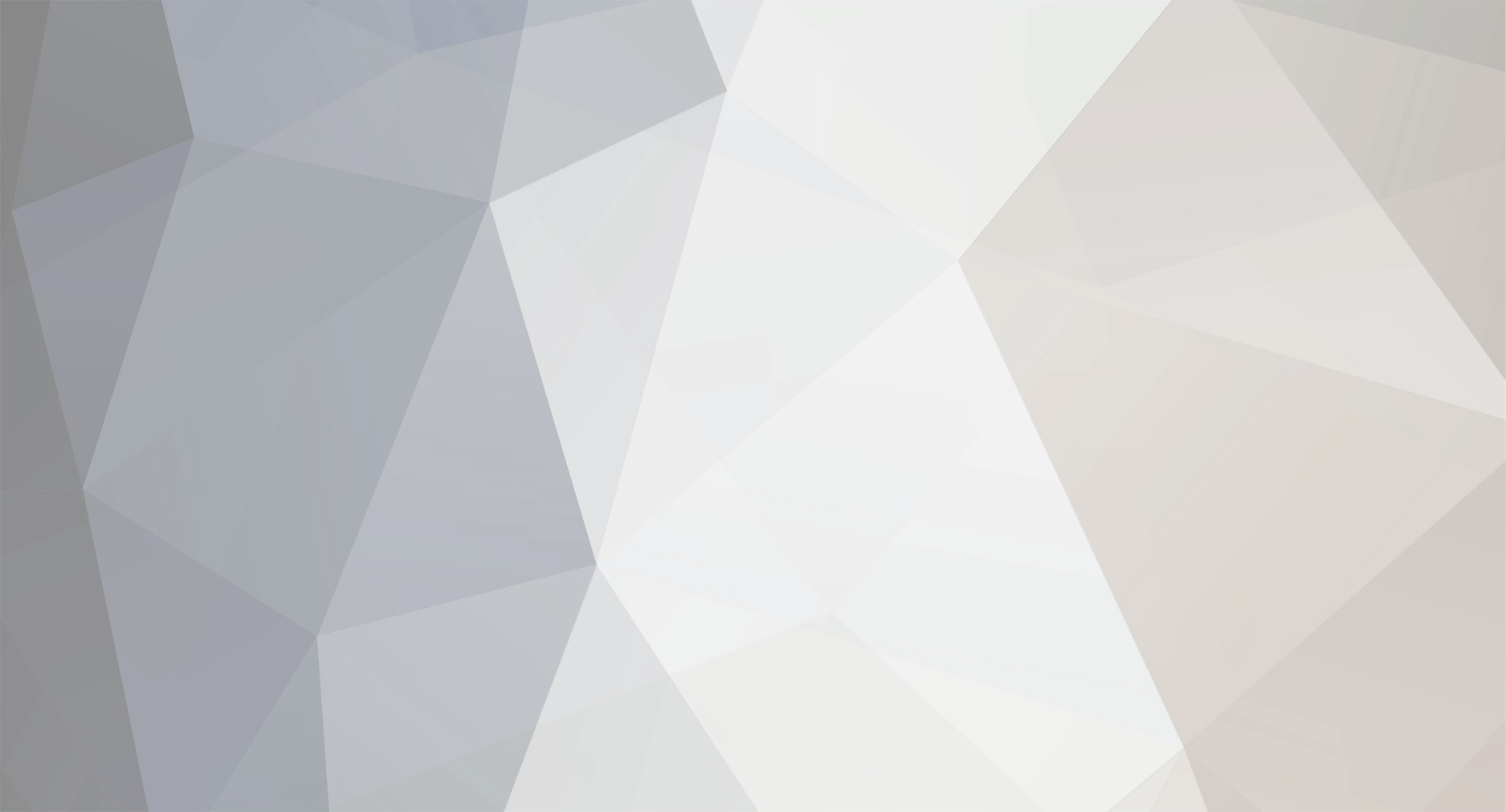
DonkeyCore
Members-
Posts
8 -
Joined
-
Last visited
Converted
-
Gender
Male
-
URL
http://www.donkeyteeth2013.comule.com/mods/
-
Location
USA
-
Personal Text
Hi
DonkeyCore's Achievements

Tree Puncher (2/8)
0
Reputation
-
That's okay. Your help was wonderful nonetheless. In other words, I'm slightly closer to figuring out the problem. In this class: package donkeycore.fusion.blocks; import net.minecraft.block.Block; import net.minecraft.block.material.Material; import net.minecraft.block.state.IBlockState; import net.minecraft.client.Minecraft; import net.minecraft.client.resources.model.ModelResourceLocation; import net.minecraft.entity.EntityLivingBase; import net.minecraft.init.Blocks; import net.minecraft.init.Items; import net.minecraft.item.ItemStack; import net.minecraft.util.BlockPos; import net.minecraft.util.EnumFacing; import net.minecraft.world.Explosion; import net.minecraft.world.World; import net.minecraftforge.fml.common.registry.GameRegistry; import net.minecraftforge.fml.relauncher.Side; import donkeycore.fusion.Fusion; import donkeycore.fusion.IFusionObject; public class FusionBomb extends Block implements IFusionObject { public static final String name = "fusion_bomb"; public FusionBomb() { super(Material.tnt); setHarvestLevel(null, 0); setStepSound(Block.soundTypeMetal); setHardness(2F); setLightLevel(1F); setCreativeTab(Fusion.tabFusion); setUnlocalizedName(name); } @Override public void onBlockDestroyedByExplosion(World w, BlockPos p, Explosion e) { explode(w, p); } @Override public IBlockState onBlockPlaced(World w, BlockPos p, EnumFacing f, float hitx, float hity, float hitz, int meta, EntityLivingBase e) { Fusion.info(w.getClass().getSimpleName()); if (w.isBlockIndirectlyGettingPowered(p) > 0) { Fusion.info("Passing: " + w.getClass().getSimpleName()); explode(w, p); w.notifyNeighborsOfStateChange(p, this); return Blocks.air.getDefaultState(); } return super.onBlockPlaced(w, p, f, hitx, hity, hitz, meta, e); } @Override public void onNeighborBlockChange(World w, BlockPos p, IBlockState s, Block b) { Fusion.info(w.getClass().getSimpleName()); if (w.isBlockIndirectlyGettingPowered(p) > 0) { Fusion.info("Passing: " + w.getClass().getSimpleName()); explode(w, p); w.notifyNeighborsOfStateChange(p, this); } } public static void explode(World w, BlockPos p) { Fusion.createFusionExplosion(w, p.getX(), p.getY(), p.getZ(), 6F, true, true, true); } @Override public void preinit() { GameRegistry.registerBlock(this, name); GameRegistry.addShapedRecipe(new ItemStack(this), "RAR", "FTF", "RAR", 'F', Fusion.getItemFromId("fusion_ingot"), 'A', Fusion.getItemFromId("active_fusion_ingot"), 'T', Blocks.tnt, 'R', Items.redstone); GameRegistry.addShapedRecipe(new ItemStack(this), "RFR", "ATA", "RFR", 'F', Fusion.getItemFromId("fusion_ingot"), 'A', Fusion.getItemFromId("active_fusion_ingot"), 'T', Blocks.tnt, 'R', Items.redstone); } @Override public void init() { if (Fusion.SIDE == Side.CLIENT) Minecraft.getMinecraft().getRenderItem().getItemModelMesher().register(GameRegistry.findItem(Fusion.MODID, name), 0, new ModelResourceLocation(Fusion.MODID + ":" + name, "inventory")); } } You can see I have a couple methods that call explode(). I have 2 of them set to tell me what world was being passed in. In onBlockPlaced, I noticed that it says: [09:18:24] [Client thread/INFO] [FML]: [Fusion] WorldClient [09:18:24] [Client thread/INFO] [FML]: [Fusion] Passing: WorldClient [09:18:24] [server thread/INFO] [FML]: [Fusion] WorldServer [09:18:24] [server thread/INFO] [FML]: [Fusion] Passing: WorldServer If I place a redstone torch THEN my custom block, it works perfectly and the explosion particles are clearly visible! However, you can see onNeighborBlockChange which displays: [09:18:59] [Client thread/INFO] [FML]: [Fusion] WorldClient [09:18:59] [server thread/INFO] [FML]: [Fusion] WorldServer [09:19:00] [server thread/INFO] [FML]: [Fusion] WorldServer [09:19:00] [server thread/INFO] [FML]: [Fusion] Passing: WorldServer [09:19:00] [server thread/INFO] [FML]: [Fusion] WorldServer [09:19:00] [server thread/INFO] [FML]: [Fusion] Passing: WorldServer [09:19:00] [server thread/INFO] [FML]: [Fusion] WorldServer [09:19:00] [server thread/INFO] [FML]: [Fusion] WorldServer Which clearly does not seem to work properly. Only WorldServer is getting passed in for some reason, but the calls that the method makes is nearly identical to that of onBlockPlaced... hmm.....
