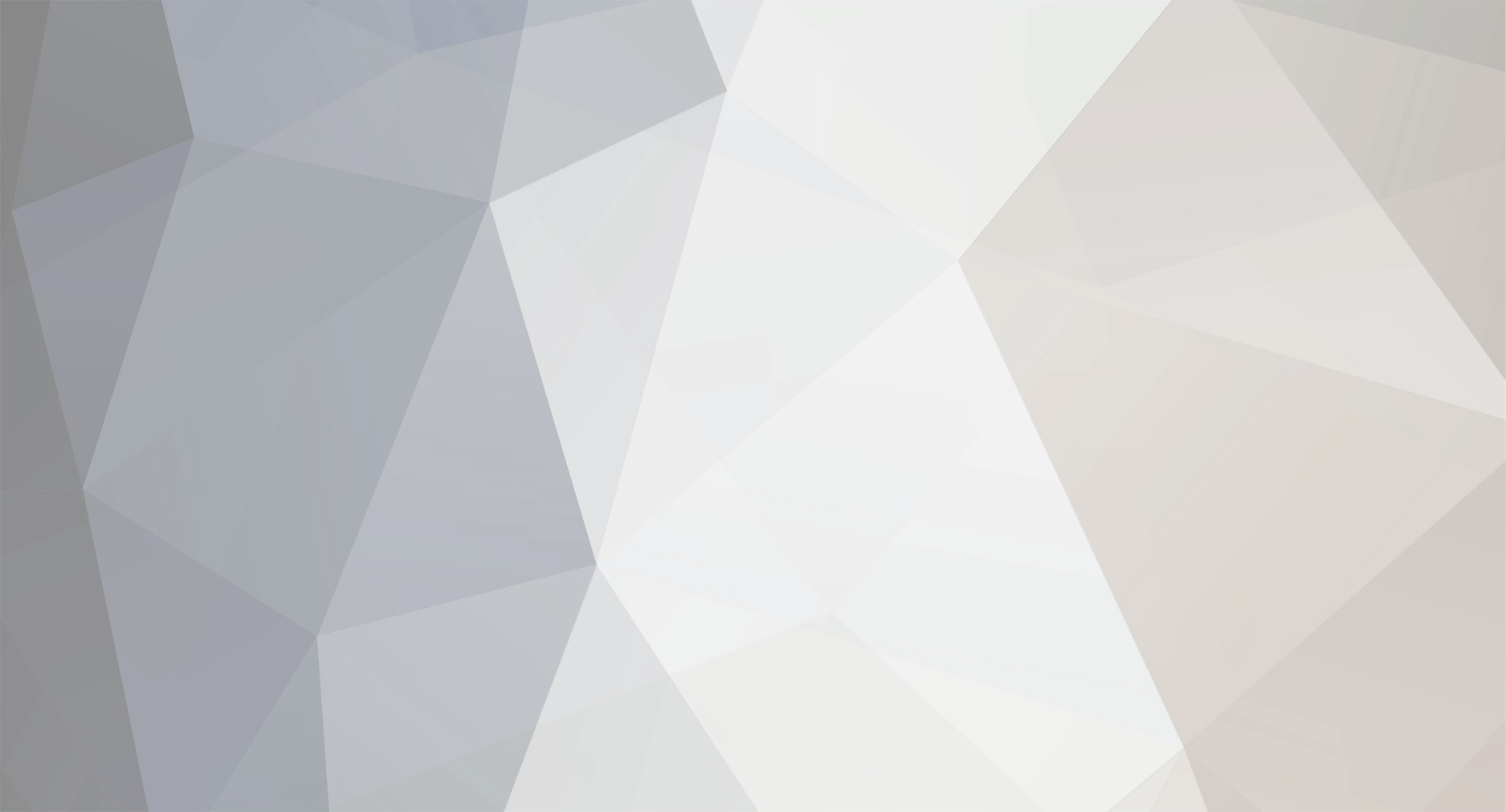
chipoodle
-
Posts
10 -
Joined
-
Last visited
Posts posted by chipoodle
-
-
I have a block already registered in my mod, which I have used in some worlds. The problem arises when in code, I add a property called CURRENT_AGE, when running Minecraft it freezes. In the console it doesn't appear any excpetion except that it stays in this phase:
[Render thread/DEBUG] [ne.mi.co.ca.CapabilityManager/CAPABILITIES]: Attempting to automatically register: Lnet/minecraftforge/items/IItemHandler;
Does anyone have an idea what it could be? I show the block and its registration
public class SoulLichenBlock extends MultifaceBlock implements SimpleWaterloggedBlock, EntityBlock { public static final BooleanProperty WATERLOGGED = BlockStateProperties.WATERLOGGED; public static final IntegerProperty SKILL_LEVEL = IntegerProperty.create("soullichen_level", 0, 30); public static final DirectionProperty FACE = DirectionProperty.create("soullichen_face"); public static final DirectionProperty DIRECTION = DirectionProperty.create("soullichen_direction"); public static final IntegerProperty CURRENT_AGE = BlockStateProperties.AGE_25; private final MultifaceSpreader spreader = new MultifaceSpreader(this); private final MultifaceSpreader.DefaultSpreaderConfig config = new MultifaceSpreader.DefaultSpreaderConfig(this); private LivingEntity owner; //private static final Integer MAX_AGE = 25; public SoulLichenBlock(Properties properties) { super(properties); this.registerDefaultState(this .defaultBlockState() .setValue(WATERLOGGED, Boolean.FALSE) .setValue(SKILL_LEVEL, 0) .setValue(FACE, Direction.DOWN) .setValue(DIRECTION, Direction.DOWN) .trySetValue(CURRENT_AGE, 0) ); } public static ToIntFunction<BlockState> emission(int p_181223_) { return (p_181221_) -> MultifaceBlock.hasAnyFace(p_181221_) ? p_181223_ : 0; } public static boolean hasFace(BlockState p_153901_, @NotNull Direction p_153902_) { BooleanProperty booleanproperty = getFaceProperty(p_153902_); return p_153901_.hasProperty(booleanproperty) && p_153901_.getValue(booleanproperty); } protected void createBlockStateDefinition(StateDefinition.@NotNull Builder<Block, BlockState> stateDefinition) { stateDefinition.add(WATERLOGGED).add(SKILL_LEVEL).add(FACE).add(DIRECTION).add(CURRENT_AGE); super.createBlockStateDefinition(stateDefinition); } public @NotNull BlockState updateShape(BlockState p_153302_, @NotNull Direction p_153303_, @NotNull BlockState p_153304_, @NotNull LevelAccessor p_153305_, @NotNull BlockPos p_153306_, @NotNull BlockPos p_153307_) { if (p_153302_.getValue(WATERLOGGED)) { p_153305_.scheduleTick(p_153306_, Fluids.WATER, Fluids.WATER.getTickDelay(p_153305_)); } return super.updateShape(p_153302_, p_153303_, p_153304_, p_153305_, p_153306_, p_153307_); } @SuppressWarnings("deprecation") public @NotNull FluidState getFluidState(BlockState fluidState) { return fluidState.getValue(WATERLOGGED) ? Fluids.WATER.getSource(false) : super.getFluidState(fluidState); } public boolean propagatesSkylightDown(BlockState p_181225_, @NotNull BlockGetter blockGetter, @NotNull BlockPos blockPos) { return p_181225_.getFluidState().isEmpty(); } public @NotNull MultifaceSpreader getSpreader() { return this.spreader; } public Optional<MultifaceSpreader.SpreadPos> spreadFromRandomFaceTowardRandomDirection( BlockState