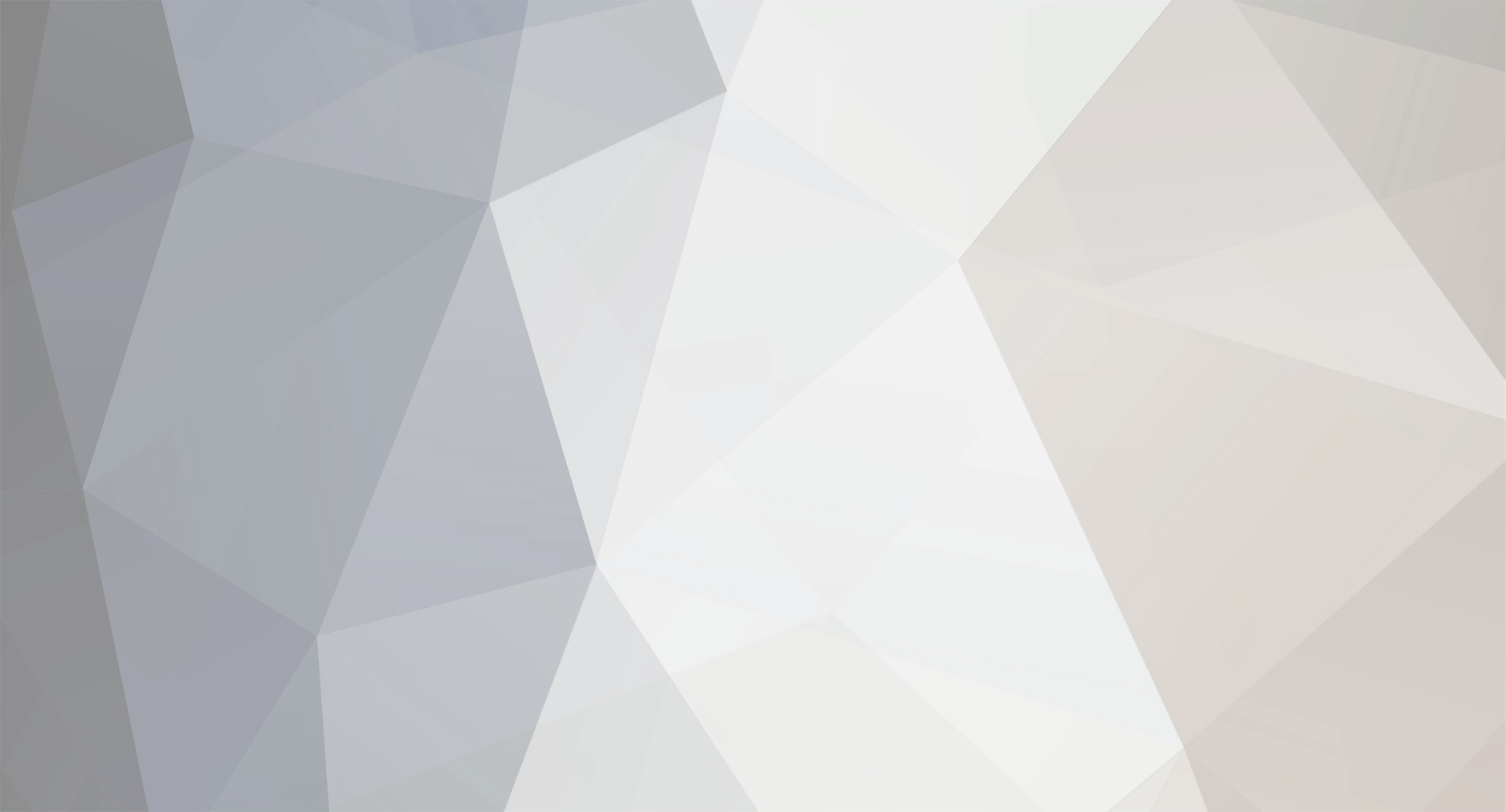
Komodo2013
-
Posts
24 -
Joined
-
Last visited
Posts posted by Komodo2013
-
-
I'm sorry... I've tried setting the unlocalized name and it is still crashing with the null pointer exception. Is this not where I would set it?
