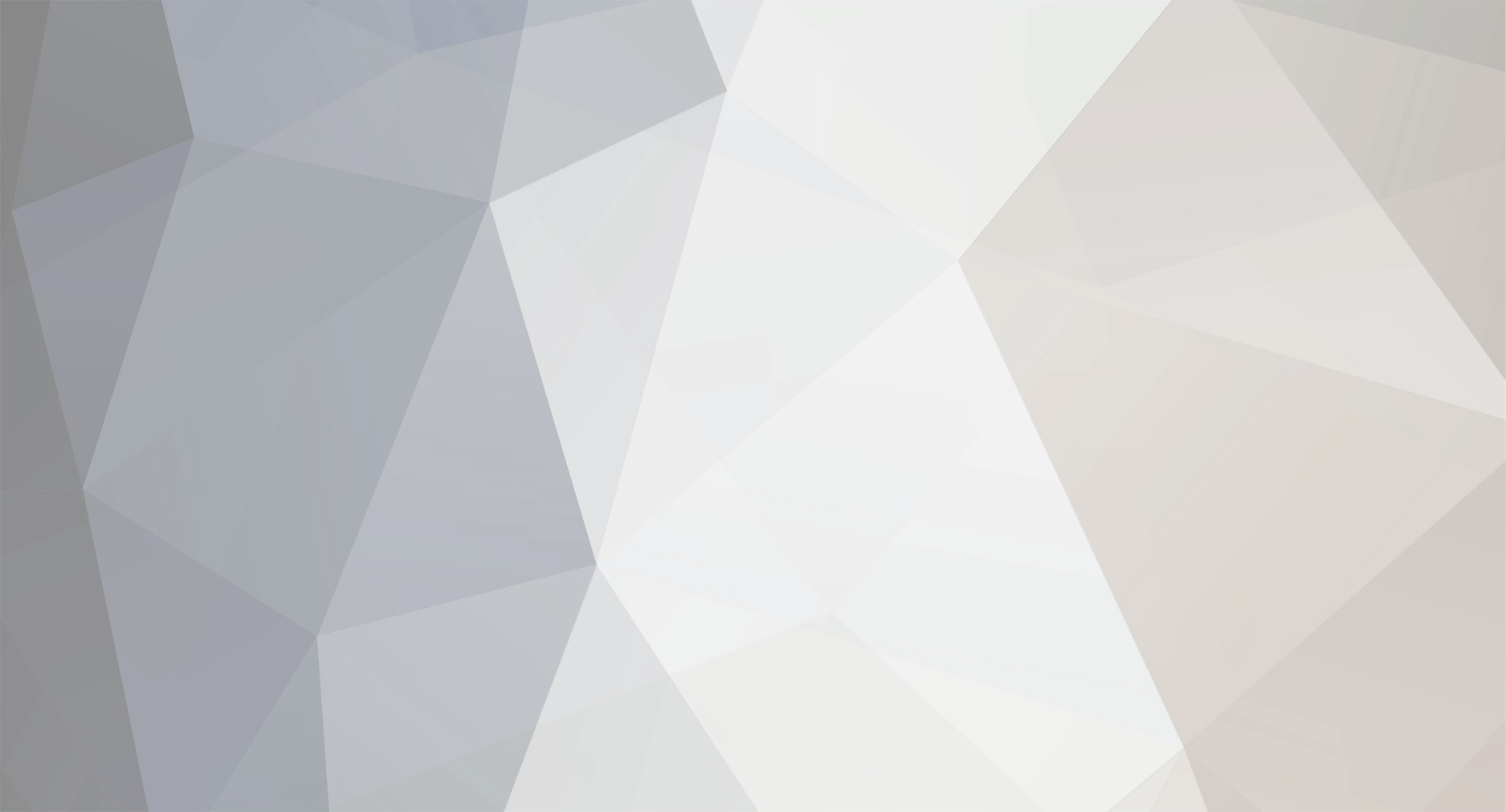
XxArchangelz
-
Posts
49 -
Joined
-
Last visited
-
Days Won
1
Posts posted by XxArchangelz
-
-
You have to pastebin us what the crash says, or we cannot help you with this.
-
That only changes the GUI if I rightclick with the item in hand, I need it to switch as I place the item in the slot which I had working, but it only changes after reopening the gui with the item already in the slot
-
A texture is just a number ( the index of the texture in the sheet).
You need to return a different number depending on whether there is an Item in the slot or not.
I'm using a custom rendered item though so I have two image files (one with one without), I got it working in the ModelAltar class, but when you disconnect/reconnect the block shows up as it were empty then switches back to having a book when you right click it opening the gui.
