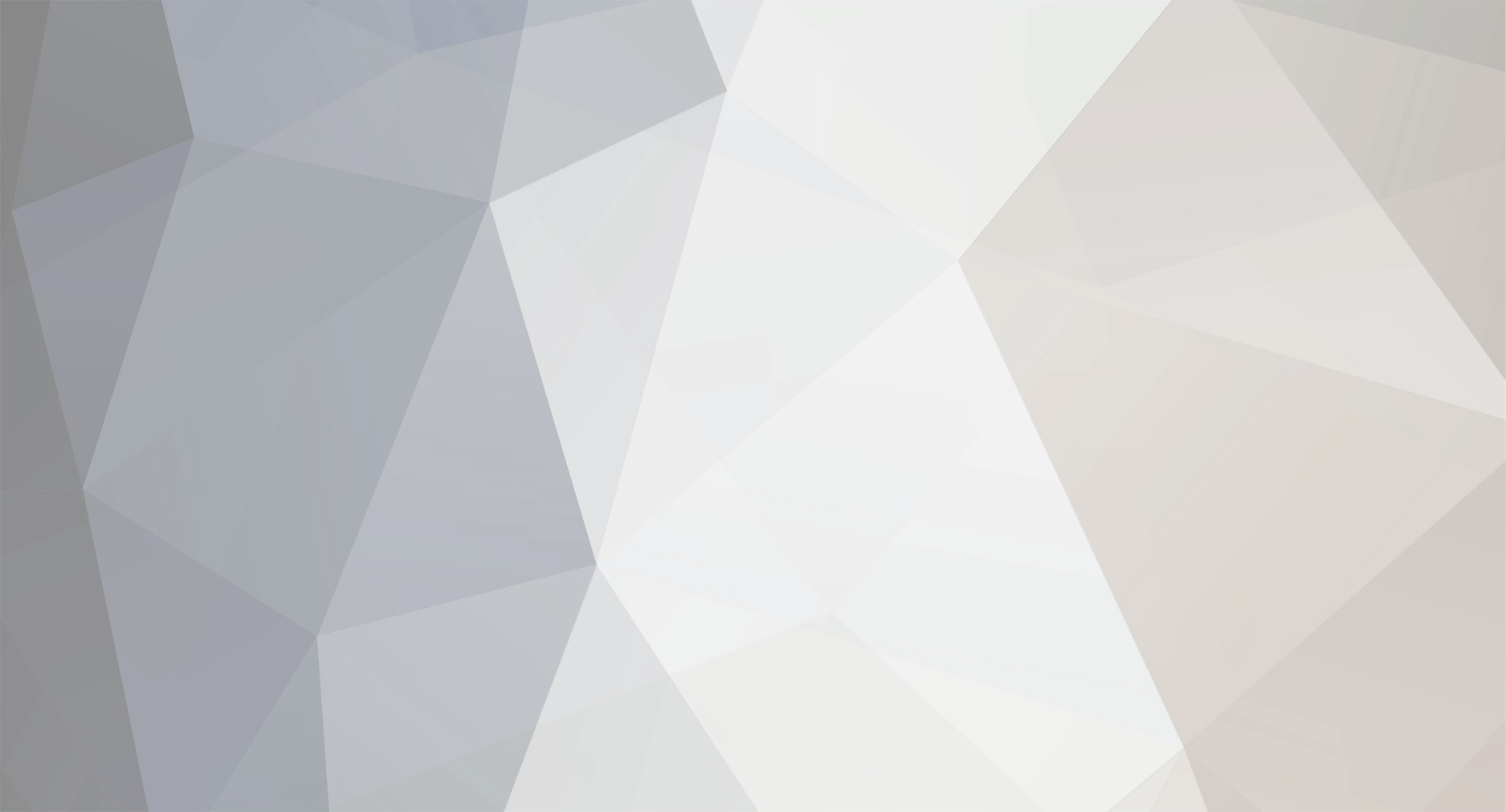
kirstym
Members-
Posts
11 -
Joined
-
Last visited
Converted
-
Gender
Undisclosed
-
Personal Text
I am new!
