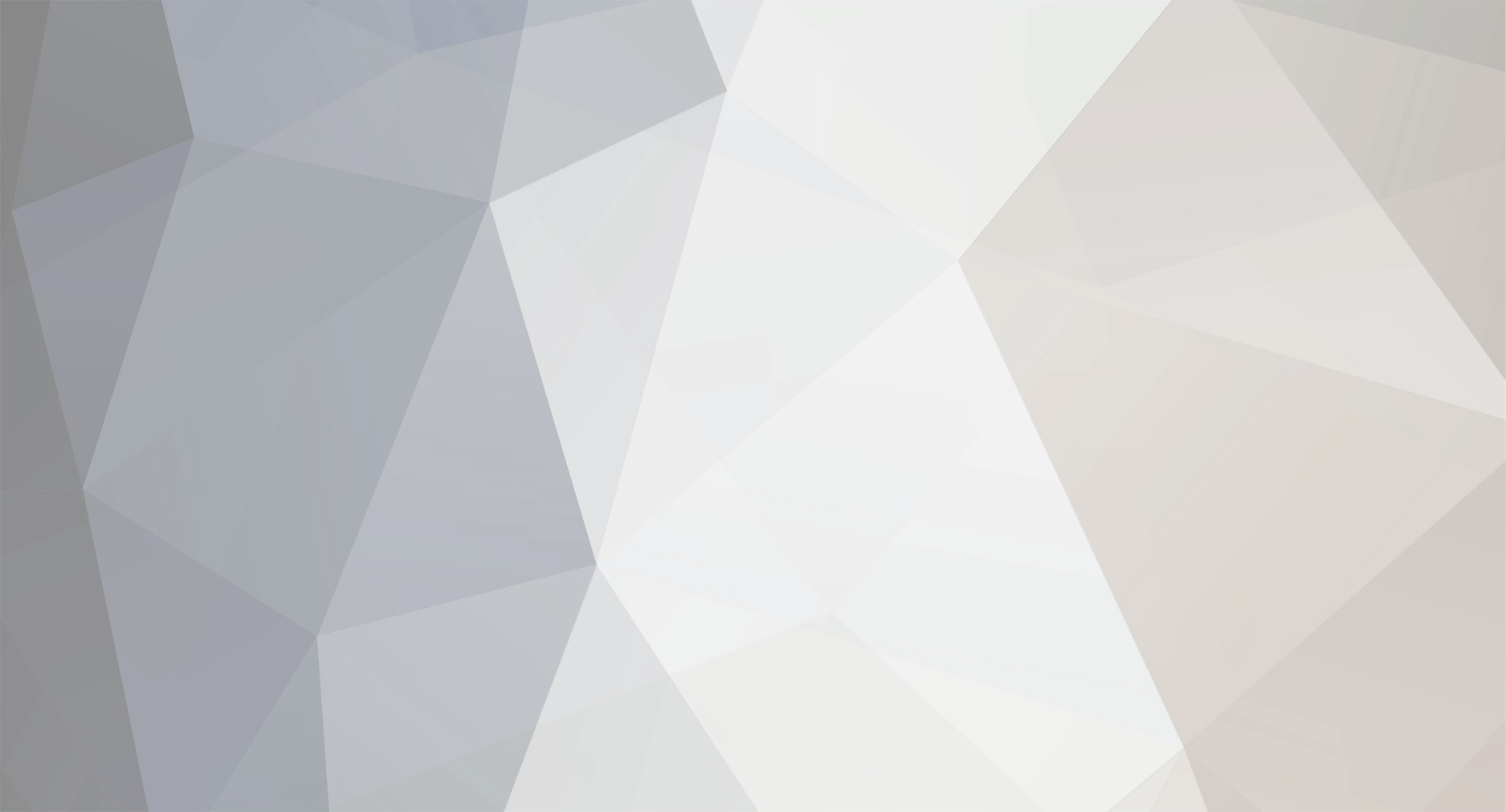
plr123
-
Posts
16 -
Joined
-
Last visited
Posts posted by plr123
-
-
Is your code in src/main/java?
