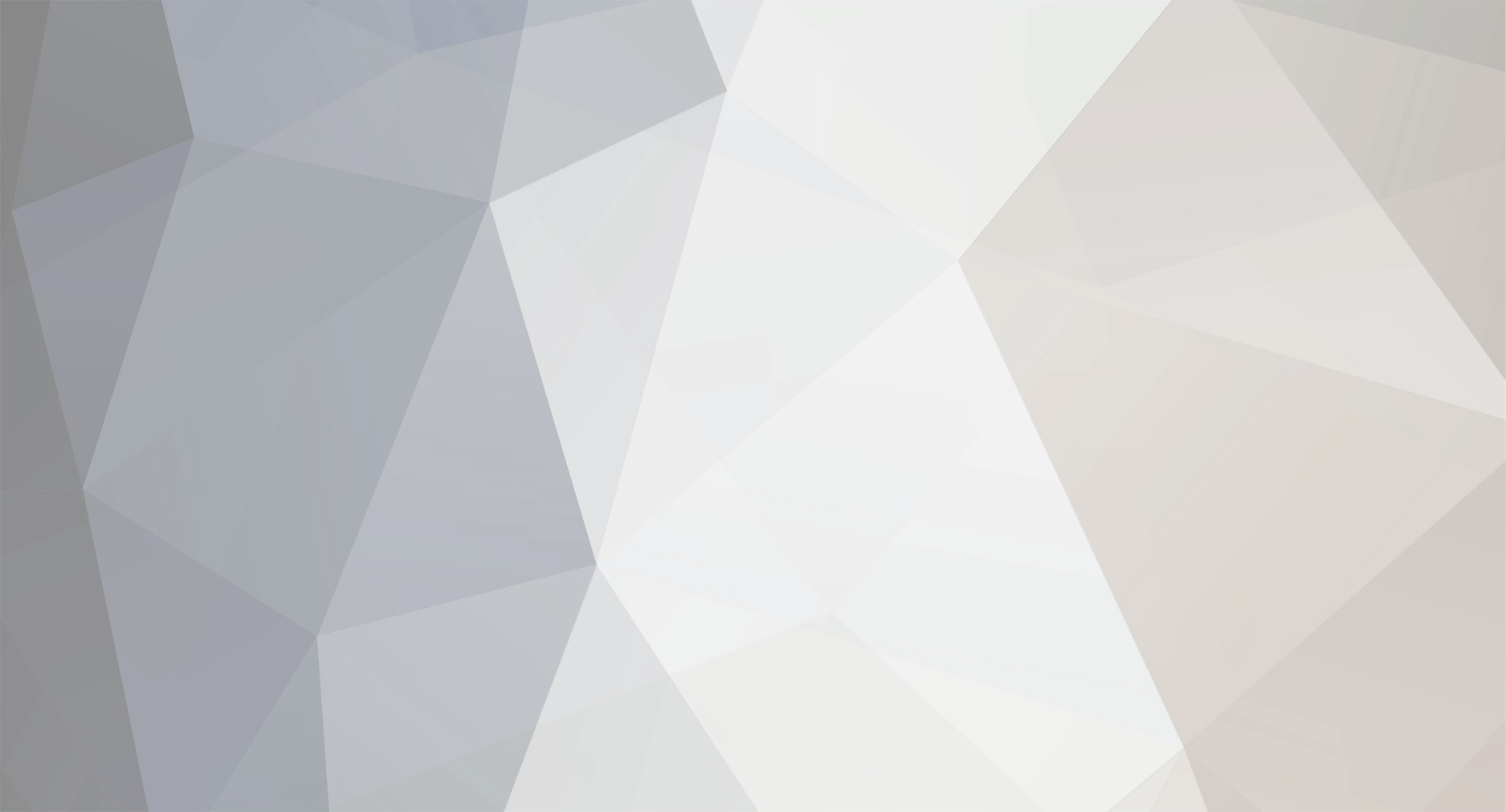
hilburn
Members-
Posts
10 -
Joined
-
Last visited
Everything posted by hilburn
-
if (storedRenderer!=null){ storedRenderer.renderItem(type, thisStack, data); } Actually handles all the custom renderers by checking for the existence of one in the Renderer Registry, all the rest of the code is basically just vanilla item rendering
-
I have tried both renderItemIntoGUI and renderItemWithEffectsIntoGUI both to no avail unfortunately Don't worry - I am a huge fan of stupid questions when it comes to debugging annoying code Here's a quick album (sorry for night screenshots)
-
Thanks for the advice - I've got closer (a lot closer) but I've hit a ridiculous wall - the easiest part of the whole thing, rendering basic items in the inventory doesn't work, no matter what method I use to try to do it: tesselator, GL, .renderIcon - all result in an empty looking inventory slot. If you have any ideas about what's going wrong in this, that'd be awesome package com.hilburn.dimensionguard.client; import net.minecraft.block.Block; import net.minecraft.client.Minecraft; import net.minecraft.client.renderer.OpenGlHelper; import net.minecraft.client.renderer.RenderBlocks; import net.minecraft.client.renderer.Tessellator; import net.minecraft.client.renderer.entity.RenderItem; import net.minecraft.client.renderer.texture.TextureManager; import net.minecraft.item.Item; import net.minecraft.item.ItemBlock; import net.minecraft.item.ItemStack; import net.minecraft.nbt.NBTTagCompound; import net.minecraft.util.IIcon; import net.minecraft.util.ResourceLocation; import net.minecraftforge.client.IItemRenderer; import net.minecraftforge.client.MinecraftForgeClient; import org.lwjgl.opengl.GL11; import org.lwjgl.opengl.GL12; public class DisabledRenderer implements IItemRenderer { private static RenderItem renderItem = new RenderItem(); private static ResourceLocation lockTexture = new ResourceLocation("dimensionguard","textures/items/lock.png"); private static Minecraft mc = Minecraft.getMinecraft(); private static RenderBlocks renderBlocksIr = new RenderBlocks(); @Override public boolean handleRenderType (ItemStack item, ItemRenderType type) { switch (type) { case ENTITY: case EQUIPPED: case EQUIPPED_FIRST_PERSON: case INVENTORY: return true; default: case FIRST_PERSON_MAP: return false; } } @Override public boolean shouldUseRenderHelper(ItemRenderType type, ItemStack item, ItemRendererHelper helper) { return true; } @Override public void renderItem (ItemRenderType type, ItemStack itemStack, Object... data) { ItemStack thisStack = ItemStack.loadItemStackFromNBT((NBTTagCompound) itemStack.stackTagCompound.getTag("ItemStack")); boolean isInventory = type == ItemRenderType.INVENTORY; if (data.length > 1) { } Tessellator tess = Tessellator.instance; TextureManager texturemanager = mc.getTextureManager(); GL11.glPushMatrix(); Item item = thisStack.getItem(); Block block = Block.getBlockFromItem(item); if (thisStack != null && block != null && block.getRenderBlockPass() != 0){ GL11.glEnable(GL11.GL_BLEND); GL11.glEnable(GL11.GL_CULL_FACE); OpenGlHelper.glBlendFunc(770, 771, 1, 0); } IItemRenderer storedRenderer = MinecraftForgeClient.getItemRenderer(thisStack, type); //======Handles Special Blocks and Items====== if (storedRenderer!