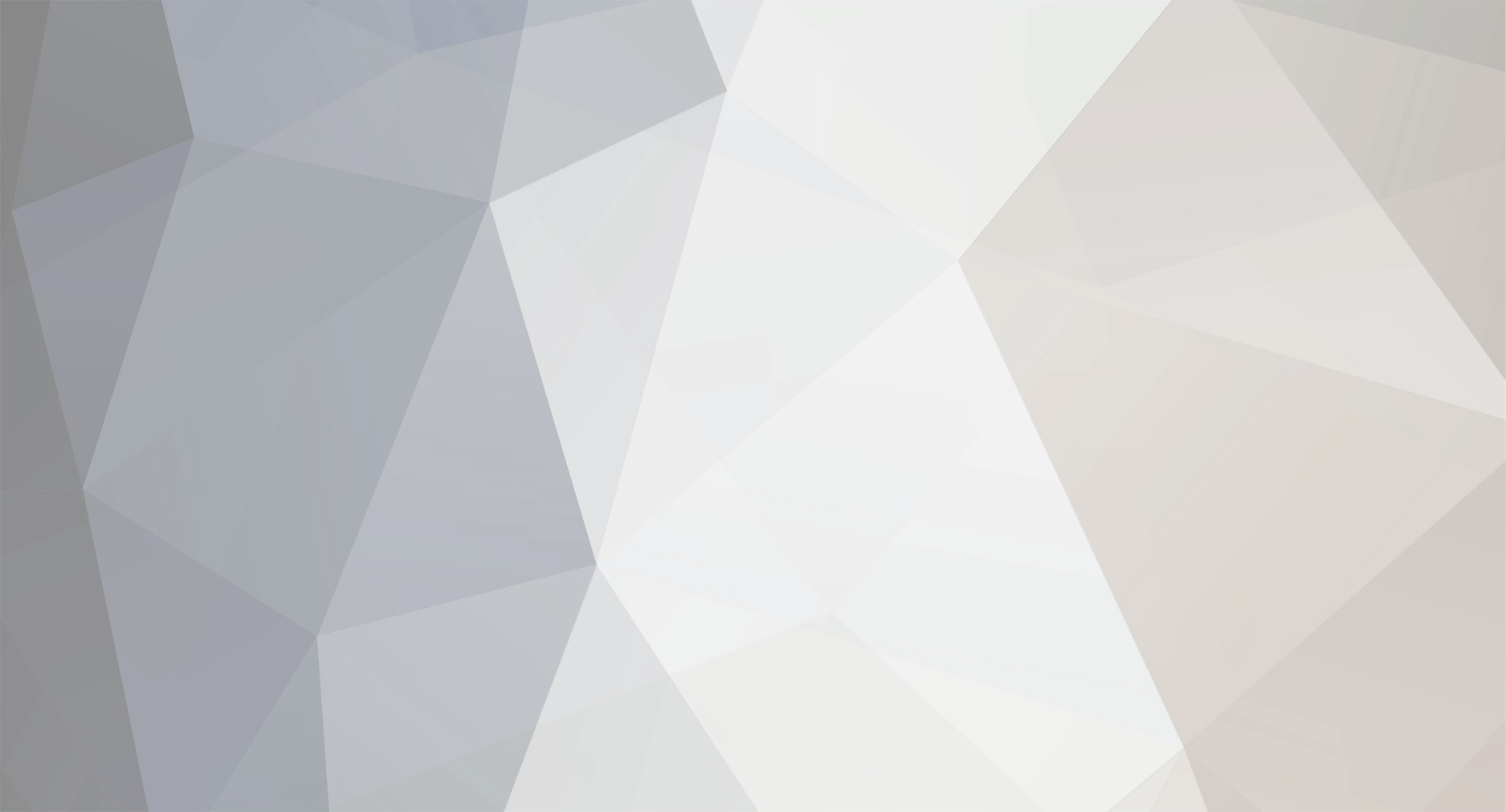
OnlyP1ng
Members-
Posts
17 -
Joined
-
Last visited
Everything posted by OnlyP1ng
-
I think you have to do the model bakery in the preInit part of the Client Proxy (if existing) and then ModelMesher part in the init method of the Client Proxy.
-
Do you put the GameRegistry part in preInit and the model part in init?
