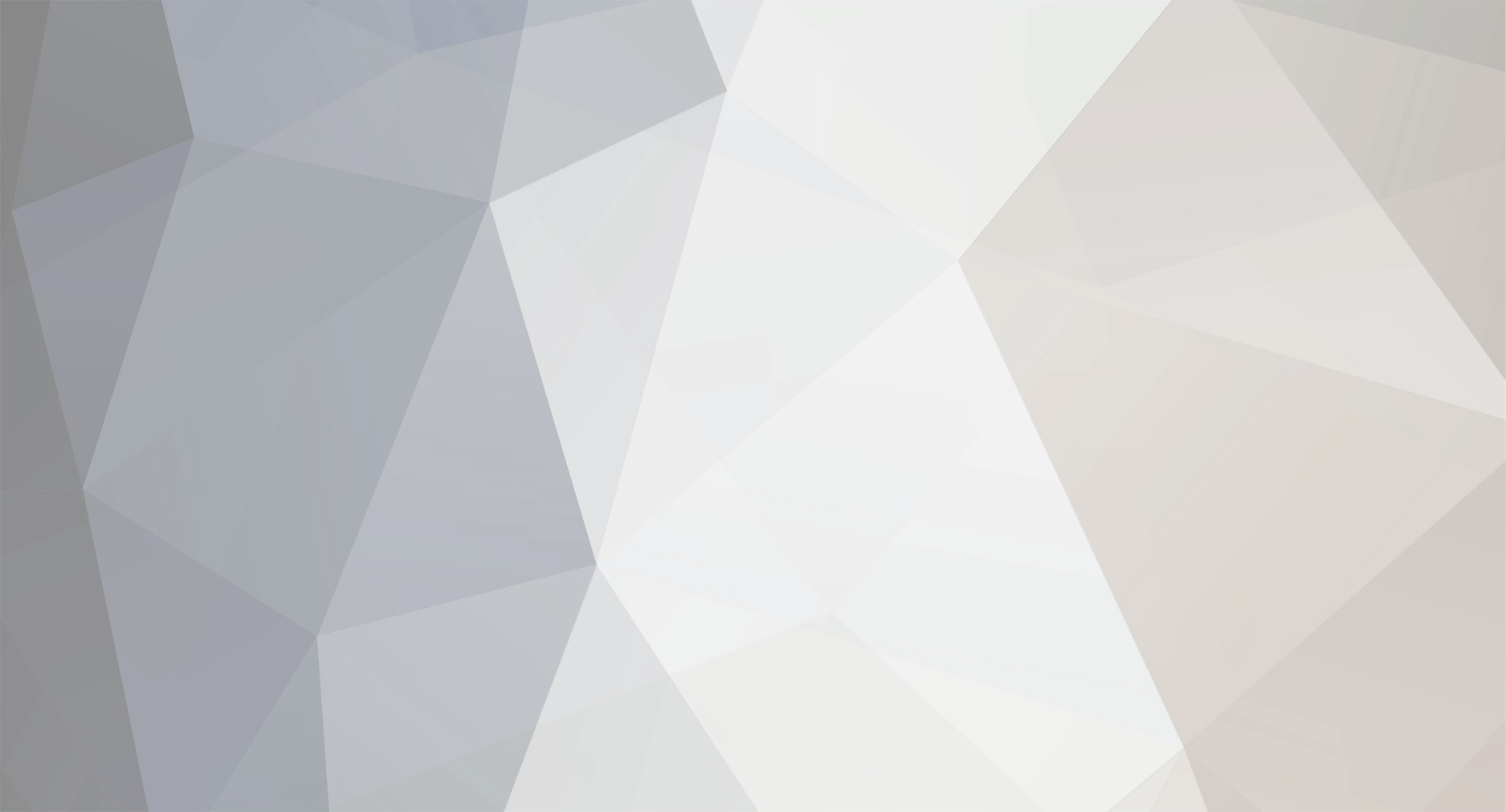
Eighty8keyGuru
Members-
Posts
16 -
Joined
-
Last visited
Everything posted by Eighty8keyGuru
-
Okay. This is my third time posting on this forum in two days. I feel soooooo dumb right now. I'm obviously new to modding. I am using wuppy's book tutorial to learn to mod with 1.8 and eclipse. I have four custom items right now, which should turn into two items with two metadata tags (meaning, I have a "key" and an item called "sam," because I have "Grey Key" and "Red Key", then I have "Sam Dust" and "Sam Ingot.") My key textures both work fine in game, but I have tried doing the exact same thing with the samingots and samdust in the json, lang, and texture files (changing the appropriate names, of course), and I get nothing but purple-black cubes no matter what I try. Here's an example of my key and sam jsons. keygrey.json { "parent": "builtin/generated", "textures": { "layer0": "nathantestmod:items/keygrey" }, "display": { "thirdperson": { "rotation": [ -90, 0, 0 ], "translation": [0, 1, -3 ], "scale": [ 1.7, 1.7, 1.7 ] } } } The red key is the same as this, just with keyred in the layer0 line. ingotsam.json { "parent": "builtin/generated", "textures": { "layer0": "nathantestmod:items/item.ingotsam" }, "display": { "thirdperson": { "rotation": [ -90, 0, 0 ], "translation": [0, 1, -3 ], "scale": [ 1.7, 1.7, 1.7 ] } } } The sam dust file is the same as this with dustsam in the layer0. The json files are all located in assets/nathantestmod/models/item My texture files are named: keygrey.png, keyred.png, ingotsam.png, dustsam.png and are located in assets/nathantestmod/textures/items The lang files I know are fine because the names of all four items are fine in game. The coding to register all the items in the mod class is as follows: @EventHandler public void PostInit(FMLPostInitializationEvent event) { if(event.getSide() == Side.CLIENT) { RenderItem renderItem = Minecraft.getMinecraft().getRenderItem(); renderItem.getItemModelMesher().register(key, 