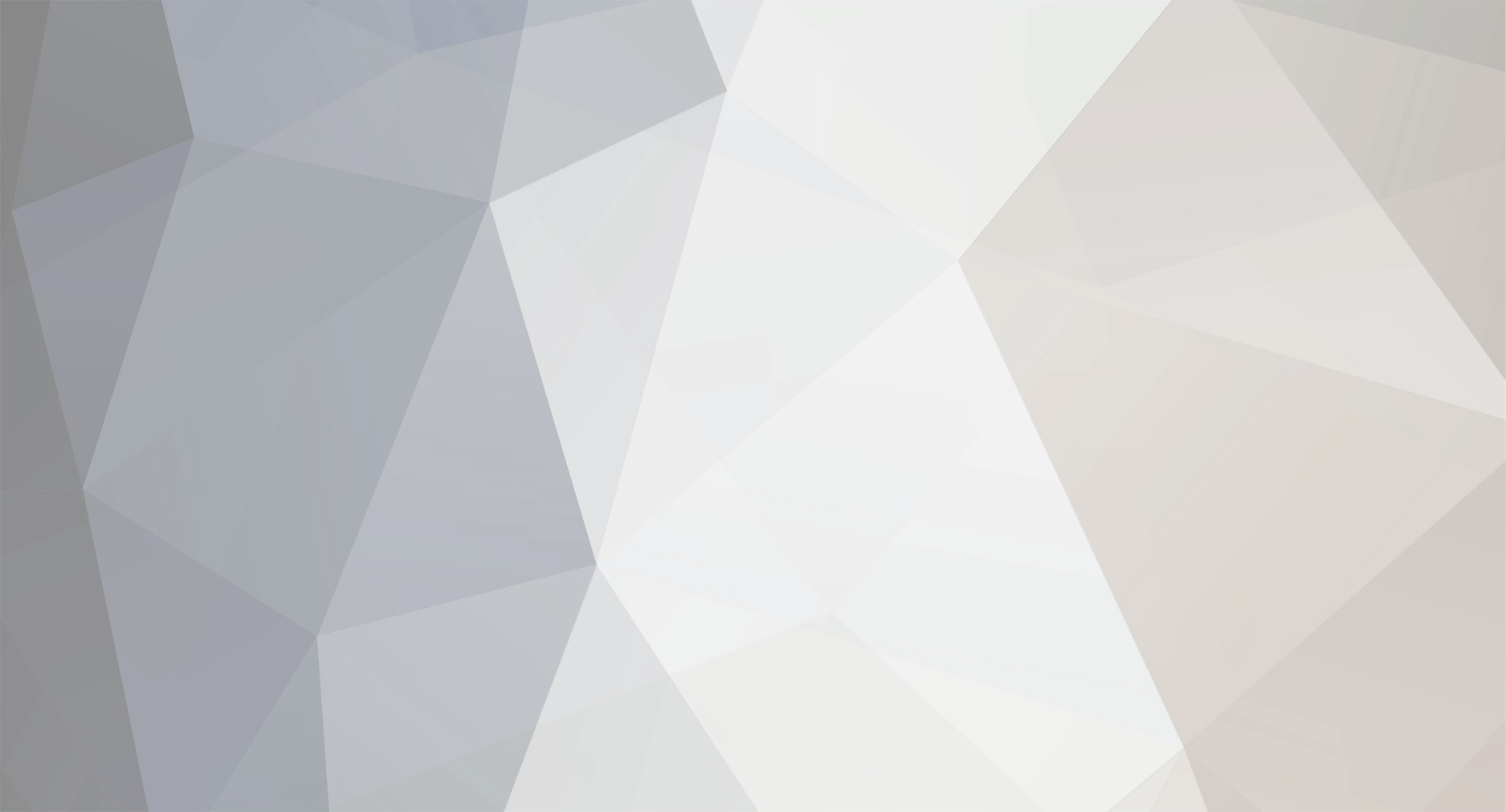
Lellson
Members-
Posts
16 -
Joined
-
Last visited
Everything posted by Lellson
-
It's a custom block. To be exactly an Ore which should look like stone if the player doesn't wear a custom helmet.
-
Hello, I'm trying to change the texture of a block in game (only if the player wears a special helmet). I only need this on the client side, because other players (without the helmet) should see the block like normal. How would I achieve something like this? Are there any events I could use? Thanks!
-
The client part works well for me. I simply did this in my server proxy: ((EntityPlayerMP) entity).interactionManager.setBlockReachDistance(range); I also had to use the PlayerInteractEvent.LeftClickEmpty event, send a packet and raytrace to attack entities from the new range.
