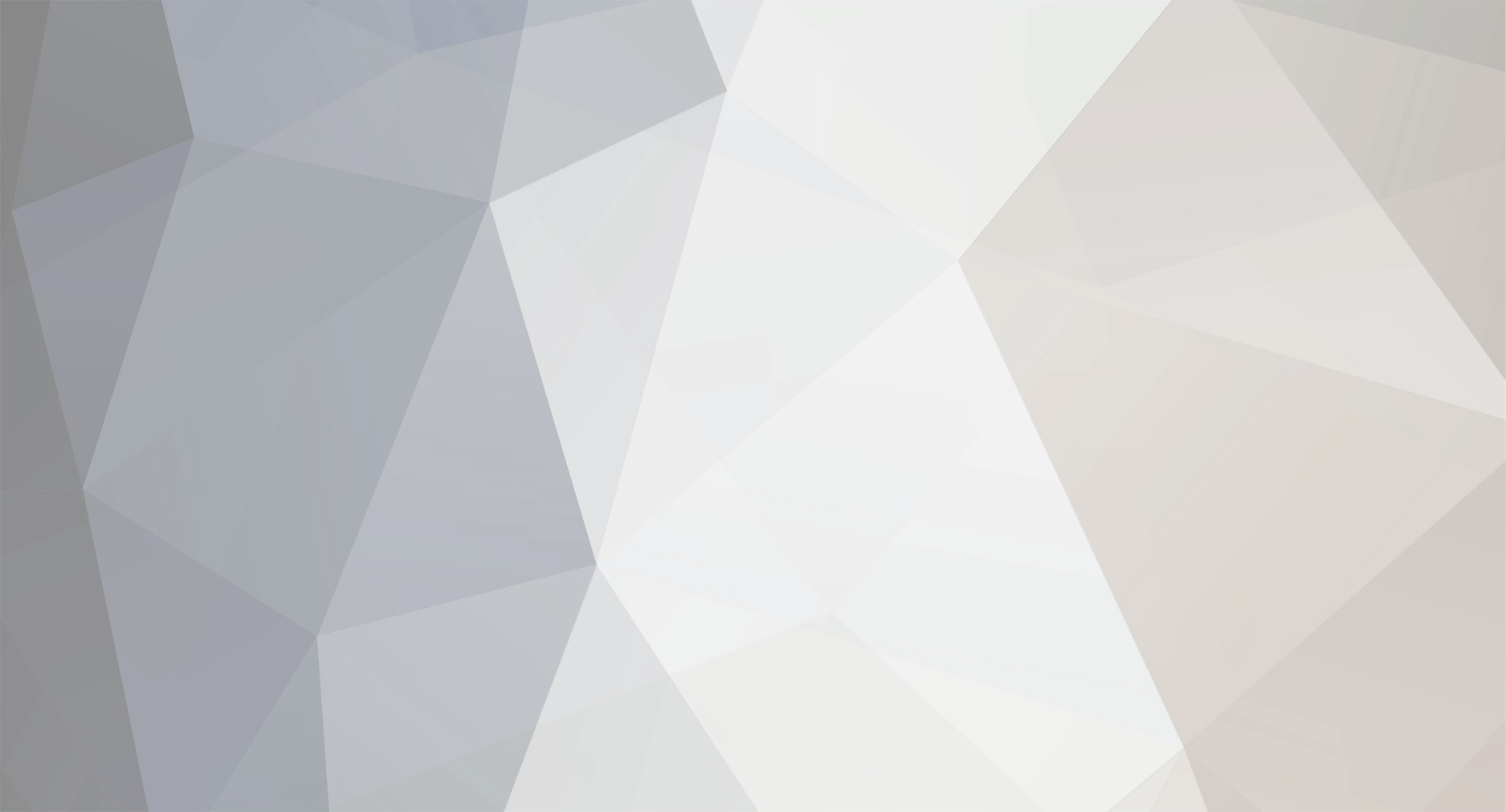
jameshyland
Members-
Posts
16 -
Joined
-
Last visited
Everything posted by jameshyland
-
This is my second time modding, and I am new to java so please cut me some slack. I am trying to create an item that give the player an item when a specific block is right clicked. The problem is, when I run the game, nothing happens, it doesnt even call onUpdate, onItemUse or onItemRightClick! Please help! Here is my main mod class package com.MoneyMod; import net.minecraft.item.Item.ToolMaterial; import net.minecraftforge.common.util.EnumHelper; import net.minecraftforge.fml.common.Mod; import net.minecraftforge.fml.common.Mod.EventHandler; import net.minecraftforge.fml.common.SidedProxy; import net.minecraftforge.fml.common.event.FMLInitializationEvent; import net.minecraftforge.fml.common.event.FMLPostInitializationEvent; import net.minecraftforge.fml.common.event.FMLPreInitializationEvent; import com.MoneyMod.init.MoneyModBlocks; import com.MoneyMod.init.MoneyModItems; import com.MoneyMod.proxy.CommonProxy; @Mod(modid = Reference.MOD_ID, name = Reference.MOD_NAME, version = Reference.VERSION) public class MoneyMod { @SidedProxy(clientSide = Reference.CLIENT_PROXY_CLASS, serverSide = Reference.SERVER_PROXY_CLASS) public static CommonProxy proxy; public static final MoneyModTab tabCreative = new MoneyModTab("tabCreative"); @EventHandler public void preInit(FMLPreInitializationEvent event) { MoneyModBlocks.init(); MoneyModBlocks.register(); MoneyModItems.init(); MoneyModItems.register(); //MoneyModItems.registerRenders(); } @EventHandler public void init (FMLInitializationEvent event) { { proxy.registerRenders(); } } @EventHandler public void postInit(FMLPostInitializationEvent event) { } Item declaration class: package com.MoneyMod.init; import net.minecraft.block.Block; import net.minecraft.client.Minecraft; import net.minecraft.client.resources.model.ModelResourceLocation; import net.minecraft.item.Item; import net.minecraft.item.Item.ToolMaterial; import net.minecraftforge.common.util.EnumHelper; import net.minecraftforge.fml.common.registry.GameRegistry; import com.MoneyMod.MoneyMod; import com.MoneyMod.Reference; public class MoneyModItems { public static Item itemPoundCoin; public static Item itemMoneyDust; public static Item itemTwoPoundCoin; public static Item itemFivePoundNote; public static Item