-
Okay I see, I got that all working now thanks so much. I have one last problem. I made it in my proxy to switch GUI's if an item is present with a similar method and separate gui classes, but the gui only switches after opening and closing the gui. Where would I have to do this to make it work instantly?
-
You need to give it the id of the slot.
Okay so now how would I call the texture from my sprites class? I have been trying to figure out a way to get that working, but It wants me to make the method a string.
-
Oh I see thank you
-
Oh duh my bad, I thought that was the entire thing so I never put it with the method. Now with SLOT_NO, I'm thinking that means SlotNecronomicon from my container class that is set to only allow a certain item in the slot, should be placed there, or no? Because when I try that it tells me that it cannot be resolved to a variable, so would I need to make a variable for it, and like how?
Also when I try to call the texture file assigned in my Sprites class, it throws me an error because the method is an int and to call it from my sprites class would require it be a string.
-
I'm getting errors on blockAccess being unable to be resolved, and the variables. Is that something missing from the TileEntity class?
-
I'm sorry not entirely sure what I am doing. I have this for my block class
package archangel.necromancy.block;
import net.minecraft.block.material.Material;
import net.minecraft.entity.player.EntityPlayer;
import net.minecraft.tileentity.TileEntity;
import net.minecraft.world.IBlockAccess;
import net.minecraft.world.World;
import archangel.necromancy.Necromancy;
import archangel.necromancy.lib.GuiIds;
import archangel.necromancy.lib.RenderIds;
import archangel.necromancy.lib.Strings;
import archangel.necromancy.tileentity.TileAltar;
public class BlockAltar extends BlockNC {
public BlockAltar(int id) {
super(id, Material.rock);
this.setBlockName(Strings.ALTAR_NAME);
this.setCreativeTab(Necromancy.tabsNecro);
this.setHardness(5F);
}
@Override
public TileEntity createNewTileEntity(World var1) {
return new TileAltar();
}
@Override
public boolean renderAsNormalBlock() {
return false;
}
@Override
public boolean isOpaqueCube() {
return false;
}
@Override
public int getRenderType() {
return RenderIds.altarRenderId;
}
@Override
public int getBlockTexture(IBlockAccess blockAccess, int x, int y, int z, int side){
this.getBlockTileEntity(int i, int j, int k)
}
// @Override
// public int getBlockTextureFromSide(int par1) {
// return 1;
// }
public boolean onBlockActivated(World world, int x, int y, int z,
EntityPlayer player, int par6, float par7, float par8, float par9) {
if (!world.isRemote) {
TileAltar tileAltar = (TileAltar) world.getBlockTileEntity(x, y, z);
if (tileAltar != null) {
player.openGui(Necromancy.instance, GuiIds.ALTAR, world, x, y,
z);
}
}
return true;
}
}
I don't know if I'm putting this correctly, but I am getting syntax errors on the variables and I don't know how to word it to make it change the texture and the gui when the item is present.
-
I have 2 items, a tile entity/block/container and a container/item. I have it so far that the block (altar) will only accept the item (book), but I want to have it so that when the book is present in the altar, the block will render a different model and the gui will switch (if book is in slot make altar have book on top and new gui otherwise show original gui with empty slot and empty altar without book)
I figure I need to do if statements but I don't know where and how to go about it. I was thinking it needed to be in GuiAltar or ItemAltarRender but I don't know.