0, new ModelResourceLocation(MODID + ":" + ((ItemKey) key).getNameFromDamage(0), "inventory")); renderItem.getItemModelMesher().register(key, 1, new ModelResourceLocation(MODID + ":" + ((ItemKey) key).getNameFromDamage(1), "inventory")); renderItem.getItemModelMesher().register(sam, 0, new ModelResourceLocation(MODID + ":" + ((ItemSamGeneric) sam).getNameFromDamage(0), "inventory")); renderItem.getItemModelMesher().register(sam, 1, new ModelResourceLocation(MODID + ":" + ((ItemSamGeneric) sam).getNameFromDamage(1), "inventory")); } Sorry about my stupidity in modding. I'm just really slow to learn stuff like this and the tutorial is not as clear as I would like it to be. Thanks for any assistance.
-
Ok I'm sorry. Here's the first method, right between the imports and the recipes in the Init Method. public class NathanTestMod { public static final String MODID = "nathantestmod"; public static final String VERSION = "1.0"; public static Item key; public static Item sam; @EventHandler public void PreInit(FMLPreInitializationEvent event) { key = new ItemKey(); sam = new ItemSamGeneric(); if(event.getSide() == Side.CLIENT) { ItemKey.registerVariants(); } and here's the postInit method: @EventHandler public void PostInit(FMLPostInitializationEvent event) { if(event.getSide() == Side.CLIENT) { RenderItem renderItem = Minecraft.getMinecraft().getRenderItem(); renderItem.getItemModelMesher().register(key, 0, new ModelResourceLocation(MODID + ":" + ((ItemKey) key).getNameFromDamage(0), "inventory")); renderItem.getItemModelMesher().register(key, 1, new ModelResourceLocation(MODID + ":" + ((ItemKey) key).getNameFromDamage(1), "inventory")); renderItem.getItemModelMesher().register(sam, 0, new ModelResourceLocation(MODID + ":" + ((ItemKey) sam).getNameFromDamage(0), "inventory")); renderItem.getItemModelMesher().register(sam, 1, new ModelResourceLocation(MODID + ":" + ((ItemKey) sam).getNameFromDamage(1), "inventory")); } } The weird thing is, eclipse showed no errors in any of the classes.