-
Yep, it is definitely being called both times. Still no particles though unfortunately.
-
Thanks for replying! I removed the !w.isRemote check, and I can hear the sound, but I still don't see any explosion particles.
-
Sorry for posting again so soon, but I have another problem. I have a bomb block that causes an explosion based on redstone signals / time settings. It works fine, but the explosion particles (EXPLOSION_LARGE / EXPLOSION_HUGE) do not appear. I have a subclass of explosion that I'm using, but it works virtually the same way. Here is the method used to summon the explosion: public static final FusionExplosion createFusionExplosion(World w, double x, double y, double z, float size, boolean fire, boolean smoke, boolean strong) { FusionExplosion explosion = new FusionExplosion(w, null, x, y, z, size, fire, smoke, strong); if (ForgeEventFactory.onExplosionStart(w, explosion)) return explosion; explosion.doExplosionA(); explosion.doExplosionB(true); return explosion; } Here is FusionExplosion.class: package donkeycore.fusion; import java.util.HashSet; import java.util.Iterator; import java.util.List; import java.util.Map; import java.util.Random; import net.minecraft.block.Block; import net.minecraft.block.material.Material; import net.minecraft.block.state.IBlockState; import net.minecraft.enchantment.EnchantmentProtection; import net.minecraft.entity.Entity; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.util.AxisAlignedBB; import net.minecraft.util.BlockPos; import net.minecraft.util.EnumParticleTypes; import net.minecraft.util.MathHelper; import net.minecraft.util.Vec3; import net.minecraft.world.Explosion; import net.minecraft.world.World; import net.minecraftforge.event.ForgeEventFactory; import com.google.common.collect.Maps; import com.google.common.collect.Sets; public class FusionExplosion extends Explosion { private final boolean f; private final boolean s; private final Random r; private final World w; private final double x; private final double y; private final double z; private final Entity e; private final float size; private final List<Object> blocks; private final Map<Object, Object> map; private final boolean strong; public FusionExplosion(World w, Entity e, double x, double y, double z, float size, List<Object> l) { this(w, e, x, y, z, size, false, true, l); } public FusionExplosion(World w, Entity e, double x, double y, double z, float size, boolean f, boolean s, List<Object> l) { this(w, e, x, y, z, size, f, s, false); blocks.addAll(l); } public FusionExplosion(World w, Entity e, double x, double y, double z, float size, boolean f, boolean s, boolean strong) { super(w, e, x, y, z, size, f, s); this.r = new Random(); this.blocks = com.google.common.collect.Lists.newArrayList(); this.map = Maps.newHashMap(); this.w = w; this.e = e; this.size = size; this.x = x; this.y = y; this.z = z; this.f = f; this.s = s; this.strong = strong; } @SuppressWarnings({"unchecked", "rawtypes"}) public void doExplosionA() { HashSet<Object> hashset = Sets.newHashSet(); for(int i = 0; i < 16; i++) { for(int j = 0; j < 16; j++) { for(int k = 0; k < 16; k++) { if ((i == 0) || (i == 15) || (j == 0) || (j == 15) || (k == 0) || (k == 15)) { double d0 = i / 15.0F * 2.0F - 1.0F; double d1 = j / 15.0F * 2.0F - 1.0F; double d2 = k / 15.0F * 2.0F - 1.0F; double d3 = Math.sqrt(d0 * d0 + d1 * d1 + d2 * d2); d0 /= d3; d1 /= d3; d2 /= d3; float f = this.size * (0.7F + this.w.rand.nextFloat() * 0.6F); double d4 = this.x; double d6 = this.y; double d8 = this.z; for(; f > 0.0F; f -= 0.22500001F) { BlockPos blockpos = new BlockPos(d4, d6, d8); IBlockState iblockstate = this.w.getBlockState(blockpos); if (iblockstate.getBlock().getMaterial() != Material.air) { float f2 = this.e != null ? this.e.getExplosionResistance(this, this.w, blockpos, iblockstate) : iblockstate.getBlock().getExplosionResistance(this.w, blockpos, (Entity) null, this); f -= (f2 + 0.3F) * 0.3F; } if ((f > 0.0F) && ((this.e == null) || (this.e.func_174816_a(this, this.w, blockpos, iblockstate, f)))) { hashset.add(blockpos); } d4 += d0 * 0.30000001192092896D; d6 += d1 * 0.30000001192092896D; d8 += d2 * 0.30000001192092896D; } } } } } this.blocks.addAll(hashset); float f3 = this.size * 2.0F; int j = MathHelper.floor_double(this.x - f3 - 1.0D); int k = MathHelper.floor_double(this.x + f3 + 1.0D); int j1 = MathHelper.floor_double(this.y - f3 - 1.0D); int l = MathHelper.floor_double(this.y + f3 + 1.0D); int k1 = MathHelper.floor_double(this.z - f3 - 1.0D); int i1 = MathHelper.floor_double(this.z + f3 + 1.0D); List list = this.w.getEntitiesWithinAABBExcludingEntity(this.e, new AxisAlignedBB(j, j1, k1, k, l, i1)); ForgeEventFactory.onExplosionDetonate(this.w, this, list, f3); Vec3 vec3 = new Vec3(this.x, this.y, this.z); for(int l1 = 0; l1 < list.size(); l1++) { Entity entity = (Entity) list.get(l1); if (!entity.func_180427_aV()) { double d12 = entity.getDistance(this.x, this.y, this.z) / f3; if (d12 <= 1.0D) { double d5 = entity.posX - this.x; double d7 = entity.posY + entity.getEyeHeight() - this.y; double d9 = entity.posZ - this.z; double d13 = MathHelper.sqrt_double(d5 * d5 + d7 * d7 + d9 * d9); if (d13 != 0.0D) { d5 /= d13; d7 /= d13; d9 /= d13; double d14 = this.w.getBlockDensity(vec3, entity.getEntityBoundingBox()); double d10 = (1.0D - d12) * d14; entity.attackEntityFrom((strong ? Fusion.sourceStrongRadiation : Fusion.sourceRadiation), (int) ((d10 * d10 + d10) / 2.0D * 8.0D * f3 + 1.0D)); double d11 = EnchantmentProtection.func_92092_a(entity, d10); entity.motionX += d5 * d11; entity.motionY += d7 * d11; entity.motionZ += d9 * d11; if ((entity instanceof EntityPlayer)) { this.map.put((EntityPlayer) entity, new Vec3(d5 * d10, d7 * d10, d9 * d10)); } } } } } } public void doExplosionB(boolean b) { this.w.playSoundEffect(this.x, this.y, this.z, "random.explode", 4.0F, (1.0F + (this.w.rand.nextFloat() - this.w.rand.nextFloat()) * 0.2F) * 0.7F); if ((this.size >= 2.0F) && (this.s)) this.w.spawnParticle(EnumParticleTypes.EXPLOSION_HUGE, this.x, this.y, this.z, 1.0D, 0.0D, 0.0D, new int[0]); else this.w.spawnParticle(EnumParticleTypes.EXPLOSION_LARGE, this.x, this.y, this.z, 1.0D, 0.0D, 0.0D, new int[0]); if (this.s) { Iterator<Object> iterator = this.blocks.iterator(); while(iterator.hasNext()) { BlockPos blockpos = (BlockPos) iterator.next(); Block block = this.w.getBlockState(blockpos).getBlock(); if (b) { double d0 = blockpos.getX() + this.w.rand.nextFloat(); double d1 = blockpos.getY() + this.w.rand.nextFloat(); double d2 = blockpos.getZ() + this.w.rand.nextFloat(); double d3 = d0 - this.x; double d4 = d1 - this.y; double d5 = d2 - this.z; double d6 = MathHelper.sqrt_double(d3 * d3 + d4 * d4 + d5 * d5); d3 /= d6; d4 /= d6; d5 /= d6; double d7 = 0.5D / (d6 / this.size + 0.1D); d7 *= (this.w.rand.nextFloat() * this.w.rand.nextFloat() + 0.3F); d3 *= d7; d4 *= d7; d5 *= d7; this.w.spawnParticle(EnumParticleTypes.EXPLOSION_NORMAL, (d0 + this.x * 1.0D) / 2.0D, (d1 + this.y * 1.0D) / 2.0D, (d2 + this.z * 1.0D) / 2.0D, d3, d4, d5, new int[0]); this.w.spawnParticle(EnumParticleTypes.SMOKE_NORMAL, d0, d1, d2, d3, d4, d5, new int[0]); } if (block.getMaterial() != Material.air) { if (block.canDropFromExplosion(this)) block.dropBlockAsItemWithChance(this.w, blockpos, this.w.getBlockState(blockpos), 1.0F / this.size, 