itemTenPoundNote; public static Item itemTwentyPoundNote; public static Block blockFiverBlock; public static Item MoneyPickaxe; public static Item itemMoneyPutty; public static Item itemMoneyIngot; public static Item itemMoneyWand; public static final Item.ToolMaterial MoneyToolMaterial = EnumHelper.addToolMaterial("MoneyToolMaterial", 2, 1250 , 8.0F, 5.0F, 10); public static void init() { System.out.println("setting up item"); itemPoundCoin = new Item().setUnlocalizedName("itemPoundCoin").setCreativeTab(MoneyMod.tabCreative); itemMoneyIngot = new Item().setUnlocalizedName("ItemMoneyIngot").setCreativeTab(MoneyMod.tabCreative); itemTwentyPoundNote = new Item().setUnlocalizedName("ItemTwentyPoundNote").setCreativeTab(MoneyMod.tabCreative); itemMoneyPutty = new Item().setUnlocalizedName("ItemMoneyPutty").setCreativeTab(MoneyMod.tabCreative); itemTwoPoundCoin = new Item().setUnlocalizedName("ItemTwoPoundCoin").setCreativeTab(MoneyMod.tabCreative); itemFivePoundNote = new Item().setUnlocalizedName("ItemFivePoundNote").setCreativeTab(MoneyMod.tabCreative); itemTenPoundNote = new Item().setUnlocalizedName("ItemTenPoundNote").setCreativeTab(MoneyMod.tabCreative); itemMoneyDust = new Item().setUnlocalizedName("ItemMoneyDust").setCreativeTab(MoneyMod.tabCreative); itemMoneyWand = new Item().setUnlocalizedName("ItemMoneyWand").setCreativeTab(MoneyMod.tabCreative); } public static void register() { GameRegistry.registerItem(itemPoundCoin, itemPoundCoin.getUnlocalizedName().substring(5)); GameRegistry.registerItem(itemMoneyIngot, itemMoneyIngot.getUnlocalizedName().substring(5)); GameRegistry.registerItem(itemTwentyPoundNote, itemTwentyPoundNote.getUnlocalizedName().substring(5)); GameRegistry.registerItem(itemMoneyPutty, itemMoneyPutty.getUnlocalizedName().substring(5)); GameRegistry.registerItem(itemFivePoundNote, itemFivePoundNote.getUnlocalizedName().substring(5)); GameRegistry.registerItem(itemTenPoundNote, itemTenPoundNote.getUnlocalizedName().substring(5)); GameRegistry.registerItem(itemTwoPoundCoin, itemTwoPoundCoin.getUnlocalizedName().substring(5)); GameRegistry.registerItem(itemMoneyWand, itemMoneyWand.getUnlocalizedName().substring(5)); GameRegistry.registerItem(itemMoneyDust, itemMoneyDust.getUnlocalizedName().substring(5)); } public static void