Yes it is. My resources are in src/main/resources
-
Unfortunately there are no obvious errors in the log.
Are you sure your
@Mod
class is present in the JAR?
I decompiled the jar and found that the only folder is the "METAINF" folder. I think that might be a problem.....
-
In you item class (where you initialize you items) try putting in this code:
public static final ToolMaterial testToolMaterial = EnumHelper.addToolMaterial(String name, int harvestLevel, int maxUses, float efficiency, float damage, int enchantablility);
name - should be the same as you variable name, but you can change it.
harvestLevel - used for which blocks can be mined
maxUses - this is what you are looking for. This is basically how much damage your tool can take. Set this value to 1 or something like that. Play around with it and see what you can do
efficiency - how fast can it break blocks (pickaxes)
damage - how much damage it does against an entity
enchantablility - how much the tool can be enchanted
Then just create a new class that extends the type of tool you want.
Ex:
public class TestTool extends ItemPickaxe
Then just let it add in the constructor. Make sure in your items class that your tool is initialized to "TestTool()" or whatever you named your tool class.
You might want to refer to this article in the future: http://bedrockminer.jimdo.com/modding-tutorials/basic-modding-1-7/custom-tools-swords/
It may be for 1.7, but it is actually the same thing for 1.8.
He actually has some great tutorials for beginners
-
I know it says that. It loads fine when I am testing it within eclipse, but when I actually put it in my mods folder, it doesn't load at all.
Then post a log from when it doesn't load (i.e. from a client with the mod in its mods folder).
The debugging suggestion was a separate recommendation to the log posting.
Log file: https://gist.github.com/anonymous/4ee059313179dc31ccd4
-
I think your modID is too short. I have a vague recollection that it has to be at least 3 characters.
I just changed my modid to a longer one, but still no change.
Here is the log: https://gist.github.com/anonymous/68638fd46aedb3d418fc
It looks like your mod loads without issue in that log.
I know it says that. It loads fine when I am testing it within eclipse, but when I actually put it in my mods folder, it doesn't load at all.
-
Where is "Reference", and what all is in it?
This is my Reference class:
package com.parth; public class Reference { public static final String MOD_ID = "tm"; public static final String MOD_NAME = "[1.8] DrVader's Robot Pet Mod"; public static final String VERSION = "1.0"; public static final String CLIENT_PROXY_CLASS = "com.parth.proxy.ClientProxy"; public static final String SERVER_PROXY_CLASS = "com.parth.proxy.CommonProxy"; }
[23:56:05] [Client thread/INFO] [FML]: FML has found a non-mod file [1.8]DrVaders Robot Pet Mod-1.0.jar in your mods directory. It will now be injected into your classpath. This could severe stability issues, it should be removed if possible.
Did you put anything in your Mods folder, found in your mod dev folder? I've seen this happen when you throw a mod (zip file / jar) into the mods folder (a non-deobfuscated file). Really, what have you in there?
I only have put my mod in there. Nothing else. Also, my mod file is a jar file.
It looks like you've posted the output from the console, but the log file should have some more information about the mod loading process in it. Could you post that? Use Gist if it's too large for Pastebin.
You should also try building a deobfuscated copy of your mod (run the
jar
Gradle task), adding that as a mod in a separate ForgeGradle project, putting some breakpoints in
JarDiscoverer#discover
and stepping through it to see what's going wrong.
Here is the log: https://gist.github.com/anonymous/68638fd46aedb3d418fc
-
You are missing your @instance annotation and field.