-
Hello everybody, first of all sorry for my bad english, im german . I created a BlockOre that has different states wich have different textures. Heres my Block class: package de.GetDeschmengd.MoreStuffMod.Blocks; import java.util.List; import net.minecraft.block.Block; import net.minecraft.block.material.Material; import net.minecraft.block.properties.IProperty; import net.minecraft.block.properties.PropertyEnum; import net.minecraft.block.state.BlockState; import net.minecraft.block.state.IBlockState; import net.minecraft.creativetab.CreativeTabs; import net.minecraft.item.Item; import net.minecraft.item.ItemStack; import net.minecraft.util.BlockPos; import net.minecraft.util.IStringSerializable; import net.minecraft.util.MovingObjectPosition; import net.minecraft.world.World; public class BlockOres extends Block implements IMetaBlockName{ public static final PropertyEnum TYPE = PropertyEnum.create("type", BlockOres.EnumType.class); protected BlockOres(Material materialIn, String unlocalizedName, String tool, int level, float hardness, float resistance) { super(materialIn); this.setUnlocalizedName(unlocalizedName); this.setHarvestLevel(tool, level); this.setHardness(hardness); this.setResistance(resistance); this.setCreativeTab(CreativeTabs.tabBlock); this.setDefaultState(this.blockState.getBaseState().withProperty(TYPE, EnumType.TIN)); } protected BlockOres(String unlocalizedName, float hardness, float resistance) { this(Material.rock, unlocalizedName, "pickaxe", 1, hardness, resistance); } protected BlockOres(String unlocalizedName) { this(unlocalizedName, 2.0F, 2.0F); } @Override protected BlockState createBlockState() { return new BlockState(this, new IProperty[] {TYPE}); } @Override public IBlockState getStateFromMeta(int meta) { switch(meta){ case 0: return getDefaultState().withProperty(TYPE, EnumType.TIN); case 1: return getDefaultState().withProperty(TYPE, EnumType.COPPER); default: return null; } } @Override public int getMetaFromState(IBlockState state) { EnumType type = (EnumType) state.getValue(TYPE); return type.getMeta(); } @Override public int damageDropped(IBlockState state) { return getMetaFromState(state); } @Override public void getSubBlocks(Item itemIn, CreativeTabs tab, List list) { list.add(new ItemStack(itemIn, 1, EnumType.TIN.getMeta())); list.add(new ItemStack(itemIn, 1, EnumType.COPPER.getMeta())); } public enum EnumType implements IStringSerializable{ TIN(0, "tin"), COPPER(1, "copper"); private int meta; private String name; private EnumType(int meta, String name) { this.meta = meta; this.name = name; } @Override public String getName() { return name; } @Override public String toString() { return getName(); } public int getMeta() { return meta; } } @Override public String getSpecialName(ItemStack stack) { return stack.getItemDamage() == 0 ? "tin" : "copper"; } @Override public ItemStack getPickBlock(MovingObjectPosition target, World world, BlockPos pos) { return new ItemStack(Item.getItemFromBlock(this), 1, this.getMetaFromState(world.getBlockState(pos))); } } With the block class i created a interface that is for getting the unlocalizedname for the items. Interface: package de.GetDeschmengd.MoreStuffMod.Blocks; import net.minecraft.item.ItemStack; public interface IMetaBlockName { String getSpecialName(ItemStack stack); } ItemBlock class: package de.GetDeschmengd.MoreStuffMod.Blocks; import net.minecraft.block.Block; import net.minecraft.item.ItemBlock; import net.minecraft.item.ItemStack; public class ItemBlockMeta extends ItemBlock{ public ItemBlockMeta(Block block) { super(block); if(!