Recent Profile Visitors
The recent visitors block is disabled and is not being shown to other users.
kirstym's Achievements

Tree Puncher (2/8)
0
Reputation
-
This was the only thread I could find when I was seeking out how to quit the current world and load another world that is already saved, so I thought I'd post a solution for anyone in the future who might have the same goal... the following is for Forge 1.14.4 and can be called during an `onKeyInput` callback. It works for a local world (i.e. using an integrated server). // Disconnect from current world Minecraft.getInstance().world.sendQuittingDisconnectingPacket(); Minecraft.getInstance().func_213231_b(new DirtMessageScreen(new StringTextComponent("Reloading"))); // Connect to another with folder name "New World" and world name "New World" String folderName = "New World"; String worldName = "New World"; WorldSettings settings = null; Minecraft.getInstance().launchIntegratedServer(folderName, worldName, settings);
-
Thanks!
-
Okay, that makes sense, thanks. I certainly wouldn't expect vanilla Minecraft to know what to do, but I wasn't sure whether having Forge installed would be sufficient - mainly because I had some users report success playing on public servers which wouldn't have the mods installed on them. Trying to limit usage to vanilla packets sounds like a good idea - where would I look to see what packets are available?
-
TL;DR: Is it possible for client-side-only mods to send messages to a server using SimpleChannel.registerMessage? If so, what server config options might allow / disallow the messages? I'm getting reports of some servers working with my mods and others won't execute any of the messages. I maintain some mods to allow disabled gamers to play Minecraft using eye gaze. These mods don't add any content (new blocks etc), they just make changes to the controls and automate some things. I've set it up to be client-side only, using the pattern recommended in the docs, e.g.: @Mod(EyeGaze.MODID) public class EyeGaze { public EyeGaze() { // Only load things if on client - this means if mod is loaded on server, nothing happens. DistExecutor.runWhenOn(Dist.CLIENT, () -> () -> { // Register ourselves for server and other game events we are interested in MinecraftForge.EVENT_BUS.register(this); FMLJavaModLoadingContext.get().getModEventBus().addListener(this::setup); // Make sure the mod being absent on the other network side does not cause the client to display the server as incompatible ModLoadingContext.get().registerExtensionPoint(ExtensionPoint.DISPLAYTEST, () -> Pair.of(() -> FMLNetworkConstants.IGNORESERVERONLY, (a, b) -> true)); ... more setup ... } ... more stuff ... } Some of the mods do need to send messages to the network, for instance to place a block programmatically. I use SimpleChannel.registerMessage in these situations and everything works fine when testing locally, or with a LAN world. Users have reported that on some remote servers they can use the mod fine, but on other servers, any of the functionality that required message passing is failing. I don't know much about Minecraft server setup/admin, so I'm not sure what to suggest. What is the basic requirement to play on a server while using a client-side mod which requires message passing? I assume it needs the same version of Forge installed on the server, but doesn't require the same mods to be installed. Is this correct? Are there any other server config options that might change this behaviour? For example, allowing cheats? Are there any other variables that might affect whether or not my mods work with a given server?