My new code:
package com.parth; import java.awt.Color; import net.minecraft.client.Minecraft; import net.minecraft.entity.Entity; import net.minecraft.entity.EntityList; import net.minecraft.init.Blocks; import net.minecraft.init.Items; import net.minecraft.item.ItemStack; import net.minecraft.util.ChatComponentText; import net.minecraft.util.ResourceLocation; import net.minecraftforge.common.MinecraftForge; import net.minecraftforge.fml.client.registry.RenderingRegistry; import net.minecraftforge.fml.common.FMLCommonHandler; import net.minecraftforge.fml.common.Mod; import net.minecraftforge.fml.common.Mod.EventHandler; import net.minecraftforge.fml.common.Mod.Instance; import net.minecraftforge.fml.common.ModClassLoader; import net.minecraftforge.fml.common.SidedProxy; import net.minecraftforge.fml.common.event.FMLInitializationEvent; import net.minecraftforge.fml.common.event.FMLPostInitializationEvent; import net.minecraftforge.fml.common.event.FMLPreInitializationEvent; import net.minecraftforge.fml.common.registry.EntityRegistry; import net.minecraftforge.fml.common.registry.GameRegistry; import net.minecraftforge.fml.common.registry.LanguageRegistry; import net.minecraftforge.fml.relauncher.Side; import net.minecraftforge.fml.relauncher.SideOnly; import com.parth.entity.Entities; import com.parth.event.PlayerJoin; import com.parth.init.RobotPetBlocks; import com.parth.init.RobotPetItems; import com.parth.proxy.CommonProxy; import com.parth.recipe.Recipes; import com.parth.worldgen.WorldRegister; @Mod(modid = Reference.MOD_ID, name = Reference.MOD_NAME, version = Reference.VERSION) public class RobotPetMod { @SidedProxy(clientSide = Reference.CLIENT_PROXY_CLASS, serverSide = Reference.SERVER_PROXY_CLASS) public static CommonProxy proxy; public static final RobotPetTab robotPetTab = new RobotPetTab("robotPetTab"); static int MyEntityID = 300; @Instance(value = Reference.MOD_ID) //What I added public static RobotPetMod instance; //What I added @EventHandler public void preInit(FMLPreInitializationEvent event) { RobotPetBlocks.init(); RobotPetBlocks.register(); RobotPetItems.init(); RobotPetItems.register(); Entities.preinit(); WorldRegister.mainRegistry(); } @EventHandler public void init(FMLInitializationEvent event) { proxy.registerRenders(); Recipes.loadRecipes(); MinecraftForge.EVENT_BUS.register(new PlayerJoin()); } @EventHandler public void postInit(FMLPostInitializationEvent event) { } }
The same thing still happens
-
This couldn't make any more sense. Your saying have a Main class with the "@Mod" annotation, including its several parameters, yet Forge / FML is not recognizing your Mod as a Mod. What is happening in the console? Did you even look in the "Mods" button at the Minecraft Main Menu? That is very basic - check the mods button, post the log, and show us your code (Main class).
I made sure each time if the mod was loaded and each time it wasn't.
My Main class:
package com.parth; import java.awt.Color; import net.minecraft.client.Minecraft; import net.minecraft.entity.Entity; import net.minecraft.entity.EntityList; import net.minecraft.init.Blocks; import net.minecraft.init.Items; import net.minecraft.item.ItemStack; import net.minecraft.util.ChatComponentText; import net.minecraft.util.ResourceLocation; import net.minecraftforge.common.MinecraftForge; import net.minecraftforge.fml.client.registry.RenderingRegistry; import net.minecraftforge.fml.common.FMLCommonHandler; import net.minecraftforge.fml.common.Mod; import net.minecraftforge.fml.common.Mod.EventHandler; import net.minecraftforge.fml.common.ModClassLoader; import net.minecraftforge.fml.common.SidedProxy; import net.minecraftforge.fml.common.event.FMLInitializationEvent; import net.minecraftforge.fml.common.event.FMLPostInitializationEvent; import net.minecraftforge.fml.common.event.FMLPreInitializationEvent; import net.minecraftforge.fml.common.registry.EntityRegistry; import net.minecraftforge.fml.common.registry.GameRegistry; import net.minecraftforge.fml.common.registry.LanguageRegistry; import