public class BettermentsBlocks { public static BlockBase marble; public static MarbleDoubleSlab marble_double_slab; public static MarbleSingleSlab marble_single_slab; public static MarbleStair marble_stair; public static void init() { marble = register(new BlockBase("marble", marble, Material.ROCK, 1.5f, 30f, 1, "Pickaxe", MapColor.SNOW).setCreativeTab(CreativeTabs.BUILDING_BLOCKS)); marble_double_slab = register(new MarbleDoubleSlab("marble_double_slab", marble_double_slab, Material.ROCK, 0.6f, 30f, 1, "Pickaxe", MapColor.SNOW)); marble_single_slab = register(new MarbleSingleSlab("marble_single_slab", marble_single_slab, Material.ROCK, 0.6f, 30f, 1, "Pickaxe", MapColor.SNOW)).setCreativeTab(CreativeTabs.BUILDING_BLOCKS); marble_stair = register(new MarbleStair(marble.getDefaultState(), "marble_stair", marble_stair, Material.ROCK, 1.5f, 30f, 1, "Pickaxe", MapColor.SNOW).setCreativeTab(CreativeTabs.BUILDING_BLOCKS)); } private static <T extends Block> T register(T block, ItemBlock itemBlock) { GameRegistry.register(block); GameRegistry.register(itemBlock); if (block instanceof BlockBase) { ((BlockBase)block).registerItemModel(itemBlock); } else if (block instanceof MarbleStair) { ((MarbleStair)block).registerItemModel(itemBlock); } return block; } private static <T extends Block> T registernoitemblock(T block) { GameRegistry.register(block); return block; } private static <T extends Block> T register(T block) { if(block instanceof MarbleSingleSlab) { GameRegistry.register(block); ItemSlab itemSlab = new ItemSlab(marble, marble_single_slab, marble_double_slab); //********* Right Here: ********** itemSlab.setUnlocalizedName(block.getUnlocalizedName()); itemSlab.setRegistryName(block.getRegistryName()); GameRegistry.register(itemSlab); ((MarbleSlab)block).registerItemModel(itemSlab); return block; } else if(!(block instanceof MarbleDoubleSlab)){ ItemBlock itemBlock = new ItemBlock(block); itemBlock.setRegistryName(block.getRegistryName()); return register(block, itemBlock); } else { return registernoitemblock(block); } } }
-
You don't have a json file named "marble_single_slab"
Right, sorry... As I try to debug, I occasionally change names of things as I re-do them. But that doesn't fix the null pointer exception...
-
I could really use some help creating this slab. I have tried moving the itemSlab as the last thing, and it is still not working. I don't see why Forge can't find the unlocalized name for the single slab block.
*Edit: just noticed I didn't post the error eclipse gave me: http://pastebin.com/Fm7JgAz0
-
Alright, fixed the issue... Turns out, all you need to do is to keep the meta at 0 and remove
("transform": "forge:default-block")
from your .json file.
-
I must have done something wrong, as now it doesn't show any texture or model for the icon.
Stair:
public class MarbleStair extends BlockStairs{ protected String name; protected MarbleStair(IBlockState modelState, String name, Block BlockIn, Material material, float hardness, float resistance, int harvest, String tool, MapColor color) { super(modelState); this.name = name; setUnlocalizedName(name); setRegistryName(name); setHardness(hardness); setResistance(resistance); setHarvestLevel(tool, harvest); useNeighborBrightness = true; } public void registerItemModel(ItemBlock itemBlock) { BettermentsMod.proxy.registerItemRenderer(itemBlock, 1, name); } @Override public MarbleStair setCreativeTab(CreativeTabs tab) { super.setCreativeTab(tab); return this; } }
ClientProxy:
public class ClientProxy extends CommonProxy{ @Override public void registerItemRenderer(Item item, int meta, String id) { ModelLoader.setCustomModelResourceLocation(item, meta, new ModelResourceLocation(Reference.MOD_ID + ":" + id, "inventory")); } }
-
Sorry I wasn't clear, the image for the item for the stairs is rotated about the y-axis by 90(or 270) degrees from what it should be. (sorry, I would post an image, but I am failing to find how to upload images)
-
There should be a simple fix for this, like a forge transformation for the block. Everything is working just fine, except that the icon for the stair is rotated 90 degrees, the inventory icon that is. In all other aspects, including handheld and 3rd person it is identical to vanilla stairs.
-
I've got the following issues with my slab:
They won't stack.
The metadata isn't being saved (upper slabs revert to lower once reloaded)
The item has no texture. (no info in log though, and all 3 blocks have their textures)
The double slab block won't drop two slabs - tried to fix this one by using the ItemSlab, which crashes the game.