GuiAltar
package archangel.necromancy.client.gui.inventory;
import net.minecraft.client.gui.inventory.GuiContainer;
import net.minecraft.entity.player.InventoryPlayer;
import net.minecraft.util.StatCollector;
import org.lwjgl.opengl.GL11;
import archangel.necromancy.inventory.ContainerAltar;
import archangel.necromancy.lib.Sprites;
import archangel.necromancy.lib.Strings;
import archangel.necromancy.tileentity.TileAltar;
import cpw.mods.fml.common.registry.LanguageRegistry;
public class GuiAltar extends GuiContainer {
private TileAltar altar;
public GuiAltar(InventoryPlayer player, TileAltar altar) {
super(new ContainerAltar(player, altar));
this.ySize = 176;
this.altar = altar;
}
protected void drawGuiContainerForegroundLayer() {
this.fontRenderer.drawString(LanguageRegistry.instance().getStringLocalization(Strings.GUI_ALTAR_NAME), 60, 6, 4210752);
this.fontRenderer.drawString(StatCollector.translateToLocal("container.inventory"), 8, this.ySize - 96 + 2, 4210752);
}
protected void drawGuiContainerBackgroundLayer(float par1, int par2, int par3) {
int var4 = this.mc.renderEngine.getTexture(Sprites.GUI_SHEET_LOCATION + Sprites.ALTAR_MODEL_TEXTURE);
GL11.glColor4f(1.0F, 1.0F, 1.0F, 1.0F);
this.mc.renderEngine.bindTexture(var4);
int var5 = (this.width - this.xSize) / 2;
int var6 = (this.height - this.ySize) / 2;
this.drawTexturedModalRect(var5, var6, 0, 0, this.xSize, this.ySize);
int var7;
}
}
ItemAltarRender
package archangel.necromancy.client.renderer;
import net.minecraft.client.renderer.Tessellator;
import net.minecraft.item.ItemStack;
import net.minecraftforge.client.ForgeHooksClient;
import net.minecraftforge.client.IItemRenderer;
import org.lwjgl.opengl.GL11;
import archangel.necromancy.client.model.ModelAltar;
import archangel.necromancy.lib.Sprites;
public class ItemAltarRenderer implements IItemRenderer {
private ModelAltar altarModel;
public ItemAltarRenderer() {
altarModel = new ModelAltar(1 / 16F);
}
@Override
public boolean handleRenderType(ItemStack item, ItemRenderType type) {
return true;
}
@Override
public boolean shouldUseRenderHelper(ItemRenderType type, ItemStack item, ItemRendererHelper helper) {
return true;
}
@Override
public void renderItem(ItemRenderType type, ItemStack item, Object... data) {
switch (type) {
case ENTITY: {
renderAltar(-0.5F, 0F, -0.5F);
break;
}
case EQUIPPED: {
renderAltar(0F, 0.4F, 0F);
break;
}
case INVENTORY: {
renderAltar(1F, 0.65F, 1F);
break;
}
default:
break;
}
}
private void renderAltar(float x, float y, float z) {
Tessellator tesselator = Tessellator.instance;
ForgeHooksClient.bindTexture(Sprites.SPRITE_SHEET_LOCATION + Sprites.ALTAR_MODEL_TEXTURE, 0);
GL11.glPushMatrix(); //start
GL11.glTranslatef(x, y, z); //size
altarModel.render(0.0625F);
GL11.glPopMatrix(); //end
}
}
I don't know squat about OpenGL and i'm still fairly new to modding so I am trying to learn as much as I can to better myself as modding and java. Thanks for the help
-
NINJA EDIT
I figured it all out. The solution is to create a custom slot (non vanilla slot) and specify it in the class to only allow said items
example
package archangel.necromancy.inventory;
import net.minecraft.inventory.IInventory;
import net.minecraft.inventory.Slot;
import net.minecraft.item.ItemStack;
import archangel.necromancy.item.ModItems;
public class SlotNecronomicon extends Slot {
public SlotNecronomicon(IInventory inventory, int par2, int par3, int par4) {
super(inventory, par2, par3, par4);
}
@Override
public boolean isItemValid(ItemStack itemstack) {
return itemstack.itemID == ModItems.necronomicon.itemID;
}
@Override
public int getSlotStackLimit() {
return 1;
}
}
the slot inside container class
// Add the book slot to the container
this.addSlotToContainer(new SlotNecronomicon(altar, 0, 56, 17));
-
1
-
-
I figured it out and fixed the shift right click crash
I use
@Override
public ItemStack transferStackInSlot(EntityPlayer entityPlayer, int slot) {
Slot slotObject = (Slot) inventorySlots.get(slot);
if(slotObject != null && slotObject.getHasStack()) {
ItemStack stackInSlot = slotObject.getStack();
ItemStack stack = stackInSlot.copy();
if(slot <= 1) {
if(!mergeItemStack(stackInSlot, 2, inventorySlots.size(), true))
return null;
} else if(slot != 1 && stack.itemID == ModItems.necronomicon.itemID && !getSlot(0).getHasStack()) {
ItemStack copy = slotObject.decrStackSize(1);
getSlot(0).putStack(copy);
return null;
} else {
return null;
}
if(stackInSlot.stackSize == 0)
slotObject.putStack(null);
else
slotObject.onSlotChanged();
return stack;
}
return null;
}
But I don't entirely understand it and I still cannot restrict ONLY allowing a specific item into the slot
-
I have a tile entity/container for a block and I want to be able to restrict what can go in its inventory slot to a specific item in my mod, but for the life of me I cannot figure out how. I also have it so that shift clicking crashes the game (not supposed to happen
) and I can see that I need to have something like
public boolean mergeItemStack(itemStack, start, end, backwards)
But I don't know exactly where to put it and what it does.