net.minecraftforge.fml.relauncher.Side; import net.minecraftforge.fml.relauncher.SideOnly; import com.parth.entity.Entities; import com.parth.event.PlayerJoin; import com.parth.init.RobotPetBlocks; import com.parth.init.RobotPetItems; import com.parth.proxy.CommonProxy; import com.parth.recipe.Recipes; import com.parth.worldgen.WorldRegister; @Mod(modid = Reference.MOD_ID, name = Reference.MOD_NAME, version = Reference.VERSION) public class RobotPetMod { @SidedProxy(clientSide = Reference.CLIENT_PROXY_CLASS, serverSide = Reference.SERVER_PROXY_CLASS) public static CommonProxy proxy; public static final RobotPetTab robotPetTab = new RobotPetTab("robotPetTab"); @EventHandler public void preInit(FMLPreInitializationEvent event) { RobotPetBlocks.init(); RobotPetBlocks.register(); RobotPetItems.init(); RobotPetItems.register(); Entities.preinit(); WorldRegister.mainRegistry(); } @EventHandler public void init(FMLInitializationEvent event) { proxy.registerRenders(); Recipes.loadRecipes(); MinecraftForge.EVENT_BUS.register(new PlayerJoin()); } @EventHandler public void postInit(FMLPostInitializationEvent event) { } }
The Game Output/Log:
The error in the log is particularly this line:
[23:56:05] [Client thread/INFO] [FML]: FML has found a non-mod file [1.8]DrVaders Robot Pet Mod-1.0.jar in your mods directory. It will now be injected into your classpath. This could severe stability issues, it should be removed if possible.
-
Hey,
I have been having this problem for about a day now. I have done some research to figure this out by myself, but I couldn't find a solution.
The error I am getting is in the game logs. It says that my mod is being recognized as a non-mod file. I have used multiple sources and they all said to add things that I already had.
In my research, many sources said that it wasn't being recognized because I didn't have a @Mod annotation in my main class. I actually do have one.
Anyway, does anyone know how to fix this?
My mcmod.info: http://pastebin.com/HJDhRxzL
My build.gradle: http://pastebin.com/zHTwmtG2
Thanks!
-
@Override public boolean onItemUse(ItemStack stack, EntityPlayer playerIn, World worldIn, BlockPos pos, EnumFacing side, float hitX, float hitY, float hitZ) { if (!worldIn.isRemote) { EntityZombie test = new EntityZombie(worldIn); worldIn.spawnEntityInWorld(test); test.setPosition(pos.getX(), pos.getY() + 1, pos.getZ()); return true; } return false; }
I actually just tried that code out right now. I still get nothing spawning when I write "!worldIn.isRemote" and I get a "still" zombie when I write "worldIn.isRemote"
This is how the zombie looks like when I write "worldIn.isRemote":
Notice how the zombie isn't even on fire or reacting to me being that close to it.
As for this:
There is probably something wrong with your EntityTest - you might not have renderer for it. Or you messed up constructors?
I have a renderer for my Entity. I am able to spawn the entity fine with an egg. I just want the player to be able to spawn the entity with an item as I dont want the entity spawning naturally and eggs are basically unattainable in survival.
-
Hey,
I have been having this problem for a couple of days. When I try to spawn an entity with my item by clicking the ground, it spawns, but it doesn't move at all, it can't get hurt, it can't be pushed, etc. I think once I waited for a little, and it disappeared (I'm not sure about this though).
NOTE: I have tried doing my own research and they all said that I needed to add
if (!world.isRemote)
in order for it to work. I have tried this, but nothing at all spawns.
This is the code I am using:
@Override public boolean onItemUse(ItemStack stack, EntityPlayer playerIn, World worldIn, BlockPos pos, EnumFacing side, float hitX, float hitY, float hitZ) { if(stack.getItem() == TestItems.test_item) { if (!worldIn.isRemote) { EntityTest test= new EntityTest(worldIn); worldIn.spawnEntityInWorld(test); test.setPosition(pos.getX(), pos.getY() + 1, pos.getZ()); return true; } } return false; }
How do I fix this?
