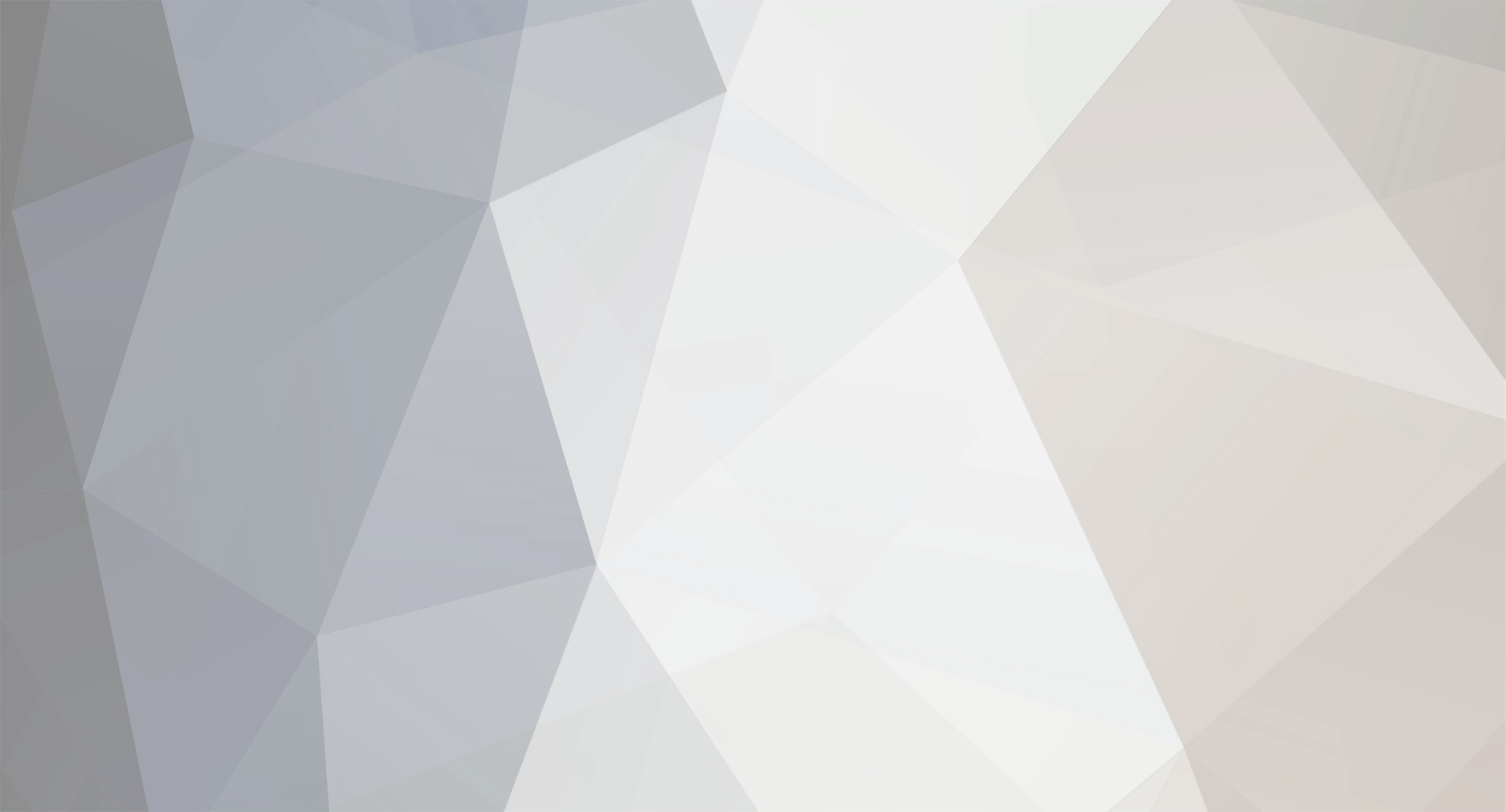
Noxyper
Members-
Posts
28 -
Joined
-
Last visited
Everything posted by Noxyper
-
[1.8] Transfering Data between two Tile Entities
Noxyper replied to Noxyper's topic in Modder Support
Although quite aggressive, thanks! It works fine. -
Hello! I've recently tried to get back to work on my mod that I've not worked on in months. Where I left off, I was working on a system to transfer steam through pipes to power my machinery. What I remember from back then was that some change in my code broke the system and now occasionally the game throws this crash: I've decoded this and found the source of the problem which is in this if statement (I will warn you, this if statement is incredibly long and will therefore look strange in this post. If you copy it and post it in a notepad document, it will look normal): The issue in the statement being this line of code: worldObj.getTileEntity(pos).getTileData().getString("liquid") == worldObj.getTileEntity(new BlockPos(pos.getX() - 1, pos.getY(), pos.getZ())).getTileData().getString("liquid") My theory is that because if the block it is checking for is not a tile entity, it doesn't know what to do and therefore crashes. I don't know how to solve this issue though and would appreciate any help I could get! If any more code is required, just ask me and I'll add it to this main post! Thanks in advance!
