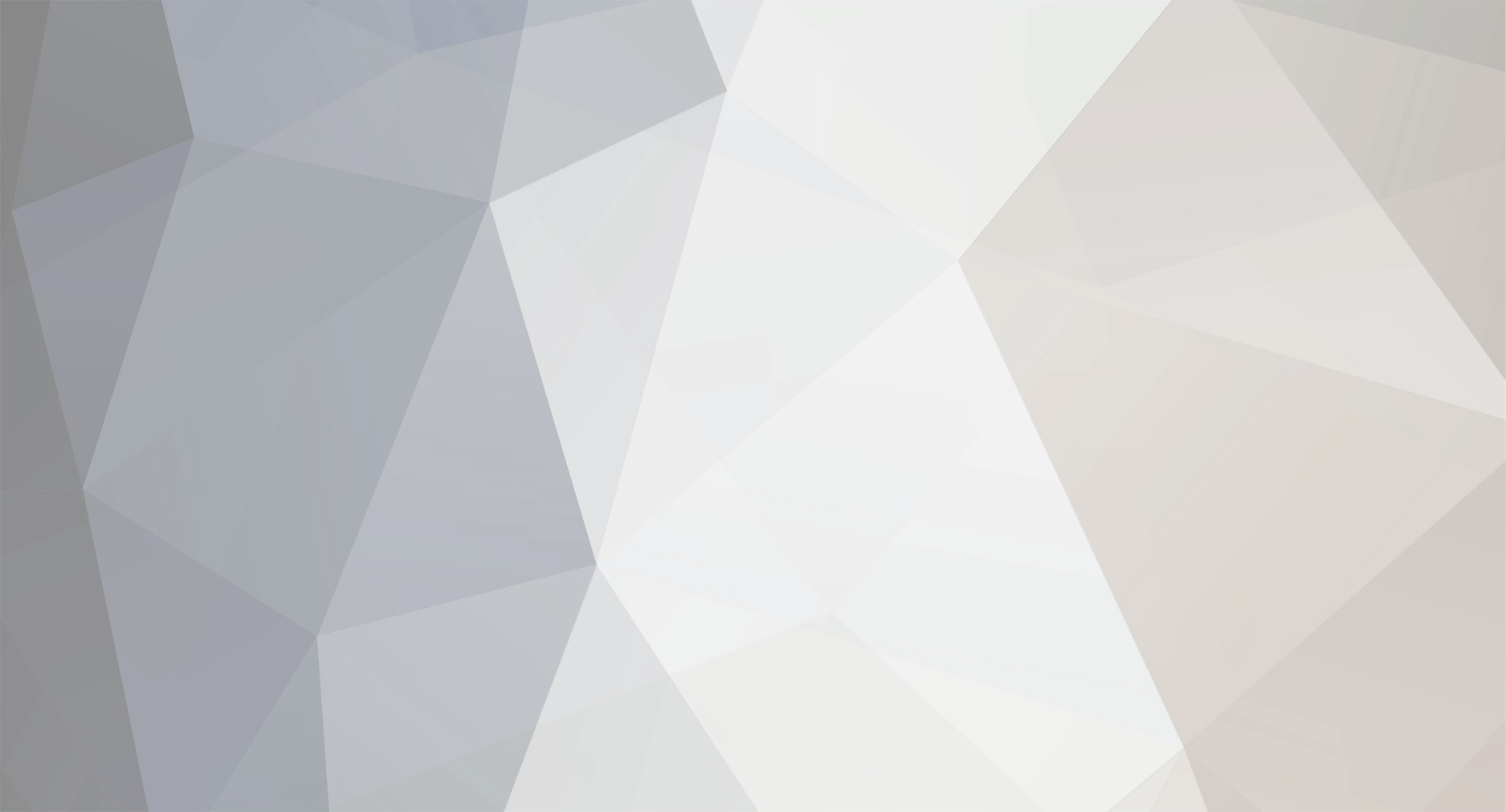
headstack
Members-
Posts
8 -
Joined
-
Last visited
Everything posted by headstack
-
1.10.2 - Custom Mobs dont work Server-side, but Singleplayer work fine!