Blocks class:
public class BettermentsBlocks { public static MarbleSlab marble_slab; public static MarbleDoubleSlab marble_double_slab; public static void init() { marble_double_slab = register(new MarbleDoubleSlab("marble_double_slab", marble_double_slab, Material.ROCK, 0.6f, 30f, 1, "Pickaxe", MapColor.SNOW)); marble_slab = register(new MarbleSlab("marble_slab", marble_slab, Material.ROCK, 0.6f, 30f, 1, "Pickaxe", MapColor.SNOW)).setCreativeTab(CreativeTabs.BUILDING_BLOCKS); } private static <T extends Block> T register(T block, ItemBlock itemBlock) { GameRegistry.register(block); GameRegistry.register(itemBlock); return block; } private static <T extends Block> T registerd(T block) { GameRegistry.register(block); return block; } private static <T extends Block> T register(T block, ItemSlab itemSlab){ GameRegistry.register(block); GameRegistry.register(itemSlab); ((MarbleSlab)block).registerItemModel(itemSlab); return block; } private static <T extends Block> T register(T block) { if(block instanceof MarbleSlab) { ItemSlab itemSlab = new ItemSlab(marble_slab, marble_slab, marble_double_slab); itemSlab.setRegistryName(Reference.MOD_ID + ":" + "marble_slab"); return register(block, itemSlab); } else if(!(block instanceof MarbleDoubleSlab)){ ItemBlock itemBlock = new ItemBlock(block); itemBlock.setRegistryName(block.getRegistryName()); return register(block, itemBlock); } else { return registerd(block); } } }
Slab and Double Slab class:
public class MarbleSlab extends BlockSlab { protected String name; public MarbleSlab(String name, Block BlockIn, Material material, float hardness, float resistance, int harvest, String tool, MapColor color) { super(material); IBlockState iblockstate = this.blockState.getBaseState(); if (!this.isDouble()){ iblockstate = iblockstate.withProperty(HALF, EnumBlockHalf.BOTTOM); } this.setDefaultState(iblockstate); this.name = name; setUnlocalizedName(name); setRegistryName(name); setHardness(hardness); setResistance(resistance); setHarvestLevel(tool, harvest); this.useNeighborBrightness = true; } @Override public String getUnlocalizedName(int meta) { return this.getLocalizedName(); } @Override public Comparable<?> getTypeForItem(ItemStack stack) { return false; } @Override public IProperty<?> getVariantProperty() { return null; } @Override public int damageDropped(IBlockState state) { return 0; } @Override public final IBlockState getStateFromMeta(final int meta) { IBlockState iblockstate = this.getDefaultState(); if (!this.isDouble()){ EnumBlockHalf value = EnumBlockHalf.BOTTOM; if ((meta & != 0){ value = EnumBlockHalf.TOP; } } return iblockstate; } @Override public final int getMetaFromState(final IBlockState state){ if (this.isDouble()){ return 0; } if ((EnumBlockHalf) state.getValue(HALF) == EnumBlockHalf.TOP){ return 8; } else { return 0; } } @Override protected final BlockStateContainer createBlockState(){ if(this.isDouble()){ return new BlockStateContainer(this, new IProperty[] {}); } else { return new BlockStateContainer(this, new IProperty[] {HALF}); } } public void registerItemModel(ItemSlab itemBlock) { BettermentsMod.proxy.registerItemRenderer(itemBlock, 0, name); } @Override public MarbleSlab setCreativeTab(CreativeTabs tab) { super.setCreativeTab(tab); return this; } @Override public MapColor getMapColor(IBlockState state){ return MapColor.SNOW; } @Override public boolean isDouble() { return false; } }
public class MarbleDoubleSlab extends MarbleSlab{ public MarbleDoubleSlab(String name, Block BlockIn, Material material, float hardness, float resistance, int harvest, String tool, MapColor color) { super(name, BlockIn, material, hardness, resistance, harvest, tool, color); } @Override public boolean isDouble() { return true; } }
.json files:
marble_slab
{ "forge_marker": 1, "variants": { "half=bottom": { "model": "betterments:marble_slab_half_bottom" }, "half=top": { "model": "betterments:marble_slab_half_top" }, "inventory": { "model": "betterments:marble_slab_half_bottom", "transform": "forge:default-block" } } }
marble_double_slab:
{ "forge_marker": 1, "defaults": { "textures": { "all": "betterments:blocks/dummy_side" } }, "variants": { "normal": { "model": "cube_all" }, "inventory": { "model": "cube_all" } } }
marble_slab_half_bottom:
{ "parent": "block/half_slab", "textures": { "bottom": "betterments:blocks/dummy_side", "top": "betterments:blocks/dummy_side", "side": "betterments:blocks/dummy_side" } }
marble_slab_half_top:
{ "parent": "block/upper_slab", "textures": { "bottom": "betterments:blocks/dummy_side", "top": "betterments:blocks/dummy_side", "side": "betterments:blocks/dummy_side" } }
And crash report for the code above:
-
All three of the methods I mentioned pass a World and BlockPos arguments.