-
Thanks! Now, lastly, how can I make these pipes transport steam from one block to the other? I understand how I can start it off, by adding like a if(world.getBlockState(pos.up) == GnCBlocks.iron_pipe) { but it is in here I'm having troubles. } What do I put in that if-statement?
-
Alright, that should be working now, but one last question before I move on to the brain of this problem. How can I make my iron pipe and brass pipe not connect?
-
One problem done, next one! I've messed around with my code to try to connect these pipes to a machine, such as my boiler. The problem here though, is that it isn't working. At all. Here's the code: package com.minenemo.gearsandclouds.blocks; import java.util.List; import java.util.stream.Collectors; import java.util.stream.Stream; import net.minecraft.block.Block; import net.minecraft.block.material.Material; import net.minecraft.block.properties.IProperty; import net.minecraft.block.properties.PropertyBool; import net.minecraft.block.state.IBlockState; import net.minecraft.entity.Entity; import net.minecraft.util.AxisAlignedBB; import net.minecraft.util.BlockPos; import net.minecraft.util.EnumFacing; import net.minecraft.util.Vec3i; import net.minecraft.world.IBlockAccess; import net.minecraft.world.World; import com.google.common.collect.ImmutableList; public abstract class BlockPipeBase extends Block { public static final float PIPE_MIN_POS = 0.3125f; public static final float PIPE_MAX_POS = 0.6875f; public static final ImmutableList<IProperty> CONNECTED_PROPERTIES = ImmutableList.copyOf(Stream.of(EnumFacing.VALUES).map(facing -> PropertyBool.create(facing.getName())).collect(Collectors.toList())); public static final ImmutableList<AxisAlignedBB> CONNECTED_BOUNDING_BOXES = ImmutableList.copyOf(Stream.of(EnumFacing.VALUES).map(facing -> {Vec3i directionVec = facing.getDirectionVec();return new AxisAlignedBB(getMinBound(directionVec.getX()), getMinBound(directionVec.getY()), getMinBound(directionVec.getZ()),getMaxBound(directionVec.getX()), getMaxBound(directionVec.getY()), getMaxBound(directionVec.getZ()));}).collect(Collectors.toList())); private static float getMinBound(int dir) { return dir == -1 ? 0 : PIPE_MIN_POS; } private static float getMaxBound(int dir) { return dir == 1 ? 1 : PIPE_MAX_POS; } protected BlockPipeBase(Material material) { super(material); } @Override public boolean isOpaqueCube() { return false; } protected boolean isValidPipe(IBlockState ownState, IBlockState neighbourState, IBlockAccess world, BlockPos ownPos, EnumFacing neighbourDirection) { return neighbourState.getBlock() instanceof BlockPipeBase || neighbourState.getBlock() instanceof BlockBoiler || neighbourState.getBlock() instanceof BlockRuster; } private boolean canConnectTo(IBlockState ownState, IBlockAccess worldIn, BlockPos ownPos, EnumFacing neighbourDirection) { BlockPos neighbourPos = ownPos.offset(neighbourDirection); IBlockState neighbourState = worldIn.getBlockState(neighbourPos); Block neighbourBlock = neighbourState.getBlock(); boolean neighbourIsValidForThis = isValidPipe(ownState, neighbourState, worldIn, ownPos, neighbourDirection); boolean pipeIsValidForNeighbour = neighbourBlock instanceof BlockPipeBase && ((BlockPipeBase) neighbourBlock).isValidPipe(neighbourState, ownState, worldIn, neighbourPos, neighbourDirection.getOpposite()); boolean rusterIsValidForNeighbour = neighbourBlock instanceof