-
1. Use World#getLoadedEntityList and iterate through each entry checking if a. is it an EntityTameable, and b. does it belong to the player; teleport them as they are found.
I tried using that, but when I do, nothing happens. This is the code I'm using:
@Override public boolean onItemUse(ItemStack stack, EntityPlayer playerIn, World worldIn, BlockPos pos, EnumFacing side, float hitX, float hitY, float hitZ) { if(stack.getItem() == RobotPetItems.bucket_oil) { Object o = worldIn.getLoadedEntityList(); if (o instanceof EntityTameable) { if (((EntityTameable) o).isOwner(playerIn)) { System.out.println("Found Entity"); return true; } else { System.out.println("No entity found"); return false; } } //playerIn.setPosition(pos.getX(), pos.getY() + 1, pos.getZ()); //return true; } return false; }
I sorry but I'm actually new to this.
-
Is it YOUR block, a vanilla block, any block? If it's your block, you can override the onBlockClicked method (or whatever it's called) and you'll have the coordinates there; if it can by any block, but your Item, you can do what you did above and use onItemUse. Either way, that will give you the Block's position.
How are you going to find the entity to teleport? Do you have some kind of system to track the entity, e.g. if the entity's unique ID is stored in the ItemStack NBT, the Block's TileEntity, or even the player's IEEP? Without such a system, it will be impossible to know which entity to look for and teleport to the block position.
If you describe how your item/block/entity teleportation should work, not in code but from the player's perspective in game, then I could give you better advice.
It is any block.
So let's assume this:
My custom item will be called test_item
My custom entity will be called EntityTest
Now for what I want to happen:
From a player's perspective, the player has item test_item in their hand. With this, they can right click any block, and all of their tamed entity/entities EntityTest teleport to that clicked block.
I don't know if this is possible. But if it is, could you please give me a walkthrough of what I would have to do? It doesn't have to be the entire code; I just want the concepts that I would have to apply to make this work.
-
You can't just create a new entity whenever you want - you need the instance of the entity that already exists in the world. Luckily, there is an Item method called whenever you right-click on an entity with your item, which you can override to do whatever you want, e.g. teleport:
@Override public boolean itemInteractionForEntity(ItemStack stack, EntityPlayer player, EntityLivingBase entity) { if (entity instanceof YourTargetEntityClass) { // put teleportation code or whatever here return true; } return false; }
That isn't exactly what I am looking for. I don't really want the entity to teleport when I right click on it. I want it to teleport to a block when I right click an item on that block. I'm just wondering if this is possible. If it is, how would I go about doing it? If it isn't, then I will just have to find another way to do something similar.
-
Hi,
I have been creating a mod with a custom entity, and I wanted to teleport it when I clicked a specific block to that block. I have tried to get it to work, but it seems that I don't exactly know how to initialize the entity properly so that it is recognized by the onItemUse() method. This is the code I have been trying with fail:
public boolean onItemUse(ItemStack stack, EntityPlayer playerIn, World worldIn, BlockPos pos, EnumFacing side, float hitX, float hitY, float hitZ) { EntityTameable entity = new EntityRobot(worldIn); //my custom entity that I attempt to initialize if(stack.getItem() == MyMod.bucket_oil) { //bucket_oil is just the name for an item entity.setLocationAndAngles((double)pos.getX(), (double)pos.getY(), (double)pos.getZ(), 1.0F, 1.0F); //attempts to teleport entity but does not do anything System.out.println(entity.getEntityId()); //check to see if my entity is "seen" return true; } return false; }
Thanks!
[1.8] Mod is not recognized as a mod
in Modder Support
Posted
This worked! Thanks!
But now I have run into another problem. When the mod loads onto the menu screen, it appears as "Tutorial Mod" which was a mod I had created when I was learning modding about 2 months ago. Do you or anyone know how to change this? Is there a file where I can change this? My mcmod.info file is set up how I want it to be, but thats not how it is.
EDIT: Sorry I just had compiled the wrong mod. But thanks to everyone that helped!