p_221620_, LevelAccessor p_221621_, BlockPos p_221622_, RandomSource p_221623_, int skillPoints, int age) { return Direction.allShuffled(p_221623_).stream().filter((p_221680_) -> { return this.config.canSpreadFrom(p_221620_, p_221680_); }).map((p_221629_) -> { return this.spreadFromFaceTowardRandomDirection(p_221620_, p_221621_, p_221622_, p_221629_, p_221623_, false, skillPoints, age); }).filter(Optional::isPresent).findFirst().orElse(Optional.empty()); } public Optional<MultifaceSpreader.SpreadPos> spreadFromFaceTowardRandomDirection( BlockState blockState, LevelAccessor levelAccessor, BlockPos blockPos, Direction face, RandomSource randomSource, boolean aBoolean, int skillPoints, int age) { return Direction.allShuffled(randomSource).stream().map((direction) -> spreadFromFaceTowardDirection(blockState, levelAccessor, blockPos, face, direction, aBoolean, skillPoints, age)) .filter(Optional::isPresent) .findFirst() .orElse(Optional.empty()); } public Optional<MultifaceSpreader.SpreadPos> spreadFromFaceTowardDirection( BlockState blockState, LevelAccessor levelAccessor, BlockPos blockPos, Direction face, Direction direction, boolean aBoolean, int skillPoints, int age) { //DevilRpg.LOGGER.debug("BEGIN ==================================== spreadFromFaceTowardDirection skillPoints {}", skillPoints); return skillPoints < 0 ? Optional.empty() : getSpreadFromFaceTowardDirection(blockState, levelAccessor, blockPos, face, direction, this::canSpreadInto) .flatMap((spreadPos) -> { //DevilRpg.LOGGER.debug("END ================================ spreadFromFaceTowardDirection spreadPos {}", spreadPos); return this.spreadToFace(levelAccessor, spreadPos, aBoolean, skillPoints, direction, age); }); } public boolean canSpreadInto(BlockGetter p_221685_, BlockPos p_221686_, MultifaceSpreader.SpreadPos p_221687_) { BlockState blockstate = p_221685_.getBlockState(p_221687_.pos()); return this.stateCanBeReplaced(p_221685_, p_221686_, p_221687_.pos(), p_221687_.face(), blockstate) && isValidStateForPlacement(p_221685_, blockstate, p_221687_.pos(), p_221687_.face()); } protected boolean stateCanBeReplaced(BlockGetter p_221688_, BlockPos p_221689_, BlockPos p_221690_, Direction p_221691_, BlockState p_221692_) { return p_221692_.isAir() || p_221692_.is(this) || p_221692_.is(Blocks.WATER) && p_221692_.getFluidState().isSource(); } public Optional<MultifaceSpreader.SpreadPos> getSpreadFromFaceTowardDirection(BlockState blockState, BlockGetter blockGetter, BlockPos blockPos, Direction face, Direction direction, MultifaceSpreader.SpreadPredicate spreadPredicate) { //DevilRpg.LOGGER.debug("--- getSpreadFromFaceTowardDirection direction.getAxis() == face.getAxis(): {}", direction.getAxis() == face.getAxis()); ArrayList<Direction> directions = new ArrayList<>(); directions.add(direction); if (direction.getAxis() == face.getAxis()) { if (direction.getAxis().isHorizontal()) { directions = Arrays.stream(Direction.values()).filter(dir -> dir.getAxis().isVertical()).collect(Collectors.toCollection(ArrayList::new)); } if (direction.getAxis().isVertical()) { directions = Arrays.stream(Direction.values()).filter(dir -> dir.getAxis().isHorizontal()).collect(Collectors.toCollection(ArrayList::new)); } } for (Direction directionElement : directions) { /*DevilRpg.LOGGER.debug("--->> getSpreadFromFaceTowardDirection