BlockRuster && ((BlockRuster) neighbourBlock).isValidPipe(neighbourState, ownState, worldIn, neighbourPos, neighbourDirection.getOpposite()); boolean boilerIsValidForNeighbour = neighbourBlock instanceof BlockBoiler && ((BlockBoiler) neighbourBlock).isValidPipe(neighbourState, ownState, worldIn, neighbourPos, neighbourDirection.getOpposite()); boolean thisIsValidForNeighbour = pipeIsValidForNeighbour || rusterIsValidForNeighbour || boilerIsValidForNeighbour; return neighbourIsValidForThis && thisIsValidForNeighbour; } @Override public IBlockState getActualState(IBlockState state, IBlockAccess world, BlockPos pos) { for (EnumFacing facing : EnumFacing.VALUES) { state = state.withProperty(CONNECTED_PROPERTIES.get(facing.getIndex()), canConnectTo(state, world, pos, facing)); } return state; } public final boolean isConnected(IBlockState state, EnumFacing facing) { return (boolean) state.getValue(CONNECTED_PROPERTIES.get(facing.getIndex())); } public void setBlockBounds(AxisAlignedBB bb) { setBlockBounds((float) bb.minX, (float) bb.minY, (float) bb.minZ, (float) bb.maxX, (float) bb.maxY, (float) bb.maxZ); } @Override public void addCollisionBoxesToList(World worldIn, BlockPos pos, IBlockState state, AxisAlignedBB mask, List list, Entity collidingEntity) { setBlockBounds(PIPE_MIN_POS, PIPE_MIN_POS, PIPE_MIN_POS, PIPE_MAX_POS, PIPE_MAX_POS, PIPE_MAX_POS); super.addCollisionBoxesToList(worldIn, pos, state, mask, list, collidingEntity); state = getActualState(state, worldIn, pos); for (EnumFacing facing : EnumFacing.VALUES) { if (isConnected(state, facing)) { AxisAlignedBB axisAlignedBB = CONNECTED_BOUNDING_BOXES.get(facing.getIndex()); setBlockBounds(axisAlignedBB); super.addCollisionBoxesToList(worldIn, pos, state, mask, list, collidingEntity); } } setBlockBounds(PIPE_MIN_POS, PIPE_MIN_POS, PIPE_MIN_POS, PIPE_MAX_POS, PIPE_MAX_POS, PIPE_MAX_POS); } }
-
So now that THAT problem is fixed, I have another issue. Choonster touched upon this briefly and it is the transport of steam power through the pipes. I would rather not use any API, but if I have to, it is fine. As this is a bit bigger, I'll need help with a few different things. First off, where in the BlockPipeBase.java code can I add in the possibility of my pipes connecting to the machines? The code is posted below: BlockPipeBase.java package com.minenemo.gearsandclouds.blocks; import java.util.List; import java.util.stream.Collectors; import java.util.stream.Stream; import net.minecraft.block.Block; import net.minecraft.block.material.Material; import net.minecraft.block.properties.IProperty; import net.minecraft.block.properties.PropertyBool; import net.minecraft.block.state.IBlockState; import net.minecraft.entity.Entity; import net.minecraft.util.AxisAlignedBB; import net.minecraft.util.BlockPos; import net.minecraft.util.EnumFacing; import net.minecraft.util.Vec3i; import net.minecraft.world.IBlockAccess; import net.minecraft.world.World; import com.google.common.collect.ImmutableList; public abstract class BlockPipeBase extends Block { public static final float PIPE_MIN_POS = 0.3125f; public static final float PIPE_MAX_POS = 0.6875f; public static final ImmutableList<IProperty> CONNECTED_PROPERTIES = ImmutableList.copyOf(Stream.of(EnumFacing.VALUES).map(facing -> PropertyBool.create(facing.getName())).collect(Collectors.toList())); public static final ImmutableList<AxisAlignedBB> CONNECTED_BOUNDING_BOXES = ImmutableList.copyOf(Stream.of(EnumFacing.VALUES).map(facing -> {Vec3i directionVec = facing.getDirectionVec();return new AxisAlignedBB(getMinBound(directionVec.getX()), getMinBound(directionVec.getY()), getMinBound(directionVec.getZ()),getMaxBound(directionVec.getX()), getMaxBound(directionVec.getY()), getMaxBound(directionVec.getZ()));}).collect(Collectors.toList())); private static float getMinBound(int dir) { return dir == -1 ? 