Here is my tile entity class
package archangel.necromancy.tileentity;
import net.minecraft.entity.player.EntityPlayer;
import net.minecraft.inventory.IInventory;
import net.minecraft.item.ItemStack;
import net.minecraft.nbt.NBTTagCompound;
import net.minecraft.nbt.NBTTagList;
import archangel.necromancy.lib.Strings;
public class TileAltar extends TileNC implements IInventory {
// Then create a ItemStack array (I.E. ItemStack[])
private ItemStack[] inventory;
// Then create a super constructor and give the inventory the amount of
// ItemStacks you want (Slots),
// I.E. (for 1 slot: inventory = new ItemStack[1];, for 5 slots: inventory =
// new ItemStack[5]
public TileAltar() {
inventory = new ItemStack[1];
}
// This returns the inventory size
@Override
public int getSizeInventory() {
return inventory.length;
}
// This returns the stack that is in the slot you picked
// This has 1 param
// @param int slotIndex is just the index you selected
@Override
public ItemStack getStackInSlot(int slotIndex) {
return inventory[slotIndex];
}
// This sets the slots contents, it has 2 params
// @param int slot, this is the slots number
// @param ItemStack stack, this is the stack you want to add
@Override
public void setInventorySlotContents(int slot, ItemStack stack) {
inventory[slot] = stack;
// This checks to make sure the stack is not nothing, and then makes
// sure the stack is not going over the limit
// Of the stack
if (stack != null && stack.stackSize > getInventoryStackLimit()) {
stack.stackSize = getInventoryStackLimit();
}
}
// This decreases the stack size
// It has 2 params
// @param int slotIndex, this is the slot number
// @param int amount, this is the amount you want to decreases by
@Override
public ItemStack decrStackSize(int slotIndex, int amount) {
// This gets the stack with the slot number you want
ItemStack stack = getStackInSlot(slotIndex);
// Then it checks to make sure it has something in it
if (stack != null) {
// Then it checks to make sure that it has something that is equal
// or lesser than the amount you want to add
if (stack.stackSize <= amount) {
setInventorySlotContents(slotIndex, null);
} else {
stack = stack.splitStack(amount);
if (stack.stackSize == 0) {
setInventorySlotContents(slotIndex, null);
}
}
}
// Then it returns the stack
return stack;
}
// This returns the stack in the slot you chose
// It has 1 param
// @param int slotIndex this is the slot number you choose to get
@Override
public ItemStack getStackInSlotOnClosing(int slotIndex) {
// This gets the stack in the slot you chose
ItemStack stack = getStackInSlot(slotIndex);
// This checks to make sure it has something in it
if (stack != null) {
setInventorySlotContents(slotIndex, null);
}
// Then it returns the stack
return stack;
}
// This gets the inventory's stack limit
// This is normally 64 but on some conditions it is something lower
@Override
public int getInventoryStackLimit() {
return 1;
}
// Basically this makes sure the player is not to far away to activate the
// block and, makes sure its the Entity you are
// Selecting
@Override
public boolean isUseableByPlayer(EntityPlayer player) {
return worldObj.getBlockTileEntity(xCoord, yCoord, zCoord) == this
&& player.getDistanceSq(xCoord + 0.5, yCoord + 0.5,
zCoord + 0.5) < 64;
}
// A dummy, mostly un-used method, for a check to do something when you open
// the Gui
@Override
public void openChest() {
}
// Another dummy, mostly un-used method, for a check to do something when
// you close the Gui
@Override
public void closeChest() {
}
@Override
public void readFromNBT(NBTTagCompound tagCompound) {
super.readFromNBT(tagCompound);
NBTTagList tagList = tagCompound.getTagList("Inventory");
for (int i = 0; i < tagList.tagCount(); i++) {
NBTTagCompound tag = (NBTTagCompound) tagList.tagAt(i);
byte slot = tag.getByte("Slot");
if (slot >= 0 && slot < inventory.length) {
inventory[slot] = ItemStack.loadItemStackFromNBT(tag);
}
}
}
@Override
public void writeToNBT(NBTTagCompound tagCompound) {
super.writeToNBT(tagCompound);
NBTTagList itemList = new NBTTagList();
for (int i = 0; i < inventory.length; i++) {
ItemStack stack = inventory;
if (stack != null) {
NBTTagCompound tag = new NBTTagCompound();
tag.setByte("Slot", (byte) i);
stack.writeToNBT(tag);
itemList.appendTag(tag);
}
}
tagCompound.setTag("Inventory", itemList);
}
// This returns the inventory's name
@Override
public String getInvName() {
return "container." + Strings.ALTAR_NAME;
}
}
and the container class
package archangel.necromancy.inventory;
import net.minecraft.entity.player.EntityPlayer;
import net.minecraft.entity.player.InventoryPlayer;
import net.minecraft.inventory.Container;