They pass type "IBlockAccess", not type "World".
*NVM just casted IBlockAccess to World and it worked perfectly. It now explodes when on fire!
*Here's the code that is working:
public class GunpowderBarrel extends BlockTNT { protected String name; public GunpowderBarrel(String name, Block BlockIn, Material material, float hardness, float resistance, int harvest, String tool) { super(); this.name = name; setUnlocalizedName(name); setRegistryName(name); setHardness(hardness); setResistance(resistance); setHarvestLevel(tool, harvest); setSoundType(SoundType.WOOD); } @Override public void neighborChanged(IBlockState state, World worldIn, BlockPos pos, Block blockIn) { if (worldIn.isBlockPowered(pos)) { this.onBlockDestroyedByPlayer(worldIn, pos, state.withProperty(EXPLODE, Boolean.valueOf(true))); worldIn.setBlockToAir(pos); } } @Override public int getFlammability(IBlockAccess world, BlockPos pos, EnumFacing face){ this.onBlockDestroyedByExplosion((World) world, pos, null); System.out.println("getFlammability overriden"); return 300; } @Override public boolean isFlammable(IBlockAccess world, BlockPos pos, EnumFacing face){ System.out.println("isFlammable overriden"); return getFlammability(world, pos, face) > 0; } @Override public int getFireSpreadSpeed(IBlockAccess world, BlockPos pos, EnumFacing face){ System.out.println("getFireSpreadSpeed overriden"); return 300; } @SideOnly(Side.CLIENT) public BlockRenderLayer getBlockLayer() { return BlockRenderLayer.SOLID; } public void registerItemModel(ItemBlock itemBlock) { BettermentsMod.proxy.registerItemRenderer(itemBlock, 0, name); } @Override public GunpowderBarrel setCreativeTab(CreativeTabs tab) { super.setCreativeTab(tab); return this; } @Override public void onBlockDestroyedByExplosion(World worldIn, BlockPos pos, Explosion explosionIn) { if (!worldIn.isRemote) { worldIn.createExplosion(null, pos.getX(), pos.getY(), pos.getZ(), 10f, true); } } @Override public void explode(World worldIn, BlockPos pos, IBlockState state, EntityLivingBase igniter) { if (!worldIn.isRemote) { if (((Boolean)state.getValue(EXPLODE)).booleanValue()) { worldIn.createExplosion(null, pos.getX(), pos.getY(), pos.getZ(), 10f, true); } } } @Override public boolean onBlockActivated(World worldIn, BlockPos pos, IBlockState state, EntityPlayer playerIn, EnumHand hand, @Nullable ItemStack heldItem, EnumFacing side, float hitX, float hitY, float hitZ) { if (heldItem != null && (heldItem.getItem() == Items.FLINT_AND_STEEL || heldItem.getItem() == Items.FIRE_CHARGE)) { this.explode(worldIn, pos, state.withProperty(EXPLODE, Boolean.valueOf(true)), playerIn); worldIn.setBlockState(pos, Blocks.AIR.getDefaultState(), 11); if (heldItem.getItem() == Items.FLINT_AND_STEEL) { heldItem.damageItem(0, playerIn); } else if (!playerIn.capabilities.isCreativeMode) { --heldItem.stackSize; } return true; } else { return super.onBlockActivated(worldIn, pos, state, playerIn, hand, heldItem, side, hitX, hitY, hitZ); } } }
-
Thank you! That helps. I've overriden those functions, but have encountered another issue...The TNT Block extends the Block class, so you will be able to override the methods from the Block class which are not overridden in the TNT Block.
I don't see a way to either set its EXPLODE boolean to true, or to create the explosion. I need the World in order to create an explosion.
Obviously I forgot that tnt extended block, is there something else I'm missing?