-
Here is my item class code. Can someone please tell me what to change? I just want to set metadata to the two items: samdust and samingot, within the class SamItemGeneric: 1 package com.nathantestmod; 2 3 import net.minecraft.item.Item; 4 import net.minecraft.item.ItemStack; 5 6 import java.util.List; 7 8 import net.minecraft.client.resources.model.ModelBakery; 9 import net.minecraft.creativetab.CreativeTabs; 10 import net.minecraftforge.fml.common.registry.GameRegistry; 11 import net.minecraftforge.fml.relauncher.Side; 12 import net.minecraftforge.fml.relauncher.SideOnly; 13 14 public class ItemSamGeneric extends Item 15 { 16 private static String name = "sam"; 17 private static String[] metaNames = { "dust", "ingot" }; 18 19 public ItemSamGeneric() 20 { 21 GameRegistry.registerItem(this, name); 22 setUnlocalizedName(NathanTestMod.MODID + "_" + name); 23 setCreativeTab(CreativeTabs.tabMisc); 24 setHasSubtypes(true); 25 } 26 27 public String getName() 28 { 29 return name; 30 } 31 32 @Override 33 public String getUnlocalizedName(ItemStack par1ItemStack) 34 { 35 return super.getUnlocalizedName() + "." + metaNames[par1ItemStack.getItemDamage()]; 36 } 37 public static String getNameFromDamage(int damage) 38 { 39 return name + metaNames[damage]; 40 } 41 public static void registerVariants() 42 { 43 String[] variantNames = new String[metaNames.length]; 44 for (int i = 0; i < metaNames.length; i++) 45 { 46 variantNames = NathanTestMod.MODID + ":" + getNameFromDamage(i); 47 } 48 ModelBakery.addVariantName(NathanTestMod.sam, variantNames); 49 } 50 @SideOnly(Side.CLIENT) 51 @Override 52 public void getSubItems(Item itemIn, CreativeTabs tab, List subItems) 53 { 54 for (int i = 0; i < metaNames.length; ++i) 55 { 56 subItems.add(new ItemStack(itemIn, 1, i)); 57 } 58 } 59 60 }
-
Hello. I am trying to use wuppy's tutorial book on modding mc 1.8 with eclipse and forge. Everything in the file and both item classes is fine compared to what is in the book, it follows it to the letter; but yet I receive this message when trying to run minecraft through the eclipse: AL lib: (EE) alc_cleanup: 1 device not closed Here is the crash report: ---- Minecraft Crash Report ---- // This is a token for 1 free hug. Redeem at your nearest Mojangsta: [~~HUG~~] Time: 6/19/16 1:08 PM Description: Initializing game java.lang.ClassCastException: com.nathantestmod.ItemSamGeneric cannot be cast to com.nathantestmod.ItemKey at com.nathantestmod.NathanTestMod.PostInit(NathanTestMod.java:104) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) at java.lang.reflect.Method.invoke(Unknown Source) at net.minecraftforge.fml.common.FMLModContainer.handleModStateEvent(FMLModContainer.java:553) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) at java.lang.reflect.Method.invoke(Unknown Source) at com.google.common.eventbus.EventSubscriber.handleEvent(EventSubscriber.java:74) at com.google.common.eventbus.SynchronizedEventSubscriber.handleEvent(SynchronizedEventSubscriber.java:47) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:322) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:304) at com.google.common.eventbus.EventBus.post(EventBus.java:275) at net.minecraftforge.fml.common.LoadController.sendEventToModContainer(LoadController.java:212) at net.minecraftforge.fml.common.LoadController.propogateStateMessage(LoadController.java:190) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) at java.lang.reflect.Method.invoke(Unknown Source) at com.google.common.eventbus.EventSubscriber.handleEvent(EventSubscriber.java:74) at com.google.common.eventbus.SynchronizedEventSubscriber.handleEvent(SynchronizedEventSubscriber.java:47) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:322) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:304) at com.google.common.eventbus.EventBus.post(EventBus.java:275) at net.minecraftforge.fml.common.LoadController.distributeStateMessage(LoadController.java:119) at net.minecraftforge.fml.common.Loader.initializeMods(Loader.java:736) at net.minecraftforge.fml.client.FMLClientHandler.finishMinecraftLoading(FMLClientHandler.java:316) at net.minecraft.client.Minecraft.startGame(Minecraft.java:528) at net.minecraft.client.Minecraft.run(Minecraft.java:356) at net.minecraft.client.main.Main.main(Main.java:117) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) at java.lang.reflect.Method.invoke(Unknown Source) at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) at net.minecraft.launchwrapper.Launch.main(Launch.java:28) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) at java.lang.reflect.Method.invoke(Unknown Source) at net.minecraftforge.gradle.GradleStartCommon.launch(Unknown Source) at GradleStart.main(Unknown Source) A detailed walkthrough