=null){ storedRenderer.renderItem(type, thisStack, data); } else{ if (thisStack.getItemSpriteNumber() == 0 && item instanceof ItemBlock && RenderBlocks.renderItemIn3d(block.getRenderType())) { //=====Handles regular blocks====== texturemanager.bindTexture(texturemanager.getResourceLocation(0)); switch (type){ case EQUIPPED_FIRST_PERSON: case EQUIPPED: GL11.glTranslatef(0.5F, 0.5F, 0.5F); break; case ENTITY: default: } if (thisStack != null && block != null && block.getRenderBlockPass() != 0) { GL11.glDepthMask(false); renderBlocksIr.renderBlockAsItem(block, thisStack.getItemDamage(), 1.0F); GL11.glDepthMask(true); } else { renderBlocksIr.renderBlockAsItem(block, thisStack.getItemDamage(), 1.0F); } } else{ //=======Handles Regular Items====== IIcon icon = thisStack.getIconIndex(); float xMax; float yMin; float xMin; float yMax; float depth = 1f / 16f; float width; float height; float xDiff; float yDiff; float xSub; float ySub; xMin = icon.getMinU(); xMax = icon.getMaxU(); yMin = icon.getMinV(); yMax = icon.getMaxV(); width = icon.getIconWidth(); height = icon.getIconHeight(); xDiff = xMin - xMax; yDiff = yMin - yMax; xSub = 0.5f * (xMax - xMin) / width; ySub = 0.5f * (yMax - yMin) / height; if (isInventory) { //=======Render Icon in inventory======== Doesn't Work renderItem.renderIcon(0, 0, icon, 16, 16); } else { GL11.glEnable(GL12.GL_RESCALE_NORMAL); switch (type) { case EQUIPPED_FIRST_PERSON: //TODO: get items to render in the right place case EQUIPPED: GL11.glRotated(90F, 0F, 1F, 0F); GL11.glTranslatef(-0.8F, 0.7F, -0.8F); //GL11.glTranslatef(0, -4 / 16f, 0); break; case ENTITY: GL11.glTranslatef(-0.5f, 0f, depth); // correction of the rotation point when items lie on the ground default: } //=====Front and back===== tess.startDrawingQuads(); tess.setNormal(0, 0, 1); tess.addVertexWithUV(0, 0, 0, xMax, yMax); tess.addVertexWithUV(1, 0, 0, xMin, yMax); tess.addVertexWithUV(1, 1, 0, xMin, yMin); tess.addVertexWithUV(0, 1, 0, xMax, yMin); tess.draw(); tess.startDrawingQuads(); tess.setNormal(0, 0, -1); tess.addVertexWithUV(0, 1, -depth, xMax, yMin); tess.addVertexWithUV(1, 1, -depth, xMin, yMin); tess.addVertexWithUV(1, 0, -depth, xMin, yMax); tess.addVertexWithUV(0, 0, -depth, xMax, yMax); tess.draw(); // =========Sides============ tess.startDrawingQuads(); tess.setNormal(-1, 0, 0); float pos; float iconPos; float w = width, m = xMax, d = xDiff, s = xSub; for (int k = 0, e = (int) w; k < e; ++k) { pos = k / w; iconPos = m + d * pos - s; tess.addVertexWithUV(pos, 0, -depth, iconPos, yMax); tess.addVertexWithUV(pos, 0, 0, iconPos, yMax); tess.addVertexWithUV(pos, 