registerRenders() { registerRender(itemPoundCoin); registerRender(itemMoneyIngot); registerRender(itemTwentyPoundNote); registerRender(itemMoneyPutty); registerRender(itemFivePoundNote); registerRender(itemTwoPoundCoin); registerRender(itemMoneyDust); registerRender(itemTenPoundNote); registerRender(itemMoneyWand); } public static void registerRender(Item item) { Minecraft.getMinecraft().getRenderItem().getItemModelMesher().register(item,0,new ModelResourceLocation(Reference.MOD_ID + ":"+ item.getUnlocalizedName().substring(5),"inventory")); } } and the item in question: package com.MoneyMod.items; import net.minecraft.entity.Entity; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.item.Item; import net.minecraft.item.ItemStack; import net.minecraft.nbt.NBTTagCompound; import net.minecraft.world.World; import com.MoneyMod.init.MoneyModItems; public class ItemMoneyWand extends Item { @Override public void onUpdate(ItemStack itemstack, World world, Entity entity, int metadata, boolean bool) { if (itemstack.getTagCompound() == null) { ItemStack s = new ItemStack(MoneyModItems.itemMoneyDust); itemstack.setTagCompound(new NBTTagCompound()); //or itemstack.setTagCompound(new NBTTagCompound()); } } public ItemStack onItemUse(ItemStack itemstack, World world, EntityPlayer player) { itemstack.getTagCompound().setInteger( "Coins", 100); ItemStack s = new ItemStack(MoneyModItems.itemMoneyDust); return itemstack; } public void onItemRightClick(ItemStack itemstack, World world, EntityPlayer playerIn, int metadata, boolean bool) { if (itemstack.getTagCompound() .getInteger("Coins") >= 100) { ItemStack s = new ItemStack(MoneyModItems.itemMoneyDust); playerIn.inventory.addItemStackToInventory(s); } } } //@Override /*public ItemStack onItemRightClick1(ItemStack itemStackIn, World worldIn, EntityPlayer playerIn) if(worldIn.isRemote); System.out.println("james smells"); ItemStack s = new ItemStack(MoneyModItems.itemMoneyDust); playerIn.inventory.addItemStackToInventory(s); return itemStackIn; } } */
-
[1.8] [UNSOLVED] Need some help with Item properties.
jameshyland replied to jameshyland's topic in Modder Support
Bumping. Still haven't sorted -
[1.8] [UNSOLVED] Need some help with Item properties.
jameshyland replied to jameshyland's topic in Modder Support
Thanks for your suggestions, I will spend some time trying to understand what you have written. Once again, thanks for your help. -
[1.8] [UNSOLVED] Need some help with Item properties.