config.isOtherBlockValidAsSource(blockState) {} || " + "hasFace(blockState, face) {} && " + "!hasFace(blockState, direction) {}", config.isOtherBlockValidAsSource(blockState), hasFace(blockState, face), !hasFace(blockState, directionElement));*/ if (config.isOtherBlockValidAsSource(blockState) || hasFace(blockState, face) && !hasFace(blockState, directionElement)) { for (MultifaceSpreader.SpreadType multifacespreader$spreadtype : config.getSpreadTypes()) { MultifaceSpreader.SpreadPos multifacespreader$spreadpos = multifacespreader$spreadtype.getSpreadPos(blockPos, directionElement, face); //DevilRpg.LOGGER.debug("--- test SpreadPos: {} direction {} face {} ", multifacespreader$spreadpos, directionElement, face); if (spreadPredicate.test(blockGetter, blockPos, multifacespreader$spreadpos)) { //DevilRpg.LOGGER.debug("--- spreadPredicate success:"); return Optional.of(multifacespreader$spreadpos); } } } } return Optional.empty(); } public boolean isValidStateForPlacement(@NotNull BlockGetter blockGetter, @NotNull BlockState blockState, @NotNull BlockPos blockPos, @NotNull Direction face) { //DevilRpg.LOGGER.debug("------ isValidStateForPlacement 1st condition: {} && ({} || {})", this.isFaceSupported(face), !blockState.is(this), !hasFace(blockState, face)); if (this.isFaceSupported(face) && (!blockState.is(this) || !hasFace(blockState, face))) { BlockPos blockpos = blockPos.relative(face); //DevilRpg.LOGGER.debug("------ isValidStateForPlacement 2nd condition: canAttachTo {} ", secondCondition); return canAttachTo(blockGetter, face, blockpos, blockGetter.getBlockState(blockpos)); } else { return false; } } @Nullable public BlockState getStateForPlacement(@NotNull BlockState blockState, @NotNull BlockGetter blockGetter, @NotNull BlockPos blockPos, @NotNull Direction face, int skillPoints, Direction direction, int age) { //DevilRpg.LOGGER.debug("--- getStateForPlacement"); boolean isNotValidStateForPlacement = !this.isValidStateForPlacement(blockGetter, blockState, blockPos, face); //DevilRpg.LOGGER.debug("------- isNotValidStateForPlacement: {}", isNotValidStateForPlacement); if (isNotValidStateForPlacement) { return null; } else { BlockState blockstate; if (blockState.is(this)) { blockstate = blockState; } else if (this.isWaterloggable() && blockState.getFluidState().isSourceOfType(Fluids.WATER)) { blockstate = this.defaultBlockState().setValue(BlockStateProperties.WATERLOGGED, Boolean.TRUE); } else { blockstate = this.defaultBlockState(); } //DevilRpg.LOGGER.debug("------- getStateForPlacement -> blockStateResult "); return blockstate .setValue(getFaceProperty(face), Boolean.TRUE) .setValue(SKILL_LEVEL, skillPoints).setValue(FACE, face) .setValue(DIRECTION, direction) .setValue(CURRENT_AGE,age) ; } } public Optional<MultifaceSpreader.SpreadPos> spreadToFace(LevelAccessor levelAccessor, MultifaceSpreader.SpreadPos spreadPos, boolean p_221596_, int skillPoints, Direction direction, int age) { BlockState blockstate = levelAccessor.getBlockState(spreadPos.pos()); //DevilRpg.LOGGER.debug("---> spreadToFace blockstate{} direction: {}", blockstate, direction); return this.placeBlock(levelAccessor, spreadPos, blockstate, p_221596_, skillPoints, direction, age) ? Optional.of(spreadPos) : Optional.empty(); } public boolean placeBlock(LevelAccessor