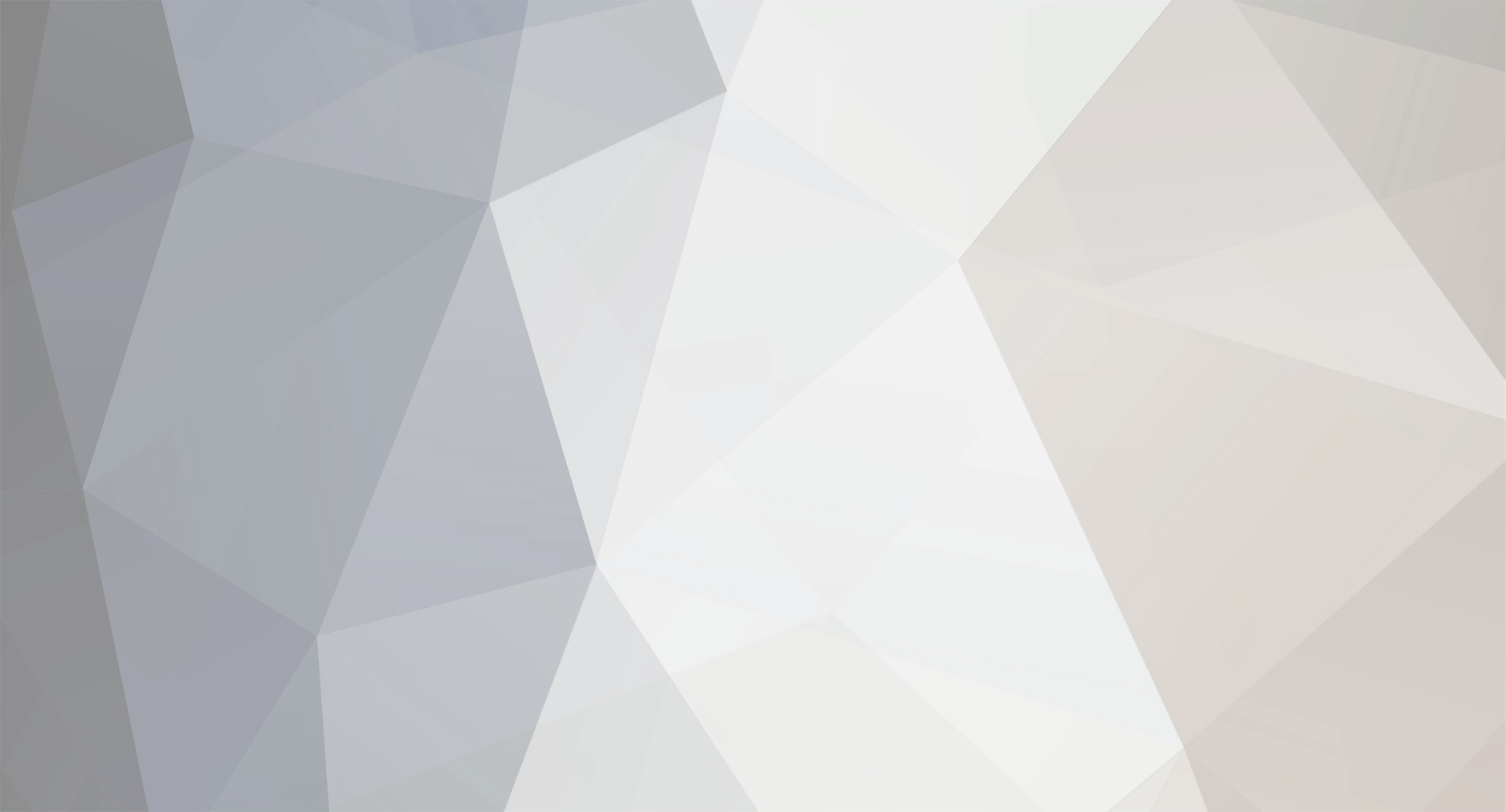
noahc3
Forge Modder-
Posts
26 -
Joined
-
Last visited
Converted
-
Gender
Male
-
Location
MB, Canada
Recent Profile Visitors
The recent visitors block is disabled and is not being shown to other users.
