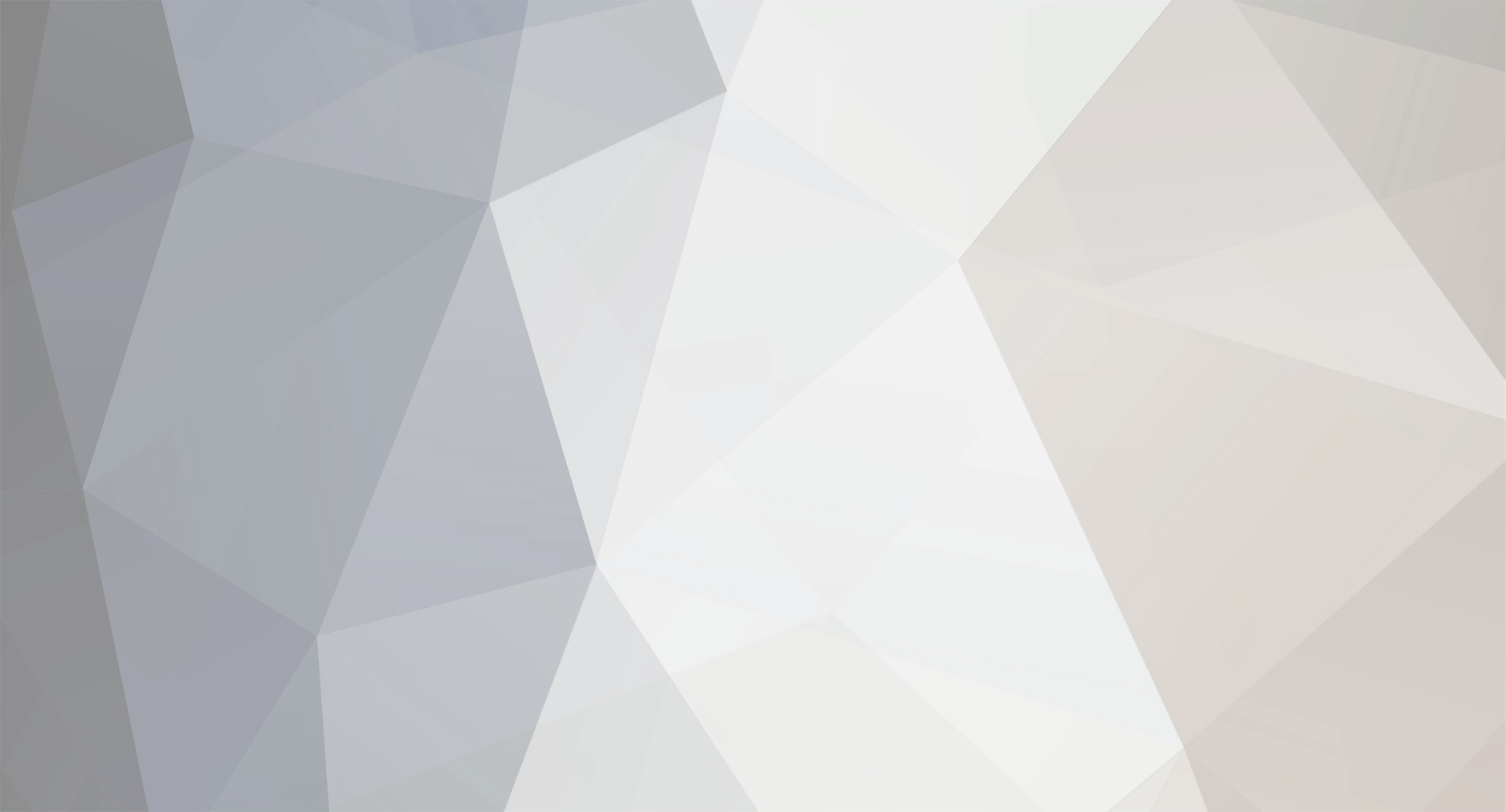
thelion1997
Members-
Posts
25 -
Joined
-
Last visited
Everything posted by thelion1997
-
I was having the same issue. Make sure that you have set up the accesstransformer.cfg file under resources/META_INF You will also need to uncomment this line in the build.gradle file and reload your gradle project. accessTransformer = file('src/main/resources/META-INF/accesstransformer.cfg') That should fix the access issue you are having.