(block instanceof IMetaBlockName)){ throw new IllegalArgumentException(String.format("The given Block %s is not an instance of ISpecialBlockName!", block.getUnlocalizedName())); } this.setMaxDamage(0); this.setHasSubtypes(true); } @Override public int getMetadata(int damage) { return damage; } @Override public String getUnlocalizedName(ItemStack stack) { return super.getUnlocalizedName(stack) + "." + ((IMetaBlockName)this.block).getSpecialName(stack); } } I registered the block in my CommonProxy: CommonProxy: package de.GetDeschmengd.MoreStuffMod.proxies; import de.GetDeschmengd.MoreStuffMod.Blocks.ModBlocks; import net.minecraftforge.fml.common.event.FMLInitializationEvent; import net.minecraftforge.fml.common.event.FMLPostInitializationEvent; import net.minecraftforge.fml.common.event.FMLPreInitializationEvent; public class CommonProxy { public void preInit(FMLPreInitializationEvent e){ ModBlocks.init(); ModBlocks.registerBlocks(); } public void init(FMLInitializationEvent e){ } public void postInit(FMLPostInitializationEvent e){ } } ModBlocks class: package de.GetDeschmengd.MoreStuffMod.Blocks; import net.minecraft.block.Block; import net.minecraft.block.material.Material; import net.minecraft.item.ItemBlock; import net.minecraftforge.fml.common.registry.GameRegistry; public class ModBlocks { public static Block ores; public static void init(){ ores = new BlockOres("block_ores"); } public static void registerBlocks(){ reg(ores, "block_ores", ItemBlockMeta.class); } public static void reg(Block block, String name, Class<? extends ItemBlock> itemclass){ GameRegistry.registerBlock(block, itemclass, name); } public static void reg(Block block, String name){ GameRegistry.registerBlock(block, name); } } Here is my rendering registry: package de.GetDeschmengd.MoreStuffMod.render.blocks; import de.GetDeschmengd.MoreStuffMod.MoreStuffMod; import de.GetDeschmengd.MoreStuffMod.Blocks.ModBlocks; import net.minecraft.block.Block; import net.minecraft.client.Minecraft; import net.minecraft.client.resources.model.ModelBakery; import net.minecraft.client.resources.model.ModelResourceLocation; import net.minecraft.item.Item; public class BlockRenderRegister { public static String modid = MoreStuffMod.MODID; public static void preInit() { ModelBakery.addVariantName(Item.getItemFromBlock(ModBlocks.ores), "morestuffmod:block_ores_tin", "morestuffmod:block_ores_copper"); } public static void registerBlockRenderer(){ reg(ModBlocks.ores, 0, "block_ores_tin"); reg(ModBlocks.ores, 1, "block_ores_copper"); } public static void reg(Block block, int meta, String file){ Minecraft.getMinecraft().getRenderItem().getItemModelMesher().register(Item.getItemFromBlock(block), meta, new ModelResourceLocation(modid + ":" + file)); } public static void reg(Block block, String file){ Minecraft.getMinecraft().getRenderItem().getItemModelMesher().register(Item.getItemFromBlock(block), 0, new ModelResourceLocation(modid + ":" + file)); } } ClientProxy: package de.GetDeschmengd.MoreStuffMod.proxies; import de.GetDeschmengd.MoreStuffMod.Blocks.ModBlocks; import de.GetDeschmengd.MoreStuffMod.render.blocks.BlockRenderRegister; import net.minecraftforge.fml.common.event.FMLInitializationEvent; import net.minecraftforge.fml.common.event.FMLPostInitializationEvent; import net.minecraftforge.fml.common.event.FMLPreInitializationEvent; public class ClientProxy extends CommonProxy{ @Override public void preInit(FMLPreInitializationEvent e) { super.preInit(e); BlockRenderRegister.preInit(); } @Override public void init(FMLInitializationEvent e) { super.init(e); BlockRenderRegister.registerBlockRenderer(); } @Override public void postInit(FMLPostInitializationEvent e) { super.postInit(e); } } so here are my jsons: blockstates: { "variants": { "type=tin": { "model":"tutorial:block_ores_tin" }, "type=copper": { "model":"tutorial:block_ores_copper" } } } block (i put only one here because its the same) { "parent":"block/cube_all", "textures": { "all": "morestuffmod:blocks/block_ores_copper" } } item(i also put only one here) { "parent":"morestuffmod:block/block_ores_copper", "display": { "thirdperson": { "rotation": [ 10, -45, 170 ], "translation": [ 0, 1.5, -2.75 ], "scale": [ 0.375, 0.375, 0.375 ] } } } so when i start the game there is no texture not found error or anything but the texture doesnt renderes in item and in block model, when i do f3 it shows that the different states are working, and the unlocalizedname is changed too. heres my console log: thanks for helping
-
ok i'm going to to that thanks for your help byw
-
so how should i do?