0 : PIPE_MIN_POS; } private static float getMaxBound(int dir) { return dir == 1 ? 1 : PIPE_MAX_POS; } protected BlockPipeBase(Material material) { super(material); } @Override public boolean isOpaqueCube() { return false; } protected boolean isValidPipe(IBlockState ownState, IBlockState neighbourState, IBlockAccess world, BlockPos ownPos, EnumFacing neighbourDirection) { return neighbourState.getBlock() instanceof BlockPipeBase; } private boolean canConnectTo(IBlockState ownState, IBlockAccess worldIn, BlockPos ownPos, EnumFacing neighbourDirection) { BlockPos neighbourPos = ownPos.offset(neighbourDirection); IBlockState neighbourState = worldIn.getBlockState(neighbourPos); Block neighbourBlock = neighbourState.getBlock(); boolean neighbourIsValidForThis = isValidPipe(ownState, neighbourState, worldIn, ownPos, neighbourDirection); boolean thisIsValidForNeighbour = neighbourBlock instanceof BlockPipeBase && ((BlockPipeBase) neighbourBlock).isValidPipe(neighbourState, ownState, worldIn, neighbourPos, neighbourDirection.getOpposite()); return neighbourIsValidForThis && thisIsValidForNeighbour; } @Override public IBlockState getActualState(IBlockState state, IBlockAccess world, BlockPos pos) { for (EnumFacing facing : EnumFacing.VALUES) { state = state.withProperty(CONNECTED_PROPERTIES.get(facing.getIndex()), canConnectTo(state, world, pos, facing)); } return state; } public final boolean isConnected(IBlockState state, EnumFacing facing) { return (boolean) state.getValue(CONNECTED_PROPERTIES.get(facing.getIndex())); } public void setBlockBounds(AxisAlignedBB bb) { setBlockBounds((float) bb.minX, (float) bb.minY, (float) bb.minZ, (float) bb.maxX, (float) bb.maxY, (float) bb.maxZ); } @Override public void addCollisionBoxesToList(World worldIn, BlockPos pos, IBlockState state, AxisAlignedBB mask, List list, Entity collidingEntity) { setBlockBounds(PIPE_MIN_POS, PIPE_MIN_POS, PIPE_MIN_POS, PIPE_MAX_POS, PIPE_MAX_POS, PIPE_MAX_POS); super.addCollisionBoxesToList(worldIn, pos, state, mask, list, collidingEntity); state = getActualState(state, worldIn, pos); for (EnumFacing facing : EnumFacing.VALUES) { if (isConnected(state, facing)) { AxisAlignedBB axisAlignedBB = CONNECTED_BOUNDING_BOXES.get(facing.getIndex()); setBlockBounds(axisAlignedBB); super.addCollisionBoxesToList(worldIn, pos, state, mask, list, collidingEntity); } } setBlockBounds(PIPE_MIN_POS, PIPE_MIN_POS, PIPE_MIN_POS, PIPE_MAX_POS, PIPE_MAX_POS, PIPE_MAX_POS); } } I'd assume it is in the canConnectTo() method (and maybe the isValidPipe() method) but I don't know how I'm supposed to go about this. I only want the pipe to be able to connect to the boiler from above.
-
Wow, now I feel stupid. Well, that's programming. Something that easy is always hiding under your nose. Thanks!
-
Here's all the pipe code https://github.com/BlackHyp3r/GnC/tree/master/Pipe And here is a debug info picture
-
Yep, it displays the properties. Do you need the full code?