-
Since I changed the infinite loop, and still had it crash, I thought that cx, which obtains its value from the bit shift, was the problem. (The best tutorial I could find used chunkx*16 method of finding the cords, and his worked). What you didn't see was the version were I changed all cx to chunkx*16 or commented them out. Once it started to work, I uncommented the references to cx, and simply haven't changed chunkx*16 to cx as this generator is a dummy generator, and thus I will be altering a different generator class.By the way, why are you using "chunkx*16" in one place, but "cx" in the others?
(I removed the actual generator out of the package so its presence didn't affect anything)
-
No. You override those methods on your TNT block so that when one of them is called you make it explode.
I can't override functions that aren't contained in the parent class. TNT does not use any of the functions getFlammability, isFlammable, or getFireSpreadSpeed. Fire does. I did try creating a getFlammability and isFlammable functions, within my TNT block, and they do nothing, because they are not even being called.
Since I'm obviously not understanding this, is it possible to see an example?
-
Yes, I realized I had made that mistake before. But you can see from my code that I've fixed it, and I still had it crash, I'm having difficulty trying to get it to crash again, so I can post the error report, but this is a good problem.x >> 4 is division.
x << 4 is multiplication.
Perhaps it simply decided to stop giving me grief?
-
In order to override that function, I need to create my own fire block (probably just copy, paste, and edit vanilla fire), right? But this block would then need to replace every instance of vanilla fire, both generated and placed. Does this require using ASM to inject the code, or is there a way to cause vanilla fire to reference my fire?
-
Alright, I got my code to work perfectly. I didn't realize that I had two "generate" functions
, so I fixed that, but it still didn't fix the error. For some reason, the game did not like using the block coordinates using the bit shift, but it is working just fine by simply multiplying them by 16. Anyway, here is my working code, which also has the working biome specific generation:
public class BettermentsWorldGenerator implements IWorldGenerator{ @Override public void generate(Random random, int chunkX, int chunkZ, World world, IChunkGenerator chunkGenerator, IChunkProvider chunkProvider) { int dim = world.provider.getDimension(); //System.out.printf("Betterments mod now loading for dimension %s", dim); if(dim == 0) {generateOverworld(random, chunkX, chunkZ, world, chunkGenerator, chunkProvider);} else if(dim == 1) {generateEnd(random, chunkX, chunkZ, world, chunkGenerator, chunkProvider);} else if(dim == -1) {generateNether(random, chunkX, chunkZ, world, chunkGenerator, chunkProvider);} } private void generateNether(Random random, int chunkX, int chunkZ, World world, IChunkGenerator chunkGenerator, IChunkProvider chunkProvider) { } private void generateEnd(Random random, int chunkX, int chunkZ, World world, IChunkGenerator chunkGenerator, IChunkProvider chunkProvider) { } private void generateOverworld(Random random, int chunkX, int chunkZ, World world, IChunkGenerator chunkGenerator, IChunkProvider chunkProvider) { int cx = chunkX << 4; int cz = chunkZ << 4; BlockPos pos = new BlockPos(cx, 70, cz); String s = world.getBiomeGenForCoords(pos).getBiomeName(); System.out.println(s); //Vanilla minecraft addition generateSeam(Blocks.IRON_ORE.getDefaultState(), world, random, chunkX*16, chunkZ*16, 1, 128, 32, 10, Blocks.STONE); //Biome specific spawns if(s.contains("Desert")) { generateSeam(Blocks.EMERALD_ORE.getDefaultState(), world, random, cx, cz, 1, 64, 16, 1, Blocks.STONE); } generateSeam(BettermentsBlocks.marble.getDefaultState(), world, random, cx, cz, 1, 128, 64, 3, Blocks.STONE); } private void generateSeam(IBlockState block, World world, Random random, int cx, int cz, int minY, int maxY, int size, int tries, Block inside){ int dy = maxY - minY; if (minY < 0 || maxY > 256 || dy < 0) { throw new IllegalArgumentException("Illegal Height Arguments for WorldGenerator"); } for (int i = 0; i<tries; i++){ int rx = cx + random.nextInt(15); int rz = cz + random.nextInt(15); int y = minY + random.nextInt(dy); //System.out.println(tries - i); if(y==0){y=1;} BlockPos pos = new BlockPos(rx, y, rz); WorldGenMinable generator = new WorldGenMinable(block, size, BlockMatcher.forBlock(inside)); generator.generate(world, random, pos); //System.out.println("Betterments World Generator called."); } } }
*Edit: Apparently I need to work on noticing my variables, but I now see that I latter use the bit shifted coordinates, and it was still working fine. I don't understand what fixed the crashes that were still occurring after I fixed my duplicate functions.