-
oh i found the error it means models.item sorry that i employed you a very silly mistake from me
-
So heres a screenshot from my packages http://www.directupload.net/file/d/4096/q7fjzo6a_png.htm
-
here
-
i doesnt get any errors
-
Hello i created a block called furnancec so here's my Block class package com.youtube.blocks; import net.minecraft.block.Block; import net.minecraft.block.material.Material; import net.minecraft.creativetab.CreativeTabs; public class BlockFurnanceC extends Block{ public BlockFurnanceC() { super(Material.rock); setUnlocalizedName("furnancec"); setCreativeTab(CreativeTabs.tabBlock); setHardness(25F); setResistance(15F); } } so then i registered the block in my main class: package com.youtube; import com.youtube.blocks.BlockFurnanceC; import net.minecraft.block.Block; import net.minecraft.block.BlockFurnace; import net.minecraft.client.Minecraft; import net.minecraft.client.resources.model.ModelResourceLocation; import net.minecraft.item.Item; import net.minecraftforge.fml.common.Mod; import net.minecraftforge.fml.common.Mod.EventHandler; import net.minecraftforge.fml.common.Mod.Instance; import net.minecraftforge.fml.common.event.FMLInitializationEvent; import net.minecraftforge.fml.common.event.FMLPostInitializationEvent; import net.minecraftforge.fml.common.event.FMLPreInitializationEvent; import net.minecraftforge.fml.common.registry.GameRegistry; @Mod(modid = ZauberMod.MODID) public class ZauberMod { public static final String MODID = "zaubermod"; @Instance public static ZauberMod instance; //Blöcke public static Block blockFurnanceC = new BlockFurnanceC(); @EventHandler public void preInit(FMLPreInitializationEvent event){ } @EventHandler public void Init(FMLInitializationEvent event){ registerBlock(blockFurnanceC, "furnancec"); } public void postInit(FMLPostInitializationEvent event){ } public void registerBlock(Block block, String name){ GameRegistry.registerBlock(block, name); Minecraft.getMinecraft().getRenderItem().getItemModelMesher().register(Item.getItemFromBlock(block), 0, new ModelResourceLocation("zaubermod:" + name, "inventory")); } } so then i created the json renderes models.block: { "parent":"block/cube_all", "textures": { "all": "zaubermod:blocks/furnancec" } } models.item { "parent": "zaubermod:block/furnancec", "display": { "thirdperson": { "rotation": [ 10, -45, 170 ], "translation": [ 0, 1.5, -2.75 ], "scale": [ 0.375, 0.375, 0.375 ] } } } blockstates: { "variants": { "normal": { "model": "zaubermod:furnancec"} } } my problem is that the block doesn't renders the item texture (when its in the inventory) the proxies i create only before exporting the mod. thanks in advance for your answers
-
Thank you very much now it works so a very silly Mistake but i'm from germany
-
Hello, I made a tower.schematic file with MC Edit so now i want to generate this in my world so first of all i created a BlockObject class wich represent a single block in the schematic public class BlockObject { private BlockPos pos; private IBlockState state; public BlockObject(BlockPos pos, IBlockState state) { this.pos = pos; this.state = state; } public BlockPos getPos() { return pos; } public IBlockState getState() { return state; } public BlockPos getPositonWithOffset(int x, int y, int z){ return new BlockPos(x + pos.getX(), y + pos.getY(), z + pos.getZ()); } } next i created a .schematic reader that makes a array of block objects: private short width; private short length; private short heigth; private int size; private BlockObject[] blockObjects; public Schematic(String name) { try{ InputStream is = Schematic.class.getResourceAsStream("/assets/zaubermod/schematics/" + name); NBTTagCompound nbtdata = CompressedStreamTools.readCompressed(is); is.close(); width = nbtdata.getShort("Width"); length = nbtdata.getShort("Length"); heigth = nbtdata.getShort("Heigth"); size = width * length * heigth; blockObjects = new BlockObject[size]; byte[] blockids = nbtdata.getByteArray("Blocks"); byte[] metadata = nbtdata.getByteArray("Data"); int counter = 0; for(int i = 0; i < heigth; i++){ for(int j = 0; j < length; j++){ for(int k = 0; k < width; k++){ BlockPos pos = new BlockPos(k, i, j); IBlockState state = Block.getBlockById(blockids[counter]).getStateFromMeta(metadata[counter]); blockObjects[counter] = new