-
Here's the getActualState code @Override public IBlockState getActualState(IBlockState state, IBlockAccess world, BlockPos pos) { for (EnumFacing facing : EnumFacing.VALUES) { state = state.withProperty(CONNECTED_PROPERTIES.get(facing.getIndex()), canConnectTo(state, world, pos, facing)); } return state; }
-
So, now I've messed around a bit with what you said and I got the bounding boxes to work, but the pipes don't connect. I attached a picture so that you could see what I mean: And here is the .json files: iron_pipe_side.json (model) { "textures": { "particle": "#side" }, "elements": [ { "name": "Cube", "from": [ 5.0, 5.0, 5.0 ], "to": [ 11.0, 11.0, 11.0 ], "faces": { "north": { "texture": "#side" }, "east": { "texture": "#side" }, "south": { "texture": "#side" }, "west": { "texture": "#side" }, "up": { "texture": "#side" }, "down": { "texture": "#side" } } } ] } iron_pipe_centre.json (model) { "textures": { "particle": "#centre" }, "elements": [ { "name": "Cube", "from": [ 5.0, 5.0, 5.0 ], "to": [ 11.0, 11.0, 11.0 ], "faces": { "north": { "texture": "#centre" }, "east": { "texture": "#centre" }, "south": { "texture": "#centre" }, "west": { "texture": "#centre" }, "up": { "texture": "#centre" }, "down": { "texture": "#centre" } } } ] } iron_pipe.json (blockstate) { "forge_marker": 1, "defaults": { "textures": { "centre": "blocks/iron_block", "side": "blocks/iron_block" }, "model": "gnc:iron_pipe_centre", "uvlock": true }, "variants": { "down": { "true": { "submodel": { "pipe_down": { "model": "gnc:iron_pipe_side", "x": 90, "uvlock": true } } }, "false": { } }, "up": { "true": { "submodel": { "pipe_up": { "model": "gnc:iron_pipe_side", "x": -90, "uvlock": true } } }, "false": { } }, "north": { "true": { "submodel": { "pipe_north": { "model": "gnc:iron_pipe_side", "uvlock": true } } }, "false": { } }, "south": { "true": { "submodel": { "pipe_south": { "model": "gnc:iron_pipe_side", "y": 180, "uvlock": true } } }, "false": { } }, "east": { "true": { "submodel": { "pipe_east": { "model": "gnc:iron_pipe_side", "y": 90, "uvlock": true } } }, "false": { } }, "west": { "true": { "submodel": { "pipe_west": { "model": "gnc:iron_pipe_side", "y": -90, "uvlock": true } } }, "false": { } } } }
-
No, how would that model look like? If it is going to be universal, how would I go about modelling it? Or am I just misunderstanding your posts?
-
So how do I go about making the pipe part? How would that look like?
-
Just so that I haven't wasted my time, I have to make .json files for all the different ways the pipe can connect, right? But 1 blockstate. That is how Mojang did with the redstone so I'm just assuming...
-
Alrighty then! So, for my mod I want to implement pipes to carry steam from a boiler to other machines. But I'm having some trouble. Maybe I am jumping straight into this, but I'm an okay programmer. I can the basics: armor, items, guis, mobs. But now I want to move on to the more advanced stuff! So, here's the deal. I'll be posting on this post everytime I run into trouble and maybe you guys could help? So my first problem is how to make these pipes connect to one another. I looked at the redstone code, but that just messes with my head. So, just to recap, my first problem is how do I make my pipes change state and connect to each other? Thank you! Hyp3r
-
Ey! That fixed it! Thanks!
-
Updated the code on GitHub: https://github.com/BlackHyp3r/GnC/blob/master/Message+Handler
-
I've localized the problem... I think... When sneding the message I write the line like this: GearsAndClouds.network.sendToServer(new GnCPacket("player invisible")); I think they mean that new. How do I work around that?