-
-
Modified again, but still isn't working. If I shift the bits the other way, then it crashes.
public class BettermentsWorldGenerator implements IWorldGenerator{ @Override public void generate(Random random, int chunkX, int chunkZ, World world, IChunkGenerator chunkGenerator, IChunkProvider chunkProvider) { int dim = world.provider.getDimension(); //System.out.printf("Betterments mod now loading for dimension %s", dim); if(dim == 0) {generateOverworld(random, chunkX, chunkZ, world, chunkGenerator, chunkProvider);} else if(dim == 1) {generateEnd(random, chunkX, chunkZ, world, chunkGenerator, chunkProvider);} else if(dim == -1) {generateNether(random, chunkX, chunkZ, world, chunkGenerator, chunkProvider);} } private void generateNether(Random random, int chunkX, int chunkZ, World world, IChunkGenerator chunkGenerator, IChunkProvider chunkProvider) { } private void generateEnd(Random random, int chunkX, int chunkZ, World world, IChunkGenerator chunkGenerator, IChunkProvider chunkProvider) { } private void generateOverworld(Random random, int chunkX, int chunkZ, World world, IChunkGenerator chunkGenerator, IChunkProvider chunkProvider) { int cx = chunkX >> 4; int cz = chunkZ >> 4; BlockPos pos = new BlockPos(cx, 70, cz); String s = world.getBiomeGenForCoords(pos).getBiomeName(); System.out.println(s); //Vanilla minecraft addition generate(Blocks.IRON_ORE.getDefaultState(), world, random, cx, cz, 1, 128, 32, 10, Blocks.STONE); //Biome specific spawns if(s.contains("Desert")) { generate(Blocks.EMERALD_ORE.getDefaultState(), world, random, cx, cz, 1, 64, 16, 1, Blocks.STONE); } generate(BettermentsBlocks.marble.getDefaultState(), world, random, cx, cz, 1, 128, 64, 3, Blocks.STONE); } private void generate(IBlockState block, World world, Random random, int cx, int cz, int minY, int maxY, int size, int tries, Block inside){ int dy = maxY - minY; if (minY < 0 || maxY > 256 || dy < 0) { throw new IllegalArgumentException("Illegal Height Arguments for WorldGenerator"); } for (int i = 0; i<tries; i++){ cx = cx + random.nextInt(16); cz = cz + random.nextInt(16); int y = minY + random.nextInt(dy); if(y==0){y=1;} BlockPos pos = new BlockPos(cx, y, cz); WorldGenMinable generator = new WorldGenMinable(block, size, BlockMatcher.forBlock(inside)); generator.generate(world, random, pos); //System.out.println("Betterments World Generator called."); } } }
-
*Face palm*
Implemented that change:
public class BettermentsWorldGenerator implements IWorldGenerator{ @Override public void generate(Random random, int chunkX, int chunkZ, World world, IChunkGenerator chunkGenerator, IChunkProvider chunkProvider) { int dim = world.provider.getDimension(); //System.out.printf("Betterments mod now loading for dimension %s", dim); if(dim == 0) {generateOverworld(random, chunkX, chunkZ, world, chunkGenerator, chunkProvider);} else if(dim == 1) {generateEnd(random, chunkX, chunkZ, world, chunkGenerator, chunkProvider);} else if(dim == -1) {generateNether(random, chunkX, chunkZ, world, chunkGenerator, chunkProvider);} } private void generateNether(Random random, int chunkX, int chunkZ, World world, IChunkGenerator chunkGenerator, IChunkProvider chunkProvider) { } private void generateEnd(Random random, int chunkX, int chunkZ, World world, IChunkGenerator chunkGenerator, IChunkProvider chunkProvider) { } private void generateOverworld(Random random, int chunkX, int chunkZ, World world, IChunkGenerator chunkGenerator, IChunkProvider chunkProvider) { BlockPos pos = new BlockPos(chunkX * 16, 70, chunkZ * 16); String s = world.getBiomeGenForCoords(pos).getBiomeName(); System.out.println(s); //Vanilla minecraft addition generate(Blocks.IRON_ORE.getDefaultState(), world, random, chunkX, chunkZ, 1, 128, 32, 10, Blocks.STONE); //Biome specific spawns if(s.contains("Desert")) { generate(Blocks.EMERALD_ORE.getDefaultState(), world, random, chunkX, chunkZ, 1, 64, 16, 1, Blocks.STONE); } generate(BettermentsBlocks.marble.getDefaultState(), world, random, chunkX, chunkZ, 1, 128, 64, 3, Blocks.STONE); } private void generate(IBlockState block, World world, Random random, int x, int z, int minY, int maxY, int size, int tries, Block inside){ int cx = x >> 4; int cz = z >> 4; int dy = maxY - minY; if (minY < 0 || maxY > 256 || dy < 0) { throw new IllegalArgumentException("Illegal Height Arguments for WorldGenerator"); } for (int i = 0; i<tries; i++){ x = x + random.nextInt(16); z = z + random.nextInt(16); int y = minY + random.nextInt(dy); if(y==0){y=1;} BlockPos pos = new BlockPos(cx, y, cz); WorldGenMinable generator = new WorldGenMinable(block, size, BlockMatcher.forBlock(inside)); generator.generate(world, random, pos); //System.out.println("Betterments World Generator called."); } } }
But it still doesn't do anything.