-
[1.16.5] New Mob Spawns Not Spawning when run via jar file
thelion1997 replied to thelion1997's topic in Modder Support
For some reason the canSpawnOn function wasn't running properly from the jar. It seems to have clashed with where I set spawn placement type to inWater. public static boolean canSpawnOn(final EntityType<? extends MobEntity> entity, final IWorld world, final SpawnReason reason, final BlockPos pos, final Random rand) { if (pos.getY() > 25) { Optional<RegistryKey<Biome>> optional = world.func_242406_i(pos); return (Objects.equals(optional, Optional.of(Biomes.OCEAN)) || Objects.equals(optional, Optional.of(Biomes.DEEP_OCEAN)) || Objects.equals(optional, Optional.of(Biomes.WARM_OCEAN)) || Objects.equals(optional, Optional.of(Biomes.DEEP_WARM_OCEAN)) || Objects.equals(optional, Optional.of(Biomes.LUKEWARM_OCEAN)) || Objects.equals(optional, Optional.of(Biomes.DEEP_LUKEWARM_OCEAN)) || Objects.equals(optional, Optional.of(Biomes.COLD_OCEAN)) || Objects.equals(optional, Optional.of(Biomes.DEEP_COLD_OCEAN)) || Objects.equals(optional, Optional.of(Biomes.DEEP_OCEAN))) && world.getFluidState(pos).isTagged(FluidTags.WATER); } else { return false; } } I got this code mostly from the dolphinEntity class but modified it slightly. I tried changing this and ended up with this. I also changed the name of the function as I think there may have been an inheritance issue. This is what I ended up with. public static boolean canHippocampusSpawnOn(final EntityType<? extends MobEntity> entity, final IWorld world, final SpawnReason reason, final BlockPos pos, final Random rand) { if (pos.getY() <= 25 || pos.getY() >= world.getSeaLevel()) { return false; } RegistryKey<Biome> biome = world.func_242406_i(pos).orElse(Biomes.PLAINS); return (BiomeDictionary.hasType(biome, BiomeDictionary.Type.OCEAN)) && world.getFluidState(pos).isTagged(FluidTags.WATER); } This seems to have fixed the issue. Thanks again! -
[1.16.5] New Mob Spawns Not Spawning when run via jar file
thelion1997 replied to thelion1997's topic in Modder Support
Nvm. I just figured it out. Not sure why it was working in the IDE. -
[1.16.5] New Mob Spawns Not Spawning when run via jar file
thelion1997 replied to thelion1997's topic in Modder Support
Just set up the repo. Here is the link: https://github.com/WindRunner7/GreekMyths -
[1.16.5] New Mob Spawns Not Spawning when run via jar file
thelion1997 replied to thelion1997's topic in Modder Support
I don't have a repo set up yet. It is the hippocampus entity which is not spawning. Here is the class which registers the entities if it is helpful. @Mod.EventBusSubscriber(modid = GreekMyths.MOD_ID, bus = Mod.EventBusSubscriber.Bus.MOD) public class ModEntities { public static final EntityClassification MOD_FREINDLY = EntityClassification.create("ModFriendly", "ModFriendly", 20, true, true, 128); public static final EntityClassification MOD_WATER_CREATURE = EntityClassification.create("ModWater", "ModWater", 20, true, true, 128); public static final EntityClassification MOD_FREINDLY_CREATURE = EntityClassification.create("ModFriendlyCreature", "ModFriendlyCreature", 10, true, true, 128); public static final RegistryObject<EntityType<?>> GREEKVILLAGER = register("greekvillager_entity", () -> EntityType.Builder.create(GreekVillagerEntity::new, MOD_FREINDLY).build(new ResourceLocation(GreekMyths.MOD_ID, "greekvillager_entity").toString())); public static final RegistryObject<EntityType<?>> HIPPOCAMPUS = register("hippocampus_entity", () -> EntityType.Builder.create(HippocampusEntity::new, MOD_WATER_CREATURE).size(1.2f, 1.3f).build(new ResourceLocation(GreekMyths.MOD_ID, "hippocampus_entity").toString())); public static final RegistryObject<EntityType<?>> PEGASUS = register("pegasus_entity", () -> EntityType.Builder.create(PegasusEntity::new, MOD_FREINDLY_CREATURE).size(1.36f, 1.6f).build(new ResourceLocation(GreekMyths.MOD_ID, "pegasus_entity").toString())); // public static final RegistryObject<Item> GREEK_VILLAGER_SPAWN_EGG = Registration.ITEMS.register("greek_villager_spawn_egg", () -> // new SpawnEggItem(GREEKVILLAGER.get(), 0x2f5882, 0x6f1499, new Item.Properties().group(GreekMyths.ITEM_GROUP))); public static void register() { } public static <T extends EntityType<?