p_221702_, MultifaceSpreader.SpreadPos p_221703_, BlockState p_221704_, boolean p_221705_, int skillPoints, Direction direction, int age) { //DevilRpg.LOGGER.debug("---> placeBlock {} direction {} ", p_221703_, direction); BlockState blockstate = this.getStateForPlacement(p_221704_, p_221702_, p_221703_.pos(), p_221703_.face(), skillPoints, direction, age); if (blockstate != null) { if (p_221705_) { p_221702_.getChunk(p_221703_.pos()).markPosForPostprocessing(p_221703_.pos()); } //DevilRpg.LOGGER.debug("------> setBlock"); return p_221702_.setBlock(p_221703_.pos(), blockstate, 2); } else { return false; } } public long spreadFromFaceTowardAllDirections( BlockState blockState, LevelAccessor levelAccessor, BlockPos blockPos, Direction face, boolean aBoolean, int skillPoints, int age) { return Direction.stream().map((p_221656_) -> spreadFromFaceTowardDirection(blockState, levelAccessor, blockPos, face, p_221656_, aBoolean, skillPoints, age)) .filter(Optional::isPresent).count(); } private boolean isWaterloggable() { return this.stateDefinition.getProperties().contains(BlockStateProperties.WATERLOGGED); } @Override public void setPlacedBy(@NotNull Level level, @NotNull BlockPos blockPos, @NotNull BlockState blockState, @Nullable LivingEntity livingEntity, @NotNull ItemStack itemStack) { super.setPlacedBy(level, blockPos, blockState, livingEntity, itemStack); this.setOwner(livingEntity); } public LivingEntity getOwner() { return this.owner; } private void setOwner(LivingEntity livingEntity) { this.owner = livingEntity; } @Deprecated @Override public void entityInside(@NotNull BlockState blockState, @NotNull Level level, @NotNull BlockPos blockPos, @NotNull Entity entity) { if (entity instanceof LivingEntity /*&& entity.getType() != EntityType.BEE*/ && entity.getType() != ModEntities.LICHEN_SEEDBALL.get()) { entity.makeStuckInBlock(blockState, new Vec3(0.8D, 0.75D, 0.8D)); if (!level.isClientSide /*&& (entity.xOld != entity.getX() || entity.zOld != entity.getZ())*/) { // double d0 = Math.abs(entity.getX() - entity.xOld); // double d1 = Math.abs(entity.getZ() - entity.zOld); // if (d0 >= (double) 0.003F || d1 >= (double) 0.003F) { entity.hurt(level.damageSources().playerAttack((Player) owner), 1.0F); // Aplicar aceleración al movimiento double speedBoost = -0.4; // Ajusta este valor según lo rápido que quieras que sea el impulso double motionX = entity.getX() - entity.xOld; double motionZ = entity.getZ() - entity.zOld; double speed = Math.sqrt(motionX * motionX + motionZ * motionZ); //if (speed > 0.0) { entity.setDeltaMovement(entity.getDeltaMovement().multiply( (motionX / speed) * speedBoost, 0.0, (motionZ / speed) * speedBoost )); // } //} } } } @Nullable @Override public BlockEntity newBlockEntity(@NotNull BlockPos pos, @NotNull BlockState state) { return ModEntityBlocks.SOUL_LICHEN_ENTITY_BLOCK.get().create(pos, state); } @Nullable @Override public <T extends BlockEntity> BlockEntityTicker<T> getTicker(Level level, @NotNull BlockState blockState, @NotNull BlockEntityType<T> type) { return level.isClientSide ? null : (alevel, pos, aBlockstate, blockEntity) -> { if (blockEntity instanceof SoulLichenBlockEntity soulLichenBlockEntity && alevel.getGameTime() % 5 == 0) { soulLichenBlockEntity.tick(blockState, (ServerLevel) alevel, pos, alevel.getRandom()); //DevilRpg.LOGGER.info("-------->tick. this: {}", this.getClass().getSimpleName()); } }; } }