jameshyland replied to jameshyland's topic in Modder Support
Thanks for you reply! As I said in my post, I really dont know java that well and this is my first mod. I appreciate your help, but if you could edit my code with your suggestions, it would greatly benefit me. As a thank you (if you do so), I will credit you as a mod author and another Thanks on your account. Thanks again. -
I am making an item that should give a specific item when blocks are clicked, however I cannot seem to get it to work. I havent actually ran the code to test it, only because I do not know the code. I am a beginner coder and so I would like some help. This is my code so far. package com.MoneyMod.items; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.item.Item; import net.minecraft.item.ItemStack; import net.minecraft.nbt.NBTTagCompound; import net.minecraft.world.World; import com.MoneyMod.init.MoneyModItems; public class ItemMoneyWand extends Item { public ItemStack onItemRightClick(ItemStack itemStackIn, World worldIn, EntityPlayer playerIn) { if(worldIn.isRemote); System.out.println("james smells"); ItemStack s = new ItemStack(MoneyModItems.itemMoneyDust); playerIn.inventory.addItemStackToInventory(s); return itemStackIn; } } {code}
-
[1.7.10] Crafting Recipe Crashes Game on startup
jameshyland replied to jameshyland's topic in Modder Support
Thanks all for the replies. The crafting recipe that doesnt work is GameRegistry.addRecipe(new ItemStack(MoneyMod.itemFivePoundNote), new Object[]{"STS", " " O " , "STS"}, 'T', itemTwoPoundCoin, 'O', itemPoundCoin, 'S', Items.string); and crashes with the same [18:19:43] [main/INFO] [GradleStart]: Extra: [] [18:19:43] [main/INFO] [GradleStart]: Running with arguments: [--userProperties, {}, --assetsDir, C:/Users/James/.gradle/caches/minecraft/assets, --assetIndex, 1.7.10, --accessToken, {REDACTED}, --version, 1.7.10, --tweakClass, cpw.mods.fml.common.launcher.FMLTweaker, --tweakClass, net.minecraftforge.gradle.tweakers.CoremodTweaker] [18:19:43] [main/INFO] [LaunchWrapper]: Loading tweak class name cpw.mods.fml.common.launcher.FMLTweaker [18:19:43] [main/INFO] [LaunchWrapper]: Using primary tweak class name cpw.mods.fml.common.launcher.FMLTweaker [18:19:43] [main/INFO] [LaunchWrapper]: Loading tweak class name net.minecraftforge.gradle.tweakers.CoremodTweaker [18:19:43] [main/INFO] [LaunchWrapper]: Calling tweak class cpw.mods.fml.common.launcher.FMLTweaker [18:19:43] [main/INFO] [FML]: Forge Mod Loader version 7.99.36.1558 for Minecraft 1.7.10 loading [18:19:43] [main/INFO] [FML]: Java is Java HotSpot(TM) 64-Bit Server VM, version 1.8.0_65, running on Windows 8.1:amd64:6.3, installed at C:\Program Files\Java\jdk1.8.0_65\jre [18:19:43] [main/INFO] [FML]: Managed to load a deobfuscated Minecraft name- we are in a deobfuscated environment. Skipping runtime deobfuscation [18:19:43] [main/INFO] [LaunchWrapper]: Calling tweak class net.minecraftforge.gradle.tweakers.CoremodTweaker [18:19:43] [main/INFO] [GradleStart]: Injecting location in coremod cpw.mods.fml.relauncher.FMLCorePlugin [18:19:43] [main/INFO] [GradleStart]: Injecting location in coremod net.minecraftforge.classloading.FMLForgePlugin [18:19:43] [main/INFO] [LaunchWrapper]: Loading tweak class name cpw.mods.fml.common.launcher.FMLInjectionAndSortingTweaker [18:19:43] [main/INFO] [LaunchWrapper]: Loading tweak class name cpw.mods.fml.common.launcher.FMLDeobfTweaker [18:19:43] [main/INFO] [LaunchWrapper]: Loading tweak class name net.minecraftforge.gradle.tweakers.AccessTransformerTweaker [18:19:43] [main/INFO] [LaunchWrapper]: Calling tweak class cpw.mods.fml.common.launcher.FMLInjectionAndSortingTweaker [18:19:43] [main/INFO] [LaunchWrapper]: Calling tweak class cpw.mods.fml.common.launcher.FMLInjectionAndSortingTweaker [18:19:43] [main/INFO] [LaunchWrapper]: Calling tweak class cpw.mods.fml.relauncher.CoreModManager$FMLPluginWrapper [18:19:43] [main/ERROR] [FML]: The binary patch set is missing. Either you are in a development environment, or things are not going to work! [18:19:44] [main/ERROR] [FML]: FML appears to be missing any signature data. This is not a good thing [18:19:44] [main/INFO] [LaunchWrapper]: Calling tweak class cpw.mods.fml.relauncher.CoreModManager$FMLPluginWrapper [18:19:44] [main/INFO] [LaunchWrapper]: Calling tweak class cpw.mods.fml.common.launcher.FMLDeobfTweaker [18:19:45] [main/INFO] [LaunchWrapper]: Calling tweak class net.minecraftforge.gradle.tweakers.AccessTransformerTweaker [18:19:45] [main/INFO] [LaunchWrapper]: Loading tweak class name cpw.mods.fml.common.launcher.TerminalTweaker [18:19:45] [main/INFO] [LaunchWrapper]: Calling tweak class cpw.mods.fml.common.launcher.TerminalTweaker [18:19:45] [main/INFO] [LaunchWrapper]: Launching wrapped minecraft {net.minecraft.client.main.Main} [18:19:46] [main/INFO]: Setting user: Player436 [18:19:48] [Client thread/INFO]: LWJGL Version: 2.9.1 [18:19:49] [Client thread/INFO] [sTDOUT]: [cpw.mods.fml.client.SplashProgress:start:188]: ---- Minecraft Crash Report ---- // Don't be sad, have a hug! <3 Time: 10/12/15 18:19 Description: Loading screen debug info This is just a prompt for computer specs to be printed. THIS IS NOT A ERROR A detailed walkthrough of the error, its code path and all known details is as follows: --------------------------------------------------------------------------------------- -- System Details -- Details: Minecraft Version: 1.7.10 Operating System: Windows 8.1 (amd64) version 6.3 Java Version: 1.8.0_65, Oracle Corporation Java VM Version: Java HotSpot(TM) 64-Bit Server VM (mixed mode), Oracle Corporation Memory: 792943328 bytes (756 MB) / 1038876672 bytes (990 MB) up to 1038876672 bytes (990 MB) JVM Flags: 3 total; -Xincgc -Xmx1024M -Xms1024M AABB Pool Size: 0 (0 bytes; 0 MB) allocated, 0 (0 bytes; 0 MB) used IntCache: cache: 0, tcache: 0, allocated: 0, tallocated: 0 FML: GL info: ' Vendor: 'NVIDIA Corporation' Version: '4.5.0 NVIDIA 347.25' Renderer: 'GeForce GTX 960/PCIe/SSE2' [18:19:49] [Client thread/INFO] [MinecraftForge]: Attempting early MinecraftForge initialization [18:19:49] [Client thread/INFO] [FML]: MinecraftForge v10.13.4.1558 Initialized [18:19:49] [Client thread/INFO] [FML]: Replaced 183 ore recipies [18:19:49] [Client thread/INFO] [MinecraftForge]: Completed early MinecraftForge initialization [18:19:50] [Client thread/INFO] [FML]: Found 0 mods from the command line. Injecting into mod discoverer [18:19:50] [Client thread/INFO] [FML]: Searching C:\Users\James\Desktop\Minecraft Mod\eclipse\mods for mods [18:19:55] [Client thread/INFO] [FML]: Forge Mod Loader has identified 4 mods to load [18:19:56] [Client thread/INFO] [FML]: Attempting connection with missing mods [mcp, FML, Forge, MnyMod] at CLIENT [18:19:56] [Client thread/INFO] [FML]: Attempting connection with missing mods [mcp, FML, Forge, MnyMod] at SERVER [18:19:56] [Client thread/INFO]: Reloading ResourceManager: Default, FMLFileResourcePack:Forge Mod Loader, FMLFileResourcePack:Minecraft Forge, FMLFileResourcePack:Money Mods [18:19:56] [Client thread/INFO] [FML]: Processing ObjectHolder annotations [18:19:56] [Client thread/INFO] [FML]: Found 341 ObjectHolder annotations [18:19:56] [Client thread/INFO] [FML]: Identifying ItemStackHolder annotations [18:19:56] [Client thread/INFO] [FML]: Found 0 ItemStackHolder annotations [18:19:56] [Client thread/INFO] [FML]: Configured a dormant chunk cache size of 0 [18:19:56] [Client thread/INFO] [FML]: Applying holder lookups [18:19:56] [Client thread/INFO] [FML]: Holder lookups applied [18:19:56] [Client thread/INFO] [FML]: Injecting itemstacks [18:19:56] [Client thread/INFO] [FML]: Itemstack injection complete [18:19:56] [sound Library Loader/INFO] [sTDOUT]: [paulscode.sound.SoundSystemLogger:message:69]: [18:19:56] [sound Library Loader/INFO] [sTDOUT]: [paulscode.sound.SoundSystemLogger:message:69]: Starting up SoundSystem... [18:19:56] [Thread-8/INFO] [sTDOUT]: [paulscode.sound.SoundSystemLogger:message:69]: Initializing LWJGL OpenAL [18:19:56] [Thread-8/INFO] [sTDOUT]: [paulscode.sound.SoundSystemLogger:message:69]: (The LWJGL binding of OpenAL. For more information, see http://www.lwjgl.org) [18:19:56] [Thread-8/INFO] [sTDOUT]: [paulscode.sound.SoundSystemLogger:message:69]: OpenAL initialized. [18:19:57] [sound Library Loader/INFO] [sTDOUT]: [paulscode.sound.SoundSystemLogger:message:69]: [18:19:57] [sound Library Loader/INFO]: Sound engine started [18:19:57] [Client thread/INFO]: Created: 16x16 textures/blocks-atlas [18:19:57] [Client thread/INFO]: Created: 16x16 textures/items-atlas [18:19:57] [Client thread/ERROR] [FML]: Fatal errors were detected during the transition from INITIALIZATION to POSTINITIALIZATION. Loading cannot continue [18:19:57] [Client thread/ERROR] [FML]: States: 'U' = Unloaded 'L' = Loaded 'C' = Constructed 'H' = Pre-initialized 'I' = Initialized 'J' = Post-initialized 'A' = Available 'D' = Disabled 'E' = Errored UCHI mcp{9.05} [Minecraft Coder Pack] (minecraft.jar) UCHI FML{7.10.99.99} [Forge Mod Loader] (forgeSrc-1.7.10-10.13.4.1558-1.7.10.jar) UCHI Forge{10.13.4.1558} [Minecraft Forge] (forgeSrc-1.7.10-10.13.4.1558-1.7.10.jar) UCHE MnyMod{1.0} [Money Mods] (bin) [18:19:57] [Client thread/ERROR] [FML]: The following problems were captured during this phase [18:19:57] [Client thread/ERROR] [FML]: Caught exception from MnyMod java.lang.ClassCastException: [Ljava.lang.Object; cannot be cast to java.lang.Character at net.minecraft.item.crafting.CraftingManager.addRecipe(CraftingManager.java:209) ~[forgeSrc-1.7.10-10.13.4.1558-1.7.10.jar:?] at cpw.mods.fml.common.registry.GameRegistry.addShapedRecipe(GameRegistry.java:250) ~[forgeSrc-1.7.10-10.13.4.1558-1.7.10.jar:?] at cpw.mods.fml.common.registry.GameRegistry.addRecipe(GameRegistry.java:245) ~[forgeSrc-1.7.10-10.13.4.1558-1.7.10.jar:?] at com.jameshyland.MoneyMod.MoneyMod.init(MoneyMod.java:95) ~[bin/:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_65] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_65] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_65] at java.lang.reflect.Method.invoke(Method.java:497) ~[?:1.8.0_65] at cpw.mods.fml.common.FMLModContainer.handleModStateEvent(FMLModContainer.java:532) ~[forgeSrc-1.7.10-10.13.4.1558-1.7.10.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_65] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_65] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_65] at java.lang.reflect.Method.invoke(Method.java:497) ~[?:1.8.0_65] at com.google.common.eventbus.EventSubscriber.handleEvent(EventSubscriber.java:74) ~[guava-17.0.jar:?] at com.google.common.eventbus.SynchronizedEventSubscriber.handleEvent(SynchronizedEventSubscriber.java:47) ~[guava-17.0.jar:?] at com.google.common.eventbus.EventBus.dispatch(EventBus.java:322) ~[guava-17.0.jar:?] at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:304) ~[guava-17.0.jar:?] at com.google.common.eventbus.EventBus.post(EventBus.java:275) ~[guava-17.0.jar:?] at cpw.mods.fml.common.LoadController.sendEventToModContainer(LoadController.java:212) ~[forgeSrc-1.7.10-10.13.4.1558-1.7.10.jar:?] at cpw.mods.fml.common.LoadController.propogateStateMessage(LoadController.java:190) ~[forgeSrc-1.7.10-10.13.4.1558-1.7.10.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_65] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_65] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_65] at java.lang.reflect.Method.invoke(Method.java:497) ~[?:1.8.0_65] at com.google.common.eventbus.EventSubscriber.handleEvent(EventSubscriber.java:74) ~[guava-17.0.jar:?] at com.google.common.eventbus.SynchronizedEventSubscriber.handleEvent(SynchronizedEventSubscriber.java:47) ~[guava-17.0.jar:?] at com.google.common.eventbus.EventBus.dispatch(EventBus.java:322) ~[guava-17.0.jar:?] at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:304) ~[guava-17.0.jar:?] at com.google.common.eventbus.EventBus.post(EventBus.java:275) ~[guava-17.0.jar:?] at cpw.mods.fml.common.LoadController.distributeStateMessage(LoadController.java:119) [LoadController.class:?] at cpw.mods.fml.common.Loader.initializeMods(Loader.java:737) [Loader.class:?] at cpw.mods.fml.client.FMLClientHandler.finishMinecraftLoading(FMLClientHandler.java:311) [FMLClientHandler.class:?] at net.minecraft.client.Minecraft.startGame(Minecraft.java:597) [Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:942) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:164) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_65] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_65] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_65] at java.lang.reflect.Method.invoke(Method.java:497) ~[?:1.8.0_65] at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) [launchwrapper-1.12.