>> RegistryObject<T> register(String name, Supplier<T> entity) { RegistryObject<T> ret = Registration.ENTITIES.register(name, entity); return ret; } @SubscribeEvent public static void setupEntityHandler(final FMLCommonSetupEvent event) { event.enqueueWork(() -> { setupEntity((EntityType<MobEntity>)GREEKVILLAGER.get(),GreekVillagerEntity::getAttributes, GreekVillagerEntity::canSpawnOn); setupEntity((EntityType<MobEntity>)HIPPOCAMPUS.get(), HippocampusEntity::getAttributes, HippocampusEntity::canSpawnOn); setupEntity((EntityType<MobEntity>)PEGASUS.get(), PegasusEntity::getAttributes, PegasusEntity::canSpawnOn); }); } public static <T extends MobEntity> void setupEntity(final EntityType<T> entityType, final Supplier<AttributeModifierMap.MutableAttribute> mapSupplier, @Nullable final EntitySpawnPlacementRegistry.IPlacementPredicate<T> placementPredicate){ GlobalEntityTypeAttributes.put((EntityType<? extends LivingEntity>) entityType, mapSupplier.get().create()); final EntitySpawnPlacementRegistry.PlacementType placementType = entityType.getClassification() == MOD_WATER_CREATURE ? EntitySpawnPlacementRegistry.PlacementType.IN_WATER : EntitySpawnPlacementRegistry.PlacementType.ON_GROUND; final EntitySpawnPlacementRegistry.IPlacementPredicate<T> placement = (entity, world, reason, pos, rand) -> placementPredicate.test(entity, world, reason, pos, rand); EntitySpawnPlacementRegistry.register(entityType, placementType, Heightmap.Type.MOTION_BLOCKING_NO_LEAVES, placement); } } -
[1.16.5] New Mob Spawns Not Spawning when run via jar file
thelion1997 replied to thelion1997's topic in Modder Support
Another this to note is that the lang file doesn't seem to load from the jar file either. Not sure if that is related or not... -
The new mob spawns properly when run from Intellij but not from the minecraft launcher using the jar. The strangest part is that the other two mobs I added spawn as expected in both cases. I have included the code used to register the spawns below. @Mod.EventBusSubscriber(modid = GreekMyths.MOD_ID, bus = Mod.EventBusSubscriber.Bus.FORGE) public class EntitySpawning { @SubscribeEvent(priority = EventPriority.HIGH) public static void addBiomeSpawns(final BiomeLoadingEvent event) { if(event.getCategory() == Biome.Category.OCEAN) { addSpawns(event, ModEntities.HIPPOCAMPUS.get(), 100, 1, 2); } addSpawns(event, ModEntities.GREEKVILLAGER.get(), 100, 1, 3); addSpawns(event, ModEntities.PEGASUS.get(), 100, 1, 3); } private static void addSpawns(final BiomeLoadingEvent biome, final EntityType<?> entity, final int chance, final int min, final int max) { if (biome.getCategory() == Biome.Category.NETHER) { } else if (biome.getCategory() == Biome.Category.THEEND) { } else { biome.getSpawns().withSpawner(entity.getClassification(), new MobSpawnInfo.Spawners(entity, chance, min, max)); } } } Also, you are still able to summon the mob using commands. Any thoughts?
-
Isn't that how you set the entity classification? For example, in order to run my code I have my entity registered through differed register as follows: public static final RegistryObject<EntityType<?>> GREEKVILLAGER = register("greekvillager_entity", () -> EntityType.Builder.create(GreekVillagerEntity::new, EntityClassification.CREATURE).build(new ResourceLocation(GreekMyths.MOD_ID, "greekvillager_entity").toString())); In that second field of the create method I am passing the EntityClassification.CREATURE What I would like is to create a different classification, lets say EntityClassification.MYMOBS and registier it as part of that instead. Is there a way to add additional Items to the EntityClassification Enum?
-
I will give this a try. Thanks for the advice.
-
I am trying to create a widget that is displayed in the corner of the screen but does not stop player movement. (much like the widget in the damage indicators mod) When I use a guiscreen it unbinds the mouse from the screen and stops the player from moving. Is there a easy way to do this?
