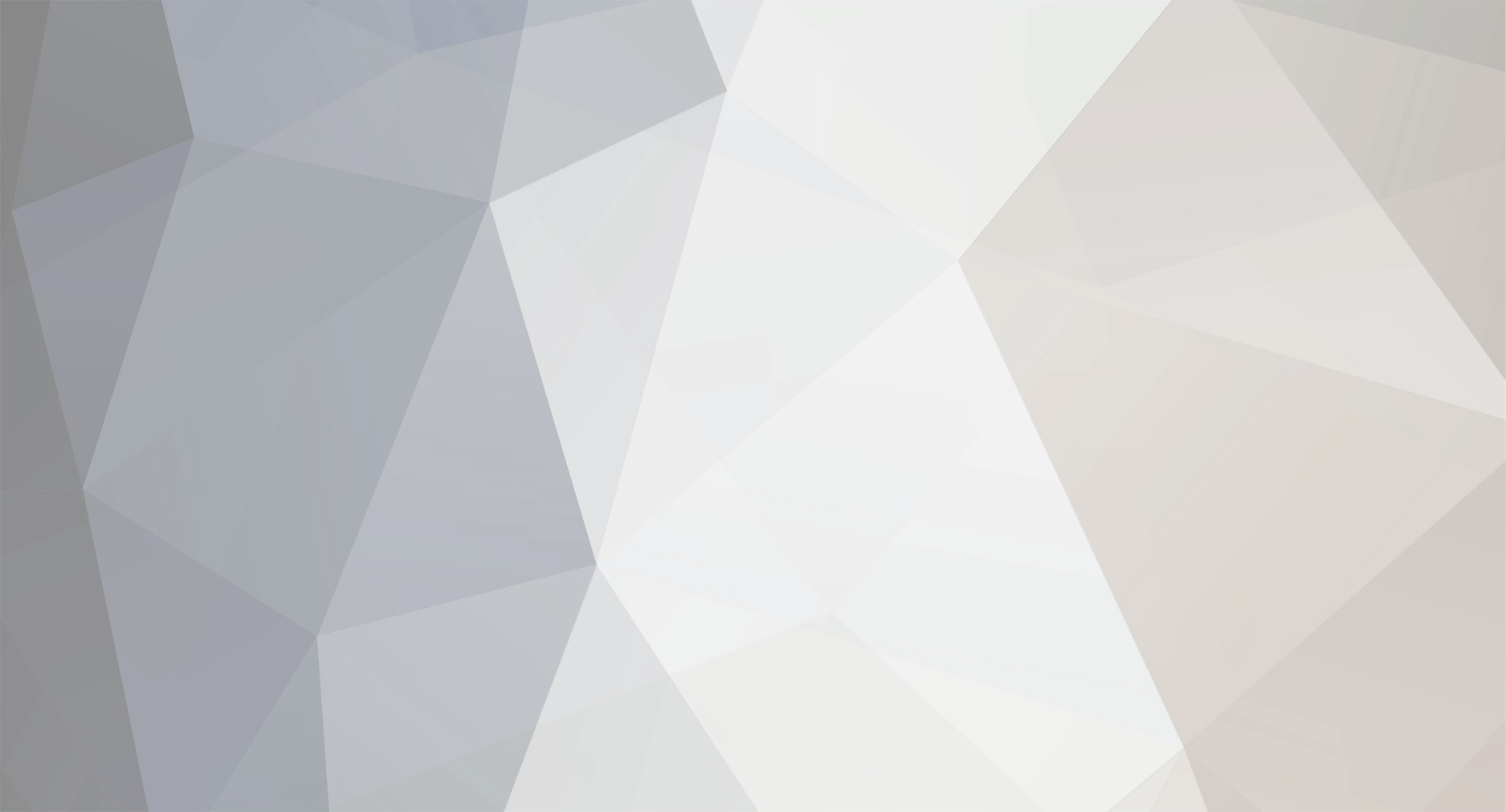
Nxs0000
-
Posts
20 -
Joined
-
Last visited
Posts posted by Nxs0000
-
-
Edit: Don't know how I managed to get it look better for a minute, but I just noticed that it actually is not
The method you gave me woks fine to get the block rendered correctly, but the orange box is still overlapping on the normal block render
-
Wow.