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.12.jar:?] at net.minecraftforge.gradle.GradleStartCommon.launch(Unknown Source) [start/:?] at GradleStart.main(Unknown Source) [start/:?] [18:19:57] [Client thread/INFO] [sTDOUT]: [net.minecraft.client.Minecraft:displayCrashReport:388]: ---- Minecraft Crash Report ---- // I just don't know what went wrong Time: 10/12/15 18:19 Description: Initializing game java.lang.ClassCastException: [Ljava.lang.Object; cannot be cast to java.lang.Character at net.minecraft.item.crafting.CraftingManager.addRecipe(CraftingManager.java:209) at cpw.mods.fml.common.registry.GameRegistry.addShapedRecipe(GameRegistry.java:250) at cpw.mods.fml.common.registry.GameRegistry.addRecipe(GameRegistry.java:245) at com.jameshyland.MoneyMod.MoneyMod.init(MoneyMod.java:95) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:497) at cpw.mods.fml.common.FMLModContainer.handleModStateEvent(FMLModContainer.java:532) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:497) at com.google.common.eventbus.EventSubscriber.handleEvent(EventSubscriber.java:74) at com.google.common.eventbus.SynchronizedEventSubscriber.handleEvent(SynchronizedEventSubscriber.java:47) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:322) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:304) at com.google.common.eventbus.EventBus.post(EventBus.java:275) at cpw.mods.fml.common.LoadController.sendEventToModContainer(LoadController.java:212) at cpw.mods.fml.common.LoadController.propogateStateMessage(LoadController.java:190) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:497) at com.google.common.eventbus.EventSubscriber.handleEvent(EventSubscriber.java:74) at com.google.common.eventbus.SynchronizedEventSubscriber.handleEvent(SynchronizedEventSubscriber.java:47) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:322) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:304) at com.google.common.eventbus.EventBus.post(EventBus.java:275) at cpw.mods.fml.common.LoadController.distributeStateMessage(LoadController.java:119) at cpw.mods.fml.common.Loader.initializeMods(Loader.java:737) at cpw.mods.fml.client.FMLClientHandler.finishMinecraftLoading(FMLClientHandler.java:311) at net.minecraft.client.Minecraft.startGame(Minecraft.java:597) at net.minecraft.client.Minecraft.run(Minecraft.java:942) at net.minecraft.client.main.Main.main(Main.java:164) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:497) at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) at net.minecraft.launchwrapper.Launch.main(Launch.java:28) at net.minecraftforge.gradle.GradleStartCommon.launch(Unknown Source) at GradleStart.main(Unknown Source) A detailed walkthrough of the error, its code path and all known details is as follows: --------------------------------------------------------------------------------------- -- Head -- Stacktrace: at net.minecraft.item.crafting.CraftingManager.addRecipe(CraftingManager.java:209) at cpw.mods.fml.common.registry.GameRegistry.addShapedRecipe(GameRegistry.java:250) at cpw.mods.fml.common.registry.GameRegistry.addRecipe(GameRegistry.java:245) at com.jameshyland.MoneyMod.MoneyMod.init(MoneyMod.java:95) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:497) at cpw.mods.fml.common.FMLModContainer.handleModStateEvent(FMLModContainer.java:532) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:497) at com.google.common.eventbus.EventSubscriber.handleEvent(EventSubscriber.java:74) at com.google.common.eventbus.SynchronizedEventSubscriber.handleEvent(SynchronizedEventSubscriber.java:47) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:322) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:304) at com.google.common.eventbus.EventBus.post(EventBus.java:275) at cpw.mods.fml.common.LoadController.sendEventToModContainer(LoadController.java:212) at cpw.mods.fml.common.LoadController.propogateStateMessage(LoadController.java:190) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:497) at