0); block.onBlockExploded(this.w, blockpos, this); } } } if (this.f) { Iterator<Object> iterator = this.blocks.iterator(); while(iterator.hasNext()) { BlockPos blockpos = (BlockPos) iterator.next(); if ((this.w.getBlockState(blockpos).getBlock().getMaterial() == Material.air) && (this.w.getBlockState(blockpos.down()).getBlock().isFullBlock()) && (this.r.nextInt(3) == 0)) this.w.setBlockState(blockpos, net.minecraft.init.Blocks.fire.getDefaultState()); } } } } And here is FusionBomb.class, which calls the first method to create the explosion: package donkeycore.fusion.blocks; import net.minecraft.block.Block; import net.minecraft.block.material.Material; import net.minecraft.block.state.IBlockState; import net.minecraft.client.Minecraft; import net.minecraft.client.resources.model.ModelResourceLocation; import net.minecraft.entity.EntityLivingBase; import net.minecraft.init.Blocks; import net.minecraft.init.Items; import net.minecraft.item.ItemStack; import net.minecraft.util.BlockPos; import net.minecraft.util.EnumFacing; import net.minecraft.world.Explosion; import net.minecraft.world.World; import net.minecraftforge.fml.common.registry.GameRegistry; import net.minecraftforge.fml.relauncher.Side; import donkeycore.fusion.Fusion; import donkeycore.fusion.IFusionObject; public class FusionBomb extends Block implements IFusionObject { public static final String name = "fusion_bomb"; public FusionBomb() { super(Material.tnt); setHarvestLevel(null, 0); setStepSound(Block.soundTypeMetal); setHardness(2F); setLightLevel(1F); setCreativeTab(Fusion.tabFusion); setUnlocalizedName(name); } @Override public void onBlockDestroyedByExplosion(World w, BlockPos p, Explosion e) { explode(w, p); } @Override public IBlockState onBlockPlaced(World w, BlockPos p, EnumFacing f, float hitx, float hity, float hitz, int meta, EntityLivingBase e) { if (w.isBlockIndirectlyGettingPowered(p) > 0) { explode(w, p); w.notifyNeighborsOfStateChange(p, this); } return super.onBlockPlaced(w, p, f, hitx, hity, hitz, meta, e); } @Override public void onNeighborBlockChange(World w, BlockPos p, IBlockState s, Block b) { if (w.isBlockIndirectlyGettingPowered(p) > 0) { explode(w, p); w.notifyNeighborsOfStateChange(p, this); } } public static void explode(World w, BlockPos p) { if(!w.isRemote) Fusion.createFusionExplosion(w, p.getX(), p.getY(), p.getZ(), 6F, true, true, true); } @Override public void preinit() { GameRegistry.registerBlock(this, name); GameRegistry.addShapedRecipe(new ItemStack(this), "RAR", "FTF", "RAR", 'F', Fusion.getItemFromId("fusion_ingot"), 'A', Fusion.getItemFromId("active_fusion_ingot"), 'T', Blocks.tnt, 'R', Items.redstone); GameRegistry.addShapedRecipe(new ItemStack(this), "RFR", "ATA", "RFR", 'F', Fusion.getItemFromId("fusion_ingot"), 'A', Fusion.getItemFromId("active_fusion_ingot"), 'T', Blocks.tnt, 'R', Items.redstone); } @Override public void init() { if (Fusion.SIDE == Side.CLIENT) Minecraft.getMinecraft().getRenderItem().getItemModelMesher().register(GameRegistry.findItem(Fusion.MODID, name), 0, new ModelResourceLocation(Fusion.MODID + ":" + name, "inventory")); } } And yes, it is shown that I checked !World.isRemote before creating the explosion. I'm stumped, thanks in advance. Edit: Added [1.8] prefix
-
Fair enough, I was aiming for neither prettiness nor fluency when coding that lol
-
Thank you all for helping! diesieben07 had the answer I needed, but you guys are all awesome for helping And yeah, the item registering code is temporary just so I could experiment with the concept... I'm not really big on reflection and I don't do it much, what was a major problem with the way I did it?