This is the registration:
public final class ModBlocks { public static final DeferredRegister<Block> BLOCKS = DeferredRegister.create(ForgeRegistries.BLOCKS, DevilRpg.MODID); ... public static final RegistryObject<SoulLichenBlock> SOUL_LICHEN_BLOCK = BLOCKS.register("soullichen", () -> new SoulLichenBlock( Block.Properties.copy(Blocks.GLOW_LICHEN).lightLevel(SoulLichenBlock.emission(7)).randomTicks() )); }
-
Hi folks,
I'm trying to generate a custom village, so i called in my proxy this:
public class MapGenEventHandler { public static void register() { MinecraftForge.TERRAIN_GEN_BUS.register(new MapGenEventHandler()); } @SubscribeEvent(priority = EventPriority.LOWEST) public void initMapGen(InitMapGenEvent event) { event.setNewGen(new MapGenRogueVillage()); } }
Next i extended MapGenStructure
public class MapGenRogueVillage extends MapGenStructure { ... }
Then i just copied vanilla class StructureVillagePieces into my class StructureRogueVillagePieces. But in the method:
public static List<StructureRogueVillagePieces.PieceWeight> getStructureVillageWeightedPieceList(Random random, int size) { List<StructureRogueVillagePieces.PieceWeight> list = Lists.<StructureRogueVillagePieces.PieceWeight>newArrayList(); list.add(new StructureRogueVillagePieces.PieceWeight(StructureRogueVillagePieces.House4Garden.class, 4, MathHelper.getInt(random, 2 + size, 4 + size * 2))); list.add(new StructureRogueVillagePieces.PieceWeight(StructureRogueVillagePieces.Church.class, 20, MathHelper.getInt(random, 0 + size, 1 + size))); list.add(new StructureRogueVillagePieces.PieceWeight(StructureRogueVillagePieces.House1.class, 20, MathHelper.getInt(random, 0 + size, 2 + size))); list.add(new StructureRogueVillagePieces.PieceWeight(StructureRogueVillagePieces.WoodHut.class, 3, MathHelper.getInt(random, 2 + size, 5 + size * 3))); list.add(new StructureRogueVillagePieces.PieceWeight(StructureRogueVillagePieces.Hall.class, 15, MathHelper.getInt(random, 0 + size, 2 + size))); list.add(new StructureRogueVillagePieces.PieceWeight(StructureRogueVillagePieces.Field1.class, 3, MathHelper.getInt(random, 1 + size, 4 + size))); list.add(new StructureRogueVillagePieces.PieceWeight(StructureRogueVillagePieces.Field2.class, 3, MathHelper.getInt(random, 2 + size, 4 + size * 2))); list.add(new StructureRogueVillagePieces.PieceWeight(StructureRogueVillagePieces.House2.class, 15, MathHelper.getInt(random, 0, 1 + size))); list.add(new StructureRogueVillagePieces.PieceWeight(StructureRogueVillagePieces.House3.class, 8, MathHelper.getInt(random, 0 + size, 3 + size * 2))); net.minecraftforge.fml.common.registry.VillagerRegistry.addExtraVillageComponents(list, random, size); Iterator<StructureRogueVillagePieces.PieceWeight> iterator = list.iterator(); while (iterator.hasNext()) { if (((StructureRogueVillagePieces.PieceWeight)iterator.next()).villagePiecesLimit == 0) { iterator.remove(); } } return list; }
i get an error in this line:
net.minecraftforge.fml.common.registry.VillagerRegistry.addExtraVillageComponents(list, random, size);
Because i can't cast from StructureRogueVillagePieces.PieceWeight to StructureVillagePieces.PieceWeight. Is there a way to solve this? for example another method to register village pieces that accept my custom PieceWeight list.