-
Looks like a nice solution! I should probably have checked this topic before I tried something new... I actually fixed it a bit differently. The problem was the method getBlockReachDistance() in PlayerControllerMP, which always returned 4.5F or 5F depending on the player's gamemode. I overrid that method in a custom PlayerControllerMP class and set the playerController field from the minecraft instance to the custom one. Client Proxy: @Override public void setWeaponRange(Entity entity, double range) { super.setWeaponRange(entity, range); class RangePlayerController extends PlayerControllerMP { private float range = Constants.DEFAULT_RANGE; public RangePlayerController(Minecraft mcIn, NetHandlerPlayClient netHandler) { super(mcIn, netHandler); } @Override public float getBlockReachDistance() { return range; } public void setBlockReachDistance(float range) { this.range = range; } } Minecraft mc = Minecraft.getMinecraft(); EntityPlayer player = mc.thePlayer; if (player == entity) { if (!(mc.playerController instanceof RangePlayerController)) { GameType gameType = ReflectionHelper.getPrivateValue(PlayerControllerMP.class, mc.playerController, "currentGameType", "field_78779_k"); NetHandlerPlayClient netClient = ReflectionHelper.getPrivateValue(PlayerControllerMP.class, mc.playerController, "connection", "field_78774_b"); RangePlayerController controller = new RangePlayerController(mc, netClient); boolean isFlying = player.capabilities.isFlying; boolean allowFlying = player.capabilities.allowFlying; controller.setGameType(gameType); player.capabilities.isFlying = isFlying; player.capabilities.allowFlying = allowFlying; mc.playerController = controller; } ((RangePlayerController) mc.playerController).setBlockReachDistance((float) range); } } Thanks anyway!
-
Hey! I'm trying to increase the player's block reach distance but it doesn't seem to work. This is my code: @Override public void onUpdate(ItemStack stack, World world, Entity entity, int itemSlot, boolean isSelected) { super.onUpdate(stack, world, entity, itemSlot, isSelected); if (!(entity instanceof EntityPlayerMP)) return; if (isSelected) { ((EntityPlayerMP) entity).interactionManager.setBlockReachDistance(20.0D); } else { ((EntityPlayerMP) entity).interactionManager.setBlockReachDistance(5.0D); } } The setters only run on the server side. Could that be the problem? Has someone a proper solution for this? Thanks!
-
Hi! I made a custom Model which extends ModelBiped and I use it as armor model at the moment. I use the method getArmorModel in ItemArmor for this. My problem is that I have a custom slot for my armor item (backpack) and I want to render the model on the player if the item is detected in that slot and not in the chestplate slot. I already tried the event RenderPlayerEvent.SetArmorModel but it is deprecated and I don't really know how to use it. Are there any good ways to do this?
-
Hello! I'm working on updating my mod (1.7.10 to 1.9). My problem is, that I don't know how to attach a custom Model (which extends ModelBase) to an item. In 1.7, I did something like that: MinecraftForgeClient.registerItemRenderer(AstralItems.spellBook, new Astral3DItemBooks(new ModelSpellBook(), "spellBook.png")); I had a custom class (Astral3DItemBooks), which implemented IItemRenderer and I just passed the Model and String (Texture Path) to render the Item. But the interface IItemRenderer and the method registerItemRenderer doesn't exists anymore... Does anyone know a good tutorial or a solution for this?
-
I know this post is old, but the problem is still a thing. I had the same issue. It's because Minecraft can only handle 4000 particles at once. If there are more than 4000, the game crashes.
-
Hey! Is there any way to save the enchantments from an ItemStack / input of a recipe and add them to the output of a recipe? I already tried this, but hadn't any success. public static void craftSword(ItemStack output, ItemStack mat, ItemStack tool) { Map enchants = EnchantmentHelper.getEnchantments(tool); EnchantmentHelper.setEnchantments(enchants, output); GameRegistry.addShapedRecipe(output, new Object[]{"M","M","T",'M', mat, 'T', tool}); } craftSword(new ItemStack(Items.stone_sword), stonePlate, new ItemStack(Items.wooden_sword)); Thanks for help!
-
[1.7.10] Need help with disappearing XP-levels and Durability.
Lellson replied to Lellson's topic in Modder Support
Thanks! I'll have to try that. -
Hey! I'm pretty new into modding, so this is probably a easy problem... I made an item, which can store players experience levels, but I have a problem. I made two variants of using this item to store and withdraw xp. The first one is made with onItemRightClick and the second one is made with onUpdate and a custom key. The first one is working nicely, but if I use the second one, the xp and the durability always reset to their normal value, if i receive more xp or after a certain time. Here is my code: package lellson.survivalgear.items; import lellson.survivalgear.util.SurvivalKeys; import baubles.api.BaubleType; import baubles.api.IBauble; import net.minecraft.entity.Entity; import net.minecraft.entity.EntityLivingBase; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.item.Item; import net.minecraft.item.ItemStack; import net.minecraft.world.World; public class ItemXpHolder extends Item { public ItemXpHolder() { this.setMaxDamage(50); this.maxStackSize = 1; } // First variant. works fine! @Override public ItemStack onItemRightClick(ItemStack item, World world, EntityPlayer player) { if (!player.isSneaking()) { if (item.getItemDamage() > 0 && item.getItemDamage() <= 50 && ((EntityPlayer) player).experienceLevel > 0) { player.experienceLevel -= 1; item.damageItem(-1, player); } } if (player.isSneaking()) { if (item.getItemDamage() < 50) { player.experienceLevel += 1; item.damageItem(1, player); } } return item; } // Second variant. The xp and the durability always reset to their normal value @Override public void onUpdate(ItemStack item, World world, Entity player, int i, boolean b) { if (SurvivalKeys.survivalActivate.isPressed()) { if (!player.isSneaking()) { if (item.getItemDamage() > 0 && item.getItemDamage() <= 50 && ((EntityPlayer) player).experienceLevel > 0) { ((EntityPlayer) player).experienceLevel -= 1; item.damageItem(-1, (EntityLivingBase) player); } } if (player.isSneaking()) { if (item.getItemDamage() < 50) { ((EntityPlayer) player).experienceLevel += 1; item.damageItem(1, (EntityLivingBase) player); } } } } } Thanks for your help!