-
[1.14.4] KeyBinding falsely reporting isPressed after using inventory
kirstym replied to kirstym's topic in Modder Support
@DragonITA sorry, I’m not sure what I’m supposed to be looking at - are you just showing an example of querying key state? Should I look in the whole repo or just the file you linked to? I gave a full mod in the spoiler in the first post. I just stripped it down so that it demonstrates the bug without any extra logic from my mods. It’s a fully functional mod class. -
[1.14.4] KeyBinding falsely reporting isPressed after using inventory
kirstym replied to kirstym's topic in Modder Support
To clarify: if my key binding is “P”, then when I press “P” with the inventory open, events immediately fire with the key code for “P”. This is expected. Then, I close the inventory and press “O” (another unrelated key). An event fires with the key code for “O”, but inside the event handler, the isPressed method for the “P” key binding wrongly returns true. -
[1.14.4] KeyBinding falsely reporting isPressed after using inventory
kirstym replied to kirstym's topic in Modder Support
I actually do usually use that myself to return early if a GUI is open - I just left it out to keep the examples really simple. Thanks for the suggestion though! This doesn’t change the behaviour I described, because the bug is happening in any Key event shortly after closing the gui. -
I've been using the following pattern to react to key presses in my mods: @SubscribeEvent public void onKeyInput(KeyInputEvent event) { if (myKeyBinding.isPressed()) { LOGGER.debug("key has been pressed!"); // Do stuff here } } This generally works as expected - isPressed() returns true on the initial event (where event.action = GLFW_PRESS). The docstring for isPressed() says "Returns true on the initial key press. For continuous querying use {@link isKeyDown()}. Should be used in key events." which makes me think it's a reasonable approach. However, I've noticed that some of my key events were being unexpectedly fired upon closing an inventory GUI. I've stripped it down to a simple example, and what I'm seeing is that if the key in question was pressed at any point while the inventory was open, then isPressed() continues to report true for a short time after. It's not firing a new event for the key in question, but it means my mod code gets triggered for any other key event (including the GLFW_RELEASE event of the Escape key from closing the inventory). This happens on both creative and survival inventories, but not the IngameMenuScreen or AdvancementsScreen. This standalone example that shows I'm not doing anything funny elsewhere in my code: Is this expected behaviour or a bug in the KeyBinding code? Is it fundamentally a broken pattern? From looking at the KeyBinding source, I don't really get the logic behind isPressed. I'm quite happy to bin this pattern and revert to the following one, which always works - but if it's a bug I wanted to document it somewhere, or at least save someone else the debugging time! @SubscribeEvent public void onKeyInput(KeyInputEvent event) { if (event.getKey() == myKeyBinding.getKey().getKeyCode() && event.getAction() == GLFW.GLFW_PRESS) { // do stuff } }
-
Hello, I'm trying to get the CraftingKeys mod working in combination with a eye tracking keyboard to make crafting more accessible to people unable to use a keyboard/mouse. One classic problem with alternative keyboard inputs is that they tend to give instantaneous key press events, rather than slow finger presses - this means that you almost never detect the key events when polling isKeyDown(). What's the best approach to detecting key events while the inventory is open? The mod's onKeyInput() method only gets called in-game (not when the inventory is open). I've tried iterating over the whole event queue, which does successfully find the events I care about, but it also consumes any other events, so that other keys don't work (such as pressing Esc to close the inventory). Is there some way I can subscribe to key events within the vanilla inventory GUI? Or should I be re-publishing events that I consume but don't care about? The latter seems a bit low-level to me. Any pointers would be greatly appreciated, Kirsty
-
Manually placing block with forge 1.8 and/or 1.8.8
kirstym replied to kirstym's topic in Modder Support
Thanks Choonster, that's really helpful. I'll take a look at diesieben07's tutorial. -
I'm producing a few very simple mods to help users who can only play minecraft via an eye-controlled keyboard. Right now I'm trying to add an action that will be equivalent to jumping and placing a block underneath you, but with a single key event. The issue I'm having is how to programmatically add a block to the world, or in particular how to make the newly-placed block persistent. I've found two ways to programmatically add a (vanilla) block to the world: world.setBlockState(...) onItemUse(...) In both cases, the block initially seems to be placed fine. However, as soon as you save/quit the game and restart, they have vanished. Also, after placing a block automatically, trying to place a block manually (with right click) against your new block will result in replacing it rather than adding it to the side. Is there something I'm supposed to be doing to make the new block persistent? I've tried: - Varying the EnumFacing passed to onItemUse(...). - Calling world.markBlockForUpdate(blockPos) after creating the block - Comparing the state of a manually placed and a programmatically placed block as given by world.getBlockState(blockPos). Nothing is obviously different. Note that I'm using a local world, and I don't necessarily need this to work on a remote server right now (though it would be good to be aware of any limitations in that regard). Many thanks,