-
How to get plant to drop both seeds and output
thelion1997 replied to thelion1997's topic in Modder Support
Not sure exactly what I did but it is working now. Thanks again. -
How to get plant to drop both seeds and output
thelion1997 replied to thelion1997's topic in Modder Support
Oh well I will keep trying thanks for the help. -
How to get plant to drop both seeds and output
thelion1997 replied to thelion1997's topic in Modder Support
I am. Here is the code. public final class RA_Blocks { public static final Block blockCoffeePlant = new BlockCoffeePlant(); public static final Block blockSpinachPlant = new BlockSpinachPlant(); public static void initBlocks() { GameRegistry.registerBlock(blockCoffeePlant, blockCoffeePlant.getUnlocalizedName().substring(5)); GameRegistry.registerBlock(blockSpinachPlant, blockSpinachPlant.getUnlocalizedName().substring(5)); } } -
How to get plant to drop both seeds and output
thelion1997 replied to thelion1997's topic in Modder Support
It doesn't look like it. It isn't hitting the break point. -
How to get plant to drop both seeds and output
thelion1997 replied to thelion1997's topic in Modder Support
public class BlockSpinachPlant extends BlockCrops { public String name = "BlockSpinachPlant"; //basic block properties public BlockSpinachPlant() { this.setBlockName(name); } @Override public ArrayList<ItemStack> getDrops(World world, int x, int y, int z, int metadata, int fortune) { ArrayList<ItemStack> drops = new ArrayList<ItemStack>(); drops.add(new ItemStack(RA_Items.itemSpinachSeeds, world.rand.nextInt(3) + 1)); drops.add(new ItemStack(RA_Items.itemSpinach, 1)); return drops; } @Override @SideOnly(Side.CLIENT) public void registerBlockIcons(IIconRegister parIIconRegister) { iIcon = new IIcon[maxGrowthStage+1]; // seems that crops like to have 8 growth icons, but okay to repeat actual texture if you want // to make generic should loop to maxGrowthStage iIcon[0] = parIIconRegister.registerIcon("ra:Coffee_stage_0"); iIcon[1] = parIIconRegister.registerIcon("ra:Coffee_stage_1"); iIcon[2] = parIIconRegister.registerIcon("ra:Coffee_stage_2"); iIcon[3] = parIIconRegister.registerIcon("ra:Coffee_stage_3"); iIcon[4] = parIIconRegister.registerIcon("ra:Coffee_stage_4"); iIcon[5] = parIIconRegister.registerIcon("ra:Coffee_stage_5"); iIcon[6] = parIIconRegister.registerIcon("ra:Coffee_stage_6"); iIcon[7] = parIIconRegister.registerIcon("ra:Coffee_stage_7"); } } and here is the BlockCrops class it implements public class BlockCrops extends BlockBush implements IGrowable { protected int maxGrowthStage = 7; @SideOnly(Side.CLIENT) protected IIcon[] iIcon; public BlockCrops() { // Basic block setup setTickRandomly(true); float f = 0.5F; setBlockBounds(0.5F - f, 0.0F, 0.5F - f, 0.5F + f, 0.25F, 0.5F + f); setCreativeTab(null); setHardness(0.0F); setStepSound(soundTypeGrass); disableStats(); } public boolean isOpaqueCube() { return false; // stops x-ray vision } //is the block grass, dirt or farmland @Override protected boolean canPlaceBlockOn(Block parBlock) { return parBlock == Blocks.farmland; } public void incrementGrowStage(World parWorld, Random parRand, int parX, int parY, int parZ) { int growStage = parWorld.getBlockMetadata(parX, parY, parZ) + MathHelper.getRandomIntegerInRange(parRand, 2, 5); if (growStage > maxGrowthStage) { growStage = maxGrowthStage; } parWorld.setBlockMetadataWithNotify(parX, parY, parZ, growStage, 2); } @Override public Item getItemDropped(int metadata, Random parRand, int parFortune) { return Item.getItemFromBlock(this); } // The type of render function that is called for this block @Override public int getRenderType() { return 1; // Cross like flowers } //Gets the block's texture. Args: side, meta @Override @SideOnly(Side.CLIENT) public IIcon getIcon(int parSide, int parGrowthStage) { return iIcon[parGrowthStage]; } //grows automatically after a random amount of ticks @Override public void updateTick(World parWorld, int parX, int parY, int parZ, Random parRand) { super.updateTick(parWorld, parX, parY, parZ, parRand); int growStage = parWorld.getBlockMetadata(parX, parY, parZ) + 1; if (growStage > 7) { growStage = 7; } parWorld.setBlockMetadataWithNotify(parX, parY, parZ, growStage, 2); } // checks if finished growing (a grow stage of 7 is final stage) @Override public boolean func_149851_a(World world, int parX, int parY, int parZ, boolean