Thanks in advance.
-
Solved. I used acquireAllLookTargets method from here: https://github.com/coolAlias/ZeldaSwordSkills/blob/1.8/src/main/java/zeldaswordskills/util/TargetUtils.java
And adjusted it to 1.11
-
How can i get entities in a cone in front of steve? 3 block length. I tried this from a tutorial:
public static List<RayTraceResult> getMouseOverExtendedList(float dist) { Minecraft mc = FMLClientHandler.instance().getClient(); Entity theRenderViewEntity = mc.getRenderViewEntity(); AxisAlignedBB theViewBoundingBox = new AxisAlignedBB( theRenderViewEntity.posX - 2.0D, theRenderViewEntity.posY - 0.0D, theRenderViewEntity.posZ - 0.0D, theRenderViewEntity.posX + 2.0D, theRenderViewEntity.posY + 1.5D, theRenderViewEntity.posZ + 2.0D); RayTraceResult returnMOP = null; List<RayTraceResult> returnMOPList = new ArrayList<>(); if (mc.world != null) { double distancia = dist; returnMOP = theRenderViewEntity.rayTrace(distancia, 0); double calcdist = distancia; Vec3d vectorPosicionOjos = theRenderViewEntity.getPositionEyes(0); distancia = calcdist; if (returnMOP != null) { calcdist = returnMOP.hitVec.distanceTo(vectorPosicionOjos); } Vec3d lookvec = theRenderViewEntity.getLook(0); Vec3d lookVectorPlusDistancia = vectorPosicionOjos.addVector(lookvec.xCoord * distancia, lookvec.yCoord * distancia, lookvec.zCoord * distancia); float var9 = 1.0F; @SuppressWarnings("unchecked") List<Entity> list = mc.world.getEntitiesWithinAABBExcludingEntity(theRenderViewEntity, theViewBoundingBox.addCoord(lookvec.xCoord * distancia, lookvec.yCoord * distancia, lookvec.zCoord * distancia) .expand(var9, var9, var9)); double d = calcdist; for (Entity entity : list) { if (entity.canBeCollidedWith()) { returnMOPList.add(new RayTraceResult(entity)); } } } return returnMOPList; }
But it didn't worked as i expected. Any help would be much apreciated.
-
Ok it worked. Here's what i did: I copied EntityPolarBear into a new class and made it extend from EntityTameable. Then i copied Model an Render into new classes and adapted them to my bear class. Tank you very much.
-
I already did. Even EntityTamable (Which is the extra class that EntityWolf inherits from unlike EntityPolarBear) and they don't seem to have any code for setting experience orbs from mobs they kill. any idea folks?
-
I did the same with EntityWolf and when my summoned wolf kills a mob it drops xp orbs. It does not happen with my Summoned bear.
-
Override the
getExperiencePoints
in your
Entity
class to control how much experience is dropped.
It worked! Now i'm trying to get the mobs drop XP points when my summonable bear kill them. Any ideas? Many Thanks.