noahc3's Achievements

Tree Puncher (2/8)
0
Reputation
-
[1.16.4] [Solved] Removing specific features from a biome
noahc3 replied to noahc3's topic in Modder Support
Thanks, that worked. I had to dig through the features recursively since they weren't always one level deep: @SubscribeEvent(priority = EventPriority.LOWEST) public static void BiomeLoadingIntercept(final BiomeLoadingEvent event) { BiomeGenerationSettingsBuilder gen = event.getGeneration(); for(Supplier<ConfiguredFeature<?, ?>> f : gen.getFeatures(GenerationStage.Decoration.UNDERGROUND_ORES)) { ConfiguredFeature<?, ?> resolved = resolve(f.get()); if (resolved.feature instanceof OreFeature) { OreFeatureConfig oreConfig = (OreFeatureConfig) resolved.config; Logger.info(oreConfig.state.getBlock().getRegistryName()); ... compare blockstates ... } } } private static ConfiguredFeature<?, ?> resolve(ConfiguredFeature<?, ?> f) { if (f.getFeature() instanceof DecoratedFeature) { ConfiguredFeature<?, ?> subFeature = ((DecoratedFeatureConfig)f.getConfig()).feature.get(); return resolve(subFeature); } else { return f; } } This seems inefficient, is this the simplest way? -
The end goal is to disable certain aspects of vanilla ore/feature generation so I can replace them with my own features with custom values. To disable vanilla ore generation, I've created an event handler for BiomeLoadingEvent. This allows me to get the BiomeGenerationSettingsBuilder which allows me to get the list of ConfiguredFeature's for the biome associated with the event call: @SubscribeEvent(priority = EventPriority.LOWEST) public static void BiomeLoadingIntercept(final BiomeLoadingEvent event) { BiomeGenerationSettingsBuilder gen = event.getGeneration(); List<Supplier<ConfiguredFeature<?, ?>>> features = gen.getFeatures(GenerationStage.Decoration.UNDERGROUND_ORES); ... } I know this list is *probably* what I need, since if I just call features.clear(), all ore generation is successfully removed from the game. However, I want to be able to remove specific ores via config file, so I need some way to either remove by registry ID, or remove by object (ex. using the objects in net.minecraft.world.gen.feature.Features). I've tried a number of things that don't seem to work: Looping through the features list, feature.get().getFeature().getRegistryName() always returns minecraft:decorated and never anything more specific. Looping through the features list, calling ForgeRegistries.getKey(feature) always returns minecraft:decorated. Looping through the features list, calling net.minecraft.util.registry.WorldGenRegistries.CONFIGURED_FEATURE.getKey(feature) always returns NULL (presumably because vanilla registries aren't used by Forge, or something). Calling features.contains(Features.ORE_COAL) (for example) is always false, like the biomes never contain the coal ore feature (but they do). I think the way features are applied and stacked causes the features to lose their original registry values. Is there any way I can remove specific features from biomes? Thanks
-
I used to track the items life by using a tick counter, however I was forced to change this due to the fact that anytime NBT is updated, the item (if held) performs the re-equip animation, which looks very strange even with longer tick delays. In 1.8 this could be dealt with by enabling some flag that disables the re-equip animation, but this has a different effect in 1.10+ in which when you swap items, it actually doesn't change what item is rendered in hand (this might be a Forge bug). Ticks existed also won't work since the item can be toggled on and off, therefore it could realistically be "alive" for an indefinite amount of time. I'll probably go ahead with what you initially suggested and just have a single static helper class that stores various NBT and player manipulation methods. Thanks!