BlockObject(pos, state); counter++; } } } }catch(Exception e){ e.printStackTrace(); } } public void generate(World world, int x, int y, int z){ for(BlockObject obj : blockObjects){ world.setBlockState(obj.getPositonWithOffset(x, y, z), obj.getState()); } } } So now i create a object of the schematic class in the main class: @Mod(modid = ZauberMod.MODID) public class ZauberMod { public static final String MODID = "zaubermod"; public static final Schematic TOWER_SCHEMATIC = new Schematic("tower.schematic"); @Instance public ZauberMod instance; @EventHandler public void preInit(FMLPreInitializationEvent event){ } @EventHandler public void Init(FMLInitializationEvent event){ GameRegistry.registerWorldGenerator(new SchematicGenereator(TOWER_SCHEMATIC), 0); } @EventHandler public void postInit(FMLPostInitializationEvent event){ } at the end i created a world generator that should create this structure every chunk: public class SchematicGenereator implements IWorldGenerator{ private Schematic schematic; public SchematicGenereator(Schematic schematic) { this.schematic = schematic; } @Override public void generate(Random random, int chunkX, int chunkZ, World world, IChunkProvider chunkGenerator, IChunkProvider chunkProvider) { int x = chunkX * 16 + random.nextInt(16); int z = chunkZ * 16 + random.nextInt(16); int y = getWorldHeightAt(world, x, z); schematic.generate(world, x, y, z); } private static int getWorldHeightAt(World worldIn, int x, int z){ int heigth = 0; for(int i = 0; i < 255; i++){ if(worldIn.getBlockState(new BlockPos(x, i, z)).getBlock().isSolidFullCube()){ heigth = i; } } return heigth; } } now my problem: it just doesnt create i can fly every where in the world but the Structure doesnt create thank you for your answers
-
[1.8] MInecraft Crashes when crafting custom Block
OnlyP1ng replied to OnlyP1ng's topic in Modder Support
Here are my json files blockstates { "variants": { "normal": { "model": "kohlewerk:maschc" } } } models.item { "parent": "kohlewerk:block/maschc", "display": { "thirdperson": { "rotation": [ 10, -45, 170 ], "translation": [ 0, 1.5, -2.75 ], "scale": [ 0.375, 0.375, 0.375 ] } } } models.block { "parent": "block/cube", "textures": { "particle": "kohlewerk:blocks/maschc_top", "up": "kohlewerk:blocks/maschc_top", "down": "kohlewerk:blocks/maschc_side", "north": "kohlewerk:blocks/maschc_front", "south": "kohlewerk:blocks/maschc_side", "west": "kohlewerk:blocks/maschc_side", "east": "kohlewerk:blocks/maschc_side" } } -
[1.8] MInecraft Crashes when crafting custom Block
OnlyP1ng replied to OnlyP1ng's topic in Modder Support
First of all thank you but is still doesnt work so now here`s my main class @Mod(modid = KohleWerk.MODID) public class KohleWerk { //Variablen public static final String MODID = "kohlewerk"; public static Item koks = new ItemKoks(); public static final int GUIMASCHC = 0; List<String> authors = Arrays.asList("Jan"); public static Block blockmaschc = new BlockMaschC(); @Instance public static KohleWerk instance; @EventHandler public void preInit(FMLPreInitializationEvent event){ GameRegistry.addShapedRecipe(new ItemStack(KohleWerk.blockmaschc), "XXX", "XYX", "ZZZ", 'X', Blocks.cobblestone, 'Y',Items.diamond, 'Z', Items.coal); //GuiHandler NetworkRegistry.INSTANCE.registerGuiHandler(instance, new GuiHandler()); //TileEntitys GameRegistry.registerTileEntity(TileEntityMaschC.class, "TileEntityMaschC"); ModMetadata ModData = event.getModMetadata(); modinfo(ModData); } @EventHandler public void Init(FMLInitializationEvent event){ GameRegistry.registerBlock(blockmaschc, "maschc"); Item itemfromblock = Item.getItemFromBlock(blockmaschc); GameRegistry.registerItem(koks, "koks"); Minecraft.getMinecraft().getRenderItem().getItemModelMesher().register(koks, 0, new ModelResourceLocation("kohlewerk:koks", "inventory")); Minecraft.getMinecraft().getRenderItem().getItemModelMesher().register(itemfromblock, 3, new ModelResourceLocation("kohlewerk:maschc", "inventory")); } @EventHandler public void postInit(FMLPostInitializationEvent event){ } public void modinfo(ModMetadata data){ //Modinfos setzten data.autogenerated = false; data.name = EnumChatFormatting.BLUE + "" + EnumChatFormatting.BOLD + "Kohle Werk"; data.authorList = authors; data.description = "Dieser Mod fügt ein Kohlewerk hinzu mit dem man Erze vervielvältigen kann."; data.credits = "HyCraftHD"; data.logoFile = "assets/kohlewerk/textures/logo/logo.png"; data.version= "0.1"; } } i set the mod data in methods becuase i don`t like the mcmod.info file so here`s my crashreport -