-
So should I fix this by putting that in its own separate method and calling the method for each package I want in order? Thanks, I'll try this!
-
I'm using 1.8 Forge and when registering my items/blocks, it appears crazily in the creative inventory. I know it sorts by item IDs, but I don't know how to register the blocks/items in the way I want so the IDs are sorted correctly. Here is a picture of how it looks now: My main mod class [Fusion.java]: package donkeycore.fusion; import java.io.File; import java.io.FileInputStream; import java.lang.reflect.Field; import java.net.URL; import java.util.ArrayList; import java.util.Collections; import java.util.List; import java.util.Random; import java.util.jar.JarEntry; import java.util.jar.JarInputStream; import net.minecraft.block.Block; import net.minecraft.creativetab.CreativeTabs; import net.minecraft.item.Item; import net.minecraft.item.ItemArmor; import net.minecraft.item.ItemArmor.ArmorMaterial; import net.minecraft.item.ItemPickaxe; import net.minecraft.util.ChatComponentText; import net.minecraft.util.DamageSource; import net.minecraft.util.EnumChatFormatting; import net.minecraft.world.World; import net.minecraftforge.common.MinecraftForge; import net.minecraftforge.common.config.Configuration; import net.minecraftforge.common.util.EnumHelper; import net.minecraftforge.event.ForgeEventFactory; import net.minecraftforge.event.world.BlockEvent; import net.minecraftforge.fml.common.FMLCommonHandler; import net.minecraftforge.fml.common.FMLLog; import net.minecraftforge.fml.common.Mod; import net.minecraftforge.fml.common.Mod.EventHandler; import net.minecraftforge.fml.common.event.FMLInitializationEvent; import net.minecraftforge.fml.common.event.FMLPostInitializationEvent; import net.minecraftforge.fml.common.event.FMLPreInitializationEvent; import net.minecraftforge.fml.common.event.FMLServerStartingEvent; import net.minecraftforge.fml.common.eventhandler.SubscribeEvent; import net.minecraftforge.fml.common.gameevent.PlayerEvent; import net.minecraftforge.fml.common.registry.GameRegistry; import net.minecraftforge.fml.relauncher.Side; import net.minecraftforge.fml.relauncher.SideOnly; import org.apache.logging.log4j.Level; import donkeycore.fusion.blocks.DegradedFusionBlock.Stages; import donkeycore.fusion.command.FusionCommand; @Mod(modid = Fusion.MODID, version = Fusion.VERSION) public final class Fusion { @Mod.Instance("fusion") public static Fusion instance; public static Configuration config; public static final String MODID = "fusion"; public static final String VERSION = "0.1"; public static final String BUILD = "beta_01"; public static final Random r = new Random(); public static Side SIDE; private static boolean initpre = false, init = false, initpost = false; public static final List<IFusionObject> fusionObjects = new ArrayList<IFusionObject>(); public static final DamageSource sourceRadiation = new DamageSource("fusion.radiation"); public static final DamageSource sourceStrongRadiation = new DamageSource("fusion.strongradiation"); public static final ArmorMaterial fusionArmorMaterial = EnumHelper.addArmorMaterial("fusionArmorMaterial", MODID + ":textures/armor/fusion", 30, new int[]{3, 8, 6, 3}, 15); public static final CreativeTabs tabFusion = new CreativeTabs("tabFusion") { @Override @SideOnly(Side.CLIENT) public Item getTabIconItem() { return getItemFromId("fusion_ingot"); } }; { instance = this; } @EventHandler public void init(FMLPreInitializationEvent event) { if (initpre) throw new IllegalStateException("Already preinitialized!"); initpre = true; SIDE = event.getSide(); info("Reading configuration..."); config = new Configuration(event.getSuggestedConfigurationFile()); Config.sync(); info("Registering blocks/items..."); try { String[] packages = new String[]{"blocks", "items.armor", "items.materials", "items.tools"}; boolean jar = Fusion.class.getResource("Fusion.class").toString().startsWith("jar:"); for(int i = 0; i < packages.length; i++) { List<Class<?>> cl = new ArrayList<Class<?