-
Hello! I have some items which have a timer that compares current system time and a stored system time while activated to determine when the item is "depleted" and should be removed from the players inventory. The problem is, if a player logs out with the item in an active state (determined by a NBT boolean value) and logs back in long enough after that the item would be considered old enough, the item depletes itself. To combat this, I figure I should detect if the player the item is held by is logging off, and when that happens, change necessary NBT values so that the item is in the disabled state by the time the player logs back in. I figure I should be using the PlayerEvent.OnPlayerLoggedOut event to do this, but I'm not sure how to implement the event so I can call an item's method. Any help is appreciated! EDIT: I basically have this working with the following in an event handler class: public class ASEventHandler { @SubscribeEvent public void PlayerLoggedOutEvent(PlayerEvent.PlayerLoggedOutEvent event) { EntityPlayer player = event.player; InventoryPlayer inv = event.player.inventory; ArrayList<ItemStack> items = new ArrayList<>(); for(int i=0; i < inv.getSizeInventory(); i++) { if (!(inv.getStackInSlot(i) == AbilityStones.blankItemStack)) { if(ModItems.isStackAbilityStone(inv.getStackInSlot(i))){ if (inv.getStackInSlot(i).getTagCompound().getInteger("Enabled") == 1) { disableRegularStone(inv.getStackInSlot(i)); } } else if (inv.getStackInSlot(i).getItem() == ModItems.advancedAbilityStone) { disableAdvancedStone(player, inv.getStackInSlot(i)); } } } } private void disableRegularStone(ItemStack stack) { --- change regular nbt to off state --- } private void disableAdvancedStone(EntityPlayer playerIn, ItemStack stack) { --- change advanced nbt to off state --- } private void cleanupEffects(ItemStack stack, Entity entity) { --- extra cleanup the advanced stone might need to perform --- } } where the code under disableRegularStone, disableAdvancedStone and cleanupEffects are near duplicates of that in their respective item classes. While it does work, I would appreciate it if anyone could let me know of a way to keep the methods in the item classes themselves, rather than duplicate the code into a static event handler.
-
Minecraft does not like me using getItem on the itemstack returned from the slot check ( this.getStackInSlot(0).getItem() ), crashes and throws a NullPointerException, even though I'm making sure to check that it isn't null before executing any code using it. Crash report: http://paste.ee/p/CnMhU Tile Entity Class where the error is thrown: http://paste.ee/p/tyuNv (getItem checks around line 204) Help would be appreciated, and sorry for any hassle. EDIT: Whoops! Needed to make sure the ItemStack itself wasn't null before setting a variable to Itemstack.getItem()
-
Simple (hopefully) question. How can I compare the itemstack in a slot with an itemstack that I am checking for. Eg. I am trying to check if slot index 0 has a diamond. I've tried just comparing the itemstack in the slot with new ItemStack(items.whatever) however that does not work presumably because certain data such as how long the item has existed for is different from a newly generated itemstack. Any help would be appreciated!
-
Hey, I have an item which has 2 timers stored in NBT, a cooldown and a time remaining counter. For some reason, if the NBT is being modified, the item does some crazy jumping thing if you hold it in your hand (Like when you right click an item on the ground, except constant). I don't remember this being an issue in 1.7. Here is my code: http://pastebin.com/C9ZrfKVY Any help would be much appreciated!