headstack replied to Keks's topic in Modder Support
Register the entity in your CommonProxy, render it in your ClientProxy. -
I have an item, which when interacted with a Block, checks if there are EntityItems around the block using World.getEntitiesWithinAABB(EntityItem.class, aabb). If the EntityItems contain a certain type of ItemStack (checked with EntityItem.getEntityItem()) then it sets a flag which will be later used. However, when compared to an ItemStack, it always returns false. I am dropping the correct items near the block, and have verified that it knows the items are there by checking the ItemStacks around using println. This leads me to believe that there is a problem with the check. Amalgamite.java (snippet, item) @Override public EnumActionResult onItemUse(ItemStack stack, EntityPlayer playerIn, World worldIn, BlockPos pos, EnumHand hand, EnumFacing facing, float hitX, float hitY, float hitZ) { if (worldIn.getBlockState(pos).getBlock() instanceof Amalgamator) { EntityItem ironIngots = null; EntityItem wool = null; if (AncepsUtil.itemsNearby(worldIn, pos, Amalgamator.detectRange)) { // function which checks if items are nearby (self-explanatory) AxisAlignedBB bb = new AxisAlignedBB(pos.getX() - 4, pos.getY() - 4, pos.getZ() - 4, pos.getX() + 4, pos.getY() + 4, pos.getZ() + 4); List<? extends EntityItem> items = worldIn.getEntitiesWithinAABB(EntityItem.class, bb); for (EntityItem i : items) { // start of problem if (!worldIn.isRemote) { if (i.getEntityItem().equals(new ItemStack(Items.iron_ingot, )) { System.out.println("iron success"); ironIngots = i; } else if (i.getEntityItem().equals(new ItemStack(Blocks.wool, 1, OreDictionary.WILDCARD_VALUE))) { System.out.println("wool success"); wool = i; } } } if (ironIngots != null && wool != null) { ironIngots.setDead(); wool.setDead(); if (!worldIn.isRemote) { worldIn.spawnEntityInWorld(new EntityItem(worldIn, pos.getX(), pos.getY() + 1, pos.getZ(), new ItemStack(AncepsMain.woolyironingot))); // result } return EnumActionResult.SUCCESS; } } } return EnumActionResult.PASS; } AncepsUtil.getEntityItem() public static boolean itemsNearby(World worldObj, BlockPos posAt, int detectRange) { AxisAlignedBB bb = new AxisAlignedBB(posAt.getX() - detectRange, posAt.getY(), posAt.getZ() - detectRange, posAt.getX() + detectRange, posAt.getY() + detectRange, posAt.getZ() + detectRange); return worldObj.getEntitiesWithinAABB(EntityItem.class, bb) != null; }
-
[1.9] Ticking block entity error with setState
headstack replied to headstack's topic in Modder Support
I'm making a block which is essentially a furnace that runs off of another fuel and it has it's own recipes. Most of it works, except when I put in the either the fuel or the item which is being changed (whichever is inserted second) the game crashes. When I relog, however, the tile entity does the smelting process perfectly. When it crashes it gives me this error: java.lang.NullPointerException: Ticking block entity at com.headstack.magijern.block.InfusionMachine.setState(InfusionMachine.java:101) at com.headstack.magijern.tileentity.TileEntityInfusionMachine.update(TileEntityInfusionMachine.java:256) at net.minecraft.world.World.updateEntities(World.java:1936) at net.minecraft.world.WorldServer.updateEntities(WorldServer.java:637) at net.minecraft.server.MinecraftServer.updateTimeLightAndEntities(MinecraftServer.java:779) at net.minecraft.server.MinecraftServer.tick(MinecraftServer.java:683) at net.minecraft.server.integrated.IntegratedServer.tick(IntegratedServer.java:155) at net.minecraft.server.MinecraftServer.run(MinecraftServer.java:532) at java.lang.Thread.run(Unknown Source) Here are the snippets where the errors happen: InfusionMachine.java (block) public static void setState(boolean active, World worldIn, BlockPos pos) { IBlockState iblockstate = worldIn.getBlockState(pos); TileEntity tileentity = worldIn.getTileEntity(pos); keepInventory = true; if (active) { worldIn.setBlockState(pos, Magijern.infusion_machine_lit.getDefaultState(), 3); <-- Line 101, where it errored worldIn.setBlockState(pos, Magijern.infusion_machine_lit.getDefaultState(), 3); } else { worldIn.setBlockState(pos, Magijern.infusion_machine.getDefaultState(), 3); worldIn.setBlockState(pos, Magijern.infusion_machine.getDefaultState(), 3); } keepInventory = false; if (tileentity != null) { tileentity.validate(); worldIn.setTileEntity(pos, tileentity); } } TileEntityInfusionMachine.java (tileentity) public void update() { boolean flag = this.isBurning(); boolean flag1 = false; if (this.isBurning()) { --this.furnaceBurnTime; } if (!this.worldObj.isRemote) { if (this.isBurning() || this.furnaceItemStacks[1] != null && this.furnaceItemStacks[0] != null) { if (!this.isBurning() && this.canSmelt()) { this.currentItemBurnTime = this.furnaceBurnTime = getItemBurnTime(this.furnaceItemStacks[1]); if (this.isBurning()) { flag1 = true; if (this.furnaceItemStacks[1] != null) { --this.furnaceItemStacks[1].stackSize; if (this.furnaceItemStacks[1].stackSize == 0) { this.furnaceItemStacks[1] = furnaceItemStacks[1].getItem().getContainerItem(furnaceItemStacks[1]); } } } } if (this.isBurning() && this.canSmelt()) { ++this.cookTime; if (this.cookTime == this.totalCookTime) { this.cookTime = 0; this.totalCookTime = this.getCookTime(this.furnaceItemStacks[0]); this.smeltItem(); flag1 = true; } } else { this.cookTime = 0; } } else if (!this.isBurning() && this.cookTime > 0) { this.cookTime = MathHelper.clamp_int(this.cookTime - 2, 0, this.totalCookTime); } if (flag != this.isBurning()) { flag1 = true; InfusionMachine.setState(this.isBurning(), this.worldObj, this.pos); // <-- Line 256, where it errored } } if (flag1) { this.markDirty(); } } -