com.google.common.eventbus.EventSubscriber.handleEvent(EventSubscriber.java:74) at com.google.common.eventbus.SynchronizedEventSubscriber.handleEvent(SynchronizedEventSubscriber.java:47) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:322) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:304) at com.google.common.eventbus.EventBus.post(EventBus.java:275) at cpw.mods.fml.common.LoadController.distributeStateMessage(LoadController.java:119) at cpw.mods.fml.common.Loader.initializeMods(Loader.java:737) at cpw.mods.fml.client.FMLClientHandler.finishMinecraftLoading(FMLClientHandler.java:311) at net.minecraft.client.Minecraft.startGame(Minecraft.java:597) -- Initialization -- Details: Stacktrace: at net.minecraft.client.Minecraft.run(Minecraft.java:942) at net.minecraft.client.main.Main.main(Main.java:164) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:497) at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) at net.minecraft.launchwrapper.Launch.main(Launch.java:28) at net.minecraftforge.gradle.GradleStartCommon.launch(Unknown Source) at GradleStart.main(Unknown Source) -- System Details -- Details: Minecraft Version: 1.7.10 Operating System: Windows 8.1 (amd64) version 6.3 Java Version: 1.8.0_65, Oracle Corporation Java VM Version: Java HotSpot(TM) 64-Bit Server VM (mixed mode), Oracle Corporation Memory: 658047544 bytes (627 MB) / 1038876672 bytes (990 MB) up to 1038876672 bytes (990 MB) JVM Flags: 3 total; -Xincgc -Xmx1024M -Xms1024M AABB Pool Size: 0 (0 bytes; 0 MB) allocated, 0 (0 bytes; 0 MB) used IntCache: cache: 0, tcache: 0, allocated: 0, tallocated: 0 FML: MCP v9.05 FML v7.10.99.99 Minecraft Forge 10.13.4.1558 4 mods loaded, 4 mods active States: 'U' = Unloaded 'L' = Loaded 'C' = Constructed 'H' = Pre-initialized 'I' = Initialized 'J' = Post-initialized 'A' = Available 'D' = Disabled 'E' = Errored UCHI mcp{9.05} [Minecraft Coder Pack] (minecraft.jar) UCHI FML{7.10.99.99} [Forge Mod Loader] (forgeSrc-1.7.10-10.13.4.1558-1.7.10.jar) UCHI Forge{10.13.4.1558} [Minecraft Forge] (forgeSrc-1.7.10-10.13.4.1558-1.7.10.jar) UCHE MnyMod{1.0} [Money Mods] (bin) GL info: ' Vendor: 'NVIDIA Corporation' Version: '4.5.0 NVIDIA 347.25' Renderer: 'GeForce GTX 960/PCIe/SSE2' Launched Version: 1.7.10 LWJGL: 2.9.1 OpenGL: GeForce GTX 960/PCIe/SSE2 GL version 4.5.0 NVIDIA 347.25, NVIDIA Corporation GL Caps: Using GL 1.3 multitexturing. Using framebuffer objects because OpenGL 3.0 is supported and separate blending is supported. Anisotropic filtering is supported and maximum anisotropy is 16. Shaders are available because OpenGL 2.1 is supported. {code} -
[1.7.10] Crafting Recipe Crashes Game on startup
jameshyland replied to jameshyland's topic in Modder Support
also how do you do shapeless crafting recipes? -
[1.7.10] Crafting Recipe Crashes Game on startup
jameshyland replied to jameshyland's topic in Modder Support
here you go -
[1.7.10] Crafting Recipe Crashes Game on startup
jameshyland replied to jameshyland's topic in Modder Support
I know but even uncommented it still doesnt work -
[1.7.10] Crafting Recipe Crashes Game on startup
jameshyland replied to jameshyland's topic in Modder Support
Ok, but what about the other one.. -
Here is my code: The problem I have is that this crafting recipe works: But the other ones do not and return this error report:
-
Minecraft 1.7.10 Ore Generation Problems. [Modding]
jameshyland replied to jameshyland's topic in Modder Support
It now looks like this: Is that better? -
Minecraft 1.7.10 Ore Generation Problems. [Modding]
jameshyland replied to jameshyland's topic in Modder Support
I already have that in the code though. -
Minecraft 1.7.10 Ore Generation Problems. [Modding]
jameshyland replied to jameshyland's topic in Modder Support
I dont know what the difference is. This is my first mod and i have little to no experience with Java programming so... Can you please elaborate? -
So i have been making a mod for the past week or so and I have stumbled upon problems when generating ores. I have entered the code, but the ores dont show up in game. Here is the code for the ore in question If you could help please do. Thanks.