-
Hi everybody,
I have a problem coding my mod. I'm doing a summonable bear by extending EntityPolarBear class and implementing IEntityOwnable interface. Everything works alright but when i kill my minion, it drop xp orbs which is a undesairable behavior. Could some help me disabling this feature? This is my code:
public class EntitySoulBear extends EntityPolarBear implements IEntityOwnable { protected static int puntosAsignados; private static double saludMaxima; private static final int SALUD_INICIAL = 30; protected static final DataParameter<Byte> TAMED = EntityDataManager.<Byte>createKey(EntityTameable.class, DataSerializers.BYTE); protected static final DataParameter<Optional<UUID>> OWNER_UNIQUE_ID = EntityDataManager .<Optional<UUID>>createKey(EntityTameable.class, DataSerializers.OPTIONAL_UNIQUE_ID); public EntitySoulBear(World worldIn) { super(worldIn); // this.setOwnerId(null); this.setTamed(false); } public EntitySoulBear(World worldIn, EntityPlayer playerIn) { this(worldIn); this.setOwnerId(playerIn.getUniqueID()); this.setTamed(true); } @Override protected void initEntityAI() { //super.initEntityAI(); this.tasks.addTask(0, new EntityAISwimming(this)); this.tasks.addTask(1, new EntityAIWatchClosest(this, EntityMob.class, 8.0F)); // this.tasks.addTask(1, new EntityPolarBear.AIMeleeAttack()); //this.tasks.addTask(2, new EntityAILeapAtTarget(this, 0.4F)); this.tasks.addTask(3, new SoulBearAIMeleeAttack(this, 1.25D, true)); // this.tasks.addTask(2, new EntityAIAttackMelee(this, 1.0D, true)); // this.tasks.addTask(1, new EntityPolarBear.AIPanic()); // this.tasks.addTask(3, new EntityAIPanic(this, 2.0D)); //this.tasks.addTask(3, new EntityAIFollowParent(this, 1.25D)); this.tasks.addTask(4, new SoulBearAIFollowOwner(this, 1.0D, 10.0F, 2.0F)); this.tasks.addTask(5, new EntityAIWander(this, 1.0D)); this.tasks.addTask(6, new EntityAIWatchClosest(this, EntityPlayer.class, 8.0F)); this.tasks.addTask(7, new EntityAILookIdle(this)); this.targetTasks.addTask(1, new SoulBearAIOwnerHurtByTarget(this)); this.targetTasks.addTask(2, new SoulBearAIOwnerHurtTarget(this)); this.targetTasks.addTask(3, new EntityAIHurtByTarget(this, true, new Class[0])); this.targetTasks.addTask(4, new EntityAINearestAttackableTarget(this, EntityMob.class, true)); this.targetTasks.addTask(4, new EntityAINearestAttackableTarget(this, EntitySlime.class, true)); } @Override protected void applyEntityAttributes() { // super.applyEntityAttributes(); EntityPlayer ep = Minecraft.getMinecraft().player; if (ep != null) { PlayerSkillCapability playerCapability = (PlayerSkillCapability) PlayerSkillCapabilityProvider.Get(ep); if (playerCapability != null) { this.puntosAsignados = playerCapability.getPaSoulBear(); /* * System.out.println( "===========>soulWolf PA:" + * this.puntosAsignados + " SERVER?: " + * !this.worldObj.isRemote); */ saludMaxima = this.puntosAsignados + SALUD_INICIAL; } } this.getAttributeMap().registerAttribute(SharedMonsterAttributes.MAX_HEALTH); this.getAttributeMap().registerAttribute(SharedMonsterAttributes.KNOCKBACK_RESISTANCE); this.getAttributeMap().registerAttribute(SharedMonsterAttributes.MOVEMENT_SPEED); this.getAttributeMap().registerAttribute(SharedMonsterAttributes.ARMOR); this.getAttributeMap().registerAttribute(SharedMonsterAttributes.ARMOR_TOUGHNESS); this.getAttributeMap().registerAttribute(SharedMonsterAttributes.FOLLOW_RANGE).setBaseValue(16.0D); this.getEntityAttribute(SharedMonsterAttributes.MAX_HEALTH).setBaseValue(30.0D); this.getEntityAttribute(SharedMonsterAttributes.FOLLOW_RANGE).setBaseValue(20.0D); this.getEntityAttribute(SharedMonsterAttributes.MOVEMENT_SPEED).setBaseValue(0.25D); this.getEntityAttribute(SharedMonsterAttributes.ARMOR).setBaseValue(1.5D); this.getAttributeMap().registerAttribute(SharedMonsterAttributes.ATTACK_DAMAGE); // this.getEntityAttribute(SharedMonsterAttributes.ATTACK_DAMAGE).setBaseValue(6.0D); this.getEntityAttribute(SharedMonsterAttributes.ATTACK_DAMAGE) .setBaseValue((1.0D * 0.35 * puntosAsignados) + 4); // 4-10 dmg // thru 20 // lvls this.getEntityAttribute(SharedMonsterAttributes.MAX_HEALTH).setBaseValue(saludMaxima); } @Override protected void entityInit() { super.entityInit(); this.dataManager.register(TAMED, Byte.valueOf((byte) 0)); this.dataManager.register(OWNER_UNIQUE_ID, Optional.