[1.8] MInecraft Crashes when crafting custom Block
OnlyP1ng replied to OnlyP1ng's topic in Modder Support
So my code is now this: @Mod(modid = KohleWerk.MODID) public class KohleWerk { //Variablen public static final String MODID = "kohlewerk"; public static Item koks = new ItemKoks(); public static final int GUIMASCHC = 0; List<String> authors = Arrays.asList("Jan"); Block blockmaschc = new BlockMaschC(); ItemStack maschc = new ItemStack(blockmaschc); @Instance public static KohleWerk instance; @EventHandler public void preInit(FMLPreInitializationEvent event){ GameRegistry.registerBlock(blockmaschc, "maschc"); Item itemfromblock = Item.getItemFromBlock(blockmaschc); Minecraft.getMinecraft().getRenderItem().getItemModelMesher().register(itemfromblock, 3, new ModelResourceLocation("kohlewerk:maschc", "inventory")); GameRegistry.registerItem(koks, "koks"); Minecraft.getMinecraft().getRenderItem().getItemModelMesher().register(koks, 0, new ModelResourceLocation("kohlewerk:koks", "inventory")); //GuiHandler NetworkRegistry.INSTANCE.registerGuiHandler(instance, new GuiHandler()); //TileEntitys GameRegistry.registerTileEntity(TileEntityMaschC.class, "TileEntityMaschC"); ModMetadata ModData = event.getModMetadata(); modinfo(ModData); } @EventHandler public void Init(FMLInitializationEvent event){ GameRegistry.addShapedRecipe(maschc, "XXX", "XYX", "ZZZ", 'X', Blocks.cobblestone, 'Y',Items.diamond, 'Z', Items.coal); } @EventHandler public void postInit(FMLPostInitializationEvent event){ } public void modinfo(ModMetadata data){ //Modinfos setzten data.autogenerated = false; data.name = EnumChatFormatting.BLUE + "" + EnumChatFormatting.BOLD + "Kohle Werk"; data.authorList = authors; data.description = "Dieser Mod fügt ein Kohlewerk hinzu mit dem man Erze vervielvältigen kann."; data.credits = "HyCraftHD"; data.logoFile = "assets/kohlewerk/textures/logo/logo.png"; data.version= "0.1"; } public void CraftingOfen(){ } } but it still crashes when i craft -
[1.8] MInecraft Crashes when crafting custom Block
OnlyP1ng replied to OnlyP1ng's topic in Modder Support
Still dont working but thanks for the tip -
Hey sorry for my bad english, i am german. So i am coding a mod where I craft a custom block but when i put the last item in the crafting gui minecraft crashes with a null pointer exception (when you need the crash report just post it) here is my main class: //Variablen public static final String MODID = "kohlewerk"; public static final int GUIMASCHC = 0; List<String> authors = Arrays.asList("Jan"); Block blockmaschc = new BlockMaschC(); ItemStack maschc = new ItemStack(blockmaschc); @Instance public static KohleWerk instance; @EventHandler public void preInit(FMLPreInitializationEvent event){ ModMetadata ModData = event.getModMetadata(); modinfo(ModData); GameRegistry.addShapedRecipe(maschc, "XXX", "XYX", "ZZZ", 'X', new ItemStack(Blocks.cobblestone), 'Y', new ItemStack(Items.diamond), 'Z', new ItemStack(Items.coal)); } @EventHandler public void Init(FMLInitializationEvent event){ GameRegistry.registerBlock(blockmaschc, "maschc"); Item itemfromblock = Item.getItemFromBlock(blockmaschc); Minecraft.getMinecraft().getRenderItem().getItemModelMesher().register(itemfromblock, 3, new ModelResourceLocation("kohlewerk:maschc", "inventory")); //GuiHandler NetworkRegistry.INSTANCE.registerGuiHandler(instance, new GuiHandler()); //TileEntitys GameRegistry.registerTileEntity(TileEntityMaschC.class, "TileEntityMaschC"); } @EventHandler public void postInit(FMLPostInitializationEvent event){ } public void modinfo(ModMetadata data){ //Modinfos setzten data.autogenerated = false; data.name = EnumChatFormatting.BLUE + "" + EnumChatFormatting.BOLD + "Kohle Werk"; data.authorList = authors; data.description = "Dieser Mod fügt ein Kohlewerk hinzu mit dem man Erze vervielvältigen kann."; data.credits = "HyCraftHD"; data.logoFile = "assets/kohlewerk/textures/logo/logo.png"; data.version= "0.1"; } public void CraftingOfen(){ } } I hope you can help me thanks in advance <3 Jan