-
Following all of your directions it should work now... if it wasn't for the game crashing. Anyone who understands it? [21:59:16] [Client thread/INFO] [sTDOUT]: [net.minecraft.init.Bootstrap:printToSYSOUT:663]: ---- Minecraft Crash Report ---- // Uh... Did I do that? Time: 2015-08-21 21:59 Description: Initializing game java.lang.RuntimeException: java.lang.InstantiationException: minenemo.gearsandclouds.packet.GnCPacket$GnCPacketHandler at com.google.common.base.Throwables.propagate(Throwables.java:160) at net.minecraftforge.fml.common.network.simpleimpl.SimpleNetworkWrapper.instantiate(SimpleNetworkWrapper.java:116) at net.minecraftforge.fml.common.network.simpleimpl.SimpleNetworkWrapper.registerMessage(SimpleNetworkWrapper.java:106) at minenemo.gearsandclouds.GearsAndClouds.Init(GearsAndClouds.java:66) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:483) at net.minecraftforge.fml.common.FMLModContainer.handleModStateEvent(FMLModContainer.java:537) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:483) at com.google.common.eventbus.EventSubscriber.handleEvent(EventSubscriber.java:74) at com.google.common.eventbus.SynchronizedEventSubscriber.handleEvent(SynchronizedEventSubscriber.java:47) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:322) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:304) at com.google.common.eventbus.EventBus.post(EventBus.java:275) at net.minecraftforge.fml.common.LoadController.sendEventToModContainer(LoadController.java:212) at net.minecraftforge.fml.common.LoadController.propogateStateMessage(LoadController.java:190) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:483) at com.google.common.eventbus.EventSubscriber.handleEvent(EventSubscriber.java:74) at com.google.common.eventbus.SynchronizedEventSubscriber.handleEvent(SynchronizedEventSubscriber.java:47) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:322) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:304) at com.google.common.eventbus.EventBus.post(EventBus.java:275) at net.minecraftforge.fml.common.LoadController.distributeStateMessage(LoadController.java:119) at net.minecraftforge.fml.common.Loader.initializeMods(Loader.java:731) at net.minecraftforge.fml.client.FMLClientHandler.finishMinecraftLoading(FMLClientHandler.java:315) at net.minecraft.client.Minecraft.startGame(Minecraft.java:528) at net.minecraft.client.Minecraft.run(Minecraft.java:356) at net.minecraft.client.main.Main.main(Main.java:117) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:483) at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) at net.minecraft.launchwrapper.Launch.main(Launch.java:28) at net.minecraftforge.gradle.GradleStartCommon.launch(Unknown Source) at GradleStart.main(Unknown Source) Caused by: java.lang.InstantiationException: minenemo.gearsandclouds.packet.GnCPacket$GnCPacketHandler at java.lang.Class.newInstance(Class.java:418) at net.minecraftforge.fml.common.network.simpleimpl.SimpleNetworkWrapper.instantiate(SimpleNetworkWrapper.java:113) ... 41 more Caused by: java.lang.NoSuchMethodException: minenemo.gearsandclouds.packet.GnCPacket$GnCPacketHandler.<init>() at java.lang.Class.getConstructor0(Class.java:2971) at java.lang.Class.newInstance(Class.java:403) ... 42 more A detailed walkthrough of the error, its code path and all known details is as follows: --------------------------------------------------------------------------------------- -- Head -- Stacktrace: at com.google.common.base.Throwables.propagate(Throwables.java:160) at net.minecraftforge.fml.common.network.simpleimpl.SimpleNetworkWrapper.instantiate(SimpleNetworkWrapper.java:116) at net.minecraftforge.fml.common.network.simpleimpl.SimpleNetworkWrapper.registerMessage(SimpleNetworkWrapper.java:106) at minenemo.gearsandclouds.GearsAndClouds.Init(GearsAndClouds.java:66) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:483) at net.minecraftforge.fml.common.FMLModContainer.handleModStateEvent(FMLModContainer.java:537) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:483) at com.google.common.eventbus.EventSubscriber.handleEvent(EventSubscriber.java:74) at com.google.common.eventbus.SynchronizedEventSubscriber.handleEvent(SynchronizedEventSubscriber.java:47) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:322) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:304) at com.google.common.eventbus.EventBus.post(EventBus.java:275) at net.minecraftforge.fml.common.LoadController.sendEventToModContainer(LoadController.java:212) at