allowed) { return world.getBlockMetadata(parX, parY, parZ) != 7; //checks if bonemeal is allowed } @Override public boolean func_149852_a(World world, Random parRand, int parX, int parY, int parZ) { return true; } @Override public void func_149853_b(World world, Random rand, int parX, int parY, int parZ) { incrementGrowStage(world, rand, parX, parY, parZ); } /* func_149851_a returns true if bonemeal is allowed, false otherwise. func_149852_a returns true at the same time bonemeal is used if conditions for a growth-tick are acceptable. func_149853_b processes the actual growth-tick logic, which is usually increasing metadata or replacing the block. */ @Override public boolean canBlockStay (World world, int x, int y, int z) { Block soil = world.getBlock(x, y - 1, z); return (world.getFullBlockLightValue(x, y, z) >= 8 || world.canBlockSeeTheSky(x, y, z)) && (soil != null && soil.canSustainPlant(world, x, y - 1, z, ForgeDirection.UP, (IPlantable) RA_Items.itemCoffeeBeans)); } } -
How to get plant to drop both seeds and output
thelion1997 replied to thelion1997's topic in Modder Support
I replaced the previous code with @Override public ArrayList<ItemStack> getDrops(World world, int x, int y, int z, int metadata, int fortune) { ArrayList<ItemStack> drops = new ArrayList<ItemStack>(); drops.add(new ItemStack(RA_Items.itemSpinachSeeds, world.rand.nextInt(3) + 1)); drops.add(new ItemStack(RA_Items.itemSpinach, 1)); return drops; } however now it doesn't drop anything. I am missing something? -
I am working on a mod and I wan't a plant to drop both the seeds and the fruit Here is my code for the get item dropped [embed=425,349]@Override public Item getItemDropped(int parMetadata, Random parRand, int parFortune) { return (RA_Items.itemSpinachSeeds); } [/embed] How would I make it return the itemSpinachSeeds and an ItemSpinach?
-
I am working on a mod and after building it none of the textures or language files show up. Any help would be much appreciated. I have already checked to make sure the mod id is the same in both the java files and the mcmod.info file The Assets folder inside of the jar file shows up as capitalized even though it is lowercase in eclipse. Is this a problem?
-
Item Drop problem Minecraft Forge 1.7.10
thelion1997 replied to thelion1997's topic in Modder Support
Thanks that fixed it!!! -
Hey, I am trying to get a throwable entity to drop itself on impact. I have basically used to code from the snow ball and changed the damage and made it drop the itself as an item on impact. It works just fine when I throw it against a mob but when I throw it against a block it drops multiple floating icons, only one of which can be picked up. The rest just float there until you reload the world. any ideas as to why? Hear is my code for EntityRangerThrowingKnife import thelion1997.test.TestItems; import net.minecraft.block.Block; import net.minecraft.block.material.Material; import net.minecraft.entity.EntityLivingBase; import net.minecraft.entity.projectile.EntityArrow; import net.minecraft.entity.projectile.EntityThrowable; import net.minecraft.item.ItemStack; import net.minecraft.util.AxisAlignedBB; import net.minecraft.util.DamageSource; import net.minecraft.util.MovingObjectPosition; import net.minecraft.util.Vec3; import net.minecraft.world.World; public class EntityRangerThrowingKnife extends EntityThrowable { private World world; public EntityRangerThrowingKnife(World world) { super(world); this.world = world; } public EntityRangerThrowingKnife(World world, EntityLivingBase entity) { super(world, entity); this.world = world; } public EntityRangerThrowingKnife(World world, double x, double y, double z) { super(world, x, y, z); this.world = world; } @Override protected void onImpact(MovingObjectPosition mop) { Block block = world.getBlock(mop.blockX, mop.blockY, mop.blockZ); if (mop.entityHit != null) { // I changed this to type 'float' and set to '15'; float knifeDamage = 15; mop.entityHit.attackEntityFrom( DamageSource.causeThrownDamage(this, this.getThrower()), knifeDamage); } //This is where I drop the item entityDropItem(new ItemStack(TestItems.itemRangerThrowingKnife), 0); // I changed particles to "crit" particles at the point of impact for (int l = 0; l < 4; ++l) { this.worldObj.spawnParticle("crit", this.posX, this.posY, this.posZ, 0.0D, 0.0D, 0.0D); } if (!worldObj.isRemote) { setDead(); } } }