1, 0, iconPos, yMin); tess.addVertexWithUV(pos, 1, -depth, iconPos, yMin); } tess.draw(); tess.startDrawingQuads(); tess.setNormal(1, 0, 0); float posEnd; w = width; m = xMax; d = xDiff; s = xSub; float d2 = 1f / w; for (int k = 0, e = (int) w; k < e; ++k) { pos = k / w; iconPos = m + d * pos - s; posEnd = pos + d2; tess.addVertexWithUV(posEnd, 1, -depth, iconPos, yMin); tess.addVertexWithUV(posEnd, 1, 0, iconPos, yMin); tess.addVertexWithUV(posEnd, 0, 0, iconPos, yMax); tess.addVertexWithUV(posEnd, 0, -depth, iconPos, yMax); } tess.draw(); tess.startDrawingQuads(); tess.setNormal(0, 1, 0); float h = height; m = yMax; d = yDiff; s = ySub; d2 = 1f / h; for (int k = 0, e = (int) h; k < e; ++k) { pos = k / h; iconPos = m + d * pos - s; posEnd = pos + d2; tess.addVertexWithUV(0, posEnd, 0, xMax, iconPos); tess.addVertexWithUV(1, posEnd, 0, xMin, iconPos); tess.addVertexWithUV(1, posEnd, -depth, xMin, iconPos); tess.addVertexWithUV(0, posEnd, -depth, xMax, iconPos); } tess.draw(); tess.startDrawingQuads(); tess.setNormal(0, -1, 0); h = height; m = yMax; d = yDiff; s = ySub; for (int k = 0, e = (int) h; k < e; ++k) { pos = k / h; iconPos = m + d * pos - s; tess.addVertexWithUV(1, pos, 0, xMin, iconPos); tess.addVertexWithUV(0, pos, 0, xMax, iconPos); tess.addVertexWithUV(0, pos, -depth, xMax, iconPos); tess.addVertexWithUV(1, pos, -depth, xMin, iconPos); } tess.draw(); GL11.glDisable(GL12.GL_RESCALE_NORMAL); } } } if (thisStack != null && block != null && block.getRenderBlockPass() != 0) { GL11.glDisable(GL11.GL_BLEND); } //TODO: Overlay lock in inventory GL11.glPopMatrix(); } }
-
I'm going to begin this right at the start because I think that'd be easier to understand overall. I'm creating a method of disabling Items in certain dimensions - to facilitate the creation of magic/tech only dimensions, prevent people quarrying in the overworld, stuff like that. The method I'm currently using detects if a disabled item is in the player's inventory and disables it from being used by removing it, then creating a new ItemStack of DisabledItem with the original ItemStack stored as an NBT tag. This Item has no interesting properties and so can't be placed in the world as a block, worn as armour, used as a weapon/tool etc. What I would like to do however is to get the "stored" item to render normally, except in the inventory where I want to overlay a small padlock icon. Here is where I am having trouble, I cannot get blocks or items with custom or multi-pass renders to display properly. If anyone could give some advice on how to approach this I'd be very appreciative.
-
Thank you very much, I can't believe I didn't think of doing that - Solved it
-
Currently having a problem with a custom biome - It is supposed to not have any weather at all, enableRain and enableSnow are both set to false, rainfall is set to 0. However I'm still having snow appearing on the top level of the ground without any weather having occurred. Is there any way to prevent this happening while keeping a cold temperature, as I need to have water freezing as a mechanic in this biome. Any advice would be appreciated