Thank you so much!
It works much better now, but I still have the issue with the block disapearing in the floor tho
-
Thanks for the reply
Here it is:
public class RenderFloatingBlock extends Render<EntityFloatingBlock> { private static final ResourceLocation textures = new ResourceLocation(Main.MODID,"textures/entity/floatingblock.png"); private ResourceLocation t = new ResourceLocation(""); private ModelBase modelEmpty = new ModelFloatingEmpty(); private ModelBase modelFull = new ModelFloatingFull(); public RenderFloatingBlock(RenderManager rendermanager, ModelBase model, float shadowsize) { super(rendermanager); } @Override protected ResourceLocation getEntityTexture(EntityFloatingBlock entity) { return textures; } public void doRender(EntityFloatingBlock entity, double x, double y, double z, float entityYaw, float partialTicks) { GlStateManager.pushMatrix(); GlStateManager.translate((float)x, (float)y-1.15, (float)z); if(entity.inv.getItem() == Item.getItemFromBlock(Blocks.glass)) // glass is a neutral block that represent the emptiness (have had the trouble with NullPointer when ItemStack was null) { // enable semi-transparent (not the cause of the problem: same result without it) GL11.glEnable(GL11.GL_BLEND); GL11.glBlendFunc(GL11.GL_SRC_ALPHA, GL11.GL_ONE_MINUS_SRC_ALPHA); GL11.glColor4f(1.5f, 1.5f, 1.5f, 0.8f); this.bindTexture(textures); this.modelEmpty.render(entity, 0.0F, 0.0F, 0.0F, 0.0F, 0.0F, 0.0625F*1.4F); // disable transparent GL11.glDisable(GL11.GL_BLEND); } else { // Getting the texture from the contaigned block (almost works correctly >.<, cause... mutli-textured blocks ^^) String a = Block.getBlockFromItem(entity.getInv().getItem()).getRegistryName(); String segments[] = a.split(":"); t = new ResourceLocation(segments[0], "textures/blocks/"+segments[1]+".png"); this.bindTexture(t); GlStateManager.translate((float)x, (float)y-1.35, (float)z); this.modelFull.render(entity, 0.0F, 0.0F, 0.0F, 0.0F, 0.0F, 0.0625F*1.4F); } GlStateManager.popMatrix(); super.doRender(entity, x, y, z, entityYaw, partialTicks); } }
Come to think of it. The issue may come from the the super.doRender() call.
I am probably doing something i should not ever do, but I'm new to rendering and have still to learn. Just tell me
-
Edit: Forgot to mention, i am currently using Forge 1.8.9
-
Hey!
I just created a custom entity (extending EntityBase) with a custom model. My idea was to use them as block display, so they look like small floating blocks:
Here is an exemple of how it looks like initially:
And here is how it gets when you "put" a block in it:
So this looks pretty cool to me, but when there are multiple of them, the render is messing up:
It only happens with "empty" ones on "containing" ones. It render correctly when those entity are of the same type.
At first I thought it could be an age-based rendering, so the older entity would render first and the younger last, which could have explained why the renders are overlapping. But no matter the way I'm facing, where the older entity is, it always occure that way.
The way i change the rendering model may be the cause: In the "doRender" method in the EntityRender class, I choose a model depending on what the entity contains then i call the Model.render().
Plus, the entity that contains a block also act wierd with real blocks:
Does someone know what the issue is/are?
-
ok... fml... I'm dumb
Thanks for the help guys! i juste messed up from the start >.<
The real issue was that I did @Override the initGui() to add my stuff and forgot to call the super back...
This caused the inventory to appear weird, which i tried to correct by changing the order in which the slots are registered.
Thanks for your time everyone,
Have a nice day !