of the error, its code path and all known details is as follows: --------------------------------------------------------------------------------------- -- Head -- Stacktrace: at com.nathantestmod.NathanTestMod.PostInit(NathanTestMod.java:104) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) at java.lang.reflect.Method.invoke(Unknown Source) at net.minecraftforge.fml.common.FMLModContainer.handleModStateEvent(FMLModContainer.java:553) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) at java.lang.reflect.Method.invoke(Unknown Source) at com.google.common.eventbus.EventSubscriber.handleEvent(EventSubscriber.java:74) at com.google.common.eventbus.SynchronizedEventSubscriber.handleEvent(SynchronizedEventSubscriber.java:47) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:322) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:304) at com.google.common.eventbus.EventBus.post(EventBus.java:275) at net.minecraftforge.fml.common.LoadController.sendEventToModContainer(LoadController.java:212) at net.minecraftforge.fml.common.LoadController.propogateStateMessage(LoadController.java:190) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) at java.lang.reflect.Method.invoke(Unknown Source) at com.google.common.eventbus.EventSubscriber.handleEvent(EventSubscriber.java:74) at com.google.common.eventbus.SynchronizedEventSubscriber.handleEvent(SynchronizedEventSubscriber.java:47) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:322) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:304) at com.google.common.eventbus.EventBus.post(EventBus.java:275) at net.minecraftforge.fml.common.LoadController.distributeStateMessage(LoadController.java:119) at net.minecraftforge.fml.common.Loader.initializeMods(Loader.java:736) at net.minecraftforge.fml.client.FMLClientHandler.finishMinecraftLoading(FMLClientHandler.java:316) at net.minecraft.client.Minecraft.startGame(Minecraft.java:528) -- Initialization -- Details: Stacktrace: at net.minecraft.client.Minecraft.run(Minecraft.java:356) at net.minecraft.client.main.Main.main(Main.java:117) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) at java.lang.reflect.Method.invoke(Unknown Source) at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) at net.minecraft.launchwrapper.Launch.main(Launch.java:28) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) at java.lang.reflect.Method.invoke(Unknown Source) at net.minecraftforge.gradle.GradleStartCommon.launch(Unknown Source) at GradleStart.main(Unknown Source) -- System Details -- Details: Minecraft Version: 1.8 Operating System: Windows 8.1 (amd64) version 6.3 Java Version: 1.8.0_92, Oracle Corporation Java VM Version: Java HotSpot 64-Bit Server VM (mixed mode), Oracle Corporation Memory: 908069384 bytes (866 MB) / 1037959168 bytes (989 MB) up to 1037959168 bytes (989 MB) JVM Flags: 3 total; -Xincgc -Xmx1024M -Xms1024M IntCache: cache: 0, tcache: 0, allocated: 0, tallocated: 0 FML: MCP v9.10 FML v8.0.99.99 Minecraft Forge 11.14.4.1563 5 mods loaded, 5 mods active States: 'U' = Unloaded 'L' = Loaded 'C' = Constructed 'H' = Pre-initialized 'I' = Initialized 'J' = Post-initialized 'A' = Available 'D' = Disabled 'E' = Errored UCHIJ mcp{9.05} [Minecraft Coder Pack] (minecraft.jar) UCHIJ FML{8.0.99.99} [Forge Mod Loader] (forgeSrc-1.8-11.14.4.1563.jar) UCHIJ Forge{11.14.4.1563} [Minecraft Forge] (forgeSrc-1.8-11.14.4.1563.jar) UCHIJ examplemod{1.0} [Example Mod] (bin) UCHIE nathantestmod{1.0} [nathantestmod] (bin) Loaded coremods (and transformers): GL info: ' Vendor: 'Intel' Version: '4.2.0 - Build 10.18.10.3308' Renderer: 'Intel® HD Graphics 4600' Launched Version: 1.8 LWJGL: 2.9.1 OpenGL: Intel® HD Graphics 4600 GL version 4.2.0 - Build 10.18.10.3308, Intel GL Caps: Using GL 1.3 multitexturing. Using GL 1.3 texture combiners. Using framebuffer objects because OpenGL 3.0 is supported and separate blending is supported. Shaders are available because OpenGL 2.1 is supported. VBOs are available because OpenGL 1.5 is supported. Using VBOs: No Is Modded: Definitely; Client brand changed to 'fml,forge' Type: Client (map_client.txt) Resource Packs: [] Current Language: English (US) Profiler Position: N/A (disabled)
-
gradlew setupDecompWorkspace not working with Eclipse Mars
Eighty8keyGuru replied to Eighty8keyGuru's topic in Modder Support
OH THANK GOD! It worked. A hearty thanks to all who helped! -
gradlew setupDecompWorkspace not working with Eclipse Mars
Eighty8keyGuru replied to Eighty8keyGuru's topic in Modder Support
Wait do you mean the one at C:\Users\[myusername] ? -
gradlew setupDecompWorkspace not working with Eclipse Mars
Eighty8keyGuru replied to Eighty8keyGuru's topic in Modder Support