-
[1.7.10][Forge-new] Block/Item Override Troubles
hilburn replied to zombiepig333's topic in Modder Support
I've tried this method (1210-new) and it does work, however it does not seem to update crafting recipes, in my case for BlockChest my alternative chest is not usable in a hopper recipe and the vanilla chest recipe produces an invalid recipe. Is this an issue that can be resolved to updating to latest or intended behaviour? -
It's definitely possible, as many mods (forestry, tinker's construct, railcraft, witchery to name a few) all add structures to villages. Now I'm no expert on the matter, but I had a quick poke around the TiC github and found the following: Village Structure Handler: https://github.com/SlimeKnights/TinkersConstruct/blob/master/src/main/java/tconstruct/world/village/VillageSmelteryHandler.java Register Handler: https://github.com/SlimeKnights/TinkersConstruct/blob/master/src/main/java/tconstruct/TConstruct.java#L150 Pretty sure you can figure what's going on from these two together
-
I'm working on something for a friend which involves a number of vanilla tweaks. Most of these are quite simple and just involve editing drops and whatnot but this one has got me stumped. Changing the inventory size of vanilla chests to 9 - Making a block to do this is pretty easy, extend TileEntityChest, change inventory size to 9, extend BlockChest, point it at the new Tile Entity. However this is the first time I've tried overriding a vanilla class since 1.6.4 when you could just set the ID the same and getting this SmallChest to register as "minecraft:chest" in 1.7.10 is giving me trouble. The slight wrinkle is that I want the chest to behave completely normally for the purposes of crafting etc, so I am wary about editing every recipe that contains a chest to instead contain a small chest so I think overriding the vanilla chest is the best option (but please feel free to correct me) Possible solutions I've come up with so far, slight escalation in complexity: [*]When the player tries to place a Chest, instead places a SmallChest, SmallChest drops a vanilla chest when broken [*]ASM to allow editing of Blocks While the first option works, it's not particularly neat and could probably be bypassed by getting some sort of block placer to place a chest so I don't particularly want to do it, the second is, well... icky. Would really appreciate your thoughts on the topic, any possible solutions to this issue that you can offer would be great. P.S. methods that don't work: public static Block registerBlock(Block block, Class<? extends ItemBlock> itemclass, String name, String modId, Object... itemCtorArgs) - deprecated public static void addAlias(String alias, String forName, GameRegistry.Type type) - not yet implemented