-
that could be the drawScreen, that doesn't display the item right since it doesn't understand my slots? Since i left if that way :
@Override public void drawScreen(int mouseX, int mouseY, float partialTicks) { super.drawScreen(mouseX, mouseY, partialTicks); }
-
yea, it seems
I open the Cotainer by right-clicking on the Vitrine Block, then using this in "onBlockActivated"
if (!worldIn.isRemote) { playerIn.openGui(Main.instance, GuiHandler.getID(15), worldIn, pos.getX(), pos.getY(), pos.getZ()); //15 correspond to my Vitrine }
GuiHandler
@Override public Object getServerGuiElement(int ID, EntityPlayer player, World world, int x, int y, int z) { if(ID == 15) return new VitrineContainer((VitrineTileEntity) world.getTileEntity(new BlockPos(x,y,z)) , player.inventory); return null; } @Override public Object getClientGuiElement(int ID, EntityPlayer player, World world, int x, int y, int z) { if(ID == 15) return new GuiVitrine((VitrineTileEntity) world.getTileEntity(new BlockPos(x,y,z)) , player.inventory); return null; }
-
btw, i don't have the armor slot and crafting slots filled up anymore... must be display/sync issue
-
the openInventory call a method from the TileEntity that needed to be override, for now i left it empty.
I tried what you said:
Before:
After:
This really is weird: The inventory looks messed up but the item are just showed in the wrong place: for exemple, when i click on the second slot from the first row i get the 59 blaze powder... Plus, even so the slot for the Container displays the wool, clicking on it does nothing.
It seem to be a display issue now
-
Hey,
I recently stared working on a TileEntity vitrine that display an item/itemBlock on it when it does contain one.
Basically the TileEntity contains 2 properties:
private ItemStack contained; private EntityItem image;
the "image" is created from the "contained" with the it's property stackSize set to 1.
Now i wanted to add a Gui that would allow me to change the contained easily, so i created both the GuiContainer class and the Container one (and registered in the GuiHandler class)
To do all that i just followed bedrockminer's tutorials, changing what needed to be changed, but now i have some wierd issues: (all tested in a single player world)
- when i open the Container, the first Item in my Hotbar gets change into the item that is contained in the TileEntity, even though the item is still in it and clicking on the item in the contained slot take the one in my hotbar.
- when i close the Gui, this first item don't gets back to what it was before openning, and, when i open my inventory (in survival) i can see that some on the items from my hotbar got copied to the armor slots and crafting slots.
- I have to relog to get my inventory back right, as before i even openned the Container.
Here is my VitrineContainer construtor. The class is extending Container, have a property theVitr where I store the VitrineTileEntity.
public VitrineContainer(VitrineTileEntity vitr, IInventory player) { this.theVitr = vitr; theVitr.openInventory(((InventoryPlayer)player).player); //Player inventory for(int x = 0; x<9; x++){ this.addSlotToContainer(new Slot(player, x, 8+x*18, 49+58)); } for(int y = 0; y<3; y++){ for(int x = 0; x<9; x++){ this.addSlotToContainer(new Slot(player, 9+x+y*9, 8+x*18, 49 +18*y)); } } this.addSlotToContainer(new Slot(vitr, 0, 8, 18)); // TileEntity's slot, top left of the Gui }
I don't think the error could come from other classes since I barely created the Gui class just to draw a 1-row chest, and my VitrineBlock and VitrineTileEntity work fine and don't have much to do with the Container, exept for the TileEntity that extends IInventory so I completed the newly genereted methods to return the correct objects (slot 0 returning the contained ItemStack).
Maybe it only is an issue of synchronizing Server and Client since reloging correct the Inventory mess.
Before:
Open the Container:
Clicking on the contained Item:
Opening the inventory after closing the Container:
What is/could be wrong ? Thank you for any help
-
Sorry if i didn't make it clear earlier...
I made a "PokeLauncher" which allow me, by right-clicking, to throw a pokeball that is in my inventory.
The way i made my "Pokeball" item is that when I capture a mob inside, the ball gets an NBT tag to tell what mob is inside it.
For now, my launcher throw every "pokeball" item I have in my inventory, no matter if the pokeball item has this specific NBT tag or not (meaning it is empty) and I want to make it only throw the balls that contain mobs.