net.minecraftforge.fml.common.LoadController.propogateStateMessage(LoadController.java:190) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:483) at com.google.common.eventbus.EventSubscriber.handleEvent(EventSubscriber.java:74) at com.google.common.eventbus.SynchronizedEventSubscriber.handleEvent(SynchronizedEventSubscriber.java:47) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:322) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:304) at com.google.common.eventbus.EventBus.post(EventBus.java:275) at net.minecraftforge.fml.common.LoadController.distributeStateMessage(LoadController.java:119) at net.minecraftforge.fml.common.Loader.initializeMods(Loader.java:731) at net.minecraftforge.fml.client.FMLClientHandler.finishMinecraftLoading(FMLClientHandler.java:315) at net.minecraft.client.Minecraft.startGame(Minecraft.java:528) -- Initialization -- Details: Stacktrace: at net.minecraft.client.Minecraft.run(Minecraft.java:356) at net.minecraft.client.main.Main.main(Main.java:117) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:483) at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) at net.minecraft.launchwrapper.Launch.main(Launch.java:28) at net.minecraftforge.gradle.GradleStartCommon.launch(Unknown Source) at GradleStart.main(Unknown Source) -- System Details -- Details: Minecraft Version: 1.8 Operating System: Windows 8.1 (amd64) version 6.3 Java Version: 1.8.0_05, Oracle Corporation Java VM Version: Java HotSpot 64-Bit Server VM (mixed mode), Oracle Corporation Memory: 648797256 bytes (618 MB) / 1037959168 bytes (989 MB) up to 1037959168 bytes (989 MB) JVM Flags: 3 total; -Xincgc -Xmx1024M -Xms1024M IntCache: cache: 0, tcache: 0, allocated: 0, tallocated: 0 FML: MCP v9.10 FML v8.0.99.99 Minecraft Forge 11.14.3.1450 4 mods loaded, 4 mods active States: 'U' = Unloaded 'L' = Loaded 'C' = Constructed 'H' = Pre-initialized 'I' = Initialized 'J' = Post-initialized 'A' = Available 'D' = Disabled 'E' = Errored UCHI mcp{9.05} [Minecraft Coder Pack] (minecraft.jar) UCHI FML{8.0.99.99} [Forge Mod Loader] (forgeSrc-1.8-11.14.3.1450.jar) UCHI Forge{11.14.3.1450} [Minecraft Forge] (forgeSrc-1.8-11.14.3.1450.jar) UCHE gnc{0.2} [Gears N' Clouds] (bin) Loaded coremods (and transformers): GL info: ' Vendor: 'NVIDIA Corporation' Version: '4.5.0 NVIDIA 355.60' Renderer: 'GeForce GTX 760/PCIe/SSE2' Launched Version: 1.8 LWJGL: 2.9.1 OpenGL: GeForce GTX 760/PCIe/SSE2 GL version 4.5.0 NVIDIA 355.60, NVIDIA Corporation GL Caps: Using GL 1.3 multitexturing. Using GL 1.3 texture combiners. Using framebuffer objects because OpenGL 3.0 is supported and separate blending is supported. Shaders are available because OpenGL 2.1 is supported. VBOs are available because OpenGL 1.5 is supported. Using VBOs: No Is Modded: Definitely; Client brand changed to 'fml,forge' Type: Client (map_client.txt) Resource Packs: [] Current Language: English (US) Profiler Position: N/A (disabled) [21:59:16] [Client thread/INFO] [sTDOUT]: [net.minecraft.init.Bootstrap:printToSYSOUT:663]: #@!@# Game crashed! Crash report saved to: #@!@# C:\Users\Nemo\Desktop\Programming\Gears N' Clouds 1.8\eclipse\.\crash-reports\crash-2015-08-21_21.59.16-client.txt AL lib: (EE) alc_cleanup: 1 device not closed Java HotSpot 64-Bit Server VM warning: Using incremental CMS is deprecated and will likely be removed in a future release
-
And I'm immediatly back for more knowledge! If you couldn't tell, I'm pretty new to packets, so how do I check what message got recieved? If I send a message saying "player invisible" then how do the MessageHandler check for that specific method?
-
Well, don't I feel stupid now... Thank you, everyone!
-
The player that presses button 'I.' If I press 'I,' I'm the one who should turn invisible, not everyone or no one on a server.
-
Alright, let me see... I might just be stupid here, but it seems as if 4/5 responses on this thread is about recieving the player from the message. In the EventHandler, I'm sending a message with said player. But how would I do that, and/or is that even necessary? If not, then how do I do this?
-
Sorry I haven't responded on this post for the past 2 days, school started again (yay ) Anyways, how do I tell the onMessage method that this message will recieve information about a player? Should I follow what delpi said or is that not necessary for what I'm doing?
-
But how do I send a message with a playerUUID, not recieve