>>(); if (jar) cl = getClassesInJar("donkeycore.fusion." + packages[i]); else cl = getClasses("donkeycore.fusion." + packages[i]); if(cl.size() < 1) bigWarning("There was a problem registering Fusion blocks/items!"); for(Class<?> c : cl) { if (ItemArmor.class.isAssignableFrom(c)) { boolean active = c.getSimpleName().contains("Active"); String[] types = new String[]{"helmet", "chestplate", "leggings", "boots"}; int x = 0; for(String type : types) fusionObjects.add((IFusionObject) c.getConstructor(String.class, int.class).newInstance((active ? "active_" : "") + "fusion_" + type, x++)); } else if(Item.class.isAssignableFrom(c) || Block.class.isAssignableFrom(c)) fusionObjects.add((IFusionObject) c.newInstance()); else warning("Unknown class: " + c.getSimpleName()); } } Collections.sort(fusionObjects); } catch(Throwable t) { bigWarning("UNABLE TO REGISTER ITEMS!!"); t.printStackTrace(); } for(IFusionObject f : fusionObjects) { f.preinit(); } } @EventHandler public void init(FMLInitializationEvent event) { if (init) throw new IllegalStateException("Already initialized!"); init = true; info("Initializing objects..."); MinecraftForge.EVENT_BUS.register(this); FMLCommonHandler.instance().bus().register(this); GameRegistry.registerWorldGenerator(new OreGenerator(), 1); for(IFusionObject f : fusionObjects) f.init(); } @EventHandler public void init(FMLPostInitializationEvent event) { if (initpost) throw new IllegalStateException("Already postinitialized!"); initpost = true; info("Fusion mod v" + VERSION + " enabled!"); } @EventHandler public void init(FMLServerStartingEvent event) { event.registerServerCommand(new FusionCommand()); } public static Item getItemFromId(String id) { return (Item) getFromId(id, Item.class); } public static ItemArmor getArmorFromId(String id) { return (ItemArmor) getFromId(id, ItemArmor.class); } public static Block getBlockFromId(String id) { return (Block) getFromId(id, Block.class); } public static Object getFromId(String id, Class<?> type) { for(IFusionObject o : fusionObjects) { try { Class<?> c = o.getClass(); if(type.isAssignableFrom(c)) { Field f = c.getDeclaredField("name"); f.setAccessible(true); String s = (String) f.get(o); if(s == null) s = (String) f.get(null); if(id.equals(s)) return o; } } catch(Throwable t) { t.printStackTrace(); return null; } } return null; } @SubscribeEvent public void onJoin(PlayerEvent.PlayerLoggedInEvent event) { if (!event.player.worldObj.isRemote && Config.loginMessage) event.player.addChatComponentMessage(new ChatComponentText(EnumChatFormatting.DARK_GREEN + "[Fusion v" + VERSION + "] " + EnumChatFormatting.GREEN + "This mod is in beta, so please report bugs!")); } @SubscribeEvent public void onBreak(BlockEvent.BreakEvent event) { if (event.getPlayer() == null || event.isCanceled()) return; if (Stages.has(event.state)) { if (event.state.getBlock().getUnlocalizedName().equals(getBlockFromId("degraded_fusion_block").getUnlocalizedName()) && Stages.from(event.state) != Stages.STABLE) explode(event); } if (event.state.getBlock().getUnlocalizedName().equals(getBlockFromId("fusion_ore").getUnlocalizedName()) && event.getPlayer().inventory.getCurrentItem() == null) { explode(event); return; } if (event.getPlayer().inventory.getCurrentItem() == null) return; Item i = event.getPlayer().inventory.getCurrentItem().getItem(); if (event.state.getBlock().getUnlocalizedName().equals(getBlockFromId("fusion_ore").getUnlocalizedName()) && ((!(i instanceof ItemPickaxe)) || (i instanceof ItemPickaxe && ((ItemPickaxe) i).getToolMaterial().getHarvestLevel() < 2))) { explode(event); return; } } private static final List<Class<?>> getClassesInJar(String packageName) { ArrayList<Class<?>> classes = new ArrayList<Class<?