Maybe did i make it the wrong way... Is the NBT tag a proper way to do so ?
-
I actually wanted to make some kind of pokeball, and when I catch a mob, the pokeball item get a NBT tag and I need to differentiate the pokeball that has an entity inside to the empty one in order to make a "Pokelauncher", which will throw my pokeballs at huge distances.
But, the thing is the launcher takes every pokeball in my inventory and I just want it to take the pokeballs with an entity.
-
Hi,
Can someone tell me how to differ an item with a NBT tag on it. I'm creating an item that can store a mob and can be launched by gun. So I need to know how to differ the ammo empty and the ammo charged which has a NBT tag.
-
Ok, I am currently trying to replicate the CuiChat methods that autocomplete the player name.
Here are the methods in GuiChat I copied:
- setText(String , boolean)
- autocompletePlayerNames()
- sendAutocompleteRequest(String , String)
- onAutocompleteResponse(String[] )
I change the differents objects names to mine (like "inputField" into "textBox" which is my textField i want te autocomplete to act)
But now, I think i need to add a Handler for the communications of Packets. autocomplete and sendAutoCompleteRequest are fired when i Tab to complete. But onAutocompleteResponse is never fired...
The packets to autocomplete is C14PacketTabComplete (I think, even so there is a BlockPos as argument) and i can't find the packet handler fired on response so I don't know how to fire the last method.
Does someone know what i have to do next ?
-
Thing is that I don't want to update to 1.9. I mean... I have my reasons to stay on 1.8 so i asked for a possible solution on that version. (just figured that i didn't mention the version i used ^^)
Still, thank you for the idee
-
Hey!
I've made a custom GUI for my mod (in 1.
, and wanted to use a TextField with autocompletion. I managed to achieve that by making my Gui class extending GuiChat.
That way, I am now able to use the method "autocompletePlayerNames()" to complete the name that I start to write in the TextField.
But, after a bit, I realized that the chat was active when this Gui was open (seems logic). I managed to remove the writing line of the chat so that when I type, the text don't appear in both the Chat box and my own Text box.
I thought it would do the whole job, but it actually leaves the chat history be. All the messages received are constantly rendered, the chat never unfocus...
So here is my question: What do I have to do to cancel this focus? Or maybe is there another way to autocomplete ( without having to re-write the whole algorithm that do it )
-
Hey,
I am working on a part of my mod which consist in rendering, on client side, some custom area.
What is needed to render those areas is sent through a channel ( I send the coordinates, the type of area, the size, ... )
Here comes my problem, the datas are recieved and correctly interpreted (I used some System.out.printl(thing) to watch is the treatement was correct). Once the datas a corectly understood, the client should create a ArrayList<Area> which contains all the areas Object (with the properties inside). But, when i call the list later, in another method in the same class (the list is part of the class), it appears empty.
Thanks to the Print I made, I saw that:
inside the method that create the list (method called when the packet is recieved), the Print return things like that:
[Netty Local Client IO #0/INFO] [sTDOUT]: [nxs.listManager:addToList:160]: area1
and in the other method, it return :
[Client thread/INFO] [sTDOUT]: [nxs.listManager:canBuild:73]: list emplty!!!
What is the problem ? What is the difference between those two?
[Netty Local Client IO #0/INFO] [Client thread/INFO]
-
Hi everyone,
I recently started to code a mod and now I'm starting the part consisting in creating the dialog between the server and the client.
But I was wondering if a mod could be different on the Client and on the Server. Let's imagine that all the dialog between those two should be correct since they register the same channels and the same type data that go through. Would the rest of the mod cause any trouble because it is different? Like, when the player try to log on a the server, will this player be able to do so ?
I ask because I know that the list of mod of the server is compared with the client's when this last one attempt to log in.
thanks in advance for your help
[Solved] Custom Entity Rendering
in Modder Support
Posted
Ok, i found the issue (even if i don't really get why it makes the block disapearing in the floor)
Apparently, the translate method was creating the whole issue.
Here is my new doRender method:
Thanks for your time and the help Silly511.
Have a nice day