import net.minecraft.inventory.Slot;
import net.minecraft.item.ItemStack;
import archangel.necromancy.tileentity.TileAltar;
public class ContainerAltar extends Container {
private TileAltar altar;
public ContainerAltar(InventoryPlayer inventoryPlayer, TileAltar altar) {
// Set the instance of the TileAltar for the container
this.altar = altar;
// Add the book slot to the container
this.addSlotToContainer(new Slot(altar, 0, 56, 17));
// Add the player's inventory slots to the container
for (int inventoryRowIndex = 0; inventoryRowIndex < 3; ++inventoryRowIndex) {
for (int inventoryColumnIndex = 0; inventoryColumnIndex < 9; ++inventoryColumnIndex) {
this.addSlotToContainer(new Slot(inventoryPlayer,
inventoryColumnIndex + inventoryRowIndex * 9 + 9,
8 + inventoryColumnIndex * 18,
94 + inventoryRowIndex * 18));
}
}
// Add the player's action bar slots to the container
for (int actionBarSlotIndex = 0; actionBarSlotIndex < 9; ++actionBarSlotIndex) {
this.addSlotToContainer(new Slot(inventoryPlayer,
actionBarSlotIndex, 8 + actionBarSlotIndex * 18, 152));
}
}
public boolean canInteractWith(EntityPlayer player) {
return true;
}
@Override
public ItemStack transferStackInSlot(EntityPlayer player, int slot) {
ItemStack stack = null;
Slot slotObject = (Slot) inventorySlots.get(slot);
// null checks and checks if the item can be stacked (maxStackSize > 1)
if (slotObject != null && slotObject.getHasStack()) {
ItemStack stackInSlot = slotObject.getStack();
stack = stackInSlot.copy();
// merges the item into player inventory since its in the tileEntity
if (slot < 9) {
if (!this.mergeItemStack(stackInSlot, 9, 45, true)) {
return null;
}
}
// places it into the tileEntity is possible since its in the player
// inventory
else if (!this.mergeItemStack(stackInSlot, 0, 9, false)) {
return null;
}
if (stackInSlot.stackSize == 0) {
slotObject.putStack(null);
} else {
slotObject.onSlotChanged();
}
if (stackInSlot.stackSize == stack.stackSize) {
return null;
}
slotObject.onPickupFromSlot(player, stackInSlot);
}
return stack;
}
}
-
Awesome then, Thank you so much for the help. It will have to be server side, odds are I will have more questions but I will try to figure this out. Again thank you so much
-
How would I go about doing that? I'm sorry I just don't see ANY tutorials whatsoever for making GUI's for items in forge. The best I found was modloader and I would prefer not using modloader. I'm trying to basically have it like in thaumcraft where you will open a book and it will have instructions/recipes to the things the player will be doing, as well as the book being used in a receptacle and when the book is in the receptacle you will have a new gui extending from the block that will act as a container/tile entity. I'm not sure if what I am trying to do with the book requires it to be a tile entity or a container, and what code is required to set it up in the guiscreen/proxy/item class or where to find references on how to do it. I have it working perfectly with the block acting as a container and it has its gui but this has me completely stumped.
-
Right, but now if its an item and I don't plan on it having a container, wouldn't I just need the GUI class and the handler? I'm sorry I'm new and completely lost trying to get this to work
-
I have that much so far in the item class, I'm just clueless to the structure/methods I need to use in the guihandler (client / server functions like for tile entity and container )
-
I figured out the GuiHandler and everything to get a block gui working, but I can't figure out the procedure for the handler for an item.
-
Or am I doing something entirely different?
-
I'm fairly sure you will have to rewrite everything if you don't have the .java files.
-
Actually I don't, I thought I made one. Would that be whats messing this all up on me?
-
What are you saying, that you only have the source files to your mod? If so install the latest forge and put your source files inside of forge/mcp/src/minecraft and run eclipse or whatever you use.
-
java.lang.ClassNotFoundException: net.trito.stc.TritoCraftBase
There is something wrong with that class in net/trito/stc/. See if you are referencing everything correctly and such. We can't really help if its only a crash report.
-
Any help? I would prefer to not have any mod loader references in my code.
How to make an item open a GUI
in Modder Support
Posted
look, this is what I use and it works just fine.
This pretty much makes it so when this item in hand is right-clicked, it will open a gui defined in my guihandler (GuiIds) class. This ONLY allows you to open the gui, you still need to make a container class, a gui class and set this up in your proxy