>>(); packageName = packageName.replaceAll("\\.", "/"); try { String jarName = new File(Fusion.class.getProtectionDomain().getCodeSource().getLocation().toURI().getPath()).getAbsolutePath(); JarInputStream jarFile = new JarInputStream(new FileInputStream(jarName)); JarEntry jarEntry; while(true) { jarEntry = jarFile.getNextJarEntry(); if (jarEntry == null) break; if ((jarEntry.getName().startsWith(packageName)) && (jarEntry.getName().endsWith(".class"))) classes.add(Class.forName(jarEntry.getName().replaceAll("/", "\\."))); } jarFile.close(); } catch(Throwable t) { t.printStackTrace(); } return classes; } public static final List<Class<?>> getClasses(String scannedPackage) { String scannedPath = scannedPackage.replace(".", "/"); URL scannedUrl = Thread.currentThread().getContextClassLoader().getResource(scannedPath); if (scannedUrl == null) throw new IllegalArgumentException(String.format("Unable to get resources from path '%s'.", scannedPath)); File scannedDir = new File(scannedUrl.getFile()); List<Class<?>> classes = new ArrayList<Class<?>>(); for(File file : scannedDir.listFiles()) classes.addAll(getClasses(file, scannedPackage)); return classes; } private static final List<Class<?>> getClasses(File file, String scannedPackage) { List<Class<?>> classes = new ArrayList<Class<?>>(); String resource = scannedPackage + "." + file.getName(); if (file.isDirectory()) { for(File child : file.listFiles()) classes.addAll(getClasses(child, resource)); } else if (resource.endsWith(".class")) { int endIndex = resource.length() - ".class".length(); String className = resource.substring(0, endIndex); if (!className.contains("$")) { try { classes.add(Class.forName(className)); } catch(ClassNotFoundException ignore) {} } } return classes; } private static final FusionExplosion explode(BlockEvent.BreakEvent event) { event.setCanceled(true); event.world.setBlockToAir(event.pos); return createFusionExplosion(event.world, event.pos.getX(), event.pos.getY(), event.pos.getZ(), 1.5F, false, false); } public static void info(String format, Object... data) { FMLLog.info("[Fusion] " + format, data); } public static void warning(String format, Object... data) { FMLLog.warning("[Fusion] " + format, data); } public static void severe(String format, Object... data) { FMLLog.severe("[Fusion] " + format, data); } public static void bigWarning(String format, Object... data) { FMLLog.bigWarning("[Fusion] " + format, data); } public static void info(Level level, String format, Object... data) { FMLLog.log(level, "[Fusion] " + format, data); } public static final FusionExplosion createFusionExplosion(World w, double x, double y, double z, float size, boolean fire, boolean smoke) { FusionExplosion explosion = new FusionExplosion(w, null, x, y, z, size, fire, smoke); if (ForgeEventFactory.onExplosionStart(w, explosion)) return explosion; explosion.doExplosionA(); explosion.doExplosionB(true); return explosion; } } And my base item template [FusionItem.java]: package donkeycore.fusion.items; import net.minecraft.client.Minecraft; import net.minecraft.client.resources.model.ModelResourceLocation; import net.minecraft.item.Item; import net.minecraft.item.ItemArmor; import net.minecraft.item.ItemTool; import net.minecraftforge.fml.common.registry.GameRegistry; import net.minecraftforge.fml.relauncher.Side; import donkeycore.fusion.Fusion; import donkeycore.fusion.IFusionObject; public abstract class FusionItem extends Item implements IFusionObject { { setMaxStackSize(64); setCreativeTab(Fusion.tabFusion); } private final String name; public FusionItem(String name) { this.name = name; setUnlocalizedName(name); } public void preinit() { GameRegistry.registerItem(this, name); } public void init() { if(Fusion.SIDE == Side.CLIENT) Minecraft.getMinecraft().getRenderItem().getItemModelMesher().register(this, 0, new ModelResourceLocation(Fusion.MODID + ":" + name, "inventory")); } public static final void init(Item i, String name) { if(Fusion.SIDE == Side.CLIENT) Minecraft.getMinecraft().getRenderItem().getItemModelMesher().register(i, 0, new ModelResourceLocation(Fusion.MODID + ":" + name, "inventory")); } @Override public int compareTo(IFusionObject o) { return o instanceof ItemArmor || o instanceof ItemTool ? -1 : 1; } } Any ideas of why the items aren't ordering correctly in the inventory? Thanks in advance