<UUID>absent()); } @Override public void onLivingUpdate() { super.onLivingUpdate(); if (this.getOwner() == null || this.getOwner().isDead) this.setDead(); } @Override public UUID getOwnerId() { return (UUID) ((Optional) this.dataManager.get(OWNER_UNIQUE_ID)).orNull(); } public void setOwnerId(@Nullable UUID p_184754_1_) { this.dataManager.set(OWNER_UNIQUE_ID, Optional.fromNullable(p_184754_1_)); } @Override @Nullable public EntityLivingBase getOwner(){ try { UUID uuid = this.getOwnerId(); return uuid == null ? null : this.world.getPlayerEntityByUUID(uuid); } catch (IllegalArgumentException var2){ return null; } } public void setTamed(boolean tamed) { // super.setTamed(tamed); byte tamedByte = ((Byte) this.dataManager.get(TAMED)).byteValue(); if (tamed) { this.dataManager.set(TAMED, Byte.valueOf((byte) (tamedByte | 4))); } else { this.dataManager.set(TAMED, Byte.valueOf((byte) (tamedByte & -5))); } } public boolean isOwner(EntityLivingBase entityIn) { return entityIn == this.getOwner(); } @Override public Team getTeam() { if (this.isTamed()) { EntityLivingBase entitylivingbase = (EntityLivingBase) this.getOwner(); if (entitylivingbase != null) { return entitylivingbase.getTeam(); } } return super.getTeam(); } @Override public boolean isOnSameTeam(Entity entityIn) { // super.isOnSameTeam(entityIn); if (this.isTamed()) { EntityLivingBase entitylivingbase = (EntityLivingBase) this.getOwner(); if (entityIn == entitylivingbase) { return true; } if (entitylivingbase != null) { boolean isOnTeam = entitylivingbase.isOnSameTeam(entityIn); boolean isSameOwner = false; if (entityIn instanceof IEntityOwnable && ((IEntityOwnable) entityIn).getOwner() != null) isSameOwner = entitylivingbase.getUniqueID() .equals(((IEntityOwnable) entityIn).getOwner().getUniqueID()); return isOnTeam || isSameOwner; } } return super.isOnSameTeam(entityIn); } public boolean isTamed() { return (((Byte) this.dataManager.get(TAMED)).byteValue() & 4) != 0; } public boolean isSitting() { return (((Byte) this.dataManager.get(TAMED)).byteValue() & 1) != 0; } @Override public void onDeath(DamageSource cause) { if (!this.world.isRemote && this.world.getGameRules().getBoolean("showDeathMessages") && this.getOwner() instanceof EntityPlayerMP) { // this.getOwner().addChatMessage(this.getCombatTracker().getDeathMessage()); this.setTamed(false); } super.onDeath(cause); } public static void setPuntosAsignados(int puntos) { puntosAsignados = puntos; } public void playWarningSound() { super.playWarningSound(); } public boolean shouldAttackEntity(EntityLivingBase p_142018_1_, EntityLivingBase p_142018_2_) { if (/* !(p_142018_1_ instanceof EntityCreeper) && */ !(p_142018_1_ instanceof EntityGhast)) { if (p_142018_1_ instanceof EntitySoulBear) { EntitySoulBear soulBear = (EntitySoulBear) p_142018_1_; if (soulBear.isTamed() && soulBear.getOwner() == p_142018_2_) { return false; } } if (p_142018_1_ instanceof EntityWolf) { EntityWolf entitywolf = (EntityWolf) p_142018_1_; if (entitywolf.isTamed() && entitywolf.getOwner() == p_142018_2_) { return false; } } return p_142018_1_ instanceof EntityPlayer && p_142018_2_ instanceof EntityPlayer && !((EntityPlayer) p_142018_2_).canAttackPlayer((EntityPlayer) p_142018_1_) ? false : !(p_142018_1_ instanceof AbstractHorse) || !((AbstractHorse) p_142018_1_).isTame(); } else { return false; } } @Override public boolean attackEntityAsMob(Entity entityIn) { super.attackEntityAsMob(entityIn); boolean flag = entityIn.attackEntityFrom(DamageSource.causeMobDamage(this), (float) ((int) this.getEntityAttribute(SharedMonsterAttributes.ATTACK_DAMAGE).getAttributeValue())); if (flag) { this.applyEnchantments(this, entityIn); // Cura 1.5 cada que ataca // this.heal(1.5F); } return flag; } }
Thanks in advance
Adding a property to a custom block freezes Minecraft without giving an Exception
in Modder Support
Posted
I've found that, when I uncomment the .add(CURRENT_AGE) in the following method, Minecraft freezes without giving any exception, otherwise it runs without any problem.