-
package noahc3.AbilityStones; import net.minecraft.creativetab.CreativeTabs; import net.minecraft.item.Item; import net.minecraft.item.ItemStack; import net.minecraftforge.common.MinecraftForge; import cpw.mods.fml.common.Mod; import cpw.mods.fml.common.Mod.EventHandler; import cpw.mods.fml.common.event.FMLInitializationEvent; import cpw.mods.fml.common.event.FMLPostInitializationEvent; import cpw.mods.fml.common.event.FMLPreInitializationEvent; import cpw.mods.fml.common.registry.GameRegistry; @Mod(modid = "abilstones", name = "Ability Stones", version = "1.0") public class AbilityStones { public static Item itemRegenerationStone; //public static Item itemFireResistStone; //public static Item itemSwiftnessStone; //public static Item itemNightVisionStone; @EventHandler public void preInit(FMLPreInitializationEvent event) { //Item/Block init and registration //Config Handling //blocks //items itemRegenerationStone = new ItemRegenerationStone().setCreativeTab(tabAbilityStones); GameRegistry.registerItem(itemRegenerationStone, itemRegenerationStone.getUnlocalizedName().substring(5)); //itemFireResistStone = new ItemFireResistStone().setUnlocalizedName("ItemFireResistStone").setTextureName("abilstones:itemFireResistStone").setMaxDamage(24000).setCreativeTab(tabAbilityStones); //GameRegistry.registerItem(itemFireResistStone, itemFireResistStone.getUnlocalizedName().substring(5)); //itemSwiftnessStone = new ItemSwiftnessStone().setUnlocalizedName("ItemSwiftnessStone").setTextureName("abilstones:itemSwiftnessStone").setMaxDamage(24000).setCreativeTab(tabAbilityStones); //GameRegistry.registerItem(itemSwiftnessStone, itemSwiftnessStone.getUnlocalizedName().substring(5)); //itemNightVisionStone = new ItemNightVisionStone().setUnlocalizedName("ItemNightVisionStone").setTextureName("abilstones:itemNightVisionStone").setMaxDamage(24000).setCreativeTab(tabAbilityStones); //GameRegistry.registerItem(itemNightVisionStone, itemNightVisionStone.getUnlocalizedName().substring(5)); //foods //tools //armor //furnace recipes } @EventHandler public void init(FMLInitializationEvent event) { //Proxy, Tileentity, entity, crafting recipes, GUI and Packet Registration } @EventHandler public void postInit(FMLPostInitializationEvent event) { } public static CreativeTabs tabAbilityStones = new CreativeTabs("tabAbilityStones"){ @Override public Item getTabIconItem(){ return new ItemStack(itemRegenerationStone).getItem(); } }; }
-
Hey, for some reason I have an item that while in the creative menu or in NEI, it's name is Unnamed. When I put it into my inventory it fixes itself. I suspect this may be due to me using NBT but I'm not sure. Here is my Item Class: package noahc3.AbilityStones; import java.util.List; import net.minecraft.client.Minecraft; import net.minecraft.entity.Entity; import net.minecraft.entity.EntityLivingBase; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.item.Item; import net.minecraft.item.ItemStack; import net.minecraft.nbt.NBTTagCompound; import net.minecraft.potion.Potion; import net.minecraft.potion.PotionEffect; import net.minecraft.world.World; public class ItemRegenerationStone extends Item { //use timer in ticks (24000) public ItemRegenerationStone() { setMaxStackSize(1); setMaxDamage(24000); setNoRepair(); setUnlocalizedName("ItemRegenerationStone"); setTextureName("abilstones:itemRegenerationStone"); } public void addInformation(ItemStack par1ItemStack, EntityPlayer par2EntityPlayer, List par3List, boolean par4) { par3List.add("Minutes Remaining: " + (((par1ItemStack.getTagCompound().getInteger("timer"))/20)/60+1)); } public ItemStack onItemRightClick(ItemStack itemstack, World par2World, EntityPlayer par3EntityPlayer) { if(itemstack.stackTagCompound.getInteger("enabled") == 1 && itemstack.stackTagCompound.getInteger("cooldown") == 0) { itemstack.stackTagCompound.setInteger("enabled", 0); itemstack.stackTagCompound.setInteger("cooldown", 