-
I've created a world generator, successfully registered it, and it is being called (as is evidence as the system log shows the print lines). Despite this, the generator itself is not working.
Code:
public class BettermentsWorldGenerator implements IWorldGenerator{ @Override public void generate(Random random, int chunkX, int chunkZ, World world, IChunkGenerator chunkGenerator, IChunkProvider chunkProvider) { int dim = world.provider.getDimension(); //System.out.printf("Betterments mod now loading for dimension %s", dim); if(dim == 0) {generateOverworld(random, chunkX, chunkZ, world, chunkGenerator, chunkProvider);} else if(dim == 1) {generateEnd(random, chunkX, chunkZ, world, chunkGenerator, chunkProvider);} else if(dim == -1) {generateNether(random, chunkX, chunkZ, world, chunkGenerator, chunkProvider);} } private void generateNether(Random random, int chunkX, int chunkZ, World world, IChunkGenerator chunkGenerator, IChunkProvider chunkProvider) { } private void generateEnd(Random random, int chunkX, int chunkZ, World world, IChunkGenerator chunkGenerator, IChunkProvider chunkProvider) { } private void generateOverworld(Random random, int chunkX, int chunkZ, World world, IChunkGenerator chunkGenerator, IChunkProvider chunkProvider) { BlockPos pos = new BlockPos(chunkX * 16, 70, chunkZ * 16); String s = world.getBiomeGenForCoords(pos).getBiomeName(); System.out.println(s); //Vanilla minecraft addition generate(Blocks.IRON_ORE.getDefaultState(), world, random, chunkX, chunkZ, 1, 128, 32, 10, Blocks.STONE); //Biome specific spawns if(s.contains("Desert")) { generate(Blocks.EMERALD_ORE.getDefaultState(), world, random, chunkX, chunkZ, 1, 64, 16, 1, Blocks.STONE); } generate(BettermentsBlocks.marble.getDefaultState(), world, random, chunkX, chunkZ, 1, 128, 64, 3, Blocks.STONE); } private void generate(IBlockState block, World world, Random random, int x, int z, int minY, int maxY, int size, int tries, Block inside){ int dy = maxY - minY; if (minY < 0 || maxY > 256 || dy < 0) { throw new IllegalArgumentException("Illegal Height Arguments for WorldGenerator"); } for (int i = 0; i<tries; i++){ x = x + random.nextInt(16); z = z + random.nextInt(16); int y = minY + random.nextInt(dy); if(y==0){y=1;} BlockPos pos = new BlockPos(x, y, z); WorldGenMinable generator = new WorldGenMinable(block, size, BlockMatcher.forBlock(inside)); generator.generate(world, random, pos); //System.out.println("Betterments World Generator called."); } } }
-
You could try to override the getFlammability method. It wouldn't be perfect, but you could randomly explode when your block's getFlammability is checked. Sometimes it will, and sometimes it will be consumed before it can. You could even return zero (never catches vanilla fire), reserving all randomness to your own explosion. That might even mimic TNT correctly.
I've tried that already... It seems to do nothing. Unless you are referencing overriding some function, as getFlammablility cannot be overridden (TNT doesn't use it).
-
Thanks, but I'm having trouble looking for a tutorial on how to replace a vanilla block/Item with my own block, that isn't for MC 1.7.10 or earlier. If you know of such a tutorial, I would greatly appreciate a link to it!