I'm making a block which is essentially a furnace that runs off of another fuel and it has it's own recipes. Most of it works, except when I put in the either the fuel or the item which is being changed (whichever is inserted second) the game crashes. When I relog, however, the tile entity does the smelting process perfectly. When it crashes it gives me this error: java.lang.NullPointerException: Ticking block entity at com.headstack.magijern.block.InfusionMachine.setState(InfusionMachine.java:101) at com.headstack.magijern.tileentity.TileEntityInfusionMachine.update(TileEntityInfusionMachine.java:256) at net.minecraft.world.World.updateEntities(World.java:1936) at net.minecraft.world.WorldServer.updateEntities(WorldServer.java:637) at net.minecraft.server.MinecraftServer.updateTimeLightAndEntities(MinecraftServer.java:779) at net.minecraft.server.MinecraftServer.tick(MinecraftServer.java:683) at net.minecraft.server.integrated.IntegratedServer.tick(IntegratedServer.java:155) at net.minecraft.server.MinecraftServer.run(MinecraftServer.java:532) at java.lang.Thread.run(Unknown Source) Here are the snippets where the errors happen: InfusionMachine.java (block) public static void setState(boolean active, World worldIn, BlockPos pos) { IBlockState iblockstate = worldIn.getBlockState(pos); TileEntity tileentity = worldIn.getTileEntity(pos); keepInventory = true; if (active) { worldIn.setBlockState(pos, Magijern.infusion_machine_lit.getDefaultState(), 3); <-- Line 101, where it errored worldIn.setBlockState(pos, Magijern.infusion_machine_lit.getDefaultState(), 3); } else { worldIn.setBlockState(pos, Magijern.infusion_machine.getDefaultState(), 3); worldIn.setBlockState(pos, Magijern.infusion_machine.getDefaultState(), 3); } keepInventory = false; if (tileentity != null) { tileentity.validate(); worldIn.setTileEntity(pos, tileentity); } } TileEntityInfusionMachine.java (tileentity) public void update() { boolean flag = this.isBurning(); boolean flag1 = false; if (this.isBurning()) { --this.furnaceBurnTime; } if (!this.worldObj.isRemote) { if (this.isBurning() || this.furnaceItemStacks[1] != null && this.furnaceItemStacks[0] != null) { if (!this.isBurning() && this.canSmelt()) { this.currentItemBurnTime = this.furnaceBurnTime = getItemBurnTime(this.furnaceItemStacks[1]); if (this.isBurning()) { flag1 = true; if (this.furnaceItemStacks[1] != null) { --this.furnaceItemStacks[1].stackSize; if (this.furnaceItemStacks[1].stackSize == 0) { this.furnaceItemStacks[1] = furnaceItemStacks[1].getItem().getContainerItem(furnaceItemStacks[1]); } } } } if (this.isBurning() && this.canSmelt()) { ++this.cookTime; if (this.cookTime == this.totalCookTime) { this.cookTime = 0; this.totalCookTime = this.getCookTime(this.furnaceItemStacks[0]); this.smeltItem(); flag1 = true; } } else { this.cookTime = 0; } } else if (!this.isBurning() && this.cookTime > 0) { this.cookTime = MathHelper.clamp_int(this.cookTime - 2, 0, this.totalCookTime); } if (flag != this.isBurning()) { flag1 = true; InfusionMachine.setState(this.isBurning(), this.worldObj, this.pos); // <-- Line 256, where it errored } } if (flag1) { this.markDirty(); } }
-
Yes, that was it! In my init function where I register my items and blocks the item was being registered last. Thank you.
-
Yes, that was it! In my init function where I register my items and blocks the item was being registered last. Thank you.
-
I am currently trying to update a mod from 1.8 to 1.9, and in the process I saw that the method for making a block drop a custom item isn't working. The method I am using is just plainly overriding the quantityDropped and getItemDropped functions from the Block class and making them return variables I have predefined in my custom block class. private int dropamountmax; private Item dropsOnHarvest; @Override public int quantityDropped(Random random) { return dropamountmax; } @Override public Item getItemDropped(IBlockState state, Random rand, int fortune) { return dropsOnHarvest; } Now, instead of dropping the Item dropsOnHarvest, it drops nothing when I break it with the correct tools and harvest level. Is there anything I might've missed in doing so?
-
I am currently trying to update a mod from 1.8 to 1.9, and in the process I saw that the method for making a block drop a custom item isn't working. The method I am using is just plainly overriding the quantityDropped and getItemDropped functions from the Block class and making them return variables I have predefined in my custom block class. private int dropamountmax; private Item dropsOnHarvest; @Override public int quantityDropped(Random random) { return dropamountmax; } @Override public Item getItemDropped(IBlockState state, Random rand, int fortune) { return dropsOnHarvest; } Now, instead of dropping the Item dropsOnHarvest, it drops nothing when I break it with the correct tools and harvest level. Is there anything I might've missed in doing so?