20); System.out.println("Was Enabled, now Disabled"); return itemstack; } else if(itemstack.stackTagCompound.getInteger("enabled") == 0 && itemstack.stackTagCompound.getInteger("cooldown") == 0) { itemstack.stackTagCompound.setInteger("enabled", 1); itemstack.stackTagCompound.setInteger("cooldown", 20); System.out.println("Was Disabled, now Enabled"); return itemstack; } else { System.out.println("Warning! For some reason the " + this + " is not enabled nor disabled. Please report this to the mod author!"); return itemstack; } } EntityPlayer player = Minecraft.getMinecraft().thePlayer; @Override public void onUpdate(ItemStack itemstack, World world, Entity entity, int i, boolean flag) { //stuff to do when active if(itemstack.stackTagCompound == null){ itemstack.stackTagCompound = new NBTTagCompound(); itemstack.stackTagCompound.setInteger("timer", 23999); itemstack.stackTagCompound.setInteger("enabled", 0); itemstack.stackTagCompound.setInteger("cooldown", 0); } EntityPlayer player = (EntityPlayer)entity; if(itemstack.stackTagCompound.getInteger("enabled") == 1) { itemstack.stackTagCompound.setInteger("timer", itemstack.getTagCompound().getInteger("timer") + -1); ((EntityLivingBase) entity).addPotionEffect(new PotionEffect(Potion.regeneration.id, 20, 1)); } if(itemstack.stackTagCompound.getInteger("timer") <= 0){player.inventory.consumeInventoryItem(AbilityStones.itemRegenerationStone);} if(itemstack.stackTagCompound.getInteger("cooldown") > 0) { itemstack.stackTagCompound.setInteger("cooldown", itemstack.getTagCompound().getInteger("cooldown") + -1); System.out.println("minus one tick"); } } } Thanks for the help.
-
Hey, I'm currently having an issue where if you hold right click for longer than maybe half a second, the if statement I use to detect right clicks is constantly activating. Here is my if statement: public ItemStack onItemRightClick(ItemStack itemstack, World par2World, EntityPlayer par3EntityPlayer) { if(itemstack.stackTagCompound.getInteger("enabled") == 1) { itemstack.stackTagCompound.setInteger("enabled", 0); System.out.println("Was Enabled, now Disabled"); return itemstack; } else { itemstack.stackTagCompound.setInteger("enabled", 1); System.out.println("Was Disabled, now Enabled"); return itemstack; } } I was wondering if there was something similar to onItemRightClick but instead detects the opposite, when I'm not right clicking with the item (Which I would then make a variable that utilizes it).
-
[Solved | 1.7.10] How to detect Right Clicks with items?
noahc3 replied to noahc3's topic in Modder Support
@AwesomeSpider Thanks, switching it to return itemstack fixed it. Yea, it was a nullpointer exception. @coolAlias I have created the NBT tag further in the code with this: if(itemstack.stackTagCompound == null){ itemstack.stackTagCompound = new NBTTagCompound(); itemstack.stackTagCompound.setInteger("timer", 24000); itemstack.stackTagCompound.setInteger("enabled", 0); } Thanks for the help. That's inside onUpdate. -
Hey, I'm having some issues with detecting right clicks with an item. Here is the code I'm using: public ItemStack onItemRightClick(ItemStack itemstack, World par2World, EntityPlayer par3EntityPlayer) { if(itemstack.stackTagCompound.getInteger("enabled") == 1) { itemstack.stackTagCompound.setInteger("enabled", 0); System.out.println("Was Enabled, now Disabled"); return null; } else { itemstack.stackTagCompound.setInteger("enabled", 1); System.out.println("Was Disabled, now Enabled"); return null; } } Minecraft successfully loads, but right clicking the item crashes the game. (But it does detect the right click as sysout prints to console) Any help would be appreciated.
-
[Solved | 1.7.10] How to make item lose durability/take damage?
noahc3 replied to noahc3's topic in Modder Support
I figured out how to do it, but yea, I decided to move the timer to NBT to stop the weird jumpy stuff it does when you hold it in your hand. Thanks anyways.