Okay. Reinstalled eclipse, forge, and jdk. All correct versions: Eclipse IDE for Java EE Developers Windows x64. forge-1.8-11.14.4.1563-mdk. jdk-1.8_92 windows x64. SAME ISSUE. This is getting frustrating cause I've had this problem for the past week. Tried googling it, there isn't anything detailing this problem. You've been helpful and I appreciate it. Do you have any more suggestions, or a site you can link me that'll help? -
gradlew setupDecompWorkspace not working with Eclipse Mars
Eighty8keyGuru replied to Eighty8keyGuru's topic in Modder Support
I tried again. 1. I downloaded the recommended version of Forge mdk. (forge-1.8-11.14.4.1563-mdk) 2. I extracted it into the Programming parent file alongside eclipse (within its own folder) 3. I copied the contents into a folder separate for the mod. (my testmod folder) 4. I opened the testmod folder and ran the command: gradlew setupDecompWorkspace 5. Step four failed, so I tried the command in the original forge extracted folder. Still failed. Same error message too. -
gradlew setupDecompWorkspace not working with Eclipse Mars
Eighty8keyGuru replied to Eighty8keyGuru's topic in Modder Support
Ok, I copied all the files from the forge folder to a new folder on my desktop for the mod, but I've now tried running the cmd prompt from both folders (where .gradlew exists) and still it fails the "mergeJars" process. -
gradlew setupDecompWorkspace not working with Eclipse Mars
Eighty8keyGuru replied to Eighty8keyGuru's topic in Modder Support
Forgive me if I sound ignorant, I just want to make sure I understood you clearly. You said to create a separate folder for my mod stuff. Is this within the forge folder, or the programming folder (would it be Desktop/Programming/forge-1.8-11.14.3.1502-src/modstuff or would it be Desktop/Programming/modstuff)? Also, the command should still be run from the forge folder? -
gradlew setupDecompWorkspace not working with Eclipse Mars
Eighty8keyGuru replied to Eighty8keyGuru's topic in Modder Support
Ok, I just tried running it from the eclipse window and it didn't recognize the command. Here are my folder hierarchies: Desktop/Programming/Eclipse Desktop/Programming/Forge-1.8-11.14.3.1502-src (both of these unpacked from the original downloaded zip files) My original attempt was to go into the Forge folder mentioned above and run the command. Also, I just noticed, the MCP data version reads: snapshot_20141130. Don't know if that's useful or not. -
gradlew setupDecompWorkspace not working with Eclipse Mars
Eighty8keyGuru replied to Eighty8keyGuru's topic in Modder Support
So, if I'm to be using the "eclipse" folder within the "forge" unpacked folder, I need to do the command: gradlew setupDecompWorkspace within the "eclipse" folder? (What I mean by that is when I pull up eclipse, the directory I choose on the very first window is the eclipse folder within the forge. And yes I've unpacked both the ZIPs.) -
gradlew setupDecompWorkspace not working with Eclipse Mars
Eighty8keyGuru replied to Eighty8keyGuru's topic in Modder Support
I was just following the tutorial to the letter hoping I had spelled it wrong the first time or something. I've tried several times in 1.8 with no luck. And yes I've installed the jdk 1.8_91 for the correct windows x64. I even did the javac command to check and it came out fine. -
Hello. I am new to modding and following a tutorial for minecraft 1.8. The thing is, I can't even setup the forge gradle files because it is unsuccessful when I run the command: gradlew setupDecompWorkspace --refresh-dependencies I type it exactly as it is written here in a cmd prompt I opened inside the extracted forge folder. Note that I did place the extracted forge and eclipse folders under the same parent folder. The error message I'm getting after the "Powered by MCP" stuff is: :extractMcpData :getVersionJson :extractUserdev :genSrgs SKIPPED :extractNatives :getAssetsIndex :getAssets :makeStart [ant:javac] warning: [options] bootstrap class path not set in conjunction with -source 1.6 [ant:javac] 1 warning :downloadMcpTools SKIPPED :downloadClient SKIPPED downloadServer SKIPPED :mergeJars FAILED FAILURE: Build failed with an exception. *What went wrong: Execution failed for task ':mergeJars'. > java.util.zip.ZipException: error in opening zip file Then it suggests I try running with stacktrace, which I've tried but like I said, I'm new to modding so I don't understand any of it. The strange thing is that when I follow the tutorial and do the same exact command for forge 1.7.10, it works perfectly. It was fine until the tutorial started using methods that were newly implemented in 1.8. Sometimes I also get the same error but with the ":splitServerJar" process. What do these errors mean, and how can I fix? Thanks for any advice.