Additionally, core modding doesn't much appeal to me... but if that is the only solution, as it seems there isn't an easy way of doing this, then I am happy to give it a try...
-
Sorry I wasn't clear... The block burns just fine... Just doesn't blow up once fire consumes it. I tried that bit of code, and nothing changed (also its deprecated). Sorry, here's my code for said block:
public class GunpowderBlock extends BlockTNT {
protected String name;
public GunpowderBlock(String name, Block BlockIn, Material material, float hardness, float resistance, int harvest, String tool) {
super();
this.name = name;
setUnlocalizedName(name);
setRegistryName(name);
setHardness(hardness);
setResistance(resistance);
setHarvestLevel(tool, harvest);
setSoundType(SoundType.SAND);
}
public int getFlammablility(IBlockAccess world, BlockPos pos, EnumFacing face) {
return net.minecraft.init.Blocks.FIRE.getFlammability(this);
}
public void registerItemModel(ItemBlock itemBlock) {
BettermentsMod.proxy.registerItemRenderer(itemBlock, 0, name);
}
@Override
public GunpowderBlock setCreativeTab(CreativeTabs tab) {
super.setCreativeTab(tab);
return this;
}
@Override
public void onBlockDestroyedByExplosion(World worldIn, BlockPos pos, Explosion explosionIn)
{
if (!worldIn.isRemote)
{
EntityTNTPrimed entitytntprimed = new EntityTNTPrimed(worldIn, (double)((float)pos.getX() + 0.5F), (double)pos.getY(), (double)((float)pos.getZ() + 0.5F), explosionIn.getExplosivePlacedBy());
entitytntprimed.setFuse((short)(0));
worldIn.spawnEntityInWorld(entitytntprimed);
}
}
@Override
public void explode(World worldIn, BlockPos pos, IBlockState state, EntityLivingBase igniter)
{
if (!worldIn.isRemote)
{
if (((Boolean)state.getValue(EXPLODE)).booleanValue())
{
EntityTNTPrimed entitytntprimed = new EntityTNTPrimed(worldIn, (double)((float)pos.getX() + 0.5F), (double)pos.getY(), (double)((float)pos.getZ() + 0.5F), igniter);
entitytntprimed.setFuse((short)(0));
worldIn.spawnEntityInWorld(entitytntprimed);
worldIn.playSound((EntityPlayer)null, entitytntprimed.posX, entitytntprimed.posY, entitytntprimed.posZ, SoundEvents.ENTITY_TNT_PRIMED, SoundCategory.BLOCKS, 1.0F, 1.0F);
}
}
}
@Override
public boolean onBlockActivated(World worldIn, BlockPos pos, IBlockState state, EntityPlayer playerIn, EnumHand hand, @Nullable ItemStack heldItem, EnumFacing side, float hitX, float hitY, float hitZ)
{
if (heldItem != null && (heldItem.getItem() == Items.FLINT_AND_STEEL || heldItem.getItem() == Items.FIRE_CHARGE))
{
this.explode(worldIn, pos, state.withProperty(EXPLODE, Boolean.valueOf(true)), playerIn);
worldIn.setBlockState(pos, Blocks.AIR.getDefaultState(), 11);
if (heldItem.getItem() == Items.FLINT_AND_STEEL)
{
heldItem.damageItem(0, playerIn);
}
else if (!playerIn.capabilities.isCreativeMode)
{
--heldItem.stackSize;
}
return true;
}
else
{
return super.onBlockActivated(worldIn, pos, state, playerIn, hand, heldItem, side, hitX, hitY, hitZ);
}
}
}
-
I have a block which extends TNT and is working perfectly except that when fire burns it, it does not explode. I believe that I see the solution, adding my block to a specific if statement within the Fire class.
Is this the only way to make it so when fire destroys this block, then it Ignites? If so, how would one go about overriding this code?
-note: I am just using the standard lit TNT entity, with 0 fuse time, to make the explosion, and am happy to leave it as such.
[MC 1.10] A few Issues with slabs
in Modder Support
Posted
Alright, so I've realized that the block that I am using is the standard block. I also fixed most things, but I still have four issues that I can't seem to resolve:
But the game no longer crashes, so that's a plus. The game is also placing marble_slab blocks, not marble_single_slab. I don't know if this is a problem or not. Updated codes:
Blocks File:
MarbleSlab:
MarbleDoubleSlab:
MarbleSingleSlab:
MarbleSlabItem:
marble_slab.json (marble_single_slab.json is the same, but the game is not using it):